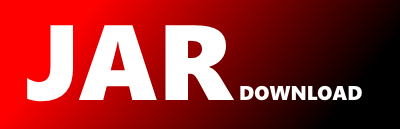
com.amazonaws.services.lookoutequipment.AmazonLookoutEquipmentAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-lookoutequipment Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.lookoutequipment;
import javax.annotation.Generated;
import com.amazonaws.services.lookoutequipment.model.*;
/**
* Interface for accessing LookoutEquipment asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.lookoutequipment.AbstractAmazonLookoutEquipmentAsync} instead.
*
*
*
* Amazon Lookout for Equipment is a machine learning service that uses advanced analytics to identify anomalies in
* machines from sensor data for use in predictive maintenance.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonLookoutEquipmentAsync extends AmazonLookoutEquipment {
/**
*
* Creates a container for a collection of data being ingested for analysis. The dataset contains the metadata
* describing where the data is and what the data actually looks like. For example, it contains the location of the
* data source, the data schema, and other information. A dataset also contains any tags associated with the
* ingested data.
*
*
* @param createDatasetRequest
* @return A Java Future containing the result of the CreateDataset operation returned by the service.
* @sample AmazonLookoutEquipmentAsync.CreateDataset
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createDatasetAsync(CreateDatasetRequest createDatasetRequest);
/**
*
* Creates a container for a collection of data being ingested for analysis. The dataset contains the metadata
* describing where the data is and what the data actually looks like. For example, it contains the location of the
* data source, the data schema, and other information. A dataset also contains any tags associated with the
* ingested data.
*
*
* @param createDatasetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDataset operation returned by the service.
* @sample AmazonLookoutEquipmentAsyncHandler.CreateDataset
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createDatasetAsync(CreateDatasetRequest createDatasetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a scheduled inference. Scheduling an inference is setting up a continuous real-time inference plan to
* analyze new measurement data. When setting up the schedule, you provide an S3 bucket location for the input data,
* assign it a delimiter between separate entries in the data, set an offset delay if desired, and set the frequency
* of inferencing. You must also provide an S3 bucket location for the output data.
*
*
* @param createInferenceSchedulerRequest
* @return A Java Future containing the result of the CreateInferenceScheduler operation returned by the service.
* @sample AmazonLookoutEquipmentAsync.CreateInferenceScheduler
* @see AWS API Documentation
*/
java.util.concurrent.Future createInferenceSchedulerAsync(CreateInferenceSchedulerRequest createInferenceSchedulerRequest);
/**
*
* Creates a scheduled inference. Scheduling an inference is setting up a continuous real-time inference plan to
* analyze new measurement data. When setting up the schedule, you provide an S3 bucket location for the input data,
* assign it a delimiter between separate entries in the data, set an offset delay if desired, and set the frequency
* of inferencing. You must also provide an S3 bucket location for the output data.
*
*
* @param createInferenceSchedulerRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateInferenceScheduler operation returned by the service.
* @sample AmazonLookoutEquipmentAsyncHandler.CreateInferenceScheduler
* @see AWS API Documentation
*/
java.util.concurrent.Future createInferenceSchedulerAsync(CreateInferenceSchedulerRequest createInferenceSchedulerRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a label for an event.
*
*
* @param createLabelRequest
* @return A Java Future containing the result of the CreateLabel operation returned by the service.
* @sample AmazonLookoutEquipmentAsync.CreateLabel
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createLabelAsync(CreateLabelRequest createLabelRequest);
/**
*
* Creates a label for an event.
*
*
* @param createLabelRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateLabel operation returned by the service.
* @sample AmazonLookoutEquipmentAsyncHandler.CreateLabel
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createLabelAsync(CreateLabelRequest createLabelRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a group of labels.
*
*
* @param createLabelGroupRequest
* @return A Java Future containing the result of the CreateLabelGroup operation returned by the service.
* @sample AmazonLookoutEquipmentAsync.CreateLabelGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future createLabelGroupAsync(CreateLabelGroupRequest createLabelGroupRequest);
/**
*
* Creates a group of labels.
*
*
* @param createLabelGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateLabelGroup operation returned by the service.
* @sample AmazonLookoutEquipmentAsyncHandler.CreateLabelGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future createLabelGroupAsync(CreateLabelGroupRequest createLabelGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a machine learning model for data inference.
*
*
* A machine-learning (ML) model is a mathematical model that finds patterns in your data. In Amazon Lookout for
* Equipment, the model learns the patterns of normal behavior and detects abnormal behavior that could be potential
* equipment failure (or maintenance events). The models are made by analyzing normal data and abnormalities in
* machine behavior that have already occurred.
*
*
* Your model is trained using a portion of the data from your dataset and uses that data to learn patterns of
* normal behavior and abnormal patterns that lead to equipment failure. Another portion of the data is used to
* evaluate the model's accuracy.
