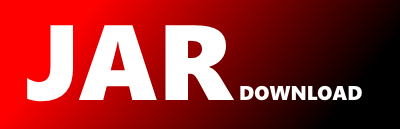
com.amazonaws.services.macie2.AmazonMacie2Async Maven / Gradle / Ivy
Show all versions of aws-java-sdk-macie2 Show documentation
/*
* Copyright 2018-2023 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.macie2;
import javax.annotation.Generated;
import com.amazonaws.services.macie2.model.*;
/**
* Interface for accessing Amazon Macie 2 asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.macie2.AbstractAmazonMacie2Async} instead.
*
*
*
* Amazon Macie
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonMacie2Async extends AmazonMacie2 {
/**
*
* Accepts an Amazon Macie membership invitation that was received from a specific account.
*
*
* @param acceptInvitationRequest
* @return A Java Future containing the result of the AcceptInvitation operation returned by the service.
* @sample AmazonMacie2Async.AcceptInvitation
* @see AWS API
* Documentation
*/
java.util.concurrent.Future acceptInvitationAsync(AcceptInvitationRequest acceptInvitationRequest);
/**
*
* Accepts an Amazon Macie membership invitation that was received from a specific account.
*
*
* @param acceptInvitationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AcceptInvitation operation returned by the service.
* @sample AmazonMacie2AsyncHandler.AcceptInvitation
* @see AWS API
* Documentation
*/
java.util.concurrent.Future acceptInvitationAsync(AcceptInvitationRequest acceptInvitationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves information about one or more custom data identifiers.
*
*
* @param batchGetCustomDataIdentifiersRequest
* @return A Java Future containing the result of the BatchGetCustomDataIdentifiers operation returned by the
* service.
* @sample AmazonMacie2Async.BatchGetCustomDataIdentifiers
* @see AWS API Documentation
*/
java.util.concurrent.Future batchGetCustomDataIdentifiersAsync(
BatchGetCustomDataIdentifiersRequest batchGetCustomDataIdentifiersRequest);
/**
*
* Retrieves information about one or more custom data identifiers.
*
*
* @param batchGetCustomDataIdentifiersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchGetCustomDataIdentifiers operation returned by the
* service.
* @sample AmazonMacie2AsyncHandler.BatchGetCustomDataIdentifiers
* @see AWS API Documentation
*/
java.util.concurrent.Future batchGetCustomDataIdentifiersAsync(
BatchGetCustomDataIdentifiersRequest batchGetCustomDataIdentifiersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates and defines the settings for an allow list.
*
*
* @param createAllowListRequest
* @return A Java Future containing the result of the CreateAllowList operation returned by the service.
* @sample AmazonMacie2Async.CreateAllowList
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createAllowListAsync(CreateAllowListRequest createAllowListRequest);
/**
*
* Creates and defines the settings for an allow list.
*
*
* @param createAllowListRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateAllowList operation returned by the service.
* @sample AmazonMacie2AsyncHandler.CreateAllowList
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createAllowListAsync(CreateAllowListRequest createAllowListRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates and defines the settings for a classification job.
*
*
* @param createClassificationJobRequest
* @return A Java Future containing the result of the CreateClassificationJob operation returned by the service.
* @sample AmazonMacie2Async.CreateClassificationJob
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createClassificationJobAsync(CreateClassificationJobRequest createClassificationJobRequest);
/**
*
* Creates and defines the settings for a classification job.
*
*
* @param createClassificationJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateClassificationJob operation returned by the service.
* @sample AmazonMacie2AsyncHandler.CreateClassificationJob
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createClassificationJobAsync(CreateClassificationJobRequest createClassificationJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates and defines the criteria and other settings for a custom data identifier.
*
*
* @param createCustomDataIdentifierRequest
* @return A Java Future containing the result of the CreateCustomDataIdentifier operation returned by the service.
* @sample AmazonMacie2Async.CreateCustomDataIdentifier
* @see AWS API Documentation
*/
java.util.concurrent.Future createCustomDataIdentifierAsync(
CreateCustomDataIdentifierRequest createCustomDataIdentifierRequest);
/**
*
* Creates and defines the criteria and other settings for a custom data identifier.
*
*
* @param createCustomDataIdentifierRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateCustomDataIdentifier operation returned by the service.
* @sample AmazonMacie2AsyncHandler.CreateCustomDataIdentifier
* @see AWS API Documentation
*/
java.util.concurrent.Future createCustomDataIdentifierAsync(
CreateCustomDataIdentifierRequest createCustomDataIdentifierRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates and defines the criteria and other settings for a findings filter.
*
*
* @param createFindingsFilterRequest
* @return A Java Future containing the result of the CreateFindingsFilter operation returned by the service.
* @sample AmazonMacie2Async.CreateFindingsFilter
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createFindingsFilterAsync(CreateFindingsFilterRequest createFindingsFilterRequest);
/**
*
* Creates and defines the criteria and other settings for a findings filter.
*
*
* @param createFindingsFilterRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateFindingsFilter operation returned by the service.
* @sample AmazonMacie2AsyncHandler.CreateFindingsFilter
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createFindingsFilterAsync(CreateFindingsFilterRequest createFindingsFilterRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Sends an Amazon Macie membership invitation to one or more accounts.
*
*
* @param createInvitationsRequest
* @return A Java Future containing the result of the CreateInvitations operation returned by the service.
* @sample AmazonMacie2Async.CreateInvitations
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createInvitationsAsync(CreateInvitationsRequest createInvitationsRequest);
/**
*
* Sends an Amazon Macie membership invitation to one or more accounts.
*
*
* @param createInvitationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateInvitations operation returned by the service.
* @sample AmazonMacie2AsyncHandler.CreateInvitations
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createInvitationsAsync(CreateInvitationsRequest createInvitationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Associates an account with an Amazon Macie administrator account.
*
*
* @param createMemberRequest
* @return A Java Future containing the result of the CreateMember operation returned by the service.
* @sample AmazonMacie2Async.CreateMember
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createMemberAsync(CreateMemberRequest createMemberRequest);
/**
*
* Associates an account with an Amazon Macie administrator account.
*
*
* @param createMemberRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateMember operation returned by the service.
* @sample AmazonMacie2AsyncHandler.CreateMember
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createMemberAsync(CreateMemberRequest createMemberRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates sample findings.
*
*
* @param createSampleFindingsRequest
* @return A Java Future containing the result of the CreateSampleFindings operation returned by the service.
* @sample AmazonMacie2Async.CreateSampleFindings
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createSampleFindingsAsync(CreateSampleFindingsRequest createSampleFindingsRequest);
/**
*
* Creates sample findings.
*
*
* @param createSampleFindingsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateSampleFindings operation returned by the service.
* @sample AmazonMacie2AsyncHandler.CreateSampleFindings
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createSampleFindingsAsync(CreateSampleFindingsRequest createSampleFindingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Declines Amazon Macie membership invitations that were received from specific accounts.
*
*
* @param declineInvitationsRequest
* @return A Java Future containing the result of the DeclineInvitations operation returned by the service.
* @sample AmazonMacie2Async.DeclineInvitations
* @see AWS API
* Documentation
*/
java.util.concurrent.Future declineInvitationsAsync(DeclineInvitationsRequest declineInvitationsRequest);
/**
*
* Declines Amazon Macie membership invitations that were received from specific accounts.
