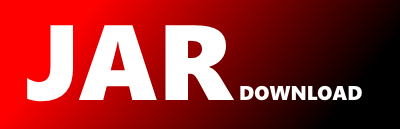
com.amazonaws.services.location.model.CreateRouteCalculatorRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-location Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.location.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateRouteCalculatorRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The name of the route calculator resource.
*
*
* Requirements:
*
*
* -
*
* Can use alphanumeric characters (A–Z, a–z, 0–9) , hyphens (-), periods (.), and underscores (_).
*
*
* -
*
* Must be a unique Route calculator resource name.
*
*
* -
*
* No spaces allowed. For example, ExampleRouteCalculator
.
*
*
*
*/
private String calculatorName;
/**
*
* Specifies the data provider of traffic and road network data.
*
*
*
* This field is case-sensitive. Enter the valid values as shown. For example, entering HERE
returns an
* error.
*
*
*
* Valid values include:
*
*
* -
*
* Esri
– For additional information about Esri's coverage in your region of
* interest, see Esri details on
* street networks and traffic coverage.
*
*
* Route calculators that use Esri as a data source only calculate routes that are shorter than 400 km.
*
*
* -
*
* Grab
– Grab provides routing functionality for Southeast Asia. For additional information about GrabMaps' coverage, see GrabMaps countries
* and areas covered.
*
*
* -
*
* Here
– For additional information about HERE Technologies' coverage in
* your region of interest, see HERE car
* routing coverage and HERE
* truck routing coverage.
*
*
*
*
* For additional information , see Data providers
* on the Amazon Location Service Developer Guide.
*
*/
private String dataSource;
/**
*
* No longer used. If included, the only allowed value is RequestBasedUsage
.
*
*/
@Deprecated
private String pricingPlan;
/**
*
* The optional description for the route calculator resource.
*
*/
private String description;
/**
*
* Applies one or more tags to the route calculator resource. A tag is a key-value pair helps manage, identify,
* search, and filter your resources by labelling them.
*
*
* -
*
* For example: { "tag1" : "value1"
, "tag2" : "value2"
*
*
*
*
* Format: "key" : "value"
*
*
* Restrictions:
*
*
* -
*
* Maximum 50 tags per resource
*
*
* -
*
* Each resource tag must be unique with a maximum of one value.
*
*
* -
*
* Maximum key length: 128 Unicode characters in UTF-8
*
*
* -
*
* Maximum value length: 256 Unicode characters in UTF-8
*
*
* -
*
* Can use alphanumeric characters (A–Z, a–z, 0–9), and the following characters: + - = . _ : / @.
*
*
* -
*
* Cannot use "aws:" as a prefix for a key.
*
*
*
*/
private java.util.Map tags;
/**
*
* The name of the route calculator resource.
*
*
* Requirements:
*
*
* -
*
* Can use alphanumeric characters (A–Z, a–z, 0–9) , hyphens (-), periods (.), and underscores (_).
*
*
* -
*
* Must be a unique Route calculator resource name.
*
*
* -
*
* No spaces allowed. For example, ExampleRouteCalculator
.
*
*
*
*
* @param calculatorName
* The name of the route calculator resource.
*
* Requirements:
*
*
* -
*
* Can use alphanumeric characters (A–Z, a–z, 0–9) , hyphens (-), periods (.), and underscores (_).
*
*
* -
*
* Must be a unique Route calculator resource name.
*
*
* -
*
* No spaces allowed. For example, ExampleRouteCalculator
.
*
*
*/
public void setCalculatorName(String calculatorName) {
this.calculatorName = calculatorName;
}
/**
*
* The name of the route calculator resource.
*
*
* Requirements:
*
*
* -
*
* Can use alphanumeric characters (A–Z, a–z, 0–9) , hyphens (-), periods (.), and underscores (_).
*
*
* -
*
* Must be a unique Route calculator resource name.
*
*
* -
*
* No spaces allowed. For example, ExampleRouteCalculator
.
