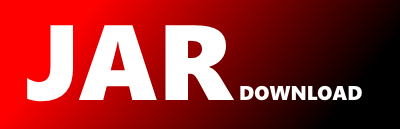
com.amazonaws.services.lookoutequipment.model.DescribeModelResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-lookoutequipment Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.lookoutequipment.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class DescribeModelResult extends com.amazonaws.AmazonWebServiceResult implements Serializable, Cloneable {
/**
*
* The name of the machine learning model being described.
*
*/
private String modelName;
/**
*
* The Amazon Resource Name (ARN) of the machine learning model being described.
*
*/
private String modelArn;
/**
*
* The name of the dataset being used by the machine learning being described.
*
*/
private String datasetName;
/**
*
* The Amazon Resouce Name (ARN) of the dataset used to create the machine learning model being described.
*
*/
private String datasetArn;
/**
*
* A JSON description of the data that is in each time series dataset, including names, column names, and data
* types.
*
*/
private String schema;
/**
*
* Specifies configuration information about the labels input, including its S3 location.
*
*/
private LabelsInputConfiguration labelsInputConfiguration;
/**
*
* Indicates the time reference in the dataset that was used to begin the subset of training data for the machine
* learning model.
*
*/
private java.util.Date trainingDataStartTime;
/**
*
* Indicates the time reference in the dataset that was used to end the subset of training data for the machine
* learning model.
*
*/
private java.util.Date trainingDataEndTime;
/**
*
* Indicates the time reference in the dataset that was used to begin the subset of evaluation data for the machine
* learning model.
*
*/
private java.util.Date evaluationDataStartTime;
/**
*
* Indicates the time reference in the dataset that was used to end the subset of evaluation data for the machine
* learning model.
*
*/
private java.util.Date evaluationDataEndTime;
/**
*
* The Amazon Resource Name (ARN) of a role with permission to access the data source for the machine learning model
* being described.
*
*/
private String roleArn;
/**
*
* The configuration is the TargetSamplingRate
, which is the sampling rate of the data after post
* processing by Amazon Lookout for Equipment. For example, if you provide data that has been collected at a 1
* second level and you want the system to resample the data at a 1 minute rate before training, the
* TargetSamplingRate
is 1 minute.
*
*
* When providing a value for the TargetSamplingRate
, you must attach the prefix "PT" to the rate you
* want. The value for a 1 second rate is therefore PT1S, the value for a 15 minute rate is PT15M, and
* the value for a 1 hour rate is PT1H
*
*/
private DataPreProcessingConfiguration dataPreProcessingConfiguration;
/**
*
* Specifies the current status of the model being described. Status describes the status of the most recent action
* of the model.
*
*/
private String status;
/**
*
* Indicates the time at which the training of the machine learning model began.
*
*/
private java.util.Date trainingExecutionStartTime;
/**
*
* Indicates the time at which the training of the machine learning model was completed.
*
*/
private java.util.Date trainingExecutionEndTime;
/**
*
* If the training of the machine learning model failed, this indicates the reason for that failure.
*
*/
private String failedReason;
/**
*
* The Model Metrics show an aggregated summary of the model's performance within the evaluation time range. This is
* the JSON content of the metrics created when evaluating the model.
*
*/
private String modelMetrics;
/**
*
* Indicates the last time the machine learning model was updated. The type of update is not specified.
*
*/
private java.util.Date lastUpdatedTime;
/**
*
* Indicates the time and date at which the machine learning model was created.
*
*/
private java.util.Date createdAt;
/**
*
* Provides the identifier of the KMS key used to encrypt model data by Amazon Lookout for Equipment.
*
*/
private String serverSideKmsKeyId;
/**
*
* Indicates that the asset associated with this sensor has been shut off. As long as this condition is met, Lookout
* for Equipment will not use data from this asset for training, evaluation, or inference.
*
*/
private String offCondition;
/**
*
* The Amazon Resource Name (ARN) of the source model version. This field appears if the active model version was
* imported.
*
*/
private String sourceModelVersionArn;
/**
*
* The date and time when the import job was started. This field appears if the active model version was imported.
*
*/
private java.util.Date importJobStartTime;
/**
*
* The date and time when the import job was completed. This field appears if the active model version was imported.
*
*/
private java.util.Date importJobEndTime;
/**
*
* The name of the model version used by the inference schedular when running a scheduled inference execution.
*
*/
private Long activeModelVersion;
/**
*
* The Amazon Resource Name (ARN) of the model version used by the inference scheduler when running a scheduled
* inference execution.
*
*/
private String activeModelVersionArn;
/**
*
* The date the active model version was activated.
*
*/
private java.util.Date modelVersionActivatedAt;
/**
*
* The model version that was set as the active model version prior to the current active model version.
*
*/
private Long previousActiveModelVersion;
/**
*
* The ARN of the model version that was set as the active model version prior to the current active model version.
*
*/
private String previousActiveModelVersionArn;
/**
*
* The date and time when the previous active model version was activated.
*
*/
private java.util.Date previousModelVersionActivatedAt;
/**
*
* If the model version was retrained, this field shows a summary of the performance of the prior model on the new
* training range. You can use the information in this JSON-formatted object to compare the new model version and
* the prior model version.
*
*/
private String priorModelMetrics;
/**
*
* If the model version was generated by retraining and the training failed, this indicates the reason for that
* failure.
*
*/
private String latestScheduledRetrainingFailedReason;
/**
*
* Indicates the status of the most recent scheduled retraining run.
*
*/
private String latestScheduledRetrainingStatus;
/**
*
* Indicates the most recent model version that was generated by retraining.
*
*/
private Long latestScheduledRetrainingModelVersion;
/**
*
* Indicates the start time of the most recent scheduled retraining run.
*
*/
private java.util.Date latestScheduledRetrainingStartTime;
/**
*
* Indicates the number of days of data used in the most recent scheduled retraining run.
*
*/
private Integer latestScheduledRetrainingAvailableDataInDays;
/**
*
* Indicates the date and time that the next scheduled retraining run will start on. Lookout for Equipment truncates
* the time you provide to the nearest UTC day.
*
*/
private java.util.Date nextScheduledRetrainingStartDate;
/**
*
* Indicates the start time of the inference data that has been accumulated.
*
*/
private java.util.Date accumulatedInferenceDataStartTime;
/**
*
* Indicates the end time of the inference data that has been accumulated.
*
*/
private java.util.Date accumulatedInferenceDataEndTime;
/**
*
* Indicates the status of the retraining scheduler.
*
*/
private String retrainingSchedulerStatus;
/**
*
* Configuration information for the model's pointwise model diagnostics.
*
*/
private ModelDiagnosticsOutputConfiguration modelDiagnosticsOutputConfiguration;
/**
*
* Provides a quality assessment for a model that uses labels. If Lookout for Equipment determines that the model
* quality is poor based on training metrics, the value is POOR_QUALITY_DETECTED
. Otherwise, the value
* is QUALITY_THRESHOLD_MET
.
*
*
* If the model is unlabeled, the model quality can't be assessed and the value of ModelQuality
is
* CANNOT_DETERMINE_QUALITY
. In this situation, you can get a model quality assessment by adding labels
* to the input dataset and retraining the model.
*
*
* For information about using labels with your models, see Understanding
* labeling.
*
*
* For information about improving the quality of a model, see Best practices with Amazon
* Lookout for Equipment.
*
*/
private String modelQuality;
/**
*
* The name of the machine learning model being described.
*
*
* @param modelName
* The name of the machine learning model being described.
*/
public void setModelName(String modelName) {
this.modelName = modelName;
}
/**
*
* The name of the machine learning model being described.
*
*
* @return The name of the machine learning model being described.
*/
public String getModelName() {
return this.modelName;
}
/**
*
* The name of the machine learning model being described.
*
*
* @param modelName
* The name of the machine learning model being described.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelResult withModelName(String modelName) {
setModelName(modelName);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the machine learning model being described.
*
*
* @param modelArn
* The Amazon Resource Name (ARN) of the machine learning model being described.
*/
public void setModelArn(String modelArn) {
this.modelArn = modelArn;
}
/**
*
* The Amazon Resource Name (ARN) of the machine learning model being described.
*
*
* @return The Amazon Resource Name (ARN) of the machine learning model being described.
*/
public String getModelArn() {
return this.modelArn;
}
/**
*
* The Amazon Resource Name (ARN) of the machine learning model being described.
*
*
* @param modelArn
* The Amazon Resource Name (ARN) of the machine learning model being described.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelResult withModelArn(String modelArn) {
setModelArn(modelArn);
return this;
}
/**
*
* The name of the dataset being used by the machine learning being described.
*
*
* @param datasetName
* The name of the dataset being used by the machine learning being described.
*/
public void setDatasetName(String datasetName) {
this.datasetName = datasetName;
}
/**
*
* The name of the dataset being used by the machine learning being described.
*
*
* @return The name of the dataset being used by the machine learning being described.
*/
public String getDatasetName() {
return this.datasetName;
}
/**
*
* The name of the dataset being used by the machine learning being described.
*
*
* @param datasetName
* The name of the dataset being used by the machine learning being described.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelResult withDatasetName(String datasetName) {
setDatasetName(datasetName);
return this;
}
/**
*
* The Amazon Resouce Name (ARN) of the dataset used to create the machine learning model being described.
*
*
* @param datasetArn
* The Amazon Resouce Name (ARN) of the dataset used to create the machine learning model being described.
*/
public void setDatasetArn(String datasetArn) {
this.datasetArn = datasetArn;
}
/**
*
* The Amazon Resouce Name (ARN) of the dataset used to create the machine learning model being described.
*
*
* @return The Amazon Resouce Name (ARN) of the dataset used to create the machine learning model being described.
*/
public String getDatasetArn() {
return this.datasetArn;
}
/**
*
* The Amazon Resouce Name (ARN) of the dataset used to create the machine learning model being described.
*
*
* @param datasetArn
* The Amazon Resouce Name (ARN) of the dataset used to create the machine learning model being described.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelResult withDatasetArn(String datasetArn) {
setDatasetArn(datasetArn);
return this;
}
/**
*
* A JSON description of the data that is in each time series dataset, including names, column names, and data
* types.