*
*
* @param createModelRequest
* @return A Java Future containing the result of the CreateModel operation returned by the service.
* @sample AmazonLookoutEquipmentAsync.CreateModel
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createModelAsync(CreateModelRequest createModelRequest);
/**
*
* Creates a machine learning model for data inference.
*
*
* A machine-learning (ML) model is a mathematical model that finds patterns in your data. In Amazon Lookout for
* Equipment, the model learns the patterns of normal behavior and detects abnormal behavior that could be potential
* equipment failure (or maintenance events). The models are made by analyzing normal data and abnormalities in
* machine behavior that have already occurred.
*
*
* Your model is trained using a portion of the data from your dataset and uses that data to learn patterns of
* normal behavior and abnormal patterns that lead to equipment failure. Another portion of the data is used to
* evaluate the model's accuracy.
*
*
* @param createModelRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateModel operation returned by the service.
* @sample AmazonLookoutEquipmentAsyncHandler.CreateModel
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createModelAsync(CreateModelRequest createModelRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a retraining scheduler on the specified model.
*
*
* @param createRetrainingSchedulerRequest
* @return A Java Future containing the result of the CreateRetrainingScheduler operation returned by the service.
* @sample AmazonLookoutEquipmentAsync.CreateRetrainingScheduler
* @see AWS API Documentation
*/
java.util.concurrent.Future createRetrainingSchedulerAsync(
CreateRetrainingSchedulerRequest createRetrainingSchedulerRequest);
/**
*
* Creates a retraining scheduler on the specified model.
*
*
* @param createRetrainingSchedulerRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateRetrainingScheduler operation returned by the service.
* @sample AmazonLookoutEquipmentAsyncHandler.CreateRetrainingScheduler
* @see AWS API Documentation
*/
java.util.concurrent.Future createRetrainingSchedulerAsync(
CreateRetrainingSchedulerRequest createRetrainingSchedulerRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a dataset and associated artifacts. The operation will check to see if any inference scheduler or data
* ingestion job is currently using the dataset, and if there isn't, the dataset, its metadata, and any associated
* data stored in S3 will be deleted. This does not affect any models that used this dataset for training and
* evaluation, but does prevent it from being used in the future.
*
*
* @param deleteDatasetRequest
* @return A Java Future containing the result of the DeleteDataset operation returned by the service.
* @sample AmazonLookoutEquipmentAsync.DeleteDataset
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteDatasetAsync(DeleteDatasetRequest deleteDatasetRequest);
/**
*
* Deletes a dataset and associated artifacts. The operation will check to see if any inference scheduler or data
* ingestion job is currently using the dataset, and if there isn't, the dataset, its metadata, and any associated
* data stored in S3 will be deleted. This does not affect any models that used this dataset for training and
* evaluation, but does prevent it from being used in the future.
*
*
* @param deleteDatasetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteDataset operation returned by the service.
* @sample AmazonLookoutEquipmentAsyncHandler.DeleteDataset
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteDatasetAsync(DeleteDatasetRequest deleteDatasetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an inference scheduler that has been set up. Prior inference results will not be deleted.
*
*
* @param deleteInferenceSchedulerRequest
* @return A Java Future containing the result of the DeleteInferenceScheduler operation returned by the service.
* @sample AmazonLookoutEquipmentAsync.DeleteInferenceScheduler
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteInferenceSchedulerAsync(DeleteInferenceSchedulerRequest deleteInferenceSchedulerRequest);
/**
*
* Deletes an inference scheduler that has been set up. Prior inference results will not be deleted.
*
*
* @param deleteInferenceSchedulerRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteInferenceScheduler operation returned by the service.
* @sample AmazonLookoutEquipmentAsyncHandler.DeleteInferenceScheduler
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteInferenceSchedulerAsync(DeleteInferenceSchedulerRequest deleteInferenceSchedulerRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a label.
*
*
* @param deleteLabelRequest
* @return A Java Future containing the result of the DeleteLabel operation returned by the service.
* @sample AmazonLookoutEquipmentAsync.DeleteLabel
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteLabelAsync(DeleteLabelRequest deleteLabelRequest);
/**
*
* Deletes a label.
*
*
* @param deleteLabelRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteLabel operation returned by the service.
* @sample AmazonLookoutEquipmentAsyncHandler.DeleteLabel
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteLabelAsync(DeleteLabelRequest deleteLabelRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a group of labels.
*
*
* @param deleteLabelGroupRequest
* @return A Java Future containing the result of the DeleteLabelGroup operation returned by the service.
* @sample AmazonLookoutEquipmentAsync.DeleteLabelGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteLabelGroupAsync(DeleteLabelGroupRequest deleteLabelGroupRequest);
/**
*
* Deletes a group of labels.
*
*
* @param deleteLabelGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteLabelGroup operation returned by the service.