*
*
* @param declineInvitationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeclineInvitations operation returned by the service.
* @sample AmazonMacie2AsyncHandler.DeclineInvitations
* @see AWS API
* Documentation
*/
java.util.concurrent.Future declineInvitationsAsync(DeclineInvitationsRequest declineInvitationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an allow list.
*
*
* @param deleteAllowListRequest
* @return A Java Future containing the result of the DeleteAllowList operation returned by the service.
* @sample AmazonMacie2Async.DeleteAllowList
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteAllowListAsync(DeleteAllowListRequest deleteAllowListRequest);
/**
*
* Deletes an allow list.
*
*
* @param deleteAllowListRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteAllowList operation returned by the service.
* @sample AmazonMacie2AsyncHandler.DeleteAllowList
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteAllowListAsync(DeleteAllowListRequest deleteAllowListRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Soft deletes a custom data identifier.
*
*
* @param deleteCustomDataIdentifierRequest
* @return A Java Future containing the result of the DeleteCustomDataIdentifier operation returned by the service.
* @sample AmazonMacie2Async.DeleteCustomDataIdentifier
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteCustomDataIdentifierAsync(
DeleteCustomDataIdentifierRequest deleteCustomDataIdentifierRequest);
/**
*
* Soft deletes a custom data identifier.
*
*
* @param deleteCustomDataIdentifierRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteCustomDataIdentifier operation returned by the service.
* @sample AmazonMacie2AsyncHandler.DeleteCustomDataIdentifier
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteCustomDataIdentifierAsync(
DeleteCustomDataIdentifierRequest deleteCustomDataIdentifierRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a findings filter.
*
*
* @param deleteFindingsFilterRequest
* @return A Java Future containing the result of the DeleteFindingsFilter operation returned by the service.
* @sample AmazonMacie2Async.DeleteFindingsFilter
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteFindingsFilterAsync(DeleteFindingsFilterRequest deleteFindingsFilterRequest);
/**
*
* Deletes a findings filter.
*
*
* @param deleteFindingsFilterRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteFindingsFilter operation returned by the service.
* @sample AmazonMacie2AsyncHandler.DeleteFindingsFilter
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteFindingsFilterAsync(DeleteFindingsFilterRequest deleteFindingsFilterRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes Amazon Macie membership invitations that were received from specific accounts.
*
*
* @param deleteInvitationsRequest
* @return A Java Future containing the result of the DeleteInvitations operation returned by the service.
* @sample AmazonMacie2Async.DeleteInvitations
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteInvitationsAsync(DeleteInvitationsRequest deleteInvitationsRequest);
/**
*
* Deletes Amazon Macie membership invitations that were received from specific accounts.
*
*
* @param deleteInvitationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteInvitations operation returned by the service.
* @sample AmazonMacie2AsyncHandler.DeleteInvitations
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteInvitationsAsync(DeleteInvitationsRequest deleteInvitationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the association between an Amazon Macie administrator account and an account.
*
*
* @param deleteMemberRequest
* @return A Java Future containing the result of the DeleteMember operation returned by the service.
* @sample AmazonMacie2Async.DeleteMember
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteMemberAsync(DeleteMemberRequest deleteMemberRequest);
/**
*
* Deletes the association between an Amazon Macie administrator account and an account.
*
*
* @param deleteMemberRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteMember operation returned by the service.
* @sample AmazonMacie2AsyncHandler.DeleteMember
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteMemberAsync(DeleteMemberRequest deleteMemberRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves (queries) statistical data and other information about one or more S3 buckets that Amazon Macie
* monitors and analyzes for an account.
*
*
* @param describeBucketsRequest
* @return A Java Future containing the result of the DescribeBuckets operation returned by the service.
* @sample AmazonMacie2Async.DescribeBuckets
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeBucketsAsync(DescribeBucketsRequest describeBucketsRequest);
/**
*
* Retrieves (queries) statistical data and other information about one or more S3 buckets that Amazon Macie
* monitors and analyzes for an account.
*
*
* @param describeBucketsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeBuckets operation returned by the service.
* @sample AmazonMacie2AsyncHandler.DescribeBuckets
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeBucketsAsync(DescribeBucketsRequest describeBucketsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the status and settings for a classification job.
*
*
* @param describeClassificationJobRequest
* @return A Java Future containing the result of the DescribeClassificationJob operation returned by the service.
* @sample AmazonMacie2Async.DescribeClassificationJob
* @see AWS API Documentation
*/
java.util.concurrent.Future describeClassificationJobAsync(
DescribeClassificationJobRequest describeClassificationJobRequest);
/**
*
* Retrieves the status and settings for a classification job.
*
*
* @param describeClassificationJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeClassificationJob operation returned by the service.
* @sample AmazonMacie2AsyncHandler.DescribeClassificationJob
* @see AWS API Documentation
*/
java.util.concurrent.Future describeClassificationJobAsync(
DescribeClassificationJobRequest describeClassificationJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the Amazon Macie configuration settings for an organization in Organizations.
*
*
* @param describeOrganizationConfigurationRequest
* @return A Java Future containing the result of the DescribeOrganizationConfiguration operation returned by the
* service.
* @sample AmazonMacie2Async.DescribeOrganizationConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future describeOrganizationConfigurationAsync(
DescribeOrganizationConfigurationRequest describeOrganizationConfigurationRequest);
/**
*
* Retrieves the Amazon Macie configuration settings for an organization in Organizations.
*
*
* @param describeOrganizationConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeOrganizationConfiguration operation returned by the
* service.
* @sample AmazonMacie2AsyncHandler.DescribeOrganizationConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future describeOrganizationConfigurationAsync(
DescribeOrganizationConfigurationRequest describeOrganizationConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disables Amazon Macie and deletes all settings and resources for a Macie account.
*
*
* @param disableMacieRequest
* @return A Java Future containing the result of the DisableMacie operation returned by the service.
* @sample AmazonMacie2Async.DisableMacie
* @see AWS API
* Documentation
*/
java.util.concurrent.Future disableMacieAsync(DisableMacieRequest disableMacieRequest);
/**
*
* Disables Amazon Macie and deletes all settings and resources for a Macie account.
*
*
* @param disableMacieRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisableMacie operation returned by the service.
* @sample AmazonMacie2AsyncHandler.DisableMacie
* @see AWS API
* Documentation
*/
java.util.concurrent.Future disableMacieAsync(DisableMacieRequest disableMacieRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disables an account as the delegated Amazon Macie administrator account for an organization in Organizations.
*
*
* @param disableOrganizationAdminAccountRequest
* @return A Java Future containing the result of the DisableOrganizationAdminAccount operation returned by the
* service.
* @sample AmazonMacie2Async.DisableOrganizationAdminAccount
* @see AWS API Documentation
*/
java.util.concurrent.Future disableOrganizationAdminAccountAsync(
DisableOrganizationAdminAccountRequest disableOrganizationAdminAccountRequest);
/**
*
* Disables an account as the delegated Amazon Macie administrator account for an organization in Organizations.
*
*
* @param disableOrganizationAdminAccountRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisableOrganizationAdminAccount operation returned by the
* service.
