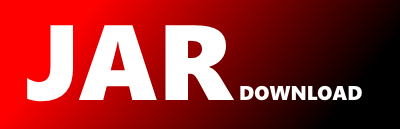
com.amazonaws.services.machinelearning.AmazonMachineLearningAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-machinelearning Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.machinelearning;
import javax.annotation.Generated;
import com.amazonaws.services.machinelearning.model.*;
/**
* Interface for accessing Amazon Machine Learning asynchronously. Each asynchronous method will return a Java Future
* object representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.machinelearning.AbstractAmazonMachineLearningAsync} instead.
*
*
* Definition of the public APIs exposed by Amazon Machine Learning
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonMachineLearningAsync extends AmazonMachineLearning {
/**
*
* Adds one or more tags to an object, up to a limit of 10. Each tag consists of a key and an optional value. If you
* add a tag using a key that is already associated with the ML object, AddTags
updates the tag's
* value.
*
*
* @param addTagsRequest
* @return A Java Future containing the result of the AddTags operation returned by the service.
* @sample AmazonMachineLearningAsync.AddTags
*/
java.util.concurrent.Future addTagsAsync(AddTagsRequest addTagsRequest);
/**
*
* Adds one or more tags to an object, up to a limit of 10. Each tag consists of a key and an optional value. If you
* add a tag using a key that is already associated with the ML object, AddTags
updates the tag's
* value.
*
*
* @param addTagsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AddTags operation returned by the service.
* @sample AmazonMachineLearningAsyncHandler.AddTags
*/
java.util.concurrent.Future addTagsAsync(AddTagsRequest addTagsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Generates predictions for a group of observations. The observations to process exist in one or more data files
* referenced by a DataSource
. This operation creates a new BatchPrediction
, and uses an
* MLModel
and the data files referenced by the DataSource
as information sources.
*
*
* CreateBatchPrediction
is an asynchronous operation. In response to
* CreateBatchPrediction
, Amazon Machine Learning (Amazon ML) immediately returns and sets the
* BatchPrediction
status to PENDING
. After the BatchPrediction
completes,
* Amazon ML sets the status to COMPLETED
.
*
*
* You can poll for status updates by using the GetBatchPrediction operation and checking the
* Status
parameter of the result. After the COMPLETED
status appears, the results are
* available in the location specified by the OutputUri
parameter.
*
*
* @param createBatchPredictionRequest
* @return A Java Future containing the result of the CreateBatchPrediction operation returned by the service.
* @sample AmazonMachineLearningAsync.CreateBatchPrediction
*/
java.util.concurrent.Future createBatchPredictionAsync(CreateBatchPredictionRequest createBatchPredictionRequest);
/**
*
* Generates predictions for a group of observations. The observations to process exist in one or more data files
* referenced by a DataSource
. This operation creates a new BatchPrediction
, and uses an
* MLModel
and the data files referenced by the DataSource
as information sources.
*
*
* CreateBatchPrediction
is an asynchronous operation. In response to
* CreateBatchPrediction
, Amazon Machine Learning (Amazon ML) immediately returns and sets the
* BatchPrediction
status to PENDING
. After the BatchPrediction
completes,
* Amazon ML sets the status to COMPLETED
.
*
*
* You can poll for status updates by using the GetBatchPrediction operation and checking the
* Status
parameter of the result. After the COMPLETED
status appears, the results are
* available in the location specified by the OutputUri
parameter.
*
*
* @param createBatchPredictionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateBatchPrediction operation returned by the service.
* @sample AmazonMachineLearningAsyncHandler.CreateBatchPrediction
*/
java.util.concurrent.Future createBatchPredictionAsync(CreateBatchPredictionRequest createBatchPredictionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a DataSource
object from an Amazon Relational Database
* Service (Amazon RDS). A DataSource
references data that can be used to perform
* CreateMLModel
, CreateEvaluation
, or CreateBatchPrediction
operations.
*
*
* CreateDataSourceFromRDS
is an asynchronous operation. In response to
* CreateDataSourceFromRDS
, Amazon Machine Learning (Amazon ML) immediately returns and sets the
* DataSource
status to PENDING
. After the DataSource
is created and ready
* for use, Amazon ML sets the Status
parameter to COMPLETED
. DataSource
in
* the COMPLETED
or PENDING
state can be used only to perform
* >CreateMLModel
>, CreateEvaluation
, or CreateBatchPrediction
* operations.
*
*
* If Amazon ML cannot accept the input source, it sets the Status
parameter to FAILED
and
* includes an error message in the Message
attribute of the GetDataSource
operation
* response.
*
*
* @param createDataSourceFromRDSRequest
* @return A Java Future containing the result of the CreateDataSourceFromRDS operation returned by the service.
* @sample AmazonMachineLearningAsync.CreateDataSourceFromRDS
*/
java.util.concurrent.Future createDataSourceFromRDSAsync(CreateDataSourceFromRDSRequest createDataSourceFromRDSRequest);
/**
*
* Creates a DataSource
object from an Amazon Relational Database
* Service (Amazon RDS). A DataSource
references data that can be used to perform
* CreateMLModel
, CreateEvaluation
, or CreateBatchPrediction
operations.
