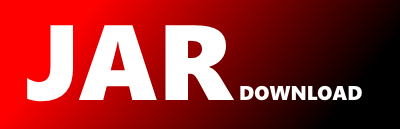
com.amazonaws.services.lookoutforvision.AmazonLookoutforVision Maven / Gradle / Ivy
Show all versions of aws-java-sdk-lookoutforvision Show documentation
/*
* Copyright 2016-2021 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.lookoutforvision;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.lookoutforvision.model.*;
/**
* Interface for accessing Amazon Lookout for Vision.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.lookoutforvision.AbstractAmazonLookoutforVision} instead.
*
*
*
* This is the Amazon Lookout for Vision API Reference. It provides descriptions of actions, data types, common
* parameters, and common errors.
*
*
* Amazon Lookout for Vision enables you to find visual defects in industrial products, accurately and at scale. It uses
* computer vision to identify missing components in an industrial product, damage to vehicles or structures,
* irregularities in production lines, and even minuscule defects in silicon wafers — or any other physical item where
* quality is important such as a missing capacitor on printed circuit boards.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonLookoutforVision {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "lookoutvision";
/**
*
* Creates a new dataset in an Amazon Lookout for Vision project. CreateDataset
can create a training
* or a test dataset from a valid dataset source (DatasetSource
).
*
*
* If you want a single dataset project, specify train
for the value of DatasetType
.
*
*
* To have a project with separate training and test datasets, call CreateDataset
twice. On the first
* call, specify train
for the value of DatasetType
. On the second call, specify
* test
for the value of DatasetType
.
*
*
* This operation requires permissions to perform the lookoutvision:CreateDataset
operation.
*
*
* @param createDatasetRequest
* @return Result of the CreateDataset operation returned by the service.
* @throws AccessDeniedException
* You are not authorized to perform the action.
* @throws InternalServerException
* Amazon Lookout for Vision experienced a service issue. Try your call again.
* @throws ValidationException
* An input validation error occured. For example, invalid characters in a project name, or if a pagination
* token is invalid.
* @throws ConflictException
* The update or deletion of a resource caused an inconsistent state.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ThrottlingException
* Amazon Lookout for Vision is temporarily unable to process the request. Try your call again.
* @throws ServiceQuotaExceededException
* A service quota was exceeded the allowed limit. For more information, see Limits in Amazon Lookout for
* Vision in the Amazon Lookout for Vision Developer Guide.
* @sample AmazonLookoutforVision.CreateDataset
* @see AWS
* API Documentation
*/
CreateDatasetResult createDataset(CreateDatasetRequest createDatasetRequest);
/**
*
* Creates a new version of a model within an an Amazon Lookout for Vision project. CreateModel
is an
* asynchronous operation in which Amazon Lookout for Vision trains, tests, and evaluates a new version of a model.
*
*
* To get the current status, check the Status
field returned in the response from
* DescribeModel.
*
*
* If the project has a single dataset, Amazon Lookout for Vision internally splits the dataset to create a training
* and a test dataset. If the project has a training and a test dataset, Lookout for Vision uses the respective
* datasets to train and test the model.
*
*
* After training completes, the evaluation metrics are stored at the location specified in
* OutputConfig
.
*
*
* This operation requires permissions to perform the lookoutvision:CreateModel
operation. If you want
* to tag your model, you also require permission to the lookoutvision:TagResource
operation.
*
*
* @param createModelRequest
* @return Result of the CreateModel operation returned by the service.
* @throws AccessDeniedException
* You are not authorized to perform the action.
* @throws InternalServerException
* Amazon Lookout for Vision experienced a service issue. Try your call again.
* @throws ValidationException
* An input validation error occured. For example, invalid characters in a project name, or if a pagination
* token is invalid.
* @throws ConflictException
* The update or deletion of a resource caused an inconsistent state.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ThrottlingException
* Amazon Lookout for Vision is temporarily unable to process the request. Try your call again.
* @throws ServiceQuotaExceededException
* A service quota was exceeded the allowed limit. For more information, see Limits in Amazon Lookout for
* Vision in the Amazon Lookout for Vision Developer Guide.
* @sample AmazonLookoutforVision.CreateModel
* @see AWS API
* Documentation
*/
CreateModelResult createModel(CreateModelRequest createModelRequest);
/**
*
* Creates an empty Amazon Lookout for Vision project. After you create the project, add a dataset by calling
* CreateDataset.
*
*
* This operation requires permissions to perform the lookoutvision:CreateProject
operation.
