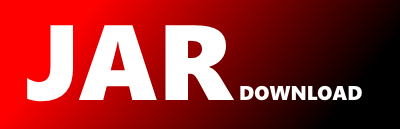
com.amazonaws.services.lookoutequipment.AmazonLookoutEquipment Maven / Gradle / Ivy
Show all versions of aws-java-sdk-lookoutequipment Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.lookoutequipment;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.lookoutequipment.model.*;
/**
* Interface for accessing LookoutEquipment.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.lookoutequipment.AbstractAmazonLookoutEquipment} instead.
*
*
*
* Amazon Lookout for Equipment is a machine learning service that uses advanced analytics to identify anomalies in
* machines from sensor data for use in predictive maintenance.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonLookoutEquipment {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "lookoutequipment";
/**
*
* Creates a container for a collection of data being ingested for analysis. The dataset contains the metadata
* describing where the data is and what the data actually looks like. For example, it contains the location of the
* data source, the data schema, and other information. A dataset also contains any tags associated with the
* ingested data.
*
*
* @param createDatasetRequest
* @return Result of the CreateDataset operation returned by the service.
* @throws ValidationException
* The input fails to satisfy constraints specified by Amazon Lookout for Equipment or a related Amazon Web
* Services service that's being utilized.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ServiceQuotaExceededException
* Resource limitations have been exceeded.
* @throws AccessDeniedException
* The request could not be completed because you do not have access to the resource.
* @throws InternalServerException
* Processing of the request has failed because of an unknown error, exception or failure.
* @sample AmazonLookoutEquipment.CreateDataset
* @see AWS
* API Documentation
*/
CreateDatasetResult createDataset(CreateDatasetRequest createDatasetRequest);
/**
*
* Creates a scheduled inference. Scheduling an inference is setting up a continuous real-time inference plan to
* analyze new measurement data. When setting up the schedule, you provide an S3 bucket location for the input data,
* assign it a delimiter between separate entries in the data, set an offset delay if desired, and set the frequency
* of inferencing. You must also provide an S3 bucket location for the output data.
*
*
* @param createInferenceSchedulerRequest
* @return Result of the CreateInferenceScheduler operation returned by the service.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @throws ResourceNotFoundException
* The resource requested could not be found. Verify the resource ID and retry your request.
* @throws ValidationException
* The input fails to satisfy constraints specified by Amazon Lookout for Equipment or a related Amazon Web
* Services service that's being utilized.
* @throws ServiceQuotaExceededException
* Resource limitations have been exceeded.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* The request could not be completed because you do not have access to the resource.
* @throws InternalServerException
* Processing of the request has failed because of an unknown error, exception or failure.
* @sample AmazonLookoutEquipment.CreateInferenceScheduler
* @see AWS API Documentation
*/
CreateInferenceSchedulerResult createInferenceScheduler(CreateInferenceSchedulerRequest createInferenceSchedulerRequest);
/**
*
* Creates a label for an event.
*
*
* @param createLabelRequest
* @return Result of the CreateLabel operation returned by the service.
* @throws ValidationException
* The input fails to satisfy constraints specified by Amazon Lookout for Equipment or a related Amazon Web
* Services service that's being utilized.
* @throws ResourceNotFoundException
* The resource requested could not be found. Verify the resource ID and retry your request.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ServiceQuotaExceededException
* Resource limitations have been exceeded.
* @throws AccessDeniedException
* The request could not be completed because you do not have access to the resource.
* @throws InternalServerException
* Processing of the request has failed because of an unknown error, exception or failure.
* @sample AmazonLookoutEquipment.CreateLabel
* @see AWS
* API Documentation
*/
CreateLabelResult createLabel(CreateLabelRequest createLabelRequest);
/**
*
* Creates a group of labels.
*
*
* @param createLabelGroupRequest
* @return Result of the CreateLabelGroup operation returned by the service.
* @throws ValidationException
* The input fails to satisfy constraints specified by Amazon Lookout for Equipment or a related Amazon Web
* Services service that's being utilized.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ServiceQuotaExceededException
* Resource limitations have been exceeded.
* @throws AccessDeniedException
* The request could not be completed because you do not have access to the resource.
* @throws InternalServerException
* Processing of the request has failed because of an unknown error, exception or failure.
* @sample AmazonLookoutEquipment.CreateLabelGroup
* @see AWS API Documentation
*/
CreateLabelGroupResult createLabelGroup(CreateLabelGroupRequest createLabelGroupRequest);
/**
*
* Creates a machine learning model for data inference.
*
*
* A machine-learning (ML) model is a mathematical model that finds patterns in your data. In Amazon Lookout for
* Equipment, the model learns the patterns of normal behavior and detects abnormal behavior that could be potential
* equipment failure (or maintenance events). The models are made by analyzing normal data and abnormalities in
* machine behavior that have already occurred.
*
*
* Your model is trained using a portion of the data from your dataset and uses that data to learn patterns of
* normal behavior and abnormal patterns that lead to equipment failure. Another portion of the data is used to
* evaluate the model's accuracy.
*
*
* @param createModelRequest
* @return Result of the CreateModel operation returned by the service.
