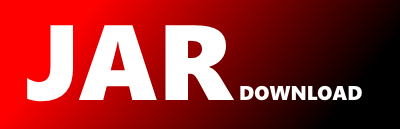
com.amazonaws.services.lookoutequipment.model.ModelSummary Maven / Gradle / Ivy
Show all versions of aws-java-sdk-lookoutequipment Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.lookoutequipment.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Provides information about the specified machine learning model, including dataset and model names and ARNs, as well
* as status.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ModelSummary implements Serializable, Cloneable, StructuredPojo {
/**
*
* The name of the machine learning model.
*
*/
private String modelName;
/**
*
* The Amazon Resource Name (ARN) of the machine learning model.
*
*/
private String modelArn;
/**
*
* The name of the dataset being used for the machine learning model.
*
*/
private String datasetName;
/**
*
* The Amazon Resource Name (ARN) of the dataset used to create the model.
*
*/
private String datasetArn;
/**
*
* Indicates the status of the machine learning model.
*
*/
private String status;
/**
*
* The time at which the specific model was created.
*
*/
private java.util.Date createdAt;
/**
*
* The model version that the inference scheduler uses to run an inference execution.
*
*/
private Long activeModelVersion;
/**
*
* The Amazon Resource Name (ARN) of the model version that is set as active. The active model version is the model
* version that the inference scheduler uses to run an inference execution.
*
*/
private String activeModelVersionArn;
/**
*
* Indicates the status of the most recent scheduled retraining run.
*
*/
private String latestScheduledRetrainingStatus;
/**
*
* Indicates the most recent model version that was generated by retraining.
*
*/
private Long latestScheduledRetrainingModelVersion;
/**
*
* Indicates the start time of the most recent scheduled retraining run.
*
*/
private java.util.Date latestScheduledRetrainingStartTime;
/**
*
* Indicates the date that the next scheduled retraining run will start on. Lookout for Equipment truncates the time
* you provide to the nearest UTC day.
*
*/
private java.util.Date nextScheduledRetrainingStartDate;
/**
*
* Indicates the status of the retraining scheduler.
*
*/
private String retrainingSchedulerStatus;
private ModelDiagnosticsOutputConfiguration modelDiagnosticsOutputConfiguration;
/**
*
* Provides a quality assessment for a model that uses labels. If Lookout for Equipment determines that the model
* quality is poor based on training metrics, the value is POOR_QUALITY_DETECTED
. Otherwise, the value
* is QUALITY_THRESHOLD_MET
.
*
*
* If the model is unlabeled, the model quality can't be assessed and the value of ModelQuality
is
* CANNOT_DETERMINE_QUALITY
. In this situation, you can get a model quality assessment by adding labels
* to the input dataset and retraining the model.
*
*
* For information about using labels with your models, see Understanding
* labeling.
*
*
* For information about improving the quality of a model, see Best practices with Amazon
* Lookout for Equipment.
*
*/
private String modelQuality;
/**
*
* The name of the machine learning model.
*
*
* @param modelName
* The name of the machine learning model.
*/
public void setModelName(String modelName) {
this.modelName = modelName;
}
/**
*
* The name of the machine learning model.
*
*
* @return The name of the machine learning model.
*/
public String getModelName() {
return this.modelName;
}
/**
*
* The name of the machine learning model.
*
*
* @param modelName
* The name of the machine learning model.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModelSummary withModelName(String modelName) {
setModelName(modelName);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the machine learning model.
*
*
* @param modelArn
* The Amazon Resource Name (ARN) of the machine learning model.
*/
public void setModelArn(String modelArn) {
this.modelArn = modelArn;
}
/**
*
* The Amazon Resource Name (ARN) of the machine learning model.
*
*
* @return The Amazon Resource Name (ARN) of the machine learning model.
*/
public String getModelArn() {
return this.modelArn;
}
/**
*
* The Amazon Resource Name (ARN) of the machine learning model.
*
*
* @param modelArn
* The Amazon Resource Name (ARN) of the machine learning model.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModelSummary withModelArn(String modelArn) {
setModelArn(modelArn);
return this;
}
/**
*
* The name of the dataset being used for the machine learning model.
*
*
* @param datasetName
* The name of the dataset being used for the machine learning model.
