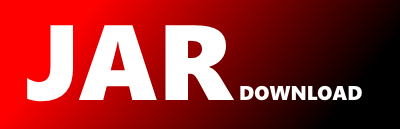
com.amazonaws.services.macie2.model.DescribeClassificationJobResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-macie2 Show documentation
/*
* Copyright 2018-2023 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.macie2.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class DescribeClassificationJobResult extends com.amazonaws.AmazonWebServiceResult implements Serializable, Cloneable {
/**
*
* An array of unique identifiers, one for each allow list that the job uses when it analyzes data.
*
*/
private java.util.List allowListIds;
/**
*
* The token that was provided to ensure the idempotency of the request to create the job.
*
*/
private String clientToken;
/**
*
* The date and time, in UTC and extended ISO 8601 format, when the job was created.
*
*/
private java.util.Date createdAt;
/**
*
* An array of unique identifiers, one for each custom data identifier that the job uses when it analyzes data. This
* value is null if the job uses only managed data identifiers to analyze data.
*
*/
private java.util.List customDataIdentifierIds;
/**
*
* The custom description of the job.
*
*/
private String description;
/**
*
* For a recurring job, specifies whether you configured the job to analyze all existing, eligible objects
* immediately after the job was created (true). If you configured the job to analyze only those objects that were
* created or changed after the job was created and before the job's first scheduled run, this value is false. This
* value is also false for a one-time job.
*
*/
private Boolean initialRun;
/**
*
* The Amazon Resource Name (ARN) of the job.
*
*/
private String jobArn;
/**
*
* The unique identifier for the job.
*
*/
private String jobId;
/**
*
* The current status of the job. Possible values are:
*
*
* -
*
* CANCELLED - You cancelled the job or, if it's a one-time job, you paused the job and didn't resume it within 30
* days.
*
*
* -
*
* COMPLETE - For a one-time job, Amazon Macie finished processing the data specified for the job. This value
* doesn't apply to recurring jobs.
*
*
* -
*
* IDLE - For a recurring job, the previous scheduled run is complete and the next scheduled run is pending. This
* value doesn't apply to one-time jobs.
*
*
* -
*
* PAUSED - Macie started running the job but additional processing would exceed the monthly sensitive data
* discovery quota for your account or one or more member accounts that the job analyzes data for.
*
*
* -
*
* RUNNING - For a one-time job, the job is in progress. For a recurring job, a scheduled run is in progress.
*
*
* -
*
* USER_PAUSED - You paused the job. If you paused the job while it had a status of RUNNING and you don't resume it
* within 30 days of pausing it, the job or job run will expire and be cancelled, depending on the job's type. To
* check the expiration date, refer to the UserPausedDetails.jobExpiresAt property.
*
*
*
*/
private String jobStatus;
/**
*
* The schedule for running the job. Possible values are:
*
*
* -
*
* ONE_TIME - The job runs only once.
*
*
* -
*
* SCHEDULED - The job runs on a daily, weekly, or monthly basis. The scheduleFrequency property indicates the
* recurrence pattern for the job.
*
*
*
*/
private String jobType;
/**
*
* Specifies whether any account- or bucket-level access errors occurred when the job ran. For a recurring job, this
* value indicates the error status of the job's most recent run.
*
*/
private LastRunErrorStatus lastRunErrorStatus;
/**
*
* The date and time, in UTC and extended ISO 8601 format, when the job started. If the job is a recurring job, this
* value indicates when the most recent run started or, if the job hasn't run yet, when the job was created.
*
*/
private java.util.Date lastRunTime;
/**
*
* An array of unique identifiers, one for each managed data identifier that the job is explicitly configured to
* include (use) or exclude (not use) when it analyzes data. Inclusion or exclusion depends on the managed data
* identifier selection type specified for the job (managedDataIdentifierSelector).
*
*
* This value is null if the job's managed data identifier selection type is ALL, NONE, or RECOMMENDED.
*
*/
private java.util.List managedDataIdentifierIds;
/**
*
* The selection type that determines which managed data identifiers the job uses when it analyzes data. Possible
* values are:
*
*
* -
*
* ALL (default) - Use all managed data identifiers.
*
*
* -
*
* EXCLUDE - Use all managed data identifiers except the ones specified by the managedDataIdentifierIds property.
*
*
* -
*
* INCLUDE - Use only the managed data identifiers specified by the managedDataIdentifierIds property.
