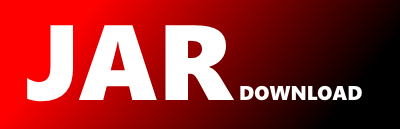
com.amazonaws.services.lookoutequipment.model.CreateModelRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-lookoutequipment Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.lookoutequipment.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateModelRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The name for the machine learning model to be created.
*
*/
private String modelName;
/**
*
* The name of the dataset for the machine learning model being created.
*
*/
private String datasetName;
/**
*
* The data schema for the machine learning model being created.
*
*/
private DatasetSchema datasetSchema;
/**
*
* The input configuration for the labels being used for the machine learning model that's being created.
*
*/
private LabelsInputConfiguration labelsInputConfiguration;
/**
*
* A unique identifier for the request. If you do not set the client request token, Amazon Lookout for Equipment
* generates one.
*
*/
private String clientToken;
/**
*
* Indicates the time reference in the dataset that should be used to begin the subset of training data for the
* machine learning model.
*
*/
private java.util.Date trainingDataStartTime;
/**
*
* Indicates the time reference in the dataset that should be used to end the subset of training data for the
* machine learning model.
*
*/
private java.util.Date trainingDataEndTime;
/**
*
* Indicates the time reference in the dataset that should be used to begin the subset of evaluation data for the
* machine learning model.
*
*/
private java.util.Date evaluationDataStartTime;
/**
*
* Indicates the time reference in the dataset that should be used to end the subset of evaluation data for the
* machine learning model.
*
*/
private java.util.Date evaluationDataEndTime;
/**
*
* The Amazon Resource Name (ARN) of a role with permission to access the data source being used to create the
* machine learning model.
*
*/
private String roleArn;
/**
*
* The configuration is the TargetSamplingRate
, which is the sampling rate of the data after post
* processing by Amazon Lookout for Equipment. For example, if you provide data that has been collected at a 1
* second level and you want the system to resample the data at a 1 minute rate before training, the
* TargetSamplingRate
is 1 minute.
*
*
* When providing a value for the TargetSamplingRate
, you must attach the prefix "PT" to the rate you
* want. The value for a 1 second rate is therefore PT1S, the value for a 15 minute rate is PT15M, and
* the value for a 1 hour rate is PT1H
*
*/
private DataPreProcessingConfiguration dataPreProcessingConfiguration;
/**
*
* Provides the identifier of the KMS key used to encrypt model data by Amazon Lookout for Equipment.
*
*/
private String serverSideKmsKeyId;
/**
*
* Any tags associated with the machine learning model being created.
*
*/
private java.util.List tags;
/**
*
* Indicates that the asset associated with this sensor has been shut off. As long as this condition is met, Lookout
* for Equipment will not use data from this asset for training, evaluation, or inference.
*
*/
private String offCondition;
/**
*
* The Amazon S3 location where you want Amazon Lookout for Equipment to save the pointwise model diagnostics. You
* must also specify the RoleArn
request parameter.
*
*/
private ModelDiagnosticsOutputConfiguration modelDiagnosticsOutputConfiguration;
/**
*
* The name for the machine learning model to be created.
*
*
* @param modelName
* The name for the machine learning model to be created.
*/
public void setModelName(String modelName) {
this.modelName = modelName;
}
/**
*
* The name for the machine learning model to be created.
*
*
* @return The name for the machine learning model to be created.
*/
public String getModelName() {
return this.modelName;
}
/**
*
* The name for the machine learning model to be created.
*
*
* @param modelName
* The name for the machine learning model to be created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateModelRequest withModelName(String modelName) {
setModelName(modelName);
return this;
}
/**
*
* The name of the dataset for the machine learning model being created.
*
*
* @param datasetName
* The name of the dataset for the machine learning model being created.
*/
public void setDatasetName(String datasetName) {
this.datasetName = datasetName;
}
/**
*
* The name of the dataset for the machine learning model being created.
*
*
* @return The name of the dataset for the machine learning model being created.
*/
public String getDatasetName() {
return this.datasetName;
}
/**
*
* The name of the dataset for the machine learning model being created.
