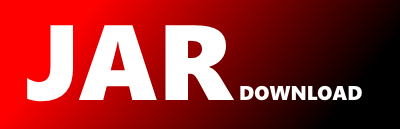
com.amazonaws.services.macie.AmazonMacie Maven / Gradle / Ivy
Show all versions of aws-java-sdk-macie Show documentation
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.macie;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.macie.model.*;
/**
* Interface for accessing Amazon Macie.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.macie.AbstractAmazonMacie} instead.
*
*
* Amazon Macie
*
* Amazon Macie is a security service that uses machine learning to automatically discover, classify, and protect
* sensitive data in AWS. Macie recognizes sensitive data such as personally identifiable information (PII) or
* intellectual property, and provides you with dashboards and alerts that give visibility into how this data is being
* accessed or moved. For more information, see the Macie User Guide.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonMacie {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "macie";
/**
*
* Associates a specified AWS account with Amazon Macie as a member account.
*
*
* @param associateMemberAccountRequest
* @return Result of the AssociateMemberAccount operation returned by the service.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current AWS account limits.
* The error code describes the limit exceeded.
* @throws InternalException
* Internal server error.
* @sample AmazonMacie.AssociateMemberAccount
* @see AWS
* API Documentation
*/
AssociateMemberAccountResult associateMemberAccount(AssociateMemberAccountRequest associateMemberAccountRequest);
/**
*
* Associates specified S3 resources with Amazon Macie for monitoring and data classification. If memberAccountId
* isn't specified, the action associates specified S3 resources with Macie for the current master account. If
* memberAccountId is specified, the action associates specified S3 resources with Macie for the specified member
* account.
*
*
* @param associateS3ResourcesRequest
* @return Result of the AssociateS3Resources operation returned by the service.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws AccessDeniedException
* You do not have required permissions to access the requested resource.
* @throws LimitExceededException
* The request was rejected because it attempted to create resources beyond the current AWS account limits.
* The error code describes the limit exceeded.
* @throws InternalException
* Internal server error.
* @sample AmazonMacie.AssociateS3Resources
* @see AWS API
* Documentation
*/
AssociateS3ResourcesResult associateS3Resources(AssociateS3ResourcesRequest associateS3ResourcesRequest);
/**
*
* Removes the specified member account from Amazon Macie.
*
*
* @param disassociateMemberAccountRequest
* @return Result of the DisassociateMemberAccount operation returned by the service.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws InternalException
* Internal server error.
* @sample AmazonMacie.DisassociateMemberAccount
* @see AWS API Documentation
*/
DisassociateMemberAccountResult disassociateMemberAccount(DisassociateMemberAccountRequest disassociateMemberAccountRequest);
/**
*
* Removes specified S3 resources from being monitored by Amazon Macie. If memberAccountId isn't specified, the
* action removes specified S3 resources from Macie for the current master account. If memberAccountId is specified,
* the action removes specified S3 resources from Macie for the specified member account.
*
*
* @param disassociateS3ResourcesRequest
* @return Result of the DisassociateS3Resources operation returned by the service.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws AccessDeniedException
* You do not have required permissions to access the requested resource.
* @throws InternalException
* Internal server error.
* @sample AmazonMacie.DisassociateS3Resources
* @see AWS
* API Documentation
*/
DisassociateS3ResourcesResult disassociateS3Resources(DisassociateS3ResourcesRequest disassociateS3ResourcesRequest);
/**
*
* Lists all Amazon Macie member accounts for the current Amazon Macie master account.
*
*
* @param listMemberAccountsRequest
* @return Result of the ListMemberAccounts operation returned by the service.
* @throws InternalException
* Internal server error.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @sample AmazonMacie.ListMemberAccounts
* @see AWS API
* Documentation
*/
ListMemberAccountsResult listMemberAccounts(ListMemberAccountsRequest listMemberAccountsRequest);
/**
*
* Lists all the S3 resources associated with Amazon Macie. If memberAccountId isn't specified, the action lists the
* S3 resources associated with Amazon Macie for the current master account. If memberAccountId is specified, the
* action lists the S3 resources associated with Amazon Macie for the specified member account.
*
*
* @param listS3ResourcesRequest
* @return Result of the ListS3Resources operation returned by the service.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws AccessDeniedException
* You do not have required permissions to access the requested resource.
* @throws InternalException
* Internal server error.
* @sample AmazonMacie.ListS3Resources
* @see AWS API
* Documentation
*/
ListS3ResourcesResult listS3Resources(ListS3ResourcesRequest listS3ResourcesRequest);
/**
*
* Updates the classification types for the specified S3 resources. If memberAccountId isn't specified, the action
* updates the classification types of the S3 resources associated with Amazon Macie for the current master account.
* If memberAccountId is specified, the action updates the classification types of the S3 resources associated with
* Amazon Macie for the specified member account.
*
*
* @param updateS3ResourcesRequest
* @return Result of the UpdateS3Resources operation returned by the service.
* @throws InvalidInputException
* The request was rejected because an invalid or out-of-range value was supplied for an input parameter.
* @throws AccessDeniedException
* You do not have required permissions to access the requested resource.
* @throws InternalException
* Internal server error.
* @sample AmazonMacie.UpdateS3Resources
* @see AWS API
* Documentation
*/
UpdateS3ResourcesResult updateS3Resources(UpdateS3ResourcesRequest updateS3ResourcesRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}