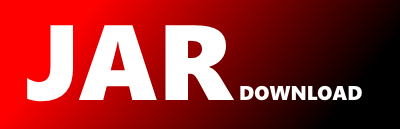
com.amazonaws.services.location.model.Step Maven / Gradle / Ivy
Show all versions of aws-java-sdk-location Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.location.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Represents an element of a leg within a route. A step contains instructions for how to move to the next step in the
* leg.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class Step implements Serializable, Cloneable, StructuredPojo {
/**
*
* The starting position of a step. If the position is the first step in the leg, this position is the same as the
* start position of the leg.
*
*/
private java.util.List startPosition;
/**
*
* The end position of a step. If the position the last step in the leg, this position is the same as the end
* position of the leg.
*
*/
private java.util.List endPosition;
/**
*
* The travel distance between the step's StartPosition
and EndPosition
.
*
*/
private Double distance;
/**
*
* The estimated travel time, in seconds, from the step's StartPosition
to the EndPosition
* . . The travel mode and departure time that you specify in the request determines the calculated time.
*
*/
private Double durationSeconds;
/**
*
* Represents the start position, or index, in a sequence of steps within the leg's line string geometry. For
* example, the index of the first step in a leg geometry is 0
.
*
*
* Included in the response for queries that set IncludeLegGeometry
to True
.
*
*/
private Integer geometryOffset;
/**
*
* The starting position of a step. If the position is the first step in the leg, this position is the same as the
* start position of the leg.
*
*
* @return The starting position of a step. If the position is the first step in the leg, this position is the same
* as the start position of the leg.
*/
public java.util.List getStartPosition() {
return startPosition;
}
/**
*
* The starting position of a step. If the position is the first step in the leg, this position is the same as the
* start position of the leg.
*
*
* @param startPosition
* The starting position of a step. If the position is the first step in the leg, this position is the same
* as the start position of the leg.
*/
public void setStartPosition(java.util.Collection startPosition) {
if (startPosition == null) {
this.startPosition = null;
return;
}
this.startPosition = new java.util.ArrayList(startPosition);
}
/**
*
* The starting position of a step. If the position is the first step in the leg, this position is the same as the
* start position of the leg.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setStartPosition(java.util.Collection)} or {@link #withStartPosition(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param startPosition
* The starting position of a step. If the position is the first step in the leg, this position is the same
* as the start position of the leg.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Step withStartPosition(Double... startPosition) {
if (this.startPosition == null) {
setStartPosition(new java.util.ArrayList(startPosition.length));
}
for (Double ele : startPosition) {
this.startPosition.add(ele);
}
return this;
}
/**
*
* The starting position of a step. If the position is the first step in the leg, this position is the same as the
* start position of the leg.
*
*
* @param startPosition
* The starting position of a step. If the position is the first step in the leg, this position is the same
* as the start position of the leg.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Step withStartPosition(java.util.Collection startPosition) {
setStartPosition(startPosition);
return this;
}
/**
*
* The end position of a step. If the position the last step in the leg, this position is the same as the end
* position of the leg.
*
*
* @return The end position of a step. If the position the last step in the leg, this position is the same as the
* end position of the leg.
*/
public java.util.List getEndPosition() {
return endPosition;
}
/**
*
* The end position of a step. If the position the last step in the leg, this position is the same as the end
* position of the leg.
*
*
* @param endPosition
* The end position of a step. If the position the last step in the leg, this position is the same as the end
* position of the leg.
*/
public void setEndPosition(java.util.Collection endPosition) {
if (endPosition == null) {
this.endPosition = null;
return;
}
this.endPosition = new java.util.ArrayList(endPosition);
}
/**
*
* The end position of a step. If the position the last step in the leg, this position is the same as the end
* position of the leg.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setEndPosition(java.util.Collection)} or {@link #withEndPosition(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param endPosition
* The end position of a step. If the position the last step in the leg, this position is the same as the end
* position of the leg.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Step withEndPosition(Double... endPosition) {
if (this.endPosition == null) {
setEndPosition(new java.util.ArrayList(endPosition.length));
}
for (Double ele : endPosition) {
this.endPosition.add(ele);
}
return this;
}
/**
*
* The end position of a step. If the position the last step in the leg, this position is the same as the end
* position of the leg.
*
*
* @param endPosition
* The end position of a step. If the position the last step in the leg, this position is the same as the end
* position of the leg.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Step withEndPosition(java.util.Collection endPosition) {
setEndPosition(endPosition);
return this;
}
/**
*
* The travel distance between the step's StartPosition
and EndPosition
.
*
*
* @param distance
* The travel distance between the step's StartPosition
and EndPosition
.
*/
public void setDistance(Double distance) {
this.distance = distance;
}
/**
*
* The travel distance between the step's StartPosition
and EndPosition
.
*
*
* @return The travel distance between the step's StartPosition
and EndPosition
.
*/
public Double getDistance() {
return this.distance;
}
/**
*
* The travel distance between the step's StartPosition
and EndPosition
.
*
*
* @param distance
* The travel distance between the step's StartPosition
and EndPosition
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Step withDistance(Double distance) {
setDistance(distance);
return this;
}
/**
*
* The estimated travel time, in seconds, from the step's StartPosition
to the EndPosition
* . . The travel mode and departure time that you specify in the request determines the calculated time.
