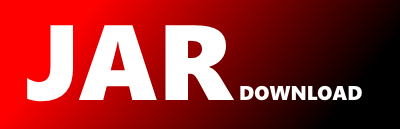
com.amazonaws.services.macie2.AmazonMacie2Client Maven / Gradle / Ivy
Show all versions of aws-java-sdk-macie2 Show documentation
/*
* Copyright 2018-2023 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.macie2;
import org.w3c.dom.*;
import java.net.*;
import java.util.*;
import javax.annotation.Generated;
import org.apache.commons.logging.*;
import com.amazonaws.*;
import com.amazonaws.annotation.SdkInternalApi;
import com.amazonaws.auth.*;
import com.amazonaws.handlers.*;
import com.amazonaws.http.*;
import com.amazonaws.internal.*;
import com.amazonaws.internal.auth.*;
import com.amazonaws.metrics.*;
import com.amazonaws.regions.*;
import com.amazonaws.transform.*;
import com.amazonaws.util.*;
import com.amazonaws.protocol.json.*;
import com.amazonaws.util.AWSRequestMetrics.Field;
import com.amazonaws.annotation.ThreadSafe;
import com.amazonaws.client.AwsSyncClientParams;
import com.amazonaws.client.builder.AdvancedConfig;
import com.amazonaws.services.macie2.AmazonMacie2ClientBuilder;
import com.amazonaws.services.macie2.waiters.AmazonMacie2Waiters;
import com.amazonaws.AmazonServiceException;
import com.amazonaws.services.macie2.model.*;
import com.amazonaws.services.macie2.model.transform.*;
/**
* Client for accessing Amazon Macie 2. All service calls made using this client are blocking, and will not return until
* the service call completes.
*
*
* Amazon Macie
*
*/
@ThreadSafe
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AmazonMacie2Client extends AmazonWebServiceClient implements AmazonMacie2 {
/** Provider for AWS credentials. */
private final AWSCredentialsProvider awsCredentialsProvider;
private static final Log log = LogFactory.getLog(AmazonMacie2.class);
/** Default signing name for the service. */
private static final String DEFAULT_SIGNING_NAME = "macie2";
private volatile AmazonMacie2Waiters waiters;
/** Client configuration factory providing ClientConfigurations tailored to this client */
protected static final ClientConfigurationFactory configFactory = new ClientConfigurationFactory();
private final AdvancedConfig advancedConfig;
private static final com.amazonaws.protocol.json.SdkJsonProtocolFactory protocolFactory = new com.amazonaws.protocol.json.SdkJsonProtocolFactory(
new JsonClientMetadata()
.withProtocolVersion("1.1")
.withSupportsCbor(false)
.withSupportsIon(false)
.withContentTypeOverride("application/json")
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ServiceQuotaExceededException").withExceptionUnmarshaller(
com.amazonaws.services.macie2.model.transform.ServiceQuotaExceededExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InternalServerException").withExceptionUnmarshaller(
com.amazonaws.services.macie2.model.transform.InternalServerExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ResourceNotFoundException").withExceptionUnmarshaller(
com.amazonaws.services.macie2.model.transform.ResourceNotFoundExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ValidationException").withExceptionUnmarshaller(
com.amazonaws.services.macie2.model.transform.ValidationExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ThrottlingException").withExceptionUnmarshaller(
com.amazonaws.services.macie2.model.transform.ThrottlingExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("AccessDeniedException").withExceptionUnmarshaller(
com.amazonaws.services.macie2.model.transform.AccessDeniedExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ConflictException").withExceptionUnmarshaller(
com.amazonaws.services.macie2.model.transform.ConflictExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("UnprocessableEntityException").withExceptionUnmarshaller(
com.amazonaws.services.macie2.model.transform.UnprocessableEntityExceptionUnmarshaller.getInstance()))
.withBaseServiceExceptionClass(com.amazonaws.services.macie2.model.AmazonMacie2Exception.class));
public static AmazonMacie2ClientBuilder builder() {
return AmazonMacie2ClientBuilder.standard();
}
/**
* Constructs a new client to invoke service methods on Amazon Macie 2 using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AmazonMacie2Client(AwsSyncClientParams clientParams) {
this(clientParams, false);
}
/**
* Constructs a new client to invoke service methods on Amazon Macie 2 using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AmazonMacie2Client(AwsSyncClientParams clientParams, boolean endpointDiscoveryEnabled) {
super(clientParams);
this.awsCredentialsProvider = clientParams.getCredentialsProvider();
this.advancedConfig = clientParams.getAdvancedConfig();
init();
}
private void init() {
setServiceNameIntern(DEFAULT_SIGNING_NAME);
setEndpointPrefix(ENDPOINT_PREFIX);
// calling this.setEndPoint(...) will also modify the signer accordingly
setEndpoint("macie2.us-east-1.amazonaws.com");
HandlerChainFactory chainFactory = new HandlerChainFactory();
requestHandler2s.addAll(chainFactory.newRequestHandlerChain("/com/amazonaws/services/macie2/request.handlers"));
requestHandler2s.addAll(chainFactory.newRequestHandler2Chain("/com/amazonaws/services/macie2/request.handler2s"));
requestHandler2s.addAll(chainFactory.getGlobalHandlers());
}
/**
*
* Accepts an Amazon Macie membership invitation that was received from a specific account.
*
*
* @param acceptInvitationRequest
* @return Result of the AcceptInvitation operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.AcceptInvitation
* @see AWS API
* Documentation
*/
@Override
public AcceptInvitationResult acceptInvitation(AcceptInvitationRequest request) {
request = beforeClientExecution(request);
return executeAcceptInvitation(request);
}
@SdkInternalApi
final AcceptInvitationResult executeAcceptInvitation(AcceptInvitationRequest acceptInvitationRequest) {
ExecutionContext executionContext = createExecutionContext(acceptInvitationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AcceptInvitationRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(acceptInvitationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "AcceptInvitation");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new AcceptInvitationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves information about one or more custom data identifiers.
*
*
* @param batchGetCustomDataIdentifiersRequest
* @return Result of the BatchGetCustomDataIdentifiers operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.BatchGetCustomDataIdentifiers
* @see AWS API Documentation
*/
@Override
public BatchGetCustomDataIdentifiersResult batchGetCustomDataIdentifiers(BatchGetCustomDataIdentifiersRequest request) {
request = beforeClientExecution(request);
return executeBatchGetCustomDataIdentifiers(request);
}
@SdkInternalApi
final BatchGetCustomDataIdentifiersResult executeBatchGetCustomDataIdentifiers(BatchGetCustomDataIdentifiersRequest batchGetCustomDataIdentifiersRequest) {
ExecutionContext executionContext = createExecutionContext(batchGetCustomDataIdentifiersRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new BatchGetCustomDataIdentifiersRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(batchGetCustomDataIdentifiersRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "BatchGetCustomDataIdentifiers");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new BatchGetCustomDataIdentifiersResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates and defines the settings for an allow list.
*
*
* @param createAllowListRequest
* @return Result of the CreateAllowList operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.CreateAllowList
* @see AWS API
* Documentation
*/
@Override
public CreateAllowListResult createAllowList(CreateAllowListRequest request) {
request = beforeClientExecution(request);
return executeCreateAllowList(request);
}
@SdkInternalApi
final CreateAllowListResult executeCreateAllowList(CreateAllowListRequest createAllowListRequest) {
ExecutionContext executionContext = createExecutionContext(createAllowListRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateAllowListRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createAllowListRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateAllowList");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateAllowListResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates and defines the settings for a classification job.
*
*
* @param createClassificationJobRequest
* @return Result of the CreateClassificationJob operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.CreateClassificationJob
* @see AWS
* API Documentation
*/
@Override
public CreateClassificationJobResult createClassificationJob(CreateClassificationJobRequest request) {
request = beforeClientExecution(request);
return executeCreateClassificationJob(request);
}
@SdkInternalApi
final CreateClassificationJobResult executeCreateClassificationJob(CreateClassificationJobRequest createClassificationJobRequest) {
ExecutionContext executionContext = createExecutionContext(createClassificationJobRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateClassificationJobRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(createClassificationJobRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateClassificationJob");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateClassificationJobResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates and defines the criteria and other settings for a custom data identifier.
