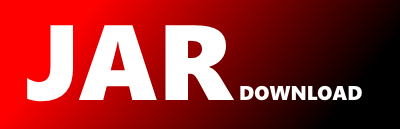
com.amazonaws.services.logs.model.CreateExportTaskRequest Maven / Gradle / Ivy
/*
* Copyright 2011-2016 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not
* use this file except in compliance with the License. A copy of the License is
* located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.logs.model;
import java.io.Serializable;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
*/
public class CreateExportTaskRequest extends AmazonWebServiceRequest implements
Serializable, Cloneable {
/**
*
* The name of the export task.
*
*/
private String taskName;
/**
*
* The name of the log group to export.
*
*/
private String logGroupName;
/**
*
* Will only export log streams that match the provided logStreamNamePrefix.
* If you don't specify a value, no prefix filter is applied.
*
*/
private String logStreamNamePrefix;
/**
*
* A point in time expressed as the number of milliseconds since Jan 1, 1970
* 00:00:00 UTC. It indicates the start time of the range for the request.
* Events with a timestamp prior to this time will not be exported.
*
*/
private Long from;
/**
*
* A point in time expressed as the number of milliseconds since Jan 1, 1970
* 00:00:00 UTC. It indicates the end time of the range for the request.
* Events with a timestamp later than this time will not be exported.
*
*/
private Long to;
/**
*
* Name of Amazon S3 bucket to which the log data will be exported.
*
*
* Note: Only buckets in the same AWS region are supported.
*
*/
private String destination;
/**
*
* Prefix that will be used as the start of Amazon S3 key for every object
* exported. If not specified, this defaults to 'exportedlogs'.
*
*/
private String destinationPrefix;
/**
*
* The name of the export task.
*
*
* @param taskName
* The name of the export task.
*/
public void setTaskName(String taskName) {
this.taskName = taskName;
}
/**
*
* The name of the export task.
*
*
* @return The name of the export task.
*/
public String getTaskName() {
return this.taskName;
}
/**
*
* The name of the export task.
*
*
* @param taskName
* The name of the export task.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public CreateExportTaskRequest withTaskName(String taskName) {
setTaskName(taskName);
return this;
}
/**
*
* The name of the log group to export.
*
*
* @param logGroupName
* The name of the log group to export.
*/
public void setLogGroupName(String logGroupName) {
this.logGroupName = logGroupName;
}
/**
*
* The name of the log group to export.
*
*
* @return The name of the log group to export.
*/
public String getLogGroupName() {
return this.logGroupName;
}
/**
*
* The name of the log group to export.
*
*
* @param logGroupName
* The name of the log group to export.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public CreateExportTaskRequest withLogGroupName(String logGroupName) {
setLogGroupName(logGroupName);
return this;
}
/**
*
* Will only export log streams that match the provided logStreamNamePrefix.
* If you don't specify a value, no prefix filter is applied.
*
*
* @param logStreamNamePrefix
* Will only export log streams that match the provided
* logStreamNamePrefix. If you don't specify a value, no prefix
* filter is applied.
*/
public void setLogStreamNamePrefix(String logStreamNamePrefix) {
this.logStreamNamePrefix = logStreamNamePrefix;
}
/**
*
* Will only export log streams that match the provided logStreamNamePrefix.
* If you don't specify a value, no prefix filter is applied.
*
*
* @return Will only export log streams that match the provided
* logStreamNamePrefix. If you don't specify a value, no prefix
* filter is applied.
*/
public String getLogStreamNamePrefix() {
return this.logStreamNamePrefix;
}
/**
*
* Will only export log streams that match the provided logStreamNamePrefix.
* If you don't specify a value, no prefix filter is applied.
*
*
* @param logStreamNamePrefix
* Will only export log streams that match the provided
* logStreamNamePrefix. If you don't specify a value, no prefix
* filter is applied.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public CreateExportTaskRequest withLogStreamNamePrefix(
String logStreamNamePrefix) {
setLogStreamNamePrefix(logStreamNamePrefix);
return this;
}
/**
*
* A point in time expressed as the number of milliseconds since Jan 1, 1970
* 00:00:00 UTC. It indicates the start time of the range for the request.