* @sample AmazonMacie2AsyncHandler.DisableOrganizationAdminAccount
* @see AWS API Documentation
*/
java.util.concurrent.Future disableOrganizationAdminAccountAsync(
DisableOrganizationAdminAccountRequest disableOrganizationAdminAccountRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disassociates a member account from its Amazon Macie administrator account.
*
*
* @param disassociateFromAdministratorAccountRequest
* @return A Java Future containing the result of the DisassociateFromAdministratorAccount operation returned by the
* service.
* @sample AmazonMacie2Async.DisassociateFromAdministratorAccount
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateFromAdministratorAccountAsync(
DisassociateFromAdministratorAccountRequest disassociateFromAdministratorAccountRequest);
/**
*
* Disassociates a member account from its Amazon Macie administrator account.
*
*
* @param disassociateFromAdministratorAccountRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisassociateFromAdministratorAccount operation returned by the
* service.
* @sample AmazonMacie2AsyncHandler.DisassociateFromAdministratorAccount
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateFromAdministratorAccountAsync(
DisassociateFromAdministratorAccountRequest disassociateFromAdministratorAccountRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* (Deprecated) Disassociates a member account from its Amazon Macie administrator account. This operation has been
* replaced by the DisassociateFromAdministratorAccount
* operation.
*
*
* @param disassociateFromMasterAccountRequest
* @return A Java Future containing the result of the DisassociateFromMasterAccount operation returned by the
* service.
* @sample AmazonMacie2Async.DisassociateFromMasterAccount
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateFromMasterAccountAsync(
DisassociateFromMasterAccountRequest disassociateFromMasterAccountRequest);
/**
*
* (Deprecated) Disassociates a member account from its Amazon Macie administrator account. This operation has been
* replaced by the DisassociateFromAdministratorAccount
* operation.
*
*
* @param disassociateFromMasterAccountRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisassociateFromMasterAccount operation returned by the
* service.
* @sample AmazonMacie2AsyncHandler.DisassociateFromMasterAccount
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateFromMasterAccountAsync(
DisassociateFromMasterAccountRequest disassociateFromMasterAccountRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disassociates an Amazon Macie administrator account from a member account.
*
*
* @param disassociateMemberRequest
* @return A Java Future containing the result of the DisassociateMember operation returned by the service.
* @sample AmazonMacie2Async.DisassociateMember
* @see AWS API
* Documentation
*/
java.util.concurrent.Future disassociateMemberAsync(DisassociateMemberRequest disassociateMemberRequest);
/**
*
* Disassociates an Amazon Macie administrator account from a member account.
*
*
* @param disassociateMemberRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisassociateMember operation returned by the service.
* @sample AmazonMacie2AsyncHandler.DisassociateMember
* @see AWS API
* Documentation
*/
java.util.concurrent.Future disassociateMemberAsync(DisassociateMemberRequest disassociateMemberRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Enables Amazon Macie and specifies the configuration settings for a Macie account.
*
*
* @param enableMacieRequest
* @return A Java Future containing the result of the EnableMacie operation returned by the service.
* @sample AmazonMacie2Async.EnableMacie
* @see AWS API
* Documentation
*/
java.util.concurrent.Future enableMacieAsync(EnableMacieRequest enableMacieRequest);
/**
*
* Enables Amazon Macie and specifies the configuration settings for a Macie account.
*
*
* @param enableMacieRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the EnableMacie operation returned by the service.
* @sample AmazonMacie2AsyncHandler.EnableMacie
* @see AWS API
* Documentation
*/
java.util.concurrent.Future enableMacieAsync(EnableMacieRequest enableMacieRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Designates an account as the delegated Amazon Macie administrator account for an organization in Organizations.
*
*
* @param enableOrganizationAdminAccountRequest
* @return A Java Future containing the result of the EnableOrganizationAdminAccount operation returned by the
* service.
* @sample AmazonMacie2Async.EnableOrganizationAdminAccount
* @see AWS API Documentation
*/
java.util.concurrent.Future enableOrganizationAdminAccountAsync(
EnableOrganizationAdminAccountRequest enableOrganizationAdminAccountRequest);
/**
*
* Designates an account as the delegated Amazon Macie administrator account for an organization in Organizations.
*
*
* @param enableOrganizationAdminAccountRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the EnableOrganizationAdminAccount operation returned by the
* service.
* @sample AmazonMacie2AsyncHandler.EnableOrganizationAdminAccount
* @see AWS API Documentation
*/
java.util.concurrent.Future enableOrganizationAdminAccountAsync(
EnableOrganizationAdminAccountRequest enableOrganizationAdminAccountRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves information about the Amazon Macie administrator account for an account.
*
*
* @param getAdministratorAccountRequest
* @return A Java Future containing the result of the GetAdministratorAccount operation returned by the service.
* @sample AmazonMacie2Async.GetAdministratorAccount
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getAdministratorAccountAsync(GetAdministratorAccountRequest getAdministratorAccountRequest);
/**
*
* Retrieves information about the Amazon Macie administrator account for an account.
*
*
* @param getAdministratorAccountRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetAdministratorAccount operation returned by the service.
* @sample AmazonMacie2AsyncHandler.GetAdministratorAccount
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getAdministratorAccountAsync(GetAdministratorAccountRequest getAdministratorAccountRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the settings and status of an allow list.
*
*
* @param getAllowListRequest
* @return A Java Future containing the result of the GetAllowList operation returned by the service.
* @sample AmazonMacie2Async.GetAllowList
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getAllowListAsync(GetAllowListRequest getAllowListRequest);
/**
*
* Retrieves the settings and status of an allow list.
*
*
* @param getAllowListRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetAllowList operation returned by the service.
* @sample AmazonMacie2AsyncHandler.GetAllowList
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getAllowListAsync(GetAllowListRequest getAllowListRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the configuration settings and status of automated sensitive data discovery for an account.
*
*
* @param getAutomatedDiscoveryConfigurationRequest
* @return A Java Future containing the result of the GetAutomatedDiscoveryConfiguration operation returned by the
* service.
* @sample AmazonMacie2Async.GetAutomatedDiscoveryConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future getAutomatedDiscoveryConfigurationAsync(
GetAutomatedDiscoveryConfigurationRequest getAutomatedDiscoveryConfigurationRequest);
/**
*
* Retrieves the configuration settings and status of automated sensitive data discovery for an account.
*
*
* @param getAutomatedDiscoveryConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetAutomatedDiscoveryConfiguration operation returned by the
* service.
* @sample AmazonMacie2AsyncHandler.GetAutomatedDiscoveryConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future getAutomatedDiscoveryConfigurationAsync(
GetAutomatedDiscoveryConfigurationRequest getAutomatedDiscoveryConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves (queries) aggregated statistical data about all the S3 buckets that Amazon Macie monitors and analyzes
* for an account.
*
*
* @param getBucketStatisticsRequest
* @return A Java Future containing the result of the GetBucketStatistics operation returned by the service.
* @sample AmazonMacie2Async.GetBucketStatistics
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getBucketStatisticsAsync(GetBucketStatisticsRequest getBucketStatisticsRequest);
/**
*
* Retrieves (queries) aggregated statistical data about all the S3 buckets that Amazon Macie monitors and analyzes
* for an account.
