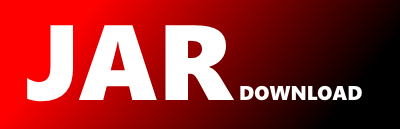
com.amazonaws.services.lookoutequipment.model.DescribeDatasetResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-lookoutequipment Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.lookoutequipment.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class DescribeDatasetResult extends com.amazonaws.AmazonWebServiceResult implements Serializable, Cloneable {
/**
*
* The name of the dataset being described.
*
*/
private String datasetName;
/**
*
* The Amazon Resource Name (ARN) of the dataset being described.
*
*/
private String datasetArn;
/**
*
* Specifies the time the dataset was created in Lookout for Equipment.
*
*/
private java.util.Date createdAt;
/**
*
* Specifies the time the dataset was last updated, if it was.
*
*/
private java.util.Date lastUpdatedAt;
/**
*
* Indicates the status of the dataset.
*
*/
private String status;
/**
*
* A JSON description of the data that is in each time series dataset, including names, column names, and data
* types.
*
*/
private String schema;
/**
*
* Provides the identifier of the KMS key used to encrypt dataset data by Amazon Lookout for Equipment.
*
*/
private String serverSideKmsKeyId;
/**
*
* Specifies the S3 location configuration for the data input for the data ingestion job.
*
*/
private IngestionInputConfiguration ingestionInputConfiguration;
/**
*
* Gives statistics associated with the given dataset for the latest successful associated ingestion job id. These
* statistics primarily relate to quantifying incorrect data such as MissingCompleteSensorData, MissingSensorData,
* UnsupportedDateFormats, InsufficientSensorData, and DuplicateTimeStamps.
*
*/
private DataQualitySummary dataQualitySummary;
/**
*
* IngestedFilesSummary associated with the given dataset for the latest successful associated ingestion job id.
*
*/
private IngestedFilesSummary ingestedFilesSummary;
/**
*
* The Amazon Resource Name (ARN) of the IAM role that you are using for this the data ingestion job.
*
*/
private String roleArn;
/**
*
* Indicates the earliest timestamp corresponding to data that was successfully ingested during the most recent
* ingestion of this particular dataset.
*
*/
private java.util.Date dataStartTime;
/**
*
* Indicates the latest timestamp corresponding to data that was successfully ingested during the most recent
* ingestion of this particular dataset.
*
*/
private java.util.Date dataEndTime;
/**
*
* The Amazon Resource Name (ARN) of the source dataset from which the current data being described was imported
* from.
*
*/
private String sourceDatasetArn;
/**
*
* The name of the dataset being described.
*
*
* @param datasetName
* The name of the dataset being described.
*/
public void setDatasetName(String datasetName) {
this.datasetName = datasetName;
}
/**
*
* The name of the dataset being described.
*
*
* @return The name of the dataset being described.
*/
public String getDatasetName() {
return this.datasetName;
}
/**
*
* The name of the dataset being described.
*
*
* @param datasetName
* The name of the dataset being described.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeDatasetResult withDatasetName(String datasetName) {
setDatasetName(datasetName);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the dataset being described.
*
*
* @param datasetArn
* The Amazon Resource Name (ARN) of the dataset being described.
*/
public void setDatasetArn(String datasetArn) {
this.datasetArn = datasetArn;
}
/**
*
* The Amazon Resource Name (ARN) of the dataset being described.
*
*
* @return The Amazon Resource Name (ARN) of the dataset being described.
*/
public String getDatasetArn() {
return this.datasetArn;
}
/**
*
* The Amazon Resource Name (ARN) of the dataset being described.
*
*
* @param datasetArn
* The Amazon Resource Name (ARN) of the dataset being described.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeDatasetResult withDatasetArn(String datasetArn) {
setDatasetArn(datasetArn);
return this;
}
/**
*
* Specifies the time the dataset was created in Lookout for Equipment.
*
*
* @param createdAt
* Specifies the time the dataset was created in Lookout for Equipment.