* @throws ValidationException
* The input fails to satisfy constraints specified by Amazon Lookout for Equipment or a related Amazon Web
* Services service that's being utilized.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ServiceQuotaExceededException
* Resource limitations have been exceeded.
* @throws InternalServerException
* Processing of the request has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The resource requested could not be found. Verify the resource ID and retry your request.
* @throws AccessDeniedException
* The request could not be completed because you do not have access to the resource.
* @sample AmazonLookoutEquipment.CreateModel
* @see AWS
* API Documentation
*/
CreateModelResult createModel(CreateModelRequest createModelRequest);
/**
*
* Creates a retraining scheduler on the specified model.
*
*
* @param createRetrainingSchedulerRequest
* @return Result of the CreateRetrainingScheduler operation returned by the service.
* @throws ValidationException
* The input fails to satisfy constraints specified by Amazon Lookout for Equipment or a related Amazon Web
* Services service that's being utilized.
* @throws ResourceNotFoundException
* The resource requested could not be found. Verify the resource ID and retry your request.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* The request could not be completed because you do not have access to the resource.
* @throws InternalServerException
* Processing of the request has failed because of an unknown error, exception or failure.
* @sample AmazonLookoutEquipment.CreateRetrainingScheduler
* @see AWS API Documentation
*/
CreateRetrainingSchedulerResult createRetrainingScheduler(CreateRetrainingSchedulerRequest createRetrainingSchedulerRequest);
/**
*
* Deletes a dataset and associated artifacts. The operation will check to see if any inference scheduler or data
* ingestion job is currently using the dataset, and if there isn't, the dataset, its metadata, and any associated
* data stored in S3 will be deleted. This does not affect any models that used this dataset for training and
* evaluation, but does prevent it from being used in the future.
*
*
* @param deleteDatasetRequest
* @return Result of the DeleteDataset operation returned by the service.
* @throws ResourceNotFoundException
* The resource requested could not be found. Verify the resource ID and retry your request.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* Processing of the request has failed because of an unknown error, exception or failure.
* @throws AccessDeniedException
* The request could not be completed because you do not have access to the resource.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @throws ValidationException
* The input fails to satisfy constraints specified by Amazon Lookout for Equipment or a related Amazon Web
* Services service that's being utilized.
* @sample AmazonLookoutEquipment.DeleteDataset
* @see AWS
* API Documentation
*/
DeleteDatasetResult deleteDataset(DeleteDatasetRequest deleteDatasetRequest);
/**
*
* Deletes an inference scheduler that has been set up. Prior inference results will not be deleted.
*
*
* @param deleteInferenceSchedulerRequest
* @return Result of the DeleteInferenceScheduler operation returned by the service.
* @throws ValidationException
* The input fails to satisfy constraints specified by Amazon Lookout for Equipment or a related Amazon Web
* Services service that's being utilized.
* @throws ResourceNotFoundException
* The resource requested could not be found. Verify the resource ID and retry your request.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* The request could not be completed because you do not have access to the resource.
* @throws InternalServerException
* Processing of the request has failed because of an unknown error, exception or failure.
* @sample AmazonLookoutEquipment.DeleteInferenceScheduler
* @see AWS API Documentation
*/
DeleteInferenceSchedulerResult deleteInferenceScheduler(DeleteInferenceSchedulerRequest deleteInferenceSchedulerRequest);
/**
*
* Deletes a label.
*
*
* @param deleteLabelRequest
* @return Result of the DeleteLabel operation returned by the service.
* @throws ResourceNotFoundException
* The resource requested could not be found. Verify the resource ID and retry your request.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* Processing of the request has failed because of an unknown error, exception or failure.
* @throws AccessDeniedException
* The request could not be completed because you do not have access to the resource.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @throws ValidationException
* The input fails to satisfy constraints specified by Amazon Lookout for Equipment or a related Amazon Web
* Services service that's being utilized.
* @sample AmazonLookoutEquipment.DeleteLabel
* @see AWS
* API Documentation
*/
DeleteLabelResult deleteLabel(DeleteLabelRequest deleteLabelRequest);
/**
*
* Deletes a group of labels.
*
*
* @param deleteLabelGroupRequest
* @return Result of the DeleteLabelGroup operation returned by the service.
* @throws ResourceNotFoundException
* The resource requested could not be found. Verify the resource ID and retry your request.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* Processing of the request has failed because of an unknown error, exception or failure.
* @throws AccessDeniedException
* The request could not be completed because you do not have access to the resource.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @throws ValidationException
* The input fails to satisfy constraints specified by Amazon Lookout for Equipment or a related Amazon Web
* Services service that's being utilized.
* @sample AmazonLookoutEquipment.DeleteLabelGroup
* @see AWS API Documentation
*/
DeleteLabelGroupResult deleteLabelGroup(DeleteLabelGroupRequest deleteLabelGroupRequest);
/**
*
* Deletes a machine learning model currently available for Amazon Lookout for Equipment. This will prevent it from
* being used with an inference scheduler, even one that is already set up.
*
*
* @param deleteModelRequest
* @return Result of the DeleteModel operation returned by the service.