*
*
* @param getBucketStatisticsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetBucketStatistics operation returned by the service.
* @sample AmazonMacie2AsyncHandler.GetBucketStatistics
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getBucketStatisticsAsync(GetBucketStatisticsRequest getBucketStatisticsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the configuration settings for storing data classification results.
*
*
* @param getClassificationExportConfigurationRequest
* @return A Java Future containing the result of the GetClassificationExportConfiguration operation returned by the
* service.
* @sample AmazonMacie2Async.GetClassificationExportConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future getClassificationExportConfigurationAsync(
GetClassificationExportConfigurationRequest getClassificationExportConfigurationRequest);
/**
*
* Retrieves the configuration settings for storing data classification results.
*
*
* @param getClassificationExportConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetClassificationExportConfiguration operation returned by the
* service.
* @sample AmazonMacie2AsyncHandler.GetClassificationExportConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future getClassificationExportConfigurationAsync(
GetClassificationExportConfigurationRequest getClassificationExportConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the classification scope settings for an account.
*
*
* @param getClassificationScopeRequest
* @return A Java Future containing the result of the GetClassificationScope operation returned by the service.
* @sample AmazonMacie2Async.GetClassificationScope
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getClassificationScopeAsync(GetClassificationScopeRequest getClassificationScopeRequest);
/**
*
* Retrieves the classification scope settings for an account.
*
*
* @param getClassificationScopeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetClassificationScope operation returned by the service.
* @sample AmazonMacie2AsyncHandler.GetClassificationScope
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getClassificationScopeAsync(GetClassificationScopeRequest getClassificationScopeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the criteria and other settings for a custom data identifier.
*
*
* @param getCustomDataIdentifierRequest
* @return A Java Future containing the result of the GetCustomDataIdentifier operation returned by the service.
* @sample AmazonMacie2Async.GetCustomDataIdentifier
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getCustomDataIdentifierAsync(GetCustomDataIdentifierRequest getCustomDataIdentifierRequest);
/**
*
* Retrieves the criteria and other settings for a custom data identifier.
*
*
* @param getCustomDataIdentifierRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetCustomDataIdentifier operation returned by the service.
* @sample AmazonMacie2AsyncHandler.GetCustomDataIdentifier
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getCustomDataIdentifierAsync(GetCustomDataIdentifierRequest getCustomDataIdentifierRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves (queries) aggregated statistical data about findings.
*
*
* @param getFindingStatisticsRequest
* @return A Java Future containing the result of the GetFindingStatistics operation returned by the service.
* @sample AmazonMacie2Async.GetFindingStatistics
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getFindingStatisticsAsync(GetFindingStatisticsRequest getFindingStatisticsRequest);
/**
*
* Retrieves (queries) aggregated statistical data about findings.
*
*
* @param getFindingStatisticsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetFindingStatistics operation returned by the service.
* @sample AmazonMacie2AsyncHandler.GetFindingStatistics
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getFindingStatisticsAsync(GetFindingStatisticsRequest getFindingStatisticsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the details of one or more findings.
*
*
* @param getFindingsRequest
* @return A Java Future containing the result of the GetFindings operation returned by the service.
* @sample AmazonMacie2Async.GetFindings
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getFindingsAsync(GetFindingsRequest getFindingsRequest);
/**
*
* Retrieves the details of one or more findings.
*
*
* @param getFindingsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetFindings operation returned by the service.
* @sample AmazonMacie2AsyncHandler.GetFindings
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getFindingsAsync(GetFindingsRequest getFindingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the criteria and other settings for a findings filter.
*
*
* @param getFindingsFilterRequest
* @return A Java Future containing the result of the GetFindingsFilter operation returned by the service.
* @sample AmazonMacie2Async.GetFindingsFilter
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getFindingsFilterAsync(GetFindingsFilterRequest getFindingsFilterRequest);
/**
*
* Retrieves the criteria and other settings for a findings filter.
*
*
* @param getFindingsFilterRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetFindingsFilter operation returned by the service.
* @sample AmazonMacie2AsyncHandler.GetFindingsFilter
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getFindingsFilterAsync(GetFindingsFilterRequest getFindingsFilterRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the configuration settings for publishing findings to Security Hub.
*
*
* @param getFindingsPublicationConfigurationRequest
* @return A Java Future containing the result of the GetFindingsPublicationConfiguration operation returned by the
* service.
* @sample AmazonMacie2Async.GetFindingsPublicationConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future getFindingsPublicationConfigurationAsync(
GetFindingsPublicationConfigurationRequest getFindingsPublicationConfigurationRequest);
/**
*
* Retrieves the configuration settings for publishing findings to Security Hub.
*
*
* @param getFindingsPublicationConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetFindingsPublicationConfiguration operation returned by the
* service.
* @sample AmazonMacie2AsyncHandler.GetFindingsPublicationConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future getFindingsPublicationConfigurationAsync(
GetFindingsPublicationConfigurationRequest getFindingsPublicationConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the count of Amazon Macie membership invitations that were received by an account.
*
*
* @param getInvitationsCountRequest
* @return A Java Future containing the result of the GetInvitationsCount operation returned by the service.
* @sample AmazonMacie2Async.GetInvitationsCount
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getInvitationsCountAsync(GetInvitationsCountRequest getInvitationsCountRequest);
/**
*
* Retrieves the count of Amazon Macie membership invitations that were received by an account.
*
*
* @param getInvitationsCountRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetInvitationsCount operation returned by the service.
* @sample AmazonMacie2AsyncHandler.GetInvitationsCount
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getInvitationsCountAsync(GetInvitationsCountRequest getInvitationsCountRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the status and configuration settings for an Amazon Macie account.
*
*
* @param getMacieSessionRequest
* @return A Java Future containing the result of the GetMacieSession operation returned by the service.
* @sample AmazonMacie2Async.GetMacieSession
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getMacieSessionAsync(GetMacieSessionRequest getMacieSessionRequest);
/**
*
* Retrieves the status and configuration settings for an Amazon Macie account.
*
*
* @param getMacieSessionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetMacieSession operation returned by the service.
* @sample AmazonMacie2AsyncHandler.GetMacieSession
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getMacieSessionAsync(GetMacieSessionRequest getMacieSessionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* (Deprecated) Retrieves information about the Amazon Macie administrator account for an account. This operation
* has been replaced by the GetAdministratorAccount operation.
*
*
* @param getMasterAccountRequest
* @return A Java Future containing the result of the GetMasterAccount operation returned by the service.
* @sample AmazonMacie2Async.GetMasterAccount
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getMasterAccountAsync(GetMasterAccountRequest getMasterAccountRequest);
/**
*
* (Deprecated) Retrieves information about the Amazon Macie administrator account for an account. This operation
* has been replaced by the GetAdministratorAccount operation.
*
*
* @param getMasterAccountRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetMasterAccount operation returned by the service.
* @sample AmazonMacie2AsyncHandler.GetMasterAccount
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getMasterAccountAsync(GetMasterAccountRequest getMasterAccountRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves information about an account that's associated with an Amazon Macie administrator account.
*
*
* @param getMemberRequest
* @return A Java Future containing the result of the GetMember operation returned by the service.
