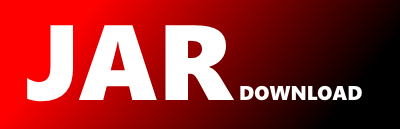
com.amazonaws.services.lookoutmetrics.AmazonLookoutMetricsClient Maven / Gradle / Ivy
Show all versions of aws-java-sdk-lookoutmetrics Show documentation
/*
* Copyright 2017-2022 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.lookoutmetrics;
import org.w3c.dom.*;
import java.net.*;
import java.util.*;
import javax.annotation.Generated;
import org.apache.commons.logging.*;
import com.amazonaws.*;
import com.amazonaws.annotation.SdkInternalApi;
import com.amazonaws.auth.*;
import com.amazonaws.handlers.*;
import com.amazonaws.http.*;
import com.amazonaws.internal.*;
import com.amazonaws.internal.auth.*;
import com.amazonaws.metrics.*;
import com.amazonaws.regions.*;
import com.amazonaws.transform.*;
import com.amazonaws.util.*;
import com.amazonaws.protocol.json.*;
import com.amazonaws.util.AWSRequestMetrics.Field;
import com.amazonaws.annotation.ThreadSafe;
import com.amazonaws.client.AwsSyncClientParams;
import com.amazonaws.client.builder.AdvancedConfig;
import com.amazonaws.services.lookoutmetrics.AmazonLookoutMetricsClientBuilder;
import com.amazonaws.AmazonServiceException;
import com.amazonaws.services.lookoutmetrics.model.*;
import com.amazonaws.services.lookoutmetrics.model.transform.*;
/**
* Client for accessing LookoutMetrics. All service calls made using this client are blocking, and will not return until
* the service call completes.
*
*
* This is the Amazon Lookout for Metrics API Reference. For an introduction to the service with tutorials for
* getting started, visit Amazon Lookout for Metrics
* Developer Guide.
*
*/
@ThreadSafe
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AmazonLookoutMetricsClient extends AmazonWebServiceClient implements AmazonLookoutMetrics {
/** Provider for AWS credentials. */
private final AWSCredentialsProvider awsCredentialsProvider;
private static final Log log = LogFactory.getLog(AmazonLookoutMetrics.class);
/** Default signing name for the service. */
private static final String DEFAULT_SIGNING_NAME = "lookoutmetrics";
/** Client configuration factory providing ClientConfigurations tailored to this client */
protected static final ClientConfigurationFactory configFactory = new ClientConfigurationFactory();
private final AdvancedConfig advancedConfig;
private static final com.amazonaws.protocol.json.SdkJsonProtocolFactory protocolFactory = new com.amazonaws.protocol.json.SdkJsonProtocolFactory(
new JsonClientMetadata()
.withProtocolVersion("1.1")
.withSupportsCbor(false)
.withSupportsIon(false)
.withContentTypeOverride("application/json")
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ServiceQuotaExceededException").withExceptionUnmarshaller(
com.amazonaws.services.lookoutmetrics.model.transform.ServiceQuotaExceededExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InternalServerException").withExceptionUnmarshaller(
com.amazonaws.services.lookoutmetrics.model.transform.InternalServerExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("AccessDeniedException").withExceptionUnmarshaller(
com.amazonaws.services.lookoutmetrics.model.transform.AccessDeniedExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ConflictException").withExceptionUnmarshaller(
com.amazonaws.services.lookoutmetrics.model.transform.ConflictExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ResourceNotFoundException").withExceptionUnmarshaller(
com.amazonaws.services.lookoutmetrics.model.transform.ResourceNotFoundExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ValidationException").withExceptionUnmarshaller(
com.amazonaws.services.lookoutmetrics.model.transform.ValidationExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("TooManyRequestsException").withExceptionUnmarshaller(
com.amazonaws.services.lookoutmetrics.model.transform.TooManyRequestsExceptionUnmarshaller.getInstance()))
.withBaseServiceExceptionClass(com.amazonaws.services.lookoutmetrics.model.AmazonLookoutMetricsException.class));
public static AmazonLookoutMetricsClientBuilder builder() {
return AmazonLookoutMetricsClientBuilder.standard();
}
/**
* Constructs a new client to invoke service methods on LookoutMetrics using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AmazonLookoutMetricsClient(AwsSyncClientParams clientParams) {
this(clientParams, false);
}
/**
* Constructs a new client to invoke service methods on LookoutMetrics using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AmazonLookoutMetricsClient(AwsSyncClientParams clientParams, boolean endpointDiscoveryEnabled) {
super(clientParams);
this.awsCredentialsProvider = clientParams.getCredentialsProvider();
this.advancedConfig = clientParams.getAdvancedConfig();
init();
}
private void init() {
setServiceNameIntern(DEFAULT_SIGNING_NAME);
setEndpointPrefix(ENDPOINT_PREFIX);
// calling this.setEndPoint(...) will also modify the signer accordingly
setEndpoint("lookoutmetrics.us-east-1.amazonaws.com");
HandlerChainFactory chainFactory = new HandlerChainFactory();
requestHandler2s.addAll(chainFactory.newRequestHandlerChain("/com/amazonaws/services/lookoutmetrics/request.handlers"));
requestHandler2s.addAll(chainFactory.newRequestHandler2Chain("/com/amazonaws/services/lookoutmetrics/request.handler2s"));
requestHandler2s.addAll(chainFactory.getGlobalHandlers());
}
/**
*
* Activates an anomaly detector.