* Events with a timestamp prior to this time will not be exported.
*
*
* @param from
* A point in time expressed as the number of milliseconds since Jan
* 1, 1970 00:00:00 UTC. It indicates the start time of the range for
* the request. Events with a timestamp prior to this time will not
* be exported.
*/
public void setFrom(Long from) {
this.from = from;
}
/**
*
* A point in time expressed as the number of milliseconds since Jan 1, 1970
* 00:00:00 UTC. It indicates the start time of the range for the request.
* Events with a timestamp prior to this time will not be exported.
*
*
* @return A point in time expressed as the number of milliseconds since Jan
* 1, 1970 00:00:00 UTC. It indicates the start time of the range
* for the request. Events with a timestamp prior to this time will
* not be exported.
*/
public Long getFrom() {
return this.from;
}
/**
*
* A point in time expressed as the number of milliseconds since Jan 1, 1970
* 00:00:00 UTC. It indicates the start time of the range for the request.
* Events with a timestamp prior to this time will not be exported.
*
*
* @param from
* A point in time expressed as the number of milliseconds since Jan
* 1, 1970 00:00:00 UTC. It indicates the start time of the range for
* the request. Events with a timestamp prior to this time will not
* be exported.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public CreateExportTaskRequest withFrom(Long from) {
setFrom(from);
return this;
}
/**
*
* A point in time expressed as the number of milliseconds since Jan 1, 1970
* 00:00:00 UTC. It indicates the end time of the range for the request.
* Events with a timestamp later than this time will not be exported.
*
*
* @param to
* A point in time expressed as the number of milliseconds since Jan
* 1, 1970 00:00:00 UTC. It indicates the end time of the range for
* the request. Events with a timestamp later than this time will not
* be exported.
*/
public void setTo(Long to) {
this.to = to;
}
/**
*
* A point in time expressed as the number of milliseconds since Jan 1, 1970
* 00:00:00 UTC. It indicates the end time of the range for the request.
* Events with a timestamp later than this time will not be exported.
*
*
* @return A point in time expressed as the number of milliseconds since Jan
* 1, 1970 00:00:00 UTC. It indicates the end time of the range for
* the request. Events with a timestamp later than this time will
* not be exported.
*/
public Long getTo() {
return this.to;
}
/**
*
* A point in time expressed as the number of milliseconds since Jan 1, 1970
* 00:00:00 UTC. It indicates the end time of the range for the request.
* Events with a timestamp later than this time will not be exported.
*
*
* @param to
* A point in time expressed as the number of milliseconds since Jan
* 1, 1970 00:00:00 UTC. It indicates the end time of the range for
* the request. Events with a timestamp later than this time will not
* be exported.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public CreateExportTaskRequest withTo(Long to) {
setTo(to);
return this;
}
/**
*
* Name of Amazon S3 bucket to which the log data will be exported.
*
*
* Note: Only buckets in the same AWS region are supported.
*
*
* @param destination
* Name of Amazon S3 bucket to which the log data will be
* exported.
*
* Note: Only buckets in the same AWS region are supported.
*/
public void setDestination(String destination) {
this.destination = destination;
}
/**
*
* Name of Amazon S3 bucket to which the log data will be exported.
*
*
* Note: Only buckets in the same AWS region are supported.
*
*
* @return Name of Amazon S3 bucket to which the log data will be
* exported.
*
* Note: Only buckets in the same AWS region are supported.
*/
public String getDestination() {
return this.destination;
}
/**
*
* Name of Amazon S3 bucket to which the log data will be exported.
*
*
* Note: Only buckets in the same AWS region are supported.
*
*
* @param destination
* Name of Amazon S3 bucket to which the log data will be
* exported.
*
* Note: Only buckets in the same AWS region are supported.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public CreateExportTaskRequest withDestination(String destination) {
setDestination(destination);
return this;
}
/**
*
* Prefix that will be used as the start of Amazon S3 key for every object
* exported. If not specified, this defaults to 'exportedlogs'.
*
*
* @param destinationPrefix
* Prefix that will be used as the start of Amazon S3 key for every
* object exported. If not specified, this defaults to
* 'exportedlogs'.