*
*
*
*
* @return The name of the route calculator resource.
*
* Requirements:
*
*
* -
*
* Can use alphanumeric characters (A–Z, a–z, 0–9) , hyphens (-), periods (.), and underscores (_).
*
*
* -
*
* Must be a unique Route calculator resource name.
*
*
* -
*
* No spaces allowed. For example, ExampleRouteCalculator
.
*
*
*/
public String getCalculatorName() {
return this.calculatorName;
}
/**
*
* The name of the route calculator resource.
*
*
* Requirements:
*
*
* -
*
* Can use alphanumeric characters (A–Z, a–z, 0–9) , hyphens (-), periods (.), and underscores (_).
*
*
* -
*
* Must be a unique Route calculator resource name.
*
*
* -
*
* No spaces allowed. For example, ExampleRouteCalculator
.
*
*
*
*
* @param calculatorName
* The name of the route calculator resource.
*
* Requirements:
*
*
* -
*
* Can use alphanumeric characters (A–Z, a–z, 0–9) , hyphens (-), periods (.), and underscores (_).
*
*
* -
*
* Must be a unique Route calculator resource name.
*
*
* -
*
* No spaces allowed. For example, ExampleRouteCalculator
.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateRouteCalculatorRequest withCalculatorName(String calculatorName) {
setCalculatorName(calculatorName);
return this;
}
/**
*
* Specifies the data provider of traffic and road network data.
*
*
*
* This field is case-sensitive. Enter the valid values as shown. For example, entering HERE
returns an
* error.
*
*
*
* Valid values include:
*
*
* -
*
* Esri
– For additional information about Esri's coverage in your region of
* interest, see Esri details on
* street networks and traffic coverage.
*
*
* Route calculators that use Esri as a data source only calculate routes that are shorter than 400 km.
*
*
* -
*
* Grab
– Grab provides routing functionality for Southeast Asia. For additional information about GrabMaps' coverage, see GrabMaps countries
* and areas covered.
*
*
* -
*
* Here
– For additional information about HERE Technologies' coverage in
* your region of interest, see HERE car
* routing coverage and HERE
* truck routing coverage.
*
*
*
*
* For additional information , see Data providers
* on the Amazon Location Service Developer Guide.
*
*
* @param dataSource
* Specifies the data provider of traffic and road network data.
*
* This field is case-sensitive. Enter the valid values as shown. For example, entering HERE
* returns an error.
*
*
*
* Valid values include:
*
*
* -
*
* Esri
– For additional information about Esri's coverage in your
* region of interest, see Esri details on street
* networks and traffic coverage.
*
*
* Route calculators that use Esri as a data source only calculate routes that are shorter than 400 km.
*
*
* -
*
* Grab
– Grab provides routing functionality for Southeast Asia. For additional information
* about GrabMaps'
* coverage, see GrabMaps
* countries and areas covered.
*
*
* -
*
* Here
– For additional information about HERE Technologies'
* coverage in your region of interest, see HERE car routing coverage and HERE truck routing coverage.
*
*
*
*
* For additional information , see Data
* providers on the Amazon Location Service Developer Guide.
*/
public void setDataSource(String dataSource) {
this.dataSource = dataSource;
}
/**
*
* Specifies the data provider of traffic and road network data.
*
*
*
* This field is case-sensitive. Enter the valid values as shown. For example, entering HERE
returns an
* error.
*
*
*
* Valid values include:
*
*
* -
*
* Esri
– For additional information about Esri's coverage in your region of
* interest, see Esri details on
* street networks and traffic coverage.
*
*
* Route calculators that use Esri as a data source only calculate routes that are shorter than 400 km.
*
*
* -
*
* Grab
– Grab provides routing functionality for Southeast Asia. For additional information about GrabMaps' coverage, see GrabMaps countries
* and areas covered.
*
*
* -
*
* Here
– For additional information about HERE Technologies' coverage in
* your region of interest, see HERE car
* routing coverage and HERE
* truck routing coverage.