*
*
* @param activateAnomalyDetectorRequest
* @return Result of the ActivateAnomalyDetector operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the AWS service. Check your input values and try
* again.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ARN of the resource and try again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action.
* @throws TooManyRequestsException
* The request was denied due to too many requests being submitted at the same time.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws ConflictException
* There was a conflict processing the request. Try your request again.
* @sample AmazonLookoutMetrics.ActivateAnomalyDetector
* @see AWS API Documentation
*/
@Override
public ActivateAnomalyDetectorResult activateAnomalyDetector(ActivateAnomalyDetectorRequest request) {
request = beforeClientExecution(request);
return executeActivateAnomalyDetector(request);
}
@SdkInternalApi
final ActivateAnomalyDetectorResult executeActivateAnomalyDetector(ActivateAnomalyDetectorRequest activateAnomalyDetectorRequest) {
ExecutionContext executionContext = createExecutionContext(activateAnomalyDetectorRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ActivateAnomalyDetectorRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(activateAnomalyDetectorRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "LookoutMetrics");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ActivateAnomalyDetector");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ActivateAnomalyDetectorResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Runs a backtest for anomaly detection for the specified resource.
*
*
* @param backTestAnomalyDetectorRequest
* @return Result of the BackTestAnomalyDetector operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the AWS service. Check your input values and try
* again.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ARN of the resource and try again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action.
* @throws TooManyRequestsException
* The request was denied due to too many requests being submitted at the same time.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @sample AmazonLookoutMetrics.BackTestAnomalyDetector
* @see AWS API Documentation
*/
@Override
public BackTestAnomalyDetectorResult backTestAnomalyDetector(BackTestAnomalyDetectorRequest request) {
request = beforeClientExecution(request);
return executeBackTestAnomalyDetector(request);
}
@SdkInternalApi
final BackTestAnomalyDetectorResult executeBackTestAnomalyDetector(BackTestAnomalyDetectorRequest backTestAnomalyDetectorRequest) {
ExecutionContext executionContext = createExecutionContext(backTestAnomalyDetectorRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new BackTestAnomalyDetectorRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(backTestAnomalyDetectorRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "LookoutMetrics");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "BackTestAnomalyDetector");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new BackTestAnomalyDetectorResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates an alert for an anomaly detector.
*
*
* @param createAlertRequest
* @return Result of the CreateAlert operation returned by the service.
* @throws ConflictException
* There was a conflict processing the request. Try your request again.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the AWS service. Check your input values and try
* again.
* @throws ServiceQuotaExceededException
* The request exceeded the service's quotas. Check the service quotas and try again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action.
* @throws TooManyRequestsException
* The request was denied due to too many requests being submitted at the same time.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ARN of the resource and try again.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @sample AmazonLookoutMetrics.CreateAlert
* @see AWS API
* Documentation
*/
@Override
public CreateAlertResult createAlert(CreateAlertRequest request) {
request = beforeClientExecution(request);
return executeCreateAlert(request);
}
@SdkInternalApi
final CreateAlertResult executeCreateAlert(CreateAlertRequest createAlertRequest) {
ExecutionContext executionContext = createExecutionContext(createAlertRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateAlertRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createAlertRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "LookoutMetrics");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateAlert");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateAlertResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates an anomaly detector.
*
*
* @param createAnomalyDetectorRequest
* @return Result of the CreateAnomalyDetector operation returned by the service.
* @throws ConflictException
* There was a conflict processing the request. Try your request again.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the AWS service. Check your input values and try
* again.
* @throws ServiceQuotaExceededException
* The request exceeded the service's quotas. Check the service quotas and try again.
* @throws ConflictException
* There was a conflict processing the request. Try your request again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action.
