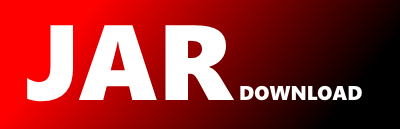
com.amazonaws.services.logs.AWSLogs Maven / Gradle / Ivy
/*
* Copyright 2011-2016 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not
* use this file except in compliance with the License. A copy of the License is
* located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.logs;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.logs.model.*;
/**
* Interface for accessing Amazon CloudWatch Logs.
*
*
* You can use Amazon CloudWatch Logs to monitor, store, and access your log
* files from Amazon Elastic Compute Cloud (Amazon EC2) instances, Amazon
* CloudTrail, or other sources. You can then retrieve the associated log data
* from CloudWatch Logs using the Amazon CloudWatch console, the CloudWatch Logs
* commands in the AWS CLI, the CloudWatch Logs API, or the CloudWatch Logs SDK.
*
*
* You can use CloudWatch Logs to:
*
*
* -
*
* Monitor Logs from Amazon EC2 Instances in Real-time: You can use
* CloudWatch Logs to monitor applications and systems using log data. For
* example, CloudWatch Logs can track the number of errors that occur in your
* application logs and send you a notification whenever the rate of errors
* exceeds a threshold you specify. CloudWatch Logs uses your log data for
* monitoring; so, no code changes are required. For example, you can monitor
* application logs for specific literal terms (such as
* "NullReferenceException") or count the number of occurrences of a literal
* term at a particular position in log data (such as "404" status codes in an
* Apache access log). When the term you are searching for is found, CloudWatch
* Logs reports the data to a Amazon CloudWatch metric that you specify.
*
*
* -
*
* Monitor Amazon CloudTrail Logged Events: You can create alarms in
* Amazon CloudWatch and receive notifications of particular API activity as
* captured by CloudTrail and use the notification to perform troubleshooting.
*
*
* -
*
* Archive Log Data: You can use CloudWatch Logs to store your log data
* in highly durable storage. You can change the log retention setting so that
* any log events older than this setting are automatically deleted. The
* CloudWatch Logs agent makes it easy to quickly send both rotated and
* non-rotated log data off of a host and into the log service. You can then
* access the raw log data when you need it.
*
*
*
*/
public interface AWSLogs {
/**
* The region metadata service name for computing region endpoints. You can
* use this value to retrieve metadata (such as supported regions) of the
* service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "logs";
/**
* Overrides the default endpoint for this client
* ("https://logs.us-east-1.amazonaws.com"). Callers can use this method to
* control which AWS region they want to work with.
*
* Callers can pass in just the endpoint (ex:
* "logs.us-east-1.amazonaws.com") or a full URL, including the protocol
* (ex: "https://logs.us-east-1.amazonaws.com"). If the protocol is not
* specified here, the default protocol from this client's
* {@link ClientConfiguration} will be used, which by default is HTTPS.
*
* For more information on using AWS regions with the AWS SDK for Java, and
* a complete list of all available endpoints for all AWS services, see: http://developer.amazonwebservices.com/connect/entry.jspa?externalID=
* 3912
*
* This method is not threadsafe. An endpoint should be configured when
* the client is created and before any service requests are made. Changing
* it afterwards creates inevitable race conditions for any service requests
* in transit or retrying.
*
* @param endpoint
* The endpoint (ex: "logs.us-east-1.amazonaws.com") or a full URL,
* including the protocol (ex:
* "https://logs.us-east-1.amazonaws.com") of the region specific AWS
* endpoint this client will communicate with.
*/
void setEndpoint(String endpoint);
/**
* An alternative to {@link AWSLogs#setEndpoint(String)}, sets the regional
* endpoint for this client's service calls. Callers can use this method to
* control which AWS region they want to work with.
*
* By default, all service endpoints in all regions use the https protocol.
* To use http instead, specify it in the {@link ClientConfiguration}
* supplied at construction.
*
* This method is not threadsafe. A region should be configured when the
* client is created and before any service requests are made. Changing it
* afterwards creates inevitable race conditions for any service requests in
* transit or retrying.