*
*
* @param datasetName
* The name of the dataset for the machine learning model being created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateModelRequest withDatasetName(String datasetName) {
setDatasetName(datasetName);
return this;
}
/**
*
* The data schema for the machine learning model being created.
*
*
* @param datasetSchema
* The data schema for the machine learning model being created.
*/
public void setDatasetSchema(DatasetSchema datasetSchema) {
this.datasetSchema = datasetSchema;
}
/**
*
* The data schema for the machine learning model being created.
*
*
* @return The data schema for the machine learning model being created.
*/
public DatasetSchema getDatasetSchema() {
return this.datasetSchema;
}
/**
*
* The data schema for the machine learning model being created.
*
*
* @param datasetSchema
* The data schema for the machine learning model being created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateModelRequest withDatasetSchema(DatasetSchema datasetSchema) {
setDatasetSchema(datasetSchema);
return this;
}
/**
*
* The input configuration for the labels being used for the machine learning model that's being created.
*
*
* @param labelsInputConfiguration
* The input configuration for the labels being used for the machine learning model that's being created.
*/
public void setLabelsInputConfiguration(LabelsInputConfiguration labelsInputConfiguration) {
this.labelsInputConfiguration = labelsInputConfiguration;
}
/**
*
* The input configuration for the labels being used for the machine learning model that's being created.
*
*
* @return The input configuration for the labels being used for the machine learning model that's being created.
*/
public LabelsInputConfiguration getLabelsInputConfiguration() {
return this.labelsInputConfiguration;
}
/**
*
* The input configuration for the labels being used for the machine learning model that's being created.
*
*
* @param labelsInputConfiguration
* The input configuration for the labels being used for the machine learning model that's being created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateModelRequest withLabelsInputConfiguration(LabelsInputConfiguration labelsInputConfiguration) {
setLabelsInputConfiguration(labelsInputConfiguration);
return this;
}
/**
*
* A unique identifier for the request. If you do not set the client request token, Amazon Lookout for Equipment
* generates one.
*
*
* @param clientToken
* A unique identifier for the request. If you do not set the client request token, Amazon Lookout for
* Equipment generates one.
*/
public void setClientToken(String clientToken) {
this.clientToken = clientToken;
}
/**
*
* A unique identifier for the request. If you do not set the client request token, Amazon Lookout for Equipment
* generates one.
*
*
* @return A unique identifier for the request. If you do not set the client request token, Amazon Lookout for
* Equipment generates one.
*/
public String getClientToken() {
return this.clientToken;
}
/**
*
* A unique identifier for the request. If you do not set the client request token, Amazon Lookout for Equipment
* generates one.
*
*
* @param clientToken
* A unique identifier for the request. If you do not set the client request token, Amazon Lookout for
* Equipment generates one.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateModelRequest withClientToken(String clientToken) {
setClientToken(clientToken);
return this;
}
/**
*
* Indicates the time reference in the dataset that should be used to begin the subset of training data for the
* machine learning model.
*
*
* @param trainingDataStartTime
* Indicates the time reference in the dataset that should be used to begin the subset of training data for
* the machine learning model.
*/
public void setTrainingDataStartTime(java.util.Date trainingDataStartTime) {
this.trainingDataStartTime = trainingDataStartTime;
}
/**
*
* Indicates the time reference in the dataset that should be used to begin the subset of training data for the
* machine learning model.
*
*
* @return Indicates the time reference in the dataset that should be used to begin the subset of training data for
* the machine learning model.
*/
public java.util.Date getTrainingDataStartTime() {
return this.trainingDataStartTime;
}
/**
*
* Indicates the time reference in the dataset that should be used to begin the subset of training data for the
* machine learning model.
*
*
* @param trainingDataStartTime
* Indicates the time reference in the dataset that should be used to begin the subset of training data for
* the machine learning model.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateModelRequest withTrainingDataStartTime(java.util.Date trainingDataStartTime) {
setTrainingDataStartTime(trainingDataStartTime);
return this;
}
/**
*
* Indicates the time reference in the dataset that should be used to end the subset of training data for the
* machine learning model.
