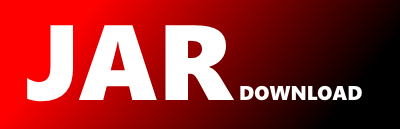
com.amazonaws.services.location.model.SearchPlaceIndexForTextSummary Maven / Gradle / Ivy
Show all versions of aws-java-sdk-location Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.location.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* A summary of the request sent by using SearchPlaceIndexForText
.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class SearchPlaceIndexForTextSummary implements Serializable, Cloneable, StructuredPojo {
/**
*
* The search text specified in the request.
*
*/
private String text;
/**
*
* Contains the coordinates for the optional bias position specified in the request.
*
*
* This parameter contains a pair of numbers. The first number represents the X coordinate, or longitude; the second
* number represents the Y coordinate, or latitude.
*
*
* For example, [-123.1174, 49.2847]
represents the position with longitude -123.1174
and
* latitude 49.2847
.
*
*/
private java.util.List biasPosition;
/**
*
* Contains the coordinates for the optional bounding box specified in the request.
*
*/
private java.util.List filterBBox;
/**
*
* Contains the optional country filter specified in the request.
*
*/
private java.util.List filterCountries;
/**
*
* Contains the optional result count limit specified in the request.
*
*/
private Integer maxResults;
/**
*
* The bounding box that fully contains all search results.
*
*
*
* If you specified the optional FilterBBox
parameter in the request, ResultBBox
is
* contained within FilterBBox
.
*
*
*/
private java.util.List resultBBox;
/**
*
* The geospatial data provider attached to the place index resource specified in the request. Values can be one of
* the following:
*
*
* -
*
* Esri
*
*
* -
*
* Grab
*
*
* -
*
* Here
*
*
*
*
* For more information about data providers, see Amazon Location
* Service data providers.
*
*/
private String dataSource;
/**
*
* The preferred language used to return results. Matches the language in the request. The value is a valid BCP 47 language tag, for example, en
for English.
*
*/
private String language;
/**
*
* The optional category filter specified in the request.
*
*/
private java.util.List filterCategories;
/**
*
* The search text specified in the request.
*
*
* @param text
* The search text specified in the request.
*/
public void setText(String text) {
this.text = text;
}
/**
*
* The search text specified in the request.
*
*
* @return The search text specified in the request.
*/
public String getText() {
return this.text;
}
/**
*
* The search text specified in the request.
*
*
* @param text
* The search text specified in the request.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SearchPlaceIndexForTextSummary withText(String text) {
setText(text);
return this;
}
/**
*
* Contains the coordinates for the optional bias position specified in the request.
*
*
* This parameter contains a pair of numbers. The first number represents the X coordinate, or longitude; the second
* number represents the Y coordinate, or latitude.
*
*
* For example, [-123.1174, 49.2847]
represents the position with longitude -123.1174
and
* latitude 49.2847
.
*
*
* @return Contains the coordinates for the optional bias position specified in the request.
*
* This parameter contains a pair of numbers. The first number represents the X coordinate, or longitude;
* the second number represents the Y coordinate, or latitude.
*
*
* For example, [-123.1174, 49.2847]
represents the position with longitude
* -123.1174
and latitude 49.2847
.
*/
public java.util.List getBiasPosition() {
return biasPosition;
}
/**
*
* Contains the coordinates for the optional bias position specified in the request.
*
*
* This parameter contains a pair of numbers. The first number represents the X coordinate, or longitude; the second
* number represents the Y coordinate, or latitude.
*
*
* For example, [-123.1174, 49.2847]
represents the position with longitude -123.1174
and
* latitude 49.2847
.
*
*
* @param biasPosition
* Contains the coordinates for the optional bias position specified in the request.
*
* This parameter contains a pair of numbers. The first number represents the X coordinate, or longitude; the
* second number represents the Y coordinate, or latitude.
*
*
* For example, [-123.1174, 49.2847]
represents the position with longitude
* -123.1174
and latitude 49.2847
.
