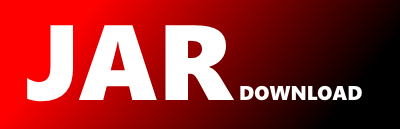
com.amazonaws.services.lookoutmetrics.AmazonLookoutMetricsAsync Maven / Gradle / Ivy
/*
* Copyright 2018-2023 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.lookoutmetrics;
import javax.annotation.Generated;
import com.amazonaws.services.lookoutmetrics.model.*;
/**
* Interface for accessing LookoutMetrics asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.lookoutmetrics.AbstractAmazonLookoutMetricsAsync} instead.
*
*
*
* This is the Amazon Lookout for Metrics API Reference. For an introduction to the service with tutorials for
* getting started, visit Amazon Lookout for Metrics
* Developer Guide.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonLookoutMetricsAsync extends AmazonLookoutMetrics {
/**
*
* Activates an anomaly detector.
*
*
* @param activateAnomalyDetectorRequest
* @return A Java Future containing the result of the ActivateAnomalyDetector operation returned by the service.
* @sample AmazonLookoutMetricsAsync.ActivateAnomalyDetector
* @see AWS API Documentation
*/
java.util.concurrent.Future activateAnomalyDetectorAsync(ActivateAnomalyDetectorRequest activateAnomalyDetectorRequest);
/**
*
* Activates an anomaly detector.
*
*
* @param activateAnomalyDetectorRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ActivateAnomalyDetector operation returned by the service.
* @sample AmazonLookoutMetricsAsyncHandler.ActivateAnomalyDetector
* @see AWS API Documentation
*/
java.util.concurrent.Future activateAnomalyDetectorAsync(ActivateAnomalyDetectorRequest activateAnomalyDetectorRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Runs a backtest for anomaly detection for the specified resource.
*
*
* @param backTestAnomalyDetectorRequest
* @return A Java Future containing the result of the BackTestAnomalyDetector operation returned by the service.
* @sample AmazonLookoutMetricsAsync.BackTestAnomalyDetector
* @see AWS API Documentation
*/
java.util.concurrent.Future backTestAnomalyDetectorAsync(BackTestAnomalyDetectorRequest backTestAnomalyDetectorRequest);
/**
*
* Runs a backtest for anomaly detection for the specified resource.
*
*
* @param backTestAnomalyDetectorRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BackTestAnomalyDetector operation returned by the service.
* @sample AmazonLookoutMetricsAsyncHandler.BackTestAnomalyDetector
* @see AWS API Documentation
*/
java.util.concurrent.Future backTestAnomalyDetectorAsync(BackTestAnomalyDetectorRequest backTestAnomalyDetectorRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an alert for an anomaly detector.
*
*
* @param createAlertRequest
* @return A Java Future containing the result of the CreateAlert operation returned by the service.
* @sample AmazonLookoutMetricsAsync.CreateAlert
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createAlertAsync(CreateAlertRequest createAlertRequest);
/**
*
* Creates an alert for an anomaly detector.
*
*
* @param createAlertRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateAlert operation returned by the service.
* @sample AmazonLookoutMetricsAsyncHandler.CreateAlert
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createAlertAsync(CreateAlertRequest createAlertRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an anomaly detector.
*
*
* @param createAnomalyDetectorRequest
* @return A Java Future containing the result of the CreateAnomalyDetector operation returned by the service.
* @sample AmazonLookoutMetricsAsync.CreateAnomalyDetector
* @see AWS API Documentation
*/
java.util.concurrent.Future createAnomalyDetectorAsync(CreateAnomalyDetectorRequest createAnomalyDetectorRequest);
/**
*
* Creates an anomaly detector.
*
*
* @param createAnomalyDetectorRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateAnomalyDetector operation returned by the service.
* @sample AmazonLookoutMetricsAsyncHandler.CreateAnomalyDetector
* @see AWS API Documentation
*/
java.util.concurrent.Future createAnomalyDetectorAsync(CreateAnomalyDetectorRequest createAnomalyDetectorRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a dataset.
