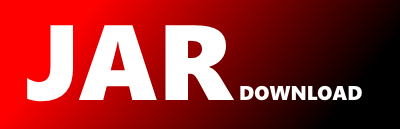
com.amazonaws.services.location.model.UpdateTrackerRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-location Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.location.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class UpdateTrackerRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The name of the tracker resource to update.
*
*/
private String trackerName;
/**
*
* No longer used. If included, the only allowed value is RequestBasedUsage
.
*
*/
@Deprecated
private String pricingPlan;
/**
*
* This parameter is no longer used.
*
*/
@Deprecated
private String pricingPlanDataSource;
/**
*
* Updates the description for the tracker resource.
*
*/
private String description;
/**
*
* Updates the position filtering for the tracker resource.
*
*
* Valid values:
*
*
* -
*
* TimeBased
- Location updates are evaluated against linked geofence collections, but not every
* location update is stored. If your update frequency is more often than 30 seconds, only one update per 30 seconds
* is stored for each unique device ID.
*
*
* -
*
* DistanceBased
- If the device has moved less than 30 m (98.4 ft), location updates are ignored.
* Location updates within this distance are neither evaluated against linked geofence collections, nor stored. This
* helps control costs by reducing the number of geofence evaluations and historical device positions to paginate
* through. Distance-based filtering can also reduce the effects of GPS noise when displaying device trajectories on
* a map.
*
*
* -
*
* AccuracyBased
- If the device has moved less than the measured accuracy, location updates are
* ignored. For example, if two consecutive updates from a device have a horizontal accuracy of 5 m and 10 m, the
* second update is ignored if the device has moved less than 15 m. Ignored location updates are neither evaluated
* against linked geofence collections, nor stored. This helps educe the effects of GPS noise when displaying device
* trajectories on a map, and can help control costs by reducing the number of geofence evaluations.
*
*
*
*/
private String positionFiltering;
/**
*
* Whether to enable position UPDATE
events from this tracker to be sent to EventBridge.
*
*
*
* You do not need enable this feature to get ENTER
and EXIT
events for geofences with
* this tracker. Those events are always sent to EventBridge.
*
*
*/
private Boolean eventBridgeEnabled;
/**
*
* Enables GeospatialQueries
for a tracker that uses a Amazon Web Services KMS customer
* managed key.
*
*
* This parameter is only used if you are using a KMS customer managed key.
*
*/
private Boolean kmsKeyEnableGeospatialQueries;
/**
*
* The name of the tracker resource to update.
*
*
* @param trackerName
* The name of the tracker resource to update.
*/
public void setTrackerName(String trackerName) {
this.trackerName = trackerName;
}
/**
*
* The name of the tracker resource to update.
*
*
* @return The name of the tracker resource to update.
*/
public String getTrackerName() {
return this.trackerName;
}
/**
*
* The name of the tracker resource to update.
*
*
* @param trackerName
* The name of the tracker resource to update.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateTrackerRequest withTrackerName(String trackerName) {
setTrackerName(trackerName);
return this;
}
/**
*
* No longer used. If included, the only allowed value is RequestBasedUsage
.
*
*
* @param pricingPlan
* No longer used. If included, the only allowed value is RequestBasedUsage
.
* @see PricingPlan
*/
@Deprecated
public void setPricingPlan(String pricingPlan) {
this.pricingPlan = pricingPlan;
}
/**
*
* No longer used. If included, the only allowed value is RequestBasedUsage
.
*
*
* @return No longer used. If included, the only allowed value is RequestBasedUsage
.
* @see PricingPlan
*/
@Deprecated
public String getPricingPlan() {
return this.pricingPlan;
}
/**
*
* No longer used. If included, the only allowed value is RequestBasedUsage
.
*
*
* @param pricingPlan
* No longer used. If included, the only allowed value is RequestBasedUsage
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see PricingPlan
*/
@Deprecated
public UpdateTrackerRequest withPricingPlan(String pricingPlan) {
setPricingPlan(pricingPlan);
return this;
}
/**
*
* No longer used. If included, the only allowed value is RequestBasedUsage
.