* @sample AmazonLookoutEquipmentAsyncHandler.DeleteLabelGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteLabelGroupAsync(DeleteLabelGroupRequest deleteLabelGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a machine learning model currently available for Amazon Lookout for Equipment. This will prevent it from
* being used with an inference scheduler, even one that is already set up.
*
*
* @param deleteModelRequest
* @return A Java Future containing the result of the DeleteModel operation returned by the service.
* @sample AmazonLookoutEquipmentAsync.DeleteModel
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteModelAsync(DeleteModelRequest deleteModelRequest);
/**
*
* Deletes a machine learning model currently available for Amazon Lookout for Equipment. This will prevent it from
* being used with an inference scheduler, even one that is already set up.
*
*
* @param deleteModelRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteModel operation returned by the service.
* @sample AmazonLookoutEquipmentAsyncHandler.DeleteModel
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteModelAsync(DeleteModelRequest deleteModelRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the resource policy attached to the resource.
*
*
* @param deleteResourcePolicyRequest
* @return A Java Future containing the result of the DeleteResourcePolicy operation returned by the service.
* @sample AmazonLookoutEquipmentAsync.DeleteResourcePolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteResourcePolicyAsync(DeleteResourcePolicyRequest deleteResourcePolicyRequest);
/**
*
* Deletes the resource policy attached to the resource.
*
*
* @param deleteResourcePolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteResourcePolicy operation returned by the service.
* @sample AmazonLookoutEquipmentAsyncHandler.DeleteResourcePolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteResourcePolicyAsync(DeleteResourcePolicyRequest deleteResourcePolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a retraining scheduler from a model. The retraining scheduler must be in the STOPPED
status.
*
*
* @param deleteRetrainingSchedulerRequest
* @return A Java Future containing the result of the DeleteRetrainingScheduler operation returned by the service.
* @sample AmazonLookoutEquipmentAsync.DeleteRetrainingScheduler
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteRetrainingSchedulerAsync(
DeleteRetrainingSchedulerRequest deleteRetrainingSchedulerRequest);
/**
*
* Deletes a retraining scheduler from a model. The retraining scheduler must be in the STOPPED
status.
*
*
* @param deleteRetrainingSchedulerRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteRetrainingScheduler operation returned by the service.
* @sample AmazonLookoutEquipmentAsyncHandler.DeleteRetrainingScheduler
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteRetrainingSchedulerAsync(
DeleteRetrainingSchedulerRequest deleteRetrainingSchedulerRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provides information on a specific data ingestion job such as creation time, dataset ARN, and status.
*
*
* @param describeDataIngestionJobRequest
* @return A Java Future containing the result of the DescribeDataIngestionJob operation returned by the service.
* @sample AmazonLookoutEquipmentAsync.DescribeDataIngestionJob
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDataIngestionJobAsync(DescribeDataIngestionJobRequest describeDataIngestionJobRequest);
/**
*
* Provides information on a specific data ingestion job such as creation time, dataset ARN, and status.
*
*
* @param describeDataIngestionJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDataIngestionJob operation returned by the service.
* @sample AmazonLookoutEquipmentAsyncHandler.DescribeDataIngestionJob
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDataIngestionJobAsync(DescribeDataIngestionJobRequest describeDataIngestionJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provides a JSON description of the data in each time series dataset, including names, column names, and data
* types.
*
*
* @param describeDatasetRequest
* @return A Java Future containing the result of the DescribeDataset operation returned by the service.
* @sample AmazonLookoutEquipmentAsync.DescribeDataset
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDatasetAsync(DescribeDatasetRequest describeDatasetRequest);
/**
*
* Provides a JSON description of the data in each time series dataset, including names, column names, and data
* types.
*
*
* @param describeDatasetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDataset operation returned by the service.
* @sample AmazonLookoutEquipmentAsyncHandler.DescribeDataset
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDatasetAsync(DescribeDatasetRequest describeDatasetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Specifies information about the inference scheduler being used, including name, model, status, and associated
* metadata
*
*
* @param describeInferenceSchedulerRequest
* @return A Java Future containing the result of the DescribeInferenceScheduler operation returned by the service.
* @sample AmazonLookoutEquipmentAsync.DescribeInferenceScheduler
* @see AWS API Documentation
*/
java.util.concurrent.Future describeInferenceSchedulerAsync(
DescribeInferenceSchedulerRequest describeInferenceSchedulerRequest);
/**
*
* Specifies information about the inference scheduler being used, including name, model, status, and associated
* metadata
*
*
* @param describeInferenceSchedulerRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeInferenceScheduler operation returned by the service.
* @sample AmazonLookoutEquipmentAsyncHandler.DescribeInferenceScheduler
* @see AWS API Documentation
*/
java.util.concurrent.Future describeInferenceSchedulerAsync(
DescribeInferenceSchedulerRequest describeInferenceSchedulerRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the name of the label.
*
*
* @param describeLabelRequest
* @return A Java Future containing the result of the DescribeLabel operation returned by the service.