*
*
* @param createMetricSetRequest
* @return A Java Future containing the result of the CreateMetricSet operation returned by the service.
* @sample AmazonLookoutMetricsAsync.CreateMetricSet
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createMetricSetAsync(CreateMetricSetRequest createMetricSetRequest);
/**
*
* Creates a dataset.
*
*
* @param createMetricSetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateMetricSet operation returned by the service.
* @sample AmazonLookoutMetricsAsyncHandler.CreateMetricSet
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createMetricSetAsync(CreateMetricSetRequest createMetricSetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deactivates an anomaly detector.
*
*
* @param deactivateAnomalyDetectorRequest
* @return A Java Future containing the result of the DeactivateAnomalyDetector operation returned by the service.
* @sample AmazonLookoutMetricsAsync.DeactivateAnomalyDetector
* @see AWS API Documentation
*/
java.util.concurrent.Future deactivateAnomalyDetectorAsync(
DeactivateAnomalyDetectorRequest deactivateAnomalyDetectorRequest);
/**
*
* Deactivates an anomaly detector.
*
*
* @param deactivateAnomalyDetectorRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeactivateAnomalyDetector operation returned by the service.
* @sample AmazonLookoutMetricsAsyncHandler.DeactivateAnomalyDetector
* @see AWS API Documentation
*/
java.util.concurrent.Future deactivateAnomalyDetectorAsync(
DeactivateAnomalyDetectorRequest deactivateAnomalyDetectorRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an alert.
*
*
* @param deleteAlertRequest
* @return A Java Future containing the result of the DeleteAlert operation returned by the service.
* @sample AmazonLookoutMetricsAsync.DeleteAlert
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteAlertAsync(DeleteAlertRequest deleteAlertRequest);
/**
*
* Deletes an alert.
*
*
* @param deleteAlertRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteAlert operation returned by the service.
* @sample AmazonLookoutMetricsAsyncHandler.DeleteAlert
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteAlertAsync(DeleteAlertRequest deleteAlertRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a detector. Deleting an anomaly detector will delete all of its corresponding resources including any
* configured datasets and alerts.
*
*
* @param deleteAnomalyDetectorRequest
* @return A Java Future containing the result of the DeleteAnomalyDetector operation returned by the service.
* @sample AmazonLookoutMetricsAsync.DeleteAnomalyDetector
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteAnomalyDetectorAsync(DeleteAnomalyDetectorRequest deleteAnomalyDetectorRequest);
/**
*
* Deletes a detector. Deleting an anomaly detector will delete all of its corresponding resources including any
* configured datasets and alerts.
*
*
* @param deleteAnomalyDetectorRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteAnomalyDetector operation returned by the service.
* @sample AmazonLookoutMetricsAsyncHandler.DeleteAnomalyDetector
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteAnomalyDetectorAsync(DeleteAnomalyDetectorRequest deleteAnomalyDetectorRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes an alert.
*
*
* Amazon Lookout for Metrics API actions are eventually consistent. If you do a read operation on a resource
* immediately after creating or modifying it, use retries to allow time for the write operation to complete.
*
*
* @param describeAlertRequest
* @return A Java Future containing the result of the DescribeAlert operation returned by the service.
* @sample AmazonLookoutMetricsAsync.DescribeAlert
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeAlertAsync(DescribeAlertRequest describeAlertRequest);
/**
*
* Describes an alert.
*
*
* Amazon Lookout for Metrics API actions are eventually consistent. If you do a read operation on a resource
* immediately after creating or modifying it, use retries to allow time for the write operation to complete.
*
*
* @param describeAlertRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeAlert operation returned by the service.
* @sample AmazonLookoutMetricsAsyncHandler.DescribeAlert
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeAlertAsync(DescribeAlertRequest describeAlertRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about the status of the specified anomaly detection jobs.
*
*
* @param describeAnomalyDetectionExecutionsRequest
* @return A Java Future containing the result of the DescribeAnomalyDetectionExecutions operation returned by the
* service.