*
*
* This field's value must be valid JSON according to RFC 7159, including the opening and closing braces. For
* example: '{"key": "value"}'.
*
*
* The AWS SDK for Java performs a Base64 encoding on this field before sending this request to the AWS service.
* Users of the SDK should not perform Base64 encoding on this field.
*
*
* @param schema
* A JSON description of the data that is in each time series dataset, including names, column names, and
* data types.
*/
public void setSchema(String schema) {
this.schema = schema;
}
/**
*
* A JSON description of the data that is in each time series dataset, including names, column names, and data
* types.
*
*
* This field's value will be valid JSON according to RFC 7159, including the opening and closing braces. For
* example: '{"key": "value"}'.
*
*
* @return A JSON description of the data that is in each time series dataset, including names, column names, and
* data types.
*/
public String getSchema() {
return this.schema;
}
/**
*
* A JSON description of the data that is in each time series dataset, including names, column names, and data
* types.
*
*
* This field's value must be valid JSON according to RFC 7159, including the opening and closing braces. For
* example: '{"key": "value"}'.
*
*
* The AWS SDK for Java performs a Base64 encoding on this field before sending this request to the AWS service.
* Users of the SDK should not perform Base64 encoding on this field.
*
*
* @param schema
* A JSON description of the data that is in each time series dataset, including names, column names, and
* data types.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelResult withSchema(String schema) {
setSchema(schema);
return this;
}
/**
*
* Specifies configuration information about the labels input, including its S3 location.
*
*
* @param labelsInputConfiguration
* Specifies configuration information about the labels input, including its S3 location.
*/
public void setLabelsInputConfiguration(LabelsInputConfiguration labelsInputConfiguration) {
this.labelsInputConfiguration = labelsInputConfiguration;
}
/**
*
* Specifies configuration information about the labels input, including its S3 location.
*
*
* @return Specifies configuration information about the labels input, including its S3 location.
*/
public LabelsInputConfiguration getLabelsInputConfiguration() {
return this.labelsInputConfiguration;
}
/**
*
* Specifies configuration information about the labels input, including its S3 location.
*
*
* @param labelsInputConfiguration
* Specifies configuration information about the labels input, including its S3 location.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelResult withLabelsInputConfiguration(LabelsInputConfiguration labelsInputConfiguration) {
setLabelsInputConfiguration(labelsInputConfiguration);
return this;
}
/**
*
* Indicates the time reference in the dataset that was used to begin the subset of training data for the machine
* learning model.
*
*
* @param trainingDataStartTime
* Indicates the time reference in the dataset that was used to begin the subset of training data for the
* machine learning model.
*/
public void setTrainingDataStartTime(java.util.Date trainingDataStartTime) {
this.trainingDataStartTime = trainingDataStartTime;
}
/**
*
* Indicates the time reference in the dataset that was used to begin the subset of training data for the machine
* learning model.
*
*
* @return Indicates the time reference in the dataset that was used to begin the subset of training data for the
* machine learning model.
*/
public java.util.Date getTrainingDataStartTime() {
return this.trainingDataStartTime;
}
/**
*
* Indicates the time reference in the dataset that was used to begin the subset of training data for the machine
* learning model.
*
*
* @param trainingDataStartTime
* Indicates the time reference in the dataset that was used to begin the subset of training data for the
* machine learning model.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelResult withTrainingDataStartTime(java.util.Date trainingDataStartTime) {
setTrainingDataStartTime(trainingDataStartTime);
return this;
}
/**
*
* Indicates the time reference in the dataset that was used to end the subset of training data for the machine
* learning model.
*
*
* @param trainingDataEndTime
* Indicates the time reference in the dataset that was used to end the subset of training data for the
* machine learning model.
*/
public void setTrainingDataEndTime(java.util.Date trainingDataEndTime) {
this.trainingDataEndTime = trainingDataEndTime;
}
/**
*
* Indicates the time reference in the dataset that was used to end the subset of training data for the machine
* learning model.
*
*
* @return Indicates the time reference in the dataset that was used to end the subset of training data for the
* machine learning model.
*/
public java.util.Date getTrainingDataEndTime() {
return this.trainingDataEndTime;
}
/**
*
* Indicates the time reference in the dataset that was used to end the subset of training data for the machine
* learning model.
*
*
* @param trainingDataEndTime
* Indicates the time reference in the dataset that was used to end the subset of training data for the
* machine learning model.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelResult withTrainingDataEndTime(java.util.Date trainingDataEndTime) {
setTrainingDataEndTime(trainingDataEndTime);
return this;
}
/**
*
* Indicates the time reference in the dataset that was used to begin the subset of evaluation data for the machine
* learning model.
*
*
* @param evaluationDataStartTime
* Indicates the time reference in the dataset that was used to begin the subset of evaluation data for the
* machine learning model.
*/
public void setEvaluationDataStartTime(java.util.Date evaluationDataStartTime) {
this.evaluationDataStartTime = evaluationDataStartTime;
}
/**
*
* Indicates the time reference in the dataset that was used to begin the subset of evaluation data for the machine
* learning model.
*
*
* @return Indicates the time reference in the dataset that was used to begin the subset of evaluation data for the
* machine learning model.
*/
public java.util.Date getEvaluationDataStartTime() {
return this.evaluationDataStartTime;
}
/**
*
* Indicates the time reference in the dataset that was used to begin the subset of evaluation data for the machine
* learning model.
*
*
* @param evaluationDataStartTime
* Indicates the time reference in the dataset that was used to begin the subset of evaluation data for the
* machine learning model.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelResult withEvaluationDataStartTime(java.util.Date evaluationDataStartTime) {
setEvaluationDataStartTime(evaluationDataStartTime);
return this;
}
/**
*
* Indicates the time reference in the dataset that was used to end the subset of evaluation data for the machine
* learning model.
*
*
* @param evaluationDataEndTime
* Indicates the time reference in the dataset that was used to end the subset of evaluation data for the
* machine learning model.
*/
public void setEvaluationDataEndTime(java.util.Date evaluationDataEndTime) {
this.evaluationDataEndTime = evaluationDataEndTime;
}
/**
*
* Indicates the time reference in the dataset that was used to end the subset of evaluation data for the machine
* learning model.
*
*
* @return Indicates the time reference in the dataset that was used to end the subset of evaluation data for the
* machine learning model.
*/
public java.util.Date getEvaluationDataEndTime() {
return this.evaluationDataEndTime;
}
/**
*
* Indicates the time reference in the dataset that was used to end the subset of evaluation data for the machine
* learning model.
*
*
* @param evaluationDataEndTime
* Indicates the time reference in the dataset that was used to end the subset of evaluation data for the
* machine learning model.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelResult withEvaluationDataEndTime(java.util.Date evaluationDataEndTime) {
setEvaluationDataEndTime(evaluationDataEndTime);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of a role with permission to access the data source for the machine learning model
* being described.
*
*
* @param roleArn
* The Amazon Resource Name (ARN) of a role with permission to access the data source for the machine
* learning model being described.
*/
public void setRoleArn(String roleArn) {
this.roleArn = roleArn;
}
/**
*
* The Amazon Resource Name (ARN) of a role with permission to access the data source for the machine learning model
* being described.
*
*
* @return The Amazon Resource Name (ARN) of a role with permission to access the data source for the machine
* learning model being described.
*/
public String getRoleArn() {
return this.roleArn;
}
/**
*
* The Amazon Resource Name (ARN) of a role with permission to access the data source for the machine learning model
* being described.
*
*
* @param roleArn
* The Amazon Resource Name (ARN) of a role with permission to access the data source for the machine
* learning model being described.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelResult withRoleArn(String roleArn) {
setRoleArn(roleArn);
return this;
}
/**
*
* The configuration is the TargetSamplingRate
, which is the sampling rate of the data after post
* processing by Amazon Lookout for Equipment. For example, if you provide data that has been collected at a 1
* second level and you want the system to resample the data at a 1 minute rate before training, the
* TargetSamplingRate
is 1 minute.
*
*
* When providing a value for the TargetSamplingRate
, you must attach the prefix "PT" to the rate you
* want. The value for a 1 second rate is therefore PT1S, the value for a 15 minute rate is PT15M, and
* the value for a 1 hour rate is PT1H
*
*
* @param dataPreProcessingConfiguration
* The configuration is the TargetSamplingRate
, which is the sampling rate of the data after
* post processing by Amazon Lookout for Equipment. For example, if you provide data that has been collected
* at a 1 second level and you want the system to resample the data at a 1 minute rate before training, the
* TargetSamplingRate
is 1 minute.
*
* When providing a value for the TargetSamplingRate
, you must attach the prefix "PT" to the
* rate you want. The value for a 1 second rate is therefore PT1S, the value for a 15 minute rate is
* PT15M, and the value for a 1 hour rate is PT1H
*/
public void setDataPreProcessingConfiguration(DataPreProcessingConfiguration dataPreProcessingConfiguration) {
this.dataPreProcessingConfiguration = dataPreProcessingConfiguration;
}
/**
*
* The configuration is the TargetSamplingRate
, which is the sampling rate of the data after post
* processing by Amazon Lookout for Equipment. For example, if you provide data that has been collected at a 1
* second level and you want the system to resample the data at a 1 minute rate before training, the
* TargetSamplingRate
is 1 minute.
*
*
* When providing a value for the TargetSamplingRate
, you must attach the prefix "PT" to the rate you
* want. The value for a 1 second rate is therefore PT1S, the value for a 15 minute rate is PT15M, and
* the value for a 1 hour rate is PT1H
*
*
* @return The configuration is the TargetSamplingRate
, which is the sampling rate of the data after
* post processing by Amazon Lookout for Equipment. For example, if you provide data that has been collected
* at a 1 second level and you want the system to resample the data at a 1 minute rate before training, the
* TargetSamplingRate
is 1 minute.
*
* When providing a value for the TargetSamplingRate
, you must attach the prefix "PT" to the
* rate you want. The value for a 1 second rate is therefore PT1S, the value for a 15 minute rate is
* PT15M, and the value for a 1 hour rate is PT1H
*/
public DataPreProcessingConfiguration getDataPreProcessingConfiguration() {
return this.dataPreProcessingConfiguration;
}
/**
*
* The configuration is the TargetSamplingRate
, which is the sampling rate of the data after post
* processing by Amazon Lookout for Equipment. For example, if you provide data that has been collected at a 1
* second level and you want the system to resample the data at a 1 minute rate before training, the
* TargetSamplingRate
is 1 minute.