*/
public void setBiasPosition(java.util.Collection biasPosition) {
if (biasPosition == null) {
this.biasPosition = null;
return;
}
this.biasPosition = new java.util.ArrayList(biasPosition);
}
/**
*
* Contains the coordinates for the optional bias position specified in the request.
*
*
* This parameter contains a pair of numbers. The first number represents the X coordinate, or longitude; the second
* number represents the Y coordinate, or latitude.
*
*
* For example, [-123.1174, 49.2847]
represents the position with longitude -123.1174
and
* latitude 49.2847
.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setBiasPosition(java.util.Collection)} or {@link #withBiasPosition(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param biasPosition
* Contains the coordinates for the optional bias position specified in the request.
*
* This parameter contains a pair of numbers. The first number represents the X coordinate, or longitude; the
* second number represents the Y coordinate, or latitude.
*
*
* For example, [-123.1174, 49.2847]
represents the position with longitude
* -123.1174
and latitude 49.2847
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SearchPlaceIndexForTextSummary withBiasPosition(Double... biasPosition) {
if (this.biasPosition == null) {
setBiasPosition(new java.util.ArrayList(biasPosition.length));
}
for (Double ele : biasPosition) {
this.biasPosition.add(ele);
}
return this;
}
/**
*
* Contains the coordinates for the optional bias position specified in the request.
*
*
* This parameter contains a pair of numbers. The first number represents the X coordinate, or longitude; the second
* number represents the Y coordinate, or latitude.
*
*
* For example, [-123.1174, 49.2847]
represents the position with longitude -123.1174
and
* latitude 49.2847
.
*
*
* @param biasPosition
* Contains the coordinates for the optional bias position specified in the request.
*
* This parameter contains a pair of numbers. The first number represents the X coordinate, or longitude; the
* second number represents the Y coordinate, or latitude.
*
*
* For example, [-123.1174, 49.2847]
represents the position with longitude
* -123.1174
and latitude 49.2847
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SearchPlaceIndexForTextSummary withBiasPosition(java.util.Collection biasPosition) {
setBiasPosition(biasPosition);
return this;
}
/**
*
* Contains the coordinates for the optional bounding box specified in the request.
*
*
* @return Contains the coordinates for the optional bounding box specified in the request.
*/
public java.util.List getFilterBBox() {
return filterBBox;
}
/**
*
* Contains the coordinates for the optional bounding box specified in the request.
*
*
* @param filterBBox
* Contains the coordinates for the optional bounding box specified in the request.
*/
public void setFilterBBox(java.util.Collection filterBBox) {
if (filterBBox == null) {
this.filterBBox = null;
return;
}
this.filterBBox = new java.util.ArrayList(filterBBox);
}
/**
*
* Contains the coordinates for the optional bounding box specified in the request.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setFilterBBox(java.util.Collection)} or {@link #withFilterBBox(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param filterBBox
* Contains the coordinates for the optional bounding box specified in the request.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SearchPlaceIndexForTextSummary withFilterBBox(Double... filterBBox) {
if (this.filterBBox == null) {
setFilterBBox(new java.util.ArrayList(filterBBox.length));
}
for (Double ele : filterBBox) {
this.filterBBox.add(ele);
}
return this;
}
/**
*
* Contains the coordinates for the optional bounding box specified in the request.
*
*
* @param filterBBox
* Contains the coordinates for the optional bounding box specified in the request.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SearchPlaceIndexForTextSummary withFilterBBox(java.util.Collection filterBBox) {
setFilterBBox(filterBBox);
return this;
}
/**
*
* Contains the optional country filter specified in the request.
*
*
* @return Contains the optional country filter specified in the request.
*/
public java.util.List getFilterCountries() {
return filterCountries;
}
/**
*
* Contains the optional country filter specified in the request.
*
*
* @param filterCountries
* Contains the optional country filter specified in the request.