* @throws ResourceNotFoundException
* The resource requested could not be found. Verify the resource ID and retry your request.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* Processing of the request has failed because of an unknown error, exception or failure.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @throws AccessDeniedException
* The request could not be completed because you do not have access to the resource.
* @throws ValidationException
* The input fails to satisfy constraints specified by Amazon Lookout for Equipment or a related Amazon Web
* Services service that's being utilized.
* @sample AmazonLookoutEquipment.DeleteModel
* @see AWS
* API Documentation
*/
DeleteModelResult deleteModel(DeleteModelRequest deleteModelRequest);
/**
*
* Deletes the resource policy attached to the resource.
*
*
* @param deleteResourcePolicyRequest
* @return Result of the DeleteResourcePolicy operation returned by the service.
* @throws ResourceNotFoundException
* The resource requested could not be found. Verify the resource ID and retry your request.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerException
* Processing of the request has failed because of an unknown error, exception or failure.
* @throws AccessDeniedException
* The request could not be completed because you do not have access to the resource.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @throws ValidationException
* The input fails to satisfy constraints specified by Amazon Lookout for Equipment or a related Amazon Web
* Services service that's being utilized.
* @sample AmazonLookoutEquipment.DeleteResourcePolicy
* @see AWS API Documentation
*/
DeleteResourcePolicyResult deleteResourcePolicy(DeleteResourcePolicyRequest deleteResourcePolicyRequest);
/**
*
* Deletes a retraining scheduler from a model. The retraining scheduler must be in the STOPPED
status.
*
*
* @param deleteRetrainingSchedulerRequest
* @return Result of the DeleteRetrainingScheduler operation returned by the service.
* @throws ValidationException
* The input fails to satisfy constraints specified by Amazon Lookout for Equipment or a related Amazon Web
* Services service that's being utilized.
* @throws ResourceNotFoundException
* The resource requested could not be found. Verify the resource ID and retry your request.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* The request could not be completed because you do not have access to the resource.
* @throws InternalServerException
* Processing of the request has failed because of an unknown error, exception or failure.
* @sample AmazonLookoutEquipment.DeleteRetrainingScheduler
* @see AWS API Documentation
*/
DeleteRetrainingSchedulerResult deleteRetrainingScheduler(DeleteRetrainingSchedulerRequest deleteRetrainingSchedulerRequest);
/**
*
* Provides information on a specific data ingestion job such as creation time, dataset ARN, and status.
*
*
* @param describeDataIngestionJobRequest
* @return Result of the DescribeDataIngestionJob operation returned by the service.
* @throws ValidationException
* The input fails to satisfy constraints specified by Amazon Lookout for Equipment or a related Amazon Web
* Services service that's being utilized.
* @throws ResourceNotFoundException
* The resource requested could not be found. Verify the resource ID and retry your request.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* The request could not be completed because you do not have access to the resource.
* @throws InternalServerException
* Processing of the request has failed because of an unknown error, exception or failure.
* @sample AmazonLookoutEquipment.DescribeDataIngestionJob
* @see AWS API Documentation
*/
DescribeDataIngestionJobResult describeDataIngestionJob(DescribeDataIngestionJobRequest describeDataIngestionJobRequest);
/**
*
* Provides a JSON description of the data in each time series dataset, including names, column names, and data
* types.
*
*
* @param describeDatasetRequest
* @return Result of the DescribeDataset operation returned by the service.
* @throws ValidationException
* The input fails to satisfy constraints specified by Amazon Lookout for Equipment or a related Amazon Web
* Services service that's being utilized.
* @throws ResourceNotFoundException
* The resource requested could not be found. Verify the resource ID and retry your request.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* The request could not be completed because you do not have access to the resource.
* @throws InternalServerException
* Processing of the request has failed because of an unknown error, exception or failure.
* @sample AmazonLookoutEquipment.DescribeDataset
* @see AWS API Documentation
*/
DescribeDatasetResult describeDataset(DescribeDatasetRequest describeDatasetRequest);
/**
*
* Specifies information about the inference scheduler being used, including name, model, status, and associated
* metadata
*
*
* @param describeInferenceSchedulerRequest
* @return Result of the DescribeInferenceScheduler operation returned by the service.
* @throws ValidationException
* The input fails to satisfy constraints specified by Amazon Lookout for Equipment or a related Amazon Web
* Services service that's being utilized.
* @throws ResourceNotFoundException
* The resource requested could not be found. Verify the resource ID and retry your request.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* The request could not be completed because you do not have access to the resource.
* @throws InternalServerException
* Processing of the request has failed because of an unknown error, exception or failure.
* @sample AmazonLookoutEquipment.DescribeInferenceScheduler
* @see AWS API Documentation
*/
DescribeInferenceSchedulerResult describeInferenceScheduler(DescribeInferenceSchedulerRequest describeInferenceSchedulerRequest);
/**
*
* Returns the name of the label.
*
*
* @param describeLabelRequest
* @return Result of the DescribeLabel operation returned by the service.
* @throws ValidationException
* The input fails to satisfy constraints specified by Amazon Lookout for Equipment or a related Amazon Web
* Services service that's being utilized.