* @throws TooManyRequestsException
* The request was denied due to too many requests being submitted at the same time.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @sample AmazonLookoutMetrics.CreateAnomalyDetector
* @see AWS API Documentation
*/
@Override
public CreateAnomalyDetectorResult createAnomalyDetector(CreateAnomalyDetectorRequest request) {
request = beforeClientExecution(request);
return executeCreateAnomalyDetector(request);
}
@SdkInternalApi
final CreateAnomalyDetectorResult executeCreateAnomalyDetector(CreateAnomalyDetectorRequest createAnomalyDetectorRequest) {
ExecutionContext executionContext = createExecutionContext(createAnomalyDetectorRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateAnomalyDetectorRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createAnomalyDetectorRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "LookoutMetrics");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateAnomalyDetector");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateAnomalyDetectorResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a dataset.
*
*
* @param createMetricSetRequest
* @return Result of the CreateMetricSet operation returned by the service.
* @throws ConflictException
* There was a conflict processing the request. Try your request again.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the AWS service. Check your input values and try
* again.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ARN of the resource and try again.
* @throws ServiceQuotaExceededException
* The request exceeded the service's quotas. Check the service quotas and try again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action.
* @throws TooManyRequestsException
* The request was denied due to too many requests being submitted at the same time.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @sample AmazonLookoutMetrics.CreateMetricSet
* @see AWS
* API Documentation
*/
@Override
public CreateMetricSetResult createMetricSet(CreateMetricSetRequest request) {
request = beforeClientExecution(request);
return executeCreateMetricSet(request);
}
@SdkInternalApi
final CreateMetricSetResult executeCreateMetricSet(CreateMetricSetRequest createMetricSetRequest) {
ExecutionContext executionContext = createExecutionContext(createMetricSetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateMetricSetRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createMetricSetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "LookoutMetrics");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateMetricSet");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateMetricSetResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deactivates an anomaly detector.
*
*
* @param deactivateAnomalyDetectorRequest
* @return Result of the DeactivateAnomalyDetector operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the AWS service. Check your input values and try
* again.
* @throws ConflictException
* There was a conflict processing the request. Try your request again.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ARN of the resource and try again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action.
* @throws TooManyRequestsException
* The request was denied due to too many requests being submitted at the same time.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @sample AmazonLookoutMetrics.DeactivateAnomalyDetector
* @see AWS API Documentation
*/
@Override
public DeactivateAnomalyDetectorResult deactivateAnomalyDetector(DeactivateAnomalyDetectorRequest request) {
request = beforeClientExecution(request);
return executeDeactivateAnomalyDetector(request);
}
@SdkInternalApi
final DeactivateAnomalyDetectorResult executeDeactivateAnomalyDetector(DeactivateAnomalyDetectorRequest deactivateAnomalyDetectorRequest) {
ExecutionContext executionContext = createExecutionContext(deactivateAnomalyDetectorRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeactivateAnomalyDetectorRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deactivateAnomalyDetectorRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "LookoutMetrics");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeactivateAnomalyDetector");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeactivateAnomalyDetectorResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes an alert.
*
*
* @param deleteAlertRequest
* @return Result of the DeleteAlert operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ARN of the resource and try again.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the AWS service. Check your input values and try
* again.
* @throws TooManyRequestsException
* The request was denied due to too many requests being submitted at the same time.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @sample AmazonLookoutMetrics.DeleteAlert
* @see AWS API
* Documentation
*/
@Override
public DeleteAlertResult deleteAlert(DeleteAlertRequest request) {
request = beforeClientExecution(request);
return executeDeleteAlert(request);
}
@SdkInternalApi
final DeleteAlertResult executeDeleteAlert(DeleteAlertRequest deleteAlertRequest) {
ExecutionContext executionContext = createExecutionContext(deleteAlertRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteAlertRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteAlertRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "LookoutMetrics");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteAlert");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteAlertResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a detector. Deleting an anomaly detector will delete all of its corresponding resources including any
* configured datasets and alerts.
*
*
* @param deleteAnomalyDetectorRequest
* @return Result of the DeleteAnomalyDetector operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ARN of the resource and try again.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the AWS service. Check your input values and try
* again.
* @throws ConflictException
* There was a conflict processing the request. Try your request again.
* @throws TooManyRequestsException
* The request was denied due to too many requests being submitted at the same time.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @sample AmazonLookoutMetrics.DeleteAnomalyDetector
* @see AWS API Documentation
*/
@Override
public DeleteAnomalyDetectorResult deleteAnomalyDetector(DeleteAnomalyDetectorRequest request) {
request = beforeClientExecution(request);
return executeDeleteAnomalyDetector(request);
}
@SdkInternalApi
final DeleteAnomalyDetectorResult executeDeleteAnomalyDetector(DeleteAnomalyDetectorRequest deleteAnomalyDetectorRequest) {
ExecutionContext executionContext = createExecutionContext(deleteAnomalyDetectorRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteAnomalyDetectorRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteAnomalyDetectorRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "LookoutMetrics");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteAnomalyDetector");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteAnomalyDetectorResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes an alert.