*
*
* CreateDataSourceFromRDS
is an asynchronous operation. In response to
* CreateDataSourceFromRDS
, Amazon Machine Learning (Amazon ML) immediately returns and sets the
* DataSource
status to PENDING
. After the DataSource
is created and ready
* for use, Amazon ML sets the Status
parameter to COMPLETED
. DataSource
in
* the COMPLETED
or PENDING
state can be used only to perform
* >CreateMLModel
>, CreateEvaluation
, or CreateBatchPrediction
* operations.
*
*
* If Amazon ML cannot accept the input source, it sets the Status
parameter to FAILED
and
* includes an error message in the Message
attribute of the GetDataSource
operation
* response.
*
*
* @param createDataSourceFromRDSRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDataSourceFromRDS operation returned by the service.
* @sample AmazonMachineLearningAsyncHandler.CreateDataSourceFromRDS
*/
java.util.concurrent.Future createDataSourceFromRDSAsync(CreateDataSourceFromRDSRequest createDataSourceFromRDSRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a DataSource
from a database hosted on an Amazon Redshift cluster. A DataSource
* references data that can be used to perform either CreateMLModel
, CreateEvaluation
, or
* CreateBatchPrediction
operations.
*
*
* CreateDataSourceFromRedshift
is an asynchronous operation. In response to
* CreateDataSourceFromRedshift
, Amazon Machine Learning (Amazon ML) immediately returns and sets the
* DataSource
status to PENDING
. After the DataSource
is created and ready
* for use, Amazon ML sets the Status
parameter to COMPLETED
. DataSource
in
* COMPLETED
or PENDING
states can be used to perform only CreateMLModel
,
* CreateEvaluation
, or CreateBatchPrediction
operations.
*
*
* If Amazon ML can't accept the input source, it sets the Status
parameter to FAILED
and
* includes an error message in the Message
attribute of the GetDataSource
operation
* response.
*
*
* The observations should be contained in the database hosted on an Amazon Redshift cluster and should be specified
* by a SelectSqlQuery
query. Amazon ML executes an Unload
command in Amazon Redshift to
* transfer the result set of the SelectSqlQuery
query to S3StagingLocation
.
*
*
* After the DataSource
has been created, it's ready for use in evaluations and batch predictions. If
* you plan to use the DataSource
to train an MLModel
, the DataSource
also
* requires a recipe. A recipe describes how each input variable will be used in training an MLModel
.
* Will the variable be included or excluded from training? Will the variable be manipulated; for example, will it
* be combined with another variable or will it be split apart into word combinations? The recipe provides answers
* to these questions.
*
*
* You can't change an existing datasource, but you can copy and modify the settings from an existing Amazon
* Redshift datasource to create a new datasource. To do so, call GetDataSource
for an existing
* datasource and copy the values to a CreateDataSource
call. Change the settings that you want to
* change and make sure that all required fields have the appropriate values.
*
*
* @param createDataSourceFromRedshiftRequest
* @return A Java Future containing the result of the CreateDataSourceFromRedshift operation returned by the
* service.
* @sample AmazonMachineLearningAsync.CreateDataSourceFromRedshift
*/
java.util.concurrent.Future createDataSourceFromRedshiftAsync(
CreateDataSourceFromRedshiftRequest createDataSourceFromRedshiftRequest);
/**
*
* Creates a DataSource
from a database hosted on an Amazon Redshift cluster. A DataSource
* references data that can be used to perform either CreateMLModel
, CreateEvaluation
, or
* CreateBatchPrediction
operations.
*
*
* CreateDataSourceFromRedshift
is an asynchronous operation. In response to
* CreateDataSourceFromRedshift
, Amazon Machine Learning (Amazon ML) immediately returns and sets the
* DataSource
status to PENDING
. After the DataSource
is created and ready
* for use, Amazon ML sets the Status
parameter to COMPLETED
. DataSource
in
* COMPLETED
or PENDING
states can be used to perform only CreateMLModel
,
* CreateEvaluation
, or CreateBatchPrediction
operations.
*
*
* If Amazon ML can't accept the input source, it sets the Status
parameter to FAILED
and
* includes an error message in the Message
attribute of the GetDataSource
operation
* response.
*
*
* The observations should be contained in the database hosted on an Amazon Redshift cluster and should be specified
* by a SelectSqlQuery
query. Amazon ML executes an Unload
command in Amazon Redshift to
* transfer the result set of the SelectSqlQuery
query to S3StagingLocation
.
*
*
* After the DataSource
has been created, it's ready for use in evaluations and batch predictions. If
* you plan to use the DataSource
to train an MLModel
, the DataSource
also
* requires a recipe. A recipe describes how each input variable will be used in training an MLModel
.
* Will the variable be included or excluded from training? Will the variable be manipulated; for example, will it
* be combined with another variable or will it be split apart into word combinations? The recipe provides answers
* to these questions.