*
*
* @param createCustomDataIdentifierRequest
* @return Result of the CreateCustomDataIdentifier operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.CreateCustomDataIdentifier
* @see AWS API Documentation
*/
@Override
public CreateCustomDataIdentifierResult createCustomDataIdentifier(CreateCustomDataIdentifierRequest request) {
request = beforeClientExecution(request);
return executeCreateCustomDataIdentifier(request);
}
@SdkInternalApi
final CreateCustomDataIdentifierResult executeCreateCustomDataIdentifier(CreateCustomDataIdentifierRequest createCustomDataIdentifierRequest) {
ExecutionContext executionContext = createExecutionContext(createCustomDataIdentifierRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateCustomDataIdentifierRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(createCustomDataIdentifierRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateCustomDataIdentifier");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateCustomDataIdentifierResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates and defines the criteria and other settings for a findings filter.
*
*
* @param createFindingsFilterRequest
* @return Result of the CreateFindingsFilter operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.CreateFindingsFilter
* @see AWS
* API Documentation
*/
@Override
public CreateFindingsFilterResult createFindingsFilter(CreateFindingsFilterRequest request) {
request = beforeClientExecution(request);
return executeCreateFindingsFilter(request);
}
@SdkInternalApi
final CreateFindingsFilterResult executeCreateFindingsFilter(CreateFindingsFilterRequest createFindingsFilterRequest) {
ExecutionContext executionContext = createExecutionContext(createFindingsFilterRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateFindingsFilterRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createFindingsFilterRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateFindingsFilter");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateFindingsFilterResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Sends an Amazon Macie membership invitation to one or more accounts.
*
*
* @param createInvitationsRequest
* @return Result of the CreateInvitations operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.CreateInvitations
* @see AWS API
* Documentation
*/
@Override
public CreateInvitationsResult createInvitations(CreateInvitationsRequest request) {
request = beforeClientExecution(request);
return executeCreateInvitations(request);
}
@SdkInternalApi
final CreateInvitationsResult executeCreateInvitations(CreateInvitationsRequest createInvitationsRequest) {
ExecutionContext executionContext = createExecutionContext(createInvitationsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateInvitationsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createInvitationsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateInvitations");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateInvitationsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Associates an account with an Amazon Macie administrator account.
*
*
* @param createMemberRequest
* @return Result of the CreateMember operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.CreateMember
* @see AWS API
* Documentation
*/
@Override
public CreateMemberResult createMember(CreateMemberRequest request) {
request = beforeClientExecution(request);
return executeCreateMember(request);
}
@SdkInternalApi
final CreateMemberResult executeCreateMember(CreateMemberRequest createMemberRequest) {
ExecutionContext executionContext = createExecutionContext(createMemberRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateMemberRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createMemberRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateMember");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateMemberResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates sample findings.
*
*
* @param createSampleFindingsRequest
* @return Result of the CreateSampleFindings operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.CreateSampleFindings
* @see AWS
* API Documentation
*/
@Override
public CreateSampleFindingsResult createSampleFindings(CreateSampleFindingsRequest request) {
request = beforeClientExecution(request);
return executeCreateSampleFindings(request);
}
@SdkInternalApi
final CreateSampleFindingsResult executeCreateSampleFindings(CreateSampleFindingsRequest createSampleFindingsRequest) {
ExecutionContext executionContext = createExecutionContext(createSampleFindingsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateSampleFindingsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createSampleFindingsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateSampleFindings");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateSampleFindingsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Declines Amazon Macie membership invitations that were received from specific accounts.
*
*
* @param declineInvitationsRequest
* @return Result of the DeclineInvitations operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.DeclineInvitations
* @see AWS API
* Documentation
*/
@Override
public DeclineInvitationsResult declineInvitations(DeclineInvitationsRequest request) {
request = beforeClientExecution(request);
return executeDeclineInvitations(request);
}
@SdkInternalApi
final DeclineInvitationsResult executeDeclineInvitations(DeclineInvitationsRequest declineInvitationsRequest) {
ExecutionContext executionContext = createExecutionContext(declineInvitationsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeclineInvitationsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(declineInvitationsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeclineInvitations");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeclineInvitationsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes an allow list.
*
*
* @param deleteAllowListRequest
* @return Result of the DeleteAllowList operation returned by the service.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @sample AmazonMacie2.DeleteAllowList
* @see AWS API
* Documentation
*/
@Override
public DeleteAllowListResult deleteAllowList(DeleteAllowListRequest request) {
request = beforeClientExecution(request);
return executeDeleteAllowList(request);
}
@SdkInternalApi
final DeleteAllowListResult executeDeleteAllowList(DeleteAllowListRequest deleteAllowListRequest) {
ExecutionContext executionContext = createExecutionContext(deleteAllowListRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteAllowListRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteAllowListRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteAllowList");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteAllowListResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Soft deletes a custom data identifier.
*
*
* @param deleteCustomDataIdentifierRequest
* @return Result of the DeleteCustomDataIdentifier operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.DeleteCustomDataIdentifier
* @see AWS API Documentation
*/
@Override
public DeleteCustomDataIdentifierResult deleteCustomDataIdentifier(DeleteCustomDataIdentifierRequest request) {
request = beforeClientExecution(request);
return executeDeleteCustomDataIdentifier(request);
}
@SdkInternalApi
final DeleteCustomDataIdentifierResult executeDeleteCustomDataIdentifier(DeleteCustomDataIdentifierRequest deleteCustomDataIdentifierRequest) {
ExecutionContext executionContext = createExecutionContext(deleteCustomDataIdentifierRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteCustomDataIdentifierRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deleteCustomDataIdentifierRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteCustomDataIdentifier");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteCustomDataIdentifierResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a findings filter.
*
*
* @param deleteFindingsFilterRequest
* @return Result of the DeleteFindingsFilter operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.DeleteFindingsFilter
* @see AWS
* API Documentation
*/
@Override
public DeleteFindingsFilterResult deleteFindingsFilter(DeleteFindingsFilterRequest request) {
request = beforeClientExecution(request);
return executeDeleteFindingsFilter(request);
}
@SdkInternalApi
final DeleteFindingsFilterResult executeDeleteFindingsFilter(DeleteFindingsFilterRequest deleteFindingsFilterRequest) {
ExecutionContext executionContext = createExecutionContext(deleteFindingsFilterRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteFindingsFilterRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteFindingsFilterRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteFindingsFilter");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteFindingsFilterResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes Amazon Macie membership invitations that were received from specific accounts.
*
*
* @param deleteInvitationsRequest
* @return Result of the DeleteInvitations operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.DeleteInvitations
* @see AWS API
* Documentation
*/
@Override
public DeleteInvitationsResult deleteInvitations(DeleteInvitationsRequest request) {
request = beforeClientExecution(request);
return executeDeleteInvitations(request);
}
@SdkInternalApi
final DeleteInvitationsResult executeDeleteInvitations(DeleteInvitationsRequest deleteInvitationsRequest) {
ExecutionContext executionContext = createExecutionContext(deleteInvitationsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteInvitationsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteInvitationsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteInvitations");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteInvitationsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the association between an Amazon Macie administrator account and an account.
*
*
* @param deleteMemberRequest
* @return Result of the DeleteMember operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.DeleteMember
* @see AWS API
* Documentation
*/
@Override
public DeleteMemberResult deleteMember(DeleteMemberRequest request) {
request = beforeClientExecution(request);
return executeDeleteMember(request);
}
@SdkInternalApi
final DeleteMemberResult executeDeleteMember(DeleteMemberRequest deleteMemberRequest) {
ExecutionContext executionContext = createExecutionContext(deleteMemberRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteMemberRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteMemberRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteMember");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteMemberResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves (queries) statistical data and other information about one or more S3 buckets that Amazon Macie
* monitors and analyzes for an account.
*
*
* @param describeBucketsRequest
* @return Result of the DescribeBuckets operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.DescribeBuckets
* @see AWS API
* Documentation
*/
@Override
public DescribeBucketsResult describeBuckets(DescribeBucketsRequest request) {
request = beforeClientExecution(request);
return executeDescribeBuckets(request);
}
@SdkInternalApi
final DescribeBucketsResult executeDescribeBuckets(DescribeBucketsRequest describeBucketsRequest) {
ExecutionContext executionContext = createExecutionContext(describeBucketsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeBucketsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeBucketsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeBuckets");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeBucketsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the status and settings for a classification job.