* @sample AmazonLookoutMetricsAsync.DescribeAnomalyDetectionExecutions
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAnomalyDetectionExecutionsAsync(
DescribeAnomalyDetectionExecutionsRequest describeAnomalyDetectionExecutionsRequest);
/**
*
* Returns information about the status of the specified anomaly detection jobs.
*
*
* @param describeAnomalyDetectionExecutionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeAnomalyDetectionExecutions operation returned by the
* service.
* @sample AmazonLookoutMetricsAsyncHandler.DescribeAnomalyDetectionExecutions
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAnomalyDetectionExecutionsAsync(
DescribeAnomalyDetectionExecutionsRequest describeAnomalyDetectionExecutionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes a detector.
*
*
* Amazon Lookout for Metrics API actions are eventually consistent. If you do a read operation on a resource
* immediately after creating or modifying it, use retries to allow time for the write operation to complete.
*
*
* @param describeAnomalyDetectorRequest
* @return A Java Future containing the result of the DescribeAnomalyDetector operation returned by the service.
* @sample AmazonLookoutMetricsAsync.DescribeAnomalyDetector
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAnomalyDetectorAsync(DescribeAnomalyDetectorRequest describeAnomalyDetectorRequest);
/**
*
* Describes a detector.
*
*
* Amazon Lookout for Metrics API actions are eventually consistent. If you do a read operation on a resource
* immediately after creating or modifying it, use retries to allow time for the write operation to complete.
*
*
* @param describeAnomalyDetectorRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeAnomalyDetector operation returned by the service.
* @sample AmazonLookoutMetricsAsyncHandler.DescribeAnomalyDetector
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAnomalyDetectorAsync(DescribeAnomalyDetectorRequest describeAnomalyDetectorRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes a dataset.
*
*
* Amazon Lookout for Metrics API actions are eventually consistent. If you do a read operation on a resource
* immediately after creating or modifying it, use retries to allow time for the write operation to complete.
*
*
* @param describeMetricSetRequest
* @return A Java Future containing the result of the DescribeMetricSet operation returned by the service.
* @sample AmazonLookoutMetricsAsync.DescribeMetricSet
* @see AWS API Documentation
*/
java.util.concurrent.Future describeMetricSetAsync(DescribeMetricSetRequest describeMetricSetRequest);
/**
*
* Describes a dataset.
*
*
* Amazon Lookout for Metrics API actions are eventually consistent. If you do a read operation on a resource
* immediately after creating or modifying it, use retries to allow time for the write operation to complete.
*
*
* @param describeMetricSetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeMetricSet operation returned by the service.
* @sample AmazonLookoutMetricsAsyncHandler.DescribeMetricSet
* @see AWS API Documentation
*/
java.util.concurrent.Future describeMetricSetAsync(DescribeMetricSetRequest describeMetricSetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Detects an Amazon S3 dataset's file format, interval, and offset.
*
*
* @param detectMetricSetConfigRequest
* @return A Java Future containing the result of the DetectMetricSetConfig operation returned by the service.
* @sample AmazonLookoutMetricsAsync.DetectMetricSetConfig
* @see AWS API Documentation
*/
java.util.concurrent.Future detectMetricSetConfigAsync(DetectMetricSetConfigRequest detectMetricSetConfigRequest);
/**
*
* Detects an Amazon S3 dataset's file format, interval, and offset.
*
*
* @param detectMetricSetConfigRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DetectMetricSetConfig operation returned by the service.
* @sample AmazonLookoutMetricsAsyncHandler.DetectMetricSetConfig
* @see AWS API Documentation
*/
java.util.concurrent.Future detectMetricSetConfigAsync(DetectMetricSetConfigRequest detectMetricSetConfigRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns details about a group of anomalous metrics.
*
*
* @param getAnomalyGroupRequest
* @return A Java Future containing the result of the GetAnomalyGroup operation returned by the service.