*
*
* You can't change an existing datasource, but you can copy and modify the settings from an existing Amazon
* Redshift datasource to create a new datasource. To do so, call GetDataSource
for an existing
* datasource and copy the values to a CreateDataSource
call. Change the settings that you want to
* change and make sure that all required fields have the appropriate values.
*
*
* @param createDataSourceFromRedshiftRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDataSourceFromRedshift operation returned by the
* service.
* @sample AmazonMachineLearningAsyncHandler.CreateDataSourceFromRedshift
*/
java.util.concurrent.Future createDataSourceFromRedshiftAsync(
CreateDataSourceFromRedshiftRequest createDataSourceFromRedshiftRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a DataSource
object. A DataSource
references data that can be used to perform
* CreateMLModel
, CreateEvaluation
, or CreateBatchPrediction
operations.
*
*
* CreateDataSourceFromS3
is an asynchronous operation. In response to
* CreateDataSourceFromS3
, Amazon Machine Learning (Amazon ML) immediately returns and sets the
* DataSource
status to PENDING
. After the DataSource
has been created and is
* ready for use, Amazon ML sets the Status
parameter to COMPLETED
.
* DataSource
in the COMPLETED
or PENDING
state can be used to perform only
* CreateMLModel
, CreateEvaluation
or CreateBatchPrediction
operations.
*
*
* If Amazon ML can't accept the input source, it sets the Status
parameter to FAILED
and
* includes an error message in the Message
attribute of the GetDataSource
operation
* response.
*
*
* The observation data used in a DataSource
should be ready to use; that is, it should have a
* consistent structure, and missing data values should be kept to a minimum. The observation data must reside in
* one or more .csv files in an Amazon Simple Storage Service (Amazon S3) location, along with a schema that
* describes the data items by name and type. The same schema must be used for all of the data files referenced by
* the DataSource
.
*
*
* After the DataSource
has been created, it's ready to use in evaluations and batch predictions. If
* you plan to use the DataSource
to train an MLModel
, the DataSource
also
* needs a recipe. A recipe describes how each input variable will be used in training an MLModel
. Will
* the variable be included or excluded from training? Will the variable be manipulated; for example, will it be
* combined with another variable or will it be split apart into word combinations? The recipe provides answers to
* these questions.
*
*
* @param createDataSourceFromS3Request
* @return A Java Future containing the result of the CreateDataSourceFromS3 operation returned by the service.
* @sample AmazonMachineLearningAsync.CreateDataSourceFromS3
*/
java.util.concurrent.Future createDataSourceFromS3Async(CreateDataSourceFromS3Request createDataSourceFromS3Request);
/**
*
* Creates a DataSource
object. A DataSource
references data that can be used to perform
* CreateMLModel
, CreateEvaluation
, or CreateBatchPrediction
operations.
*
*
* CreateDataSourceFromS3
is an asynchronous operation. In response to
* CreateDataSourceFromS3
, Amazon Machine Learning (Amazon ML) immediately returns and sets the
* DataSource
status to PENDING
. After the DataSource
has been created and is
* ready for use, Amazon ML sets the Status
parameter to COMPLETED
.
* DataSource
in the COMPLETED
or PENDING
state can be used to perform only
* CreateMLModel
, CreateEvaluation
or CreateBatchPrediction
operations.
*
*
* If Amazon ML can't accept the input source, it sets the Status
parameter to FAILED
and
* includes an error message in the Message
attribute of the GetDataSource
operation
* response.
*
*
* The observation data used in a DataSource
should be ready to use; that is, it should have a
* consistent structure, and missing data values should be kept to a minimum. The observation data must reside in
* one or more .csv files in an Amazon Simple Storage Service (Amazon S3) location, along with a schema that
* describes the data items by name and type. The same schema must be used for all of the data files referenced by
* the DataSource
.
*
*
* After the DataSource
has been created, it's ready to use in evaluations and batch predictions. If
* you plan to use the DataSource
to train an MLModel
, the DataSource
also
* needs a recipe. A recipe describes how each input variable will be used in training an MLModel
. Will
* the variable be included or excluded from training? Will the variable be manipulated; for example, will it be
* combined with another variable or will it be split apart into word combinations? The recipe provides answers to
* these questions.
*
*
* @param createDataSourceFromS3Request
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDataSourceFromS3 operation returned by the service.
* @sample AmazonMachineLearningAsyncHandler.CreateDataSourceFromS3
*/
java.util.concurrent.Future createDataSourceFromS3Async(CreateDataSourceFromS3Request createDataSourceFromS3Request,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new Evaluation
of an MLModel
. An MLModel
is evaluated on a set
* of observations associated to a DataSource
. Like a DataSource
for an
* MLModel
, the DataSource
for an Evaluation
contains values for the
* Target Variable
. The Evaluation
compares the predicted result for each observation to
* the actual outcome and provides a summary so that you know how effective the MLModel
functions on
* the test data. Evaluation generates a relevant performance metric, such as BinaryAUC, RegressionRMSE or
* MulticlassAvgFScore based on the corresponding MLModelType
: BINARY
,
* REGRESSION
or MULTICLASS
.