*
*
* @param trainingDataEndTime
* Indicates the time reference in the dataset that should be used to end the subset of training data for the
* machine learning model.
*/
public void setTrainingDataEndTime(java.util.Date trainingDataEndTime) {
this.trainingDataEndTime = trainingDataEndTime;
}
/**
*
* Indicates the time reference in the dataset that should be used to end the subset of training data for the
* machine learning model.
*
*
* @return Indicates the time reference in the dataset that should be used to end the subset of training data for
* the machine learning model.
*/
public java.util.Date getTrainingDataEndTime() {
return this.trainingDataEndTime;
}
/**
*
* Indicates the time reference in the dataset that should be used to end the subset of training data for the
* machine learning model.
*
*
* @param trainingDataEndTime
* Indicates the time reference in the dataset that should be used to end the subset of training data for the
* machine learning model.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateModelRequest withTrainingDataEndTime(java.util.Date trainingDataEndTime) {
setTrainingDataEndTime(trainingDataEndTime);
return this;
}
/**
*
* Indicates the time reference in the dataset that should be used to begin the subset of evaluation data for the
* machine learning model.
*
*
* @param evaluationDataStartTime
* Indicates the time reference in the dataset that should be used to begin the subset of evaluation data for
* the machine learning model.
*/
public void setEvaluationDataStartTime(java.util.Date evaluationDataStartTime) {
this.evaluationDataStartTime = evaluationDataStartTime;
}
/**
*
* Indicates the time reference in the dataset that should be used to begin the subset of evaluation data for the
* machine learning model.
*
*
* @return Indicates the time reference in the dataset that should be used to begin the subset of evaluation data
* for the machine learning model.
*/
public java.util.Date getEvaluationDataStartTime() {
return this.evaluationDataStartTime;
}
/**
*
* Indicates the time reference in the dataset that should be used to begin the subset of evaluation data for the
* machine learning model.
*
*
* @param evaluationDataStartTime
* Indicates the time reference in the dataset that should be used to begin the subset of evaluation data for
* the machine learning model.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateModelRequest withEvaluationDataStartTime(java.util.Date evaluationDataStartTime) {
setEvaluationDataStartTime(evaluationDataStartTime);
return this;
}
/**
*
* Indicates the time reference in the dataset that should be used to end the subset of evaluation data for the
* machine learning model.
*
*
* @param evaluationDataEndTime
* Indicates the time reference in the dataset that should be used to end the subset of evaluation data for
* the machine learning model.
*/
public void setEvaluationDataEndTime(java.util.Date evaluationDataEndTime) {
this.evaluationDataEndTime = evaluationDataEndTime;
}
/**
*
* Indicates the time reference in the dataset that should be used to end the subset of evaluation data for the
* machine learning model.
*
*
* @return Indicates the time reference in the dataset that should be used to end the subset of evaluation data for
* the machine learning model.
*/
public java.util.Date getEvaluationDataEndTime() {
return this.evaluationDataEndTime;
}
/**
*
* Indicates the time reference in the dataset that should be used to end the subset of evaluation data for the
* machine learning model.
*
*
* @param evaluationDataEndTime
* Indicates the time reference in the dataset that should be used to end the subset of evaluation data for
* the machine learning model.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateModelRequest withEvaluationDataEndTime(java.util.Date evaluationDataEndTime) {
setEvaluationDataEndTime(evaluationDataEndTime);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of a role with permission to access the data source being used to create the
* machine learning model.
*
*
* @param roleArn
* The Amazon Resource Name (ARN) of a role with permission to access the data source being used to create
* the machine learning model.
*/
public void setRoleArn(String roleArn) {
this.roleArn = roleArn;
}
/**
*
* The Amazon Resource Name (ARN) of a role with permission to access the data source being used to create the
* machine learning model.
*
*
* @return The Amazon Resource Name (ARN) of a role with permission to access the data source being used to create
* the machine learning model.
*/
public String getRoleArn() {
return this.roleArn;
}
/**
*
* The Amazon Resource Name (ARN) of a role with permission to access the data source being used to create the
* machine learning model.