*
* @param region
* The region this client will communicate with. See
* {@link Region#getRegion(com.amazonaws.regions.Regions)} for
* accessing a given region. Must not be null and must be a region
* where the service is available.
*
* @see Region#getRegion(com.amazonaws.regions.Regions)
* @see Region#createClient(Class,
* com.amazonaws.auth.AWSCredentialsProvider, ClientConfiguration)
* @see Region#isServiceSupported(String)
*/
void setRegion(Region region);
/**
*
* Cancels an export task if it is in PENDING
or
* RUNNING
state.
*
*
* @param cancelExportTaskRequest
* @return Result of the CancelExportTask operation returned by the service.
* @throws InvalidParameterException
* Returned if a parameter of the request is incorrectly specified.
* @throws ResourceNotFoundException
* Returned if the specified resource does not exist.
* @throws InvalidOperationException
* Returned if the operation is not valid on the specified resource
* @throws ServiceUnavailableException
* Returned if the service cannot complete the request.
* @sample AWSLogs.CancelExportTask
*/
CancelExportTaskResult cancelExportTask(
CancelExportTaskRequest cancelExportTaskRequest);
/**
*
* Creates an ExportTask
which allows you to efficiently export
* data from a Log Group to your Amazon S3 bucket.
*
*
* This is an asynchronous call. If all the required information is
* provided, this API will initiate an export task and respond with the task
* Id. Once started, DescribeExportTasks
can be used to get the
* status of an export task. You can only have one active (
* RUNNING
or PENDING
) export task at a time, per
* account.
*
*
* You can export logs from multiple log groups or multiple time ranges to
* the same Amazon S3 bucket. To separate out log data for each export task,
* you can specify a prefix that will be used as the Amazon S3 key prefix
* for all exported objects.
*
*
* @param createExportTaskRequest
* @return Result of the CreateExportTask operation returned by the service.
* @throws InvalidParameterException
* Returned if a parameter of the request is incorrectly specified.
* @throws LimitExceededException
* Returned if you have reached the maximum number of resources that
* can be created.
* @throws OperationAbortedException
* Returned if multiple requests to update the same resource were in
* conflict.
* @throws ServiceUnavailableException
* Returned if the service cannot complete the request.
* @throws ResourceNotFoundException
* Returned if the specified resource does not exist.
* @throws ResourceAlreadyExistsException
* Returned if the specified resource already exists.
* @sample AWSLogs.CreateExportTask
*/
CreateExportTaskResult createExportTask(
CreateExportTaskRequest createExportTaskRequest);
/**
*
* Creates a new log group with the specified name. The name of the log
* group must be unique within a region for an AWS account. You can create
* up to 500 log groups per account.
*
*
* You must use the following guidelines when naming a log group:
*
*
* -
*
* Log group names can be between 1 and 512 characters long.
*
*
* -
*
* Allowed characters are a-z, A-Z, 0-9, '_' (underscore), '-' (hyphen), '/'
* (forward slash), and '.' (period).
*
*
*
*
* @param createLogGroupRequest
* @return Result of the CreateLogGroup operation returned by the service.
* @throws InvalidParameterException
* Returned if a parameter of the request is incorrectly specified.
* @throws ResourceAlreadyExistsException
* Returned if the specified resource already exists.
* @throws LimitExceededException
* Returned if you have reached the maximum number of resources that
* can be created.
* @throws OperationAbortedException
* Returned if multiple requests to update the same resource were in
* conflict.
* @throws ServiceUnavailableException
* Returned if the service cannot complete the request.
* @sample AWSLogs.CreateLogGroup
*/
CreateLogGroupResult createLogGroup(
CreateLogGroupRequest createLogGroupRequest);
/**
*
* Creates a new log stream in the specified log group. The name of the log
* stream must be unique within the log group. There is no limit on the
* number of log streams that can exist in a log group.
*
*
* You must use the following guidelines when naming a log stream:
*
*
* -
*
* Log stream names can be between 1 and 512 characters long.
*
*
* -
*
* The ':' colon character is not allowed.
*
*
*
*
* @param createLogStreamRequest
* @return Result of the CreateLogStream operation returned by the service.
* @throws InvalidParameterException
* Returned if a parameter of the request is incorrectly specified.