*
*
*
*
* For additional information , see Data providers
* on the Amazon Location Service Developer Guide.
*
*
* @return Specifies the data provider of traffic and road network data.
*
* This field is case-sensitive. Enter the valid values as shown. For example, entering HERE
* returns an error.
*
*
*
* Valid values include:
*
*
* -
*
* Esri
– For additional information about Esri's coverage in your
* region of interest, see Esri details on street
* networks and traffic coverage.
*
*
* Route calculators that use Esri as a data source only calculate routes that are shorter than 400 km.
*
*
* -
*
* Grab
– Grab provides routing functionality for Southeast Asia. For additional information
* about GrabMaps'
* coverage, see GrabMaps
* countries and areas covered.
*
*
* -
*
* Here
– For additional information about HERE Technologies'
* coverage in your region of interest, see HERE car routing coverage and HERE
* truck routing coverage.
*
*
*
*
* For additional information , see Data
* providers on the Amazon Location Service Developer Guide.
*/
public String getDataSource() {
return this.dataSource;
}
/**
*
* Specifies the data provider of traffic and road network data.
*
*
*
* This field is case-sensitive. Enter the valid values as shown. For example, entering HERE
returns an
* error.
*
*
*
* Valid values include:
*
*
* -
*
* Esri
– For additional information about Esri's coverage in your region of
* interest, see Esri details on
* street networks and traffic coverage.
*
*
* Route calculators that use Esri as a data source only calculate routes that are shorter than 400 km.
*
*
* -
*
* Grab
– Grab provides routing functionality for Southeast Asia. For additional information about GrabMaps' coverage, see GrabMaps countries
* and areas covered.
*
*
* -
*
* Here
– For additional information about HERE Technologies' coverage in
* your region of interest, see HERE car
* routing coverage and HERE
* truck routing coverage.
*
*
*
*
* For additional information , see Data providers
* on the Amazon Location Service Developer Guide.
*
*
* @param dataSource
* Specifies the data provider of traffic and road network data.
*
* This field is case-sensitive. Enter the valid values as shown. For example, entering HERE
* returns an error.
*
*
*
* Valid values include:
*
*
* -
*
* Esri
– For additional information about Esri's coverage in your
* region of interest, see Esri details on street
* networks and traffic coverage.
*
*
* Route calculators that use Esri as a data source only calculate routes that are shorter than 400 km.
*
*
* -
*
* Grab
– Grab provides routing functionality for Southeast Asia. For additional information
* about GrabMaps'
* coverage, see GrabMaps
* countries and areas covered.
*
*
* -
*
* Here
– For additional information about HERE Technologies'
* coverage in your region of interest, see HERE car routing coverage and HERE truck routing coverage.
*
*
*
*
* For additional information , see Data
* providers on the Amazon Location Service Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateRouteCalculatorRequest withDataSource(String dataSource) {
setDataSource(dataSource);
return this;
}
/**
*
* No longer used. If included, the only allowed value is RequestBasedUsage
.
*
*
* @param pricingPlan
* No longer used. If included, the only allowed value is RequestBasedUsage
.
* @see PricingPlan
*/
@Deprecated
public void setPricingPlan(String pricingPlan) {
this.pricingPlan = pricingPlan;
}
/**
*
* No longer used. If included, the only allowed value is RequestBasedUsage
.
*
*
* @return No longer used. If included, the only allowed value is RequestBasedUsage
.
* @see PricingPlan
*/
@Deprecated
public String getPricingPlan() {
return this.pricingPlan;
}
/**
*
* No longer used. If included, the only allowed value is RequestBasedUsage
.
*
*
* @param pricingPlan
* No longer used. If included, the only allowed value is RequestBasedUsage
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see PricingPlan
*/
@Deprecated
public CreateRouteCalculatorRequest withPricingPlan(String pricingPlan) {
setPricingPlan(pricingPlan);
return this;
}
/**
*
* No longer used. If included, the only allowed value is RequestBasedUsage
.