*
*
* @param roleArn
* The Amazon Resource Name (ARN) of a role with permission to access the data source being used to create
* the machine learning model.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateModelRequest withRoleArn(String roleArn) {
setRoleArn(roleArn);
return this;
}
/**
*
* The configuration is the TargetSamplingRate
, which is the sampling rate of the data after post
* processing by Amazon Lookout for Equipment. For example, if you provide data that has been collected at a 1
* second level and you want the system to resample the data at a 1 minute rate before training, the
* TargetSamplingRate
is 1 minute.
*
*
* When providing a value for the TargetSamplingRate
, you must attach the prefix "PT" to the rate you
* want. The value for a 1 second rate is therefore PT1S, the value for a 15 minute rate is PT15M, and
* the value for a 1 hour rate is PT1H
*
*
* @param dataPreProcessingConfiguration
* The configuration is the TargetSamplingRate
, which is the sampling rate of the data after
* post processing by Amazon Lookout for Equipment. For example, if you provide data that has been collected
* at a 1 second level and you want the system to resample the data at a 1 minute rate before training, the
* TargetSamplingRate
is 1 minute.
*
* When providing a value for the TargetSamplingRate
, you must attach the prefix "PT" to the
* rate you want. The value for a 1 second rate is therefore PT1S, the value for a 15 minute rate is
* PT15M, and the value for a 1 hour rate is PT1H
*/
public void setDataPreProcessingConfiguration(DataPreProcessingConfiguration dataPreProcessingConfiguration) {
this.dataPreProcessingConfiguration = dataPreProcessingConfiguration;
}
/**
*
* The configuration is the TargetSamplingRate
, which is the sampling rate of the data after post
* processing by Amazon Lookout for Equipment. For example, if you provide data that has been collected at a 1
* second level and you want the system to resample the data at a 1 minute rate before training, the
* TargetSamplingRate
is 1 minute.
*
*
* When providing a value for the TargetSamplingRate
, you must attach the prefix "PT" to the rate you
* want. The value for a 1 second rate is therefore PT1S, the value for a 15 minute rate is PT15M, and
* the value for a 1 hour rate is PT1H
*
*
* @return The configuration is the TargetSamplingRate
, which is the sampling rate of the data after
* post processing by Amazon Lookout for Equipment. For example, if you provide data that has been collected
* at a 1 second level and you want the system to resample the data at a 1 minute rate before training, the
* TargetSamplingRate
is 1 minute.
*
* When providing a value for the TargetSamplingRate
, you must attach the prefix "PT" to the
* rate you want. The value for a 1 second rate is therefore PT1S, the value for a 15 minute rate is
* PT15M, and the value for a 1 hour rate is PT1H
*/
public DataPreProcessingConfiguration getDataPreProcessingConfiguration() {
return this.dataPreProcessingConfiguration;
}
/**
*
* The configuration is the TargetSamplingRate
, which is the sampling rate of the data after post
* processing by Amazon Lookout for Equipment. For example, if you provide data that has been collected at a 1
* second level and you want the system to resample the data at a 1 minute rate before training, the
* TargetSamplingRate
is 1 minute.
*
*
* When providing a value for the TargetSamplingRate
, you must attach the prefix "PT" to the rate you
* want. The value for a 1 second rate is therefore PT1S, the value for a 15 minute rate is PT15M, and
* the value for a 1 hour rate is PT1H
*
*
* @param dataPreProcessingConfiguration
* The configuration is the TargetSamplingRate
, which is the sampling rate of the data after
* post processing by Amazon Lookout for Equipment. For example, if you provide data that has been collected
* at a 1 second level and you want the system to resample the data at a 1 minute rate before training, the
* TargetSamplingRate
is 1 minute.
*
* When providing a value for the TargetSamplingRate
, you must attach the prefix "PT" to the
* rate you want. The value for a 1 second rate is therefore PT1S, the value for a 15 minute rate is
* PT15M, and the value for a 1 hour rate is PT1H
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateModelRequest withDataPreProcessingConfiguration(DataPreProcessingConfiguration dataPreProcessingConfiguration) {
setDataPreProcessingConfiguration(dataPreProcessingConfiguration);
return this;
}
/**
*
* Provides the identifier of the KMS key used to encrypt model data by Amazon Lookout for Equipment.