*/
public void setCreatedAt(java.util.Date createdAt) {
this.createdAt = createdAt;
}
/**
*
* Specifies the time the dataset was created in Lookout for Equipment.
*
*
* @return Specifies the time the dataset was created in Lookout for Equipment.
*/
public java.util.Date getCreatedAt() {
return this.createdAt;
}
/**
*
* Specifies the time the dataset was created in Lookout for Equipment.
*
*
* @param createdAt
* Specifies the time the dataset was created in Lookout for Equipment.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeDatasetResult withCreatedAt(java.util.Date createdAt) {
setCreatedAt(createdAt);
return this;
}
/**
*
* Specifies the time the dataset was last updated, if it was.
*
*
* @param lastUpdatedAt
* Specifies the time the dataset was last updated, if it was.
*/
public void setLastUpdatedAt(java.util.Date lastUpdatedAt) {
this.lastUpdatedAt = lastUpdatedAt;
}
/**
*
* Specifies the time the dataset was last updated, if it was.
*
*
* @return Specifies the time the dataset was last updated, if it was.
*/
public java.util.Date getLastUpdatedAt() {
return this.lastUpdatedAt;
}
/**
*
* Specifies the time the dataset was last updated, if it was.
*
*
* @param lastUpdatedAt
* Specifies the time the dataset was last updated, if it was.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeDatasetResult withLastUpdatedAt(java.util.Date lastUpdatedAt) {
setLastUpdatedAt(lastUpdatedAt);
return this;
}
/**
*
* Indicates the status of the dataset.
*
*
* @param status
* Indicates the status of the dataset.
* @see DatasetStatus
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* Indicates the status of the dataset.
*
*
* @return Indicates the status of the dataset.
* @see DatasetStatus
*/
public String getStatus() {
return this.status;
}
/**
*
* Indicates the status of the dataset.
*
*
* @param status
* Indicates the status of the dataset.
* @return Returns a reference to this object so that method calls can be chained together.
* @see DatasetStatus
*/
public DescribeDatasetResult withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* Indicates the status of the dataset.
*
*
* @param status
* Indicates the status of the dataset.
* @return Returns a reference to this object so that method calls can be chained together.
* @see DatasetStatus
*/
public DescribeDatasetResult withStatus(DatasetStatus status) {
this.status = status.toString();
return this;
}
/**
*
* A JSON description of the data that is in each time series dataset, including names, column names, and data
* types.
*
*
* This field's value must be valid JSON according to RFC 7159, including the opening and closing braces. For
* example: '{"key": "value"}'.
*
*
* The AWS SDK for Java performs a Base64 encoding on this field before sending this request to the AWS service.
* Users of the SDK should not perform Base64 encoding on this field.
*
*
* @param schema
* A JSON description of the data that is in each time series dataset, including names, column names, and
* data types.
*/
public void setSchema(String schema) {
this.schema = schema;
}
/**
*
* A JSON description of the data that is in each time series dataset, including names, column names, and data
* types.
*
*
* This field's value will be valid JSON according to RFC 7159, including the opening and closing braces. For
* example: '{"key": "value"}'.
*
*
* @return A JSON description of the data that is in each time series dataset, including names, column names, and
* data types.
*/
public String getSchema() {
return this.schema;
}
/**
*
* A JSON description of the data that is in each time series dataset, including names, column names, and data
* types.
*
*
* This field's value must be valid JSON according to RFC 7159, including the opening and closing braces. For
* example: '{"key": "value"}'.
*
*
* The AWS SDK for Java performs a Base64 encoding on this field before sending this request to the AWS service.
* Users of the SDK should not perform Base64 encoding on this field.
*
*
* @param schema
* A JSON description of the data that is in each time series dataset, including names, column names, and
* data types.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeDatasetResult withSchema(String schema) {
setSchema(schema);
return this;
}
/**
*
* Provides the identifier of the KMS key used to encrypt dataset data by Amazon Lookout for Equipment.