* @sample AmazonMacie2Async.GetMember
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getMemberAsync(GetMemberRequest getMemberRequest);
/**
*
* Retrieves information about an account that's associated with an Amazon Macie administrator account.
*
*
* @param getMemberRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetMember operation returned by the service.
* @sample AmazonMacie2AsyncHandler.GetMember
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getMemberAsync(GetMemberRequest getMemberRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves (queries) sensitive data discovery statistics and the sensitivity score for an S3 bucket.
*
*
* @param getResourceProfileRequest
* @return A Java Future containing the result of the GetResourceProfile operation returned by the service.
* @sample AmazonMacie2Async.GetResourceProfile
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getResourceProfileAsync(GetResourceProfileRequest getResourceProfileRequest);
/**
*
* Retrieves (queries) sensitive data discovery statistics and the sensitivity score for an S3 bucket.
*
*
* @param getResourceProfileRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetResourceProfile operation returned by the service.
* @sample AmazonMacie2AsyncHandler.GetResourceProfile
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getResourceProfileAsync(GetResourceProfileRequest getResourceProfileRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the status and configuration settings for retrieving occurrences of sensitive data reported by
* findings.
*
*
* @param getRevealConfigurationRequest
* @return A Java Future containing the result of the GetRevealConfiguration operation returned by the service.
* @sample AmazonMacie2Async.GetRevealConfiguration
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getRevealConfigurationAsync(GetRevealConfigurationRequest getRevealConfigurationRequest);
/**
*
* Retrieves the status and configuration settings for retrieving occurrences of sensitive data reported by
* findings.
*
*
* @param getRevealConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetRevealConfiguration operation returned by the service.
* @sample AmazonMacie2AsyncHandler.GetRevealConfiguration
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getRevealConfigurationAsync(GetRevealConfigurationRequest getRevealConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves occurrences of sensitive data reported by a finding.
*
*
* @param getSensitiveDataOccurrencesRequest
* @return A Java Future containing the result of the GetSensitiveDataOccurrences operation returned by the service.
* @sample AmazonMacie2Async.GetSensitiveDataOccurrences
* @see AWS API Documentation
*/
java.util.concurrent.Future getSensitiveDataOccurrencesAsync(
GetSensitiveDataOccurrencesRequest getSensitiveDataOccurrencesRequest);
/**
*
* Retrieves occurrences of sensitive data reported by a finding.
*
*
* @param getSensitiveDataOccurrencesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetSensitiveDataOccurrences operation returned by the service.
* @sample AmazonMacie2AsyncHandler.GetSensitiveDataOccurrences
* @see AWS API Documentation
*/
java.util.concurrent.Future getSensitiveDataOccurrencesAsync(
GetSensitiveDataOccurrencesRequest getSensitiveDataOccurrencesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Checks whether occurrences of sensitive data can be retrieved for a finding.
*
*
* @param getSensitiveDataOccurrencesAvailabilityRequest
* @return A Java Future containing the result of the GetSensitiveDataOccurrencesAvailability operation returned by
* the service.
* @sample AmazonMacie2Async.GetSensitiveDataOccurrencesAvailability
* @see AWS API Documentation
*/
java.util.concurrent.Future getSensitiveDataOccurrencesAvailabilityAsync(
GetSensitiveDataOccurrencesAvailabilityRequest getSensitiveDataOccurrencesAvailabilityRequest);
/**
*
* Checks whether occurrences of sensitive data can be retrieved for a finding.
*
*
* @param getSensitiveDataOccurrencesAvailabilityRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetSensitiveDataOccurrencesAvailability operation returned by
* the service.
* @sample AmazonMacie2AsyncHandler.GetSensitiveDataOccurrencesAvailability
* @see AWS API Documentation
*/
java.util.concurrent.Future getSensitiveDataOccurrencesAvailabilityAsync(
GetSensitiveDataOccurrencesAvailabilityRequest getSensitiveDataOccurrencesAvailabilityRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the settings for the sensitivity inspection template for an account.
*
*
* @param getSensitivityInspectionTemplateRequest
* @return A Java Future containing the result of the GetSensitivityInspectionTemplate operation returned by the
* service.
* @sample AmazonMacie2Async.GetSensitivityInspectionTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future getSensitivityInspectionTemplateAsync(
GetSensitivityInspectionTemplateRequest getSensitivityInspectionTemplateRequest);
/**
*
* Retrieves the settings for the sensitivity inspection template for an account.
*
*
* @param getSensitivityInspectionTemplateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetSensitivityInspectionTemplate operation returned by the
* service.
* @sample AmazonMacie2AsyncHandler.GetSensitivityInspectionTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future getSensitivityInspectionTemplateAsync(
GetSensitivityInspectionTemplateRequest getSensitivityInspectionTemplateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves (queries) quotas and aggregated usage data for one or more accounts.
*
*
* @param getUsageStatisticsRequest
* @return A Java Future containing the result of the GetUsageStatistics operation returned by the service.
* @sample AmazonMacie2Async.GetUsageStatistics
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getUsageStatisticsAsync(GetUsageStatisticsRequest getUsageStatisticsRequest);
/**
*
* Retrieves (queries) quotas and aggregated usage data for one or more accounts.
*
*
* @param getUsageStatisticsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetUsageStatistics operation returned by the service.
* @sample AmazonMacie2AsyncHandler.GetUsageStatistics
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getUsageStatisticsAsync(GetUsageStatisticsRequest getUsageStatisticsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves (queries) aggregated usage data for an account.
*
*
* @param getUsageTotalsRequest
* @return A Java Future containing the result of the GetUsageTotals operation returned by the service.
* @sample AmazonMacie2Async.GetUsageTotals
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getUsageTotalsAsync(GetUsageTotalsRequest getUsageTotalsRequest);
/**
*
* Retrieves (queries) aggregated usage data for an account.
*
*
* @param getUsageTotalsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetUsageTotals operation returned by the service.
* @sample AmazonMacie2AsyncHandler.GetUsageTotals
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getUsageTotalsAsync(GetUsageTotalsRequest getUsageTotalsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a subset of information about all the allow lists for an account.
*
*
* @param listAllowListsRequest
* @return A Java Future containing the result of the ListAllowLists operation returned by the service.
* @sample AmazonMacie2Async.ListAllowLists
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listAllowListsAsync(ListAllowListsRequest listAllowListsRequest);
/**
*
* Retrieves a subset of information about all the allow lists for an account.
*
*
* @param listAllowListsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListAllowLists operation returned by the service.
* @sample AmazonMacie2AsyncHandler.ListAllowLists
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listAllowListsAsync(ListAllowListsRequest listAllowListsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a subset of information about one or more classification jobs.
*
*
* @param listClassificationJobsRequest
* @return A Java Future containing the result of the ListClassificationJobs operation returned by the service.
* @sample AmazonMacie2Async.ListClassificationJobs
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listClassificationJobsAsync(ListClassificationJobsRequest listClassificationJobsRequest);
/**
*
* Retrieves a subset of information about one or more classification jobs.
*
*
* @param listClassificationJobsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListClassificationJobs operation returned by the service.
* @sample AmazonMacie2AsyncHandler.ListClassificationJobs
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listClassificationJobsAsync(ListClassificationJobsRequest listClassificationJobsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a subset of information about the classification scope for an account.