*
*
* @param pricingPlan
* No longer used. If included, the only allowed value is RequestBasedUsage
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see PricingPlan
*/
@Deprecated
public UpdateTrackerRequest withPricingPlan(PricingPlan pricingPlan) {
this.pricingPlan = pricingPlan.toString();
return this;
}
/**
*
* This parameter is no longer used.
*
*
* @param pricingPlanDataSource
* This parameter is no longer used.
*/
@Deprecated
public void setPricingPlanDataSource(String pricingPlanDataSource) {
this.pricingPlanDataSource = pricingPlanDataSource;
}
/**
*
* This parameter is no longer used.
*
*
* @return This parameter is no longer used.
*/
@Deprecated
public String getPricingPlanDataSource() {
return this.pricingPlanDataSource;
}
/**
*
* This parameter is no longer used.
*
*
* @param pricingPlanDataSource
* This parameter is no longer used.
* @return Returns a reference to this object so that method calls can be chained together.
*/
@Deprecated
public UpdateTrackerRequest withPricingPlanDataSource(String pricingPlanDataSource) {
setPricingPlanDataSource(pricingPlanDataSource);
return this;
}
/**
*
* Updates the description for the tracker resource.
*
*
* @param description
* Updates the description for the tracker resource.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* Updates the description for the tracker resource.
*
*
* @return Updates the description for the tracker resource.
*/
public String getDescription() {
return this.description;
}
/**
*
* Updates the description for the tracker resource.
*
*
* @param description
* Updates the description for the tracker resource.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateTrackerRequest withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* Updates the position filtering for the tracker resource.
*
*
* Valid values:
*
*
* -
*
* TimeBased
- Location updates are evaluated against linked geofence collections, but not every
* location update is stored. If your update frequency is more often than 30 seconds, only one update per 30 seconds
* is stored for each unique device ID.
*
*
* -
*
* DistanceBased
- If the device has moved less than 30 m (98.4 ft), location updates are ignored.
* Location updates within this distance are neither evaluated against linked geofence collections, nor stored. This
* helps control costs by reducing the number of geofence evaluations and historical device positions to paginate
* through. Distance-based filtering can also reduce the effects of GPS noise when displaying device trajectories on
* a map.
*
*
* -
*
* AccuracyBased
- If the device has moved less than the measured accuracy, location updates are
* ignored. For example, if two consecutive updates from a device have a horizontal accuracy of 5 m and 10 m, the
* second update is ignored if the device has moved less than 15 m. Ignored location updates are neither evaluated
* against linked geofence collections, nor stored. This helps educe the effects of GPS noise when displaying device
* trajectories on a map, and can help control costs by reducing the number of geofence evaluations.
*
*
*
*
* @param positionFiltering
* Updates the position filtering for the tracker resource.
*
* Valid values:
*
*
* -
*
* TimeBased
- Location updates are evaluated against linked geofence collections, but not every
* location update is stored. If your update frequency is more often than 30 seconds, only one update per 30
* seconds is stored for each unique device ID.
*
*
* -
*
* DistanceBased
- If the device has moved less than 30 m (98.4 ft), location updates are
* ignored. Location updates within this distance are neither evaluated against linked geofence collections,
* nor stored. This helps control costs by reducing the number of geofence evaluations and historical device
* positions to paginate through. Distance-based filtering can also reduce the effects of GPS noise when
* displaying device trajectories on a map.
*
*
* -
*
* AccuracyBased
- If the device has moved less than the measured accuracy, location updates are
* ignored. For example, if two consecutive updates from a device have a horizontal accuracy of 5 m and 10 m,
* the second update is ignored if the device has moved less than 15 m. Ignored location updates are neither
* evaluated against linked geofence collections, nor stored. This helps educe the effects of GPS noise when
* displaying device trajectories on a map, and can help control costs by reducing the number of geofence
* evaluations.