* @throws ResourceAlreadyExistsException
* Returned if the specified resource already exists.
* @throws ResourceNotFoundException
* Returned if the specified resource does not exist.
* @throws ServiceUnavailableException
* Returned if the service cannot complete the request.
* @sample AWSLogs.CreateLogStream
*/
CreateLogStreamResult createLogStream(
CreateLogStreamRequest createLogStreamRequest);
/**
*
* Deletes the destination with the specified name and eventually disables
* all the subscription filters that publish to it. This will not delete the
* physical resource encapsulated by the destination.
*
*
* @param deleteDestinationRequest
* @return Result of the DeleteDestination operation returned by the
* service.
* @throws InvalidParameterException
* Returned if a parameter of the request is incorrectly specified.
* @throws ResourceNotFoundException
* Returned if the specified resource does not exist.
* @throws OperationAbortedException
* Returned if multiple requests to update the same resource were in
* conflict.
* @throws ServiceUnavailableException
* Returned if the service cannot complete the request.
* @sample AWSLogs.DeleteDestination
*/
DeleteDestinationResult deleteDestination(
DeleteDestinationRequest deleteDestinationRequest);
/**
*
* Deletes the log group with the specified name and permanently deletes all
* the archived log events associated with it.
*
*
* @param deleteLogGroupRequest
* @return Result of the DeleteLogGroup operation returned by the service.
* @throws InvalidParameterException
* Returned if a parameter of the request is incorrectly specified.
* @throws ResourceNotFoundException
* Returned if the specified resource does not exist.
* @throws OperationAbortedException
* Returned if multiple requests to update the same resource were in
* conflict.
* @throws ServiceUnavailableException
* Returned if the service cannot complete the request.
* @sample AWSLogs.DeleteLogGroup
*/
DeleteLogGroupResult deleteLogGroup(
DeleteLogGroupRequest deleteLogGroupRequest);
/**
*
* Deletes a log stream and permanently deletes all the archived log events
* associated with it.
*
*
* @param deleteLogStreamRequest
* @return Result of the DeleteLogStream operation returned by the service.
* @throws InvalidParameterException
* Returned if a parameter of the request is incorrectly specified.
* @throws ResourceNotFoundException
* Returned if the specified resource does not exist.
* @throws OperationAbortedException
* Returned if multiple requests to update the same resource were in
* conflict.
* @throws ServiceUnavailableException
* Returned if the service cannot complete the request.
* @sample AWSLogs.DeleteLogStream
*/
DeleteLogStreamResult deleteLogStream(
DeleteLogStreamRequest deleteLogStreamRequest);
/**
*
* Deletes a metric filter associated with the specified log group.
*
*
* @param deleteMetricFilterRequest
* @return Result of the DeleteMetricFilter operation returned by the
* service.
* @throws InvalidParameterException
* Returned if a parameter of the request is incorrectly specified.
* @throws ResourceNotFoundException
* Returned if the specified resource does not exist.
* @throws OperationAbortedException
* Returned if multiple requests to update the same resource were in
* conflict.
* @throws ServiceUnavailableException
* Returned if the service cannot complete the request.
* @sample AWSLogs.DeleteMetricFilter
*/
DeleteMetricFilterResult deleteMetricFilter(
DeleteMetricFilterRequest deleteMetricFilterRequest);
/**
*
* Deletes the retention policy of the specified log group. Log events would
* not expire if they belong to log groups without a retention policy.
*
*
* @param deleteRetentionPolicyRequest
* @return Result of the DeleteRetentionPolicy operation returned by the
* service.
* @throws InvalidParameterException
* Returned if a parameter of the request is incorrectly specified.
* @throws ResourceNotFoundException
* Returned if the specified resource does not exist.
* @throws OperationAbortedException
* Returned if multiple requests to update the same resource were in
* conflict.
* @throws ServiceUnavailableException
* Returned if the service cannot complete the request.
* @sample AWSLogs.DeleteRetentionPolicy
*/
DeleteRetentionPolicyResult deleteRetentionPolicy(
DeleteRetentionPolicyRequest deleteRetentionPolicyRequest);
/**
*
* Deletes a subscription filter associated with the specified log group.