*
*
* @param serverSideKmsKeyId
* Provides the identifier of the KMS key used to encrypt dataset data by Amazon Lookout for Equipment.
*/
public void setServerSideKmsKeyId(String serverSideKmsKeyId) {
this.serverSideKmsKeyId = serverSideKmsKeyId;
}
/**
*
* Provides the identifier of the KMS key used to encrypt dataset data by Amazon Lookout for Equipment.
*
*
* @return Provides the identifier of the KMS key used to encrypt dataset data by Amazon Lookout for Equipment.
*/
public String getServerSideKmsKeyId() {
return this.serverSideKmsKeyId;
}
/**
*
* Provides the identifier of the KMS key used to encrypt dataset data by Amazon Lookout for Equipment.
*
*
* @param serverSideKmsKeyId
* Provides the identifier of the KMS key used to encrypt dataset data by Amazon Lookout for Equipment.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeDatasetResult withServerSideKmsKeyId(String serverSideKmsKeyId) {
setServerSideKmsKeyId(serverSideKmsKeyId);
return this;
}
/**
*
* Specifies the S3 location configuration for the data input for the data ingestion job.
*
*
* @param ingestionInputConfiguration
* Specifies the S3 location configuration for the data input for the data ingestion job.
*/
public void setIngestionInputConfiguration(IngestionInputConfiguration ingestionInputConfiguration) {
this.ingestionInputConfiguration = ingestionInputConfiguration;
}
/**
*
* Specifies the S3 location configuration for the data input for the data ingestion job.
*
*
* @return Specifies the S3 location configuration for the data input for the data ingestion job.
*/
public IngestionInputConfiguration getIngestionInputConfiguration() {
return this.ingestionInputConfiguration;
}
/**
*
* Specifies the S3 location configuration for the data input for the data ingestion job.
*
*
* @param ingestionInputConfiguration
* Specifies the S3 location configuration for the data input for the data ingestion job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeDatasetResult withIngestionInputConfiguration(IngestionInputConfiguration ingestionInputConfiguration) {
setIngestionInputConfiguration(ingestionInputConfiguration);
return this;
}
/**
*
* Gives statistics associated with the given dataset for the latest successful associated ingestion job id. These
* statistics primarily relate to quantifying incorrect data such as MissingCompleteSensorData, MissingSensorData,
* UnsupportedDateFormats, InsufficientSensorData, and DuplicateTimeStamps.
*
*
* @param dataQualitySummary
* Gives statistics associated with the given dataset for the latest successful associated ingestion job id.
* These statistics primarily relate to quantifying incorrect data such as MissingCompleteSensorData,
* MissingSensorData, UnsupportedDateFormats, InsufficientSensorData, and DuplicateTimeStamps.
*/
public void setDataQualitySummary(DataQualitySummary dataQualitySummary) {
this.dataQualitySummary = dataQualitySummary;
}
/**
*
* Gives statistics associated with the given dataset for the latest successful associated ingestion job id. These
* statistics primarily relate to quantifying incorrect data such as MissingCompleteSensorData, MissingSensorData,
* UnsupportedDateFormats, InsufficientSensorData, and DuplicateTimeStamps.
*
*
* @return Gives statistics associated with the given dataset for the latest successful associated ingestion job id.
* These statistics primarily relate to quantifying incorrect data such as MissingCompleteSensorData,
* MissingSensorData, UnsupportedDateFormats, InsufficientSensorData, and DuplicateTimeStamps.
*/
public DataQualitySummary getDataQualitySummary() {
return this.dataQualitySummary;
}
/**
*
* Gives statistics associated with the given dataset for the latest successful associated ingestion job id. These
* statistics primarily relate to quantifying incorrect data such as MissingCompleteSensorData, MissingSensorData,
* UnsupportedDateFormats, InsufficientSensorData, and DuplicateTimeStamps.
*
*
* @param dataQualitySummary
* Gives statistics associated with the given dataset for the latest successful associated ingestion job id.