*/
public void setDatasetName(String datasetName) {
this.datasetName = datasetName;
}
/**
*
* The name of the dataset being used for the machine learning model.
*
*
* @return The name of the dataset being used for the machine learning model.
*/
public String getDatasetName() {
return this.datasetName;
}
/**
*
* The name of the dataset being used for the machine learning model.
*
*
* @param datasetName
* The name of the dataset being used for the machine learning model.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModelSummary withDatasetName(String datasetName) {
setDatasetName(datasetName);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the dataset used to create the model.
*
*
* @param datasetArn
* The Amazon Resource Name (ARN) of the dataset used to create the model.
*/
public void setDatasetArn(String datasetArn) {
this.datasetArn = datasetArn;
}
/**
*
* The Amazon Resource Name (ARN) of the dataset used to create the model.
*
*
* @return The Amazon Resource Name (ARN) of the dataset used to create the model.
*/
public String getDatasetArn() {
return this.datasetArn;
}
/**
*
* The Amazon Resource Name (ARN) of the dataset used to create the model.
*
*
* @param datasetArn
* The Amazon Resource Name (ARN) of the dataset used to create the model.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModelSummary withDatasetArn(String datasetArn) {
setDatasetArn(datasetArn);
return this;
}
/**
*
* Indicates the status of the machine learning model.
*
*
* @param status
* Indicates the status of the machine learning model.
* @see ModelStatus
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* Indicates the status of the machine learning model.
*
*
* @return Indicates the status of the machine learning model.
* @see ModelStatus
*/
public String getStatus() {
return this.status;
}
/**
*
* Indicates the status of the machine learning model.
*
*
* @param status
* Indicates the status of the machine learning model.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ModelStatus
*/
public ModelSummary withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* Indicates the status of the machine learning model.
*
*
* @param status
* Indicates the status of the machine learning model.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ModelStatus
*/
public ModelSummary withStatus(ModelStatus status) {
this.status = status.toString();
return this;
}
/**
*
* The time at which the specific model was created.
*
*
* @param createdAt
* The time at which the specific model was created.
*/
public void setCreatedAt(java.util.Date createdAt) {
this.createdAt = createdAt;
}
/**
*
* The time at which the specific model was created.
*
*
* @return The time at which the specific model was created.
*/
public java.util.Date getCreatedAt() {
return this.createdAt;
}
/**
*
* The time at which the specific model was created.
*
*
* @param createdAt
* The time at which the specific model was created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModelSummary withCreatedAt(java.util.Date createdAt) {
setCreatedAt(createdAt);
return this;
}
/**
*
* The model version that the inference scheduler uses to run an inference execution.
*
*
* @param activeModelVersion
* The model version that the inference scheduler uses to run an inference execution.
*/
public void setActiveModelVersion(Long activeModelVersion) {
this.activeModelVersion = activeModelVersion;
}
/**
*
* The model version that the inference scheduler uses to run an inference execution.
*
*
* @return The model version that the inference scheduler uses to run an inference execution.
*/
public Long getActiveModelVersion() {
return this.activeModelVersion;
}
/**
*
* The model version that the inference scheduler uses to run an inference execution.
*
*
* @param activeModelVersion
* The model version that the inference scheduler uses to run an inference execution.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModelSummary withActiveModelVersion(Long activeModelVersion) {
setActiveModelVersion(activeModelVersion);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the model version that is set as active. The active model version is the model
* version that the inference scheduler uses to run an inference execution.
*
*
* @param activeModelVersionArn
* The Amazon Resource Name (ARN) of the model version that is set as active. The active model version is the
* model version that the inference scheduler uses to run an inference execution.
*/
public void setActiveModelVersionArn(String activeModelVersionArn) {
this.activeModelVersionArn = activeModelVersionArn;
}
/**
*
* The Amazon Resource Name (ARN) of the model version that is set as active. The active model version is the model
* version that the inference scheduler uses to run an inference execution.
*
*
* @return The Amazon Resource Name (ARN) of the model version that is set as active. The active model version is
* the model version that the inference scheduler uses to run an inference execution.
*/
public String getActiveModelVersionArn() {
return this.activeModelVersionArn;
}
/**
*
* The Amazon Resource Name (ARN) of the model version that is set as active. The active model version is the model
* version that the inference scheduler uses to run an inference execution.
