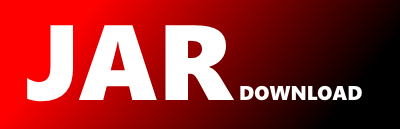
com.amazonaws.services.lookoutmetrics.model.UpdateMetricSetRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-lookoutmetrics Show documentation
/*
* Copyright 2018-2023 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.lookoutmetrics.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class UpdateMetricSetRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The ARN of the dataset to update.
*
*/
private String metricSetArn;
/**
*
* The dataset's description.
*
*/
private String metricSetDescription;
/**
*
* The metric list.
*
*/
private java.util.List metricList;
/**
*
* After an interval ends, the amount of seconds that the detector waits before importing data. Offset is only
* supported for S3, Redshift, Athena and datasources.
*
*/
private Integer offset;
/**
*
* The timestamp column.
*
*/
private TimestampColumn timestampColumn;
/**
*
* The dimension list.
*
*/
private java.util.List dimensionList;
/**
*
* The dataset's interval.
*
*/
private String metricSetFrequency;
private MetricSource metricSource;
/**
*
* Describes a list of filters for choosing specific dimensions and specific values. Each filter consists of the
* dimension and one of its values that you want to include. When multiple dimensions or values are specified, the
* dimensions are joined with an AND operation and the values are joined with an OR operation.
*
*/
private java.util.List dimensionFilterList;
/**
*
* The ARN of the dataset to update.
*
*
* @param metricSetArn
* The ARN of the dataset to update.
*/
public void setMetricSetArn(String metricSetArn) {
this.metricSetArn = metricSetArn;
}
/**
*
* The ARN of the dataset to update.
*
*
* @return The ARN of the dataset to update.
*/
public String getMetricSetArn() {
return this.metricSetArn;
}
/**
*
* The ARN of the dataset to update.
*
*
* @param metricSetArn
* The ARN of the dataset to update.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateMetricSetRequest withMetricSetArn(String metricSetArn) {
setMetricSetArn(metricSetArn);
return this;
}
/**
*
* The dataset's description.
*
*
* @param metricSetDescription
* The dataset's description.
*/
public void setMetricSetDescription(String metricSetDescription) {
this.metricSetDescription = metricSetDescription;
}
/**
*
* The dataset's description.
*
*
* @return The dataset's description.
*/
public String getMetricSetDescription() {
return this.metricSetDescription;
}
/**
*
* The dataset's description.
*
*
* @param metricSetDescription
* The dataset's description.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateMetricSetRequest withMetricSetDescription(String metricSetDescription) {
setMetricSetDescription(metricSetDescription);
return this;
}
/**
*
* The metric list.
*
*
* @return The metric list.
*/
public java.util.List getMetricList() {
return metricList;
}
/**
*
* The metric list.
*
*
* @param metricList
* The metric list.
*/
public void setMetricList(java.util.Collection metricList) {
if (metricList == null) {
this.metricList = null;
return;
}
this.metricList = new java.util.ArrayList(metricList);
}
/**
*
* The metric list.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setMetricList(java.util.Collection)} or {@link #withMetricList(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param metricList
* The metric list.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateMetricSetRequest withMetricList(Metric... metricList) {
if (this.metricList == null) {
setMetricList(new java.util.ArrayList(metricList.length));
}
for (Metric ele : metricList) {
this.metricList.add(ele);
}
return this;
}
/**
*
* The metric list.
*
*
* @param metricList
* The metric list.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateMetricSetRequest withMetricList(java.util.Collection metricList) {
setMetricList(metricList);
return this;
}
/**
*
* After an interval ends, the amount of seconds that the detector waits before importing data. Offset is only
* supported for S3, Redshift, Athena and datasources.
*
*
* @param offset
* After an interval ends, the amount of seconds that the detector waits before importing data. Offset is
* only supported for S3, Redshift, Athena and datasources.
*/
public void setOffset(Integer offset) {
this.offset = offset;
}
/**
*
* After an interval ends, the amount of seconds that the detector waits before importing data. Offset is only
* supported for S3, Redshift, Athena and datasources.
*
*
* @return After an interval ends, the amount of seconds that the detector waits before importing data. Offset is
* only supported for S3, Redshift, Athena and datasources.
*/
public Integer getOffset() {
return this.offset;
}
/**
*
* After an interval ends, the amount of seconds that the detector waits before importing data. Offset is only
* supported for S3, Redshift, Athena and datasources.
*
*
* @param offset
* After an interval ends, the amount of seconds that the detector waits before importing data. Offset is
* only supported for S3, Redshift, Athena and datasources.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateMetricSetRequest withOffset(Integer offset) {
setOffset(offset);
return this;
}
/**
*
* The timestamp column.
*
*
* @param timestampColumn
* The timestamp column.
*/
public void setTimestampColumn(TimestampColumn timestampColumn) {
this.timestampColumn = timestampColumn;
}
/**
*
* The timestamp column.
*
*
* @return The timestamp column.
