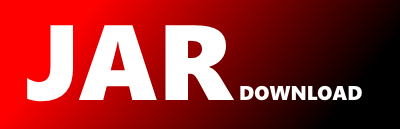
com.amazonaws.services.location.model.PutGeofenceRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-location Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.location.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class PutGeofenceRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The geofence collection to store the geofence in.
*
*/
private String collectionName;
/**
*
* An identifier for the geofence. For example, ExampleGeofence-1
.
*
*/
private String geofenceId;
/**
*
* Contains the details to specify the position of the geofence. Can be a polygon, a circle or a polygon encoded in
* Geobuf format. Including multiple selections will return a validation error.
*
*
*
* The
* geofence polygon format supports a maximum of 1,000 vertices. The Geofence
* Geobuf format supports a maximum of 100,000 vertices.
*
*
*/
private GeofenceGeometry geometry;
/**
*
* Associates one of more properties with the geofence. A property is a key-value pair stored with the geofence and
* added to any geofence event triggered with that geofence.
*
*
* Format: "key" : "value"
*
*/
private java.util.Map geofenceProperties;
/**
*
* The geofence collection to store the geofence in.
*
*
* @param collectionName
* The geofence collection to store the geofence in.
*/
public void setCollectionName(String collectionName) {
this.collectionName = collectionName;
}
/**
*
* The geofence collection to store the geofence in.
*
*
* @return The geofence collection to store the geofence in.
*/
public String getCollectionName() {
return this.collectionName;
}
/**
*
* The geofence collection to store the geofence in.
*
*
* @param collectionName
* The geofence collection to store the geofence in.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutGeofenceRequest withCollectionName(String collectionName) {
setCollectionName(collectionName);
return this;
}
/**
*
* An identifier for the geofence. For example, ExampleGeofence-1
.
*
*
* @param geofenceId
* An identifier for the geofence. For example, ExampleGeofence-1
.
*/
public void setGeofenceId(String geofenceId) {
this.geofenceId = geofenceId;
}
/**
*
* An identifier for the geofence. For example, ExampleGeofence-1
.
*
*
* @return An identifier for the geofence. For example, ExampleGeofence-1
.
*/
public String getGeofenceId() {
return this.geofenceId;
}
/**
*
* An identifier for the geofence. For example, ExampleGeofence-1
.
*
*
* @param geofenceId
* An identifier for the geofence. For example, ExampleGeofence-1
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutGeofenceRequest withGeofenceId(String geofenceId) {
setGeofenceId(geofenceId);
return this;
}
/**
*
* Contains the details to specify the position of the geofence. Can be a polygon, a circle or a polygon encoded in
* Geobuf format. Including multiple selections will return a validation error.
*
*
*
* The
* geofence polygon format supports a maximum of 1,000 vertices. The Geofence
* Geobuf format supports a maximum of 100,000 vertices.
*
*
*
* @param geometry
* Contains the details to specify the position of the geofence. Can be a polygon, a circle or a polygon
* encoded in Geobuf format. Including multiple selections will return a validation error.
*
* The
* geofence polygon format supports a maximum of 1,000 vertices. The Geofence Geobuf format supports a maximum of 100,000 vertices.
*
*/
public void setGeometry(GeofenceGeometry geometry) {
this.geometry = geometry;
}
/**
*
* Contains the details to specify the position of the geofence. Can be a polygon, a circle or a polygon encoded in
* Geobuf format. Including multiple selections will return a validation error.
*
*
*
* The
* geofence polygon format supports a maximum of 1,000 vertices. The Geofence
* Geobuf format supports a maximum of 100,000 vertices.
*
*
*
* @return Contains the details to specify the position of the geofence. Can be a polygon, a circle or a polygon
* encoded in Geobuf format. Including multiple selections will return a validation error.
*
* The
* geofence polygon format supports a maximum of 1,000 vertices. The Geofence Geobuf format supports a maximum of 100,000 vertices.
*
*/
public GeofenceGeometry getGeometry() {
return this.geometry;
}
/**
*
* Contains the details to specify the position of the geofence. Can be a polygon, a circle or a polygon encoded in
* Geobuf format. Including multiple selections will return a validation error.
*
*
*
* The
* geofence polygon format supports a maximum of 1,000 vertices. The Geofence
* Geobuf format supports a maximum of 100,000 vertices.