*
*
* @param activeModelVersionArn
* The Amazon Resource Name (ARN) of the model version that is set as active. The active model version is the
* model version that the inference scheduler uses to run an inference execution.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModelSummary withActiveModelVersionArn(String activeModelVersionArn) {
setActiveModelVersionArn(activeModelVersionArn);
return this;
}
/**
*
* Indicates the status of the most recent scheduled retraining run.
*
*
* @param latestScheduledRetrainingStatus
* Indicates the status of the most recent scheduled retraining run.
* @see ModelVersionStatus
*/
public void setLatestScheduledRetrainingStatus(String latestScheduledRetrainingStatus) {
this.latestScheduledRetrainingStatus = latestScheduledRetrainingStatus;
}
/**
*
* Indicates the status of the most recent scheduled retraining run.
*
*
* @return Indicates the status of the most recent scheduled retraining run.
* @see ModelVersionStatus
*/
public String getLatestScheduledRetrainingStatus() {
return this.latestScheduledRetrainingStatus;
}
/**
*
* Indicates the status of the most recent scheduled retraining run.
*
*
* @param latestScheduledRetrainingStatus
* Indicates the status of the most recent scheduled retraining run.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ModelVersionStatus
*/
public ModelSummary withLatestScheduledRetrainingStatus(String latestScheduledRetrainingStatus) {
setLatestScheduledRetrainingStatus(latestScheduledRetrainingStatus);
return this;
}
/**
*
* Indicates the status of the most recent scheduled retraining run.
*
*
* @param latestScheduledRetrainingStatus
* Indicates the status of the most recent scheduled retraining run.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ModelVersionStatus
*/
public ModelSummary withLatestScheduledRetrainingStatus(ModelVersionStatus latestScheduledRetrainingStatus) {
this.latestScheduledRetrainingStatus = latestScheduledRetrainingStatus.toString();
return this;
}
/**
*
* Indicates the most recent model version that was generated by retraining.
*
*
* @param latestScheduledRetrainingModelVersion
* Indicates the most recent model version that was generated by retraining.
*/
public void setLatestScheduledRetrainingModelVersion(Long latestScheduledRetrainingModelVersion) {
this.latestScheduledRetrainingModelVersion = latestScheduledRetrainingModelVersion;
}
/**
*
* Indicates the most recent model version that was generated by retraining.
*
*
* @return Indicates the most recent model version that was generated by retraining.
*/
public Long getLatestScheduledRetrainingModelVersion() {
return this.latestScheduledRetrainingModelVersion;
}
/**
*
* Indicates the most recent model version that was generated by retraining.
*
*
* @param latestScheduledRetrainingModelVersion
* Indicates the most recent model version that was generated by retraining.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModelSummary withLatestScheduledRetrainingModelVersion(Long latestScheduledRetrainingModelVersion) {
setLatestScheduledRetrainingModelVersion(latestScheduledRetrainingModelVersion);
return this;
}
/**
*
* Indicates the start time of the most recent scheduled retraining run.
*
*
* @param latestScheduledRetrainingStartTime
* Indicates the start time of the most recent scheduled retraining run.
*/
public void setLatestScheduledRetrainingStartTime(java.util.Date latestScheduledRetrainingStartTime) {
this.latestScheduledRetrainingStartTime = latestScheduledRetrainingStartTime;
}
/**
*
* Indicates the start time of the most recent scheduled retraining run.
*
*
* @return Indicates the start time of the most recent scheduled retraining run.
*/
public java.util.Date getLatestScheduledRetrainingStartTime() {
return this.latestScheduledRetrainingStartTime;
}
/**
*
* Indicates the start time of the most recent scheduled retraining run.
*
*
* @param latestScheduledRetrainingStartTime
* Indicates the start time of the most recent scheduled retraining run.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModelSummary withLatestScheduledRetrainingStartTime(java.util.Date latestScheduledRetrainingStartTime) {
setLatestScheduledRetrainingStartTime(latestScheduledRetrainingStartTime);
return this;
}
/**
*
* Indicates the date that the next scheduled retraining run will start on. Lookout for Equipment truncates the time
* you provide to the nearest UTC day.
*
*
* @param nextScheduledRetrainingStartDate
* Indicates the date that the next scheduled retraining run will start on. Lookout for Equipment truncates
* the time you provide to the nearest UTC day.