* @sample AmazonLookoutEquipmentAsync.DescribeLabel
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeLabelAsync(DescribeLabelRequest describeLabelRequest);
/**
*
* Returns the name of the label.
*
*
* @param describeLabelRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeLabel operation returned by the service.
* @sample AmazonLookoutEquipmentAsyncHandler.DescribeLabel
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeLabelAsync(DescribeLabelRequest describeLabelRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about the label group.
*
*
* @param describeLabelGroupRequest
* @return A Java Future containing the result of the DescribeLabelGroup operation returned by the service.
* @sample AmazonLookoutEquipmentAsync.DescribeLabelGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future describeLabelGroupAsync(DescribeLabelGroupRequest describeLabelGroupRequest);
/**
*
* Returns information about the label group.
*
*
* @param describeLabelGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeLabelGroup operation returned by the service.
* @sample AmazonLookoutEquipmentAsyncHandler.DescribeLabelGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future describeLabelGroupAsync(DescribeLabelGroupRequest describeLabelGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provides a JSON containing the overall information about a specific machine learning model, including model name
* and ARN, dataset, training and evaluation information, status, and so on.
*
*
* @param describeModelRequest
* @return A Java Future containing the result of the DescribeModel operation returned by the service.
* @sample AmazonLookoutEquipmentAsync.DescribeModel
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeModelAsync(DescribeModelRequest describeModelRequest);
/**
*
* Provides a JSON containing the overall information about a specific machine learning model, including model name
* and ARN, dataset, training and evaluation information, status, and so on.
*
*
* @param describeModelRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeModel operation returned by the service.
* @sample AmazonLookoutEquipmentAsyncHandler.DescribeModel
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeModelAsync(DescribeModelRequest describeModelRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves information about a specific machine learning model version.
*
*
* @param describeModelVersionRequest
* @return A Java Future containing the result of the DescribeModelVersion operation returned by the service.
* @sample AmazonLookoutEquipmentAsync.DescribeModelVersion
* @see AWS API Documentation
*/
java.util.concurrent.Future describeModelVersionAsync(DescribeModelVersionRequest describeModelVersionRequest);
/**
*
* Retrieves information about a specific machine learning model version.
*
*
* @param describeModelVersionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeModelVersion operation returned by the service.
* @sample AmazonLookoutEquipmentAsyncHandler.DescribeModelVersion
* @see AWS API Documentation
*/
java.util.concurrent.Future describeModelVersionAsync(DescribeModelVersionRequest describeModelVersionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provides the details of a resource policy attached to a resource.
*
*
* @param describeResourcePolicyRequest
* @return A Java Future containing the result of the DescribeResourcePolicy operation returned by the service.
* @sample AmazonLookoutEquipmentAsync.DescribeResourcePolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future describeResourcePolicyAsync(DescribeResourcePolicyRequest describeResourcePolicyRequest);
/**
*
* Provides the details of a resource policy attached to a resource.
*
*
* @param describeResourcePolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeResourcePolicy operation returned by the service.
* @sample AmazonLookoutEquipmentAsyncHandler.DescribeResourcePolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future describeResourcePolicyAsync(DescribeResourcePolicyRequest describeResourcePolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provides a description of the retraining scheduler, including information such as the model name and retraining
* parameters.
*
*
* @param describeRetrainingSchedulerRequest
* @return A Java Future containing the result of the DescribeRetrainingScheduler operation returned by the service.
* @sample AmazonLookoutEquipmentAsync.DescribeRetrainingScheduler
* @see AWS API Documentation
*/
java.util.concurrent.Future describeRetrainingSchedulerAsync(
DescribeRetrainingSchedulerRequest describeRetrainingSchedulerRequest);
/**
*
* Provides a description of the retraining scheduler, including information such as the model name and retraining
* parameters.
*
*
* @param describeRetrainingSchedulerRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeRetrainingScheduler operation returned by the service.
* @sample AmazonLookoutEquipmentAsyncHandler.DescribeRetrainingScheduler
* @see AWS API Documentation
*/
java.util.concurrent.Future describeRetrainingSchedulerAsync(
DescribeRetrainingSchedulerRequest describeRetrainingSchedulerRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Imports a dataset.
*
*
* @param importDatasetRequest
* @return A Java Future containing the result of the ImportDataset operation returned by the service.
* @sample AmazonLookoutEquipmentAsync.ImportDataset
* @see AWS
* API Documentation
*/
java.util.concurrent.Future importDatasetAsync(ImportDatasetRequest importDatasetRequest);
/**
*
* Imports a dataset.
*
*
* @param importDatasetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ImportDataset operation returned by the service.
* @sample AmazonLookoutEquipmentAsyncHandler.ImportDataset
* @see AWS
* API Documentation
*/
java.util.concurrent.Future importDatasetAsync(ImportDatasetRequest importDatasetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Imports a model that has been trained successfully.