*
*
* Amazon Lookout for Metrics API actions are eventually consistent. If you do a read operation on a resource
* immediately after creating or modifying it, use retries to allow time for the write operation to complete.
*
*
* @param describeAlertRequest
* @return Result of the DescribeAlert operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ARN of the resource and try again.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the AWS service. Check your input values and try
* again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws TooManyRequestsException
* The request was denied due to too many requests being submitted at the same time.
* @sample AmazonLookoutMetrics.DescribeAlert
* @see AWS
* API Documentation
*/
@Override
public DescribeAlertResult describeAlert(DescribeAlertRequest request) {
request = beforeClientExecution(request);
return executeDescribeAlert(request);
}
@SdkInternalApi
final DescribeAlertResult executeDescribeAlert(DescribeAlertRequest describeAlertRequest) {
ExecutionContext executionContext = createExecutionContext(describeAlertRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeAlertRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeAlertRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "LookoutMetrics");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeAlert");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeAlertResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns information about the status of the specified anomaly detection jobs.
*
*
* @param describeAnomalyDetectionExecutionsRequest
* @return Result of the DescribeAnomalyDetectionExecutions operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ARN of the resource and try again.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the AWS service. Check your input values and try
* again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action.
* @throws TooManyRequestsException
* The request was denied due to too many requests being submitted at the same time.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @sample AmazonLookoutMetrics.DescribeAnomalyDetectionExecutions
* @see AWS API Documentation
*/
@Override
public DescribeAnomalyDetectionExecutionsResult describeAnomalyDetectionExecutions(DescribeAnomalyDetectionExecutionsRequest request) {
request = beforeClientExecution(request);
return executeDescribeAnomalyDetectionExecutions(request);
}
@SdkInternalApi
final DescribeAnomalyDetectionExecutionsResult executeDescribeAnomalyDetectionExecutions(
DescribeAnomalyDetectionExecutionsRequest describeAnomalyDetectionExecutionsRequest) {
ExecutionContext executionContext = createExecutionContext(describeAnomalyDetectionExecutionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeAnomalyDetectionExecutionsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeAnomalyDetectionExecutionsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "LookoutMetrics");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeAnomalyDetectionExecutions");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeAnomalyDetectionExecutionsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes a detector.
*
*
* Amazon Lookout for Metrics API actions are eventually consistent. If you do a read operation on a resource
* immediately after creating or modifying it, use retries to allow time for the write operation to complete.
*
*
* @param describeAnomalyDetectorRequest
* @return Result of the DescribeAnomalyDetector operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ARN of the resource and try again.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the AWS service. Check your input values and try
* again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws TooManyRequestsException
* The request was denied due to too many requests being submitted at the same time.
* @sample AmazonLookoutMetrics.DescribeAnomalyDetector
* @see AWS API Documentation
*/
@Override
public DescribeAnomalyDetectorResult describeAnomalyDetector(DescribeAnomalyDetectorRequest request) {
request = beforeClientExecution(request);
return executeDescribeAnomalyDetector(request);
}
@SdkInternalApi
final DescribeAnomalyDetectorResult executeDescribeAnomalyDetector(DescribeAnomalyDetectorRequest describeAnomalyDetectorRequest) {
ExecutionContext executionContext = createExecutionContext(describeAnomalyDetectorRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeAnomalyDetectorRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeAnomalyDetectorRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "LookoutMetrics");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeAnomalyDetector");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeAnomalyDetectorResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes a dataset.
*
*
* Amazon Lookout for Metrics API actions are eventually consistent. If you do a read operation on a resource
* immediately after creating or modifying it, use retries to allow time for the write operation to complete.
*
*
* @param describeMetricSetRequest
* @return Result of the DescribeMetricSet operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ARN of the resource and try again.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the AWS service. Check your input values and try
* again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action.
* @throws TooManyRequestsException
* The request was denied due to too many requests being submitted at the same time.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @sample AmazonLookoutMetrics.DescribeMetricSet
* @see AWS API Documentation
*/
@Override
public DescribeMetricSetResult describeMetricSet(DescribeMetricSetRequest request) {
request = beforeClientExecution(request);
return executeDescribeMetricSet(request);
}
@SdkInternalApi
final DescribeMetricSetResult executeDescribeMetricSet(DescribeMetricSetRequest describeMetricSetRequest) {
ExecutionContext executionContext = createExecutionContext(describeMetricSetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeMetricSetRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeMetricSetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "LookoutMetrics");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeMetricSet");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeMetricSetResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Detects an Amazon S3 dataset's file format, interval, and offset.
*
*
* @param detectMetricSetConfigRequest
* @return Result of the DetectMetricSetConfig operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ARN of the resource and try again.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the AWS service. Check your input values and try
* again.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action.
* @throws TooManyRequestsException
* The request was denied due to too many requests being submitted at the same time.