*/
public void setNextScheduledRetrainingStartDate(java.util.Date nextScheduledRetrainingStartDate) {
this.nextScheduledRetrainingStartDate = nextScheduledRetrainingStartDate;
}
/**
*
* Indicates the date that the next scheduled retraining run will start on. Lookout for Equipment truncates the time
* you provide to the nearest UTC day.
*
*
* @return Indicates the date that the next scheduled retraining run will start on. Lookout for Equipment truncates
* the time you provide to the nearest UTC day.
*/
public java.util.Date getNextScheduledRetrainingStartDate() {
return this.nextScheduledRetrainingStartDate;
}
/**
*
* Indicates the date that the next scheduled retraining run will start on. Lookout for Equipment truncates the time
* you provide to the nearest UTC day.
*
*
* @param nextScheduledRetrainingStartDate
* Indicates the date that the next scheduled retraining run will start on. Lookout for Equipment truncates
* the time you provide to the nearest UTC day.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModelSummary withNextScheduledRetrainingStartDate(java.util.Date nextScheduledRetrainingStartDate) {
setNextScheduledRetrainingStartDate(nextScheduledRetrainingStartDate);
return this;
}
/**
*
* Indicates the status of the retraining scheduler.
*
*
* @param retrainingSchedulerStatus
* Indicates the status of the retraining scheduler.
* @see RetrainingSchedulerStatus
*/
public void setRetrainingSchedulerStatus(String retrainingSchedulerStatus) {
this.retrainingSchedulerStatus = retrainingSchedulerStatus;
}
/**
*
* Indicates the status of the retraining scheduler.
*
*
* @return Indicates the status of the retraining scheduler.
* @see RetrainingSchedulerStatus
*/
public String getRetrainingSchedulerStatus() {
return this.retrainingSchedulerStatus;
}
/**
*
* Indicates the status of the retraining scheduler.
*
*
* @param retrainingSchedulerStatus
* Indicates the status of the retraining scheduler.
* @return Returns a reference to this object so that method calls can be chained together.
* @see RetrainingSchedulerStatus
*/
public ModelSummary withRetrainingSchedulerStatus(String retrainingSchedulerStatus) {
setRetrainingSchedulerStatus(retrainingSchedulerStatus);
return this;
}
/**
*
* Indicates the status of the retraining scheduler.
*
*
* @param retrainingSchedulerStatus
* Indicates the status of the retraining scheduler.
* @return Returns a reference to this object so that method calls can be chained together.
* @see RetrainingSchedulerStatus
*/
public ModelSummary withRetrainingSchedulerStatus(RetrainingSchedulerStatus retrainingSchedulerStatus) {
this.retrainingSchedulerStatus = retrainingSchedulerStatus.toString();
return this;
}
/**
* @param modelDiagnosticsOutputConfiguration
*/
public void setModelDiagnosticsOutputConfiguration(ModelDiagnosticsOutputConfiguration modelDiagnosticsOutputConfiguration) {
this.modelDiagnosticsOutputConfiguration = modelDiagnosticsOutputConfiguration;
}
/**
* @return
*/
public ModelDiagnosticsOutputConfiguration getModelDiagnosticsOutputConfiguration() {
return this.modelDiagnosticsOutputConfiguration;
}
/**
* @param modelDiagnosticsOutputConfiguration
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModelSummary withModelDiagnosticsOutputConfiguration(ModelDiagnosticsOutputConfiguration modelDiagnosticsOutputConfiguration) {
setModelDiagnosticsOutputConfiguration(modelDiagnosticsOutputConfiguration);
return this;
}
/**
*
* Provides a quality assessment for a model that uses labels. If Lookout for Equipment determines that the model
* quality is poor based on training metrics, the value is POOR_QUALITY_DETECTED
. Otherwise, the value
* is QUALITY_THRESHOLD_MET
.
*
*
* If the model is unlabeled, the model quality can't be assessed and the value of ModelQuality
is
* CANNOT_DETERMINE_QUALITY
. In this situation, you can get a model quality assessment by adding labels
* to the input dataset and retraining the model.
*
*
* For information about using labels with your models, see Understanding
* labeling.