* @throws ResourceNotFoundException
* The resource requested could not be found. Verify the resource ID and retry your request.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* The request could not be completed because you do not have access to the resource.
* @throws InternalServerException
* Processing of the request has failed because of an unknown error, exception or failure.
* @sample AmazonLookoutEquipment.DescribeLabel
* @see AWS
* API Documentation
*/
DescribeLabelResult describeLabel(DescribeLabelRequest describeLabelRequest);
/**
*
* Returns information about the label group.
*
*
* @param describeLabelGroupRequest
* @return Result of the DescribeLabelGroup operation returned by the service.
* @throws ValidationException
* The input fails to satisfy constraints specified by Amazon Lookout for Equipment or a related Amazon Web
* Services service that's being utilized.
* @throws ResourceNotFoundException
* The resource requested could not be found. Verify the resource ID and retry your request.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* The request could not be completed because you do not have access to the resource.
* @throws InternalServerException
* Processing of the request has failed because of an unknown error, exception or failure.
* @sample AmazonLookoutEquipment.DescribeLabelGroup
* @see AWS API Documentation
*/
DescribeLabelGroupResult describeLabelGroup(DescribeLabelGroupRequest describeLabelGroupRequest);
/**
*
* Provides a JSON containing the overall information about a specific machine learning model, including model name
* and ARN, dataset, training and evaluation information, status, and so on.
*
*
* @param describeModelRequest
* @return Result of the DescribeModel operation returned by the service.
* @throws ValidationException
* The input fails to satisfy constraints specified by Amazon Lookout for Equipment or a related Amazon Web
* Services service that's being utilized.
* @throws ResourceNotFoundException
* The resource requested could not be found. Verify the resource ID and retry your request.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* The request could not be completed because you do not have access to the resource.
* @throws InternalServerException
* Processing of the request has failed because of an unknown error, exception or failure.
* @sample AmazonLookoutEquipment.DescribeModel
* @see AWS
* API Documentation
*/
DescribeModelResult describeModel(DescribeModelRequest describeModelRequest);
/**
*
* Retrieves information about a specific machine learning model version.
*
*
* @param describeModelVersionRequest
* @return Result of the DescribeModelVersion operation returned by the service.
* @throws ValidationException
* The input fails to satisfy constraints specified by Amazon Lookout for Equipment or a related Amazon Web
* Services service that's being utilized.
* @throws ResourceNotFoundException
* The resource requested could not be found. Verify the resource ID and retry your request.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* The request could not be completed because you do not have access to the resource.
* @throws InternalServerException
* Processing of the request has failed because of an unknown error, exception or failure.
* @sample AmazonLookoutEquipment.DescribeModelVersion
* @see AWS API Documentation
*/
DescribeModelVersionResult describeModelVersion(DescribeModelVersionRequest describeModelVersionRequest);
/**
*
* Provides the details of a resource policy attached to a resource.
*
*
* @param describeResourcePolicyRequest
* @return Result of the DescribeResourcePolicy operation returned by the service.
* @throws ValidationException
* The input fails to satisfy constraints specified by Amazon Lookout for Equipment or a related Amazon Web
* Services service that's being utilized.
* @throws ResourceNotFoundException
* The resource requested could not be found. Verify the resource ID and retry your request.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* The request could not be completed because you do not have access to the resource.
* @throws InternalServerException
* Processing of the request has failed because of an unknown error, exception or failure.
* @sample AmazonLookoutEquipment.DescribeResourcePolicy
* @see AWS API Documentation
*/
DescribeResourcePolicyResult describeResourcePolicy(DescribeResourcePolicyRequest describeResourcePolicyRequest);
/**
*
* Provides a description of the retraining scheduler, including information such as the model name and retraining
* parameters.
*
*
* @param describeRetrainingSchedulerRequest
* @return Result of the DescribeRetrainingScheduler operation returned by the service.
* @throws ValidationException
* The input fails to satisfy constraints specified by Amazon Lookout for Equipment or a related Amazon Web
* Services service that's being utilized.
* @throws ResourceNotFoundException
* The resource requested could not be found. Verify the resource ID and retry your request.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* The request could not be completed because you do not have access to the resource.
* @throws InternalServerException
* Processing of the request has failed because of an unknown error, exception or failure.
* @sample AmazonLookoutEquipment.DescribeRetrainingScheduler
* @see AWS API Documentation
*/
DescribeRetrainingSchedulerResult describeRetrainingScheduler(DescribeRetrainingSchedulerRequest describeRetrainingSchedulerRequest);
/**
*
* Imports a dataset.
*
*
* @param importDatasetRequest
* @return Result of the ImportDataset operation returned by the service.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @throws ResourceNotFoundException
* The resource requested could not be found. Verify the resource ID and retry your request.
* @throws ValidationException
* The input fails to satisfy constraints specified by Amazon Lookout for Equipment or a related Amazon Web
* Services service that's being utilized.
* @throws ServiceQuotaExceededException
* Resource limitations have been exceeded.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* The request could not be completed because you do not have access to the resource.
* @throws InternalServerException
* Processing of the request has failed because of an unknown error, exception or failure.