*
*
* @param createProjectRequest
* @return Result of the CreateProject operation returned by the service.
* @throws AccessDeniedException
* You are not authorized to perform the action.
* @throws InternalServerException
* Amazon Lookout for Vision experienced a service issue. Try your call again.
* @throws ValidationException
* An input validation error occured. For example, invalid characters in a project name, or if a pagination
* token is invalid.
* @throws ConflictException
* The update or deletion of a resource caused an inconsistent state.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ThrottlingException
* Amazon Lookout for Vision is temporarily unable to process the request. Try your call again.
* @throws ServiceQuotaExceededException
* A service quota was exceeded the allowed limit. For more information, see Limits in Amazon Lookout for
* Vision in the Amazon Lookout for Vision Developer Guide.
* @sample AmazonLookoutforVision.CreateProject
* @see AWS
* API Documentation
*/
CreateProjectResult createProject(CreateProjectRequest createProjectRequest);
/**
*
* Deletes an existing Amazon Lookout for Vision dataset
.
*
*
* If your the project has a single dataset, you must create a new dataset before you can create a model.
*
*
* If you project has a training dataset and a test dataset consider the following.
*
*
* -
*
* If you delete the test dataset, your project reverts to a single dataset project. If you then train the model,
* Amazon Lookout for Vision internally splits the remaining dataset into a training and test dataset.
*
*
* -
*
* If you delete the training dataset, you must create a training dataset before you can create a model.
*
*
*
*
* This operation requires permissions to perform the lookoutvision:DeleteDataset
operation.
*
*
* @param deleteDatasetRequest
* @return Result of the DeleteDataset operation returned by the service.
* @throws AccessDeniedException
* You are not authorized to perform the action.
* @throws InternalServerException
* Amazon Lookout for Vision experienced a service issue. Try your call again.
* @throws ValidationException
* An input validation error occured. For example, invalid characters in a project name, or if a pagination
* token is invalid.
* @throws ConflictException
* The update or deletion of a resource caused an inconsistent state.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ThrottlingException
* Amazon Lookout for Vision is temporarily unable to process the request. Try your call again.
* @sample AmazonLookoutforVision.DeleteDataset
* @see AWS
* API Documentation
*/
DeleteDatasetResult deleteDataset(DeleteDatasetRequest deleteDatasetRequest);
/**
*
* Deletes an Amazon Lookout for Vision model. You can't delete a running model. To stop a running model, use the
* StopModel operation.
*
*
* It might take a few seconds to delete a model. To determine if a model has been deleted, call ListProjects
* and check if the version of the model (ModelVersion
) is in the Models
array.
*
*
* This operation requires permissions to perform the lookoutvision:DeleteModel
operation.
*
*
* @param deleteModelRequest
* @return Result of the DeleteModel operation returned by the service.
* @throws AccessDeniedException
* You are not authorized to perform the action.
* @throws InternalServerException
* Amazon Lookout for Vision experienced a service issue. Try your call again.
* @throws ValidationException
* An input validation error occured. For example, invalid characters in a project name, or if a pagination
* token is invalid.
* @throws ConflictException
* The update or deletion of a resource caused an inconsistent state.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ThrottlingException
* Amazon Lookout for Vision is temporarily unable to process the request. Try your call again.
* @sample AmazonLookoutforVision.DeleteModel
* @see AWS API
* Documentation
*/
DeleteModelResult deleteModel(DeleteModelRequest deleteModelRequest);
/**
*
* Deletes an Amazon Lookout for Vision project.
*
*
* To delete a project, you must first delete each version of the model associated with the project. To delete a
* model use the DeleteModel operation.
*
*
* You also have to delete the dataset(s) associated with the model. For more information, see DeleteDataset.
* The images referenced by the training and test datasets aren't deleted.
*
*
* This operation requires permissions to perform the lookoutvision:DeleteProject
operation.
*
*
* @param deleteProjectRequest
* @return Result of the DeleteProject operation returned by the service.
* @throws AccessDeniedException
* You are not authorized to perform the action.
* @throws InternalServerException
* Amazon Lookout for Vision experienced a service issue. Try your call again.
* @throws ValidationException
* An input validation error occured. For example, invalid characters in a project name, or if a pagination
* token is invalid.
* @throws ConflictException
* The update or deletion of a resource caused an inconsistent state.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ThrottlingException
* Amazon Lookout for Vision is temporarily unable to process the request. Try your call again.