* @sample AmazonLookoutMetrics.DetectMetricSetConfig
* @see AWS API Documentation
*/
@Override
public DetectMetricSetConfigResult detectMetricSetConfig(DetectMetricSetConfigRequest request) {
request = beforeClientExecution(request);
return executeDetectMetricSetConfig(request);
}
@SdkInternalApi
final DetectMetricSetConfigResult executeDetectMetricSetConfig(DetectMetricSetConfigRequest detectMetricSetConfigRequest) {
ExecutionContext executionContext = createExecutionContext(detectMetricSetConfigRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DetectMetricSetConfigRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(detectMetricSetConfigRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "LookoutMetrics");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DetectMetricSetConfig");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DetectMetricSetConfigResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns details about a group of anomalous metrics.
*
*
* @param getAnomalyGroupRequest
* @return Result of the GetAnomalyGroup operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ARN of the resource and try again.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the AWS service. Check your input values and try
* again.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action.
* @throws TooManyRequestsException
* The request was denied due to too many requests being submitted at the same time.
* @sample AmazonLookoutMetrics.GetAnomalyGroup
* @see AWS
* API Documentation
*/
@Override
public GetAnomalyGroupResult getAnomalyGroup(GetAnomalyGroupRequest request) {
request = beforeClientExecution(request);
return executeGetAnomalyGroup(request);
}
@SdkInternalApi
final GetAnomalyGroupResult executeGetAnomalyGroup(GetAnomalyGroupRequest getAnomalyGroupRequest) {
ExecutionContext executionContext = createExecutionContext(getAnomalyGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetAnomalyGroupRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getAnomalyGroupRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "LookoutMetrics");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetAnomalyGroup");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetAnomalyGroupResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns details about the requested data quality metrics.
*
*
* @param getDataQualityMetricsRequest
* @return Result of the GetDataQualityMetrics operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ARN of the resource and try again.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the AWS service. Check your input values and try
* again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action.
* @throws TooManyRequestsException
* The request was denied due to too many requests being submitted at the same time.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @sample AmazonLookoutMetrics.GetDataQualityMetrics
* @see AWS API Documentation
*/
@Override
public GetDataQualityMetricsResult getDataQualityMetrics(GetDataQualityMetricsRequest request) {
request = beforeClientExecution(request);
return executeGetDataQualityMetrics(request);
}
@SdkInternalApi
final GetDataQualityMetricsResult executeGetDataQualityMetrics(GetDataQualityMetricsRequest getDataQualityMetricsRequest) {
ExecutionContext executionContext = createExecutionContext(getDataQualityMetricsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetDataQualityMetricsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getDataQualityMetricsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "LookoutMetrics");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetDataQualityMetrics");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetDataQualityMetricsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Get feedback for an anomaly group.
*
*
* @param getFeedbackRequest
* @return Result of the GetFeedback operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ARN of the resource and try again.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the AWS service. Check your input values and try
* again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws TooManyRequestsException
* The request was denied due to too many requests being submitted at the same time.
* @sample AmazonLookoutMetrics.GetFeedback
* @see AWS API
* Documentation
*/
@Override
public GetFeedbackResult getFeedback(GetFeedbackRequest request) {
request = beforeClientExecution(request);
return executeGetFeedback(request);
}
@SdkInternalApi
final GetFeedbackResult executeGetFeedback(GetFeedbackRequest getFeedbackRequest) {
ExecutionContext executionContext = createExecutionContext(getFeedbackRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetFeedbackRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getFeedbackRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "LookoutMetrics");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetFeedback");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetFeedbackResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a selection of sample records from an Amazon S3 datasource.
*
*
* @param getSampleDataRequest
* @return Result of the GetSampleData operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the AWS service. Check your input values and try
* again.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ARN of the resource and try again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action.
* @throws TooManyRequestsException
* The request was denied due to too many requests being submitted at the same time.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @sample AmazonLookoutMetrics.GetSampleData
* @see AWS
* API Documentation
*/
@Override
public GetSampleDataResult getSampleData(GetSampleDataRequest request) {
request = beforeClientExecution(request);
return executeGetSampleData(request);
}
@SdkInternalApi
final GetSampleDataResult executeGetSampleData(GetSampleDataRequest getSampleDataRequest) {
ExecutionContext executionContext = createExecutionContext(getSampleDataRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetSampleDataRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getSampleDataRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "LookoutMetrics");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetSampleData");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetSampleDataResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the alerts attached to a detector.
*
*
* Amazon Lookout for Metrics API actions are eventually consistent. If you do a read operation on a resource
* immediately after creating or modifying it, use retries to allow time for the write operation to complete.