*
*
* @param describeClassificationJobRequest
* @return Result of the DescribeClassificationJob operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.DescribeClassificationJob
* @see AWS API Documentation
*/
@Override
public DescribeClassificationJobResult describeClassificationJob(DescribeClassificationJobRequest request) {
request = beforeClientExecution(request);
return executeDescribeClassificationJob(request);
}
@SdkInternalApi
final DescribeClassificationJobResult executeDescribeClassificationJob(DescribeClassificationJobRequest describeClassificationJobRequest) {
ExecutionContext executionContext = createExecutionContext(describeClassificationJobRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeClassificationJobRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeClassificationJobRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeClassificationJob");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeClassificationJobResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the Amazon Macie configuration settings for an organization in Organizations.
*
*
* @param describeOrganizationConfigurationRequest
* @return Result of the DescribeOrganizationConfiguration operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.DescribeOrganizationConfiguration
* @see AWS API Documentation
*/
@Override
public DescribeOrganizationConfigurationResult describeOrganizationConfiguration(DescribeOrganizationConfigurationRequest request) {
request = beforeClientExecution(request);
return executeDescribeOrganizationConfiguration(request);
}
@SdkInternalApi
final DescribeOrganizationConfigurationResult executeDescribeOrganizationConfiguration(
DescribeOrganizationConfigurationRequest describeOrganizationConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(describeOrganizationConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeOrganizationConfigurationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeOrganizationConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeOrganizationConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeOrganizationConfigurationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Disables Amazon Macie and deletes all settings and resources for a Macie account.
*
*
* @param disableMacieRequest
* @return Result of the DisableMacie operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.DisableMacie
* @see AWS API
* Documentation
*/
@Override
public DisableMacieResult disableMacie(DisableMacieRequest request) {
request = beforeClientExecution(request);
return executeDisableMacie(request);
}
@SdkInternalApi
final DisableMacieResult executeDisableMacie(DisableMacieRequest disableMacieRequest) {
ExecutionContext executionContext = createExecutionContext(disableMacieRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DisableMacieRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(disableMacieRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DisableMacie");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DisableMacieResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Disables an account as the delegated Amazon Macie administrator account for an organization in Organizations.
*
*
* @param disableOrganizationAdminAccountRequest
* @return Result of the DisableOrganizationAdminAccount operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.DisableOrganizationAdminAccount
* @see AWS API Documentation
*/
@Override
public DisableOrganizationAdminAccountResult disableOrganizationAdminAccount(DisableOrganizationAdminAccountRequest request) {
request = beforeClientExecution(request);
return executeDisableOrganizationAdminAccount(request);
}
@SdkInternalApi
final DisableOrganizationAdminAccountResult executeDisableOrganizationAdminAccount(
DisableOrganizationAdminAccountRequest disableOrganizationAdminAccountRequest) {
ExecutionContext executionContext = createExecutionContext(disableOrganizationAdminAccountRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DisableOrganizationAdminAccountRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(disableOrganizationAdminAccountRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DisableOrganizationAdminAccount");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DisableOrganizationAdminAccountResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Disassociates a member account from its Amazon Macie administrator account.
*
*
* @param disassociateFromAdministratorAccountRequest
* @return Result of the DisassociateFromAdministratorAccount operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.DisassociateFromAdministratorAccount
* @see AWS API Documentation
*/
@Override
public DisassociateFromAdministratorAccountResult disassociateFromAdministratorAccount(DisassociateFromAdministratorAccountRequest request) {
request = beforeClientExecution(request);
return executeDisassociateFromAdministratorAccount(request);
}
@SdkInternalApi
final DisassociateFromAdministratorAccountResult executeDisassociateFromAdministratorAccount(
DisassociateFromAdministratorAccountRequest disassociateFromAdministratorAccountRequest) {
ExecutionContext executionContext = createExecutionContext(disassociateFromAdministratorAccountRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DisassociateFromAdministratorAccountRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(disassociateFromAdministratorAccountRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DisassociateFromAdministratorAccount");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DisassociateFromAdministratorAccountResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* (Deprecated) Disassociates a member account from its Amazon Macie administrator account. This operation has been
* replaced by the DisassociateFromAdministratorAccount
* operation.
*
*
* @param disassociateFromMasterAccountRequest
* @return Result of the DisassociateFromMasterAccount operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.DisassociateFromMasterAccount
* @see AWS API Documentation
*/
@Override
public DisassociateFromMasterAccountResult disassociateFromMasterAccount(DisassociateFromMasterAccountRequest request) {
request = beforeClientExecution(request);
return executeDisassociateFromMasterAccount(request);
}
@SdkInternalApi
final DisassociateFromMasterAccountResult executeDisassociateFromMasterAccount(DisassociateFromMasterAccountRequest disassociateFromMasterAccountRequest) {
ExecutionContext executionContext = createExecutionContext(disassociateFromMasterAccountRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DisassociateFromMasterAccountRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(disassociateFromMasterAccountRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DisassociateFromMasterAccount");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DisassociateFromMasterAccountResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Disassociates an Amazon Macie administrator account from a member account.
*
*
* @param disassociateMemberRequest
* @return Result of the DisassociateMember operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.DisassociateMember
* @see AWS API
* Documentation
*/
@Override
public DisassociateMemberResult disassociateMember(DisassociateMemberRequest request) {
request = beforeClientExecution(request);
return executeDisassociateMember(request);
}
@SdkInternalApi
final DisassociateMemberResult executeDisassociateMember(DisassociateMemberRequest disassociateMemberRequest) {
ExecutionContext executionContext = createExecutionContext(disassociateMemberRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DisassociateMemberRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(disassociateMemberRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DisassociateMember");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DisassociateMemberResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Enables Amazon Macie and specifies the configuration settings for a Macie account.
*
*
* @param enableMacieRequest
* @return Result of the EnableMacie operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.EnableMacie
* @see AWS API
* Documentation
*/
@Override
public EnableMacieResult enableMacie(EnableMacieRequest request) {
request = beforeClientExecution(request);
return executeEnableMacie(request);
}
@SdkInternalApi
final EnableMacieResult executeEnableMacie(EnableMacieRequest enableMacieRequest) {
ExecutionContext executionContext = createExecutionContext(enableMacieRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new EnableMacieRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(enableMacieRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "EnableMacie");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new EnableMacieResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Designates an account as the delegated Amazon Macie administrator account for an organization in Organizations.
*
*
* @param enableOrganizationAdminAccountRequest
* @return Result of the EnableOrganizationAdminAccount operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.EnableOrganizationAdminAccount
* @see AWS API Documentation
*/
@Override
public EnableOrganizationAdminAccountResult enableOrganizationAdminAccount(EnableOrganizationAdminAccountRequest request) {
request = beforeClientExecution(request);
return executeEnableOrganizationAdminAccount(request);
}
@SdkInternalApi
final EnableOrganizationAdminAccountResult executeEnableOrganizationAdminAccount(EnableOrganizationAdminAccountRequest enableOrganizationAdminAccountRequest) {
ExecutionContext executionContext = createExecutionContext(enableOrganizationAdminAccountRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new EnableOrganizationAdminAccountRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(enableOrganizationAdminAccountRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "EnableOrganizationAdminAccount");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new EnableOrganizationAdminAccountResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves information about the Amazon Macie administrator account for an account.
*
*
* @param getAdministratorAccountRequest
* @return Result of the GetAdministratorAccount operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.GetAdministratorAccount
* @see AWS
* API Documentation
*/
@Override
public GetAdministratorAccountResult getAdministratorAccount(GetAdministratorAccountRequest request) {
request = beforeClientExecution(request);
return executeGetAdministratorAccount(request);
}
@SdkInternalApi
final GetAdministratorAccountResult executeGetAdministratorAccount(GetAdministratorAccountRequest getAdministratorAccountRequest) {
ExecutionContext executionContext = createExecutionContext(getAdministratorAccountRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetAdministratorAccountRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getAdministratorAccountRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetAdministratorAccount");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetAdministratorAccountResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the settings and status of an allow list.
*
*
* @param getAllowListRequest
* @return Result of the GetAllowList operation returned by the service.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @sample AmazonMacie2.GetAllowList
* @see AWS API
* Documentation
*/
@Override
public GetAllowListResult getAllowList(GetAllowListRequest request) {
request = beforeClientExecution(request);
return executeGetAllowList(request);
}
@SdkInternalApi
final GetAllowListResult executeGetAllowList(GetAllowListRequest getAllowListRequest) {
ExecutionContext executionContext = createExecutionContext(getAllowListRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetAllowListRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getAllowListRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetAllowList");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetAllowListResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the configuration settings and status of automated sensitive data discovery for an account.