* @sample AmazonLookoutMetricsAsync.GetAnomalyGroup
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getAnomalyGroupAsync(GetAnomalyGroupRequest getAnomalyGroupRequest);
/**
*
* Returns details about a group of anomalous metrics.
*
*
* @param getAnomalyGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetAnomalyGroup operation returned by the service.
* @sample AmazonLookoutMetricsAsyncHandler.GetAnomalyGroup
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getAnomalyGroupAsync(GetAnomalyGroupRequest getAnomalyGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns details about the requested data quality metrics.
*
*
* @param getDataQualityMetricsRequest
* @return A Java Future containing the result of the GetDataQualityMetrics operation returned by the service.
* @sample AmazonLookoutMetricsAsync.GetDataQualityMetrics
* @see AWS API Documentation
*/
java.util.concurrent.Future getDataQualityMetricsAsync(GetDataQualityMetricsRequest getDataQualityMetricsRequest);
/**
*
* Returns details about the requested data quality metrics.
*
*
* @param getDataQualityMetricsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetDataQualityMetrics operation returned by the service.
* @sample AmazonLookoutMetricsAsyncHandler.GetDataQualityMetrics
* @see AWS API Documentation
*/
java.util.concurrent.Future getDataQualityMetricsAsync(GetDataQualityMetricsRequest getDataQualityMetricsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Get feedback for an anomaly group.
*
*
* @param getFeedbackRequest
* @return A Java Future containing the result of the GetFeedback operation returned by the service.
* @sample AmazonLookoutMetricsAsync.GetFeedback
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getFeedbackAsync(GetFeedbackRequest getFeedbackRequest);
/**
*
* Get feedback for an anomaly group.
*
*
* @param getFeedbackRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetFeedback operation returned by the service.
* @sample AmazonLookoutMetricsAsyncHandler.GetFeedback
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getFeedbackAsync(GetFeedbackRequest getFeedbackRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a selection of sample records from an Amazon S3 datasource.
*
*
* @param getSampleDataRequest
* @return A Java Future containing the result of the GetSampleData operation returned by the service.
* @sample AmazonLookoutMetricsAsync.GetSampleData
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getSampleDataAsync(GetSampleDataRequest getSampleDataRequest);
/**
*
* Returns a selection of sample records from an Amazon S3 datasource.
*
*
* @param getSampleDataRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetSampleData operation returned by the service.
* @sample AmazonLookoutMetricsAsyncHandler.GetSampleData
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getSampleDataAsync(GetSampleDataRequest getSampleDataRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the alerts attached to a detector.
*
*
* Amazon Lookout for Metrics API actions are eventually consistent. If you do a read operation on a resource
* immediately after creating or modifying it, use retries to allow time for the write operation to complete.
*
*
* @param listAlertsRequest
* @return A Java Future containing the result of the ListAlerts operation returned by the service.
* @sample AmazonLookoutMetricsAsync.ListAlerts
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listAlertsAsync(ListAlertsRequest listAlertsRequest);
/**
*
* Lists the alerts attached to a detector.
*
*
* Amazon Lookout for Metrics API actions are eventually consistent. If you do a read operation on a resource
* immediately after creating or modifying it, use retries to allow time for the write operation to complete.
*
*
* @param listAlertsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListAlerts operation returned by the service.
* @sample AmazonLookoutMetricsAsyncHandler.ListAlerts
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listAlertsAsync(ListAlertsRequest listAlertsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the detectors in the current AWS Region.
*
*
* Amazon Lookout for Metrics API actions are eventually consistent. If you do a read operation on a resource
* immediately after creating or modifying it, use retries to allow time for the write operation to complete.
*
*
* @param listAnomalyDetectorsRequest
* @return A Java Future containing the result of the ListAnomalyDetectors operation returned by the service.
* @sample AmazonLookoutMetricsAsync.ListAnomalyDetectors
* @see AWS API Documentation
*/
java.util.concurrent.Future listAnomalyDetectorsAsync(ListAnomalyDetectorsRequest listAnomalyDetectorsRequest);
/**
*
* Lists the detectors in the current AWS Region.