*
*
* @param listClassificationScopesRequest
* @return A Java Future containing the result of the ListClassificationScopes operation returned by the service.
* @sample AmazonMacie2Async.ListClassificationScopes
* @see AWS API Documentation
*/
java.util.concurrent.Future listClassificationScopesAsync(ListClassificationScopesRequest listClassificationScopesRequest);
/**
*
* Retrieves a subset of information about the classification scope for an account.
*
*
* @param listClassificationScopesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListClassificationScopes operation returned by the service.
* @sample AmazonMacie2AsyncHandler.ListClassificationScopes
* @see AWS API Documentation
*/
java.util.concurrent.Future listClassificationScopesAsync(ListClassificationScopesRequest listClassificationScopesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a subset of information about all the custom data identifiers for an account.
*
*
* @param listCustomDataIdentifiersRequest
* @return A Java Future containing the result of the ListCustomDataIdentifiers operation returned by the service.
* @sample AmazonMacie2Async.ListCustomDataIdentifiers
* @see AWS API Documentation
*/
java.util.concurrent.Future listCustomDataIdentifiersAsync(
ListCustomDataIdentifiersRequest listCustomDataIdentifiersRequest);
/**
*
* Retrieves a subset of information about all the custom data identifiers for an account.
*
*
* @param listCustomDataIdentifiersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListCustomDataIdentifiers operation returned by the service.
* @sample AmazonMacie2AsyncHandler.ListCustomDataIdentifiers
* @see AWS API Documentation
*/
java.util.concurrent.Future listCustomDataIdentifiersAsync(
ListCustomDataIdentifiersRequest listCustomDataIdentifiersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a subset of information about one or more findings.
*
*
* @param listFindingsRequest
* @return A Java Future containing the result of the ListFindings operation returned by the service.
* @sample AmazonMacie2Async.ListFindings
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listFindingsAsync(ListFindingsRequest listFindingsRequest);
/**
*
* Retrieves a subset of information about one or more findings.
*
*
* @param listFindingsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListFindings operation returned by the service.
* @sample AmazonMacie2AsyncHandler.ListFindings
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listFindingsAsync(ListFindingsRequest listFindingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a subset of information about all the findings filters for an account.
*
*
* @param listFindingsFiltersRequest
* @return A Java Future containing the result of the ListFindingsFilters operation returned by the service.
* @sample AmazonMacie2Async.ListFindingsFilters
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listFindingsFiltersAsync(ListFindingsFiltersRequest listFindingsFiltersRequest);
/**
*
* Retrieves a subset of information about all the findings filters for an account.
*
*
* @param listFindingsFiltersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListFindingsFilters operation returned by the service.
* @sample AmazonMacie2AsyncHandler.ListFindingsFilters
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listFindingsFiltersAsync(ListFindingsFiltersRequest listFindingsFiltersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves information about the Amazon Macie membership invitations that were received by an account.
*
*
* @param listInvitationsRequest
* @return A Java Future containing the result of the ListInvitations operation returned by the service.
* @sample AmazonMacie2Async.ListInvitations
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listInvitationsAsync(ListInvitationsRequest listInvitationsRequest);
/**
*
* Retrieves information about the Amazon Macie membership invitations that were received by an account.
*
*
* @param listInvitationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListInvitations operation returned by the service.
* @sample AmazonMacie2AsyncHandler.ListInvitations
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listInvitationsAsync(ListInvitationsRequest listInvitationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves information about all the managed data identifiers that Amazon Macie currently provides.
*
*
* @param listManagedDataIdentifiersRequest
* @return A Java Future containing the result of the ListManagedDataIdentifiers operation returned by the service.
* @sample AmazonMacie2Async.ListManagedDataIdentifiers
* @see AWS API Documentation
*/
java.util.concurrent.Future listManagedDataIdentifiersAsync(
ListManagedDataIdentifiersRequest listManagedDataIdentifiersRequest);
/**
*
* Retrieves information about all the managed data identifiers that Amazon Macie currently provides.
*
*
* @param listManagedDataIdentifiersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListManagedDataIdentifiers operation returned by the service.
* @sample AmazonMacie2AsyncHandler.ListManagedDataIdentifiers
* @see AWS API Documentation
*/
java.util.concurrent.Future listManagedDataIdentifiersAsync(
ListManagedDataIdentifiersRequest listManagedDataIdentifiersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves information about the accounts that are associated with an Amazon Macie administrator account.
*
*
* @param listMembersRequest
* @return A Java Future containing the result of the ListMembers operation returned by the service.
* @sample AmazonMacie2Async.ListMembers
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listMembersAsync(ListMembersRequest listMembersRequest);
/**
*
* Retrieves information about the accounts that are associated with an Amazon Macie administrator account.
*
*
* @param listMembersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListMembers operation returned by the service.
* @sample AmazonMacie2AsyncHandler.ListMembers
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listMembersAsync(ListMembersRequest listMembersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves information about the delegated Amazon Macie administrator account for an organization in
* Organizations.
*
*
* @param listOrganizationAdminAccountsRequest
* @return A Java Future containing the result of the ListOrganizationAdminAccounts operation returned by the
* service.
* @sample AmazonMacie2Async.ListOrganizationAdminAccounts
* @see AWS API Documentation
*/
java.util.concurrent.Future listOrganizationAdminAccountsAsync(
ListOrganizationAdminAccountsRequest listOrganizationAdminAccountsRequest);
/**
*
* Retrieves information about the delegated Amazon Macie administrator account for an organization in
* Organizations.
*
*
* @param listOrganizationAdminAccountsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListOrganizationAdminAccounts operation returned by the
* service.
* @sample AmazonMacie2AsyncHandler.ListOrganizationAdminAccounts
* @see AWS API Documentation
*/
java.util.concurrent.Future listOrganizationAdminAccountsAsync(
ListOrganizationAdminAccountsRequest listOrganizationAdminAccountsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves information about objects that were selected from an S3 bucket for automated sensitive data discovery.
*
*
* @param listResourceProfileArtifactsRequest
* @return A Java Future containing the result of the ListResourceProfileArtifacts operation returned by the
* service.
* @sample AmazonMacie2Async.ListResourceProfileArtifacts
* @see AWS API Documentation
*/
java.util.concurrent.Future listResourceProfileArtifactsAsync(
ListResourceProfileArtifactsRequest listResourceProfileArtifactsRequest);
/**
*
* Retrieves information about objects that were selected from an S3 bucket for automated sensitive data discovery.
*
*
* @param listResourceProfileArtifactsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListResourceProfileArtifacts operation returned by the
* service.
* @sample AmazonMacie2AsyncHandler.ListResourceProfileArtifacts
* @see AWS API Documentation
*/
java.util.concurrent.Future listResourceProfileArtifactsAsync(
ListResourceProfileArtifactsRequest listResourceProfileArtifactsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves information about the types and amount of sensitive data that Amazon Macie found in an S3 bucket.
*
*
* @param listResourceProfileDetectionsRequest
* @return A Java Future containing the result of the ListResourceProfileDetections operation returned by the
* service.