* @sample AmazonLookoutEquipment.ImportDataset
* @see AWS
* API Documentation
*/
ImportDatasetResult importDataset(ImportDatasetRequest importDatasetRequest);
/**
*
* Imports a model that has been trained successfully.
*
*
* @param importModelVersionRequest
* @return Result of the ImportModelVersion operation returned by the service.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @throws ResourceNotFoundException
* The resource requested could not be found. Verify the resource ID and retry your request.
* @throws ValidationException
* The input fails to satisfy constraints specified by Amazon Lookout for Equipment or a related Amazon Web
* Services service that's being utilized.
* @throws ServiceQuotaExceededException
* Resource limitations have been exceeded.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* The request could not be completed because you do not have access to the resource.
* @throws InternalServerException
* Processing of the request has failed because of an unknown error, exception or failure.
* @sample AmazonLookoutEquipment.ImportModelVersion
* @see AWS API Documentation
*/
ImportModelVersionResult importModelVersion(ImportModelVersionRequest importModelVersionRequest);
/**
*
* Provides a list of all data ingestion jobs, including dataset name and ARN, S3 location of the input data,
* status, and so on.
*
*
* @param listDataIngestionJobsRequest
* @return Result of the ListDataIngestionJobs operation returned by the service.
* @throws ValidationException
* The input fails to satisfy constraints specified by Amazon Lookout for Equipment or a related Amazon Web
* Services service that's being utilized.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* The request could not be completed because you do not have access to the resource.
* @throws InternalServerException
* Processing of the request has failed because of an unknown error, exception or failure.
* @sample AmazonLookoutEquipment.ListDataIngestionJobs
* @see AWS API Documentation
*/
ListDataIngestionJobsResult listDataIngestionJobs(ListDataIngestionJobsRequest listDataIngestionJobsRequest);
/**
*
* Lists all datasets currently available in your account, filtering on the dataset name.
*
*
* @param listDatasetsRequest
* @return Result of the ListDatasets operation returned by the service.
* @throws ValidationException
* The input fails to satisfy constraints specified by Amazon Lookout for Equipment or a related Amazon Web
* Services service that's being utilized.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* The request could not be completed because you do not have access to the resource.
* @throws InternalServerException
* Processing of the request has failed because of an unknown error, exception or failure.
* @sample AmazonLookoutEquipment.ListDatasets
* @see AWS
* API Documentation
*/
ListDatasetsResult listDatasets(ListDatasetsRequest listDatasetsRequest);
/**
*
* Lists all inference events that have been found for the specified inference scheduler.
*
*
* @param listInferenceEventsRequest
* @return Result of the ListInferenceEvents operation returned by the service.
* @throws ValidationException
* The input fails to satisfy constraints specified by Amazon Lookout for Equipment or a related Amazon Web
* Services service that's being utilized.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ResourceNotFoundException
* The resource requested could not be found. Verify the resource ID and retry your request.
* @throws AccessDeniedException
* The request could not be completed because you do not have access to the resource.
* @throws InternalServerException
* Processing of the request has failed because of an unknown error, exception or failure.
* @sample AmazonLookoutEquipment.ListInferenceEvents
* @see AWS API Documentation
*/
ListInferenceEventsResult listInferenceEvents(ListInferenceEventsRequest listInferenceEventsRequest);
/**
*
* Lists all inference executions that have been performed by the specified inference scheduler.
*
*
* @param listInferenceExecutionsRequest
* @return Result of the ListInferenceExecutions operation returned by the service.
* @throws ValidationException
* The input fails to satisfy constraints specified by Amazon Lookout for Equipment or a related Amazon Web
* Services service that's being utilized.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ResourceNotFoundException
* The resource requested could not be found. Verify the resource ID and retry your request.
* @throws AccessDeniedException
* The request could not be completed because you do not have access to the resource.
* @throws InternalServerException
* Processing of the request has failed because of an unknown error, exception or failure.
* @sample AmazonLookoutEquipment.ListInferenceExecutions
* @see AWS API Documentation
*/
ListInferenceExecutionsResult listInferenceExecutions(ListInferenceExecutionsRequest listInferenceExecutionsRequest);
/**
*
* Retrieves a list of all inference schedulers currently available for your account.
*
*
* @param listInferenceSchedulersRequest
* @return Result of the ListInferenceSchedulers operation returned by the service.
* @throws ValidationException
* The input fails to satisfy constraints specified by Amazon Lookout for Equipment or a related Amazon Web
* Services service that's being utilized.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* The request could not be completed because you do not have access to the resource.
* @throws InternalServerException
* Processing of the request has failed because of an unknown error, exception or failure.
* @sample AmazonLookoutEquipment.ListInferenceSchedulers
* @see AWS API Documentation
*/
ListInferenceSchedulersResult listInferenceSchedulers(ListInferenceSchedulersRequest listInferenceSchedulersRequest);
/**
*
* Returns a list of the label groups.
*
*
* @param listLabelGroupsRequest
* @return Result of the ListLabelGroups operation returned by the service.
* @throws ValidationException
* The input fails to satisfy constraints specified by Amazon Lookout for Equipment or a related Amazon Web
* Services service that's being utilized.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* The request could not be completed because you do not have access to the resource.