* @sample AmazonLookoutforVision.DeleteProject
* @see AWS
* API Documentation
*/
DeleteProjectResult deleteProject(DeleteProjectRequest deleteProjectRequest);
/**
*
* Describe an Amazon Lookout for Vision dataset.
*
*
* This operation requires permissions to perform the lookoutvision:DescribeDataset
operation.
*
*
* @param describeDatasetRequest
* @return Result of the DescribeDataset operation returned by the service.
* @throws AccessDeniedException
* You are not authorized to perform the action.
* @throws InternalServerException
* Amazon Lookout for Vision experienced a service issue. Try your call again.
* @throws ValidationException
* An input validation error occured. For example, invalid characters in a project name, or if a pagination
* token is invalid.
* @throws ConflictException
* The update or deletion of a resource caused an inconsistent state.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ThrottlingException
* Amazon Lookout for Vision is temporarily unable to process the request. Try your call again.
* @sample AmazonLookoutforVision.DescribeDataset
* @see AWS
* API Documentation
*/
DescribeDatasetResult describeDataset(DescribeDatasetRequest describeDatasetRequest);
/**
*
* Describes a version of an Amazon Lookout for Vision model.
*
*
* This operation requires permissions to perform the lookoutvision:DescribeModel
operation.
*
*
* @param describeModelRequest
* @return Result of the DescribeModel operation returned by the service.
* @throws AccessDeniedException
* You are not authorized to perform the action.
* @throws InternalServerException
* Amazon Lookout for Vision experienced a service issue. Try your call again.
* @throws ValidationException
* An input validation error occured. For example, invalid characters in a project name, or if a pagination
* token is invalid.
* @throws ConflictException
* The update or deletion of a resource caused an inconsistent state.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ThrottlingException
* Amazon Lookout for Vision is temporarily unable to process the request. Try your call again.
* @sample AmazonLookoutforVision.DescribeModel
* @see AWS
* API Documentation
*/
DescribeModelResult describeModel(DescribeModelRequest describeModelRequest);
/**
*
* Describes an Amazon Lookout for Vision project.
*
*
* This operation requires permissions to perform the lookoutvision:DescribeProject
operation.
*
*
* @param describeProjectRequest
* @return Result of the DescribeProject operation returned by the service.
* @throws AccessDeniedException
* You are not authorized to perform the action.
* @throws InternalServerException
* Amazon Lookout for Vision experienced a service issue. Try your call again.
* @throws ValidationException
* An input validation error occured. For example, invalid characters in a project name, or if a pagination
* token is invalid.
* @throws ConflictException
* The update or deletion of a resource caused an inconsistent state.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ThrottlingException
* Amazon Lookout for Vision is temporarily unable to process the request. Try your call again.
* @sample AmazonLookoutforVision.DescribeProject
* @see AWS
* API Documentation
*/
DescribeProjectResult describeProject(DescribeProjectRequest describeProjectRequest);
/**
*
* Detects anomalies in an image that you supply.
*
*
* The response from DetectAnomalies
includes a boolean prediction that the image contains one or more
* anomalies and a confidence value for the prediction.
*
*
*
* Before calling DetectAnomalies
, you must first start your model with the StartModel
* operation. You are charged for the amount of time, in minutes, that a model runs and for the number of anomaly
* detection units that your model uses. If you are not using a model, use the StopModel operation to stop
* your model.
*
*
*
* This operation requires permissions to perform the lookoutvision:DetectAnomalies
operation.
*
*
* @param detectAnomaliesRequest
* @return Result of the DetectAnomalies operation returned by the service.
* @throws AccessDeniedException
* You are not authorized to perform the action.
* @throws InternalServerException
* Amazon Lookout for Vision experienced a service issue. Try your call again.
* @throws ValidationException
* An input validation error occured. For example, invalid characters in a project name, or if a pagination
* token is invalid.
* @throws ConflictException
* The update or deletion of a resource caused an inconsistent state.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ThrottlingException
* Amazon Lookout for Vision is temporarily unable to process the request. Try your call again.
* @sample AmazonLookoutforVision.DetectAnomalies
* @see AWS
* API Documentation
*/
DetectAnomaliesResult detectAnomalies(DetectAnomaliesRequest detectAnomaliesRequest);
/**
*
* Lists the JSON Lines within a dataset. An Amazon Lookout for Vision JSON Line contains the anomaly information
* for a single image, including the image location and the assigned label.
*
*
* This operation requires permissions to perform the lookoutvision:ListDatasetEntries
operation.