*
*
* @param durationSeconds
* The estimated travel time, in seconds, from the step's StartPosition
to the
* EndPosition
. . The travel mode and departure time that you specify in the request determines
* the calculated time.
*/
public void setDurationSeconds(Double durationSeconds) {
this.durationSeconds = durationSeconds;
}
/**
*
* The estimated travel time, in seconds, from the step's StartPosition
to the EndPosition
* . . The travel mode and departure time that you specify in the request determines the calculated time.
*
*
* @return The estimated travel time, in seconds, from the step's StartPosition
to the
* EndPosition
. . The travel mode and departure time that you specify in the request determines
* the calculated time.
*/
public Double getDurationSeconds() {
return this.durationSeconds;
}
/**
*
* The estimated travel time, in seconds, from the step's StartPosition
to the EndPosition
* . . The travel mode and departure time that you specify in the request determines the calculated time.
*
*
* @param durationSeconds
* The estimated travel time, in seconds, from the step's StartPosition
to the
* EndPosition
. . The travel mode and departure time that you specify in the request determines
* the calculated time.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Step withDurationSeconds(Double durationSeconds) {
setDurationSeconds(durationSeconds);
return this;
}
/**
*
* Represents the start position, or index, in a sequence of steps within the leg's line string geometry. For
* example, the index of the first step in a leg geometry is 0
.
*
*
* Included in the response for queries that set IncludeLegGeometry
to True
.
*
*
* @param geometryOffset
* Represents the start position, or index, in a sequence of steps within the leg's line string geometry. For
* example, the index of the first step in a leg geometry is 0
.
*
* Included in the response for queries that set IncludeLegGeometry
to True
.
*/
public void setGeometryOffset(Integer geometryOffset) {
this.geometryOffset = geometryOffset;
}
/**
*
* Represents the start position, or index, in a sequence of steps within the leg's line string geometry. For
* example, the index of the first step in a leg geometry is 0
.
*
*
* Included in the response for queries that set IncludeLegGeometry
to True
.
*
*
* @return Represents the start position, or index, in a sequence of steps within the leg's line string geometry.
* For example, the index of the first step in a leg geometry is 0
.
*
* Included in the response for queries that set IncludeLegGeometry
to True
.
*/
public Integer getGeometryOffset() {
return this.geometryOffset;
}
/**
*
* Represents the start position, or index, in a sequence of steps within the leg's line string geometry. For
* example, the index of the first step in a leg geometry is 0
.
*
*
* Included in the response for queries that set IncludeLegGeometry
to True
.
*
*
* @param geometryOffset
* Represents the start position, or index, in a sequence of steps within the leg's line string geometry. For
* example, the index of the first step in a leg geometry is 0
.
*
* Included in the response for queries that set IncludeLegGeometry
to True
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Step withGeometryOffset(Integer geometryOffset) {
setGeometryOffset(geometryOffset);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getStartPosition() != null)
sb.append("StartPosition: ").append("***Sensitive Data Redacted***").append(",");
if (getEndPosition() != null)
sb.append("EndPosition: ").append("***Sensitive Data Redacted***").append(",");
if (getDistance() != null)
sb.append("Distance: ").append(getDistance()).append(",");
if (getDurationSeconds() != null)
sb.append("DurationSeconds: ").append(getDurationSeconds()).append(",");
if (getGeometryOffset() != null)
sb.append("GeometryOffset: ").append(getGeometryOffset());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof Step == false)
return false;
Step other = (Step) obj;
if (other.getStartPosition() == null ^ this.getStartPosition() == null)
return false;
if (other.getStartPosition() != null && other.getStartPosition().equals(this.getStartPosition()) == false)
return false;
if (other.getEndPosition() == null ^ this.getEndPosition() == null)
return false;
if (other.getEndPosition() != null && other.getEndPosition().equals(this.getEndPosition()) == false)
return false;
if (other.getDistance() == null ^ this.getDistance() == null)
return false;
if (other.getDistance() != null && other.getDistance().equals(this.getDistance()) == false)
return false;
if (other.getDurationSeconds() == null ^ this.getDurationSeconds() == null)
return false;
if (other.getDurationSeconds() != null && other.getDurationSeconds().equals(this.getDurationSeconds()) == false)
return false;
if (other.getGeometryOffset() == null ^ this.getGeometryOffset() == null)
return false;
if (other.getGeometryOffset() != null && other.getGeometryOffset().equals(this.getGeometryOffset()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getStartPosition() == null) ? 0 : getStartPosition().hashCode());
hashCode = prime * hashCode + ((getEndPosition() == null) ? 0 : getEndPosition().hashCode());
hashCode = prime * hashCode + ((getDistance() == null) ? 0 : getDistance().hashCode());
hashCode = prime * hashCode + ((getDurationSeconds() == null) ? 0 : getDurationSeconds().hashCode());
hashCode = prime * hashCode + ((getGeometryOffset() == null) ? 0 : getGeometryOffset().hashCode());
return hashCode;
}
@Override
public Step clone() {
try {
return (Step) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.location.model.transform.StepMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}