*
*
* For information about improving the quality of a model, see Best practices with Amazon
* Lookout for Equipment.
*
*
* @param modelQuality
* Provides a quality assessment for a model that uses labels. If Lookout for Equipment determines that the
* model quality is poor based on training metrics, the value is POOR_QUALITY_DETECTED
.
* Otherwise, the value is QUALITY_THRESHOLD_MET
.
*
* If the model is unlabeled, the model quality can't be assessed and the value of ModelQuality
* is CANNOT_DETERMINE_QUALITY
. In this situation, you can get a model quality assessment by
* adding labels to the input dataset and retraining the model.
*
*
* For information about using labels with your models, see Understanding labeling.
*
*
* For information about improving the quality of a model, see Best practices with
* Amazon Lookout for Equipment.
* @see ModelQuality
*/
public void setModelQuality(String modelQuality) {
this.modelQuality = modelQuality;
}
/**
*
* Provides a quality assessment for a model that uses labels. If Lookout for Equipment determines that the model
* quality is poor based on training metrics, the value is POOR_QUALITY_DETECTED
. Otherwise, the value
* is QUALITY_THRESHOLD_MET
.
*
*
* If the model is unlabeled, the model quality can't be assessed and the value of ModelQuality
is
* CANNOT_DETERMINE_QUALITY
. In this situation, you can get a model quality assessment by adding labels
* to the input dataset and retraining the model.
*
*
* For information about using labels with your models, see Understanding
* labeling.
*
*
* For information about improving the quality of a model, see Best practices with Amazon
* Lookout for Equipment.
*
*
* @return Provides a quality assessment for a model that uses labels. If Lookout for Equipment determines that the
* model quality is poor based on training metrics, the value is POOR_QUALITY_DETECTED
.
* Otherwise, the value is QUALITY_THRESHOLD_MET
.
*
* If the model is unlabeled, the model quality can't be assessed and the value of ModelQuality
* is CANNOT_DETERMINE_QUALITY
. In this situation, you can get a model quality assessment by
* adding labels to the input dataset and retraining the model.
*
*
* For information about using labels with your models, see Understanding labeling.
*
*
* For information about improving the quality of a model, see Best practices
* with Amazon Lookout for Equipment.
* @see ModelQuality
*/
public String getModelQuality() {
return this.modelQuality;
}
/**
*
* Provides a quality assessment for a model that uses labels. If Lookout for Equipment determines that the model
* quality is poor based on training metrics, the value is POOR_QUALITY_DETECTED
. Otherwise, the value
* is QUALITY_THRESHOLD_MET
.
*
*
* If the model is unlabeled, the model quality can't be assessed and the value of ModelQuality
is
* CANNOT_DETERMINE_QUALITY
. In this situation, you can get a model quality assessment by adding labels
* to the input dataset and retraining the model.
*
*
* For information about using labels with your models, see Understanding
* labeling.
*
*
* For information about improving the quality of a model, see Best practices with Amazon
* Lookout for Equipment.
*
*
* @param modelQuality
* Provides a quality assessment for a model that uses labels. If Lookout for Equipment determines that the
* model quality is poor based on training metrics, the value is POOR_QUALITY_DETECTED
.
* Otherwise, the value is QUALITY_THRESHOLD_MET
.
*
* If the model is unlabeled, the model quality can't be assessed and the value of ModelQuality
* is CANNOT_DETERMINE_QUALITY
. In this situation, you can get a model quality assessment by
* adding labels to the input dataset and retraining the model.
*
*
* For information about using labels with your models, see Understanding labeling.
*
*
* For information about improving the quality of a model, see Best practices with
* Amazon Lookout for Equipment.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ModelQuality
*/
public ModelSummary withModelQuality(String modelQuality) {
setModelQuality(modelQuality);
return this;
}
/**
*
* Provides a quality assessment for a model that uses labels. If Lookout for Equipment determines that the model
* quality is poor based on training metrics, the value is POOR_QUALITY_DETECTED
. Otherwise, the value
* is QUALITY_THRESHOLD_MET
.
*
*
* If the model is unlabeled, the model quality can't be assessed and the value of ModelQuality
is
* CANNOT_DETERMINE_QUALITY
. In this situation, you can get a model quality assessment by adding labels
* to the input dataset and retraining the model.