*/
public void setDestinationPrefix(String destinationPrefix) {
this.destinationPrefix = destinationPrefix;
}
/**
*
* Prefix that will be used as the start of Amazon S3 key for every object
* exported. If not specified, this defaults to 'exportedlogs'.
*
*
* @return Prefix that will be used as the start of Amazon S3 key for every
* object exported. If not specified, this defaults to
* 'exportedlogs'.
*/
public String getDestinationPrefix() {
return this.destinationPrefix;
}
/**
*
* Prefix that will be used as the start of Amazon S3 key for every object
* exported. If not specified, this defaults to 'exportedlogs'.
*
*
* @param destinationPrefix
* Prefix that will be used as the start of Amazon S3 key for every
* object exported. If not specified, this defaults to
* 'exportedlogs'.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public CreateExportTaskRequest withDestinationPrefix(
String destinationPrefix) {
setDestinationPrefix(destinationPrefix);
return this;
}
/**
* Returns a string representation of this object; useful for testing and
* debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getTaskName() != null)
sb.append("TaskName: " + getTaskName() + ",");
if (getLogGroupName() != null)
sb.append("LogGroupName: " + getLogGroupName() + ",");
if (getLogStreamNamePrefix() != null)
sb.append("LogStreamNamePrefix: " + getLogStreamNamePrefix() + ",");
if (getFrom() != null)
sb.append("From: " + getFrom() + ",");
if (getTo() != null)
sb.append("To: " + getTo() + ",");
if (getDestination() != null)
sb.append("Destination: " + getDestination() + ",");
if (getDestinationPrefix() != null)
sb.append("DestinationPrefix: " + getDestinationPrefix());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateExportTaskRequest == false)
return false;
CreateExportTaskRequest other = (CreateExportTaskRequest) obj;
if (other.getTaskName() == null ^ this.getTaskName() == null)
return false;
if (other.getTaskName() != null
&& other.getTaskName().equals(this.getTaskName()) == false)
return false;
if (other.getLogGroupName() == null ^ this.getLogGroupName() == null)
return false;
if (other.getLogGroupName() != null
&& other.getLogGroupName().equals(this.getLogGroupName()) == false)
return false;
if (other.getLogStreamNamePrefix() == null
^ this.getLogStreamNamePrefix() == null)
return false;
if (other.getLogStreamNamePrefix() != null
&& other.getLogStreamNamePrefix().equals(
this.getLogStreamNamePrefix()) == false)
return false;
if (other.getFrom() == null ^ this.getFrom() == null)
return false;
if (other.getFrom() != null
&& other.getFrom().equals(this.getFrom()) == false)
return false;
if (other.getTo() == null ^ this.getTo() == null)
return false;
if (other.getTo() != null
&& other.getTo().equals(this.getTo()) == false)
return false;
if (other.getDestination() == null ^ this.getDestination() == null)
return false;
if (other.getDestination() != null
&& other.getDestination().equals(this.getDestination()) == false)
return false;
if (other.getDestinationPrefix() == null
^ this.getDestinationPrefix() == null)
return false;
if (other.getDestinationPrefix() != null
&& other.getDestinationPrefix().equals(
this.getDestinationPrefix()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode
+ ((getTaskName() == null) ? 0 : getTaskName().hashCode());
hashCode = prime
* hashCode
+ ((getLogGroupName() == null) ? 0 : getLogGroupName()
.hashCode());
hashCode = prime
* hashCode
+ ((getLogStreamNamePrefix() == null) ? 0
: getLogStreamNamePrefix().hashCode());
hashCode = prime * hashCode
+ ((getFrom() == null) ? 0 : getFrom().hashCode());
hashCode = prime * hashCode
+ ((getTo() == null) ? 0 : getTo().hashCode());
hashCode = prime
* hashCode
+ ((getDestination() == null) ? 0 : getDestination().hashCode());
hashCode = prime
* hashCode
+ ((getDestinationPrefix() == null) ? 0
: getDestinationPrefix().hashCode());
return hashCode;
}
@Override
public CreateExportTaskRequest clone() {
return (CreateExportTaskRequest) super.clone();
}
}