*
*
* @param importModelVersionRequest
* @return A Java Future containing the result of the ImportModelVersion operation returned by the service.
* @sample AmazonLookoutEquipmentAsync.ImportModelVersion
* @see AWS API Documentation
*/
java.util.concurrent.Future importModelVersionAsync(ImportModelVersionRequest importModelVersionRequest);
/**
*
* Imports a model that has been trained successfully.
*
*
* @param importModelVersionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ImportModelVersion operation returned by the service.
* @sample AmazonLookoutEquipmentAsyncHandler.ImportModelVersion
* @see AWS API Documentation
*/
java.util.concurrent.Future importModelVersionAsync(ImportModelVersionRequest importModelVersionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provides a list of all data ingestion jobs, including dataset name and ARN, S3 location of the input data,
* status, and so on.
*
*
* @param listDataIngestionJobsRequest
* @return A Java Future containing the result of the ListDataIngestionJobs operation returned by the service.
* @sample AmazonLookoutEquipmentAsync.ListDataIngestionJobs
* @see AWS API Documentation
*/
java.util.concurrent.Future listDataIngestionJobsAsync(ListDataIngestionJobsRequest listDataIngestionJobsRequest);
/**
*
* Provides a list of all data ingestion jobs, including dataset name and ARN, S3 location of the input data,
* status, and so on.
*
*
* @param listDataIngestionJobsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListDataIngestionJobs operation returned by the service.
* @sample AmazonLookoutEquipmentAsyncHandler.ListDataIngestionJobs
* @see AWS API Documentation
*/
java.util.concurrent.Future listDataIngestionJobsAsync(ListDataIngestionJobsRequest listDataIngestionJobsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all datasets currently available in your account, filtering on the dataset name.
*
*
* @param listDatasetsRequest
* @return A Java Future containing the result of the ListDatasets operation returned by the service.
* @sample AmazonLookoutEquipmentAsync.ListDatasets
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listDatasetsAsync(ListDatasetsRequest listDatasetsRequest);
/**
*
* Lists all datasets currently available in your account, filtering on the dataset name.
*
*
* @param listDatasetsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListDatasets operation returned by the service.
* @sample AmazonLookoutEquipmentAsyncHandler.ListDatasets
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listDatasetsAsync(ListDatasetsRequest listDatasetsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all inference events that have been found for the specified inference scheduler.
*
*
* @param listInferenceEventsRequest
* @return A Java Future containing the result of the ListInferenceEvents operation returned by the service.
* @sample AmazonLookoutEquipmentAsync.ListInferenceEvents
* @see AWS API Documentation
*/
java.util.concurrent.Future listInferenceEventsAsync(ListInferenceEventsRequest listInferenceEventsRequest);
/**
*
* Lists all inference events that have been found for the specified inference scheduler.
*
*
* @param listInferenceEventsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListInferenceEvents operation returned by the service.
* @sample AmazonLookoutEquipmentAsyncHandler.ListInferenceEvents
* @see AWS API Documentation
*/
java.util.concurrent.Future listInferenceEventsAsync(ListInferenceEventsRequest listInferenceEventsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all inference executions that have been performed by the specified inference scheduler.
*
*
* @param listInferenceExecutionsRequest
* @return A Java Future containing the result of the ListInferenceExecutions operation returned by the service.
* @sample AmazonLookoutEquipmentAsync.ListInferenceExecutions
* @see AWS API Documentation
*/
java.util.concurrent.Future listInferenceExecutionsAsync(ListInferenceExecutionsRequest listInferenceExecutionsRequest);
/**
*
* Lists all inference executions that have been performed by the specified inference scheduler.
*
*
* @param listInferenceExecutionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListInferenceExecutions operation returned by the service.
* @sample AmazonLookoutEquipmentAsyncHandler.ListInferenceExecutions
* @see AWS API Documentation
*/
java.util.concurrent.Future listInferenceExecutionsAsync(ListInferenceExecutionsRequest listInferenceExecutionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a list of all inference schedulers currently available for your account.
*
*
* @param listInferenceSchedulersRequest
* @return A Java Future containing the result of the ListInferenceSchedulers operation returned by the service.
* @sample AmazonLookoutEquipmentAsync.ListInferenceSchedulers
* @see AWS API Documentation
*/
java.util.concurrent.Future listInferenceSchedulersAsync(ListInferenceSchedulersRequest listInferenceSchedulersRequest);
/**
*
* Retrieves a list of all inference schedulers currently available for your account.
*
*
* @param listInferenceSchedulersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListInferenceSchedulers operation returned by the service.
* @sample AmazonLookoutEquipmentAsyncHandler.ListInferenceSchedulers
* @see AWS API Documentation
*/
java.util.concurrent.Future listInferenceSchedulersAsync(ListInferenceSchedulersRequest listInferenceSchedulersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of the label groups.