*/
public TimestampColumn getTimestampColumn() {
return this.timestampColumn;
}
/**
*
* The timestamp column.
*
*
* @param timestampColumn
* The timestamp column.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateMetricSetRequest withTimestampColumn(TimestampColumn timestampColumn) {
setTimestampColumn(timestampColumn);
return this;
}
/**
*
* The dimension list.
*
*
* @return The dimension list.
*/
public java.util.List getDimensionList() {
return dimensionList;
}
/**
*
* The dimension list.
*
*
* @param dimensionList
* The dimension list.
*/
public void setDimensionList(java.util.Collection dimensionList) {
if (dimensionList == null) {
this.dimensionList = null;
return;
}
this.dimensionList = new java.util.ArrayList(dimensionList);
}
/**
*
* The dimension list.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setDimensionList(java.util.Collection)} or {@link #withDimensionList(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param dimensionList
* The dimension list.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateMetricSetRequest withDimensionList(String... dimensionList) {
if (this.dimensionList == null) {
setDimensionList(new java.util.ArrayList(dimensionList.length));
}
for (String ele : dimensionList) {
this.dimensionList.add(ele);
}
return this;
}
/**
*
* The dimension list.
*
*
* @param dimensionList
* The dimension list.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateMetricSetRequest withDimensionList(java.util.Collection dimensionList) {
setDimensionList(dimensionList);
return this;
}
/**
*
* The dataset's interval.
*
*
* @param metricSetFrequency
* The dataset's interval.
* @see Frequency
*/
public void setMetricSetFrequency(String metricSetFrequency) {
this.metricSetFrequency = metricSetFrequency;
}
/**
*
* The dataset's interval.
*
*
* @return The dataset's interval.
* @see Frequency
*/
public String getMetricSetFrequency() {
return this.metricSetFrequency;
}
/**
*
* The dataset's interval.
*
*
* @param metricSetFrequency
* The dataset's interval.
* @return Returns a reference to this object so that method calls can be chained together.
* @see Frequency
*/
public UpdateMetricSetRequest withMetricSetFrequency(String metricSetFrequency) {
setMetricSetFrequency(metricSetFrequency);
return this;
}
/**
*
* The dataset's interval.
*
*
* @param metricSetFrequency
* The dataset's interval.
* @return Returns a reference to this object so that method calls can be chained together.
* @see Frequency
*/
public UpdateMetricSetRequest withMetricSetFrequency(Frequency metricSetFrequency) {
this.metricSetFrequency = metricSetFrequency.toString();
return this;
}
/**
* @param metricSource
*/
public void setMetricSource(MetricSource metricSource) {
this.metricSource = metricSource;
}
/**
* @return
*/
public MetricSource getMetricSource() {
return this.metricSource;
}
/**
* @param metricSource
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateMetricSetRequest withMetricSource(MetricSource metricSource) {
setMetricSource(metricSource);
return this;
}
/**
*
* Describes a list of filters for choosing specific dimensions and specific values. Each filter consists of the
* dimension and one of its values that you want to include. When multiple dimensions or values are specified, the
* dimensions are joined with an AND operation and the values are joined with an OR operation.
*
*
* @return Describes a list of filters for choosing specific dimensions and specific values. Each filter consists of
* the dimension and one of its values that you want to include. When multiple dimensions or values are
* specified, the dimensions are joined with an AND operation and the values are joined with an OR
* operation.
*/
public java.util.List getDimensionFilterList() {
return dimensionFilterList;
}
/**
*
* Describes a list of filters for choosing specific dimensions and specific values. Each filter consists of the
* dimension and one of its values that you want to include. When multiple dimensions or values are specified, the
* dimensions are joined with an AND operation and the values are joined with an OR operation.
*
*
* @param dimensionFilterList
* Describes a list of filters for choosing specific dimensions and specific values. Each filter consists of
* the dimension and one of its values that you want to include. When multiple dimensions or values are
* specified, the dimensions are joined with an AND operation and the values are joined with an OR operation.
*/
public void setDimensionFilterList(java.util.Collection dimensionFilterList) {
if (dimensionFilterList == null) {
this.dimensionFilterList = null;
return;
}
this.dimensionFilterList = new java.util.ArrayList(dimensionFilterList);
}
/**
*
* Describes a list of filters for choosing specific dimensions and specific values. Each filter consists of the
* dimension and one of its values that you want to include. When multiple dimensions or values are specified, the
* dimensions are joined with an AND operation and the values are joined with an OR operation.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setDimensionFilterList(java.util.Collection)} or {@link #withDimensionFilterList(java.util.Collection)}
* if you want to override the existing values.