*
*
* @see PositionFiltering
*/
public void setPositionFiltering(String positionFiltering) {
this.positionFiltering = positionFiltering;
}
/**
*
* Updates the position filtering for the tracker resource.
*
*
* Valid values:
*
*
* -
*
* TimeBased
- Location updates are evaluated against linked geofence collections, but not every
* location update is stored. If your update frequency is more often than 30 seconds, only one update per 30 seconds
* is stored for each unique device ID.
*
*
* -
*
* DistanceBased
- If the device has moved less than 30 m (98.4 ft), location updates are ignored.
* Location updates within this distance are neither evaluated against linked geofence collections, nor stored. This
* helps control costs by reducing the number of geofence evaluations and historical device positions to paginate
* through. Distance-based filtering can also reduce the effects of GPS noise when displaying device trajectories on
* a map.
*
*
* -
*
* AccuracyBased
- If the device has moved less than the measured accuracy, location updates are
* ignored. For example, if two consecutive updates from a device have a horizontal accuracy of 5 m and 10 m, the
* second update is ignored if the device has moved less than 15 m. Ignored location updates are neither evaluated
* against linked geofence collections, nor stored. This helps educe the effects of GPS noise when displaying device
* trajectories on a map, and can help control costs by reducing the number of geofence evaluations.
*
*
*
*
* @return Updates the position filtering for the tracker resource.
*
* Valid values:
*
*
* -
*
* TimeBased
- Location updates are evaluated against linked geofence collections, but not
* every location update is stored. If your update frequency is more often than 30 seconds, only one update
* per 30 seconds is stored for each unique device ID.
*
*
* -
*
* DistanceBased
- If the device has moved less than 30 m (98.4 ft), location updates are
* ignored. Location updates within this distance are neither evaluated against linked geofence collections,
* nor stored. This helps control costs by reducing the number of geofence evaluations and historical device
* positions to paginate through. Distance-based filtering can also reduce the effects of GPS noise when
* displaying device trajectories on a map.
*
*
* -
*
* AccuracyBased
- If the device has moved less than the measured accuracy, location updates
* are ignored. For example, if two consecutive updates from a device have a horizontal accuracy of 5 m and
* 10 m, the second update is ignored if the device has moved less than 15 m. Ignored location updates are
* neither evaluated against linked geofence collections, nor stored. This helps educe the effects of GPS
* noise when displaying device trajectories on a map, and can help control costs by reducing the number of
* geofence evaluations.
*
*
* @see PositionFiltering
*/
public String getPositionFiltering() {
return this.positionFiltering;
}
/**
*
* Updates the position filtering for the tracker resource.
*
*
* Valid values:
*
*
* -
*
* TimeBased
- Location updates are evaluated against linked geofence collections, but not every
* location update is stored. If your update frequency is more often than 30 seconds, only one update per 30 seconds
* is stored for each unique device ID.
*
*
* -
*
* DistanceBased
- If the device has moved less than 30 m (98.4 ft), location updates are ignored.
* Location updates within this distance are neither evaluated against linked geofence collections, nor stored. This
* helps control costs by reducing the number of geofence evaluations and historical device positions to paginate
* through. Distance-based filtering can also reduce the effects of GPS noise when displaying device trajectories on
* a map.
*
*
* -
*
* AccuracyBased
- If the device has moved less than the measured accuracy, location updates are
* ignored. For example, if two consecutive updates from a device have a horizontal accuracy of 5 m and 10 m, the
* second update is ignored if the device has moved less than 15 m. Ignored location updates are neither evaluated
* against linked geofence collections, nor stored. This helps educe the effects of GPS noise when displaying device
* trajectories on a map, and can help control costs by reducing the number of geofence evaluations.
*
*
*
*
* @param positionFiltering
* Updates the position filtering for the tracker resource.