*
*
* When providing a value for the TargetSamplingRate
, you must attach the prefix "PT" to the rate you
* want. The value for a 1 second rate is therefore PT1S, the value for a 15 minute rate is PT15M, and
* the value for a 1 hour rate is PT1H
*
*
* @param dataPreProcessingConfiguration
* The configuration is the TargetSamplingRate
, which is the sampling rate of the data after
* post processing by Amazon Lookout for Equipment. For example, if you provide data that has been collected
* at a 1 second level and you want the system to resample the data at a 1 minute rate before training, the
* TargetSamplingRate
is 1 minute.
*
* When providing a value for the TargetSamplingRate
, you must attach the prefix "PT" to the
* rate you want. The value for a 1 second rate is therefore PT1S, the value for a 15 minute rate is
* PT15M, and the value for a 1 hour rate is PT1H
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelResult withDataPreProcessingConfiguration(DataPreProcessingConfiguration dataPreProcessingConfiguration) {
setDataPreProcessingConfiguration(dataPreProcessingConfiguration);
return this;
}
/**
*
* Specifies the current status of the model being described. Status describes the status of the most recent action
* of the model.
*
*
* @param status
* Specifies the current status of the model being described. Status describes the status of the most recent
* action of the model.
* @see ModelStatus
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* Specifies the current status of the model being described. Status describes the status of the most recent action
* of the model.
*
*
* @return Specifies the current status of the model being described. Status describes the status of the most recent
* action of the model.
* @see ModelStatus
*/
public String getStatus() {
return this.status;
}
/**
*
* Specifies the current status of the model being described. Status describes the status of the most recent action
* of the model.
*
*
* @param status
* Specifies the current status of the model being described. Status describes the status of the most recent
* action of the model.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ModelStatus
*/
public DescribeModelResult withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* Specifies the current status of the model being described. Status describes the status of the most recent action
* of the model.
*
*
* @param status
* Specifies the current status of the model being described. Status describes the status of the most recent
* action of the model.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ModelStatus
*/
public DescribeModelResult withStatus(ModelStatus status) {
this.status = status.toString();
return this;
}
/**
*
* Indicates the time at which the training of the machine learning model began.
*
*
* @param trainingExecutionStartTime
* Indicates the time at which the training of the machine learning model began.
*/
public void setTrainingExecutionStartTime(java.util.Date trainingExecutionStartTime) {
this.trainingExecutionStartTime = trainingExecutionStartTime;
}
/**
*
* Indicates the time at which the training of the machine learning model began.
*
*
* @return Indicates the time at which the training of the machine learning model began.
*/
public java.util.Date getTrainingExecutionStartTime() {
return this.trainingExecutionStartTime;
}
/**
*
* Indicates the time at which the training of the machine learning model began.
*
*
* @param trainingExecutionStartTime
* Indicates the time at which the training of the machine learning model began.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelResult withTrainingExecutionStartTime(java.util.Date trainingExecutionStartTime) {
setTrainingExecutionStartTime(trainingExecutionStartTime);
return this;
}
/**
*
* Indicates the time at which the training of the machine learning model was completed.
*
*
* @param trainingExecutionEndTime
* Indicates the time at which the training of the machine learning model was completed.
*/
public void setTrainingExecutionEndTime(java.util.Date trainingExecutionEndTime) {
this.trainingExecutionEndTime = trainingExecutionEndTime;
}
/**
*
* Indicates the time at which the training of the machine learning model was completed.
*
*
* @return Indicates the time at which the training of the machine learning model was completed.
*/
public java.util.Date getTrainingExecutionEndTime() {
return this.trainingExecutionEndTime;
}
/**
*
* Indicates the time at which the training of the machine learning model was completed.
*
*
* @param trainingExecutionEndTime
* Indicates the time at which the training of the machine learning model was completed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelResult withTrainingExecutionEndTime(java.util.Date trainingExecutionEndTime) {
setTrainingExecutionEndTime(trainingExecutionEndTime);
return this;
}
/**
*
* If the training of the machine learning model failed, this indicates the reason for that failure.
*
*
* @param failedReason
* If the training of the machine learning model failed, this indicates the reason for that failure.
*/
public void setFailedReason(String failedReason) {
this.failedReason = failedReason;
}
/**
*
* If the training of the machine learning model failed, this indicates the reason for that failure.
*
*
* @return If the training of the machine learning model failed, this indicates the reason for that failure.
*/
public String getFailedReason() {
return this.failedReason;
}
/**
*
* If the training of the machine learning model failed, this indicates the reason for that failure.
*
*
* @param failedReason
* If the training of the machine learning model failed, this indicates the reason for that failure.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelResult withFailedReason(String failedReason) {
setFailedReason(failedReason);
return this;
}
/**
*
* The Model Metrics show an aggregated summary of the model's performance within the evaluation time range. This is
* the JSON content of the metrics created when evaluating the model.
*
*
* This field's value must be valid JSON according to RFC 7159, including the opening and closing braces. For
* example: '{"key": "value"}'.
*
*
* The AWS SDK for Java performs a Base64 encoding on this field before sending this request to the AWS service.
* Users of the SDK should not perform Base64 encoding on this field.
*
*
* @param modelMetrics
* The Model Metrics show an aggregated summary of the model's performance within the evaluation time range.
* This is the JSON content of the metrics created when evaluating the model.
*/
public void setModelMetrics(String modelMetrics) {
this.modelMetrics = modelMetrics;
}
/**
*
* The Model Metrics show an aggregated summary of the model's performance within the evaluation time range. This is
* the JSON content of the metrics created when evaluating the model.
*
*
* This field's value will be valid JSON according to RFC 7159, including the opening and closing braces. For
* example: '{"key": "value"}'.
*
*
* @return The Model Metrics show an aggregated summary of the model's performance within the evaluation time range.
* This is the JSON content of the metrics created when evaluating the model.
*/
public String getModelMetrics() {
return this.modelMetrics;
}
/**
*
* The Model Metrics show an aggregated summary of the model's performance within the evaluation time range. This is
* the JSON content of the metrics created when evaluating the model.
*
*
* This field's value must be valid JSON according to RFC 7159, including the opening and closing braces. For
* example: '{"key": "value"}'.
*
*
* The AWS SDK for Java performs a Base64 encoding on this field before sending this request to the AWS service.
* Users of the SDK should not perform Base64 encoding on this field.
*
*
* @param modelMetrics
* The Model Metrics show an aggregated summary of the model's performance within the evaluation time range.
* This is the JSON content of the metrics created when evaluating the model.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelResult withModelMetrics(String modelMetrics) {
setModelMetrics(modelMetrics);
return this;
}
/**
*
* Indicates the last time the machine learning model was updated. The type of update is not specified.
*
*
* @param lastUpdatedTime
* Indicates the last time the machine learning model was updated. The type of update is not specified.
*/
public void setLastUpdatedTime(java.util.Date lastUpdatedTime) {
this.lastUpdatedTime = lastUpdatedTime;
}
/**
*
* Indicates the last time the machine learning model was updated. The type of update is not specified.
*
*
* @return Indicates the last time the machine learning model was updated. The type of update is not specified.
*/
public java.util.Date getLastUpdatedTime() {
return this.lastUpdatedTime;
}
/**
*
* Indicates the last time the machine learning model was updated. The type of update is not specified.
*
*
* @param lastUpdatedTime
* Indicates the last time the machine learning model was updated. The type of update is not specified.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelResult withLastUpdatedTime(java.util.Date lastUpdatedTime) {
setLastUpdatedTime(lastUpdatedTime);
return this;
}
/**
*
* Indicates the time and date at which the machine learning model was created.
*
*
* @param createdAt
* Indicates the time and date at which the machine learning model was created.
*/
public void setCreatedAt(java.util.Date createdAt) {
this.createdAt = createdAt;
}
/**
*
* Indicates the time and date at which the machine learning model was created.
*
*
* @return Indicates the time and date at which the machine learning model was created.
*/
public java.util.Date getCreatedAt() {
return this.createdAt;
}
/**
*
* Indicates the time and date at which the machine learning model was created.
*
*
* @param createdAt
* Indicates the time and date at which the machine learning model was created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelResult withCreatedAt(java.util.Date createdAt) {
setCreatedAt(createdAt);
return this;
}
/**
*
* Provides the identifier of the KMS key used to encrypt model data by Amazon Lookout for Equipment.
*
*
* @param serverSideKmsKeyId
* Provides the identifier of the KMS key used to encrypt model data by Amazon Lookout for Equipment.
*/
public void setServerSideKmsKeyId(String serverSideKmsKeyId) {
this.serverSideKmsKeyId = serverSideKmsKeyId;
}
/**
*
* Provides the identifier of the KMS key used to encrypt model data by Amazon Lookout for Equipment.
*
*
* @return Provides the identifier of the KMS key used to encrypt model data by Amazon Lookout for Equipment.
*/
public String getServerSideKmsKeyId() {
return this.serverSideKmsKeyId;
}
/**
*
* Provides the identifier of the KMS key used to encrypt model data by Amazon Lookout for Equipment.
*
*
* @param serverSideKmsKeyId
* Provides the identifier of the KMS key used to encrypt model data by Amazon Lookout for Equipment.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelResult withServerSideKmsKeyId(String serverSideKmsKeyId) {
setServerSideKmsKeyId(serverSideKmsKeyId);
return this;
}
/**
*
* Indicates that the asset associated with this sensor has been shut off. As long as this condition is met, Lookout
* for Equipment will not use data from this asset for training, evaluation, or inference.
*
*
* @param offCondition
* Indicates that the asset associated with this sensor has been shut off. As long as this condition is met,
* Lookout for Equipment will not use data from this asset for training, evaluation, or inference.
*/
public void setOffCondition(String offCondition) {
this.offCondition = offCondition;
}
/**
*
* Indicates that the asset associated with this sensor has been shut off. As long as this condition is met, Lookout
* for Equipment will not use data from this asset for training, evaluation, or inference.
*
*
* @return Indicates that the asset associated with this sensor has been shut off. As long as this condition is met,
* Lookout for Equipment will not use data from this asset for training, evaluation, or inference.