*
*
* @param getAutomatedDiscoveryConfigurationRequest
* @return Result of the GetAutomatedDiscoveryConfiguration operation returned by the service.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @sample AmazonMacie2.GetAutomatedDiscoveryConfiguration
* @see AWS API Documentation
*/
@Override
public GetAutomatedDiscoveryConfigurationResult getAutomatedDiscoveryConfiguration(GetAutomatedDiscoveryConfigurationRequest request) {
request = beforeClientExecution(request);
return executeGetAutomatedDiscoveryConfiguration(request);
}
@SdkInternalApi
final GetAutomatedDiscoveryConfigurationResult executeGetAutomatedDiscoveryConfiguration(
GetAutomatedDiscoveryConfigurationRequest getAutomatedDiscoveryConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(getAutomatedDiscoveryConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetAutomatedDiscoveryConfigurationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getAutomatedDiscoveryConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetAutomatedDiscoveryConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetAutomatedDiscoveryConfigurationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves (queries) aggregated statistical data about all the S3 buckets that Amazon Macie monitors and analyzes
* for an account.
*
*
* @param getBucketStatisticsRequest
* @return Result of the GetBucketStatistics operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.GetBucketStatistics
* @see AWS API
* Documentation
*/
@Override
public GetBucketStatisticsResult getBucketStatistics(GetBucketStatisticsRequest request) {
request = beforeClientExecution(request);
return executeGetBucketStatistics(request);
}
@SdkInternalApi
final GetBucketStatisticsResult executeGetBucketStatistics(GetBucketStatisticsRequest getBucketStatisticsRequest) {
ExecutionContext executionContext = createExecutionContext(getBucketStatisticsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetBucketStatisticsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getBucketStatisticsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetBucketStatistics");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetBucketStatisticsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the configuration settings for storing data classification results.
*
*
* @param getClassificationExportConfigurationRequest
* @return Result of the GetClassificationExportConfiguration operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.GetClassificationExportConfiguration
* @see AWS API Documentation
*/
@Override
public GetClassificationExportConfigurationResult getClassificationExportConfiguration(GetClassificationExportConfigurationRequest request) {
request = beforeClientExecution(request);
return executeGetClassificationExportConfiguration(request);
}
@SdkInternalApi
final GetClassificationExportConfigurationResult executeGetClassificationExportConfiguration(
GetClassificationExportConfigurationRequest getClassificationExportConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(getClassificationExportConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetClassificationExportConfigurationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getClassificationExportConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetClassificationExportConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetClassificationExportConfigurationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the classification scope settings for an account.
*
*
* @param getClassificationScopeRequest
* @return Result of the GetClassificationScope operation returned by the service.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @sample AmazonMacie2.GetClassificationScope
* @see AWS
* API Documentation
*/
@Override
public GetClassificationScopeResult getClassificationScope(GetClassificationScopeRequest request) {
request = beforeClientExecution(request);
return executeGetClassificationScope(request);
}
@SdkInternalApi
final GetClassificationScopeResult executeGetClassificationScope(GetClassificationScopeRequest getClassificationScopeRequest) {
ExecutionContext executionContext = createExecutionContext(getClassificationScopeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetClassificationScopeRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getClassificationScopeRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetClassificationScope");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetClassificationScopeResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the criteria and other settings for a custom data identifier.
*
*
* @param getCustomDataIdentifierRequest
* @return Result of the GetCustomDataIdentifier operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.GetCustomDataIdentifier
* @see AWS
* API Documentation
*/
@Override
public GetCustomDataIdentifierResult getCustomDataIdentifier(GetCustomDataIdentifierRequest request) {
request = beforeClientExecution(request);
return executeGetCustomDataIdentifier(request);
}
@SdkInternalApi
final GetCustomDataIdentifierResult executeGetCustomDataIdentifier(GetCustomDataIdentifierRequest getCustomDataIdentifierRequest) {
ExecutionContext executionContext = createExecutionContext(getCustomDataIdentifierRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetCustomDataIdentifierRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getCustomDataIdentifierRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetCustomDataIdentifier");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetCustomDataIdentifierResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves (queries) aggregated statistical data about findings.
*
*
* @param getFindingStatisticsRequest
* @return Result of the GetFindingStatistics operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.GetFindingStatistics
* @see AWS
* API Documentation
*/
@Override
public GetFindingStatisticsResult getFindingStatistics(GetFindingStatisticsRequest request) {
request = beforeClientExecution(request);
return executeGetFindingStatistics(request);
}
@SdkInternalApi
final GetFindingStatisticsResult executeGetFindingStatistics(GetFindingStatisticsRequest getFindingStatisticsRequest) {
ExecutionContext executionContext = createExecutionContext(getFindingStatisticsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetFindingStatisticsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getFindingStatisticsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetFindingStatistics");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetFindingStatisticsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the details of one or more findings.
*
*
* @param getFindingsRequest
* @return Result of the GetFindings operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.GetFindings
* @see AWS API
* Documentation
*/
@Override
public GetFindingsResult getFindings(GetFindingsRequest request) {
request = beforeClientExecution(request);
return executeGetFindings(request);
}
@SdkInternalApi
final GetFindingsResult executeGetFindings(GetFindingsRequest getFindingsRequest) {
ExecutionContext executionContext = createExecutionContext(getFindingsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetFindingsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getFindingsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetFindings");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetFindingsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the criteria and other settings for a findings filter.
*
*
* @param getFindingsFilterRequest
* @return Result of the GetFindingsFilter operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.GetFindingsFilter
* @see AWS API
* Documentation
*/
@Override
public GetFindingsFilterResult getFindingsFilter(GetFindingsFilterRequest request) {
request = beforeClientExecution(request);
return executeGetFindingsFilter(request);
}
@SdkInternalApi
final GetFindingsFilterResult executeGetFindingsFilter(GetFindingsFilterRequest getFindingsFilterRequest) {
ExecutionContext executionContext = createExecutionContext(getFindingsFilterRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetFindingsFilterRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getFindingsFilterRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetFindingsFilter");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetFindingsFilterResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the configuration settings for publishing findings to Security Hub.
*
*
* @param getFindingsPublicationConfigurationRequest
* @return Result of the GetFindingsPublicationConfiguration operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.GetFindingsPublicationConfiguration
* @see AWS API Documentation
*/
@Override
public GetFindingsPublicationConfigurationResult getFindingsPublicationConfiguration(GetFindingsPublicationConfigurationRequest request) {
request = beforeClientExecution(request);
return executeGetFindingsPublicationConfiguration(request);
}
@SdkInternalApi
final GetFindingsPublicationConfigurationResult executeGetFindingsPublicationConfiguration(
GetFindingsPublicationConfigurationRequest getFindingsPublicationConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(getFindingsPublicationConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetFindingsPublicationConfigurationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getFindingsPublicationConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetFindingsPublicationConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetFindingsPublicationConfigurationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the count of Amazon Macie membership invitations that were received by an account.
*
*
* @param getInvitationsCountRequest
* @return Result of the GetInvitationsCount operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.GetInvitationsCount
* @see AWS API
* Documentation
*/
@Override
public GetInvitationsCountResult getInvitationsCount(GetInvitationsCountRequest request) {
request = beforeClientExecution(request);
return executeGetInvitationsCount(request);
}
@SdkInternalApi
final GetInvitationsCountResult executeGetInvitationsCount(GetInvitationsCountRequest getInvitationsCountRequest) {
ExecutionContext executionContext = createExecutionContext(getInvitationsCountRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetInvitationsCountRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getInvitationsCountRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetInvitationsCount");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetInvitationsCountResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the status and configuration settings for an Amazon Macie account.
*
*
* @param getMacieSessionRequest
* @return Result of the GetMacieSession operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.GetMacieSession
* @see AWS API
* Documentation
*/
@Override
public GetMacieSessionResult getMacieSession(GetMacieSessionRequest request) {
request = beforeClientExecution(request);
return executeGetMacieSession(request);
}
@SdkInternalApi
final GetMacieSessionResult executeGetMacieSession(GetMacieSessionRequest getMacieSessionRequest) {
ExecutionContext executionContext = createExecutionContext(getMacieSessionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetMacieSessionRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getMacieSessionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetMacieSession");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetMacieSessionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* (Deprecated) Retrieves information about the Amazon Macie administrator account for an account. This operation
* has been replaced by the GetAdministratorAccount operation.