*/
public void setFilterCountries(java.util.Collection filterCountries) {
if (filterCountries == null) {
this.filterCountries = null;
return;
}
this.filterCountries = new java.util.ArrayList(filterCountries);
}
/**
*
* Contains the optional country filter specified in the request.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setFilterCountries(java.util.Collection)} or {@link #withFilterCountries(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param filterCountries
* Contains the optional country filter specified in the request.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SearchPlaceIndexForTextSummary withFilterCountries(String... filterCountries) {
if (this.filterCountries == null) {
setFilterCountries(new java.util.ArrayList(filterCountries.length));
}
for (String ele : filterCountries) {
this.filterCountries.add(ele);
}
return this;
}
/**
*
* Contains the optional country filter specified in the request.
*
*
* @param filterCountries
* Contains the optional country filter specified in the request.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SearchPlaceIndexForTextSummary withFilterCountries(java.util.Collection filterCountries) {
setFilterCountries(filterCountries);
return this;
}
/**
*
* Contains the optional result count limit specified in the request.
*
*
* @param maxResults
* Contains the optional result count limit specified in the request.
*/
public void setMaxResults(Integer maxResults) {
this.maxResults = maxResults;
}
/**
*
* Contains the optional result count limit specified in the request.
*
*
* @return Contains the optional result count limit specified in the request.
*/
public Integer getMaxResults() {
return this.maxResults;
}
/**
*
* Contains the optional result count limit specified in the request.
*
*
* @param maxResults
* Contains the optional result count limit specified in the request.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SearchPlaceIndexForTextSummary withMaxResults(Integer maxResults) {
setMaxResults(maxResults);
return this;
}
/**
*
* The bounding box that fully contains all search results.
*
*
*
* If you specified the optional FilterBBox
parameter in the request, ResultBBox
is
* contained within FilterBBox
.
*
*
*
* @return The bounding box that fully contains all search results.
*
* If you specified the optional FilterBBox
parameter in the request, ResultBBox
* is contained within FilterBBox
.
*
*/
public java.util.List getResultBBox() {
return resultBBox;
}
/**
*
* The bounding box that fully contains all search results.
*
*
*
* If you specified the optional FilterBBox
parameter in the request, ResultBBox
is
* contained within FilterBBox
.
*
*
*
* @param resultBBox
* The bounding box that fully contains all search results.
*
* If you specified the optional FilterBBox
parameter in the request, ResultBBox
is
* contained within FilterBBox
.
*
*/
public void setResultBBox(java.util.Collection resultBBox) {
if (resultBBox == null) {
this.resultBBox = null;
return;
}
this.resultBBox = new java.util.ArrayList(resultBBox);
}
/**
*
* The bounding box that fully contains all search results.
*
*
*
* If you specified the optional FilterBBox
parameter in the request, ResultBBox
is
* contained within FilterBBox
.
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setResultBBox(java.util.Collection)} or {@link #withResultBBox(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param resultBBox
* The bounding box that fully contains all search results.
*
* If you specified the optional FilterBBox
parameter in the request, ResultBBox
is
* contained within FilterBBox
.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SearchPlaceIndexForTextSummary withResultBBox(Double... resultBBox) {
if (this.resultBBox == null) {
setResultBBox(new java.util.ArrayList(resultBBox.length));
}
for (Double ele : resultBBox) {
this.resultBBox.add(ele);
}
return this;
}
/**
*
* The bounding box that fully contains all search results.
*
*
*
* If you specified the optional FilterBBox
parameter in the request, ResultBBox
is
* contained within FilterBBox
.
*
*
*
* @param resultBBox
* The bounding box that fully contains all search results.
*
* If you specified the optional FilterBBox
parameter in the request, ResultBBox
is
* contained within FilterBBox
.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SearchPlaceIndexForTextSummary withResultBBox(java.util.Collection resultBBox) {
setResultBBox(resultBBox);
return this;
}
/**
*
* The geospatial data provider attached to the place index resource specified in the request. Values can be one of
* the following:
*
*
* -
*
* Esri
*
*
* -
*
* Grab
*
*
* -
*
* Here
*
*
*
*
* For more information about data providers, see Amazon Location
* Service data providers.