*/
public String getOffCondition() {
return this.offCondition;
}
/**
*
* Indicates that the asset associated with this sensor has been shut off. As long as this condition is met, Lookout
* for Equipment will not use data from this asset for training, evaluation, or inference.
*
*
* @param offCondition
* Indicates that the asset associated with this sensor has been shut off. As long as this condition is met,
* Lookout for Equipment will not use data from this asset for training, evaluation, or inference.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelResult withOffCondition(String offCondition) {
setOffCondition(offCondition);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the source model version. This field appears if the active model version was
* imported.
*
*
* @param sourceModelVersionArn
* The Amazon Resource Name (ARN) of the source model version. This field appears if the active model version
* was imported.
*/
public void setSourceModelVersionArn(String sourceModelVersionArn) {
this.sourceModelVersionArn = sourceModelVersionArn;
}
/**
*
* The Amazon Resource Name (ARN) of the source model version. This field appears if the active model version was
* imported.
*
*
* @return The Amazon Resource Name (ARN) of the source model version. This field appears if the active model
* version was imported.
*/
public String getSourceModelVersionArn() {
return this.sourceModelVersionArn;
}
/**
*
* The Amazon Resource Name (ARN) of the source model version. This field appears if the active model version was
* imported.
*
*
* @param sourceModelVersionArn
* The Amazon Resource Name (ARN) of the source model version. This field appears if the active model version
* was imported.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelResult withSourceModelVersionArn(String sourceModelVersionArn) {
setSourceModelVersionArn(sourceModelVersionArn);
return this;
}
/**
*
* The date and time when the import job was started. This field appears if the active model version was imported.
*
*
* @param importJobStartTime
* The date and time when the import job was started. This field appears if the active model version was
* imported.
*/
public void setImportJobStartTime(java.util.Date importJobStartTime) {
this.importJobStartTime = importJobStartTime;
}
/**
*
* The date and time when the import job was started. This field appears if the active model version was imported.
*
*
* @return The date and time when the import job was started. This field appears if the active model version was
* imported.
*/
public java.util.Date getImportJobStartTime() {
return this.importJobStartTime;
}
/**
*
* The date and time when the import job was started. This field appears if the active model version was imported.
*
*
* @param importJobStartTime
* The date and time when the import job was started. This field appears if the active model version was
* imported.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelResult withImportJobStartTime(java.util.Date importJobStartTime) {
setImportJobStartTime(importJobStartTime);
return this;
}
/**
*
* The date and time when the import job was completed. This field appears if the active model version was imported.
*
*
* @param importJobEndTime
* The date and time when the import job was completed. This field appears if the active model version was
* imported.
*/
public void setImportJobEndTime(java.util.Date importJobEndTime) {
this.importJobEndTime = importJobEndTime;
}
/**
*
* The date and time when the import job was completed. This field appears if the active model version was imported.
*
*
* @return The date and time when the import job was completed. This field appears if the active model version was
* imported.
*/
public java.util.Date getImportJobEndTime() {
return this.importJobEndTime;
}
/**
*
* The date and time when the import job was completed. This field appears if the active model version was imported.
*
*
* @param importJobEndTime
* The date and time when the import job was completed. This field appears if the active model version was
* imported.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelResult withImportJobEndTime(java.util.Date importJobEndTime) {
setImportJobEndTime(importJobEndTime);
return this;
}
/**
*
* The name of the model version used by the inference schedular when running a scheduled inference execution.
*
*
* @param activeModelVersion
* The name of the model version used by the inference schedular when running a scheduled inference
* execution.
*/
public void setActiveModelVersion(Long activeModelVersion) {
this.activeModelVersion = activeModelVersion;
}
/**
*
* The name of the model version used by the inference schedular when running a scheduled inference execution.
*
*
* @return The name of the model version used by the inference schedular when running a scheduled inference
* execution.
*/
public Long getActiveModelVersion() {
return this.activeModelVersion;
}
/**
*
* The name of the model version used by the inference schedular when running a scheduled inference execution.
*
*
* @param activeModelVersion
* The name of the model version used by the inference schedular when running a scheduled inference
* execution.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelResult withActiveModelVersion(Long activeModelVersion) {
setActiveModelVersion(activeModelVersion);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the model version used by the inference scheduler when running a scheduled
* inference execution.
*
*
* @param activeModelVersionArn
* The Amazon Resource Name (ARN) of the model version used by the inference scheduler when running a
* scheduled inference execution.
*/
public void setActiveModelVersionArn(String activeModelVersionArn) {
this.activeModelVersionArn = activeModelVersionArn;
}
/**
*
* The Amazon Resource Name (ARN) of the model version used by the inference scheduler when running a scheduled
* inference execution.
*
*
* @return The Amazon Resource Name (ARN) of the model version used by the inference scheduler when running a
* scheduled inference execution.
*/
public String getActiveModelVersionArn() {
return this.activeModelVersionArn;
}
/**
*
* The Amazon Resource Name (ARN) of the model version used by the inference scheduler when running a scheduled
* inference execution.
*
*
* @param activeModelVersionArn
* The Amazon Resource Name (ARN) of the model version used by the inference scheduler when running a
* scheduled inference execution.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelResult withActiveModelVersionArn(String activeModelVersionArn) {
setActiveModelVersionArn(activeModelVersionArn);
return this;
}
/**
*
* The date the active model version was activated.
*
*
* @param modelVersionActivatedAt
* The date the active model version was activated.
*/
public void setModelVersionActivatedAt(java.util.Date modelVersionActivatedAt) {
this.modelVersionActivatedAt = modelVersionActivatedAt;
}
/**
*
* The date the active model version was activated.
*
*
* @return The date the active model version was activated.
*/
public java.util.Date getModelVersionActivatedAt() {
return this.modelVersionActivatedAt;
}
/**
*
* The date the active model version was activated.
*
*
* @param modelVersionActivatedAt
* The date the active model version was activated.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelResult withModelVersionActivatedAt(java.util.Date modelVersionActivatedAt) {
setModelVersionActivatedAt(modelVersionActivatedAt);
return this;
}
/**
*
* The model version that was set as the active model version prior to the current active model version.
*
*
* @param previousActiveModelVersion
* The model version that was set as the active model version prior to the current active model version.
*/
public void setPreviousActiveModelVersion(Long previousActiveModelVersion) {
this.previousActiveModelVersion = previousActiveModelVersion;
}
/**
*
* The model version that was set as the active model version prior to the current active model version.
*
*
* @return The model version that was set as the active model version prior to the current active model version.
*/
public Long getPreviousActiveModelVersion() {
return this.previousActiveModelVersion;
}
/**
*
* The model version that was set as the active model version prior to the current active model version.
*
*
* @param previousActiveModelVersion
* The model version that was set as the active model version prior to the current active model version.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelResult withPreviousActiveModelVersion(Long previousActiveModelVersion) {
setPreviousActiveModelVersion(previousActiveModelVersion);
return this;
}
/**
*
* The ARN of the model version that was set as the active model version prior to the current active model version.
*
*
* @param previousActiveModelVersionArn
* The ARN of the model version that was set as the active model version prior to the current active model
* version.
*/
public void setPreviousActiveModelVersionArn(String previousActiveModelVersionArn) {
this.previousActiveModelVersionArn = previousActiveModelVersionArn;
}
/**
*
* The ARN of the model version that was set as the active model version prior to the current active model version.
*
*
* @return The ARN of the model version that was set as the active model version prior to the current active model
* version.
*/
public String getPreviousActiveModelVersionArn() {
return this.previousActiveModelVersionArn;
}
/**
*
* The ARN of the model version that was set as the active model version prior to the current active model version.
*
*
* @param previousActiveModelVersionArn
* The ARN of the model version that was set as the active model version prior to the current active model
* version.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelResult withPreviousActiveModelVersionArn(String previousActiveModelVersionArn) {
setPreviousActiveModelVersionArn(previousActiveModelVersionArn);
return this;
}
/**
*
* The date and time when the previous active model version was activated.
*
*
* @param previousModelVersionActivatedAt
* The date and time when the previous active model version was activated.
*/
public void setPreviousModelVersionActivatedAt(java.util.Date previousModelVersionActivatedAt) {
this.previousModelVersionActivatedAt = previousModelVersionActivatedAt;
}
/**
*
* The date and time when the previous active model version was activated.
*
*
* @return The date and time when the previous active model version was activated.
*/
public java.util.Date getPreviousModelVersionActivatedAt() {
return this.previousModelVersionActivatedAt;
}
/**
*
* The date and time when the previous active model version was activated.
*
*
* @param previousModelVersionActivatedAt
* The date and time when the previous active model version was activated.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelResult withPreviousModelVersionActivatedAt(java.util.Date previousModelVersionActivatedAt) {
setPreviousModelVersionActivatedAt(previousModelVersionActivatedAt);
return this;
}
/**
*
* If the model version was retrained, this field shows a summary of the performance of the prior model on the new
* training range. You can use the information in this JSON-formatted object to compare the new model version and
* the prior model version.
*
*
* This field's value must be valid JSON according to RFC 7159, including the opening and closing braces. For
* example: '{"key": "value"}'.
*
*
* The AWS SDK for Java performs a Base64 encoding on this field before sending this request to the AWS service.
* Users of the SDK should not perform Base64 encoding on this field.
*
*
* @param priorModelMetrics
* If the model version was retrained, this field shows a summary of the performance of the prior model on
* the new training range. You can use the information in this JSON-formatted object to compare the new model
* version and the prior model version.
*/
public void setPriorModelMetrics(String priorModelMetrics) {
this.priorModelMetrics = priorModelMetrics;
}
/**
*
* If the model version was retrained, this field shows a summary of the performance of the prior model on the new
* training range. You can use the information in this JSON-formatted object to compare the new model version and
* the prior model version.
*
*
* This field's value will be valid JSON according to RFC 7159, including the opening and closing braces. For
* example: '{"key": "value"}'.
*
*
* @return If the model version was retrained, this field shows a summary of the performance of the prior model on
* the new training range. You can use the information in this JSON-formatted object to compare the new
* model version and the prior model version.