*
*
* -
*
* NONE - Don't use any managed data identifiers. Use only custom data identifiers (customDataIdentifierIds).
*
*
* -
*
* RECOMMENDED - Use only the set of managed data identifiers that Amazon Web Services recommends for jobs.
*
*
*
*
* If this value is null, the job uses all managed data identifiers.
*
*
* If the job is a recurring job and this value is null, ALL, or EXCLUDE, each job run automatically uses new
* managed data identifiers that are released after the job was created or the preceding run ended. If this value is
* RECOMMENDED for a recurring job, each job run uses all the managed data identifiers that are in the recommended
* set when the run starts.
*
*
* For information about individual managed data identifiers or to determine which ones are in the recommended set,
* see Using managed data
* identifiers and Recommended managed
* data identifiers in the Amazon Macie User Guide.
*
*/
private String managedDataIdentifierSelector;
/**
*
* The custom name of the job.
*
*/
private String name;
/**
*
* The S3 buckets that contain the objects to analyze, and the scope of that analysis.
*
*/
private S3JobDefinition s3JobDefinition;
/**
*
* The sampling depth, as a percentage, that determines the percentage of eligible objects that the job analyzes.
*
*/
private Integer samplingPercentage;
/**
*
* The recurrence pattern for running the job. This value is null if the job is configured to run only once.
*
*/
private JobScheduleFrequency scheduleFrequency;
/**
*
* The number of times that the job has run and processing statistics for the job's current run.
*
*/
private Statistics statistics;
/**
*
* A map of key-value pairs that specifies which tags (keys and values) are associated with the classification job.
*
*/
private java.util.Map tags;
/**
*
* If the current status of the job is USER_PAUSED, specifies when the job was paused and when the job or job run
* will expire and be cancelled if it isn't resumed. This value is present only if the value for jobStatus is
* USER_PAUSED.
*
*/
private UserPausedDetails userPausedDetails;
/**
*
* An array of unique identifiers, one for each allow list that the job uses when it analyzes data.
*
*
* @return An array of unique identifiers, one for each allow list that the job uses when it analyzes data.
*/
public java.util.List getAllowListIds() {
return allowListIds;
}
/**
*
* An array of unique identifiers, one for each allow list that the job uses when it analyzes data.
*
*
* @param allowListIds
* An array of unique identifiers, one for each allow list that the job uses when it analyzes data.
*/
public void setAllowListIds(java.util.Collection allowListIds) {
if (allowListIds == null) {
this.allowListIds = null;
return;
}
this.allowListIds = new java.util.ArrayList(allowListIds);
}
/**
*
* An array of unique identifiers, one for each allow list that the job uses when it analyzes data.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setAllowListIds(java.util.Collection)} or {@link #withAllowListIds(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param allowListIds
* An array of unique identifiers, one for each allow list that the job uses when it analyzes data.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeClassificationJobResult withAllowListIds(String... allowListIds) {
if (this.allowListIds == null) {
setAllowListIds(new java.util.ArrayList(allowListIds.length));
}
for (String ele : allowListIds) {
this.allowListIds.add(ele);
}
return this;
}
/**
*
* An array of unique identifiers, one for each allow list that the job uses when it analyzes data.
*
*
* @param allowListIds
* An array of unique identifiers, one for each allow list that the job uses when it analyzes data.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeClassificationJobResult withAllowListIds(java.util.Collection allowListIds) {
setAllowListIds(allowListIds);
return this;
}
/**
*
* The token that was provided to ensure the idempotency of the request to create the job.
*
*
* @param clientToken
* The token that was provided to ensure the idempotency of the request to create the job.
*/
public void setClientToken(String clientToken) {
this.clientToken = clientToken;
}
/**
*
* The token that was provided to ensure the idempotency of the request to create the job.
*
*
* @return The token that was provided to ensure the idempotency of the request to create the job.
*/
public String getClientToken() {
return this.clientToken;
}
/**
*
* The token that was provided to ensure the idempotency of the request to create the job.
*
*
* @param clientToken
* The token that was provided to ensure the idempotency of the request to create the job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeClassificationJobResult withClientToken(String clientToken) {
setClientToken(clientToken);
return this;
}
/**
*
* The date and time, in UTC and extended ISO 8601 format, when the job was created.