*
*
* @param dataSource
* The geospatial data provider attached to the place index resource specified in the request. Values can be
* one of the following:
*
* -
*
* Esri
*
*
* -
*
* Grab
*
*
* -
*
* Here
*
*
*
*
* For more information about data providers, see Amazon
* Location Service data providers.
*/
public void setDataSource(String dataSource) {
this.dataSource = dataSource;
}
/**
*
* The geospatial data provider attached to the place index resource specified in the request. Values can be one of
* the following:
*
*
* -
*
* Esri
*
*
* -
*
* Grab
*
*
* -
*
* Here
*
*
*
*
* For more information about data providers, see Amazon Location
* Service data providers.
*
*
* @return The geospatial data provider attached to the place index resource specified in the request. Values can be
* one of the following:
*
* -
*
* Esri
*
*
* -
*
* Grab
*
*
* -
*
* Here
*
*
*
*
* For more information about data providers, see Amazon
* Location Service data providers.
*/
public String getDataSource() {
return this.dataSource;
}
/**
*
* The geospatial data provider attached to the place index resource specified in the request. Values can be one of
* the following:
*
*
* -
*
* Esri
*
*
* -
*
* Grab
*
*
* -
*
* Here
*
*
*
*
* For more information about data providers, see Amazon Location
* Service data providers.
*
*
* @param dataSource
* The geospatial data provider attached to the place index resource specified in the request. Values can be
* one of the following:
*
* -
*
* Esri
*
*
* -
*
* Grab
*
*
* -
*
* Here
*
*
*
*
* For more information about data providers, see Amazon
* Location Service data providers.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SearchPlaceIndexForTextSummary withDataSource(String dataSource) {
setDataSource(dataSource);
return this;
}
/**
*
* The preferred language used to return results. Matches the language in the request. The value is a valid BCP 47 language tag, for example, en
for English.
*
*
* @param language
* The preferred language used to return results. Matches the language in the request. The value is a valid
* BCP 47 language tag, for example, en
for
* English.
*/
public void setLanguage(String language) {
this.language = language;
}
/**
*
* The preferred language used to return results. Matches the language in the request. The value is a valid BCP 47 language tag, for example, en
for English.
*
*
* @return The preferred language used to return results. Matches the language in the request. The value is a valid
* BCP 47 language tag, for example, en
for
* English.
*/
public String getLanguage() {
return this.language;
}
/**
*
* The preferred language used to return results. Matches the language in the request. The value is a valid BCP 47 language tag, for example, en
for English.
*
*
* @param language
* The preferred language used to return results. Matches the language in the request. The value is a valid
* BCP 47 language tag, for example, en
for
* English.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SearchPlaceIndexForTextSummary withLanguage(String language) {
setLanguage(language);
return this;
}
/**
*
* The optional category filter specified in the request.
*
*
* @return The optional category filter specified in the request.
*/
public java.util.List getFilterCategories() {
return filterCategories;
}
/**
*
* The optional category filter specified in the request.
*
*
* @param filterCategories
* The optional category filter specified in the request.
*/
public void setFilterCategories(java.util.Collection filterCategories) {
if (filterCategories == null) {
this.filterCategories = null;
return;
}
this.filterCategories = new java.util.ArrayList(filterCategories);
}
/**
*
* The optional category filter specified in the request.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setFilterCategories(java.util.Collection)} or {@link #withFilterCategories(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param filterCategories
* The optional category filter specified in the request.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SearchPlaceIndexForTextSummary withFilterCategories(String... filterCategories) {
if (this.filterCategories == null) {
setFilterCategories(new java.util.ArrayList(filterCategories.length));
}
for (String ele : filterCategories) {
this.filterCategories.add(ele);
}
return this;
}
/**
*
* The optional category filter specified in the request.