*
*
* Amazon Lookout for Metrics API actions are eventually consistent. If you do a read operation on a resource
* immediately after creating or modifying it, use retries to allow time for the write operation to complete.
*
*
* @param listAnomalyDetectorsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListAnomalyDetectors operation returned by the service.
* @sample AmazonLookoutMetricsAsyncHandler.ListAnomalyDetectors
* @see AWS API Documentation
*/
java.util.concurrent.Future listAnomalyDetectorsAsync(ListAnomalyDetectorsRequest listAnomalyDetectorsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of measures that are potential causes or effects of an anomaly group.
*
*
* @param listAnomalyGroupRelatedMetricsRequest
* @return A Java Future containing the result of the ListAnomalyGroupRelatedMetrics operation returned by the
* service.
* @sample AmazonLookoutMetricsAsync.ListAnomalyGroupRelatedMetrics
* @see AWS API Documentation
*/
java.util.concurrent.Future listAnomalyGroupRelatedMetricsAsync(
ListAnomalyGroupRelatedMetricsRequest listAnomalyGroupRelatedMetricsRequest);
/**
*
* Returns a list of measures that are potential causes or effects of an anomaly group.
*
*
* @param listAnomalyGroupRelatedMetricsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListAnomalyGroupRelatedMetrics operation returned by the
* service.
* @sample AmazonLookoutMetricsAsyncHandler.ListAnomalyGroupRelatedMetrics
* @see AWS API Documentation
*/
java.util.concurrent.Future listAnomalyGroupRelatedMetricsAsync(
ListAnomalyGroupRelatedMetricsRequest listAnomalyGroupRelatedMetricsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of anomaly groups.
*
*
* @param listAnomalyGroupSummariesRequest
* @return A Java Future containing the result of the ListAnomalyGroupSummaries operation returned by the service.
* @sample AmazonLookoutMetricsAsync.ListAnomalyGroupSummaries
* @see AWS API Documentation
*/
java.util.concurrent.Future listAnomalyGroupSummariesAsync(
ListAnomalyGroupSummariesRequest listAnomalyGroupSummariesRequest);
/**
*
* Returns a list of anomaly groups.
*
*
* @param listAnomalyGroupSummariesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListAnomalyGroupSummaries operation returned by the service.
* @sample AmazonLookoutMetricsAsyncHandler.ListAnomalyGroupSummaries
* @see AWS API Documentation
*/
java.util.concurrent.Future listAnomalyGroupSummariesAsync(
ListAnomalyGroupSummariesRequest listAnomalyGroupSummariesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a list of anomalous metrics for a measure in an anomaly group.
*
*
* @param listAnomalyGroupTimeSeriesRequest
* @return A Java Future containing the result of the ListAnomalyGroupTimeSeries operation returned by the service.
* @sample AmazonLookoutMetricsAsync.ListAnomalyGroupTimeSeries
* @see AWS API Documentation
*/
java.util.concurrent.Future listAnomalyGroupTimeSeriesAsync(
ListAnomalyGroupTimeSeriesRequest listAnomalyGroupTimeSeriesRequest);
/**
*
* Gets a list of anomalous metrics for a measure in an anomaly group.
*
*
* @param listAnomalyGroupTimeSeriesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListAnomalyGroupTimeSeries operation returned by the service.
* @sample AmazonLookoutMetricsAsyncHandler.ListAnomalyGroupTimeSeries
* @see AWS API Documentation
*/
java.util.concurrent.Future listAnomalyGroupTimeSeriesAsync(
ListAnomalyGroupTimeSeriesRequest listAnomalyGroupTimeSeriesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the datasets in the current AWS Region.
*
*
* Amazon Lookout for Metrics API actions are eventually consistent. If you do a read operation on a resource
* immediately after creating or modifying it, use retries to allow time for the write operation to complete.
*
*
* @param listMetricSetsRequest
* @return A Java Future containing the result of the ListMetricSets operation returned by the service.