*
*
* @param createdAt
* The date and time, in UTC and extended ISO 8601 format, when the job was created.
*/
public void setCreatedAt(java.util.Date createdAt) {
this.createdAt = createdAt;
}
/**
*
* The date and time, in UTC and extended ISO 8601 format, when the job was created.
*
*
* @return The date and time, in UTC and extended ISO 8601 format, when the job was created.
*/
public java.util.Date getCreatedAt() {
return this.createdAt;
}
/**
*
* The date and time, in UTC and extended ISO 8601 format, when the job was created.
*
*
* @param createdAt
* The date and time, in UTC and extended ISO 8601 format, when the job was created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeClassificationJobResult withCreatedAt(java.util.Date createdAt) {
setCreatedAt(createdAt);
return this;
}
/**
*
* An array of unique identifiers, one for each custom data identifier that the job uses when it analyzes data. This
* value is null if the job uses only managed data identifiers to analyze data.
*
*
* @return An array of unique identifiers, one for each custom data identifier that the job uses when it analyzes
* data. This value is null if the job uses only managed data identifiers to analyze data.
*/
public java.util.List getCustomDataIdentifierIds() {
return customDataIdentifierIds;
}
/**
*
* An array of unique identifiers, one for each custom data identifier that the job uses when it analyzes data. This
* value is null if the job uses only managed data identifiers to analyze data.
*
*
* @param customDataIdentifierIds
* An array of unique identifiers, one for each custom data identifier that the job uses when it analyzes
* data. This value is null if the job uses only managed data identifiers to analyze data.
*/
public void setCustomDataIdentifierIds(java.util.Collection customDataIdentifierIds) {
if (customDataIdentifierIds == null) {
this.customDataIdentifierIds = null;
return;
}
this.customDataIdentifierIds = new java.util.ArrayList(customDataIdentifierIds);
}
/**
*
* An array of unique identifiers, one for each custom data identifier that the job uses when it analyzes data. This
* value is null if the job uses only managed data identifiers to analyze data.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setCustomDataIdentifierIds(java.util.Collection)} or
* {@link #withCustomDataIdentifierIds(java.util.Collection)} if you want to override the existing values.
*
*
* @param customDataIdentifierIds
* An array of unique identifiers, one for each custom data identifier that the job uses when it analyzes
* data. This value is null if the job uses only managed data identifiers to analyze data.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeClassificationJobResult withCustomDataIdentifierIds(String... customDataIdentifierIds) {
if (this.customDataIdentifierIds == null) {
setCustomDataIdentifierIds(new java.util.ArrayList(customDataIdentifierIds.length));
}
for (String ele : customDataIdentifierIds) {
this.customDataIdentifierIds.add(ele);
}
return this;
}
/**
*
* An array of unique identifiers, one for each custom data identifier that the job uses when it analyzes data. This
* value is null if the job uses only managed data identifiers to analyze data.
*
*
* @param customDataIdentifierIds
* An array of unique identifiers, one for each custom data identifier that the job uses when it analyzes
* data. This value is null if the job uses only managed data identifiers to analyze data.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeClassificationJobResult withCustomDataIdentifierIds(java.util.Collection customDataIdentifierIds) {
setCustomDataIdentifierIds(customDataIdentifierIds);
return this;
}
/**
*
* The custom description of the job.
*
*
* @param description
* The custom description of the job.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* The custom description of the job.
*
*
* @return The custom description of the job.
*/
public String getDescription() {
return this.description;
}
/**
*
* The custom description of the job.
*
*
* @param description
* The custom description of the job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeClassificationJobResult withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* For a recurring job, specifies whether you configured the job to analyze all existing, eligible objects
* immediately after the job was created (true). If you configured the job to analyze only those objects that were
* created or changed after the job was created and before the job's first scheduled run, this value is false. This
* value is also false for a one-time job.
*
*
* @param initialRun
* For a recurring job, specifies whether you configured the job to analyze all existing, eligible objects
* immediately after the job was created (true). If you configured the job to analyze only those objects that
* were created or changed after the job was created and before the job's first scheduled run, this value is
* false. This value is also false for a one-time job.
*/
public void setInitialRun(Boolean initialRun) {
this.initialRun = initialRun;
}
/**
*
* For a recurring job, specifies whether you configured the job to analyze all existing, eligible objects
* immediately after the job was created (true). If you configured the job to analyze only those objects that were
* created or changed after the job was created and before the job's first scheduled run, this value is false. This
* value is also false for a one-time job.