*
*
* @param deleteSubscriptionFilterRequest
* @return Result of the DeleteSubscriptionFilter operation returned by the
* service.
* @throws InvalidParameterException
* Returned if a parameter of the request is incorrectly specified.
* @throws ResourceNotFoundException
* Returned if the specified resource does not exist.
* @throws OperationAbortedException
* Returned if multiple requests to update the same resource were in
* conflict.
* @throws ServiceUnavailableException
* Returned if the service cannot complete the request.
* @sample AWSLogs.DeleteSubscriptionFilter
*/
DeleteSubscriptionFilterResult deleteSubscriptionFilter(
DeleteSubscriptionFilterRequest deleteSubscriptionFilterRequest);
/**
*
* Returns all the destinations that are associated with the AWS account
* making the request. The list returned in the response is ASCII-sorted by
* destination name.
*
*
* By default, this operation returns up to 50 destinations. If there are
* more destinations to list, the response would contain a
* nextToken
value in the response body. You can also limit the
* number of destinations returned in the response by specifying the
* limit
parameter in the request.
*
*
* @param describeDestinationsRequest
* @return Result of the DescribeDestinations operation returned by the
* service.
* @throws InvalidParameterException
* Returned if a parameter of the request is incorrectly specified.
* @throws ServiceUnavailableException
* Returned if the service cannot complete the request.
* @sample AWSLogs.DescribeDestinations
*/
DescribeDestinationsResult describeDestinations(
DescribeDestinationsRequest describeDestinationsRequest);
/**
* Simplified method form for invoking the DescribeDestinations operation.
*
* @see #describeDestinations(DescribeDestinationsRequest)
*/
DescribeDestinationsResult describeDestinations();
/**
*
* Returns all the export tasks that are associated with the AWS account
* making the request. The export tasks can be filtered based on
* TaskId
or TaskStatus
.
*
*
* By default, this operation returns up to 50 export tasks that satisfy the
* specified filters. If there are more export tasks to list, the response
* would contain a nextToken
value in the response body. You
* can also limit the number of export tasks returned in the response by
* specifying the limit
parameter in the request.
*
*
* @param describeExportTasksRequest
* @return Result of the DescribeExportTasks operation returned by the
* service.
* @throws InvalidParameterException
* Returned if a parameter of the request is incorrectly specified.
* @throws ServiceUnavailableException
* Returned if the service cannot complete the request.
* @sample AWSLogs.DescribeExportTasks
*/
DescribeExportTasksResult describeExportTasks(
DescribeExportTasksRequest describeExportTasksRequest);
/**
*
* Returns all the log groups that are associated with the AWS account
* making the request. The list returned in the response is ASCII-sorted by
* log group name.
*
*
* By default, this operation returns up to 50 log groups. If there are more
* log groups to list, the response would contain a nextToken
* value in the response body. You can also limit the number of log groups
* returned in the response by specifying the limit
parameter
* in the request.
*
*
* @param describeLogGroupsRequest
* @return Result of the DescribeLogGroups operation returned by the
* service.
* @throws InvalidParameterException
* Returned if a parameter of the request is incorrectly specified.
* @throws ServiceUnavailableException
* Returned if the service cannot complete the request.
* @sample AWSLogs.DescribeLogGroups
*/
DescribeLogGroupsResult describeLogGroups(
DescribeLogGroupsRequest describeLogGroupsRequest);
/**
* Simplified method form for invoking the DescribeLogGroups operation.
*
* @see #describeLogGroups(DescribeLogGroupsRequest)
*/
DescribeLogGroupsResult describeLogGroups();
/**
*
* Returns all the log streams that are associated with the specified log
* group. The list returned in the response is ASCII-sorted by log stream
* name.
*
*
* By default, this operation returns up to 50 log streams. If there are
* more log streams to list, the response would contain a
* nextToken
value in the response body. You can also limit the
* number of log streams returned in the response by specifying the
* limit
parameter in the request. This operation has a limit
* of five transactions per second, after which transactions are throttled.
*
*
* @param describeLogStreamsRequest
* @return Result of the DescribeLogStreams operation returned by the
* service.