*/
public String getPriorModelMetrics() {
return this.priorModelMetrics;
}
/**
*
* If the model version was retrained, this field shows a summary of the performance of the prior model on the new
* training range. You can use the information in this JSON-formatted object to compare the new model version and
* the prior model version.
*
*
* This field's value must be valid JSON according to RFC 7159, including the opening and closing braces. For
* example: '{"key": "value"}'.
*
*
* The AWS SDK for Java performs a Base64 encoding on this field before sending this request to the AWS service.
* Users of the SDK should not perform Base64 encoding on this field.
*
*
* @param priorModelMetrics
* If the model version was retrained, this field shows a summary of the performance of the prior model on
* the new training range. You can use the information in this JSON-formatted object to compare the new model
* version and the prior model version.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelResult withPriorModelMetrics(String priorModelMetrics) {
setPriorModelMetrics(priorModelMetrics);
return this;
}
/**
*
* If the model version was generated by retraining and the training failed, this indicates the reason for that
* failure.
*
*
* @param latestScheduledRetrainingFailedReason
* If the model version was generated by retraining and the training failed, this indicates the reason for
* that failure.
*/
public void setLatestScheduledRetrainingFailedReason(String latestScheduledRetrainingFailedReason) {
this.latestScheduledRetrainingFailedReason = latestScheduledRetrainingFailedReason;
}
/**
*
* If the model version was generated by retraining and the training failed, this indicates the reason for that
* failure.
*
*
* @return If the model version was generated by retraining and the training failed, this indicates the reason for
* that failure.
*/
public String getLatestScheduledRetrainingFailedReason() {
return this.latestScheduledRetrainingFailedReason;
}
/**
*
* If the model version was generated by retraining and the training failed, this indicates the reason for that
* failure.
*
*
* @param latestScheduledRetrainingFailedReason
* If the model version was generated by retraining and the training failed, this indicates the reason for
* that failure.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelResult withLatestScheduledRetrainingFailedReason(String latestScheduledRetrainingFailedReason) {
setLatestScheduledRetrainingFailedReason(latestScheduledRetrainingFailedReason);
return this;
}
/**
*
* Indicates the status of the most recent scheduled retraining run.
*
*
* @param latestScheduledRetrainingStatus
* Indicates the status of the most recent scheduled retraining run.
* @see ModelVersionStatus
*/
public void setLatestScheduledRetrainingStatus(String latestScheduledRetrainingStatus) {
this.latestScheduledRetrainingStatus = latestScheduledRetrainingStatus;
}
/**
*
* Indicates the status of the most recent scheduled retraining run.
*
*
* @return Indicates the status of the most recent scheduled retraining run.
* @see ModelVersionStatus
*/
public String getLatestScheduledRetrainingStatus() {
return this.latestScheduledRetrainingStatus;
}
/**
*
* Indicates the status of the most recent scheduled retraining run.
*
*
* @param latestScheduledRetrainingStatus
* Indicates the status of the most recent scheduled retraining run.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ModelVersionStatus
*/
public DescribeModelResult withLatestScheduledRetrainingStatus(String latestScheduledRetrainingStatus) {
setLatestScheduledRetrainingStatus(latestScheduledRetrainingStatus);
return this;
}
/**
*
* Indicates the status of the most recent scheduled retraining run.
*
*
* @param latestScheduledRetrainingStatus
* Indicates the status of the most recent scheduled retraining run.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ModelVersionStatus
*/
public DescribeModelResult withLatestScheduledRetrainingStatus(ModelVersionStatus latestScheduledRetrainingStatus) {
this.latestScheduledRetrainingStatus = latestScheduledRetrainingStatus.toString();
return this;
}
/**
*
* Indicates the most recent model version that was generated by retraining.
*
*
* @param latestScheduledRetrainingModelVersion
* Indicates the most recent model version that was generated by retraining.
*/
public void setLatestScheduledRetrainingModelVersion(Long latestScheduledRetrainingModelVersion) {
this.latestScheduledRetrainingModelVersion = latestScheduledRetrainingModelVersion;
}
/**
*
* Indicates the most recent model version that was generated by retraining.
*
*
* @return Indicates the most recent model version that was generated by retraining.
*/
public Long getLatestScheduledRetrainingModelVersion() {
return this.latestScheduledRetrainingModelVersion;
}
/**
*
* Indicates the most recent model version that was generated by retraining.
*
*
* @param latestScheduledRetrainingModelVersion
* Indicates the most recent model version that was generated by retraining.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelResult withLatestScheduledRetrainingModelVersion(Long latestScheduledRetrainingModelVersion) {
setLatestScheduledRetrainingModelVersion(latestScheduledRetrainingModelVersion);
return this;
}
/**
*
* Indicates the start time of the most recent scheduled retraining run.
*
*
* @param latestScheduledRetrainingStartTime
* Indicates the start time of the most recent scheduled retraining run.
*/
public void setLatestScheduledRetrainingStartTime(java.util.Date latestScheduledRetrainingStartTime) {
this.latestScheduledRetrainingStartTime = latestScheduledRetrainingStartTime;
}
/**
*
* Indicates the start time of the most recent scheduled retraining run.
*
*
* @return Indicates the start time of the most recent scheduled retraining run.
*/
public java.util.Date getLatestScheduledRetrainingStartTime() {
return this.latestScheduledRetrainingStartTime;
}
/**
*
* Indicates the start time of the most recent scheduled retraining run.
*
*
* @param latestScheduledRetrainingStartTime
* Indicates the start time of the most recent scheduled retraining run.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelResult withLatestScheduledRetrainingStartTime(java.util.Date latestScheduledRetrainingStartTime) {
setLatestScheduledRetrainingStartTime(latestScheduledRetrainingStartTime);
return this;
}
/**
*
* Indicates the number of days of data used in the most recent scheduled retraining run.
*
*
* @param latestScheduledRetrainingAvailableDataInDays
* Indicates the number of days of data used in the most recent scheduled retraining run.
*/
public void setLatestScheduledRetrainingAvailableDataInDays(Integer latestScheduledRetrainingAvailableDataInDays) {
this.latestScheduledRetrainingAvailableDataInDays = latestScheduledRetrainingAvailableDataInDays;
}
/**
*
* Indicates the number of days of data used in the most recent scheduled retraining run.
*
*
* @return Indicates the number of days of data used in the most recent scheduled retraining run.
*/
public Integer getLatestScheduledRetrainingAvailableDataInDays() {
return this.latestScheduledRetrainingAvailableDataInDays;
}
/**
*
* Indicates the number of days of data used in the most recent scheduled retraining run.
*
*
* @param latestScheduledRetrainingAvailableDataInDays
* Indicates the number of days of data used in the most recent scheduled retraining run.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelResult withLatestScheduledRetrainingAvailableDataInDays(Integer latestScheduledRetrainingAvailableDataInDays) {
setLatestScheduledRetrainingAvailableDataInDays(latestScheduledRetrainingAvailableDataInDays);
return this;
}
/**
*
* Indicates the date and time that the next scheduled retraining run will start on. Lookout for Equipment truncates
* the time you provide to the nearest UTC day.
*
*
* @param nextScheduledRetrainingStartDate
* Indicates the date and time that the next scheduled retraining run will start on. Lookout for Equipment
* truncates the time you provide to the nearest UTC day.
*/
public void setNextScheduledRetrainingStartDate(java.util.Date nextScheduledRetrainingStartDate) {
this.nextScheduledRetrainingStartDate = nextScheduledRetrainingStartDate;
}
/**
*
* Indicates the date and time that the next scheduled retraining run will start on. Lookout for Equipment truncates
* the time you provide to the nearest UTC day.
*
*
* @return Indicates the date and time that the next scheduled retraining run will start on. Lookout for Equipment
* truncates the time you provide to the nearest UTC day.
*/
public java.util.Date getNextScheduledRetrainingStartDate() {
return this.nextScheduledRetrainingStartDate;
}
/**
*
* Indicates the date and time that the next scheduled retraining run will start on. Lookout for Equipment truncates
* the time you provide to the nearest UTC day.
*
*
* @param nextScheduledRetrainingStartDate
* Indicates the date and time that the next scheduled retraining run will start on. Lookout for Equipment
* truncates the time you provide to the nearest UTC day.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelResult withNextScheduledRetrainingStartDate(java.util.Date nextScheduledRetrainingStartDate) {
setNextScheduledRetrainingStartDate(nextScheduledRetrainingStartDate);
return this;
}
/**
*
* Indicates the start time of the inference data that has been accumulated.
*
*
* @param accumulatedInferenceDataStartTime
* Indicates the start time of the inference data that has been accumulated.
*/
public void setAccumulatedInferenceDataStartTime(java.util.Date accumulatedInferenceDataStartTime) {
this.accumulatedInferenceDataStartTime = accumulatedInferenceDataStartTime;
}
/**
*
* Indicates the start time of the inference data that has been accumulated.
*
*
* @return Indicates the start time of the inference data that has been accumulated.
*/
public java.util.Date getAccumulatedInferenceDataStartTime() {
return this.accumulatedInferenceDataStartTime;
}
/**
*
* Indicates the start time of the inference data that has been accumulated.
*
*
* @param accumulatedInferenceDataStartTime
* Indicates the start time of the inference data that has been accumulated.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelResult withAccumulatedInferenceDataStartTime(java.util.Date accumulatedInferenceDataStartTime) {
setAccumulatedInferenceDataStartTime(accumulatedInferenceDataStartTime);
return this;
}
/**
*
* Indicates the end time of the inference data that has been accumulated.
*
*
* @param accumulatedInferenceDataEndTime
* Indicates the end time of the inference data that has been accumulated.
*/
public void setAccumulatedInferenceDataEndTime(java.util.Date accumulatedInferenceDataEndTime) {
this.accumulatedInferenceDataEndTime = accumulatedInferenceDataEndTime;
}
/**
*
* Indicates the end time of the inference data that has been accumulated.
*
*
* @return Indicates the end time of the inference data that has been accumulated.
*/
public java.util.Date getAccumulatedInferenceDataEndTime() {
return this.accumulatedInferenceDataEndTime;
}
/**
*
* Indicates the end time of the inference data that has been accumulated.