*
*
* CreateEvaluation
is an asynchronous operation. In response to CreateEvaluation
, Amazon
* Machine Learning (Amazon ML) immediately returns and sets the evaluation status to PENDING
. After
* the Evaluation
is created and ready for use, Amazon ML sets the status to COMPLETED
.
*
*
* You can use the GetEvaluation
operation to check progress of the evaluation during the creation
* operation.
*
*
* @param createEvaluationRequest
* @return A Java Future containing the result of the CreateEvaluation operation returned by the service.
* @sample AmazonMachineLearningAsync.CreateEvaluation
*/
java.util.concurrent.Future createEvaluationAsync(CreateEvaluationRequest createEvaluationRequest);
/**
*
* Creates a new Evaluation
of an MLModel
. An MLModel
is evaluated on a set
* of observations associated to a DataSource
. Like a DataSource
for an
* MLModel
, the DataSource
for an Evaluation
contains values for the
* Target Variable
. The Evaluation
compares the predicted result for each observation to
* the actual outcome and provides a summary so that you know how effective the MLModel
functions on
* the test data. Evaluation generates a relevant performance metric, such as BinaryAUC, RegressionRMSE or
* MulticlassAvgFScore based on the corresponding MLModelType
: BINARY
,
* REGRESSION
or MULTICLASS
.
*
*
* CreateEvaluation
is an asynchronous operation. In response to CreateEvaluation
, Amazon
* Machine Learning (Amazon ML) immediately returns and sets the evaluation status to PENDING
. After
* the Evaluation
is created and ready for use, Amazon ML sets the status to COMPLETED
.
*
*
* You can use the GetEvaluation
operation to check progress of the evaluation during the creation
* operation.
*
*
* @param createEvaluationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateEvaluation operation returned by the service.
* @sample AmazonMachineLearningAsyncHandler.CreateEvaluation
*/
java.util.concurrent.Future createEvaluationAsync(CreateEvaluationRequest createEvaluationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new MLModel
using the DataSource
and the recipe as information sources.
*
*
* An MLModel
is nearly immutable. Users can update only the MLModelName
and the
* ScoreThreshold
in an MLModel
without creating a new MLModel
.
*
*
* CreateMLModel
is an asynchronous operation. In response to CreateMLModel
, Amazon
* Machine Learning (Amazon ML) immediately returns and sets the MLModel
status to PENDING
* . After the MLModel
has been created and ready is for use, Amazon ML sets the status to
* COMPLETED
.
*
*
* You can use the GetMLModel
operation to check the progress of the MLModel
during the
* creation operation.
*
*
* CreateMLModel
requires a DataSource
with computed statistics, which can be created by
* setting ComputeStatistics
to true
in CreateDataSourceFromRDS
,
* CreateDataSourceFromS3
, or CreateDataSourceFromRedshift
operations.
*
*
* @param createMLModelRequest
* @return A Java Future containing the result of the CreateMLModel operation returned by the service.
* @sample AmazonMachineLearningAsync.CreateMLModel
*/
java.util.concurrent.Future createMLModelAsync(CreateMLModelRequest createMLModelRequest);
/**
*
* Creates a new MLModel
using the DataSource
and the recipe as information sources.
*
*
* An MLModel
is nearly immutable. Users can update only the MLModelName
and the
* ScoreThreshold
in an MLModel
without creating a new MLModel
.
*
*
* CreateMLModel
is an asynchronous operation. In response to CreateMLModel
, Amazon
* Machine Learning (Amazon ML) immediately returns and sets the MLModel
status to PENDING
* . After the MLModel
has been created and ready is for use, Amazon ML sets the status to
* COMPLETED
.
*
*
* You can use the GetMLModel
operation to check the progress of the MLModel
during the
* creation operation.
*
*
* CreateMLModel
requires a DataSource
with computed statistics, which can be created by
* setting ComputeStatistics
to true
in CreateDataSourceFromRDS
,
* CreateDataSourceFromS3
, or CreateDataSourceFromRedshift
operations.
*
*
* @param createMLModelRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateMLModel operation returned by the service.
* @sample AmazonMachineLearningAsyncHandler.CreateMLModel
*/
java.util.concurrent.Future createMLModelAsync(CreateMLModelRequest createMLModelRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a real-time endpoint for the MLModel
. The endpoint contains the URI of the
* MLModel
; that is, the location to send real-time prediction requests for the specified
* MLModel
.
*
*
* @param createRealtimeEndpointRequest
* @return A Java Future containing the result of the CreateRealtimeEndpoint operation returned by the service.
* @sample AmazonMachineLearningAsync.CreateRealtimeEndpoint
*/
java.util.concurrent.Future createRealtimeEndpointAsync(CreateRealtimeEndpointRequest createRealtimeEndpointRequest);
/**
*
* Creates a real-time endpoint for the MLModel
. The endpoint contains the URI of the
* MLModel
; that is, the location to send real-time prediction requests for the specified
* MLModel
.