*
*
* @param listLabelGroupsRequest
* @return A Java Future containing the result of the ListLabelGroups operation returned by the service.
* @sample AmazonLookoutEquipmentAsync.ListLabelGroups
* @see AWS API Documentation
*/
java.util.concurrent.Future listLabelGroupsAsync(ListLabelGroupsRequest listLabelGroupsRequest);
/**
*
* Returns a list of the label groups.
*
*
* @param listLabelGroupsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListLabelGroups operation returned by the service.
* @sample AmazonLookoutEquipmentAsyncHandler.ListLabelGroups
* @see AWS API Documentation
*/
java.util.concurrent.Future listLabelGroupsAsync(ListLabelGroupsRequest listLabelGroupsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provides a list of labels.
*
*
* @param listLabelsRequest
* @return A Java Future containing the result of the ListLabels operation returned by the service.
* @sample AmazonLookoutEquipmentAsync.ListLabels
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listLabelsAsync(ListLabelsRequest listLabelsRequest);
/**
*
* Provides a list of labels.
*
*
* @param listLabelsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListLabels operation returned by the service.
* @sample AmazonLookoutEquipmentAsyncHandler.ListLabels
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listLabelsAsync(ListLabelsRequest listLabelsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Generates a list of all model versions for a given model, including the model version, model version ARN, and
* status. To list a subset of versions, use the MaxModelVersion
and MinModelVersion
* fields.
*
*
* @param listModelVersionsRequest
* @return A Java Future containing the result of the ListModelVersions operation returned by the service.
* @sample AmazonLookoutEquipmentAsync.ListModelVersions
* @see AWS API Documentation
*/
java.util.concurrent.Future listModelVersionsAsync(ListModelVersionsRequest listModelVersionsRequest);
/**
*
* Generates a list of all model versions for a given model, including the model version, model version ARN, and
* status. To list a subset of versions, use the MaxModelVersion
and MinModelVersion
* fields.
*
*
* @param listModelVersionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListModelVersions operation returned by the service.
* @sample AmazonLookoutEquipmentAsyncHandler.ListModelVersions
* @see AWS API Documentation
*/
java.util.concurrent.Future listModelVersionsAsync(ListModelVersionsRequest listModelVersionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Generates a list of all models in the account, including model name and ARN, dataset, and status.
*
*
* @param listModelsRequest
* @return A Java Future containing the result of the ListModels operation returned by the service.
* @sample AmazonLookoutEquipmentAsync.ListModels
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listModelsAsync(ListModelsRequest listModelsRequest);
/**
*
* Generates a list of all models in the account, including model name and ARN, dataset, and status.
*
*
* @param listModelsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListModels operation returned by the service.
* @sample AmazonLookoutEquipmentAsyncHandler.ListModels
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listModelsAsync(ListModelsRequest listModelsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all retraining schedulers in your account, filtering by model name prefix and status.
*
*
* @param listRetrainingSchedulersRequest
* @return A Java Future containing the result of the ListRetrainingSchedulers operation returned by the service.
* @sample AmazonLookoutEquipmentAsync.ListRetrainingSchedulers
* @see AWS API Documentation
*/
java.util.concurrent.Future listRetrainingSchedulersAsync(ListRetrainingSchedulersRequest listRetrainingSchedulersRequest);
/**
*
* Lists all retraining schedulers in your account, filtering by model name prefix and status.
*
*
* @param listRetrainingSchedulersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListRetrainingSchedulers operation returned by the service.
* @sample AmazonLookoutEquipmentAsyncHandler.ListRetrainingSchedulers
* @see AWS API Documentation
*/
java.util.concurrent.Future listRetrainingSchedulersAsync(ListRetrainingSchedulersRequest listRetrainingSchedulersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists statistics about the data collected for each of the sensors that have been successfully ingested in the
* particular dataset. Can also be used to retreive Sensor Statistics for a previous ingestion job.
*
*
* @param listSensorStatisticsRequest
* @return A Java Future containing the result of the ListSensorStatistics operation returned by the service.
* @sample AmazonLookoutEquipmentAsync.ListSensorStatistics
* @see AWS API Documentation
*/
java.util.concurrent.Future listSensorStatisticsAsync(ListSensorStatisticsRequest listSensorStatisticsRequest);
/**
*
* Lists statistics about the data collected for each of the sensors that have been successfully ingested in the
* particular dataset. Can also be used to retreive Sensor Statistics for a previous ingestion job.
*
*
* @param listSensorStatisticsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListSensorStatistics operation returned by the service.
* @sample AmazonLookoutEquipmentAsyncHandler.ListSensorStatistics
* @see AWS API Documentation
*/
java.util.concurrent.Future listSensorStatisticsAsync(ListSensorStatisticsRequest listSensorStatisticsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all the tags for a specified resource, including key and value.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AmazonLookoutEquipmentAsync.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Lists all the tags for a specified resource, including key and value.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AmazonLookoutEquipmentAsyncHandler.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a resource control policy for a given resource.