*
*
* @param listDatasetEntriesRequest
* @return Result of the ListDatasetEntries operation returned by the service.
* @throws AccessDeniedException
* You are not authorized to perform the action.
* @throws InternalServerException
* Amazon Lookout for Vision experienced a service issue. Try your call again.
* @throws ValidationException
* An input validation error occured. For example, invalid characters in a project name, or if a pagination
* token is invalid.
* @throws ConflictException
* The update or deletion of a resource caused an inconsistent state.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ThrottlingException
* Amazon Lookout for Vision is temporarily unable to process the request. Try your call again.
* @sample AmazonLookoutforVision.ListDatasetEntries
* @see AWS API Documentation
*/
ListDatasetEntriesResult listDatasetEntries(ListDatasetEntriesRequest listDatasetEntriesRequest);
/**
*
* Lists the versions of a model in an Amazon Lookout for Vision project.
*
*
* This operation requires permissions to perform the lookoutvision:ListModels
operation.
*
*
* @param listModelsRequest
* @return Result of the ListModels operation returned by the service.
* @throws AccessDeniedException
* You are not authorized to perform the action.
* @throws InternalServerException
* Amazon Lookout for Vision experienced a service issue. Try your call again.
* @throws ValidationException
* An input validation error occured. For example, invalid characters in a project name, or if a pagination
* token is invalid.
* @throws ConflictException
* The update or deletion of a resource caused an inconsistent state.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ThrottlingException
* Amazon Lookout for Vision is temporarily unable to process the request. Try your call again.
* @sample AmazonLookoutforVision.ListModels
* @see AWS API
* Documentation
*/
ListModelsResult listModels(ListModelsRequest listModelsRequest);
/**
*
* Lists the Amazon Lookout for Vision projects in your AWS account.
*
*
* This operation requires permissions to perform the lookoutvision:ListProjects
operation.
*
*
* @param listProjectsRequest
* @return Result of the ListProjects operation returned by the service.
* @throws AccessDeniedException
* You are not authorized to perform the action.
* @throws InternalServerException
* Amazon Lookout for Vision experienced a service issue. Try your call again.
* @throws ValidationException
* An input validation error occured. For example, invalid characters in a project name, or if a pagination
* token is invalid.
* @throws ConflictException
* The update or deletion of a resource caused an inconsistent state.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ThrottlingException
* Amazon Lookout for Vision is temporarily unable to process the request. Try your call again.
* @sample AmazonLookoutforVision.ListProjects
* @see AWS API
* Documentation
*/
ListProjectsResult listProjects(ListProjectsRequest listProjectsRequest);
/**
*
* Returns a list of tags attached to the specified Amazon Lookout for Vision model.
*
*
* This operation requires permissions to perform the lookoutvision:ListTagsForResource
operation.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws AccessDeniedException
* You are not authorized to perform the action.
* @throws InternalServerException
* Amazon Lookout for Vision experienced a service issue. Try your call again.
* @throws ValidationException
* An input validation error occured. For example, invalid characters in a project name, or if a pagination
* token is invalid.
* @throws ConflictException
* The update or deletion of a resource caused an inconsistent state.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ThrottlingException
* Amazon Lookout for Vision is temporarily unable to process the request. Try your call again.
* @sample AmazonLookoutforVision.ListTagsForResource
* @see AWS API Documentation
*/
ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Starts the running of the version of an Amazon Lookout for Vision model. Starting a model takes a while to
* complete. To check the current state of the model, use DescribeModel.
*
*
* A model is ready to use when its status is HOSTED
.
*
*
* Once the model is running, you can detect custom labels in new images by calling DetectAnomalies.
*
*
*
* You are charged for the amount of time that the model is running. To stop a running model, call StopModel.
*
*
*
* This operation requires permissions to perform the lookoutvision:StartModel
operation.
*
*
* @param startModelRequest
* @return Result of the StartModel operation returned by the service.
* @throws AccessDeniedException
* You are not authorized to perform the action.
* @throws InternalServerException
* Amazon Lookout for Vision experienced a service issue. Try your call again.
* @throws ValidationException
* An input validation error occured. For example, invalid characters in a project name, or if a pagination
* token is invalid.
* @throws ConflictException
* The update or deletion of a resource caused an inconsistent state.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ThrottlingException
* Amazon Lookout for Vision is temporarily unable to process the request. Try your call again.