*
*
* @param createRealtimeEndpointRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateRealtimeEndpoint operation returned by the service.
* @sample AmazonMachineLearningAsyncHandler.CreateRealtimeEndpoint
*/
java.util.concurrent.Future createRealtimeEndpointAsync(CreateRealtimeEndpointRequest createRealtimeEndpointRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Assigns the DELETED status to a BatchPrediction
, rendering it unusable.
*
*
* After using the DeleteBatchPrediction
operation, you can use the GetBatchPrediction operation
* to verify that the status of the BatchPrediction
changed to DELETED.
*
*
* Caution: The result of the DeleteBatchPrediction
operation is irreversible.
*
*
* @param deleteBatchPredictionRequest
* @return A Java Future containing the result of the DeleteBatchPrediction operation returned by the service.
* @sample AmazonMachineLearningAsync.DeleteBatchPrediction
*/
java.util.concurrent.Future deleteBatchPredictionAsync(DeleteBatchPredictionRequest deleteBatchPredictionRequest);
/**
*
* Assigns the DELETED status to a BatchPrediction
, rendering it unusable.
*
*
* After using the DeleteBatchPrediction
operation, you can use the GetBatchPrediction operation
* to verify that the status of the BatchPrediction
changed to DELETED.
*
*
* Caution: The result of the DeleteBatchPrediction
operation is irreversible.
*
*
* @param deleteBatchPredictionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteBatchPrediction operation returned by the service.
* @sample AmazonMachineLearningAsyncHandler.DeleteBatchPrediction
*/
java.util.concurrent.Future deleteBatchPredictionAsync(DeleteBatchPredictionRequest deleteBatchPredictionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Assigns the DELETED status to a DataSource
, rendering it unusable.
*
*
* After using the DeleteDataSource
operation, you can use the GetDataSource operation to verify
* that the status of the DataSource
changed to DELETED.
*
*
* Caution: The results of the DeleteDataSource
operation are irreversible.
*
*
* @param deleteDataSourceRequest
* @return A Java Future containing the result of the DeleteDataSource operation returned by the service.
* @sample AmazonMachineLearningAsync.DeleteDataSource
*/
java.util.concurrent.Future deleteDataSourceAsync(DeleteDataSourceRequest deleteDataSourceRequest);
/**
*
* Assigns the DELETED status to a DataSource
, rendering it unusable.
*
*
* After using the DeleteDataSource
operation, you can use the GetDataSource operation to verify
* that the status of the DataSource
changed to DELETED.
*
*
* Caution: The results of the DeleteDataSource
operation are irreversible.
*
*
* @param deleteDataSourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteDataSource operation returned by the service.
* @sample AmazonMachineLearningAsyncHandler.DeleteDataSource
*/
java.util.concurrent.Future deleteDataSourceAsync(DeleteDataSourceRequest deleteDataSourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Assigns the DELETED
status to an Evaluation
, rendering it unusable.
*
*
* After invoking the DeleteEvaluation
operation, you can use the GetEvaluation
operation
* to verify that the status of the Evaluation
changed to DELETED
.
*
*
* Caution: The results of the DeleteEvaluation
operation are irreversible.
*
*
* @param deleteEvaluationRequest
* @return A Java Future containing the result of the DeleteEvaluation operation returned by the service.
* @sample AmazonMachineLearningAsync.DeleteEvaluation
*/
java.util.concurrent.Future deleteEvaluationAsync(DeleteEvaluationRequest deleteEvaluationRequest);
/**
*
* Assigns the DELETED
status to an Evaluation
, rendering it unusable.
*
*
* After invoking the DeleteEvaluation
operation, you can use the GetEvaluation
operation
* to verify that the status of the Evaluation
changed to DELETED
.
*
*
* Caution: The results of the DeleteEvaluation
operation are irreversible.
*
*
* @param deleteEvaluationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteEvaluation operation returned by the service.
* @sample AmazonMachineLearningAsyncHandler.DeleteEvaluation
*/
java.util.concurrent.Future deleteEvaluationAsync(DeleteEvaluationRequest deleteEvaluationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Assigns the DELETED
status to an MLModel
, rendering it unusable.
*
*
* After using the DeleteMLModel
operation, you can use the GetMLModel
operation to verify
* that the status of the MLModel
changed to DELETED.
*
*
* Caution: The result of the DeleteMLModel
operation is irreversible.
*
*
* @param deleteMLModelRequest
* @return A Java Future containing the result of the DeleteMLModel operation returned by the service.
* @sample AmazonMachineLearningAsync.DeleteMLModel
*/
java.util.concurrent.Future deleteMLModelAsync(DeleteMLModelRequest deleteMLModelRequest);
/**
*
* Assigns the DELETED
status to an MLModel
, rendering it unusable.