*
*
* @param putResourcePolicyRequest
* @return A Java Future containing the result of the PutResourcePolicy operation returned by the service.
* @sample AmazonLookoutEquipmentAsync.PutResourcePolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future putResourcePolicyAsync(PutResourcePolicyRequest putResourcePolicyRequest);
/**
*
* Creates a resource control policy for a given resource.
*
*
* @param putResourcePolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutResourcePolicy operation returned by the service.
* @sample AmazonLookoutEquipmentAsyncHandler.PutResourcePolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future putResourcePolicyAsync(PutResourcePolicyRequest putResourcePolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Starts a data ingestion job. Amazon Lookout for Equipment returns the job status.
*
*
* @param startDataIngestionJobRequest
* @return A Java Future containing the result of the StartDataIngestionJob operation returned by the service.
* @sample AmazonLookoutEquipmentAsync.StartDataIngestionJob
* @see AWS API Documentation
*/
java.util.concurrent.Future startDataIngestionJobAsync(StartDataIngestionJobRequest startDataIngestionJobRequest);
/**
*
* Starts a data ingestion job. Amazon Lookout for Equipment returns the job status.
*
*
* @param startDataIngestionJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StartDataIngestionJob operation returned by the service.
* @sample AmazonLookoutEquipmentAsyncHandler.StartDataIngestionJob
* @see AWS API Documentation
*/
java.util.concurrent.Future startDataIngestionJobAsync(StartDataIngestionJobRequest startDataIngestionJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Starts an inference scheduler.
*
*
* @param startInferenceSchedulerRequest
* @return A Java Future containing the result of the StartInferenceScheduler operation returned by the service.
* @sample AmazonLookoutEquipmentAsync.StartInferenceScheduler
* @see AWS API Documentation
*/
java.util.concurrent.Future startInferenceSchedulerAsync(StartInferenceSchedulerRequest startInferenceSchedulerRequest);
/**
*
* Starts an inference scheduler.
*
*
* @param startInferenceSchedulerRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StartInferenceScheduler operation returned by the service.
* @sample AmazonLookoutEquipmentAsyncHandler.StartInferenceScheduler
* @see AWS API Documentation
*/
java.util.concurrent.Future startInferenceSchedulerAsync(StartInferenceSchedulerRequest startInferenceSchedulerRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Starts a retraining scheduler.
*
*
* @param startRetrainingSchedulerRequest
* @return A Java Future containing the result of the StartRetrainingScheduler operation returned by the service.
* @sample AmazonLookoutEquipmentAsync.StartRetrainingScheduler
* @see AWS API Documentation
*/
java.util.concurrent.Future startRetrainingSchedulerAsync(StartRetrainingSchedulerRequest startRetrainingSchedulerRequest);
/**
*
* Starts a retraining scheduler.
*
*
* @param startRetrainingSchedulerRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StartRetrainingScheduler operation returned by the service.
* @sample AmazonLookoutEquipmentAsyncHandler.StartRetrainingScheduler
* @see AWS API Documentation
*/
java.util.concurrent.Future startRetrainingSchedulerAsync(StartRetrainingSchedulerRequest startRetrainingSchedulerRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Stops an inference scheduler.
*
*
* @param stopInferenceSchedulerRequest
* @return A Java Future containing the result of the StopInferenceScheduler operation returned by the service.
* @sample AmazonLookoutEquipmentAsync.StopInferenceScheduler
* @see AWS API Documentation
*/
java.util.concurrent.Future stopInferenceSchedulerAsync(StopInferenceSchedulerRequest stopInferenceSchedulerRequest);
/**
*
* Stops an inference scheduler.
*
*
* @param stopInferenceSchedulerRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StopInferenceScheduler operation returned by the service.
* @sample AmazonLookoutEquipmentAsyncHandler.StopInferenceScheduler
* @see AWS API Documentation
*/
java.util.concurrent.Future stopInferenceSchedulerAsync(StopInferenceSchedulerRequest stopInferenceSchedulerRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Stops a retraining scheduler.
*
*
* @param stopRetrainingSchedulerRequest
* @return A Java Future containing the result of the StopRetrainingScheduler operation returned by the service.
* @sample AmazonLookoutEquipmentAsync.StopRetrainingScheduler
* @see AWS API Documentation
*/
java.util.concurrent.Future stopRetrainingSchedulerAsync(StopRetrainingSchedulerRequest stopRetrainingSchedulerRequest);
/**
*
* Stops a retraining scheduler.
*
*
* @param stopRetrainingSchedulerRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StopRetrainingScheduler operation returned by the service.