* @throws InvalidParameterException
* Returned if a parameter of the request is incorrectly specified.
* @throws ResourceNotFoundException
* Returned if the specified resource does not exist.
* @throws ServiceUnavailableException
* Returned if the service cannot complete the request.
* @sample AWSLogs.DescribeLogStreams
*/
DescribeLogStreamsResult describeLogStreams(
DescribeLogStreamsRequest describeLogStreamsRequest);
/**
*
* Returns all the metrics filters associated with the specified log group.
* The list returned in the response is ASCII-sorted by filter name.
*
*
* By default, this operation returns up to 50 metric filters. If there are
* more metric filters to list, the response would contain a
* nextToken
value in the response body. You can also limit the
* number of metric filters returned in the response by specifying the
* limit
parameter in the request.
*
*
* @param describeMetricFiltersRequest
* @return Result of the DescribeMetricFilters operation returned by the
* service.
* @throws InvalidParameterException
* Returned if a parameter of the request is incorrectly specified.
* @throws ResourceNotFoundException
* Returned if the specified resource does not exist.
* @throws ServiceUnavailableException
* Returned if the service cannot complete the request.
* @sample AWSLogs.DescribeMetricFilters
*/
DescribeMetricFiltersResult describeMetricFilters(
DescribeMetricFiltersRequest describeMetricFiltersRequest);
/**
*
* Returns all the subscription filters associated with the specified log
* group. The list returned in the response is ASCII-sorted by filter name.
*
*
* By default, this operation returns up to 50 subscription filters. If
* there are more subscription filters to list, the response would contain a
* nextToken
value in the response body. You can also limit the
* number of subscription filters returned in the response by specifying the
* limit
parameter in the request.
*
*
* @param describeSubscriptionFiltersRequest
* @return Result of the DescribeSubscriptionFilters operation returned by
* the service.
* @throws InvalidParameterException
* Returned if a parameter of the request is incorrectly specified.
* @throws ResourceNotFoundException
* Returned if the specified resource does not exist.
* @throws ServiceUnavailableException
* Returned if the service cannot complete the request.
* @sample AWSLogs.DescribeSubscriptionFilters
*/
DescribeSubscriptionFiltersResult describeSubscriptionFilters(
DescribeSubscriptionFiltersRequest describeSubscriptionFiltersRequest);
/**
*
* Retrieves log events, optionally filtered by a filter pattern from the
* specified log group. You can provide an optional time range to filter the
* results on the event timestamp
. You can limit the streams
* searched to an explicit list of logStreamNames
.
*
*
* By default, this operation returns as much matching log events as can fit
* in a response size of 1MB, up to 10,000 log events, or all the events
* found within a time-bounded scan window. If the response includes a
* nextToken
, then there is more data to search, and the search
* can be resumed with a new request providing the nextToken. The response
* will contain a list of searchedLogStreams
that contains
* information about which streams were searched in the request and whether
* they have been searched completely or require further pagination. The
* limit
parameter in the request can be used to specify the
* maximum number of events to return in a page.
*
*
* @param filterLogEventsRequest
* @return Result of the FilterLogEvents operation returned by the service.
* @throws InvalidParameterException
* Returned if a parameter of the request is incorrectly specified.
* @throws ResourceNotFoundException
* Returned if the specified resource does not exist.
* @throws ServiceUnavailableException
* Returned if the service cannot complete the request.
* @sample AWSLogs.FilterLogEvents
*/
FilterLogEventsResult filterLogEvents(
FilterLogEventsRequest filterLogEventsRequest);
/**
*
* Retrieves log events from the specified log stream. You can provide an
* optional time range to filter the results on the event
* timestamp
.
*
*
* By default, this operation returns as much log events as can fit in a
* response size of 1MB, up to 10,000 log events. The response will always
* include a nextForwardToken
and a
* nextBackwardToken
in the response body. You can use any of
* these tokens in subsequent GetLogEvents
requests to paginate
* through events in either forward or backward direction. You can also
* limit the number of log events returned in the response by specifying the
* limit
parameter in the request.
*
*
* @param getLogEventsRequest
* @return Result of the GetLogEvents operation returned by the service.