*
*
* For information about using labels with your models, see Understanding
* labeling.
*
*
* For information about improving the quality of a model, see Best practices with Amazon
* Lookout for Equipment.
*
*
* @param modelQuality
* Provides a quality assessment for a model that uses labels. If Lookout for Equipment determines that the
* model quality is poor based on training metrics, the value is POOR_QUALITY_DETECTED
.
* Otherwise, the value is QUALITY_THRESHOLD_MET
.
*
* If the model is unlabeled, the model quality can't be assessed and the value of ModelQuality
* is CANNOT_DETERMINE_QUALITY
. In this situation, you can get a model quality assessment by
* adding labels to the input dataset and retraining the model.
*
*
* For information about using labels with your models, see Understanding labeling.
*
*
* For information about improving the quality of a model, see Best practices with
* Amazon Lookout for Equipment.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ModelQuality
*/
public ModelSummary withModelQuality(ModelQuality modelQuality) {
this.modelQuality = modelQuality.toString();
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getModelName() != null)
sb.append("ModelName: ").append(getModelName()).append(",");
if (getModelArn() != null)
sb.append("ModelArn: ").append(getModelArn()).append(",");
if (getDatasetName() != null)
sb.append("DatasetName: ").append(getDatasetName()).append(",");
if (getDatasetArn() != null)
sb.append("DatasetArn: ").append(getDatasetArn()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getCreatedAt() != null)
sb.append("CreatedAt: ").append(getCreatedAt()).append(",");
if (getActiveModelVersion() != null)
sb.append("ActiveModelVersion: ").append(getActiveModelVersion()).append(",");
if (getActiveModelVersionArn() != null)
sb.append("ActiveModelVersionArn: ").append(getActiveModelVersionArn()).append(",");
if (getLatestScheduledRetrainingStatus() != null)
sb.append("LatestScheduledRetrainingStatus: ").append(getLatestScheduledRetrainingStatus()).append(",");
if (getLatestScheduledRetrainingModelVersion() != null)
sb.append("LatestScheduledRetrainingModelVersion: ").append(getLatestScheduledRetrainingModelVersion()).append(",");
if (getLatestScheduledRetrainingStartTime() != null)
sb.append("LatestScheduledRetrainingStartTime: ").append(getLatestScheduledRetrainingStartTime()).append(",");
if (getNextScheduledRetrainingStartDate() != null)
sb.append("NextScheduledRetrainingStartDate: ").append(getNextScheduledRetrainingStartDate()).append(",");
if (getRetrainingSchedulerStatus() != null)
sb.append("RetrainingSchedulerStatus: ").append(getRetrainingSchedulerStatus()).append(",");
if (getModelDiagnosticsOutputConfiguration() != null)
sb.append("ModelDiagnosticsOutputConfiguration: ").append(getModelDiagnosticsOutputConfiguration()).append(",");
if (getModelQuality() != null)
sb.append("ModelQuality: ").append(getModelQuality());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ModelSummary == false)
return false;
ModelSummary other = (ModelSummary) obj;
if (other.getModelName() == null ^ this.getModelName() == null)
return false;
if (other.getModelName() != null && other.getModelName().equals(this.getModelName()) == false)
return false;
if (other.getModelArn() == null ^ this.getModelArn() == null)
return false;
if (other.getModelArn() != null && other.getModelArn().equals(this.getModelArn()) == false)
return false;
if (other.getDatasetName() == null ^ this.getDatasetName() == null)
return false;
if (other.getDatasetName() != null && other.getDatasetName().equals(this.getDatasetName()) == false)
return false;
if (other.getDatasetArn() == null ^ this.getDatasetArn() == null)
return false;
if (other.getDatasetArn() != null && other.getDatasetArn().equals(this.getDatasetArn()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getCreatedAt() == null ^ this.getCreatedAt() == null)
return false;
if (other.getCreatedAt() != null && other.getCreatedAt().equals(this.getCreatedAt()) == false)
return false;
if (other.getActiveModelVersion() == null ^ this.getActiveModelVersion() == null)
return false;
if (other.getActiveModelVersion() != null && other.getActiveModelVersion().equals(this.getActiveModelVersion()) == false)