*
*
* After using the DeleteMLModel
operation, you can use the GetMLModel
operation to verify
* that the status of the MLModel
changed to DELETED.
*
*
* Caution: The result of the DeleteMLModel
operation is irreversible.
*
*
* @param deleteMLModelRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteMLModel operation returned by the service.
* @sample AmazonMachineLearningAsyncHandler.DeleteMLModel
*/
java.util.concurrent.Future deleteMLModelAsync(DeleteMLModelRequest deleteMLModelRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a real time endpoint of an MLModel
.
*
*
* @param deleteRealtimeEndpointRequest
* @return A Java Future containing the result of the DeleteRealtimeEndpoint operation returned by the service.
* @sample AmazonMachineLearningAsync.DeleteRealtimeEndpoint
*/
java.util.concurrent.Future deleteRealtimeEndpointAsync(DeleteRealtimeEndpointRequest deleteRealtimeEndpointRequest);
/**
*
* Deletes a real time endpoint of an MLModel
.
*
*
* @param deleteRealtimeEndpointRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteRealtimeEndpoint operation returned by the service.
* @sample AmazonMachineLearningAsyncHandler.DeleteRealtimeEndpoint
*/
java.util.concurrent.Future deleteRealtimeEndpointAsync(DeleteRealtimeEndpointRequest deleteRealtimeEndpointRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified tags associated with an ML object. After this operation is complete, you can't recover
* deleted tags.
*
*
* If you specify a tag that doesn't exist, Amazon ML ignores it.
*
*
* @param deleteTagsRequest
* @return A Java Future containing the result of the DeleteTags operation returned by the service.
* @sample AmazonMachineLearningAsync.DeleteTags
*/
java.util.concurrent.Future deleteTagsAsync(DeleteTagsRequest deleteTagsRequest);
/**
*
* Deletes the specified tags associated with an ML object. After this operation is complete, you can't recover
* deleted tags.
*
*
* If you specify a tag that doesn't exist, Amazon ML ignores it.
*
*
* @param deleteTagsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteTags operation returned by the service.
* @sample AmazonMachineLearningAsyncHandler.DeleteTags
*/
java.util.concurrent.Future deleteTagsAsync(DeleteTagsRequest deleteTagsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of BatchPrediction
operations that match the search criteria in the request.
*
*
* @param describeBatchPredictionsRequest
* @return A Java Future containing the result of the DescribeBatchPredictions operation returned by the service.
* @sample AmazonMachineLearningAsync.DescribeBatchPredictions
*/
java.util.concurrent.Future describeBatchPredictionsAsync(DescribeBatchPredictionsRequest describeBatchPredictionsRequest);
/**
*
* Returns a list of BatchPrediction
operations that match the search criteria in the request.
*
*
* @param describeBatchPredictionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeBatchPredictions operation returned by the service.
* @sample AmazonMachineLearningAsyncHandler.DescribeBatchPredictions
*/
java.util.concurrent.Future describeBatchPredictionsAsync(DescribeBatchPredictionsRequest describeBatchPredictionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeBatchPredictions operation.
*
* @see #describeBatchPredictionsAsync(DescribeBatchPredictionsRequest)
*/
java.util.concurrent.Future describeBatchPredictionsAsync();
/**
* Simplified method form for invoking the DescribeBatchPredictions operation with an AsyncHandler.
*
* @see #describeBatchPredictionsAsync(DescribeBatchPredictionsRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeBatchPredictionsAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of DataSource
that match the search criteria in the request.
*
*
* @param describeDataSourcesRequest
* @return A Java Future containing the result of the DescribeDataSources operation returned by the service.
* @sample AmazonMachineLearningAsync.DescribeDataSources
*/
java.util.concurrent.Future describeDataSourcesAsync(DescribeDataSourcesRequest describeDataSourcesRequest);
/**
*
* Returns a list of DataSource
that match the search criteria in the request.
*
*
* @param describeDataSourcesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDataSources operation returned by the service.
* @sample AmazonMachineLearningAsyncHandler.DescribeDataSources
*/
java.util.concurrent.Future describeDataSourcesAsync(DescribeDataSourcesRequest describeDataSourcesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeDataSources operation.
*
* @see #describeDataSourcesAsync(DescribeDataSourcesRequest)
*/
java.util.concurrent.Future describeDataSourcesAsync();
/**
* Simplified method form for invoking the DescribeDataSources operation with an AsyncHandler.
*
* @see #describeDataSourcesAsync(DescribeDataSourcesRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeDataSourcesAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of DescribeEvaluations
that match the search criteria in the request.
*
*
* @param describeEvaluationsRequest
* @return A Java Future containing the result of the DescribeEvaluations operation returned by the service.
* @sample AmazonMachineLearningAsync.DescribeEvaluations
*/
java.util.concurrent.Future describeEvaluationsAsync(DescribeEvaluationsRequest describeEvaluationsRequest);
/**
*
* Returns a list of DescribeEvaluations
that match the search criteria in the request.
*
*
* @param describeEvaluationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeEvaluations operation returned by the service.
