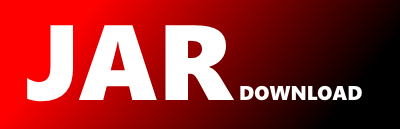
com.amazonaws.services.lookoutequipment.model.CreateInferenceSchedulerResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-lookoutequipment Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.lookoutequipment.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateInferenceSchedulerResult extends com.amazonaws.AmazonWebServiceResult implements Serializable, Cloneable {
/**
*
* The Amazon Resource Name (ARN) of the inference scheduler being created.
*
*/
private String inferenceSchedulerArn;
/**
*
* The name of inference scheduler being created.
*
*/
private String inferenceSchedulerName;
/**
*
* Indicates the status of the CreateInferenceScheduler
operation.
*
*/
private String status;
/**
*
* Provides a quality assessment for a model that uses labels. If Lookout for Equipment determines that the model
* quality is poor based on training metrics, the value is POOR_QUALITY_DETECTED
. Otherwise, the value
* is QUALITY_THRESHOLD_MET
.
*
*
* If the model is unlabeled, the model quality can't be assessed and the value of ModelQuality
is
* CANNOT_DETERMINE_QUALITY
. In this situation, you can get a model quality assessment by adding labels
* to the input dataset and retraining the model.
*
*
* For information about using labels with your models, see Understanding
* labeling.
*
*
* For information about improving the quality of a model, see Best practices with Amazon
* Lookout for Equipment.
*
*/
private String modelQuality;
/**
*
* The Amazon Resource Name (ARN) of the inference scheduler being created.
*
*
* @param inferenceSchedulerArn
* The Amazon Resource Name (ARN) of the inference scheduler being created.
*/
public void setInferenceSchedulerArn(String inferenceSchedulerArn) {
this.inferenceSchedulerArn = inferenceSchedulerArn;
}
/**
*
* The Amazon Resource Name (ARN) of the inference scheduler being created.
*
*
* @return The Amazon Resource Name (ARN) of the inference scheduler being created.
*/
public String getInferenceSchedulerArn() {
return this.inferenceSchedulerArn;
}
/**
*
* The Amazon Resource Name (ARN) of the inference scheduler being created.
*
*
* @param inferenceSchedulerArn
* The Amazon Resource Name (ARN) of the inference scheduler being created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateInferenceSchedulerResult withInferenceSchedulerArn(String inferenceSchedulerArn) {
setInferenceSchedulerArn(inferenceSchedulerArn);
return this;
}
/**
*
* The name of inference scheduler being created.
*
*
* @param inferenceSchedulerName
* The name of inference scheduler being created.
*/
public void setInferenceSchedulerName(String inferenceSchedulerName) {
this.inferenceSchedulerName = inferenceSchedulerName;
}
/**
*
* The name of inference scheduler being created.
*
*
* @return The name of inference scheduler being created.
*/
public String getInferenceSchedulerName() {
return this.inferenceSchedulerName;
}
/**
*
* The name of inference scheduler being created.
*
*
* @param inferenceSchedulerName
* The name of inference scheduler being created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateInferenceSchedulerResult withInferenceSchedulerName(String inferenceSchedulerName) {
setInferenceSchedulerName(inferenceSchedulerName);
return this;
}
/**
*
* Indicates the status of the CreateInferenceScheduler
operation.
*
*
* @param status
* Indicates the status of the CreateInferenceScheduler
operation.
* @see InferenceSchedulerStatus
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* Indicates the status of the CreateInferenceScheduler
operation.
*
*
* @return Indicates the status of the CreateInferenceScheduler
operation.
* @see InferenceSchedulerStatus
*/
public String getStatus() {
return this.status;
}
/**
*
* Indicates the status of the CreateInferenceScheduler
operation.
*
*
* @param status
* Indicates the status of the CreateInferenceScheduler
operation.
* @return Returns a reference to this object so that method calls can be chained together.