*
*
* @return For a recurring job, specifies whether you configured the job to analyze all existing, eligible objects
* immediately after the job was created (true). If you configured the job to analyze only those objects
* that were created or changed after the job was created and before the job's first scheduled run, this
* value is false. This value is also false for a one-time job.
*/
public Boolean getInitialRun() {
return this.initialRun;
}
/**
*
* For a recurring job, specifies whether you configured the job to analyze all existing, eligible objects
* immediately after the job was created (true). If you configured the job to analyze only those objects that were
* created or changed after the job was created and before the job's first scheduled run, this value is false. This
* value is also false for a one-time job.
*
*
* @param initialRun
* For a recurring job, specifies whether you configured the job to analyze all existing, eligible objects
* immediately after the job was created (true). If you configured the job to analyze only those objects that
* were created or changed after the job was created and before the job's first scheduled run, this value is
* false. This value is also false for a one-time job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeClassificationJobResult withInitialRun(Boolean initialRun) {
setInitialRun(initialRun);
return this;
}
/**
*
* For a recurring job, specifies whether you configured the job to analyze all existing, eligible objects
* immediately after the job was created (true). If you configured the job to analyze only those objects that were
* created or changed after the job was created and before the job's first scheduled run, this value is false. This
* value is also false for a one-time job.
*
*
* @return For a recurring job, specifies whether you configured the job to analyze all existing, eligible objects
* immediately after the job was created (true). If you configured the job to analyze only those objects
* that were created or changed after the job was created and before the job's first scheduled run, this
* value is false. This value is also false for a one-time job.
*/
public Boolean isInitialRun() {
return this.initialRun;
}
/**
*
* The Amazon Resource Name (ARN) of the job.
*
*
* @param jobArn
* The Amazon Resource Name (ARN) of the job.
*/
public void setJobArn(String jobArn) {
this.jobArn = jobArn;
}
/**
*
* The Amazon Resource Name (ARN) of the job.
*
*
* @return The Amazon Resource Name (ARN) of the job.
*/
public String getJobArn() {
return this.jobArn;
}
/**
*
* The Amazon Resource Name (ARN) of the job.
*
*
* @param jobArn
* The Amazon Resource Name (ARN) of the job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeClassificationJobResult withJobArn(String jobArn) {
setJobArn(jobArn);
return this;
}
/**
*
* The unique identifier for the job.
*
*
* @param jobId
* The unique identifier for the job.
*/
public void setJobId(String jobId) {
this.jobId = jobId;
}
/**
*
* The unique identifier for the job.
*
*
* @return The unique identifier for the job.
*/
public String getJobId() {
return this.jobId;
}
/**
*
* The unique identifier for the job.
*
*
* @param jobId
* The unique identifier for the job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeClassificationJobResult withJobId(String jobId) {
setJobId(jobId);
return this;
}
/**
*
* The current status of the job. Possible values are:
*
*
* -
*
* CANCELLED - You cancelled the job or, if it's a one-time job, you paused the job and didn't resume it within 30
* days.
*
*
* -
*
* COMPLETE - For a one-time job, Amazon Macie finished processing the data specified for the job. This value
* doesn't apply to recurring jobs.
*
*
* -
*
* IDLE - For a recurring job, the previous scheduled run is complete and the next scheduled run is pending. This
* value doesn't apply to one-time jobs.
*
*
* -
*
* PAUSED - Macie started running the job but additional processing would exceed the monthly sensitive data
* discovery quota for your account or one or more member accounts that the job analyzes data for.
*
*
* -
*
* RUNNING - For a one-time job, the job is in progress. For a recurring job, a scheduled run is in progress.
*
*
* -
*
* USER_PAUSED - You paused the job. If you paused the job while it had a status of RUNNING and you don't resume it
* within 30 days of pausing it, the job or job run will expire and be cancelled, depending on the job's type. To
* check the expiration date, refer to the UserPausedDetails.jobExpiresAt property.
*
*
*
*
* @param jobStatus
* The current status of the job. Possible values are:
*
* -
*
* CANCELLED - You cancelled the job or, if it's a one-time job, you paused the job and didn't resume it
* within 30 days.
*
*
* -
*
* COMPLETE - For a one-time job, Amazon Macie finished processing the data specified for the job. This value
* doesn't apply to recurring jobs.