*
* Valid values:
*
*
* -
*
* TimeBased
- Location updates are evaluated against linked geofence collections, but not every
* location update is stored. If your update frequency is more often than 30 seconds, only one update per 30
* seconds is stored for each unique device ID.
*
*
* -
*
* DistanceBased
- If the device has moved less than 30 m (98.4 ft), location updates are
* ignored. Location updates within this distance are neither evaluated against linked geofence collections,
* nor stored. This helps control costs by reducing the number of geofence evaluations and historical device
* positions to paginate through. Distance-based filtering can also reduce the effects of GPS noise when
* displaying device trajectories on a map.
*
*
* -
*
* AccuracyBased
- If the device has moved less than the measured accuracy, location updates are
* ignored. For example, if two consecutive updates from a device have a horizontal accuracy of 5 m and 10 m,
* the second update is ignored if the device has moved less than 15 m. Ignored location updates are neither
* evaluated against linked geofence collections, nor stored. This helps educe the effects of GPS noise when
* displaying device trajectories on a map, and can help control costs by reducing the number of geofence
* evaluations.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see PositionFiltering
*/
public UpdateTrackerRequest withPositionFiltering(String positionFiltering) {
setPositionFiltering(positionFiltering);
return this;
}
/**
*
* Updates the position filtering for the tracker resource.
*
*
* Valid values:
*
*
* -
*
* TimeBased
- Location updates are evaluated against linked geofence collections, but not every
* location update is stored. If your update frequency is more often than 30 seconds, only one update per 30 seconds
* is stored for each unique device ID.
*
*
* -
*
* DistanceBased
- If the device has moved less than 30 m (98.4 ft), location updates are ignored.
* Location updates within this distance are neither evaluated against linked geofence collections, nor stored. This
* helps control costs by reducing the number of geofence evaluations and historical device positions to paginate
* through. Distance-based filtering can also reduce the effects of GPS noise when displaying device trajectories on
* a map.
*
*
* -
*
* AccuracyBased
- If the device has moved less than the measured accuracy, location updates are
* ignored. For example, if two consecutive updates from a device have a horizontal accuracy of 5 m and 10 m, the
* second update is ignored if the device has moved less than 15 m. Ignored location updates are neither evaluated
* against linked geofence collections, nor stored. This helps educe the effects of GPS noise when displaying device
* trajectories on a map, and can help control costs by reducing the number of geofence evaluations.
*
*
*
*
* @param positionFiltering
* Updates the position filtering for the tracker resource.
*
* Valid values:
*
*
* -
*
* TimeBased
- Location updates are evaluated against linked geofence collections, but not every
* location update is stored. If your update frequency is more often than 30 seconds, only one update per 30
* seconds is stored for each unique device ID.
*
*
* -
*
* DistanceBased
- If the device has moved less than 30 m (98.4 ft), location updates are
* ignored. Location updates within this distance are neither evaluated against linked geofence collections,
* nor stored. This helps control costs by reducing the number of geofence evaluations and historical device
* positions to paginate through. Distance-based filtering can also reduce the effects of GPS noise when
* displaying device trajectories on a map.
*
*
* -
*
* AccuracyBased
- If the device has moved less than the measured accuracy, location updates are
* ignored. For example, if two consecutive updates from a device have a horizontal accuracy of 5 m and 10 m,
* the second update is ignored if the device has moved less than 15 m. Ignored location updates are neither
* evaluated against linked geofence collections, nor stored. This helps educe the effects of GPS noise when
* displaying device trajectories on a map, and can help control costs by reducing the number of geofence
* evaluations.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see PositionFiltering
*/
public UpdateTrackerRequest withPositionFiltering(PositionFiltering positionFiltering) {
this.positionFiltering = positionFiltering.toString();
return this;
}
/**
*
* Whether to enable position UPDATE
events from this tracker to be sent to EventBridge.