*
*
*
* @param geometry
* Contains the details to specify the position of the geofence. Can be a polygon, a circle or a polygon
* encoded in Geobuf format. Including multiple selections will return a validation error.
*
* The
* geofence polygon format supports a maximum of 1,000 vertices. The Geofence Geobuf format supports a maximum of 100,000 vertices.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutGeofenceRequest withGeometry(GeofenceGeometry geometry) {
setGeometry(geometry);
return this;
}
/**
*
* Associates one of more properties with the geofence. A property is a key-value pair stored with the geofence and
* added to any geofence event triggered with that geofence.
*
*
* Format: "key" : "value"
*
*
* @return Associates one of more properties with the geofence. A property is a key-value pair stored with the
* geofence and added to any geofence event triggered with that geofence.
*
* Format: "key" : "value"
*/
public java.util.Map getGeofenceProperties() {
return geofenceProperties;
}
/**
*
* Associates one of more properties with the geofence. A property is a key-value pair stored with the geofence and
* added to any geofence event triggered with that geofence.
*
*
* Format: "key" : "value"
*
*
* @param geofenceProperties
* Associates one of more properties with the geofence. A property is a key-value pair stored with the
* geofence and added to any geofence event triggered with that geofence.
*
* Format: "key" : "value"
*/
public void setGeofenceProperties(java.util.Map geofenceProperties) {
this.geofenceProperties = geofenceProperties;
}
/**
*
* Associates one of more properties with the geofence. A property is a key-value pair stored with the geofence and
* added to any geofence event triggered with that geofence.
*
*
* Format: "key" : "value"
*
*
* @param geofenceProperties
* Associates one of more properties with the geofence. A property is a key-value pair stored with the
* geofence and added to any geofence event triggered with that geofence.
*
* Format: "key" : "value"
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutGeofenceRequest withGeofenceProperties(java.util.Map geofenceProperties) {
setGeofenceProperties(geofenceProperties);
return this;
}
/**
* Add a single GeofenceProperties entry
*
* @see PutGeofenceRequest#withGeofenceProperties
* @returns a reference to this object so that method calls can be chained together.
*/
public PutGeofenceRequest addGeofencePropertiesEntry(String key, String value) {
if (null == this.geofenceProperties) {
this.geofenceProperties = new java.util.HashMap();
}
if (this.geofenceProperties.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.geofenceProperties.put(key, value);
return this;
}
/**
* Removes all the entries added into GeofenceProperties.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutGeofenceRequest clearGeofencePropertiesEntries() {
this.geofenceProperties = null;
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getCollectionName() != null)
sb.append("CollectionName: ").append(getCollectionName()).append(",");
if (getGeofenceId() != null)
sb.append("GeofenceId: ").append(getGeofenceId()).append(",");
if (getGeometry() != null)
sb.append("Geometry: ").append(getGeometry()).append(",");
if (getGeofenceProperties() != null)
sb.append("GeofenceProperties: ").append("***Sensitive Data Redacted***");
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof PutGeofenceRequest == false)
return false;
PutGeofenceRequest other = (PutGeofenceRequest) obj;
if (other.getCollectionName() == null ^ this.getCollectionName() == null)
return false;
if (other.getCollectionName() != null && other.getCollectionName().equals(this.getCollectionName()) == false)
return false;
if (other.getGeofenceId() == null ^ this.getGeofenceId() == null)
return false;
if (other.getGeofenceId() != null && other.getGeofenceId().equals(this.getGeofenceId()) == false)
return false;
if (other.getGeometry() == null ^ this.getGeometry() == null)
return false;
if (other.getGeometry() != null && other.getGeometry().equals(this.getGeometry()) == false)
return false;
if (other.getGeofenceProperties() == null ^ this.getGeofenceProperties() == null)
return false;
if (other.getGeofenceProperties() != null && other.getGeofenceProperties().equals(this.getGeofenceProperties()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getCollectionName() == null) ? 0 : getCollectionName().hashCode());
hashCode = prime * hashCode + ((getGeofenceId() == null) ? 0 : getGeofenceId().hashCode());
hashCode = prime * hashCode + ((getGeometry() == null) ? 0 : getGeometry().hashCode());
hashCode = prime * hashCode + ((getGeofenceProperties() == null) ? 0 : getGeofenceProperties().hashCode());
return hashCode;
}
@Override
public PutGeofenceRequest clone() {
return (PutGeofenceRequest) super.clone();
}
}