* These statistics primarily relate to quantifying incorrect data such as MissingCompleteSensorData,
* MissingSensorData, UnsupportedDateFormats, InsufficientSensorData, and DuplicateTimeStamps.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeDatasetResult withDataQualitySummary(DataQualitySummary dataQualitySummary) {
setDataQualitySummary(dataQualitySummary);
return this;
}
/**
*
* IngestedFilesSummary associated with the given dataset for the latest successful associated ingestion job id.
*
*
* @param ingestedFilesSummary
* IngestedFilesSummary associated with the given dataset for the latest successful associated ingestion job
* id.
*/
public void setIngestedFilesSummary(IngestedFilesSummary ingestedFilesSummary) {
this.ingestedFilesSummary = ingestedFilesSummary;
}
/**
*
* IngestedFilesSummary associated with the given dataset for the latest successful associated ingestion job id.
*
*
* @return IngestedFilesSummary associated with the given dataset for the latest successful associated ingestion job
* id.
*/
public IngestedFilesSummary getIngestedFilesSummary() {
return this.ingestedFilesSummary;
}
/**
*
* IngestedFilesSummary associated with the given dataset for the latest successful associated ingestion job id.
*
*
* @param ingestedFilesSummary
* IngestedFilesSummary associated with the given dataset for the latest successful associated ingestion job
* id.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeDatasetResult withIngestedFilesSummary(IngestedFilesSummary ingestedFilesSummary) {
setIngestedFilesSummary(ingestedFilesSummary);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the IAM role that you are using for this the data ingestion job.
*
*
* @param roleArn
* The Amazon Resource Name (ARN) of the IAM role that you are using for this the data ingestion job.
*/
public void setRoleArn(String roleArn) {
this.roleArn = roleArn;
}
/**
*
* The Amazon Resource Name (ARN) of the IAM role that you are using for this the data ingestion job.
*
*
* @return The Amazon Resource Name (ARN) of the IAM role that you are using for this the data ingestion job.
*/
public String getRoleArn() {
return this.roleArn;
}
/**
*
* The Amazon Resource Name (ARN) of the IAM role that you are using for this the data ingestion job.
*
*
* @param roleArn
* The Amazon Resource Name (ARN) of the IAM role that you are using for this the data ingestion job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeDatasetResult withRoleArn(String roleArn) {
setRoleArn(roleArn);
return this;
}
/**
*
* Indicates the earliest timestamp corresponding to data that was successfully ingested during the most recent
* ingestion of this particular dataset.
*
*
* @param dataStartTime
* Indicates the earliest timestamp corresponding to data that was successfully ingested during the most
* recent ingestion of this particular dataset.
*/
public void setDataStartTime(java.util.Date dataStartTime) {
this.dataStartTime = dataStartTime;
}
/**
*
* Indicates the earliest timestamp corresponding to data that was successfully ingested during the most recent
* ingestion of this particular dataset.
*
*
* @return Indicates the earliest timestamp corresponding to data that was successfully ingested during the most
* recent ingestion of this particular dataset.
*/
public java.util.Date getDataStartTime() {
return this.dataStartTime;
}
/**
*
* Indicates the earliest timestamp corresponding to data that was successfully ingested during the most recent
* ingestion of this particular dataset.
*
*
* @param dataStartTime
* Indicates the earliest timestamp corresponding to data that was successfully ingested during the most
* recent ingestion of this particular dataset.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeDatasetResult withDataStartTime(java.util.Date dataStartTime) {
setDataStartTime(dataStartTime);
return this;
}
/**
*
* Indicates the latest timestamp corresponding to data that was successfully ingested during the most recent
* ingestion of this particular dataset.
*
*
* @param dataEndTime
* Indicates the latest timestamp corresponding to data that was successfully ingested during the most recent
* ingestion of this particular dataset.