*
*
*
* You do not need enable this feature to get ENTER
and EXIT
events for geofences with
* this tracker. Those events are always sent to EventBridge.
*
*
*
* @param eventBridgeEnabled
* Whether to enable position UPDATE
events from this tracker to be sent to EventBridge.
*
*
* You do not need enable this feature to get ENTER
and EXIT
events for geofences
* with this tracker. Those events are always sent to EventBridge.
*
*/
public void setEventBridgeEnabled(Boolean eventBridgeEnabled) {
this.eventBridgeEnabled = eventBridgeEnabled;
}
/**
*
* Whether to enable position UPDATE
events from this tracker to be sent to EventBridge.
*
*
*
* You do not need enable this feature to get ENTER
and EXIT
events for geofences with
* this tracker. Those events are always sent to EventBridge.
*
*
*
* @return Whether to enable position UPDATE
events from this tracker to be sent to EventBridge.
*
*
* You do not need enable this feature to get ENTER
and EXIT
events for geofences
* with this tracker. Those events are always sent to EventBridge.
*
*/
public Boolean getEventBridgeEnabled() {
return this.eventBridgeEnabled;
}
/**
*
* Whether to enable position UPDATE
events from this tracker to be sent to EventBridge.
*
*
*
* You do not need enable this feature to get ENTER
and EXIT
events for geofences with
* this tracker. Those events are always sent to EventBridge.
*
*
*
* @param eventBridgeEnabled
* Whether to enable position UPDATE
events from this tracker to be sent to EventBridge.
*
*
* You do not need enable this feature to get ENTER
and EXIT
events for geofences
* with this tracker. Those events are always sent to EventBridge.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateTrackerRequest withEventBridgeEnabled(Boolean eventBridgeEnabled) {
setEventBridgeEnabled(eventBridgeEnabled);
return this;
}
/**
*
* Whether to enable position UPDATE
events from this tracker to be sent to EventBridge.
*
*
*
* You do not need enable this feature to get ENTER
and EXIT
events for geofences with
* this tracker. Those events are always sent to EventBridge.
*
*
*
* @return Whether to enable position UPDATE
events from this tracker to be sent to EventBridge.
*
*
* You do not need enable this feature to get ENTER
and EXIT
events for geofences
* with this tracker. Those events are always sent to EventBridge.
*
*/
public Boolean isEventBridgeEnabled() {
return this.eventBridgeEnabled;
}
/**
*
* Enables GeospatialQueries
for a tracker that uses a Amazon Web Services KMS customer
* managed key.
*
*
* This parameter is only used if you are using a KMS customer managed key.
*
*
* @param kmsKeyEnableGeospatialQueries
* Enables GeospatialQueries
for a tracker that uses a Amazon Web Services KMS
* customer managed key.
*
* This parameter is only used if you are using a KMS customer managed key.
*/
public void setKmsKeyEnableGeospatialQueries(Boolean kmsKeyEnableGeospatialQueries) {
this.kmsKeyEnableGeospatialQueries = kmsKeyEnableGeospatialQueries;
}
/**
*
* Enables GeospatialQueries
for a tracker that uses a Amazon Web Services KMS customer
* managed key.
*
*
* This parameter is only used if you are using a KMS customer managed key.
*
*
* @return Enables GeospatialQueries
for a tracker that uses a Amazon Web Services KMS
* customer managed key.
*
* This parameter is only used if you are using a KMS customer managed key.
*/
public Boolean getKmsKeyEnableGeospatialQueries() {
return this.kmsKeyEnableGeospatialQueries;
}
/**
*
* Enables GeospatialQueries
for a tracker that uses a Amazon Web Services KMS customer
* managed key.
*
*
* This parameter is only used if you are using a KMS customer managed key.
*
*
* @param kmsKeyEnableGeospatialQueries
* Enables GeospatialQueries
for a tracker that uses a Amazon Web Services KMS
* customer managed key.