* @see InferenceSchedulerStatus
*/
public CreateInferenceSchedulerResult withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* Indicates the status of the CreateInferenceScheduler
operation.
*
*
* @param status
* Indicates the status of the CreateInferenceScheduler
operation.
* @return Returns a reference to this object so that method calls can be chained together.
* @see InferenceSchedulerStatus
*/
public CreateInferenceSchedulerResult withStatus(InferenceSchedulerStatus status) {
this.status = status.toString();
return this;
}
/**
*
* Provides a quality assessment for a model that uses labels. If Lookout for Equipment determines that the model
* quality is poor based on training metrics, the value is POOR_QUALITY_DETECTED
. Otherwise, the value
* is QUALITY_THRESHOLD_MET
.
*
*
* If the model is unlabeled, the model quality can't be assessed and the value of ModelQuality
is
* CANNOT_DETERMINE_QUALITY
. In this situation, you can get a model quality assessment by adding labels
* to the input dataset and retraining the model.
*
*
* For information about using labels with your models, see Understanding
* labeling.
*
*
* For information about improving the quality of a model, see Best practices with Amazon
* Lookout for Equipment.
*
*
* @param modelQuality
* Provides a quality assessment for a model that uses labels. If Lookout for Equipment determines that the
* model quality is poor based on training metrics, the value is POOR_QUALITY_DETECTED
.
* Otherwise, the value is QUALITY_THRESHOLD_MET
.
*
* If the model is unlabeled, the model quality can't be assessed and the value of ModelQuality
* is CANNOT_DETERMINE_QUALITY
. In this situation, you can get a model quality assessment by
* adding labels to the input dataset and retraining the model.
*
*
* For information about using labels with your models, see Understanding labeling.
*
*
* For information about improving the quality of a model, see Best practices with
* Amazon Lookout for Equipment.
* @see ModelQuality
*/
public void setModelQuality(String modelQuality) {
this.modelQuality = modelQuality;
}
/**
*
* Provides a quality assessment for a model that uses labels. If Lookout for Equipment determines that the model
* quality is poor based on training metrics, the value is POOR_QUALITY_DETECTED
. Otherwise, the value
* is QUALITY_THRESHOLD_MET
.
*
*
* If the model is unlabeled, the model quality can't be assessed and the value of ModelQuality
is
* CANNOT_DETERMINE_QUALITY
. In this situation, you can get a model quality assessment by adding labels
* to the input dataset and retraining the model.
*
*
* For information about using labels with your models, see Understanding
* labeling.
*
*
* For information about improving the quality of a model, see Best practices with Amazon
* Lookout for Equipment.
*
*
* @return Provides a quality assessment for a model that uses labels. If Lookout for Equipment determines that the
* model quality is poor based on training metrics, the value is POOR_QUALITY_DETECTED
.
* Otherwise, the value is QUALITY_THRESHOLD_MET
.
*
* If the model is unlabeled, the model quality can't be assessed and the value of ModelQuality
* is CANNOT_DETERMINE_QUALITY
. In this situation, you can get a model quality assessment by
* adding labels to the input dataset and retraining the model.
*
*
* For information about using labels with your models, see Understanding labeling.
*
*
* For information about improving the quality of a model, see Best practices
* with Amazon Lookout for Equipment.
* @see ModelQuality
*/
public String getModelQuality() {
return this.modelQuality;
}
/**
*
* Provides a quality assessment for a model that uses labels. If Lookout for Equipment determines that the model
* quality is poor based on training metrics, the value is POOR_QUALITY_DETECTED
. Otherwise, the value
* is QUALITY_THRESHOLD_MET
.
*
*
* If the model is unlabeled, the model quality can't be assessed and the value of ModelQuality
is
* CANNOT_DETERMINE_QUALITY
. In this situation, you can get a model quality assessment by adding labels
* to the input dataset and retraining the model.
*
*
* For information about using labels with your models, see Understanding
* labeling.
*
*
* For information about improving the quality of a model, see Best practices with Amazon
* Lookout for Equipment.