*
*
* @param listAlertsRequest
* @return Result of the ListAlerts operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ARN of the resource and try again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws TooManyRequestsException
* The request was denied due to too many requests being submitted at the same time.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the AWS service. Check your input values and try
* again.
* @sample AmazonLookoutMetrics.ListAlerts
* @see AWS API
* Documentation
*/
@Override
public ListAlertsResult listAlerts(ListAlertsRequest request) {
request = beforeClientExecution(request);
return executeListAlerts(request);
}
@SdkInternalApi
final ListAlertsResult executeListAlerts(ListAlertsRequest listAlertsRequest) {
ExecutionContext executionContext = createExecutionContext(listAlertsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListAlertsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listAlertsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "LookoutMetrics");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListAlerts");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListAlertsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the detectors in the current AWS Region.
*
*
* Amazon Lookout for Metrics API actions are eventually consistent. If you do a read operation on a resource
* immediately after creating or modifying it, use retries to allow time for the write operation to complete.
*
*
* @param listAnomalyDetectorsRequest
* @return Result of the ListAnomalyDetectors operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ARN of the resource and try again.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the AWS service. Check your input values and try
* again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action.
* @throws TooManyRequestsException
* The request was denied due to too many requests being submitted at the same time.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @sample AmazonLookoutMetrics.ListAnomalyDetectors
* @see AWS API Documentation
*/
@Override
public ListAnomalyDetectorsResult listAnomalyDetectors(ListAnomalyDetectorsRequest request) {
request = beforeClientExecution(request);
return executeListAnomalyDetectors(request);
}
@SdkInternalApi
final ListAnomalyDetectorsResult executeListAnomalyDetectors(ListAnomalyDetectorsRequest listAnomalyDetectorsRequest) {
ExecutionContext executionContext = createExecutionContext(listAnomalyDetectorsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListAnomalyDetectorsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listAnomalyDetectorsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "LookoutMetrics");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListAnomalyDetectors");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListAnomalyDetectorsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of measures that are potential causes or effects of an anomaly group.
*
*
* @param listAnomalyGroupRelatedMetricsRequest
* @return Result of the ListAnomalyGroupRelatedMetrics operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ARN of the resource and try again.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the AWS service. Check your input values and try
* again.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action.
* @throws TooManyRequestsException
* The request was denied due to too many requests being submitted at the same time.
* @sample AmazonLookoutMetrics.ListAnomalyGroupRelatedMetrics
* @see AWS API Documentation
*/
@Override
public ListAnomalyGroupRelatedMetricsResult listAnomalyGroupRelatedMetrics(ListAnomalyGroupRelatedMetricsRequest request) {
request = beforeClientExecution(request);
return executeListAnomalyGroupRelatedMetrics(request);
}
@SdkInternalApi
final ListAnomalyGroupRelatedMetricsResult executeListAnomalyGroupRelatedMetrics(ListAnomalyGroupRelatedMetricsRequest listAnomalyGroupRelatedMetricsRequest) {
ExecutionContext executionContext = createExecutionContext(listAnomalyGroupRelatedMetricsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListAnomalyGroupRelatedMetricsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listAnomalyGroupRelatedMetricsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "LookoutMetrics");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListAnomalyGroupRelatedMetrics");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListAnomalyGroupRelatedMetricsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of anomaly groups.
*
*
* @param listAnomalyGroupSummariesRequest
* @return Result of the ListAnomalyGroupSummaries operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ARN of the resource and try again.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the AWS service. Check your input values and try
* again.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action.
* @throws TooManyRequestsException
* The request was denied due to too many requests being submitted at the same time.
* @sample AmazonLookoutMetrics.ListAnomalyGroupSummaries
* @see AWS API Documentation
*/
@Override
public ListAnomalyGroupSummariesResult listAnomalyGroupSummaries(ListAnomalyGroupSummariesRequest request) {
request = beforeClientExecution(request);
return executeListAnomalyGroupSummaries(request);
}
@SdkInternalApi
final ListAnomalyGroupSummariesResult executeListAnomalyGroupSummaries(ListAnomalyGroupSummariesRequest listAnomalyGroupSummariesRequest) {
ExecutionContext executionContext = createExecutionContext(listAnomalyGroupSummariesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListAnomalyGroupSummariesRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listAnomalyGroupSummariesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "LookoutMetrics");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListAnomalyGroupSummaries");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListAnomalyGroupSummariesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets a list of anomalous metrics for a measure in an anomaly group.
*
*
* @param listAnomalyGroupTimeSeriesRequest
* @return Result of the ListAnomalyGroupTimeSeries operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the AWS service. Check your input values and try
* again.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ARN of the resource and try again.
* @throws TooManyRequestsException
* The request was denied due to too many requests being submitted at the same time.