*
* This parameter is only used if you are using a KMS customer managed key.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateTrackerRequest withKmsKeyEnableGeospatialQueries(Boolean kmsKeyEnableGeospatialQueries) {
setKmsKeyEnableGeospatialQueries(kmsKeyEnableGeospatialQueries);
return this;
}
/**
*
* Enables GeospatialQueries
for a tracker that uses a Amazon Web Services KMS customer
* managed key.
*
*
* This parameter is only used if you are using a KMS customer managed key.
*
*
* @return Enables GeospatialQueries
for a tracker that uses a Amazon Web Services KMS
* customer managed key.
*
* This parameter is only used if you are using a KMS customer managed key.
*/
public Boolean isKmsKeyEnableGeospatialQueries() {
return this.kmsKeyEnableGeospatialQueries;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getTrackerName() != null)
sb.append("TrackerName: ").append(getTrackerName()).append(",");
if (getPricingPlan() != null)
sb.append("PricingPlan: ").append(getPricingPlan()).append(",");
if (getPricingPlanDataSource() != null)
sb.append("PricingPlanDataSource: ").append(getPricingPlanDataSource()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getPositionFiltering() != null)
sb.append("PositionFiltering: ").append(getPositionFiltering()).append(",");
if (getEventBridgeEnabled() != null)
sb.append("EventBridgeEnabled: ").append(getEventBridgeEnabled()).append(",");
if (getKmsKeyEnableGeospatialQueries() != null)
sb.append("KmsKeyEnableGeospatialQueries: ").append(getKmsKeyEnableGeospatialQueries());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof UpdateTrackerRequest == false)
return false;
UpdateTrackerRequest other = (UpdateTrackerRequest) obj;
if (other.getTrackerName() == null ^ this.getTrackerName() == null)
return false;
if (other.getTrackerName() != null && other.getTrackerName().equals(this.getTrackerName()) == false)
return false;
if (other.getPricingPlan() == null ^ this.getPricingPlan() == null)
return false;
if (other.getPricingPlan() != null && other.getPricingPlan().equals(this.getPricingPlan()) == false)
return false;
if (other.getPricingPlanDataSource() == null ^ this.getPricingPlanDataSource() == null)
return false;
if (other.getPricingPlanDataSource() != null && other.getPricingPlanDataSource().equals(this.getPricingPlanDataSource()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getPositionFiltering() == null ^ this.getPositionFiltering() == null)
return false;
if (other.getPositionFiltering() != null && other.getPositionFiltering().equals(this.getPositionFiltering()) == false)
return false;
if (other.getEventBridgeEnabled() == null ^ this.getEventBridgeEnabled() == null)
return false;
if (other.getEventBridgeEnabled() != null && other.getEventBridgeEnabled().equals(this.getEventBridgeEnabled()) == false)
return false;
if (other.getKmsKeyEnableGeospatialQueries() == null ^ this.getKmsKeyEnableGeospatialQueries() == null)
return false;
if (other.getKmsKeyEnableGeospatialQueries() != null
&& other.getKmsKeyEnableGeospatialQueries().equals(this.getKmsKeyEnableGeospatialQueries()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getTrackerName() == null) ? 0 : getTrackerName().hashCode());
hashCode = prime * hashCode + ((getPricingPlan() == null) ? 0 : getPricingPlan().hashCode());
hashCode = prime * hashCode + ((getPricingPlanDataSource() == null) ? 0 : getPricingPlanDataSource().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getPositionFiltering() == null) ? 0 : getPositionFiltering().hashCode());
hashCode = prime * hashCode + ((getEventBridgeEnabled() == null) ? 0 : getEventBridgeEnabled().hashCode());
hashCode = prime * hashCode + ((getKmsKeyEnableGeospatialQueries() == null) ? 0 : getKmsKeyEnableGeospatialQueries().hashCode());
return hashCode;
}
@Override
public UpdateTrackerRequest clone() {
return (UpdateTrackerRequest) super.clone();
}
}