*
*
* @param modelQuality
* Provides a quality assessment for a model that uses labels. If Lookout for Equipment determines that the
* model quality is poor based on training metrics, the value is POOR_QUALITY_DETECTED
.
* Otherwise, the value is QUALITY_THRESHOLD_MET
.
*
* If the model is unlabeled, the model quality can't be assessed and the value of ModelQuality
* is CANNOT_DETERMINE_QUALITY
. In this situation, you can get a model quality assessment by
* adding labels to the input dataset and retraining the model.
*
*
* For information about using labels with your models, see Understanding labeling.
*
*
* For information about improving the quality of a model, see Best practices with
* Amazon Lookout for Equipment.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ModelQuality
*/
public CreateInferenceSchedulerResult withModelQuality(String modelQuality) {
setModelQuality(modelQuality);
return this;
}
/**
*
* Provides a quality assessment for a model that uses labels. If Lookout for Equipment determines that the model
* quality is poor based on training metrics, the value is POOR_QUALITY_DETECTED
. Otherwise, the value
* is QUALITY_THRESHOLD_MET
.
*
*
* If the model is unlabeled, the model quality can't be assessed and the value of ModelQuality
is
* CANNOT_DETERMINE_QUALITY
. In this situation, you can get a model quality assessment by adding labels
* to the input dataset and retraining the model.
*
*
* For information about using labels with your models, see Understanding
* labeling.
*
*
* For information about improving the quality of a model, see Best practices with Amazon
* Lookout for Equipment.
*
*
* @param modelQuality
* Provides a quality assessment for a model that uses labels. If Lookout for Equipment determines that the
* model quality is poor based on training metrics, the value is POOR_QUALITY_DETECTED
.
* Otherwise, the value is QUALITY_THRESHOLD_MET
.
*
* If the model is unlabeled, the model quality can't be assessed and the value of ModelQuality
* is CANNOT_DETERMINE_QUALITY
. In this situation, you can get a model quality assessment by
* adding labels to the input dataset and retraining the model.
*
*
* For information about using labels with your models, see Understanding labeling.
*
*
* For information about improving the quality of a model, see Best practices with
* Amazon Lookout for Equipment.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ModelQuality
*/
public CreateInferenceSchedulerResult withModelQuality(ModelQuality modelQuality) {
this.modelQuality = modelQuality.toString();
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getInferenceSchedulerArn() != null)
sb.append("InferenceSchedulerArn: ").append(getInferenceSchedulerArn()).append(",");
if (getInferenceSchedulerName() != null)
sb.append("InferenceSchedulerName: ").append(getInferenceSchedulerName()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getModelQuality() != null)
sb.append("ModelQuality: ").append(getModelQuality());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateInferenceSchedulerResult == false)
return false;
CreateInferenceSchedulerResult other = (CreateInferenceSchedulerResult) obj;
if (other.getInferenceSchedulerArn() == null ^ this.getInferenceSchedulerArn() == null)
return false;
if (other.getInferenceSchedulerArn() != null && other.getInferenceSchedulerArn().equals(this.getInferenceSchedulerArn()) == false)
return false;
if (other.getInferenceSchedulerName() == null ^ this.getInferenceSchedulerName() == null)
return false;
if (other.getInferenceSchedulerName() != null && other.getInferenceSchedulerName().equals(this.getInferenceSchedulerName()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getModelQuality() == null ^ this.getModelQuality() == null)
return false;
if (other.getModelQuality() != null && other.getModelQuality().equals(this.getModelQuality()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getInferenceSchedulerArn() == null) ? 0 : getInferenceSchedulerArn().hashCode());
hashCode = prime * hashCode + ((getInferenceSchedulerName() == null) ? 0 : getInferenceSchedulerName().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getModelQuality() == null) ? 0 : getModelQuality().hashCode());
return hashCode;
}
@Override
public CreateInferenceSchedulerResult clone() {
try {
return (CreateInferenceSchedulerResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}