* @throws InternalServerException
* Processing of the request has failed because of an unknown error, exception or failure.
* @sample AmazonLookoutEquipment.ListLabelGroups
* @see AWS API Documentation
*/
ListLabelGroupsResult listLabelGroups(ListLabelGroupsRequest listLabelGroupsRequest);
/**
*
* Provides a list of labels.
*
*
* @param listLabelsRequest
* @return Result of the ListLabels operation returned by the service.
* @throws ValidationException
* The input fails to satisfy constraints specified by Amazon Lookout for Equipment or a related Amazon Web
* Services service that's being utilized.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* The request could not be completed because you do not have access to the resource.
* @throws InternalServerException
* Processing of the request has failed because of an unknown error, exception or failure.
* @sample AmazonLookoutEquipment.ListLabels
* @see AWS
* API Documentation
*/
ListLabelsResult listLabels(ListLabelsRequest listLabelsRequest);
/**
*
* Generates a list of all model versions for a given model, including the model version, model version ARN, and
* status. To list a subset of versions, use the MaxModelVersion
and MinModelVersion
* fields.
*
*
* @param listModelVersionsRequest
* @return Result of the ListModelVersions operation returned by the service.
* @throws ValidationException
* The input fails to satisfy constraints specified by Amazon Lookout for Equipment or a related Amazon Web
* Services service that's being utilized.
* @throws ResourceNotFoundException
* The resource requested could not be found. Verify the resource ID and retry your request.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* The request could not be completed because you do not have access to the resource.
* @throws InternalServerException
* Processing of the request has failed because of an unknown error, exception or failure.
* @sample AmazonLookoutEquipment.ListModelVersions
* @see AWS API Documentation
*/
ListModelVersionsResult listModelVersions(ListModelVersionsRequest listModelVersionsRequest);
/**
*
* Generates a list of all models in the account, including model name and ARN, dataset, and status.
*
*
* @param listModelsRequest
* @return Result of the ListModels operation returned by the service.
* @throws ValidationException
* The input fails to satisfy constraints specified by Amazon Lookout for Equipment or a related Amazon Web
* Services service that's being utilized.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* The request could not be completed because you do not have access to the resource.
* @throws InternalServerException
* Processing of the request has failed because of an unknown error, exception or failure.
* @sample AmazonLookoutEquipment.ListModels
* @see AWS
* API Documentation
*/
ListModelsResult listModels(ListModelsRequest listModelsRequest);
/**
*
* Lists all retraining schedulers in your account, filtering by model name prefix and status.
*
*
* @param listRetrainingSchedulersRequest
* @return Result of the ListRetrainingSchedulers operation returned by the service.
* @throws ValidationException
* The input fails to satisfy constraints specified by Amazon Lookout for Equipment or a related Amazon Web
* Services service that's being utilized.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* The request could not be completed because you do not have access to the resource.
* @throws InternalServerException
* Processing of the request has failed because of an unknown error, exception or failure.
* @sample AmazonLookoutEquipment.ListRetrainingSchedulers
* @see AWS API Documentation
*/
ListRetrainingSchedulersResult listRetrainingSchedulers(ListRetrainingSchedulersRequest listRetrainingSchedulersRequest);
/**
*
* Lists statistics about the data collected for each of the sensors that have been successfully ingested in the
* particular dataset. Can also be used to retreive Sensor Statistics for a previous ingestion job.
*
*
* @param listSensorStatisticsRequest
* @return Result of the ListSensorStatistics operation returned by the service.
* @throws ValidationException
* The input fails to satisfy constraints specified by Amazon Lookout for Equipment or a related Amazon Web
* Services service that's being utilized.
* @throws ResourceNotFoundException
* The resource requested could not be found. Verify the resource ID and retry your request.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* The request could not be completed because you do not have access to the resource.
* @throws InternalServerException
* Processing of the request has failed because of an unknown error, exception or failure.
* @sample AmazonLookoutEquipment.ListSensorStatistics
* @see AWS API Documentation
*/
ListSensorStatisticsResult listSensorStatistics(ListSensorStatisticsRequest listSensorStatisticsRequest);
/**
*
* Lists all the tags for a specified resource, including key and value.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws ValidationException
* The input fails to satisfy constraints specified by Amazon Lookout for Equipment or a related Amazon Web
* Services service that's being utilized.
* @throws ResourceNotFoundException
* The resource requested could not be found. Verify the resource ID and retry your request.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* The request could not be completed because you do not have access to the resource.
* @throws InternalServerException
* Processing of the request has failed because of an unknown error, exception or failure.
* @sample AmazonLookoutEquipment.ListTagsForResource
* @see AWS API Documentation
*/
ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Creates a resource control policy for a given resource.
*
*
* @param putResourcePolicyRequest
* @return Result of the PutResourcePolicy operation returned by the service.
* @throws ValidationException
* The input fails to satisfy constraints specified by Amazon Lookout for Equipment or a related Amazon Web
* Services service that's being utilized.
* @throws ResourceNotFoundException
* The resource requested could not be found. Verify the resource ID and retry your request.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ServiceQuotaExceededException
* Resource limitations have been exceeded.