return false;
if (other.getActiveModelVersionArn() == null ^ this.getActiveModelVersionArn() == null)
return false;
if (other.getActiveModelVersionArn() != null && other.getActiveModelVersionArn().equals(this.getActiveModelVersionArn()) == false)
return false;
if (other.getLatestScheduledRetrainingStatus() == null ^ this.getLatestScheduledRetrainingStatus() == null)
return false;
if (other.getLatestScheduledRetrainingStatus() != null
&& other.getLatestScheduledRetrainingStatus().equals(this.getLatestScheduledRetrainingStatus()) == false)
return false;
if (other.getLatestScheduledRetrainingModelVersion() == null ^ this.getLatestScheduledRetrainingModelVersion() == null)
return false;
if (other.getLatestScheduledRetrainingModelVersion() != null
&& other.getLatestScheduledRetrainingModelVersion().equals(this.getLatestScheduledRetrainingModelVersion()) == false)
return false;
if (other.getLatestScheduledRetrainingStartTime() == null ^ this.getLatestScheduledRetrainingStartTime() == null)
return false;
if (other.getLatestScheduledRetrainingStartTime() != null
&& other.getLatestScheduledRetrainingStartTime().equals(this.getLatestScheduledRetrainingStartTime()) == false)
return false;
if (other.getNextScheduledRetrainingStartDate() == null ^ this.getNextScheduledRetrainingStartDate() == null)
return false;
if (other.getNextScheduledRetrainingStartDate() != null
&& other.getNextScheduledRetrainingStartDate().equals(this.getNextScheduledRetrainingStartDate()) == false)
return false;
if (other.getRetrainingSchedulerStatus() == null ^ this.getRetrainingSchedulerStatus() == null)
return false;
if (other.getRetrainingSchedulerStatus() != null && other.getRetrainingSchedulerStatus().equals(this.getRetrainingSchedulerStatus()) == false)
return false;
if (other.getModelDiagnosticsOutputConfiguration() == null ^ this.getModelDiagnosticsOutputConfiguration() == null)
return false;
if (other.getModelDiagnosticsOutputConfiguration() != null
&& other.getModelDiagnosticsOutputConfiguration().equals(this.getModelDiagnosticsOutputConfiguration()) == false)
return false;
if (other.getModelQuality() == null ^ this.getModelQuality() == null)
return false;
if (other.getModelQuality() != null && other.getModelQuality().equals(this.getModelQuality()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getModelName() == null) ? 0 : getModelName().hashCode());
hashCode = prime * hashCode + ((getModelArn() == null) ? 0 : getModelArn().hashCode());
hashCode = prime * hashCode + ((getDatasetName() == null) ? 0 : getDatasetName().hashCode());
hashCode = prime * hashCode + ((getDatasetArn() == null) ? 0 : getDatasetArn().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getCreatedAt() == null) ? 0 : getCreatedAt().hashCode());
hashCode = prime * hashCode + ((getActiveModelVersion() == null) ? 0 : getActiveModelVersion().hashCode());
hashCode = prime * hashCode + ((getActiveModelVersionArn() == null) ? 0 : getActiveModelVersionArn().hashCode());
hashCode = prime * hashCode + ((getLatestScheduledRetrainingStatus() == null) ? 0 : getLatestScheduledRetrainingStatus().hashCode());
hashCode = prime * hashCode + ((getLatestScheduledRetrainingModelVersion() == null) ? 0 : getLatestScheduledRetrainingModelVersion().hashCode());
hashCode = prime * hashCode + ((getLatestScheduledRetrainingStartTime() == null) ? 0 : getLatestScheduledRetrainingStartTime().hashCode());
hashCode = prime * hashCode + ((getNextScheduledRetrainingStartDate() == null) ? 0 : getNextScheduledRetrainingStartDate().hashCode());
hashCode = prime * hashCode + ((getRetrainingSchedulerStatus() == null) ? 0 : getRetrainingSchedulerStatus().hashCode());
hashCode = prime * hashCode + ((getModelDiagnosticsOutputConfiguration() == null) ? 0 : getModelDiagnosticsOutputConfiguration().hashCode());
hashCode = prime * hashCode + ((getModelQuality() == null) ? 0 : getModelQuality().hashCode());
return hashCode;
}
@Override
public ModelSummary clone() {
try {
return (ModelSummary) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.lookoutequipment.model.transform.ModelSummaryMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}