*
*
* @param dimensionFilterList
* Describes a list of filters for choosing specific dimensions and specific values. Each filter consists of
* the dimension and one of its values that you want to include. When multiple dimensions or values are
* specified, the dimensions are joined with an AND operation and the values are joined with an OR operation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateMetricSetRequest withDimensionFilterList(MetricSetDimensionFilter... dimensionFilterList) {
if (this.dimensionFilterList == null) {
setDimensionFilterList(new java.util.ArrayList(dimensionFilterList.length));
}
for (MetricSetDimensionFilter ele : dimensionFilterList) {
this.dimensionFilterList.add(ele);
}
return this;
}
/**
*
* Describes a list of filters for choosing specific dimensions and specific values. Each filter consists of the
* dimension and one of its values that you want to include. When multiple dimensions or values are specified, the
* dimensions are joined with an AND operation and the values are joined with an OR operation.
*
*
* @param dimensionFilterList
* Describes a list of filters for choosing specific dimensions and specific values. Each filter consists of
* the dimension and one of its values that you want to include. When multiple dimensions or values are
* specified, the dimensions are joined with an AND operation and the values are joined with an OR operation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateMetricSetRequest withDimensionFilterList(java.util.Collection dimensionFilterList) {
setDimensionFilterList(dimensionFilterList);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getMetricSetArn() != null)
sb.append("MetricSetArn: ").append(getMetricSetArn()).append(",");
if (getMetricSetDescription() != null)
sb.append("MetricSetDescription: ").append(getMetricSetDescription()).append(",");
if (getMetricList() != null)
sb.append("MetricList: ").append(getMetricList()).append(",");
if (getOffset() != null)
sb.append("Offset: ").append(getOffset()).append(",");
if (getTimestampColumn() != null)
sb.append("TimestampColumn: ").append(getTimestampColumn()).append(",");
if (getDimensionList() != null)
sb.append("DimensionList: ").append(getDimensionList()).append(",");
if (getMetricSetFrequency() != null)
sb.append("MetricSetFrequency: ").append(getMetricSetFrequency()).append(",");
if (getMetricSource() != null)
sb.append("MetricSource: ").append(getMetricSource()).append(",");
if (getDimensionFilterList() != null)
sb.append("DimensionFilterList: ").append(getDimensionFilterList());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof UpdateMetricSetRequest == false)
return false;
UpdateMetricSetRequest other = (UpdateMetricSetRequest) obj;
if (other.getMetricSetArn() == null ^ this.getMetricSetArn() == null)
return false;
if (other.getMetricSetArn() != null && other.getMetricSetArn().equals(this.getMetricSetArn()) == false)
return false;
if (other.getMetricSetDescription() == null ^ this.getMetricSetDescription() == null)
return false;
if (other.getMetricSetDescription() != null && other.getMetricSetDescription().equals(this.getMetricSetDescription()) == false)
return false;
if (other.getMetricList() == null ^ this.getMetricList() == null)
return false;
if (other.getMetricList() != null && other.getMetricList().equals(this.getMetricList()) == false)
return false;
if (other.getOffset() == null ^ this.getOffset() == null)
return false;
if (other.getOffset() != null && other.getOffset().equals(this.getOffset()) == false)
return false;
if (other.getTimestampColumn() == null ^ this.getTimestampColumn() == null)
return false;
if (other.getTimestampColumn() != null && other.getTimestampColumn().equals(this.getTimestampColumn()) == false)
return false;
if (other.getDimensionList() == null ^ this.getDimensionList() == null)
return false;
if (other.getDimensionList() != null && other.getDimensionList().equals(this.getDimensionList()) == false)
return false;
if (other.getMetricSetFrequency() == null ^ this.getMetricSetFrequency() == null)
return false;
if (other.getMetricSetFrequency() != null && other.getMetricSetFrequency().equals(this.getMetricSetFrequency()) == false)
return false;
if (other.getMetricSource() == null ^ this.getMetricSource() == null)
return false;
if (other.getMetricSource() != null && other.getMetricSource().equals(this.getMetricSource()) == false)
return false;
if (other.getDimensionFilterList() == null ^ this.getDimensionFilterList() == null)
return false;
if (other.getDimensionFilterList() != null && other.getDimensionFilterList().equals(this.getDimensionFilterList()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getMetricSetArn() == null) ? 0 : getMetricSetArn().hashCode());
hashCode = prime * hashCode + ((getMetricSetDescription() == null) ? 0 : getMetricSetDescription().hashCode());
hashCode = prime * hashCode + ((getMetricList() == null) ? 0 : getMetricList().hashCode());
hashCode = prime * hashCode + ((getOffset() == null) ? 0 : getOffset().hashCode());
hashCode = prime * hashCode + ((getTimestampColumn() == null) ? 0 : getTimestampColumn().hashCode());
hashCode = prime * hashCode + ((getDimensionList() == null) ? 0 : getDimensionList().hashCode());
hashCode = prime * hashCode + ((getMetricSetFrequency() == null) ? 0 : getMetricSetFrequency().hashCode());
hashCode = prime * hashCode + ((getMetricSource() == null) ? 0 : getMetricSource().hashCode());
hashCode = prime * hashCode + ((getDimensionFilterList() == null) ? 0 : getDimensionFilterList().hashCode());
return hashCode;
}
@Override
public UpdateMetricSetRequest clone() {
return (UpdateMetricSetRequest) super.clone();
}
}