*
*
* @param filterCategories
* The optional category filter specified in the request.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SearchPlaceIndexForTextSummary withFilterCategories(java.util.Collection filterCategories) {
setFilterCategories(filterCategories);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getText() != null)
sb.append("Text: ").append("***Sensitive Data Redacted***").append(",");
if (getBiasPosition() != null)
sb.append("BiasPosition: ").append("***Sensitive Data Redacted***").append(",");
if (getFilterBBox() != null)
sb.append("FilterBBox: ").append("***Sensitive Data Redacted***").append(",");
if (getFilterCountries() != null)
sb.append("FilterCountries: ").append(getFilterCountries()).append(",");
if (getMaxResults() != null)
sb.append("MaxResults: ").append(getMaxResults()).append(",");
if (getResultBBox() != null)
sb.append("ResultBBox: ").append("***Sensitive Data Redacted***").append(",");
if (getDataSource() != null)
sb.append("DataSource: ").append(getDataSource()).append(",");
if (getLanguage() != null)
sb.append("Language: ").append(getLanguage()).append(",");
if (getFilterCategories() != null)
sb.append("FilterCategories: ").append(getFilterCategories());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof SearchPlaceIndexForTextSummary == false)
return false;
SearchPlaceIndexForTextSummary other = (SearchPlaceIndexForTextSummary) obj;
if (other.getText() == null ^ this.getText() == null)
return false;
if (other.getText() != null && other.getText().equals(this.getText()) == false)
return false;
if (other.getBiasPosition() == null ^ this.getBiasPosition() == null)
return false;
if (other.getBiasPosition() != null && other.getBiasPosition().equals(this.getBiasPosition()) == false)
return false;
if (other.getFilterBBox() == null ^ this.getFilterBBox() == null)
return false;
if (other.getFilterBBox() != null && other.getFilterBBox().equals(this.getFilterBBox()) == false)
return false;
if (other.getFilterCountries() == null ^ this.getFilterCountries() == null)
return false;
if (other.getFilterCountries() != null && other.getFilterCountries().equals(this.getFilterCountries()) == false)
return false;
if (other.getMaxResults() == null ^ this.getMaxResults() == null)
return false;
if (other.getMaxResults() != null && other.getMaxResults().equals(this.getMaxResults()) == false)
return false;
if (other.getResultBBox() == null ^ this.getResultBBox() == null)
return false;
if (other.getResultBBox() != null && other.getResultBBox().equals(this.getResultBBox()) == false)
return false;
if (other.getDataSource() == null ^ this.getDataSource() == null)
return false;
if (other.getDataSource() != null && other.getDataSource().equals(this.getDataSource()) == false)
return false;
if (other.getLanguage() == null ^ this.getLanguage() == null)
return false;
if (other.getLanguage() != null && other.getLanguage().equals(this.getLanguage()) == false)
return false;
if (other.getFilterCategories() == null ^ this.getFilterCategories() == null)
return false;
if (other.getFilterCategories() != null && other.getFilterCategories().equals(this.getFilterCategories()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getText() == null) ? 0 : getText().hashCode());
hashCode = prime * hashCode + ((getBiasPosition() == null) ? 0 : getBiasPosition().hashCode());
hashCode = prime * hashCode + ((getFilterBBox() == null) ? 0 : getFilterBBox().hashCode());
hashCode = prime * hashCode + ((getFilterCountries() == null) ? 0 : getFilterCountries().hashCode());
hashCode = prime * hashCode + ((getMaxResults() == null) ? 0 : getMaxResults().hashCode());
hashCode = prime * hashCode + ((getResultBBox() == null) ? 0 : getResultBBox().hashCode());
hashCode = prime * hashCode + ((getDataSource() == null) ? 0 : getDataSource().hashCode());
hashCode = prime * hashCode + ((getLanguage() == null) ? 0 : getLanguage().hashCode());
hashCode = prime * hashCode + ((getFilterCategories() == null) ? 0 : getFilterCategories().hashCode());
return hashCode;
}
@Override
public SearchPlaceIndexForTextSummary clone() {
try {
return (SearchPlaceIndexForTextSummary) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.location.model.transform.SearchPlaceIndexForTextSummaryMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}