*
*
* @param getMasterAccountRequest
* @return Result of the GetMasterAccount operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.GetMasterAccount
* @see AWS API
* Documentation
*/
@Override
public GetMasterAccountResult getMasterAccount(GetMasterAccountRequest request) {
request = beforeClientExecution(request);
return executeGetMasterAccount(request);
}
@SdkInternalApi
final GetMasterAccountResult executeGetMasterAccount(GetMasterAccountRequest getMasterAccountRequest) {
ExecutionContext executionContext = createExecutionContext(getMasterAccountRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetMasterAccountRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getMasterAccountRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetMasterAccount");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetMasterAccountResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves information about an account that's associated with an Amazon Macie administrator account.
*
*
* @param getMemberRequest
* @return Result of the GetMember operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.GetMember
* @see AWS API
* Documentation
*/
@Override
public GetMemberResult getMember(GetMemberRequest request) {
request = beforeClientExecution(request);
return executeGetMember(request);
}
@SdkInternalApi
final GetMemberResult executeGetMember(GetMemberRequest getMemberRequest) {
ExecutionContext executionContext = createExecutionContext(getMemberRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetMemberRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getMemberRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetMember");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetMemberResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves (queries) sensitive data discovery statistics and the sensitivity score for an S3 bucket.
*
*
* @param getResourceProfileRequest
* @return Result of the GetResourceProfile operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @sample AmazonMacie2.GetResourceProfile
* @see AWS API
* Documentation
*/
@Override
public GetResourceProfileResult getResourceProfile(GetResourceProfileRequest request) {
request = beforeClientExecution(request);
return executeGetResourceProfile(request);
}
@SdkInternalApi
final GetResourceProfileResult executeGetResourceProfile(GetResourceProfileRequest getResourceProfileRequest) {
ExecutionContext executionContext = createExecutionContext(getResourceProfileRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetResourceProfileRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getResourceProfileRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetResourceProfile");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetResourceProfileResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the status and configuration settings for retrieving occurrences of sensitive data reported by
* findings.
*
*
* @param getRevealConfigurationRequest
* @return Result of the GetRevealConfiguration operation returned by the service.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @sample AmazonMacie2.GetRevealConfiguration
* @see AWS
* API Documentation
*/
@Override
public GetRevealConfigurationResult getRevealConfiguration(GetRevealConfigurationRequest request) {
request = beforeClientExecution(request);
return executeGetRevealConfiguration(request);
}
@SdkInternalApi
final GetRevealConfigurationResult executeGetRevealConfiguration(GetRevealConfigurationRequest getRevealConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(getRevealConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetRevealConfigurationRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getRevealConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetRevealConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetRevealConfigurationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves occurrences of sensitive data reported by a finding.
*
*
* @param getSensitiveDataOccurrencesRequest
* @return Result of the GetSensitiveDataOccurrences operation returned by the service.
* @throws UnprocessableEntityException
* The request failed because it contains instructions that Amazon Macie can't process (Unprocessable
* Entity).
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @sample AmazonMacie2.GetSensitiveDataOccurrences
* @see AWS API Documentation
*/
@Override
public GetSensitiveDataOccurrencesResult getSensitiveDataOccurrences(GetSensitiveDataOccurrencesRequest request) {
request = beforeClientExecution(request);
return executeGetSensitiveDataOccurrences(request);
}
@SdkInternalApi
final GetSensitiveDataOccurrencesResult executeGetSensitiveDataOccurrences(GetSensitiveDataOccurrencesRequest getSensitiveDataOccurrencesRequest) {
ExecutionContext executionContext = createExecutionContext(getSensitiveDataOccurrencesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetSensitiveDataOccurrencesRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getSensitiveDataOccurrencesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetSensitiveDataOccurrences");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetSensitiveDataOccurrencesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Checks whether occurrences of sensitive data can be retrieved for a finding.
*
*
* @param getSensitiveDataOccurrencesAvailabilityRequest
* @return Result of the GetSensitiveDataOccurrencesAvailability operation returned by the service.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @sample AmazonMacie2.GetSensitiveDataOccurrencesAvailability
* @see AWS API Documentation
*/
@Override
public GetSensitiveDataOccurrencesAvailabilityResult getSensitiveDataOccurrencesAvailability(GetSensitiveDataOccurrencesAvailabilityRequest request) {
request = beforeClientExecution(request);
return executeGetSensitiveDataOccurrencesAvailability(request);
}
@SdkInternalApi
final GetSensitiveDataOccurrencesAvailabilityResult executeGetSensitiveDataOccurrencesAvailability(
GetSensitiveDataOccurrencesAvailabilityRequest getSensitiveDataOccurrencesAvailabilityRequest) {
ExecutionContext executionContext = createExecutionContext(getSensitiveDataOccurrencesAvailabilityRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetSensitiveDataOccurrencesAvailabilityRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getSensitiveDataOccurrencesAvailabilityRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetSensitiveDataOccurrencesAvailability");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetSensitiveDataOccurrencesAvailabilityResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the settings for the sensitivity inspection template for an account.
*
*
* @param getSensitivityInspectionTemplateRequest
* @return Result of the GetSensitivityInspectionTemplate operation returned by the service.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @sample AmazonMacie2.GetSensitivityInspectionTemplate
* @see AWS API Documentation
*/
@Override
public GetSensitivityInspectionTemplateResult getSensitivityInspectionTemplate(GetSensitivityInspectionTemplateRequest request) {
request = beforeClientExecution(request);
return executeGetSensitivityInspectionTemplate(request);
}
@SdkInternalApi
final GetSensitivityInspectionTemplateResult executeGetSensitivityInspectionTemplate(
GetSensitivityInspectionTemplateRequest getSensitivityInspectionTemplateRequest) {
ExecutionContext executionContext = createExecutionContext(getSensitivityInspectionTemplateRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetSensitivityInspectionTemplateRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getSensitivityInspectionTemplateRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetSensitivityInspectionTemplate");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetSensitivityInspectionTemplateResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves (queries) quotas and aggregated usage data for one or more accounts.
*
*
* @param getUsageStatisticsRequest
* @return Result of the GetUsageStatistics operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.GetUsageStatistics
* @see AWS API
* Documentation
*/
@Override
public GetUsageStatisticsResult getUsageStatistics(GetUsageStatisticsRequest request) {
request = beforeClientExecution(request);
return executeGetUsageStatistics(request);
}
@SdkInternalApi
final GetUsageStatisticsResult executeGetUsageStatistics(GetUsageStatisticsRequest getUsageStatisticsRequest) {
ExecutionContext executionContext = createExecutionContext(getUsageStatisticsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetUsageStatisticsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getUsageStatisticsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetUsageStatistics");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetUsageStatisticsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves (queries) aggregated usage data for an account.
*
*
* @param getUsageTotalsRequest
* @return Result of the GetUsageTotals operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.GetUsageTotals
* @see AWS API
* Documentation
*/
@Override
public GetUsageTotalsResult getUsageTotals(GetUsageTotalsRequest request) {
request = beforeClientExecution(request);
return executeGetUsageTotals(request);
}
@SdkInternalApi
final GetUsageTotalsResult executeGetUsageTotals(GetUsageTotalsRequest getUsageTotalsRequest) {
ExecutionContext executionContext = createExecutionContext(getUsageTotalsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetUsageTotalsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getUsageTotalsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetUsageTotals");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetUsageTotalsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves a subset of information about all the allow lists for an account.
*
*
* @param listAllowListsRequest
* @return Result of the ListAllowLists operation returned by the service.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @sample AmazonMacie2.ListAllowLists
* @see AWS API
* Documentation
*/
@Override
public ListAllowListsResult listAllowLists(ListAllowListsRequest request) {
request = beforeClientExecution(request);
return executeListAllowLists(request);
}
@SdkInternalApi
final ListAllowListsResult executeListAllowLists(ListAllowListsRequest listAllowListsRequest) {
ExecutionContext executionContext = createExecutionContext(listAllowListsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListAllowListsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listAllowListsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListAllowLists");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListAllowListsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves a subset of information about one or more classification jobs.