* @sample AmazonLookoutMetricsAsync.ListMetricSets
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listMetricSetsAsync(ListMetricSetsRequest listMetricSetsRequest);
/**
*
* Lists the datasets in the current AWS Region.
*
*
* Amazon Lookout for Metrics API actions are eventually consistent. If you do a read operation on a resource
* immediately after creating or modifying it, use retries to allow time for the write operation to complete.
*
*
* @param listMetricSetsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListMetricSets operation returned by the service.
* @sample AmazonLookoutMetricsAsyncHandler.ListMetricSets
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listMetricSetsAsync(ListMetricSetsRequest listMetricSetsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a list of tags for a
* detector, dataset, or alert.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AmazonLookoutMetricsAsync.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Gets a list of tags for a
* detector, dataset, or alert.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AmazonLookoutMetricsAsyncHandler.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Add feedback for an anomalous metric.
*
*
* @param putFeedbackRequest
* @return A Java Future containing the result of the PutFeedback operation returned by the service.
* @sample AmazonLookoutMetricsAsync.PutFeedback
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putFeedbackAsync(PutFeedbackRequest putFeedbackRequest);
/**
*
* Add feedback for an anomalous metric.
*
*
* @param putFeedbackRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutFeedback operation returned by the service.
* @sample AmazonLookoutMetricsAsyncHandler.PutFeedback
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putFeedbackAsync(PutFeedbackRequest putFeedbackRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds tags to a detector,
* dataset, or alert.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AmazonLookoutMetricsAsync.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* Adds tags to a detector,
* dataset, or alert.
*
*
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AmazonLookoutMetricsAsyncHandler.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes tags from a
* detector, dataset, or alert.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AmazonLookoutMetricsAsync.UntagResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* Removes tags from a
* detector, dataset, or alert.
*
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AmazonLookoutMetricsAsyncHandler.UntagResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Make changes to an existing alert.
*
*
* @param updateAlertRequest
* @return A Java Future containing the result of the UpdateAlert operation returned by the service.
* @sample AmazonLookoutMetricsAsync.UpdateAlert
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateAlertAsync(UpdateAlertRequest updateAlertRequest);
/**
*
* Make changes to an existing alert.
*
*
* @param updateAlertRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateAlert operation returned by the service.
* @sample AmazonLookoutMetricsAsyncHandler.UpdateAlert
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateAlertAsync(UpdateAlertRequest updateAlertRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates a detector. After activation, you can only change a detector's ingestion delay and description.
*
*
* @param updateAnomalyDetectorRequest
* @return A Java Future containing the result of the UpdateAnomalyDetector operation returned by the service.
* @sample AmazonLookoutMetricsAsync.UpdateAnomalyDetector
* @see AWS API Documentation
*/
java.util.concurrent.Future updateAnomalyDetectorAsync(UpdateAnomalyDetectorRequest updateAnomalyDetectorRequest);
/**
*
* Updates a detector. After activation, you can only change a detector's ingestion delay and description.
*
*
* @param updateAnomalyDetectorRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateAnomalyDetector operation returned by the service.
* @sample AmazonLookoutMetricsAsyncHandler.UpdateAnomalyDetector
* @see AWS API Documentation
*/
java.util.concurrent.Future updateAnomalyDetectorAsync(UpdateAnomalyDetectorRequest updateAnomalyDetectorRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates a dataset.
*
*
* @param updateMetricSetRequest
* @return A Java Future containing the result of the UpdateMetricSet operation returned by the service.
* @sample AmazonLookoutMetricsAsync.UpdateMetricSet
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateMetricSetAsync(UpdateMetricSetRequest updateMetricSetRequest);
/**
*
* Updates a dataset.
*
*
* @param updateMetricSetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateMetricSet operation returned by the service.
* @sample AmazonLookoutMetricsAsyncHandler.UpdateMetricSet
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateMetricSetAsync(UpdateMetricSetRequest updateMetricSetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}