*
*
* -
*
* IDLE - For a recurring job, the previous scheduled run is complete and the next scheduled run is pending.
* This value doesn't apply to one-time jobs.
*
*
* -
*
* PAUSED - Macie started running the job but additional processing would exceed the monthly sensitive data
* discovery quota for your account or one or more member accounts that the job analyzes data for.
*
*
* -
*
* RUNNING - For a one-time job, the job is in progress. For a recurring job, a scheduled run is in progress.
*
*
* -
*
* USER_PAUSED - You paused the job. If you paused the job while it had a status of RUNNING and you don't
* resume it within 30 days of pausing it, the job or job run will expire and be cancelled, depending on the
* job's type. To check the expiration date, refer to the UserPausedDetails.jobExpiresAt property.
*
*
* @see JobStatus
*/
public void setJobStatus(String jobStatus) {
this.jobStatus = jobStatus;
}
/**
*
* The current status of the job. Possible values are:
*
*
* -
*
* CANCELLED - You cancelled the job or, if it's a one-time job, you paused the job and didn't resume it within 30
* days.
*
*
* -
*
* COMPLETE - For a one-time job, Amazon Macie finished processing the data specified for the job. This value
* doesn't apply to recurring jobs.
*
*
* -
*
* IDLE - For a recurring job, the previous scheduled run is complete and the next scheduled run is pending. This
* value doesn't apply to one-time jobs.
*
*
* -
*
* PAUSED - Macie started running the job but additional processing would exceed the monthly sensitive data
* discovery quota for your account or one or more member accounts that the job analyzes data for.
*
*
* -
*
* RUNNING - For a one-time job, the job is in progress. For a recurring job, a scheduled run is in progress.
*
*
* -
*
* USER_PAUSED - You paused the job. If you paused the job while it had a status of RUNNING and you don't resume it
* within 30 days of pausing it, the job or job run will expire and be cancelled, depending on the job's type. To
* check the expiration date, refer to the UserPausedDetails.jobExpiresAt property.
*
*
*
*
* @return The current status of the job. Possible values are:
*
* -
*
* CANCELLED - You cancelled the job or, if it's a one-time job, you paused the job and didn't resume it
* within 30 days.
*
*
* -
*
* COMPLETE - For a one-time job, Amazon Macie finished processing the data specified for the job. This
* value doesn't apply to recurring jobs.
*
*
* -
*
* IDLE - For a recurring job, the previous scheduled run is complete and the next scheduled run is pending.
* This value doesn't apply to one-time jobs.
*
*
* -
*
* PAUSED - Macie started running the job but additional processing would exceed the monthly sensitive data
* discovery quota for your account or one or more member accounts that the job analyzes data for.
*
*
* -
*
* RUNNING - For a one-time job, the job is in progress. For a recurring job, a scheduled run is in
* progress.
*
*
* -
*
* USER_PAUSED - You paused the job. If you paused the job while it had a status of RUNNING and you don't
* resume it within 30 days of pausing it, the job or job run will expire and be cancelled, depending on the
* job's type. To check the expiration date, refer to the UserPausedDetails.jobExpiresAt property.
*
*
* @see JobStatus
*/
public String getJobStatus() {
return this.jobStatus;
}
/**
*
* The current status of the job. Possible values are:
*
*
* -
*
* CANCELLED - You cancelled the job or, if it's a one-time job, you paused the job and didn't resume it within 30
* days.
*
*
* -
*
* COMPLETE - For a one-time job, Amazon Macie finished processing the data specified for the job. This value
* doesn't apply to recurring jobs.
*
*
* -
*
* IDLE - For a recurring job, the previous scheduled run is complete and the next scheduled run is pending. This
* value doesn't apply to one-time jobs.
*
*
* -
*
* PAUSED - Macie started running the job but additional processing would exceed the monthly sensitive data
* discovery quota for your account or one or more member accounts that the job analyzes data for.
*
*
* -
*
* RUNNING - For a one-time job, the job is in progress. For a recurring job, a scheduled run is in progress.
*
*
* -
*
* USER_PAUSED - You paused the job. If you paused the job while it had a status of RUNNING and you don't resume it
* within 30 days of pausing it, the job or job run will expire and be cancelled, depending on the job's type. To
* check the expiration date, refer to the UserPausedDetails.jobExpiresAt property.