*
*
* @param listClassificationJobsRequest
* @return Result of the ListClassificationJobs operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.ListClassificationJobs
* @see AWS
* API Documentation
*/
@Override
public ListClassificationJobsResult listClassificationJobs(ListClassificationJobsRequest request) {
request = beforeClientExecution(request);
return executeListClassificationJobs(request);
}
@SdkInternalApi
final ListClassificationJobsResult executeListClassificationJobs(ListClassificationJobsRequest listClassificationJobsRequest) {
ExecutionContext executionContext = createExecutionContext(listClassificationJobsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListClassificationJobsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listClassificationJobsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListClassificationJobs");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListClassificationJobsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves a subset of information about the classification scope for an account.
*
*
* @param listClassificationScopesRequest
* @return Result of the ListClassificationScopes operation returned by the service.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @sample AmazonMacie2.ListClassificationScopes
* @see AWS API Documentation
*/
@Override
public ListClassificationScopesResult listClassificationScopes(ListClassificationScopesRequest request) {
request = beforeClientExecution(request);
return executeListClassificationScopes(request);
}
@SdkInternalApi
final ListClassificationScopesResult executeListClassificationScopes(ListClassificationScopesRequest listClassificationScopesRequest) {
ExecutionContext executionContext = createExecutionContext(listClassificationScopesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListClassificationScopesRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listClassificationScopesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListClassificationScopes");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListClassificationScopesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves a subset of information about all the custom data identifiers for an account.
*
*
* @param listCustomDataIdentifiersRequest
* @return Result of the ListCustomDataIdentifiers operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.ListCustomDataIdentifiers
* @see AWS API Documentation
*/
@Override
public ListCustomDataIdentifiersResult listCustomDataIdentifiers(ListCustomDataIdentifiersRequest request) {
request = beforeClientExecution(request);
return executeListCustomDataIdentifiers(request);
}
@SdkInternalApi
final ListCustomDataIdentifiersResult executeListCustomDataIdentifiers(ListCustomDataIdentifiersRequest listCustomDataIdentifiersRequest) {
ExecutionContext executionContext = createExecutionContext(listCustomDataIdentifiersRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListCustomDataIdentifiersRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listCustomDataIdentifiersRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListCustomDataIdentifiers");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListCustomDataIdentifiersResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves a subset of information about one or more findings.
*
*
* @param listFindingsRequest
* @return Result of the ListFindings operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.ListFindings
* @see AWS API
* Documentation
*/
@Override
public ListFindingsResult listFindings(ListFindingsRequest request) {
request = beforeClientExecution(request);
return executeListFindings(request);
}
@SdkInternalApi
final ListFindingsResult executeListFindings(ListFindingsRequest listFindingsRequest) {
ExecutionContext executionContext = createExecutionContext(listFindingsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListFindingsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listFindingsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListFindings");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListFindingsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves a subset of information about all the findings filters for an account.
*
*
* @param listFindingsFiltersRequest
* @return Result of the ListFindingsFilters operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.ListFindingsFilters
* @see AWS API
* Documentation
*/
@Override
public ListFindingsFiltersResult listFindingsFilters(ListFindingsFiltersRequest request) {
request = beforeClientExecution(request);
return executeListFindingsFilters(request);
}
@SdkInternalApi
final ListFindingsFiltersResult executeListFindingsFilters(ListFindingsFiltersRequest listFindingsFiltersRequest) {
ExecutionContext executionContext = createExecutionContext(listFindingsFiltersRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListFindingsFiltersRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listFindingsFiltersRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListFindingsFilters");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListFindingsFiltersResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves information about the Amazon Macie membership invitations that were received by an account.
*
*
* @param listInvitationsRequest
* @return Result of the ListInvitations operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.ListInvitations
* @see AWS API
* Documentation
*/
@Override
public ListInvitationsResult listInvitations(ListInvitationsRequest request) {
request = beforeClientExecution(request);
return executeListInvitations(request);
}
@SdkInternalApi
final ListInvitationsResult executeListInvitations(ListInvitationsRequest listInvitationsRequest) {
ExecutionContext executionContext = createExecutionContext(listInvitationsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListInvitationsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listInvitationsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListInvitations");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListInvitationsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves information about all the managed data identifiers that Amazon Macie currently provides.
*
*
* @param listManagedDataIdentifiersRequest
* @return Result of the ListManagedDataIdentifiers operation returned by the service.
* @sample AmazonMacie2.ListManagedDataIdentifiers
* @see AWS API Documentation
*/
@Override
public ListManagedDataIdentifiersResult listManagedDataIdentifiers(ListManagedDataIdentifiersRequest request) {
request = beforeClientExecution(request);
return executeListManagedDataIdentifiers(request);
}
@SdkInternalApi
final ListManagedDataIdentifiersResult executeListManagedDataIdentifiers(ListManagedDataIdentifiersRequest listManagedDataIdentifiersRequest) {
ExecutionContext executionContext = createExecutionContext(listManagedDataIdentifiersRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListManagedDataIdentifiersRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listManagedDataIdentifiersRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListManagedDataIdentifiers");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListManagedDataIdentifiersResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves information about the accounts that are associated with an Amazon Macie administrator account.
*
*
* @param listMembersRequest
* @return Result of the ListMembers operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.ListMembers
* @see AWS API
* Documentation
*/
@Override
public ListMembersResult listMembers(ListMembersRequest request) {
request = beforeClientExecution(request);
return executeListMembers(request);
}
@SdkInternalApi
final ListMembersResult executeListMembers(ListMembersRequest listMembersRequest) {
ExecutionContext executionContext = createExecutionContext(listMembersRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListMembersRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listMembersRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListMembers");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListMembersResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves information about the delegated Amazon Macie administrator account for an organization in
* Organizations.
*
*
* @param listOrganizationAdminAccountsRequest
* @return Result of the ListOrganizationAdminAccounts operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.ListOrganizationAdminAccounts
* @see AWS API Documentation
*/
@Override
public ListOrganizationAdminAccountsResult listOrganizationAdminAccounts(ListOrganizationAdminAccountsRequest request) {
request = beforeClientExecution(request);
return executeListOrganizationAdminAccounts(request);
}
@SdkInternalApi
final ListOrganizationAdminAccountsResult executeListOrganizationAdminAccounts(ListOrganizationAdminAccountsRequest listOrganizationAdminAccountsRequest) {
ExecutionContext executionContext = createExecutionContext(listOrganizationAdminAccountsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListOrganizationAdminAccountsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listOrganizationAdminAccountsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListOrganizationAdminAccounts");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListOrganizationAdminAccountsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves information about objects that were selected from an S3 bucket for automated sensitive data discovery.
*
*
* @param listResourceProfileArtifactsRequest
* @return Result of the ListResourceProfileArtifacts operation returned by the service.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @sample AmazonMacie2.ListResourceProfileArtifacts
* @see AWS API Documentation
*/
@Override
public ListResourceProfileArtifactsResult listResourceProfileArtifacts(ListResourceProfileArtifactsRequest request) {
request = beforeClientExecution(request);
return executeListResourceProfileArtifacts(request);
}
@SdkInternalApi
final ListResourceProfileArtifactsResult executeListResourceProfileArtifacts(ListResourceProfileArtifactsRequest listResourceProfileArtifactsRequest) {
ExecutionContext executionContext = createExecutionContext(listResourceProfileArtifactsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListResourceProfileArtifactsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listResourceProfileArtifactsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListResourceProfileArtifacts");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListResourceProfileArtifactsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves information about the types and amount of sensitive data that Amazon Macie found in an S3 bucket.
*
*
* @param listResourceProfileDetectionsRequest
* @return Result of the ListResourceProfileDetections operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @sample AmazonMacie2.ListResourceProfileDetections
* @see AWS API Documentation
*/
@Override
public ListResourceProfileDetectionsResult listResourceProfileDetections(ListResourceProfileDetectionsRequest request) {
request = beforeClientExecution(request);
return executeListResourceProfileDetections(request);
}
@SdkInternalApi
final ListResourceProfileDetectionsResult executeListResourceProfileDetections(ListResourceProfileDetectionsRequest listResourceProfileDetectionsRequest) {
ExecutionContext executionContext = createExecutionContext(listResourceProfileDetectionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListResourceProfileDetectionsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listResourceProfileDetectionsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListResourceProfileDetections");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListResourceProfileDetectionsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves a subset of information about the sensitivity inspection template for an account.