* @sample AmazonMacie2Async.ListResourceProfileDetections
* @see AWS API Documentation
*/
java.util.concurrent.Future listResourceProfileDetectionsAsync(
ListResourceProfileDetectionsRequest listResourceProfileDetectionsRequest);
/**
*
* Retrieves information about the types and amount of sensitive data that Amazon Macie found in an S3 bucket.
*
*
* @param listResourceProfileDetectionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListResourceProfileDetections operation returned by the
* service.
* @sample AmazonMacie2AsyncHandler.ListResourceProfileDetections
* @see AWS API Documentation
*/
java.util.concurrent.Future listResourceProfileDetectionsAsync(
ListResourceProfileDetectionsRequest listResourceProfileDetectionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a subset of information about the sensitivity inspection template for an account.
*
*
* @param listSensitivityInspectionTemplatesRequest
* @return A Java Future containing the result of the ListSensitivityInspectionTemplates operation returned by the
* service.
* @sample AmazonMacie2Async.ListSensitivityInspectionTemplates
* @see AWS API Documentation
*/
java.util.concurrent.Future listSensitivityInspectionTemplatesAsync(
ListSensitivityInspectionTemplatesRequest listSensitivityInspectionTemplatesRequest);
/**
*
* Retrieves a subset of information about the sensitivity inspection template for an account.
*
*
* @param listSensitivityInspectionTemplatesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListSensitivityInspectionTemplates operation returned by the
* service.
* @sample AmazonMacie2AsyncHandler.ListSensitivityInspectionTemplates
* @see AWS API Documentation
*/
java.util.concurrent.Future listSensitivityInspectionTemplatesAsync(
ListSensitivityInspectionTemplatesRequest listSensitivityInspectionTemplatesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the tags (keys and values) that are associated with an Amazon Macie resource.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AmazonMacie2Async.ListTagsForResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Retrieves the tags (keys and values) that are associated with an Amazon Macie resource.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AmazonMacie2AsyncHandler.ListTagsForResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates or updates the configuration settings for storing data classification results.
*
*
* @param putClassificationExportConfigurationRequest
* @return A Java Future containing the result of the PutClassificationExportConfiguration operation returned by the
* service.
* @sample AmazonMacie2Async.PutClassificationExportConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future putClassificationExportConfigurationAsync(
PutClassificationExportConfigurationRequest putClassificationExportConfigurationRequest);
/**
*
* Creates or updates the configuration settings for storing data classification results.
*
*
* @param putClassificationExportConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutClassificationExportConfiguration operation returned by the
* service.
* @sample AmazonMacie2AsyncHandler.PutClassificationExportConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future putClassificationExportConfigurationAsync(
PutClassificationExportConfigurationRequest putClassificationExportConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the configuration settings for publishing findings to Security Hub.
*
*
* @param putFindingsPublicationConfigurationRequest
* @return A Java Future containing the result of the PutFindingsPublicationConfiguration operation returned by the
* service.
* @sample AmazonMacie2Async.PutFindingsPublicationConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future putFindingsPublicationConfigurationAsync(
PutFindingsPublicationConfigurationRequest putFindingsPublicationConfigurationRequest);
/**
*
* Updates the configuration settings for publishing findings to Security Hub.
*
*
* @param putFindingsPublicationConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutFindingsPublicationConfiguration operation returned by the
* service.
* @sample AmazonMacie2AsyncHandler.PutFindingsPublicationConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future putFindingsPublicationConfigurationAsync(
PutFindingsPublicationConfigurationRequest putFindingsPublicationConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves (queries) statistical data and other information about Amazon Web Services resources that Amazon Macie
* monitors and analyzes.
*
*
* @param searchResourcesRequest
* @return A Java Future containing the result of the SearchResources operation returned by the service.
* @sample AmazonMacie2Async.SearchResources
* @see AWS API
* Documentation
*/
java.util.concurrent.Future searchResourcesAsync(SearchResourcesRequest searchResourcesRequest);
/**
*
* Retrieves (queries) statistical data and other information about Amazon Web Services resources that Amazon Macie
* monitors and analyzes.
*
*
* @param searchResourcesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the SearchResources operation returned by the service.
* @sample AmazonMacie2AsyncHandler.SearchResources
* @see AWS API
* Documentation
*/
java.util.concurrent.Future searchResourcesAsync(SearchResourcesRequest searchResourcesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds or updates one or more tags (keys and values) that are associated with an Amazon Macie resource.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AmazonMacie2Async.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* Adds or updates one or more tags (keys and values) that are associated with an Amazon Macie resource.
*
*
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AmazonMacie2AsyncHandler.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Tests a custom data identifier.
*
*
* @param testCustomDataIdentifierRequest
* @return A Java Future containing the result of the TestCustomDataIdentifier operation returned by the service.
* @sample AmazonMacie2Async.TestCustomDataIdentifier
* @see AWS API Documentation
*/
java.util.concurrent.Future testCustomDataIdentifierAsync(TestCustomDataIdentifierRequest testCustomDataIdentifierRequest);
/**
*
* Tests a custom data identifier.
*
*
* @param testCustomDataIdentifierRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TestCustomDataIdentifier operation returned by the service.
* @sample AmazonMacie2AsyncHandler.TestCustomDataIdentifier
* @see AWS API Documentation
*/
java.util.concurrent.Future testCustomDataIdentifierAsync(TestCustomDataIdentifierRequest testCustomDataIdentifierRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes one or more tags (keys and values) from an Amazon Macie resource.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AmazonMacie2Async.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* Removes one or more tags (keys and values) from an Amazon Macie resource.
*
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AmazonMacie2AsyncHandler.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the settings for an allow list.
*
*
* @param updateAllowListRequest
* @return A Java Future containing the result of the UpdateAllowList operation returned by the service.
* @sample AmazonMacie2Async.UpdateAllowList
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateAllowListAsync(UpdateAllowListRequest updateAllowListRequest);
/**
*
* Updates the settings for an allow list.
*
*
* @param updateAllowListRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateAllowList operation returned by the service.
* @sample AmazonMacie2AsyncHandler.UpdateAllowList
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateAllowListAsync(UpdateAllowListRequest updateAllowListRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Enables or disables automated sensitive data discovery for an account.
*
*
* @param updateAutomatedDiscoveryConfigurationRequest
* @return A Java Future containing the result of the UpdateAutomatedDiscoveryConfiguration operation returned by
* the service.
* @sample AmazonMacie2Async.UpdateAutomatedDiscoveryConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future updateAutomatedDiscoveryConfigurationAsync(
UpdateAutomatedDiscoveryConfigurationRequest updateAutomatedDiscoveryConfigurationRequest);
/**
*
* Enables or disables automated sensitive data discovery for an account.
*
*
* @param updateAutomatedDiscoveryConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateAutomatedDiscoveryConfiguration operation returned by
* the service.
* @sample AmazonMacie2AsyncHandler.UpdateAutomatedDiscoveryConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future updateAutomatedDiscoveryConfigurationAsync(
UpdateAutomatedDiscoveryConfigurationRequest updateAutomatedDiscoveryConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Changes the status of a classification job.
*
*
* @param updateClassificationJobRequest
* @return A Java Future containing the result of the UpdateClassificationJob operation returned by the service.