* @sample AmazonLookoutMetrics.ListAnomalyGroupTimeSeries
* @see AWS API Documentation
*/
@Override
public ListAnomalyGroupTimeSeriesResult listAnomalyGroupTimeSeries(ListAnomalyGroupTimeSeriesRequest request) {
request = beforeClientExecution(request);
return executeListAnomalyGroupTimeSeries(request);
}
@SdkInternalApi
final ListAnomalyGroupTimeSeriesResult executeListAnomalyGroupTimeSeries(ListAnomalyGroupTimeSeriesRequest listAnomalyGroupTimeSeriesRequest) {
ExecutionContext executionContext = createExecutionContext(listAnomalyGroupTimeSeriesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListAnomalyGroupTimeSeriesRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listAnomalyGroupTimeSeriesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "LookoutMetrics");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListAnomalyGroupTimeSeries");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListAnomalyGroupTimeSeriesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the datasets in the current AWS Region.
*
*
* Amazon Lookout for Metrics API actions are eventually consistent. If you do a read operation on a resource
* immediately after creating or modifying it, use retries to allow time for the write operation to complete.
*
*
* @param listMetricSetsRequest
* @return Result of the ListMetricSets operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ARN of the resource and try again.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the AWS service. Check your input values and try
* again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action.
* @throws TooManyRequestsException
* The request was denied due to too many requests being submitted at the same time.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @sample AmazonLookoutMetrics.ListMetricSets
* @see AWS
* API Documentation
*/
@Override
public ListMetricSetsResult listMetricSets(ListMetricSetsRequest request) {
request = beforeClientExecution(request);
return executeListMetricSets(request);
}
@SdkInternalApi
final ListMetricSetsResult executeListMetricSets(ListMetricSetsRequest listMetricSetsRequest) {
ExecutionContext executionContext = createExecutionContext(listMetricSetsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListMetricSetsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listMetricSetsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "LookoutMetrics");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListMetricSets");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListMetricSetsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets a list of tags for a
* detector, dataset, or alert.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the AWS service. Check your input values and try
* again.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ARN of the resource and try again.
* @sample AmazonLookoutMetrics.ListTagsForResource
* @see AWS API Documentation
*/
@Override
public ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest request) {
request = beforeClientExecution(request);
return executeListTagsForResource(request);
}
@SdkInternalApi
final ListTagsForResourceResult executeListTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest) {
ExecutionContext executionContext = createExecutionContext(listTagsForResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListTagsForResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listTagsForResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "LookoutMetrics");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListTagsForResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListTagsForResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Add feedback for an anomalous metric.
*
*
* @param putFeedbackRequest
* @return Result of the PutFeedback operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ARN of the resource and try again.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the AWS service. Check your input values and try
* again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws TooManyRequestsException
* The request was denied due to too many requests being submitted at the same time.
* @sample AmazonLookoutMetrics.PutFeedback
* @see AWS API
* Documentation
*/
@Override
public PutFeedbackResult putFeedback(PutFeedbackRequest request) {
request = beforeClientExecution(request);
return executePutFeedback(request);
}
@SdkInternalApi
final PutFeedbackResult executePutFeedback(PutFeedbackRequest putFeedbackRequest) {
ExecutionContext executionContext = createExecutionContext(putFeedbackRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new PutFeedbackRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(putFeedbackRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "LookoutMetrics");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "PutFeedback");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new PutFeedbackResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Adds tags to a detector,
* dataset, or alert.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the AWS service. Check your input values and try
* again.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ARN of the resource and try again.
* @sample AmazonLookoutMetrics.TagResource
* @see AWS API
* Documentation
*/
@Override
public TagResourceResult tagResource(TagResourceRequest request) {
request = beforeClientExecution(request);
return executeTagResource(request);
}
@SdkInternalApi
final TagResourceResult executeTagResource(TagResourceRequest tagResourceRequest) {
ExecutionContext executionContext = createExecutionContext(tagResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new TagResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(tagResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "LookoutMetrics");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "TagResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new TagResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Removes tags from a
* detector, dataset, or alert.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the AWS service. Check your input values and try
* again.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ARN of the resource and try again.
* @sample AmazonLookoutMetrics.UntagResource
* @see AWS
* API Documentation
*/
@Override
public UntagResourceResult untagResource(UntagResourceRequest request) {
request = beforeClientExecution(request);
return executeUntagResource(request);
}
@SdkInternalApi
final UntagResourceResult executeUntagResource(UntagResourceRequest untagResourceRequest) {
ExecutionContext executionContext = createExecutionContext(untagResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UntagResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(untagResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "LookoutMetrics");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UntagResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UntagResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Make changes to an existing alert.
*
*
* @param updateAlertRequest
* @return Result of the UpdateAlert operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the AWS service. Check your input values and try
* again.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ARN of the resource and try again.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action.