*
*
* @param listSensitivityInspectionTemplatesRequest
* @return Result of the ListSensitivityInspectionTemplates operation returned by the service.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @sample AmazonMacie2.ListSensitivityInspectionTemplates
* @see AWS API Documentation
*/
@Override
public ListSensitivityInspectionTemplatesResult listSensitivityInspectionTemplates(ListSensitivityInspectionTemplatesRequest request) {
request = beforeClientExecution(request);
return executeListSensitivityInspectionTemplates(request);
}
@SdkInternalApi
final ListSensitivityInspectionTemplatesResult executeListSensitivityInspectionTemplates(
ListSensitivityInspectionTemplatesRequest listSensitivityInspectionTemplatesRequest) {
ExecutionContext executionContext = createExecutionContext(listSensitivityInspectionTemplatesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListSensitivityInspectionTemplatesRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listSensitivityInspectionTemplatesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListSensitivityInspectionTemplates");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListSensitivityInspectionTemplatesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the tags (keys and values) that are associated with an Amazon Macie resource.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @sample AmazonMacie2.ListTagsForResource
* @see AWS API
* Documentation
*/
@Override
public ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest request) {
request = beforeClientExecution(request);
return executeListTagsForResource(request);
}
@SdkInternalApi
final ListTagsForResourceResult executeListTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest) {
ExecutionContext executionContext = createExecutionContext(listTagsForResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListTagsForResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listTagsForResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListTagsForResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListTagsForResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates or updates the configuration settings for storing data classification results.
*
*
* @param putClassificationExportConfigurationRequest
* @return Result of the PutClassificationExportConfiguration operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.PutClassificationExportConfiguration
* @see AWS API Documentation
*/
@Override
public PutClassificationExportConfigurationResult putClassificationExportConfiguration(PutClassificationExportConfigurationRequest request) {
request = beforeClientExecution(request);
return executePutClassificationExportConfiguration(request);
}
@SdkInternalApi
final PutClassificationExportConfigurationResult executePutClassificationExportConfiguration(
PutClassificationExportConfigurationRequest putClassificationExportConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(putClassificationExportConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new PutClassificationExportConfigurationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(putClassificationExportConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "PutClassificationExportConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new PutClassificationExportConfigurationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates the configuration settings for publishing findings to Security Hub.
*
*
* @param putFindingsPublicationConfigurationRequest
* @return Result of the PutFindingsPublicationConfiguration operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.PutFindingsPublicationConfiguration
* @see AWS API Documentation
*/
@Override
public PutFindingsPublicationConfigurationResult putFindingsPublicationConfiguration(PutFindingsPublicationConfigurationRequest request) {
request = beforeClientExecution(request);
return executePutFindingsPublicationConfiguration(request);
}
@SdkInternalApi
final PutFindingsPublicationConfigurationResult executePutFindingsPublicationConfiguration(
PutFindingsPublicationConfigurationRequest putFindingsPublicationConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(putFindingsPublicationConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new PutFindingsPublicationConfigurationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(putFindingsPublicationConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "PutFindingsPublicationConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new PutFindingsPublicationConfigurationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves (queries) statistical data and other information about Amazon Web Services resources that Amazon Macie
* monitors and analyzes.
*
*
* @param searchResourcesRequest
* @return Result of the SearchResources operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.SearchResources
* @see AWS API
* Documentation
*/
@Override
public SearchResourcesResult searchResources(SearchResourcesRequest request) {
request = beforeClientExecution(request);
return executeSearchResources(request);
}
@SdkInternalApi
final SearchResourcesResult executeSearchResources(SearchResourcesRequest searchResourcesRequest) {
ExecutionContext executionContext = createExecutionContext(searchResourcesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new SearchResourcesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(searchResourcesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "SearchResources");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new SearchResourcesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Adds or updates one or more tags (keys and values) that are associated with an Amazon Macie resource.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @sample AmazonMacie2.TagResource
* @see AWS API
* Documentation
*/
@Override
public TagResourceResult tagResource(TagResourceRequest request) {
request = beforeClientExecution(request);
return executeTagResource(request);
}
@SdkInternalApi
final TagResourceResult executeTagResource(TagResourceRequest tagResourceRequest) {
ExecutionContext executionContext = createExecutionContext(tagResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new TagResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(tagResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "TagResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new TagResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Tests a custom data identifier.
*
*
* @param testCustomDataIdentifierRequest
* @return Result of the TestCustomDataIdentifier operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.TestCustomDataIdentifier
* @see AWS API Documentation
*/
@Override
public TestCustomDataIdentifierResult testCustomDataIdentifier(TestCustomDataIdentifierRequest request) {
request = beforeClientExecution(request);
return executeTestCustomDataIdentifier(request);
}
@SdkInternalApi
final TestCustomDataIdentifierResult executeTestCustomDataIdentifier(TestCustomDataIdentifierRequest testCustomDataIdentifierRequest) {
ExecutionContext executionContext = createExecutionContext(testCustomDataIdentifierRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new TestCustomDataIdentifierRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(testCustomDataIdentifierRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "TestCustomDataIdentifier");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new TestCustomDataIdentifierResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Removes one or more tags (keys and values) from an Amazon Macie resource.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @sample AmazonMacie2.UntagResource
* @see AWS API
* Documentation
*/
@Override
public UntagResourceResult untagResource(UntagResourceRequest request) {
request = beforeClientExecution(request);
return executeUntagResource(request);
}
@SdkInternalApi
final UntagResourceResult executeUntagResource(UntagResourceRequest untagResourceRequest) {
ExecutionContext executionContext = createExecutionContext(untagResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UntagResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(untagResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UntagResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UntagResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates the settings for an allow list.
*
*
* @param updateAllowListRequest
* @return Result of the UpdateAllowList operation returned by the service.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @sample AmazonMacie2.UpdateAllowList
* @see AWS API
* Documentation
*/
@Override
public UpdateAllowListResult updateAllowList(UpdateAllowListRequest request) {
request = beforeClientExecution(request);
return executeUpdateAllowList(request);
}
@SdkInternalApi
final UpdateAllowListResult executeUpdateAllowList(UpdateAllowListRequest updateAllowListRequest) {
ExecutionContext executionContext = createExecutionContext(updateAllowListRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateAllowListRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateAllowListRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateAllowList");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateAllowListResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Enables or disables automated sensitive data discovery for an account.
*
*
* @param updateAutomatedDiscoveryConfigurationRequest
* @return Result of the UpdateAutomatedDiscoveryConfiguration operation returned by the service.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @sample AmazonMacie2.UpdateAutomatedDiscoveryConfiguration
* @see AWS API Documentation
*/
@Override
public UpdateAutomatedDiscoveryConfigurationResult updateAutomatedDiscoveryConfiguration(UpdateAutomatedDiscoveryConfigurationRequest request) {
request = beforeClientExecution(request);
return executeUpdateAutomatedDiscoveryConfiguration(request);
}
@SdkInternalApi
final UpdateAutomatedDiscoveryConfigurationResult executeUpdateAutomatedDiscoveryConfiguration(
UpdateAutomatedDiscoveryConfigurationRequest updateAutomatedDiscoveryConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(updateAutomatedDiscoveryConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateAutomatedDiscoveryConfigurationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(updateAutomatedDiscoveryConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateAutomatedDiscoveryConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new UpdateAutomatedDiscoveryConfigurationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Changes the status of a classification job.
*
*
* @param updateClassificationJobRequest
* @return Result of the UpdateClassificationJob operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.UpdateClassificationJob
* @see AWS
* API Documentation
*/
@Override
public UpdateClassificationJobResult updateClassificationJob(UpdateClassificationJobRequest request) {
request = beforeClientExecution(request);
return executeUpdateClassificationJob(request);
}
@SdkInternalApi
final UpdateClassificationJobResult executeUpdateClassificationJob(UpdateClassificationJobRequest updateClassificationJobRequest) {
ExecutionContext executionContext = createExecutionContext(updateClassificationJobRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateClassificationJobRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(updateClassificationJobRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateClassificationJob");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new UpdateClassificationJobResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates the classification scope settings for an account.