*/
public void setDataEndTime(java.util.Date dataEndTime) {
this.dataEndTime = dataEndTime;
}
/**
*
* Indicates the latest timestamp corresponding to data that was successfully ingested during the most recent
* ingestion of this particular dataset.
*
*
* @return Indicates the latest timestamp corresponding to data that was successfully ingested during the most
* recent ingestion of this particular dataset.
*/
public java.util.Date getDataEndTime() {
return this.dataEndTime;
}
/**
*
* Indicates the latest timestamp corresponding to data that was successfully ingested during the most recent
* ingestion of this particular dataset.
*
*
* @param dataEndTime
* Indicates the latest timestamp corresponding to data that was successfully ingested during the most recent
* ingestion of this particular dataset.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeDatasetResult withDataEndTime(java.util.Date dataEndTime) {
setDataEndTime(dataEndTime);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the source dataset from which the current data being described was imported
* from.
*
*
* @param sourceDatasetArn
* The Amazon Resource Name (ARN) of the source dataset from which the current data being described was
* imported from.
*/
public void setSourceDatasetArn(String sourceDatasetArn) {
this.sourceDatasetArn = sourceDatasetArn;
}
/**
*
* The Amazon Resource Name (ARN) of the source dataset from which the current data being described was imported
* from.
*
*
* @return The Amazon Resource Name (ARN) of the source dataset from which the current data being described was
* imported from.
*/
public String getSourceDatasetArn() {
return this.sourceDatasetArn;
}
/**
*
* The Amazon Resource Name (ARN) of the source dataset from which the current data being described was imported
* from.
*
*
* @param sourceDatasetArn
* The Amazon Resource Name (ARN) of the source dataset from which the current data being described was
* imported from.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeDatasetResult withSourceDatasetArn(String sourceDatasetArn) {
setSourceDatasetArn(sourceDatasetArn);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getDatasetName() != null)
sb.append("DatasetName: ").append(getDatasetName()).append(",");
if (getDatasetArn() != null)
sb.append("DatasetArn: ").append(getDatasetArn()).append(",");
if (getCreatedAt() != null)
sb.append("CreatedAt: ").append(getCreatedAt()).append(",");
if (getLastUpdatedAt() != null)
sb.append("LastUpdatedAt: ").append(getLastUpdatedAt()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getSchema() != null)
sb.append("Schema: ").append(getSchema()).append(",");
if (getServerSideKmsKeyId() != null)
sb.append("ServerSideKmsKeyId: ").append(getServerSideKmsKeyId()).append(",");
if (getIngestionInputConfiguration() != null)
sb.append("IngestionInputConfiguration: ").append(getIngestionInputConfiguration()).append(",");
if (getDataQualitySummary() != null)
sb.append("DataQualitySummary: ").append(getDataQualitySummary()).append(",");
if (getIngestedFilesSummary() != null)
sb.append("IngestedFilesSummary: ").append(getIngestedFilesSummary()).append(",");
if (getRoleArn() != null)
sb.append("RoleArn: ").append(getRoleArn()).append(",");
if (getDataStartTime() != null)
sb.append("DataStartTime: ").append(getDataStartTime()).append(",");
if (getDataEndTime() != null)
sb.append("DataEndTime: ").append(getDataEndTime()).append(",");
if (getSourceDatasetArn() != null)
sb.append("SourceDatasetArn: ").append(getSourceDatasetArn());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof DescribeDatasetResult == false)
return false;
DescribeDatasetResult other = (DescribeDatasetResult) obj;
if (other.getDatasetName() == null ^ this.getDatasetName() == null)
return false;
if (other.getDatasetName() != null && other.getDatasetName().equals(this.getDatasetName()) == false)
return false;
if (other.getDatasetArn() == null ^ this.getDatasetArn() == null)