*
*
* @param accumulatedInferenceDataEndTime
* Indicates the end time of the inference data that has been accumulated.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelResult withAccumulatedInferenceDataEndTime(java.util.Date accumulatedInferenceDataEndTime) {
setAccumulatedInferenceDataEndTime(accumulatedInferenceDataEndTime);
return this;
}
/**
*
* Indicates the status of the retraining scheduler.
*
*
* @param retrainingSchedulerStatus
* Indicates the status of the retraining scheduler.
* @see RetrainingSchedulerStatus
*/
public void setRetrainingSchedulerStatus(String retrainingSchedulerStatus) {
this.retrainingSchedulerStatus = retrainingSchedulerStatus;
}
/**
*
* Indicates the status of the retraining scheduler.
*
*
* @return Indicates the status of the retraining scheduler.
* @see RetrainingSchedulerStatus
*/
public String getRetrainingSchedulerStatus() {
return this.retrainingSchedulerStatus;
}
/**
*
* Indicates the status of the retraining scheduler.
*
*
* @param retrainingSchedulerStatus
* Indicates the status of the retraining scheduler.
* @return Returns a reference to this object so that method calls can be chained together.
* @see RetrainingSchedulerStatus
*/
public DescribeModelResult withRetrainingSchedulerStatus(String retrainingSchedulerStatus) {
setRetrainingSchedulerStatus(retrainingSchedulerStatus);
return this;
}
/**
*
* Indicates the status of the retraining scheduler.
*
*
* @param retrainingSchedulerStatus
* Indicates the status of the retraining scheduler.
* @return Returns a reference to this object so that method calls can be chained together.
* @see RetrainingSchedulerStatus
*/
public DescribeModelResult withRetrainingSchedulerStatus(RetrainingSchedulerStatus retrainingSchedulerStatus) {
this.retrainingSchedulerStatus = retrainingSchedulerStatus.toString();
return this;
}
/**
*
* Configuration information for the model's pointwise model diagnostics.
*
*
* @param modelDiagnosticsOutputConfiguration
* Configuration information for the model's pointwise model diagnostics.
*/
public void setModelDiagnosticsOutputConfiguration(ModelDiagnosticsOutputConfiguration modelDiagnosticsOutputConfiguration) {
this.modelDiagnosticsOutputConfiguration = modelDiagnosticsOutputConfiguration;
}
/**
*
* Configuration information for the model's pointwise model diagnostics.
*
*
* @return Configuration information for the model's pointwise model diagnostics.
*/
public ModelDiagnosticsOutputConfiguration getModelDiagnosticsOutputConfiguration() {
return this.modelDiagnosticsOutputConfiguration;
}
/**
*
* Configuration information for the model's pointwise model diagnostics.
*
*
* @param modelDiagnosticsOutputConfiguration
* Configuration information for the model's pointwise model diagnostics.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelResult withModelDiagnosticsOutputConfiguration(ModelDiagnosticsOutputConfiguration modelDiagnosticsOutputConfiguration) {
setModelDiagnosticsOutputConfiguration(modelDiagnosticsOutputConfiguration);
return this;
}
/**
*
* Provides a quality assessment for a model that uses labels. If Lookout for Equipment determines that the model
* quality is poor based on training metrics, the value is POOR_QUALITY_DETECTED
. Otherwise, the value
* is QUALITY_THRESHOLD_MET
.
*
*
* If the model is unlabeled, the model quality can't be assessed and the value of ModelQuality
is
* CANNOT_DETERMINE_QUALITY
. In this situation, you can get a model quality assessment by adding labels
* to the input dataset and retraining the model.
*
*
* For information about using labels with your models, see Understanding
* labeling.
*
*
* For information about improving the quality of a model, see Best practices with Amazon
* Lookout for Equipment.
*
*
* @param modelQuality
* Provides a quality assessment for a model that uses labels. If Lookout for Equipment determines that the
* model quality is poor based on training metrics, the value is POOR_QUALITY_DETECTED
.
* Otherwise, the value is QUALITY_THRESHOLD_MET
.
*
* If the model is unlabeled, the model quality can't be assessed and the value of ModelQuality
* is CANNOT_DETERMINE_QUALITY
. In this situation, you can get a model quality assessment by
* adding labels to the input dataset and retraining the model.
*
*
* For information about using labels with your models, see Understanding labeling.
*
*
* For information about improving the quality of a model, see Best practices with
* Amazon Lookout for Equipment.
* @see ModelQuality
*/
public void setModelQuality(String modelQuality) {
this.modelQuality = modelQuality;
}
/**
*
* Provides a quality assessment for a model that uses labels. If Lookout for Equipment determines that the model
* quality is poor based on training metrics, the value is POOR_QUALITY_DETECTED
. Otherwise, the value
* is QUALITY_THRESHOLD_MET
.
*
*
* If the model is unlabeled, the model quality can't be assessed and the value of ModelQuality
is
* CANNOT_DETERMINE_QUALITY
. In this situation, you can get a model quality assessment by adding labels
* to the input dataset and retraining the model.
*
*
* For information about using labels with your models, see Understanding
* labeling.
*
*
* For information about improving the quality of a model, see Best practices with Amazon
* Lookout for Equipment.
*
*
* @return Provides a quality assessment for a model that uses labels. If Lookout for Equipment determines that the
* model quality is poor based on training metrics, the value is POOR_QUALITY_DETECTED
.
* Otherwise, the value is QUALITY_THRESHOLD_MET
.
*
* If the model is unlabeled, the model quality can't be assessed and the value of ModelQuality
* is CANNOT_DETERMINE_QUALITY
. In this situation, you can get a model quality assessment by
* adding labels to the input dataset and retraining the model.
*
*
* For information about using labels with your models, see Understanding labeling.
*
*
* For information about improving the quality of a model, see Best practices
* with Amazon Lookout for Equipment.
* @see ModelQuality
*/
public String getModelQuality() {
return this.modelQuality;
}
/**
*
* Provides a quality assessment for a model that uses labels. If Lookout for Equipment determines that the model
* quality is poor based on training metrics, the value is POOR_QUALITY_DETECTED
. Otherwise, the value
* is QUALITY_THRESHOLD_MET
.
*
*
* If the model is unlabeled, the model quality can't be assessed and the value of ModelQuality
is
* CANNOT_DETERMINE_QUALITY
. In this situation, you can get a model quality assessment by adding labels
* to the input dataset and retraining the model.
*
*
* For information about using labels with your models, see Understanding
* labeling.
*
*
* For information about improving the quality of a model, see Best practices with Amazon
* Lookout for Equipment.
*
*
* @param modelQuality
* Provides a quality assessment for a model that uses labels. If Lookout for Equipment determines that the
* model quality is poor based on training metrics, the value is POOR_QUALITY_DETECTED
.
* Otherwise, the value is QUALITY_THRESHOLD_MET
.
*
* If the model is unlabeled, the model quality can't be assessed and the value of ModelQuality
* is CANNOT_DETERMINE_QUALITY
. In this situation, you can get a model quality assessment by
* adding labels to the input dataset and retraining the model.
*
*
* For information about using labels with your models, see Understanding labeling.
*
*
* For information about improving the quality of a model, see Best practices with
* Amazon Lookout for Equipment.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ModelQuality
*/
public DescribeModelResult withModelQuality(String modelQuality) {
setModelQuality(modelQuality);
return this;
}
/**
*
* Provides a quality assessment for a model that uses labels. If Lookout for Equipment determines that the model
* quality is poor based on training metrics, the value is POOR_QUALITY_DETECTED
. Otherwise, the value
* is QUALITY_THRESHOLD_MET
.
*
*
* If the model is unlabeled, the model quality can't be assessed and the value of ModelQuality
is
* CANNOT_DETERMINE_QUALITY
. In this situation, you can get a model quality assessment by adding labels
* to the input dataset and retraining the model.
*
*
* For information about using labels with your models, see Understanding
* labeling.
*
*
* For information about improving the quality of a model, see Best practices with Amazon
* Lookout for Equipment.
*
*
* @param modelQuality
* Provides a quality assessment for a model that uses labels. If Lookout for Equipment determines that the
* model quality is poor based on training metrics, the value is POOR_QUALITY_DETECTED
.
* Otherwise, the value is QUALITY_THRESHOLD_MET
.
*
* If the model is unlabeled, the model quality can't be assessed and the value of ModelQuality
* is CANNOT_DETERMINE_QUALITY
. In this situation, you can get a model quality assessment by
* adding labels to the input dataset and retraining the model.
*
*
* For information about using labels with your models, see Understanding labeling.