*
*
* @param serverSideKmsKeyId
* Provides the identifier of the KMS key used to encrypt model data by Amazon Lookout for Equipment.
*/
public void setServerSideKmsKeyId(String serverSideKmsKeyId) {
this.serverSideKmsKeyId = serverSideKmsKeyId;
}
/**
*
* Provides the identifier of the KMS key used to encrypt model data by Amazon Lookout for Equipment.
*
*
* @return Provides the identifier of the KMS key used to encrypt model data by Amazon Lookout for Equipment.
*/
public String getServerSideKmsKeyId() {
return this.serverSideKmsKeyId;
}
/**
*
* Provides the identifier of the KMS key used to encrypt model data by Amazon Lookout for Equipment.
*
*
* @param serverSideKmsKeyId
* Provides the identifier of the KMS key used to encrypt model data by Amazon Lookout for Equipment.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateModelRequest withServerSideKmsKeyId(String serverSideKmsKeyId) {
setServerSideKmsKeyId(serverSideKmsKeyId);
return this;
}
/**
*
* Any tags associated with the machine learning model being created.
*
*
* @return Any tags associated with the machine learning model being created.
*/
public java.util.List getTags() {
return tags;
}
/**
*
* Any tags associated with the machine learning model being created.
*
*
* @param tags
* Any tags associated with the machine learning model being created.
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new java.util.ArrayList(tags);
}
/**
*
* Any tags associated with the machine learning model being created.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* Any tags associated with the machine learning model being created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateModelRequest withTags(Tag... tags) {
if (this.tags == null) {
setTags(new java.util.ArrayList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* Any tags associated with the machine learning model being created.
*
*
* @param tags
* Any tags associated with the machine learning model being created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateModelRequest withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
*
* Indicates that the asset associated with this sensor has been shut off. As long as this condition is met, Lookout
* for Equipment will not use data from this asset for training, evaluation, or inference.
*
*
* @param offCondition
* Indicates that the asset associated with this sensor has been shut off. As long as this condition is met,
* Lookout for Equipment will not use data from this asset for training, evaluation, or inference.
*/
public void setOffCondition(String offCondition) {
this.offCondition = offCondition;
}
/**
*
* Indicates that the asset associated with this sensor has been shut off. As long as this condition is met, Lookout
* for Equipment will not use data from this asset for training, evaluation, or inference.
*
*
* @return Indicates that the asset associated with this sensor has been shut off. As long as this condition is met,
* Lookout for Equipment will not use data from this asset for training, evaluation, or inference.
*/
public String getOffCondition() {
return this.offCondition;
}
/**
*
* Indicates that the asset associated with this sensor has been shut off. As long as this condition is met, Lookout
* for Equipment will not use data from this asset for training, evaluation, or inference.
*
*
* @param offCondition
* Indicates that the asset associated with this sensor has been shut off. As long as this condition is met,
* Lookout for Equipment will not use data from this asset for training, evaluation, or inference.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateModelRequest withOffCondition(String offCondition) {
setOffCondition(offCondition);
return this;
}
/**
*
* The Amazon S3 location where you want Amazon Lookout for Equipment to save the pointwise model diagnostics. You
* must also specify the RoleArn
request parameter.
*
*
* @param modelDiagnosticsOutputConfiguration
* The Amazon S3 location where you want Amazon Lookout for Equipment to save the pointwise model
* diagnostics. You must also specify the RoleArn
request parameter.
*/
public void setModelDiagnosticsOutputConfiguration(ModelDiagnosticsOutputConfiguration modelDiagnosticsOutputConfiguration) {
this.modelDiagnosticsOutputConfiguration = modelDiagnosticsOutputConfiguration;
}
/**
*
* The Amazon S3 location where you want Amazon Lookout for Equipment to save the pointwise model diagnostics. You
* must also specify the RoleArn
request parameter.