* @throws InvalidParameterException
* Returned if a parameter of the request is incorrectly specified.
* @throws ResourceNotFoundException
* Returned if the specified resource does not exist.
* @throws ServiceUnavailableException
* Returned if the service cannot complete the request.
* @sample AWSLogs.GetLogEvents
*/
GetLogEventsResult getLogEvents(GetLogEventsRequest getLogEventsRequest);
/**
*
* Creates or updates a Destination
. A destination encapsulates
* a physical resource (such as a Kinesis stream) and allows you to
* subscribe to a real-time stream of log events of a different account,
* ingested through PutLogEvents
requests. Currently, the only
* supported physical resource is a Amazon Kinesis stream belonging to the
* same account as the destination.
*
*
* A destination controls what is written to its Amazon Kinesis stream
* through an access policy. By default, PutDestination does not set any
* access policy with the destination, which means a cross-account user will
* not be able to call PutSubscriptionFilter
against this
* destination. To enable that, the destination owner must call
* PutDestinationPolicy
after PutDestination.
*
*
* @param putDestinationRequest
* @return Result of the PutDestination operation returned by the service.
* @throws InvalidParameterException
* Returned if a parameter of the request is incorrectly specified.
* @throws OperationAbortedException
* Returned if multiple requests to update the same resource were in
* conflict.
* @throws ServiceUnavailableException
* Returned if the service cannot complete the request.
* @sample AWSLogs.PutDestination
*/
PutDestinationResult putDestination(
PutDestinationRequest putDestinationRequest);
/**
*
* Creates or updates an access policy associated with an existing
* Destination
. An access policy is an IAM policy document that is used to authorize claims to register a
* subscription filter against a given destination.
*
*
* @param putDestinationPolicyRequest
* @return Result of the PutDestinationPolicy operation returned by the
* service.
* @throws InvalidParameterException
* Returned if a parameter of the request is incorrectly specified.
* @throws OperationAbortedException
* Returned if multiple requests to update the same resource were in
* conflict.
* @throws ServiceUnavailableException
* Returned if the service cannot complete the request.
* @sample AWSLogs.PutDestinationPolicy
*/
PutDestinationPolicyResult putDestinationPolicy(
PutDestinationPolicyRequest putDestinationPolicyRequest);
/**
*
* Uploads a batch of log events to the specified log stream.
*
*
* Every PutLogEvents request must include the sequenceToken
* obtained from the response of the previous request. An upload in a newly
* created log stream does not require a sequenceToken
. You can
* also get the sequenceToken
using DescribeLogStreams.
*
*
* The batch of events must satisfy the following constraints:
*
*
* -
*
* The maximum batch size is 1,048,576 bytes, and this size is calculated as
* the sum of all event messages in UTF-8, plus 26 bytes for each log event.
*
*
* -
*
* None of the log events in the batch can be more than 2 hours in the
* future.
*
*
* -
*
* None of the log events in the batch can be older than 14 days or the
* retention period of the log group.
*
*
* -
*
* The log events in the batch must be in chronological ordered by their
* timestamp
.
*
*
* -
*
* The maximum number of log events in a batch is 10,000.
*
*
* -
*
* A batch of log events in a single PutLogEvents request cannot span more
* than 24 hours. Otherwise, the PutLogEvents operation will fail.
*
*
*
*
* @param putLogEventsRequest
* @return Result of the PutLogEvents operation returned by the service.
* @throws InvalidParameterException
* Returned if a parameter of the request is incorrectly specified.
* @throws InvalidSequenceTokenException
* @throws DataAlreadyAcceptedException
* @throws ResourceNotFoundException
* Returned if the specified resource does not exist.
* @throws ServiceUnavailableException
* Returned if the service cannot complete the request.
* @sample AWSLogs.PutLogEvents
*/
PutLogEventsResult putLogEvents(PutLogEventsRequest putLogEventsRequest);
/**
*
* Creates or updates a metric filter and associates it with the specified
* log group. Metric filters allow you to configure rules to extract metric
* data from log events ingested through PutLogEvents
requests.
*
*
* The maximum number of metric filters that can be associated with a log
* group is 100.