* @sample AmazonMachineLearningAsyncHandler.DescribeEvaluations
*/
java.util.concurrent.Future describeEvaluationsAsync(DescribeEvaluationsRequest describeEvaluationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeEvaluations operation.
*
* @see #describeEvaluationsAsync(DescribeEvaluationsRequest)
*/
java.util.concurrent.Future describeEvaluationsAsync();
/**
* Simplified method form for invoking the DescribeEvaluations operation with an AsyncHandler.
*
* @see #describeEvaluationsAsync(DescribeEvaluationsRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeEvaluationsAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of MLModel
that match the search criteria in the request.
*
*
* @param describeMLModelsRequest
* @return A Java Future containing the result of the DescribeMLModels operation returned by the service.
* @sample AmazonMachineLearningAsync.DescribeMLModels
*/
java.util.concurrent.Future describeMLModelsAsync(DescribeMLModelsRequest describeMLModelsRequest);
/**
*
* Returns a list of MLModel
that match the search criteria in the request.
*
*
* @param describeMLModelsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeMLModels operation returned by the service.
* @sample AmazonMachineLearningAsyncHandler.DescribeMLModels
*/
java.util.concurrent.Future describeMLModelsAsync(DescribeMLModelsRequest describeMLModelsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeMLModels operation.
*
* @see #describeMLModelsAsync(DescribeMLModelsRequest)
*/
java.util.concurrent.Future describeMLModelsAsync();
/**
* Simplified method form for invoking the DescribeMLModels operation with an AsyncHandler.
*
* @see #describeMLModelsAsync(DescribeMLModelsRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeMLModelsAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes one or more of the tags for your Amazon ML object.
*
*
* @param describeTagsRequest
* @return A Java Future containing the result of the DescribeTags operation returned by the service.
* @sample AmazonMachineLearningAsync.DescribeTags
*/
java.util.concurrent.Future describeTagsAsync(DescribeTagsRequest describeTagsRequest);
/**
*
* Describes one or more of the tags for your Amazon ML object.
*
*
* @param describeTagsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeTags operation returned by the service.
* @sample AmazonMachineLearningAsyncHandler.DescribeTags
*/
java.util.concurrent.Future describeTagsAsync(DescribeTagsRequest describeTagsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a BatchPrediction
that includes detailed metadata, status, and data file information for a
* Batch Prediction
request.
*
*
* @param getBatchPredictionRequest
* @return A Java Future containing the result of the GetBatchPrediction operation returned by the service.
* @sample AmazonMachineLearningAsync.GetBatchPrediction
*/
java.util.concurrent.Future getBatchPredictionAsync(GetBatchPredictionRequest getBatchPredictionRequest);
/**
*
* Returns a BatchPrediction
that includes detailed metadata, status, and data file information for a
* Batch Prediction
request.
*
*
* @param getBatchPredictionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetBatchPrediction operation returned by the service.
* @sample AmazonMachineLearningAsyncHandler.GetBatchPrediction
*/
java.util.concurrent.Future getBatchPredictionAsync(GetBatchPredictionRequest getBatchPredictionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a DataSource
that includes metadata and data file information, as well as the current status
* of the DataSource
.
*
*
* GetDataSource
provides results in normal or verbose format. The verbose format adds the schema
* description and the list of files pointed to by the DataSource to the normal format.
*
*
* @param getDataSourceRequest
* @return A Java Future containing the result of the GetDataSource operation returned by the service.
* @sample AmazonMachineLearningAsync.GetDataSource
*/
java.util.concurrent.Future getDataSourceAsync(GetDataSourceRequest getDataSourceRequest);
/**
*
* Returns a DataSource
that includes metadata and data file information, as well as the current status
* of the DataSource
.
*
*
* GetDataSource
provides results in normal or verbose format. The verbose format adds the schema
* description and the list of files pointed to by the DataSource to the normal format.
*
*
* @param getDataSourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetDataSource operation returned by the service.
* @sample AmazonMachineLearningAsyncHandler.GetDataSource
*/
java.util.concurrent.Future getDataSourceAsync(GetDataSourceRequest getDataSourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns an Evaluation
that includes metadata as well as the current status of the
* Evaluation
.
*
*
* @param getEvaluationRequest
* @return A Java Future containing the result of the GetEvaluation operation returned by the service.
* @sample AmazonMachineLearningAsync.GetEvaluation
*/
java.util.concurrent.Future getEvaluationAsync(GetEvaluationRequest getEvaluationRequest);
/**
*
* Returns an Evaluation
that includes metadata as well as the current status of the
* Evaluation
.
*
*
* @param getEvaluationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetEvaluation operation returned by the service.
* @sample AmazonMachineLearningAsyncHandler.GetEvaluation
*/
java.util.concurrent.Future getEvaluationAsync(GetEvaluationRequest getEvaluationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns an MLModel
that includes detailed metadata, data source information, and the current status
* of the MLModel
.
*
*
* GetMLModel
provides results in normal or verbose format.