*
*
* @return The Amazon S3 location where you want Amazon Lookout for Equipment to save the pointwise model
* diagnostics. You must also specify the RoleArn
request parameter.
*/
public ModelDiagnosticsOutputConfiguration getModelDiagnosticsOutputConfiguration() {
return this.modelDiagnosticsOutputConfiguration;
}
/**
*
* The Amazon S3 location where you want Amazon Lookout for Equipment to save the pointwise model diagnostics. You
* must also specify the RoleArn
request parameter.
*
*
* @param modelDiagnosticsOutputConfiguration
* The Amazon S3 location where you want Amazon Lookout for Equipment to save the pointwise model
* diagnostics. You must also specify the RoleArn
request parameter.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateModelRequest withModelDiagnosticsOutputConfiguration(ModelDiagnosticsOutputConfiguration modelDiagnosticsOutputConfiguration) {
setModelDiagnosticsOutputConfiguration(modelDiagnosticsOutputConfiguration);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getModelName() != null)
sb.append("ModelName: ").append(getModelName()).append(",");
if (getDatasetName() != null)
sb.append("DatasetName: ").append(getDatasetName()).append(",");
if (getDatasetSchema() != null)
sb.append("DatasetSchema: ").append(getDatasetSchema()).append(",");
if (getLabelsInputConfiguration() != null)
sb.append("LabelsInputConfiguration: ").append(getLabelsInputConfiguration()).append(",");
if (getClientToken() != null)
sb.append("ClientToken: ").append(getClientToken()).append(",");
if (getTrainingDataStartTime() != null)
sb.append("TrainingDataStartTime: ").append(getTrainingDataStartTime()).append(",");
if (getTrainingDataEndTime() != null)
sb.append("TrainingDataEndTime: ").append(getTrainingDataEndTime()).append(",");
if (getEvaluationDataStartTime() != null)
sb.append("EvaluationDataStartTime: ").append(getEvaluationDataStartTime()).append(",");
if (getEvaluationDataEndTime() != null)
sb.append("EvaluationDataEndTime: ").append(getEvaluationDataEndTime()).append(",");
if (getRoleArn() != null)
sb.append("RoleArn: ").append(getRoleArn()).append(",");
if (getDataPreProcessingConfiguration() != null)
sb.append("DataPreProcessingConfiguration: ").append(getDataPreProcessingConfiguration()).append(",");
if (getServerSideKmsKeyId() != null)
sb.append("ServerSideKmsKeyId: ").append(getServerSideKmsKeyId()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags()).append(",");
if (getOffCondition() != null)
sb.append("OffCondition: ").append(getOffCondition()).append(",");
if (getModelDiagnosticsOutputConfiguration() != null)
sb.append("ModelDiagnosticsOutputConfiguration: ").append(getModelDiagnosticsOutputConfiguration());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateModelRequest == false)
return false;
CreateModelRequest other = (CreateModelRequest) obj;
if (other.getModelName() == null ^ this.getModelName() == null)
return false;
if (other.getModelName() != null && other.getModelName().equals(this.getModelName()) == false)
return false;
if (other.getDatasetName() == null ^ this.getDatasetName() == null)
return false;
if (other.getDatasetName() != null && other.getDatasetName().equals(this.getDatasetName()) == false)
return false;
if (other.getDatasetSchema() == null ^ this.getDatasetSchema() == null)
return false;
if (other.getDatasetSchema() != null && other.getDatasetSchema().equals(this.getDatasetSchema()) == false)
return false;
if (other.getLabelsInputConfiguration() == null ^ this.getLabelsInputConfiguration() == null)
return false;
if (other.getLabelsInputConfiguration() != null && other.getLabelsInputConfiguration().equals(this.getLabelsInputConfiguration()) == false)
return false;
if (other.getClientToken() == null ^ this.getClientToken() == null)
return false;
if (other.getClientToken() != null && other.getClientToken().equals(this.getClientToken()) == false)
return false;