* @sample AmazonMacie2Async.UpdateClassificationJob
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateClassificationJobAsync(UpdateClassificationJobRequest updateClassificationJobRequest);
/**
*
* Changes the status of a classification job.
*
*
* @param updateClassificationJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateClassificationJob operation returned by the service.
* @sample AmazonMacie2AsyncHandler.UpdateClassificationJob
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateClassificationJobAsync(UpdateClassificationJobRequest updateClassificationJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the classification scope settings for an account.
*
*
* @param updateClassificationScopeRequest
* @return A Java Future containing the result of the UpdateClassificationScope operation returned by the service.
* @sample AmazonMacie2Async.UpdateClassificationScope
* @see AWS API Documentation
*/
java.util.concurrent.Future updateClassificationScopeAsync(
UpdateClassificationScopeRequest updateClassificationScopeRequest);
/**
*
* Updates the classification scope settings for an account.
*
*
* @param updateClassificationScopeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateClassificationScope operation returned by the service.
* @sample AmazonMacie2AsyncHandler.UpdateClassificationScope
* @see AWS API Documentation
*/
java.util.concurrent.Future updateClassificationScopeAsync(
UpdateClassificationScopeRequest updateClassificationScopeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the criteria and other settings for a findings filter.
*
*
* @param updateFindingsFilterRequest
* @return A Java Future containing the result of the UpdateFindingsFilter operation returned by the service.
* @sample AmazonMacie2Async.UpdateFindingsFilter
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateFindingsFilterAsync(UpdateFindingsFilterRequest updateFindingsFilterRequest);
/**
*
* Updates the criteria and other settings for a findings filter.
*
*
* @param updateFindingsFilterRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateFindingsFilter operation returned by the service.
* @sample AmazonMacie2AsyncHandler.UpdateFindingsFilter
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateFindingsFilterAsync(UpdateFindingsFilterRequest updateFindingsFilterRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Suspends or re-enables Amazon Macie, or updates the configuration settings for a Macie account.
*
*
* @param updateMacieSessionRequest
* @return A Java Future containing the result of the UpdateMacieSession operation returned by the service.
* @sample AmazonMacie2Async.UpdateMacieSession
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateMacieSessionAsync(UpdateMacieSessionRequest updateMacieSessionRequest);
/**
*
* Suspends or re-enables Amazon Macie, or updates the configuration settings for a Macie account.
*
*
* @param updateMacieSessionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateMacieSession operation returned by the service.
* @sample AmazonMacie2AsyncHandler.UpdateMacieSession
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateMacieSessionAsync(UpdateMacieSessionRequest updateMacieSessionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Enables an Amazon Macie administrator to suspend or re-enable Macie for a member account.
*
*
* @param updateMemberSessionRequest
* @return A Java Future containing the result of the UpdateMemberSession operation returned by the service.
* @sample AmazonMacie2Async.UpdateMemberSession
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateMemberSessionAsync(UpdateMemberSessionRequest updateMemberSessionRequest);
/**
*
* Enables an Amazon Macie administrator to suspend or re-enable Macie for a member account.
*
*
* @param updateMemberSessionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateMemberSession operation returned by the service.
* @sample AmazonMacie2AsyncHandler.UpdateMemberSession
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateMemberSessionAsync(UpdateMemberSessionRequest updateMemberSessionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the Amazon Macie configuration settings for an organization in Organizations.
*
*
* @param updateOrganizationConfigurationRequest
* @return A Java Future containing the result of the UpdateOrganizationConfiguration operation returned by the
* service.
* @sample AmazonMacie2Async.UpdateOrganizationConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future updateOrganizationConfigurationAsync(
UpdateOrganizationConfigurationRequest updateOrganizationConfigurationRequest);
/**
*
* Updates the Amazon Macie configuration settings for an organization in Organizations.
*
*
* @param updateOrganizationConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateOrganizationConfiguration operation returned by the
* service.
* @sample AmazonMacie2AsyncHandler.UpdateOrganizationConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future updateOrganizationConfigurationAsync(
UpdateOrganizationConfigurationRequest updateOrganizationConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the sensitivity score for an S3 bucket.
*
*
* @param updateResourceProfileRequest
* @return A Java Future containing the result of the UpdateResourceProfile operation returned by the service.
* @sample AmazonMacie2Async.UpdateResourceProfile
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateResourceProfileAsync(UpdateResourceProfileRequest updateResourceProfileRequest);
/**
*
* Updates the sensitivity score for an S3 bucket.
*
*
* @param updateResourceProfileRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateResourceProfile operation returned by the service.
* @sample AmazonMacie2AsyncHandler.UpdateResourceProfile
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateResourceProfileAsync(UpdateResourceProfileRequest updateResourceProfileRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the sensitivity scoring settings for an S3 bucket.
*
*
* @param updateResourceProfileDetectionsRequest
* @return A Java Future containing the result of the UpdateResourceProfileDetections operation returned by the
* service.
* @sample AmazonMacie2Async.UpdateResourceProfileDetections
* @see AWS API Documentation
*/
java.util.concurrent.Future updateResourceProfileDetectionsAsync(
UpdateResourceProfileDetectionsRequest updateResourceProfileDetectionsRequest);
/**
*
* Updates the sensitivity scoring settings for an S3 bucket.
*
*
* @param updateResourceProfileDetectionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateResourceProfileDetections operation returned by the
* service.
* @sample AmazonMacie2AsyncHandler.UpdateResourceProfileDetections
* @see AWS API Documentation
*/
java.util.concurrent.Future updateResourceProfileDetectionsAsync(
UpdateResourceProfileDetectionsRequest updateResourceProfileDetectionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the status and configuration settings for retrieving occurrences of sensitive data reported by findings.
*
*
* @param updateRevealConfigurationRequest
* @return A Java Future containing the result of the UpdateRevealConfiguration operation returned by the service.
* @sample AmazonMacie2Async.UpdateRevealConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future updateRevealConfigurationAsync(
UpdateRevealConfigurationRequest updateRevealConfigurationRequest);
/**
*
* Updates the status and configuration settings for retrieving occurrences of sensitive data reported by findings.
*
*
* @param updateRevealConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateRevealConfiguration operation returned by the service.
* @sample AmazonMacie2AsyncHandler.UpdateRevealConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future updateRevealConfigurationAsync(
UpdateRevealConfigurationRequest updateRevealConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the settings for the sensitivity inspection template for an account.
*
*
* @param updateSensitivityInspectionTemplateRequest
* @return A Java Future containing the result of the UpdateSensitivityInspectionTemplate operation returned by the
* service.
* @sample AmazonMacie2Async.UpdateSensitivityInspectionTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future updateSensitivityInspectionTemplateAsync(
UpdateSensitivityInspectionTemplateRequest updateSensitivityInspectionTemplateRequest);
/**
*
* Updates the settings for the sensitivity inspection template for an account.
*
*
* @param updateSensitivityInspectionTemplateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateSensitivityInspectionTemplate operation returned by the
* service.
* @sample AmazonMacie2AsyncHandler.UpdateSensitivityInspectionTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future updateSensitivityInspectionTemplateAsync(
UpdateSensitivityInspectionTemplateRequest updateSensitivityInspectionTemplateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}