return false;
if (other.getDatasetArn() != null && other.getDatasetArn().equals(this.getDatasetArn()) == false)
return false;
if (other.getCreatedAt() == null ^ this.getCreatedAt() == null)
return false;
if (other.getCreatedAt() != null && other.getCreatedAt().equals(this.getCreatedAt()) == false)
return false;
if (other.getLastUpdatedAt() == null ^ this.getLastUpdatedAt() == null)
return false;
if (other.getLastUpdatedAt() != null && other.getLastUpdatedAt().equals(this.getLastUpdatedAt()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getSchema() == null ^ this.getSchema() == null)
return false;
if (other.getSchema() != null && other.getSchema().equals(this.getSchema()) == false)
return false;
if (other.getServerSideKmsKeyId() == null ^ this.getServerSideKmsKeyId() == null)
return false;
if (other.getServerSideKmsKeyId() != null && other.getServerSideKmsKeyId().equals(this.getServerSideKmsKeyId()) == false)
return false;
if (other.getIngestionInputConfiguration() == null ^ this.getIngestionInputConfiguration() == null)
return false;
if (other.getIngestionInputConfiguration() != null && other.getIngestionInputConfiguration().equals(this.getIngestionInputConfiguration()) == false)
return false;
if (other.getDataQualitySummary() == null ^ this.getDataQualitySummary() == null)
return false;
if (other.getDataQualitySummary() != null && other.getDataQualitySummary().equals(this.getDataQualitySummary()) == false)
return false;
if (other.getIngestedFilesSummary() == null ^ this.getIngestedFilesSummary() == null)
return false;
if (other.getIngestedFilesSummary() != null && other.getIngestedFilesSummary().equals(this.getIngestedFilesSummary()) == false)
return false;
if (other.getRoleArn() == null ^ this.getRoleArn() == null)
return false;
if (other.getRoleArn() != null && other.getRoleArn().equals(this.getRoleArn()) == false)
return false;
if (other.getDataStartTime() == null ^ this.getDataStartTime() == null)
return false;
if (other.getDataStartTime() != null && other.getDataStartTime().equals(this.getDataStartTime()) == false)
return false;
if (other.getDataEndTime() == null ^ this.getDataEndTime() == null)
return false;
if (other.getDataEndTime() != null && other.getDataEndTime().equals(this.getDataEndTime()) == false)
return false;
if (other.getSourceDatasetArn() == null ^ this.getSourceDatasetArn() == null)
return false;
if (other.getSourceDatasetArn() != null && other.getSourceDatasetArn().equals(this.getSourceDatasetArn()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getDatasetName() == null) ? 0 : getDatasetName().hashCode());
hashCode = prime * hashCode + ((getDatasetArn() == null) ? 0 : getDatasetArn().hashCode());
hashCode = prime * hashCode + ((getCreatedAt() == null) ? 0 : getCreatedAt().hashCode());
hashCode = prime * hashCode + ((getLastUpdatedAt() == null) ? 0 : getLastUpdatedAt().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getSchema() == null) ? 0 : getSchema().hashCode());
hashCode = prime * hashCode + ((getServerSideKmsKeyId() == null) ? 0 : getServerSideKmsKeyId().hashCode());
hashCode = prime * hashCode + ((getIngestionInputConfiguration() == null) ? 0 : getIngestionInputConfiguration().hashCode());
hashCode = prime * hashCode + ((getDataQualitySummary() == null) ? 0 : getDataQualitySummary().hashCode());
hashCode = prime * hashCode + ((getIngestedFilesSummary() == null) ? 0 : getIngestedFilesSummary().hashCode());
hashCode = prime * hashCode + ((getRoleArn() == null) ? 0 : getRoleArn().hashCode());
hashCode = prime * hashCode + ((getDataStartTime() == null) ? 0 : getDataStartTime().hashCode());
hashCode = prime * hashCode + ((getDataEndTime() == null) ? 0 : getDataEndTime().hashCode());
hashCode = prime * hashCode + ((getSourceDatasetArn() == null) ? 0 : getSourceDatasetArn().hashCode());
return hashCode;
}
@Override
public DescribeDatasetResult clone() {
try {
return (DescribeDatasetResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}