if (other.getTrainingDataStartTime() == null ^ this.getTrainingDataStartTime() == null)
return false;
if (other.getTrainingDataStartTime() != null && other.getTrainingDataStartTime().equals(this.getTrainingDataStartTime()) == false)
return false;
if (other.getTrainingDataEndTime() == null ^ this.getTrainingDataEndTime() == null)
return false;
if (other.getTrainingDataEndTime() != null && other.getTrainingDataEndTime().equals(this.getTrainingDataEndTime()) == false)
return false;
if (other.getEvaluationDataStartTime() == null ^ this.getEvaluationDataStartTime() == null)
return false;
if (other.getEvaluationDataStartTime() != null && other.getEvaluationDataStartTime().equals(this.getEvaluationDataStartTime()) == false)
return false;
if (other.getEvaluationDataEndTime() == null ^ this.getEvaluationDataEndTime() == null)
return false;
if (other.getEvaluationDataEndTime() != null && other.getEvaluationDataEndTime().equals(this.getEvaluationDataEndTime()) == false)
return false;
if (other.getRoleArn() == null ^ this.getRoleArn() == null)
return false;
if (other.getRoleArn() != null && other.getRoleArn().equals(this.getRoleArn()) == false)
return false;
if (other.getDataPreProcessingConfiguration() == null ^ this.getDataPreProcessingConfiguration() == null)
return false;
if (other.getDataPreProcessingConfiguration() != null
&& other.getDataPreProcessingConfiguration().equals(this.getDataPreProcessingConfiguration()) == false)
return false;
if (other.getServerSideKmsKeyId() == null ^ this.getServerSideKmsKeyId() == null)
return false;
if (other.getServerSideKmsKeyId() != null && other.getServerSideKmsKeyId().equals(this.getServerSideKmsKeyId()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
if (other.getOffCondition() == null ^ this.getOffCondition() == null)
return false;
if (other.getOffCondition() != null && other.getOffCondition().equals(this.getOffCondition()) == false)
return false;
if (other.getModelDiagnosticsOutputConfiguration() == null ^ this.getModelDiagnosticsOutputConfiguration() == null)
return false;
if (other.getModelDiagnosticsOutputConfiguration() != null
&& other.getModelDiagnosticsOutputConfiguration().equals(this.getModelDiagnosticsOutputConfiguration()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getModelName() == null) ? 0 : getModelName().hashCode());
hashCode = prime * hashCode + ((getDatasetName() == null) ? 0 : getDatasetName().hashCode());
hashCode = prime * hashCode + ((getDatasetSchema() == null) ? 0 : getDatasetSchema().hashCode());
hashCode = prime * hashCode + ((getLabelsInputConfiguration() == null) ? 0 : getLabelsInputConfiguration().hashCode());
hashCode = prime * hashCode + ((getClientToken() == null) ? 0 : getClientToken().hashCode());
hashCode = prime * hashCode + ((getTrainingDataStartTime() == null) ? 0 : getTrainingDataStartTime().hashCode());
hashCode = prime * hashCode + ((getTrainingDataEndTime() == null) ? 0 : getTrainingDataEndTime().hashCode());
hashCode = prime * hashCode + ((getEvaluationDataStartTime() == null) ? 0 : getEvaluationDataStartTime().hashCode());
hashCode = prime * hashCode + ((getEvaluationDataEndTime() == null) ? 0 : getEvaluationDataEndTime().hashCode());
hashCode = prime * hashCode + ((getRoleArn() == null) ? 0 : getRoleArn().hashCode());
hashCode = prime * hashCode + ((getDataPreProcessingConfiguration() == null) ? 0 : getDataPreProcessingConfiguration().hashCode());
hashCode = prime * hashCode + ((getServerSideKmsKeyId() == null) ? 0 : getServerSideKmsKeyId().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
hashCode = prime * hashCode + ((getOffCondition() == null) ? 0 : getOffCondition().hashCode());
hashCode = prime * hashCode + ((getModelDiagnosticsOutputConfiguration() == null) ? 0 : getModelDiagnosticsOutputConfiguration().hashCode());
return hashCode;
}
@Override
public CreateModelRequest clone() {
return (CreateModelRequest) super.clone();
}
}