*
*
* @param putMetricFilterRequest
* @return Result of the PutMetricFilter operation returned by the service.
* @throws InvalidParameterException
* Returned if a parameter of the request is incorrectly specified.
* @throws ResourceNotFoundException
* Returned if the specified resource does not exist.
* @throws OperationAbortedException
* Returned if multiple requests to update the same resource were in
* conflict.
* @throws LimitExceededException
* Returned if you have reached the maximum number of resources that
* can be created.
* @throws ServiceUnavailableException
* Returned if the service cannot complete the request.
* @sample AWSLogs.PutMetricFilter
*/
PutMetricFilterResult putMetricFilter(
PutMetricFilterRequest putMetricFilterRequest);
/**
*
* Sets the retention of the specified log group. A retention policy allows
* you to configure the number of days you want to retain log events in the
* specified log group.
*
*
* @param putRetentionPolicyRequest
* @return Result of the PutRetentionPolicy operation returned by the
* service.
* @throws InvalidParameterException
* Returned if a parameter of the request is incorrectly specified.
* @throws ResourceNotFoundException
* Returned if the specified resource does not exist.
* @throws OperationAbortedException
* Returned if multiple requests to update the same resource were in
* conflict.
* @throws ServiceUnavailableException
* Returned if the service cannot complete the request.
* @sample AWSLogs.PutRetentionPolicy
*/
PutRetentionPolicyResult putRetentionPolicy(
PutRetentionPolicyRequest putRetentionPolicyRequest);
/**
*
* Creates or updates a subscription filter and associates it with the
* specified log group. Subscription filters allow you to subscribe to a
* real-time stream of log events ingested through PutLogEvents
* requests and have them delivered to a specific destination. Currently,
* the supported destinations are:
*
*
* -
*
* An Amazon Kinesis stream belonging to the same account as the
* subscription filter, for same-account delivery.
*
*
* -
*
* A logical destination (used via an ARN of Destination
)
* belonging to a different account, for cross-account delivery.
*
*
* -
*
* An Amazon Kinesis Firehose stream belonging to the same account as the
* subscription filter, for same-account delivery.
*
*
* -
*
* An AWS Lambda function belonging to the same account as the subscription
* filter, for same-account delivery.
*
*
*
*
* Currently there can only be one subscription filter associated with a log
* group.
*
*
* @param putSubscriptionFilterRequest
* @return Result of the PutSubscriptionFilter operation returned by the
* service.
* @throws InvalidParameterException
* Returned if a parameter of the request is incorrectly specified.
* @throws ResourceNotFoundException
* Returned if the specified resource does not exist.
* @throws OperationAbortedException
* Returned if multiple requests to update the same resource were in
* conflict.
* @throws LimitExceededException
* Returned if you have reached the maximum number of resources that
* can be created.
* @throws ServiceUnavailableException
* Returned if the service cannot complete the request.
* @sample AWSLogs.PutSubscriptionFilter
*/
PutSubscriptionFilterResult putSubscriptionFilter(
PutSubscriptionFilterRequest putSubscriptionFilterRequest);
/**
*
* Tests the filter pattern of a metric filter against a sample of log event
* messages. You can use this operation to validate the correctness of a
* metric filter pattern.
*
*
* @param testMetricFilterRequest
* @return Result of the TestMetricFilter operation returned by the service.
* @throws InvalidParameterException
* Returned if a parameter of the request is incorrectly specified.
* @throws ServiceUnavailableException
* Returned if the service cannot complete the request.
* @sample AWSLogs.TestMetricFilter
*/
TestMetricFilterResult testMetricFilter(
TestMetricFilterRequest testMetricFilterRequest);
/**
* Shuts down this client object, releasing any resources that might be held
* open. This is an optional method, and callers are not expected to call
* it, but can if they want to explicitly release any open resources. Once a
* client has been shutdown, it should not be used to make any more
* requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request,
* typically used for debugging issues where a service isn't acting as
* expected. This data isn't considered part of the result data returned by
* an operation, so it's available through this separate, diagnostic
* interface.
*
* Response metadata is only cached for a limited period of time, so if you
* need to access this extra diagnostic information for an executed request,
* you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none
* is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}