*
*
* @param pricingPlan
* No longer used. If included, the only allowed value is RequestBasedUsage
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see PricingPlan
*/
@Deprecated
public CreateRouteCalculatorRequest withPricingPlan(PricingPlan pricingPlan) {
this.pricingPlan = pricingPlan.toString();
return this;
}
/**
*
* The optional description for the route calculator resource.
*
*
* @param description
* The optional description for the route calculator resource.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* The optional description for the route calculator resource.
*
*
* @return The optional description for the route calculator resource.
*/
public String getDescription() {
return this.description;
}
/**
*
* The optional description for the route calculator resource.
*
*
* @param description
* The optional description for the route calculator resource.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateRouteCalculatorRequest withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* Applies one or more tags to the route calculator resource. A tag is a key-value pair helps manage, identify,
* search, and filter your resources by labelling them.
*
*
* -
*
* For example: { "tag1" : "value1"
, "tag2" : "value2"
*
*
*
*
* Format: "key" : "value"
*
*
* Restrictions:
*
*
* -
*
* Maximum 50 tags per resource
*
*
* -
*
* Each resource tag must be unique with a maximum of one value.
*
*
* -
*
* Maximum key length: 128 Unicode characters in UTF-8
*
*
* -
*
* Maximum value length: 256 Unicode characters in UTF-8
*
*
* -
*
* Can use alphanumeric characters (A–Z, a–z, 0–9), and the following characters: + - = . _ : / @.
*
*
* -
*
* Cannot use "aws:" as a prefix for a key.
*
*
*
*
* @return Applies one or more tags to the route calculator resource. A tag is a key-value pair helps manage,
* identify, search, and filter your resources by labelling them.
*
* -
*
* For example: { "tag1" : "value1"
, "tag2" : "value2"
*
*
*
*
* Format: "key" : "value"
*
*
* Restrictions:
*
*
* -
*
* Maximum 50 tags per resource
*
*
* -
*
* Each resource tag must be unique with a maximum of one value.
*
*
* -
*
* Maximum key length: 128 Unicode characters in UTF-8
*
*
* -
*
* Maximum value length: 256 Unicode characters in UTF-8
*
*
* -
*
* Can use alphanumeric characters (A–Z, a–z, 0–9), and the following characters: + - = . _ : / @.
*
*
* -
*
* Cannot use "aws:" as a prefix for a key.
*
*
*/
public java.util.Map getTags() {
return tags;
}
/**
*
* Applies one or more tags to the route calculator resource. A tag is a key-value pair helps manage, identify,
* search, and filter your resources by labelling them.
*
*
* -
*
* For example: { "tag1" : "value1"
, "tag2" : "value2"
*
*
*
*
* Format: "key" : "value"
*
*
* Restrictions:
*
*
* -
*
* Maximum 50 tags per resource
*
*
* -
*
* Each resource tag must be unique with a maximum of one value.
*
*
* -
*
* Maximum key length: 128 Unicode characters in UTF-8
*
*
* -
*
* Maximum value length: 256 Unicode characters in UTF-8
*
*
* -
*
* Can use alphanumeric characters (A–Z, a–z, 0–9), and the following characters: + - = . _ : / @.
*
*
* -
*
* Cannot use "aws:" as a prefix for a key.
*
*
*
*
* @param tags
* Applies one or more tags to the route calculator resource. A tag is a key-value pair helps manage,
* identify, search, and filter your resources by labelling them.
*
* -
*
* For example: { "tag1" : "value1"
, "tag2" : "value2"
*
*
*
*
* Format: "key" : "value"
*
*
* Restrictions:
*
*
* -
*
* Maximum 50 tags per resource
*
*
* -
*
* Each resource tag must be unique with a maximum of one value.
*
*
* -
*
* Maximum key length: 128 Unicode characters in UTF-8
*
*
* -
*
* Maximum value length: 256 Unicode characters in UTF-8
*
*
* -
*
* Can use alphanumeric characters (A–Z, a–z, 0–9), and the following characters: + - = . _ : / @.