*
*
* For information about improving the quality of a model, see Best practices with
* Amazon Lookout for Equipment.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ModelQuality
*/
public DescribeModelResult withModelQuality(ModelQuality modelQuality) {
this.modelQuality = modelQuality.toString();
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getModelName() != null)
sb.append("ModelName: ").append(getModelName()).append(",");
if (getModelArn() != null)
sb.append("ModelArn: ").append(getModelArn()).append(",");
if (getDatasetName() != null)
sb.append("DatasetName: ").append(getDatasetName()).append(",");
if (getDatasetArn() != null)
sb.append("DatasetArn: ").append(getDatasetArn()).append(",");
if (getSchema() != null)
sb.append("Schema: ").append(getSchema()).append(",");
if (getLabelsInputConfiguration() != null)
sb.append("LabelsInputConfiguration: ").append(getLabelsInputConfiguration()).append(",");
if (getTrainingDataStartTime() != null)
sb.append("TrainingDataStartTime: ").append(getTrainingDataStartTime()).append(",");
if (getTrainingDataEndTime() != null)
sb.append("TrainingDataEndTime: ").append(getTrainingDataEndTime()).append(",");
if (getEvaluationDataStartTime() != null)
sb.append("EvaluationDataStartTime: ").append(getEvaluationDataStartTime()).append(",");
if (getEvaluationDataEndTime() != null)
sb.append("EvaluationDataEndTime: ").append(getEvaluationDataEndTime()).append(",");
if (getRoleArn() != null)
sb.append("RoleArn: ").append(getRoleArn()).append(",");
if (getDataPreProcessingConfiguration() != null)
sb.append("DataPreProcessingConfiguration: ").append(getDataPreProcessingConfiguration()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getTrainingExecutionStartTime() != null)
sb.append("TrainingExecutionStartTime: ").append(getTrainingExecutionStartTime()).append(",");
if (getTrainingExecutionEndTime() != null)
sb.append("TrainingExecutionEndTime: ").append(getTrainingExecutionEndTime()).append(",");
if (getFailedReason() != null)
sb.append("FailedReason: ").append(getFailedReason()).append(",");
if (getModelMetrics() != null)
sb.append("ModelMetrics: ").append(getModelMetrics()).append(",");
if (getLastUpdatedTime() != null)
sb.append("LastUpdatedTime: ").append(getLastUpdatedTime()).append(",");
if (getCreatedAt() != null)
sb.append("CreatedAt: ").append(getCreatedAt()).append(",");
if (getServerSideKmsKeyId() != null)
sb.append("ServerSideKmsKeyId: ").append(getServerSideKmsKeyId()).append(",");
if (getOffCondition() != null)
sb.append("OffCondition: ").append(getOffCondition()).append(",");
if (getSourceModelVersionArn() != null)
sb.append("SourceModelVersionArn: ").append(getSourceModelVersionArn()).append(",");
if (getImportJobStartTime() != null)
sb.append("ImportJobStartTime: ").append(getImportJobStartTime()).append(",");
if (getImportJobEndTime() != null)
sb.append("ImportJobEndTime: ").append(getImportJobEndTime()).append(",");
if (getActiveModelVersion() != null)
sb.append("ActiveModelVersion: ").append(getActiveModelVersion()).append(",");
if (getActiveModelVersionArn() != null)
sb.append("ActiveModelVersionArn: ").append(getActiveModelVersionArn()).append(",");
if (getModelVersionActivatedAt() != null)
sb.append("ModelVersionActivatedAt: ").append(getModelVersionActivatedAt()).append(",");
if (getPreviousActiveModelVersion() != null)
sb.append("PreviousActiveModelVersion: ").append(getPreviousActiveModelVersion()).append(",");
if (getPreviousActiveModelVersionArn() != null)
sb.append("PreviousActiveModelVersionArn: ").append(getPreviousActiveModelVersionArn()).append(",");
if (getPreviousModelVersionActivatedAt() != null)
sb.append("PreviousModelVersionActivatedAt: ").append(getPreviousModelVersionActivatedAt()).append(",");
if (getPriorModelMetrics() != null)
sb.append("PriorModelMetrics: ").append(getPriorModelMetrics()).append(",");
if (getLatestScheduledRetrainingFailedReason() != null)
sb.append("LatestScheduledRetrainingFailedReason: ").append(getLatestScheduledRetrainingFailedReason()).append(",");
if (getLatestScheduledRetrainingStatus() != null)
sb.append("LatestScheduledRetrainingStatus: ").append(getLatestScheduledRetrainingStatus()).append(",");
if (getLatestScheduledRetrainingModelVersion() != null)
sb.append("LatestScheduledRetrainingModelVersion: ").append(getLatestScheduledRetrainingModelVersion()).append(",");
if (getLatestScheduledRetrainingStartTime() != null)
sb.append("LatestScheduledRetrainingStartTime: ").append(getLatestScheduledRetrainingStartTime()).append(",");
if (getLatestScheduledRetrainingAvailableDataInDays() != null)
sb.append("LatestScheduledRetrainingAvailableDataInDays: ").append(getLatestScheduledRetrainingAvailableDataInDays()).append(",");
if (getNextScheduledRetrainingStartDate() != null)
sb.append("NextScheduledRetrainingStartDate: ").append(getNextScheduledRetrainingStartDate()).append(",");
if (getAccumulatedInferenceDataStartTime() != null)
sb.append("AccumulatedInferenceDataStartTime: ").append(getAccumulatedInferenceDataStartTime()).append(",");
if (getAccumulatedInferenceDataEndTime() != null)
sb.append("AccumulatedInferenceDataEndTime: ").append(getAccumulatedInferenceDataEndTime()).append(",");
if (getRetrainingSchedulerStatus() != null)
sb.append("RetrainingSchedulerStatus: ").append(getRetrainingSchedulerStatus()).append(",");
if (getModelDiagnosticsOutputConfiguration() != null)
sb.append("ModelDiagnosticsOutputConfiguration: ").append(getModelDiagnosticsOutputConfiguration()).append(",");
if (getModelQuality() != null)
sb.append("ModelQuality: ").append(getModelQuality());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof DescribeModelResult == false)
return false;
DescribeModelResult other = (DescribeModelResult) obj;
if (other.getModelName() == null ^ this.getModelName() == null)
return false;
if (other.getModelName() != null && other.getModelName().equals(this.getModelName()) == false)
return false;
if (other.getModelArn() == null ^ this.getModelArn() == null)
return false;
if (other.getModelArn() != null && other.getModelArn().equals(this.getModelArn()) == false)
return false;
if (other.getDatasetName() == null ^ this.getDatasetName() == null)
return false;
if (other.getDatasetName() != null && other.getDatasetName().equals(this.getDatasetName()) == false)
return false;
if (other.getDatasetArn() == null ^ this.getDatasetArn() == null)
return false;
if (other.getDatasetArn() != null && other.getDatasetArn().equals(this.getDatasetArn()) == false)
return false;
if (other.getSchema() == null ^ this.getSchema() == null)
return false;
if (other.getSchema() != null && other.getSchema().equals(this.getSchema()) == false)
return false;
if (other.getLabelsInputConfiguration() == null ^ this.getLabelsInputConfiguration() == null)
return false;
if (other.getLabelsInputConfiguration() != null && other.getLabelsInputConfiguration().equals(this.getLabelsInputConfiguration()) == false)
return false;
if (other.getTrainingDataStartTime() == null ^ this.getTrainingDataStartTime() == null)
return false;
if (other.getTrainingDataStartTime() != null && other.getTrainingDataStartTime().equals(this.getTrainingDataStartTime()) == false)
return false;
if (other.getTrainingDataEndTime() == null ^ this.getTrainingDataEndTime() == null)
return false;
if (other.getTrainingDataEndTime() != null && other.getTrainingDataEndTime().equals(this.getTrainingDataEndTime()) == false)
return false;
if (other.getEvaluationDataStartTime() == null ^ this.getEvaluationDataStartTime() == null)
return false;
if (other.getEvaluationDataStartTime() != null && other.getEvaluationDataStartTime().equals(this.getEvaluationDataStartTime()) == false)
return false;
if (other.getEvaluationDataEndTime() == null ^ this.getEvaluationDataEndTime() == null)
return false;
if (other.getEvaluationDataEndTime() != null && other.getEvaluationDataEndTime().equals(this.getEvaluationDataEndTime()) == false)
return false;
if (other.getRoleArn() == null ^ this.getRoleArn() == null)
return false;
if (other.getRoleArn() != null && other.getRoleArn().equals(this.getRoleArn()) == false)
return false;
if (other.getDataPreProcessingConfiguration() == null ^ this.getDataPreProcessingConfiguration() == null)
return false;
if (other.getDataPreProcessingConfiguration() != null
&& other.getDataPreProcessingConfiguration().equals(this.getDataPreProcessingConfiguration()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getTrainingExecutionStartTime() == null ^ this.getTrainingExecutionStartTime() == null)
return false;
if (other.getTrainingExecutionStartTime() != null && other.getTrainingExecutionStartTime().equals(this.getTrainingExecutionStartTime()) == false)
return false;
if (other.getTrainingExecutionEndTime() == null ^ this.getTrainingExecutionEndTime() == null)
return false;
if (other.getTrainingExecutionEndTime() != null && other.getTrainingExecutionEndTime().equals(this.getTrainingExecutionEndTime()) == false)
return false;
if (other.getFailedReason() == null ^ this.getFailedReason() == null)
return false;
if (other.getFailedReason() != null && other.getFailedReason().equals(this.getFailedReason()) == false)
return false;
if (other.getModelMetrics() == null ^ this.getModelMetrics() == null)
return false;
if (other.getModelMetrics() != null && other.getModelMetrics().equals(this.getModelMetrics()) == false)
return false;
if (other.getLastUpdatedTime() == null ^ this.getLastUpdatedTime() == null)
return false;
if (other.getLastUpdatedTime() != null && other.getLastUpdatedTime().equals(this.getLastUpdatedTime()) == false)
return false;
if (other.getCreatedAt() == null ^ this.getCreatedAt() == null)
return false;
if (other.getCreatedAt() != null && other.getCreatedAt().equals(this.getCreatedAt()) == false)
return false;
if (other.getServerSideKmsKeyId() == null ^ this.getServerSideKmsKeyId() == null)
return false;
if (other.getServerSideKmsKeyId() != null && other.getServerSideKmsKeyId().equals(this.getServerSideKmsKeyId()) == false)
return false;
if (other.getOffCondition() == null ^ this.getOffCondition() == null)
return false;
if (other.getOffCondition() != null && other.getOffCondition().equals(this.getOffCondition()) == false)
return false;
if (other.getSourceModelVersionArn() == null ^ this.getSourceModelVersionArn() == null)
return false;
if (other.getSourceModelVersionArn() != null && other.getSourceModelVersionArn().equals(this.getSourceModelVersionArn()) == false)
return false;