* @throws ServiceQuotaExceededException
* A service quota was exceeded the allowed limit. For more information, see Limits in Amazon Lookout for
* Vision in the Amazon Lookout for Vision Developer Guide.
* @sample AmazonLookoutforVision.StartModel
* @see AWS API
* Documentation
*/
StartModelResult startModel(StartModelRequest startModelRequest);
/**
*
* Stops the hosting of a running model. The operation might take a while to complete. To check the current status,
* call DescribeModel.
*
*
* After the model hosting stops, the Status
of the model is TRAINED
.
*
*
* This operation requires permissions to perform the lookoutvision:StopModel
operation.
*
*
* @param stopModelRequest
* @return Result of the StopModel operation returned by the service.
* @throws AccessDeniedException
* You are not authorized to perform the action.
* @throws InternalServerException
* Amazon Lookout for Vision experienced a service issue. Try your call again.
* @throws ValidationException
* An input validation error occured. For example, invalid characters in a project name, or if a pagination
* token is invalid.
* @throws ConflictException
* The update or deletion of a resource caused an inconsistent state.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ThrottlingException
* Amazon Lookout for Vision is temporarily unable to process the request. Try your call again.
* @sample AmazonLookoutforVision.StopModel
* @see AWS API
* Documentation
*/
StopModelResult stopModel(StopModelRequest stopModelRequest);
/**
*
* Adds one or more key-value tags to an Amazon Lookout for Vision model. For more information, see Tagging a
* model in the Amazon Lookout for Vision Developer Guide.
*
*
* This operation requires permissions to perform the lookoutvision:TagResource
operation.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws AccessDeniedException
* You are not authorized to perform the action.
* @throws InternalServerException
* Amazon Lookout for Vision experienced a service issue. Try your call again.
* @throws ValidationException
* An input validation error occured. For example, invalid characters in a project name, or if a pagination
* token is invalid.
* @throws ConflictException
* The update or deletion of a resource caused an inconsistent state.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ThrottlingException
* Amazon Lookout for Vision is temporarily unable to process the request. Try your call again.
* @throws ServiceQuotaExceededException
* A service quota was exceeded the allowed limit. For more information, see Limits in Amazon Lookout for
* Vision in the Amazon Lookout for Vision Developer Guide.
* @sample AmazonLookoutforVision.TagResource
* @see AWS API
* Documentation
*/
TagResourceResult tagResource(TagResourceRequest tagResourceRequest);
/**
*
* Removes one or more tags from an Amazon Lookout for Vision model. For more information, see Tagging a
* model in the Amazon Lookout for Vision Developer Guide.
*
*
* This operation requires permissions to perform the lookoutvision:UntagResource
operation.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws AccessDeniedException
* You are not authorized to perform the action.
* @throws InternalServerException
* Amazon Lookout for Vision experienced a service issue. Try your call again.
* @throws ValidationException
* An input validation error occured. For example, invalid characters in a project name, or if a pagination
* token is invalid.
* @throws ConflictException
* The update or deletion of a resource caused an inconsistent state.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ThrottlingException
* Amazon Lookout for Vision is temporarily unable to process the request. Try your call again.
* @sample AmazonLookoutforVision.UntagResource
* @see AWS
* API Documentation
*/
UntagResourceResult untagResource(UntagResourceRequest untagResourceRequest);
/**
*
* Adds one or more JSON Line entries to a dataset. A JSON Line includes information about an image used for
* training or testing an Amazon Lookout for Vision model. The following is an example JSON Line.
*
*
* Updating a dataset might take a while to complete. To check the current status, call DescribeDataset and
* check the Status
field in the response.
*
*
* This operation requires permissions to perform the lookoutvision:UpdateDatasetEntries
operation.
*
*
* @param updateDatasetEntriesRequest
* @return Result of the UpdateDatasetEntries operation returned by the service.
* @throws AccessDeniedException
* You are not authorized to perform the action.
* @throws InternalServerException
* Amazon Lookout for Vision experienced a service issue. Try your call again.
* @throws ValidationException
* An input validation error occured. For example, invalid characters in a project name, or if a pagination
* token is invalid.
* @throws ConflictException
* The update or deletion of a resource caused an inconsistent state.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ThrottlingException
* Amazon Lookout for Vision is temporarily unable to process the request. Try your call again.
* @sample AmazonLookoutforVision.UpdateDatasetEntries
* @see AWS API Documentation
*/
UpdateDatasetEntriesResult updateDatasetEntries(UpdateDatasetEntriesRequest updateDatasetEntriesRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}