* @sample AmazonLookoutEquipmentAsyncHandler.StopRetrainingScheduler
* @see AWS API Documentation
*/
java.util.concurrent.Future stopRetrainingSchedulerAsync(StopRetrainingSchedulerRequest stopRetrainingSchedulerRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Associates a given tag to a resource in your account. A tag is a key-value pair which can be added to an Amazon
* Lookout for Equipment resource as metadata. Tags can be used for organizing your resources as well as helping you
* to search and filter by tag. Multiple tags can be added to a resource, either when you create it, or later. Up to
* 50 tags can be associated with each resource.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AmazonLookoutEquipmentAsync.TagResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* Associates a given tag to a resource in your account. A tag is a key-value pair which can be added to an Amazon
* Lookout for Equipment resource as metadata. Tags can be used for organizing your resources as well as helping you
* to search and filter by tag. Multiple tags can be added to a resource, either when you create it, or later. Up to
* 50 tags can be associated with each resource.
*
*
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AmazonLookoutEquipmentAsyncHandler.TagResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes a specific tag from a given resource. The tag is specified by its key.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AmazonLookoutEquipmentAsync.UntagResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* Removes a specific tag from a given resource. The tag is specified by its key.
*
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AmazonLookoutEquipmentAsyncHandler.UntagResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Sets the active model version for a given machine learning model.
*
*
* @param updateActiveModelVersionRequest
* @return A Java Future containing the result of the UpdateActiveModelVersion operation returned by the service.
* @sample AmazonLookoutEquipmentAsync.UpdateActiveModelVersion
* @see AWS API Documentation
*/
java.util.concurrent.Future updateActiveModelVersionAsync(UpdateActiveModelVersionRequest updateActiveModelVersionRequest);
/**
*
* Sets the active model version for a given machine learning model.
*
*
* @param updateActiveModelVersionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateActiveModelVersion operation returned by the service.
* @sample AmazonLookoutEquipmentAsyncHandler.UpdateActiveModelVersion
* @see AWS API Documentation
*/
java.util.concurrent.Future updateActiveModelVersionAsync(UpdateActiveModelVersionRequest updateActiveModelVersionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates an inference scheduler.
*
*
* @param updateInferenceSchedulerRequest
* @return A Java Future containing the result of the UpdateInferenceScheduler operation returned by the service.
* @sample AmazonLookoutEquipmentAsync.UpdateInferenceScheduler
* @see AWS API Documentation
*/
java.util.concurrent.Future updateInferenceSchedulerAsync(UpdateInferenceSchedulerRequest updateInferenceSchedulerRequest);
/**
*
* Updates an inference scheduler.
*
*
* @param updateInferenceSchedulerRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateInferenceScheduler operation returned by the service.
* @sample AmazonLookoutEquipmentAsyncHandler.UpdateInferenceScheduler
* @see AWS API Documentation
*/
java.util.concurrent.Future updateInferenceSchedulerAsync(UpdateInferenceSchedulerRequest updateInferenceSchedulerRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the label group.
*
*
* @param updateLabelGroupRequest
* @return A Java Future containing the result of the UpdateLabelGroup operation returned by the service.
* @sample AmazonLookoutEquipmentAsync.UpdateLabelGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future updateLabelGroupAsync(UpdateLabelGroupRequest updateLabelGroupRequest);
/**
*
* Updates the label group.
*
*
* @param updateLabelGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateLabelGroup operation returned by the service.
* @sample AmazonLookoutEquipmentAsyncHandler.UpdateLabelGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future updateLabelGroupAsync(UpdateLabelGroupRequest updateLabelGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates a model in the account.
*
*
* @param updateModelRequest
* @return A Java Future containing the result of the UpdateModel operation returned by the service.
* @sample AmazonLookoutEquipmentAsync.UpdateModel
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateModelAsync(UpdateModelRequest updateModelRequest);
/**
*
* Updates a model in the account.
*
*
* @param updateModelRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateModel operation returned by the service.
* @sample AmazonLookoutEquipmentAsyncHandler.UpdateModel
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateModelAsync(UpdateModelRequest updateModelRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates a retraining scheduler.
*
*
* @param updateRetrainingSchedulerRequest
* @return A Java Future containing the result of the UpdateRetrainingScheduler operation returned by the service.
* @sample AmazonLookoutEquipmentAsync.UpdateRetrainingScheduler
* @see AWS API Documentation
*/
java.util.concurrent.Future updateRetrainingSchedulerAsync(
UpdateRetrainingSchedulerRequest updateRetrainingSchedulerRequest);
/**
*
* Updates a retraining scheduler.
*
*
* @param updateRetrainingSchedulerRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateRetrainingScheduler operation returned by the service.
* @sample AmazonLookoutEquipmentAsyncHandler.UpdateRetrainingScheduler
* @see AWS API Documentation
*/
java.util.concurrent.Future updateRetrainingSchedulerAsync(
UpdateRetrainingSchedulerRequest updateRetrainingSchedulerRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}