if (other.getImportJobStartTime() == null ^ this.getImportJobStartTime() == null)
return false;
if (other.getImportJobStartTime() != null && other.getImportJobStartTime().equals(this.getImportJobStartTime()) == false)
return false;
if (other.getImportJobEndTime() == null ^ this.getImportJobEndTime() == null)
return false;
if (other.getImportJobEndTime() != null && other.getImportJobEndTime().equals(this.getImportJobEndTime()) == false)
return false;
if (other.getActiveModelVersion() == null ^ this.getActiveModelVersion() == null)
return false;
if (other.getActiveModelVersion() != null && other.getActiveModelVersion().equals(this.getActiveModelVersion()) == false)
return false;
if (other.getActiveModelVersionArn() == null ^ this.getActiveModelVersionArn() == null)
return false;
if (other.getActiveModelVersionArn() != null && other.getActiveModelVersionArn().equals(this.getActiveModelVersionArn()) == false)
return false;
if (other.getModelVersionActivatedAt() == null ^ this.getModelVersionActivatedAt() == null)
return false;
if (other.getModelVersionActivatedAt() != null && other.getModelVersionActivatedAt().equals(this.getModelVersionActivatedAt()) == false)
return false;
if (other.getPreviousActiveModelVersion() == null ^ this.getPreviousActiveModelVersion() == null)
return false;
if (other.getPreviousActiveModelVersion() != null && other.getPreviousActiveModelVersion().equals(this.getPreviousActiveModelVersion()) == false)
return false;
if (other.getPreviousActiveModelVersionArn() == null ^ this.getPreviousActiveModelVersionArn() == null)
return false;
if (other.getPreviousActiveModelVersionArn() != null
&& other.getPreviousActiveModelVersionArn().equals(this.getPreviousActiveModelVersionArn()) == false)
return false;
if (other.getPreviousModelVersionActivatedAt() == null ^ this.getPreviousModelVersionActivatedAt() == null)
return false;
if (other.getPreviousModelVersionActivatedAt() != null
&& other.getPreviousModelVersionActivatedAt().equals(this.getPreviousModelVersionActivatedAt()) == false)
return false;
if (other.getPriorModelMetrics() == null ^ this.getPriorModelMetrics() == null)
return false;
if (other.getPriorModelMetrics() != null && other.getPriorModelMetrics().equals(this.getPriorModelMetrics()) == false)
return false;
if (other.getLatestScheduledRetrainingFailedReason() == null ^ this.getLatestScheduledRetrainingFailedReason() == null)
return false;
if (other.getLatestScheduledRetrainingFailedReason() != null
&& other.getLatestScheduledRetrainingFailedReason().equals(this.getLatestScheduledRetrainingFailedReason()) == false)
return false;
if (other.getLatestScheduledRetrainingStatus() == null ^ this.getLatestScheduledRetrainingStatus() == null)
return false;
if (other.getLatestScheduledRetrainingStatus() != null
&& other.getLatestScheduledRetrainingStatus().equals(this.getLatestScheduledRetrainingStatus()) == false)
return false;
if (other.getLatestScheduledRetrainingModelVersion() == null ^ this.getLatestScheduledRetrainingModelVersion() == null)
return false;
if (other.getLatestScheduledRetrainingModelVersion() != null
&& other.getLatestScheduledRetrainingModelVersion().equals(this.getLatestScheduledRetrainingModelVersion()) == false)
return false;
if (other.getLatestScheduledRetrainingStartTime() == null ^ this.getLatestScheduledRetrainingStartTime() == null)
return false;
if (other.getLatestScheduledRetrainingStartTime() != null
&& other.getLatestScheduledRetrainingStartTime().equals(this.getLatestScheduledRetrainingStartTime()) == false)
return false;
if (other.getLatestScheduledRetrainingAvailableDataInDays() == null ^ this.getLatestScheduledRetrainingAvailableDataInDays() == null)
return false;
if (other.getLatestScheduledRetrainingAvailableDataInDays() != null
&& other.getLatestScheduledRetrainingAvailableDataInDays().equals(this.getLatestScheduledRetrainingAvailableDataInDays()) == false)
return false;
if (other.getNextScheduledRetrainingStartDate() == null ^ this.getNextScheduledRetrainingStartDate() == null)
return false;
if (other.getNextScheduledRetrainingStartDate() != null
&& other.getNextScheduledRetrainingStartDate().equals(this.getNextScheduledRetrainingStartDate()) == false)
return false;
if (other.getAccumulatedInferenceDataStartTime() == null ^ this.getAccumulatedInferenceDataStartTime() == null)
return false;
if (other.getAccumulatedInferenceDataStartTime() != null
&& other.getAccumulatedInferenceDataStartTime().equals(this.getAccumulatedInferenceDataStartTime()) == false)
return false;
if (other.getAccumulatedInferenceDataEndTime() == null ^ this.getAccumulatedInferenceDataEndTime() == null)
return false;
if (other.getAccumulatedInferenceDataEndTime() != null
&& other.getAccumulatedInferenceDataEndTime().equals(this.getAccumulatedInferenceDataEndTime()) == false)
return false;
if (other.getRetrainingSchedulerStatus() == null ^ this.getRetrainingSchedulerStatus() == null)
return false;
if (other.getRetrainingSchedulerStatus() != null && other.getRetrainingSchedulerStatus().equals(this.getRetrainingSchedulerStatus()) == false)
return false;
if (other.getModelDiagnosticsOutputConfiguration() == null ^ this.getModelDiagnosticsOutputConfiguration() == null)
return false;
if (other.getModelDiagnosticsOutputConfiguration() != null
&& other.getModelDiagnosticsOutputConfiguration().equals(this.getModelDiagnosticsOutputConfiguration()) == false)
return false;
if (other.getModelQuality() == null ^ this.getModelQuality() == null)
return false;
if (other.getModelQuality() != null && other.getModelQuality().equals(this.getModelQuality()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getModelName() == null) ? 0 : getModelName().hashCode());
hashCode = prime * hashCode + ((getModelArn() == null) ? 0 : getModelArn().hashCode());
hashCode = prime * hashCode + ((getDatasetName() == null) ? 0 : getDatasetName().hashCode());
hashCode = prime * hashCode + ((getDatasetArn() == null) ? 0 : getDatasetArn().hashCode());
hashCode = prime * hashCode + ((getSchema() == null) ? 0 : getSchema().hashCode());
hashCode = prime * hashCode + ((getLabelsInputConfiguration() == null) ? 0 : getLabelsInputConfiguration().hashCode());
hashCode = prime * hashCode + ((getTrainingDataStartTime() == null) ? 0 : getTrainingDataStartTime().hashCode());
hashCode = prime * hashCode + ((getTrainingDataEndTime() == null) ? 0 : getTrainingDataEndTime().hashCode());
hashCode = prime * hashCode + ((getEvaluationDataStartTime() == null) ? 0 : getEvaluationDataStartTime().hashCode());
hashCode = prime * hashCode + ((getEvaluationDataEndTime() == null) ? 0 : getEvaluationDataEndTime().hashCode());
hashCode = prime * hashCode + ((getRoleArn() == null) ? 0 : getRoleArn().hashCode());
hashCode = prime * hashCode + ((getDataPreProcessingConfiguration() == null) ? 0 : getDataPreProcessingConfiguration().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getTrainingExecutionStartTime() == null) ? 0 : getTrainingExecutionStartTime().hashCode());
hashCode = prime * hashCode + ((getTrainingExecutionEndTime() == null) ? 0 : getTrainingExecutionEndTime().hashCode());
hashCode = prime * hashCode + ((getFailedReason() == null) ? 0 : getFailedReason().hashCode());
hashCode = prime * hashCode + ((getModelMetrics() == null) ? 0 : getModelMetrics().hashCode());
hashCode = prime * hashCode + ((getLastUpdatedTime() == null) ? 0 : getLastUpdatedTime().hashCode());
hashCode = prime * hashCode + ((getCreatedAt() == null) ? 0 : getCreatedAt().hashCode());
hashCode = prime * hashCode + ((getServerSideKmsKeyId() == null) ? 0 : getServerSideKmsKeyId().hashCode());
hashCode = prime * hashCode + ((getOffCondition() == null) ? 0 : getOffCondition().hashCode());
hashCode = prime * hashCode + ((getSourceModelVersionArn() == null) ? 0 : getSourceModelVersionArn().hashCode());
hashCode = prime * hashCode + ((getImportJobStartTime() == null) ? 0 : getImportJobStartTime().hashCode());
hashCode = prime * hashCode + ((getImportJobEndTime() == null) ? 0 : getImportJobEndTime().hashCode());
hashCode = prime * hashCode + ((getActiveModelVersion() == null) ? 0 : getActiveModelVersion().hashCode());
hashCode = prime * hashCode + ((getActiveModelVersionArn() == null) ? 0 : getActiveModelVersionArn().hashCode());
hashCode = prime * hashCode + ((getModelVersionActivatedAt() == null) ? 0 : getModelVersionActivatedAt().hashCode());
hashCode = prime * hashCode + ((getPreviousActiveModelVersion() == null) ? 0 : getPreviousActiveModelVersion().hashCode());
hashCode = prime * hashCode + ((getPreviousActiveModelVersionArn() == null) ? 0 : getPreviousActiveModelVersionArn().hashCode());
hashCode = prime * hashCode + ((getPreviousModelVersionActivatedAt() == null) ? 0 : getPreviousModelVersionActivatedAt().hashCode());
hashCode = prime * hashCode + ((getPriorModelMetrics() == null) ? 0 : getPriorModelMetrics().hashCode());
hashCode = prime * hashCode + ((getLatestScheduledRetrainingFailedReason() == null) ? 0 : getLatestScheduledRetrainingFailedReason().hashCode());
hashCode = prime * hashCode + ((getLatestScheduledRetrainingStatus() == null) ? 0 : getLatestScheduledRetrainingStatus().hashCode());
hashCode = prime * hashCode + ((getLatestScheduledRetrainingModelVersion() == null) ? 0 : getLatestScheduledRetrainingModelVersion().hashCode());
hashCode = prime * hashCode + ((getLatestScheduledRetrainingStartTime() == null) ? 0 : getLatestScheduledRetrainingStartTime().hashCode());
hashCode = prime * hashCode
+ ((getLatestScheduledRetrainingAvailableDataInDays() == null) ? 0 : getLatestScheduledRetrainingAvailableDataInDays().hashCode());
hashCode = prime * hashCode + ((getNextScheduledRetrainingStartDate() == null) ? 0 : getNextScheduledRetrainingStartDate().hashCode());
hashCode = prime * hashCode + ((getAccumulatedInferenceDataStartTime() == null) ? 0 : getAccumulatedInferenceDataStartTime().hashCode());
hashCode = prime * hashCode + ((getAccumulatedInferenceDataEndTime() == null) ? 0 : getAccumulatedInferenceDataEndTime().hashCode());
hashCode = prime * hashCode + ((getRetrainingSchedulerStatus() == null) ? 0 : getRetrainingSchedulerStatus().hashCode());
hashCode = prime * hashCode + ((getModelDiagnosticsOutputConfiguration() == null) ? 0 : getModelDiagnosticsOutputConfiguration().hashCode());
hashCode = prime * hashCode + ((getModelQuality() == null) ? 0 : getModelQuality().hashCode());
return hashCode;
}
@Override
public DescribeModelResult clone() {
try {
return (DescribeModelResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}