* @throws AccessDeniedException
* The request could not be completed because you do not have access to the resource.
* @throws InternalServerException
* Processing of the request has failed because of an unknown error, exception or failure.
* @sample AmazonLookoutEquipment.PutResourcePolicy
* @see AWS API Documentation
*/
PutResourcePolicyResult putResourcePolicy(PutResourcePolicyRequest putResourcePolicyRequest);
/**
*
* Starts a data ingestion job. Amazon Lookout for Equipment returns the job status.
*
*
* @param startDataIngestionJobRequest
* @return Result of the StartDataIngestionJob operation returned by the service.
* @throws ValidationException
* The input fails to satisfy constraints specified by Amazon Lookout for Equipment or a related Amazon Web
* Services service that's being utilized.
* @throws ResourceNotFoundException
* The resource requested could not be found. Verify the resource ID and retry your request.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws ServiceQuotaExceededException
* Resource limitations have been exceeded.
* @throws AccessDeniedException
* The request could not be completed because you do not have access to the resource.
* @throws InternalServerException
* Processing of the request has failed because of an unknown error, exception or failure.
* @sample AmazonLookoutEquipment.StartDataIngestionJob
* @see AWS API Documentation
*/
StartDataIngestionJobResult startDataIngestionJob(StartDataIngestionJobRequest startDataIngestionJobRequest);
/**
*
* Starts an inference scheduler.
*
*
* @param startInferenceSchedulerRequest
* @return Result of the StartInferenceScheduler operation returned by the service.
* @throws ValidationException
* The input fails to satisfy constraints specified by Amazon Lookout for Equipment or a related Amazon Web
* Services service that's being utilized.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @throws ResourceNotFoundException
* The resource requested could not be found. Verify the resource ID and retry your request.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* The request could not be completed because you do not have access to the resource.
* @throws InternalServerException
* Processing of the request has failed because of an unknown error, exception or failure.
* @sample AmazonLookoutEquipment.StartInferenceScheduler
* @see AWS API Documentation
*/
StartInferenceSchedulerResult startInferenceScheduler(StartInferenceSchedulerRequest startInferenceSchedulerRequest);
/**
*
* Starts a retraining scheduler.
*
*
* @param startRetrainingSchedulerRequest
* @return Result of the StartRetrainingScheduler operation returned by the service.
* @throws ValidationException
* The input fails to satisfy constraints specified by Amazon Lookout for Equipment or a related Amazon Web
* Services service that's being utilized.
* @throws ResourceNotFoundException
* The resource requested could not be found. Verify the resource ID and retry your request.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* The request could not be completed because you do not have access to the resource.
* @throws InternalServerException
* Processing of the request has failed because of an unknown error, exception or failure.
* @sample AmazonLookoutEquipment.StartRetrainingScheduler
* @see AWS API Documentation
*/
StartRetrainingSchedulerResult startRetrainingScheduler(StartRetrainingSchedulerRequest startRetrainingSchedulerRequest);
/**
*
* Stops an inference scheduler.
*
*
* @param stopInferenceSchedulerRequest
* @return Result of the StopInferenceScheduler operation returned by the service.
* @throws ValidationException
* The input fails to satisfy constraints specified by Amazon Lookout for Equipment or a related Amazon Web
* Services service that's being utilized.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @throws ResourceNotFoundException
* The resource requested could not be found. Verify the resource ID and retry your request.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* The request could not be completed because you do not have access to the resource.
* @throws InternalServerException
* Processing of the request has failed because of an unknown error, exception or failure.
* @sample AmazonLookoutEquipment.StopInferenceScheduler
* @see AWS API Documentation
*/
StopInferenceSchedulerResult stopInferenceScheduler(StopInferenceSchedulerRequest stopInferenceSchedulerRequest);
/**
*
* Stops a retraining scheduler.
*
*
* @param stopRetrainingSchedulerRequest
* @return Result of the StopRetrainingScheduler operation returned by the service.
* @throws ValidationException
* The input fails to satisfy constraints specified by Amazon Lookout for Equipment or a related Amazon Web
* Services service that's being utilized.
* @throws ResourceNotFoundException
* The resource requested could not be found. Verify the resource ID and retry your request.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* The request could not be completed because you do not have access to the resource.
* @throws InternalServerException
* Processing of the request has failed because of an unknown error, exception or failure.
* @sample AmazonLookoutEquipment.StopRetrainingScheduler
* @see AWS API Documentation
*/
StopRetrainingSchedulerResult stopRetrainingScheduler(StopRetrainingSchedulerRequest stopRetrainingSchedulerRequest);
/**
*
* Associates a given tag to a resource in your account. A tag is a key-value pair which can be added to an Amazon
* Lookout for Equipment resource as metadata. Tags can be used for organizing your resources as well as helping you
* to search and filter by tag. Multiple tags can be added to a resource, either when you create it, or later. Up to
* 50 tags can be associated with each resource.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws ValidationException
* The input fails to satisfy constraints specified by Amazon Lookout for Equipment or a related Amazon Web
* Services service that's being utilized.