*
*
* -
*
* Cannot use "aws:" as a prefix for a key.
*
*
*/
public void setTags(java.util.Map tags) {
this.tags = tags;
}
/**
*
* Applies one or more tags to the route calculator resource. A tag is a key-value pair helps manage, identify,
* search, and filter your resources by labelling them.
*
*
* -
*
* For example: { "tag1" : "value1"
, "tag2" : "value2"
*
*
*
*
* Format: "key" : "value"
*
*
* Restrictions:
*
*
* -
*
* Maximum 50 tags per resource
*
*
* -
*
* Each resource tag must be unique with a maximum of one value.
*
*
* -
*
* Maximum key length: 128 Unicode characters in UTF-8
*
*
* -
*
* Maximum value length: 256 Unicode characters in UTF-8
*
*
* -
*
* Can use alphanumeric characters (A–Z, a–z, 0–9), and the following characters: + - = . _ : / @.
*
*
* -
*
* Cannot use "aws:" as a prefix for a key.
*
*
*
*
* @param tags
* Applies one or more tags to the route calculator resource. A tag is a key-value pair helps manage,
* identify, search, and filter your resources by labelling them.
*
* -
*
* For example: { "tag1" : "value1"
, "tag2" : "value2"
*
*
*
*
* Format: "key" : "value"
*
*
* Restrictions:
*
*
* -
*
* Maximum 50 tags per resource
*
*
* -
*
* Each resource tag must be unique with a maximum of one value.
*
*
* -
*
* Maximum key length: 128 Unicode characters in UTF-8
*
*
* -
*
* Maximum value length: 256 Unicode characters in UTF-8
*
*
* -
*
* Can use alphanumeric characters (A–Z, a–z, 0–9), and the following characters: + - = . _ : / @.
*
*
* -
*
* Cannot use "aws:" as a prefix for a key.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateRouteCalculatorRequest withTags(java.util.Map tags) {
setTags(tags);
return this;
}
/**
* Add a single Tags entry
*
* @see CreateRouteCalculatorRequest#withTags
* @returns a reference to this object so that method calls can be chained together.
*/
public CreateRouteCalculatorRequest addTagsEntry(String key, String value) {
if (null == this.tags) {
this.tags = new java.util.HashMap();
}
if (this.tags.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.tags.put(key, value);
return this;
}
/**
* Removes all the entries added into Tags.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateRouteCalculatorRequest clearTagsEntries() {
this.tags = null;
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getCalculatorName() != null)
sb.append("CalculatorName: ").append(getCalculatorName()).append(",");
if (getDataSource() != null)
sb.append("DataSource: ").append(getDataSource()).append(",");
if (getPricingPlan() != null)
sb.append("PricingPlan: ").append(getPricingPlan()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateRouteCalculatorRequest == false)
return false;
CreateRouteCalculatorRequest other = (CreateRouteCalculatorRequest) obj;
if (other.getCalculatorName() == null ^ this.getCalculatorName() == null)
return false;
if (other.getCalculatorName() != null && other.getCalculatorName().equals(this.getCalculatorName()) == false)
return false;
if (other.getDataSource() == null ^ this.getDataSource() == null)
return false;
if (other.getDataSource() != null && other.getDataSource().equals(this.getDataSource()) == false)
return false;
if (other.getPricingPlan() == null ^ this.getPricingPlan() == null)
return false;
if (other.getPricingPlan() != null && other.getPricingPlan().equals(this.getPricingPlan()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getCalculatorName() == null) ? 0 : getCalculatorName().hashCode());
hashCode = prime * hashCode + ((getDataSource() == null) ? 0 : getDataSource().hashCode());
hashCode = prime * hashCode + ((getPricingPlan() == null) ? 0 : getPricingPlan().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
return hashCode;
}
@Override
public CreateRouteCalculatorRequest clone() {
return (CreateRouteCalculatorRequest) super.clone();
}
}