*
*
* @param getMLModelRequest
* @return A Java Future containing the result of the GetMLModel operation returned by the service.
* @sample AmazonMachineLearningAsync.GetMLModel
*/
java.util.concurrent.Future getMLModelAsync(GetMLModelRequest getMLModelRequest);
/**
*
* Returns an MLModel
that includes detailed metadata, data source information, and the current status
* of the MLModel
.
*
*
* GetMLModel
provides results in normal or verbose format.
*
*
* @param getMLModelRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetMLModel operation returned by the service.
* @sample AmazonMachineLearningAsyncHandler.GetMLModel
*/
java.util.concurrent.Future getMLModelAsync(GetMLModelRequest getMLModelRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Generates a prediction for the observation using the specified ML Model
.
*
*
* Note: Not all response parameters will be populated. Whether a response parameter is populated depends on
* the type of model requested.
*
*
* @param predictRequest
* @return A Java Future containing the result of the Predict operation returned by the service.
* @sample AmazonMachineLearningAsync.Predict
*/
java.util.concurrent.Future predictAsync(PredictRequest predictRequest);
/**
*
* Generates a prediction for the observation using the specified ML Model
.
*
*
* Note: Not all response parameters will be populated. Whether a response parameter is populated depends on
* the type of model requested.
*
*
* @param predictRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the Predict operation returned by the service.
* @sample AmazonMachineLearningAsyncHandler.Predict
*/
java.util.concurrent.Future predictAsync(PredictRequest predictRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the BatchPredictionName
of a BatchPrediction
.
*
*
* You can use the GetBatchPrediction
operation to view the contents of the updated data element.
*
*
* @param updateBatchPredictionRequest
* @return A Java Future containing the result of the UpdateBatchPrediction operation returned by the service.
* @sample AmazonMachineLearningAsync.UpdateBatchPrediction
*/
java.util.concurrent.Future updateBatchPredictionAsync(UpdateBatchPredictionRequest updateBatchPredictionRequest);
/**
*
* Updates the BatchPredictionName
of a BatchPrediction
.
*
*
* You can use the GetBatchPrediction
operation to view the contents of the updated data element.
*
*
* @param updateBatchPredictionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateBatchPrediction operation returned by the service.
* @sample AmazonMachineLearningAsyncHandler.UpdateBatchPrediction
*/
java.util.concurrent.Future updateBatchPredictionAsync(UpdateBatchPredictionRequest updateBatchPredictionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the DataSourceName
of a DataSource
.
*
*
* You can use the GetDataSource
operation to view the contents of the updated data element.
*
*
* @param updateDataSourceRequest
* @return A Java Future containing the result of the UpdateDataSource operation returned by the service.
* @sample AmazonMachineLearningAsync.UpdateDataSource
*/
java.util.concurrent.Future updateDataSourceAsync(UpdateDataSourceRequest updateDataSourceRequest);
/**
*
* Updates the DataSourceName
of a DataSource
.
*
*
* You can use the GetDataSource
operation to view the contents of the updated data element.
*
*
* @param updateDataSourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateDataSource operation returned by the service.
* @sample AmazonMachineLearningAsyncHandler.UpdateDataSource
*/
java.util.concurrent.Future updateDataSourceAsync(UpdateDataSourceRequest updateDataSourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the EvaluationName
of an Evaluation
.
*
*
* You can use the GetEvaluation
operation to view the contents of the updated data element.
*
*
* @param updateEvaluationRequest
* @return A Java Future containing the result of the UpdateEvaluation operation returned by the service.
* @sample AmazonMachineLearningAsync.UpdateEvaluation
*/
java.util.concurrent.Future updateEvaluationAsync(UpdateEvaluationRequest updateEvaluationRequest);
/**
*
* Updates the EvaluationName
of an Evaluation
.
*
*
* You can use the GetEvaluation
operation to view the contents of the updated data element.
*
*
* @param updateEvaluationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateEvaluation operation returned by the service.
* @sample AmazonMachineLearningAsyncHandler.UpdateEvaluation
*/
java.util.concurrent.Future updateEvaluationAsync(UpdateEvaluationRequest updateEvaluationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the MLModelName
and the ScoreThreshold
of an MLModel
.
*
*
* You can use the GetMLModel
operation to view the contents of the updated data element.
*
*
* @param updateMLModelRequest
* @return A Java Future containing the result of the UpdateMLModel operation returned by the service.
* @sample AmazonMachineLearningAsync.UpdateMLModel
*/
java.util.concurrent.Future updateMLModelAsync(UpdateMLModelRequest updateMLModelRequest);
/**
*
* Updates the MLModelName
and the ScoreThreshold
of an MLModel
.
*
*
* You can use the GetMLModel
operation to view the contents of the updated data element.
*
*
* @param updateMLModelRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateMLModel operation returned by the service.
* @sample AmazonMachineLearningAsyncHandler.UpdateMLModel
*/
java.util.concurrent.Future updateMLModelAsync(UpdateMLModelRequest updateMLModelRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}