*
*
* @param updateClassificationScopeRequest
* @return Result of the UpdateClassificationScope operation returned by the service.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @sample AmazonMacie2.UpdateClassificationScope
* @see AWS API Documentation
*/
@Override
public UpdateClassificationScopeResult updateClassificationScope(UpdateClassificationScopeRequest request) {
request = beforeClientExecution(request);
return executeUpdateClassificationScope(request);
}
@SdkInternalApi
final UpdateClassificationScopeResult executeUpdateClassificationScope(UpdateClassificationScopeRequest updateClassificationScopeRequest) {
ExecutionContext executionContext = createExecutionContext(updateClassificationScopeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateClassificationScopeRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(updateClassificationScopeRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateClassificationScope");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new UpdateClassificationScopeResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates the criteria and other settings for a findings filter.
*
*
* @param updateFindingsFilterRequest
* @return Result of the UpdateFindingsFilter operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.UpdateFindingsFilter
* @see AWS
* API Documentation
*/
@Override
public UpdateFindingsFilterResult updateFindingsFilter(UpdateFindingsFilterRequest request) {
request = beforeClientExecution(request);
return executeUpdateFindingsFilter(request);
}
@SdkInternalApi
final UpdateFindingsFilterResult executeUpdateFindingsFilter(UpdateFindingsFilterRequest updateFindingsFilterRequest) {
ExecutionContext executionContext = createExecutionContext(updateFindingsFilterRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateFindingsFilterRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateFindingsFilterRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateFindingsFilter");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateFindingsFilterResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Suspends or re-enables Amazon Macie, or updates the configuration settings for a Macie account.
*
*
* @param updateMacieSessionRequest
* @return Result of the UpdateMacieSession operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.UpdateMacieSession
* @see AWS API
* Documentation
*/
@Override
public UpdateMacieSessionResult updateMacieSession(UpdateMacieSessionRequest request) {
request = beforeClientExecution(request);
return executeUpdateMacieSession(request);
}
@SdkInternalApi
final UpdateMacieSessionResult executeUpdateMacieSession(UpdateMacieSessionRequest updateMacieSessionRequest) {
ExecutionContext executionContext = createExecutionContext(updateMacieSessionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateMacieSessionRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateMacieSessionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateMacieSession");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateMacieSessionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Enables an Amazon Macie administrator to suspend or re-enable Macie for a member account.
*
*
* @param updateMemberSessionRequest
* @return Result of the UpdateMemberSession operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.UpdateMemberSession
* @see AWS API
* Documentation
*/
@Override
public UpdateMemberSessionResult updateMemberSession(UpdateMemberSessionRequest request) {
request = beforeClientExecution(request);
return executeUpdateMemberSession(request);
}
@SdkInternalApi
final UpdateMemberSessionResult executeUpdateMemberSession(UpdateMemberSessionRequest updateMemberSessionRequest) {
ExecutionContext executionContext = createExecutionContext(updateMemberSessionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateMemberSessionRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateMemberSessionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateMemberSession");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateMemberSessionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates the Amazon Macie configuration settings for an organization in Organizations.
*
*
* @param updateOrganizationConfigurationRequest
* @return Result of the UpdateOrganizationConfiguration operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.UpdateOrganizationConfiguration
* @see AWS API Documentation
*/
@Override
public UpdateOrganizationConfigurationResult updateOrganizationConfiguration(UpdateOrganizationConfigurationRequest request) {
request = beforeClientExecution(request);
return executeUpdateOrganizationConfiguration(request);
}
@SdkInternalApi
final UpdateOrganizationConfigurationResult executeUpdateOrganizationConfiguration(
UpdateOrganizationConfigurationRequest updateOrganizationConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(updateOrganizationConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateOrganizationConfigurationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(updateOrganizationConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateOrganizationConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new UpdateOrganizationConfigurationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates the sensitivity score for an S3 bucket.
*
*
* @param updateResourceProfileRequest
* @return Result of the UpdateResourceProfile operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @sample AmazonMacie2.UpdateResourceProfile
* @see AWS
* API Documentation
*/
@Override
public UpdateResourceProfileResult updateResourceProfile(UpdateResourceProfileRequest request) {
request = beforeClientExecution(request);
return executeUpdateResourceProfile(request);
}
@SdkInternalApi
final UpdateResourceProfileResult executeUpdateResourceProfile(UpdateResourceProfileRequest updateResourceProfileRequest) {
ExecutionContext executionContext = createExecutionContext(updateResourceProfileRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateResourceProfileRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateResourceProfileRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateResourceProfile");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new UpdateResourceProfileResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates the sensitivity scoring settings for an S3 bucket.
*
*
* @param updateResourceProfileDetectionsRequest
* @return Result of the UpdateResourceProfileDetections operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @sample AmazonMacie2.UpdateResourceProfileDetections
* @see AWS API Documentation
*/
@Override
public UpdateResourceProfileDetectionsResult updateResourceProfileDetections(UpdateResourceProfileDetectionsRequest request) {
request = beforeClientExecution(request);
return executeUpdateResourceProfileDetections(request);
}
@SdkInternalApi
final UpdateResourceProfileDetectionsResult executeUpdateResourceProfileDetections(
UpdateResourceProfileDetectionsRequest updateResourceProfileDetectionsRequest) {
ExecutionContext executionContext = createExecutionContext(updateResourceProfileDetectionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateResourceProfileDetectionsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(updateResourceProfileDetectionsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateResourceProfileDetections");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new UpdateResourceProfileDetectionsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates the status and configuration settings for retrieving occurrences of sensitive data reported by findings.
*
*
* @param updateRevealConfigurationRequest
* @return Result of the UpdateRevealConfiguration operation returned by the service.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @sample AmazonMacie2.UpdateRevealConfiguration
* @see AWS API Documentation
*/
@Override
public UpdateRevealConfigurationResult updateRevealConfiguration(UpdateRevealConfigurationRequest request) {
request = beforeClientExecution(request);
return executeUpdateRevealConfiguration(request);
}
@SdkInternalApi
final UpdateRevealConfigurationResult executeUpdateRevealConfiguration(UpdateRevealConfigurationRequest updateRevealConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(updateRevealConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateRevealConfigurationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(updateRevealConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateRevealConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new UpdateRevealConfigurationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates the settings for the sensitivity inspection template for an account.
*
*
* @param updateSensitivityInspectionTemplateRequest
* @return Result of the UpdateSensitivityInspectionTemplate operation returned by the service.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @sample AmazonMacie2.UpdateSensitivityInspectionTemplate
* @see AWS API Documentation
*/
@Override
public UpdateSensitivityInspectionTemplateResult updateSensitivityInspectionTemplate(UpdateSensitivityInspectionTemplateRequest request) {
request = beforeClientExecution(request);
return executeUpdateSensitivityInspectionTemplate(request);
}
@SdkInternalApi
final UpdateSensitivityInspectionTemplateResult executeUpdateSensitivityInspectionTemplate(
UpdateSensitivityInspectionTemplateRequest updateSensitivityInspectionTemplateRequest) {
ExecutionContext executionContext = createExecutionContext(updateSensitivityInspectionTemplateRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateSensitivityInspectionTemplateRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(updateSensitivityInspectionTemplateRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Macie2");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateSensitivityInspectionTemplate");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new UpdateSensitivityInspectionTemplateResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
* Returns additional metadata for a previously executed successful, request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing the request.
*
* @param request
* The originally executed request
*
* @return The response metadata for the specified request, or null if none is available.
*/
public ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request) {
return client.getResponseMetadataForRequest(request);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext) {
return invoke(request, responseHandler, executionContext, null, null);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI cachedEndpoint, URI uriFromEndpointTrait) {
executionContext.setCredentialsProvider(CredentialUtils.getCredentialsProvider(request.getOriginalRequest(), awsCredentialsProvider));
return doInvoke(request, responseHandler, executionContext, cachedEndpoint, uriFromEndpointTrait);
}
/**
* Invoke with no authentication. Credentials are not required and any credentials set on the client or request will
* be ignored for this operation.
**/
private Response anonymousInvoke(Request request,
HttpResponseHandler> responseHandler, ExecutionContext executionContext) {
return doInvoke(request, responseHandler, executionContext, null, null);
}
/**
* Invoke the request using the http client. Assumes credentials (or lack thereof) have been configured in the
* ExecutionContext beforehand.
**/
private