* @throws ResourceNotFoundException
* The resource requested could not be found. Verify the resource ID and retry your request.
* @throws ServiceQuotaExceededException
* Resource limitations have been exceeded.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* The request could not be completed because you do not have access to the resource.
* @throws InternalServerException
* Processing of the request has failed because of an unknown error, exception or failure.
* @sample AmazonLookoutEquipment.TagResource
* @see AWS
* API Documentation
*/
TagResourceResult tagResource(TagResourceRequest tagResourceRequest);
/**
*
* Removes a specific tag from a given resource. The tag is specified by its key.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws ValidationException
* The input fails to satisfy constraints specified by Amazon Lookout for Equipment or a related Amazon Web
* Services service that's being utilized.
* @throws ResourceNotFoundException
* The resource requested could not be found. Verify the resource ID and retry your request.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* The request could not be completed because you do not have access to the resource.
* @throws InternalServerException
* Processing of the request has failed because of an unknown error, exception or failure.
* @sample AmazonLookoutEquipment.UntagResource
* @see AWS
* API Documentation
*/
UntagResourceResult untagResource(UntagResourceRequest untagResourceRequest);
/**
*
* Sets the active model version for a given machine learning model.
*
*
* @param updateActiveModelVersionRequest
* @return Result of the UpdateActiveModelVersion operation returned by the service.
* @throws ValidationException
* The input fails to satisfy constraints specified by Amazon Lookout for Equipment or a related Amazon Web
* Services service that's being utilized.
* @throws ResourceNotFoundException
* The resource requested could not be found. Verify the resource ID and retry your request.
* @throws InternalServerException
* Processing of the request has failed because of an unknown error, exception or failure.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* The request could not be completed because you do not have access to the resource.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @sample AmazonLookoutEquipment.UpdateActiveModelVersion
* @see AWS API Documentation
*/
UpdateActiveModelVersionResult updateActiveModelVersion(UpdateActiveModelVersionRequest updateActiveModelVersionRequest);
/**
*
* Updates an inference scheduler.
*
*
* @param updateInferenceSchedulerRequest
* @return Result of the UpdateInferenceScheduler operation returned by the service.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @throws ResourceNotFoundException
* The resource requested could not be found. Verify the resource ID and retry your request.
* @throws ValidationException
* The input fails to satisfy constraints specified by Amazon Lookout for Equipment or a related Amazon Web
* Services service that's being utilized.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* The request could not be completed because you do not have access to the resource.
* @throws InternalServerException
* Processing of the request has failed because of an unknown error, exception or failure.
* @sample AmazonLookoutEquipment.UpdateInferenceScheduler
* @see AWS API Documentation
*/
UpdateInferenceSchedulerResult updateInferenceScheduler(UpdateInferenceSchedulerRequest updateInferenceSchedulerRequest);
/**
*
* Updates the label group.
*
*
* @param updateLabelGroupRequest
* @return Result of the UpdateLabelGroup operation returned by the service.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @throws ResourceNotFoundException
* The resource requested could not be found. Verify the resource ID and retry your request.
* @throws ValidationException
* The input fails to satisfy constraints specified by Amazon Lookout for Equipment or a related Amazon Web
* Services service that's being utilized.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* The request could not be completed because you do not have access to the resource.
* @throws InternalServerException
* Processing of the request has failed because of an unknown error, exception or failure.
* @sample AmazonLookoutEquipment.UpdateLabelGroup
* @see AWS API Documentation
*/
UpdateLabelGroupResult updateLabelGroup(UpdateLabelGroupRequest updateLabelGroupRequest);
/**
*
* Updates a model in the account.
*
*
* @param updateModelRequest
* @return Result of the UpdateModel operation returned by the service.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @throws ResourceNotFoundException
* The resource requested could not be found. Verify the resource ID and retry your request.
* @throws ValidationException
* The input fails to satisfy constraints specified by Amazon Lookout for Equipment or a related Amazon Web
* Services service that's being utilized.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* The request could not be completed because you do not have access to the resource.
* @throws InternalServerException
* Processing of the request has failed because of an unknown error, exception or failure.
* @sample AmazonLookoutEquipment.UpdateModel
* @see AWS
* API Documentation
*/
UpdateModelResult updateModel(UpdateModelRequest updateModelRequest);
/**
*
* Updates a retraining scheduler.
*
*
* @param updateRetrainingSchedulerRequest
* @return Result of the UpdateRetrainingScheduler operation returned by the service.
* @throws ValidationException
* The input fails to satisfy constraints specified by Amazon Lookout for Equipment or a related Amazon Web
* Services service that's being utilized.
* @throws ResourceNotFoundException
* The resource requested could not be found. Verify the resource ID and retry your request.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws AccessDeniedException
* The request could not be completed because you do not have access to the resource.
* @throws InternalServerException
* Processing of the request has failed because of an unknown error, exception or failure.
* @sample AmazonLookoutEquipment.UpdateRetrainingScheduler
* @see AWS API Documentation
*/
UpdateRetrainingSchedulerResult updateRetrainingScheduler(UpdateRetrainingSchedulerRequest updateRetrainingSchedulerRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}