* @throws TooManyRequestsException
* The request was denied due to too many requests being submitted at the same time.
* @sample AmazonLookoutMetrics.UpdateAlert
* @see AWS API
* Documentation
*/
@Override
public UpdateAlertResult updateAlert(UpdateAlertRequest request) {
request = beforeClientExecution(request);
return executeUpdateAlert(request);
}
@SdkInternalApi
final UpdateAlertResult executeUpdateAlert(UpdateAlertRequest updateAlertRequest) {
ExecutionContext executionContext = createExecutionContext(updateAlertRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateAlertRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateAlertRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "LookoutMetrics");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateAlert");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateAlertResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates a detector. After activation, you can only change a detector's ingestion delay and description.
*
*
* @param updateAnomalyDetectorRequest
* @return Result of the UpdateAnomalyDetector operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ARN of the resource and try again.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the AWS service. Check your input values and try
* again.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action.
* @throws TooManyRequestsException
* The request was denied due to too many requests being submitted at the same time.
* @sample AmazonLookoutMetrics.UpdateAnomalyDetector
* @see AWS API Documentation
*/
@Override
public UpdateAnomalyDetectorResult updateAnomalyDetector(UpdateAnomalyDetectorRequest request) {
request = beforeClientExecution(request);
return executeUpdateAnomalyDetector(request);
}
@SdkInternalApi
final UpdateAnomalyDetectorResult executeUpdateAnomalyDetector(UpdateAnomalyDetectorRequest updateAnomalyDetectorRequest) {
ExecutionContext executionContext = createExecutionContext(updateAnomalyDetectorRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateAnomalyDetectorRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateAnomalyDetectorRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "LookoutMetrics");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateAnomalyDetector");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new UpdateAnomalyDetectorResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates a dataset.
*
*
* @param updateMetricSetRequest
* @return Result of the UpdateMetricSet operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ARN of the resource and try again.
* @throws ValidationException
* The input fails to satisfy the constraints specified by the AWS service. Check your input values and try
* again.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action.
* @throws TooManyRequestsException
* The request was denied due to too many requests being submitted at the same time.
* @throws ServiceQuotaExceededException
* The request exceeded the service's quotas. Check the service quotas and try again.
* @sample AmazonLookoutMetrics.UpdateMetricSet
* @see AWS
* API Documentation
*/
@Override
public UpdateMetricSetResult updateMetricSet(UpdateMetricSetRequest request) {
request = beforeClientExecution(request);
return executeUpdateMetricSet(request);
}
@SdkInternalApi
final UpdateMetricSetResult executeUpdateMetricSet(UpdateMetricSetRequest updateMetricSetRequest) {
ExecutionContext executionContext = createExecutionContext(updateMetricSetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateMetricSetRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateMetricSetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "LookoutMetrics");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateMetricSet");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateMetricSetResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
* Returns additional metadata for a previously executed successful, request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing the request.
*
* @param request
* The originally executed request
*
* @return The response metadata for the specified request, or null if none is available.
*/
public ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request) {
return client.getResponseMetadataForRequest(request);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext) {
return invoke(request, responseHandler, executionContext, null, null);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI cachedEndpoint, URI uriFromEndpointTrait) {
executionContext.setCredentialsProvider(CredentialUtils.getCredentialsProvider(request.getOriginalRequest(), awsCredentialsProvider));
return doInvoke(request, responseHandler, executionContext, cachedEndpoint, uriFromEndpointTrait);
}
/**
* Invoke with no authentication. Credentials are not required and any credentials set on the client or request will
* be ignored for this operation.
**/
private Response anonymousInvoke(Request request,
HttpResponseHandler> responseHandler, ExecutionContext executionContext) {
return doInvoke(request, responseHandler, executionContext, null, null);
}
/**
* Invoke the request using the http client. Assumes credentials (or lack thereof) have been configured in the
* ExecutionContext beforehand.
**/
private Response doInvoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI discoveredEndpoint, URI uriFromEndpointTrait) {
if (discoveredEndpoint != null) {
request.setEndpoint(discoveredEndpoint);
request.getOriginalRequest().getRequestClientOptions().appendUserAgent("endpoint-discovery");
} else if (uriFromEndpointTrait != null) {
request.setEndpoint(uriFromEndpointTrait);
} else {
request.setEndpoint(endpoint);
}
request.setTimeOffset(timeOffset);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler(new JsonErrorResponseMetadata());
return client.execute(request, responseHandler, errorResponseHandler, executionContext);
}
@com.amazonaws.annotation.SdkInternalApi
static com.amazonaws.protocol.json.SdkJsonProtocolFactory getProtocolFactory() {
return protocolFactory;
}
@Override
public void shutdown() {
super.shutdown();
}
}