*
*
*
*
* @param jobStatus
* The current status of the job. Possible values are:
*
* -
*
* CANCELLED - You cancelled the job or, if it's a one-time job, you paused the job and didn't resume it
* within 30 days.
*
*
* -
*
* COMPLETE - For a one-time job, Amazon Macie finished processing the data specified for the job. This value
* doesn't apply to recurring jobs.
*
*
* -
*
* IDLE - For a recurring job, the previous scheduled run is complete and the next scheduled run is pending.
* This value doesn't apply to one-time jobs.
*
*
* -
*
* PAUSED - Macie started running the job but additional processing would exceed the monthly sensitive data
* discovery quota for your account or one or more member accounts that the job analyzes data for.
*
*
* -
*
* RUNNING - For a one-time job, the job is in progress. For a recurring job, a scheduled run is in progress.
*
*
* -
*
* USER_PAUSED - You paused the job. If you paused the job while it had a status of RUNNING and you don't
* resume it within 30 days of pausing it, the job or job run will expire and be cancelled, depending on the
* job's type. To check the expiration date, refer to the UserPausedDetails.jobExpiresAt property.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see JobStatus
*/
public DescribeClassificationJobResult withJobStatus(String jobStatus) {
setJobStatus(jobStatus);
return this;
}
/**
*
* The current status of the job. Possible values are:
*
*
* -
*
* CANCELLED - You cancelled the job or, if it's a one-time job, you paused the job and didn't resume it within 30
* days.
*
*
* -
*
* COMPLETE - For a one-time job, Amazon Macie finished processing the data specified for the job. This value
* doesn't apply to recurring jobs.
*
*
* -
*
* IDLE - For a recurring job, the previous scheduled run is complete and the next scheduled run is pending. This
* value doesn't apply to one-time jobs.
*
*
* -
*
* PAUSED - Macie started running the job but additional processing would exceed the monthly sensitive data
* discovery quota for your account or one or more member accounts that the job analyzes data for.
*
*
* -
*
* RUNNING - For a one-time job, the job is in progress. For a recurring job, a scheduled run is in progress.
*
*
* -
*
* USER_PAUSED - You paused the job. If you paused the job while it had a status of RUNNING and you don't resume it
* within 30 days of pausing it, the job or job run will expire and be cancelled, depending on the job's type. To
* check the expiration date, refer to the UserPausedDetails.jobExpiresAt property.
*
*
*
*
* @param jobStatus
* The current status of the job. Possible values are:
*
* -
*
* CANCELLED - You cancelled the job or, if it's a one-time job, you paused the job and didn't resume it
* within 30 days.
*
*
* -
*
* COMPLETE - For a one-time job, Amazon Macie finished processing the data specified for the job. This value
* doesn't apply to recurring jobs.
*
*
* -
*
* IDLE - For a recurring job, the previous scheduled run is complete and the next scheduled run is pending.
* This value doesn't apply to one-time jobs.
*
*
* -
*
* PAUSED - Macie started running the job but additional processing would exceed the monthly sensitive data
* discovery quota for your account or one or more member accounts that the job analyzes data for.
*
*
* -
*
* RUNNING - For a one-time job, the job is in progress. For a recurring job, a scheduled run is in progress.
*
*
* -
*
* USER_PAUSED - You paused the job. If you paused the job while it had a status of RUNNING and you don't
* resume it within 30 days of pausing it, the job or job run will expire and be cancelled, depending on the
* job's type. To check the expiration date, refer to the UserPausedDetails.jobExpiresAt property.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see JobStatus
*/
public DescribeClassificationJobResult withJobStatus(JobStatus jobStatus) {
this.jobStatus = jobStatus.toString();
return this;
}
/**
*
* The schedule for running the job. Possible values are:
*
*
* -
*
* ONE_TIME - The job runs only once.
*
*
* -
*
* SCHEDULED - The job runs on a daily, weekly, or monthly basis. The scheduleFrequency property indicates the
* recurrence pattern for the job.
*
*
*
*
* @param jobType
* The schedule for running the job. Possible values are:
*
* -
*
* ONE_TIME - The job runs only once.
*
*
* -
*
* SCHEDULED - The job runs on a daily, weekly, or monthly basis. The scheduleFrequency property indicates
* the recurrence pattern for the job.
*
*
* @see JobType
*/
public void setJobType(String jobType) {
this.jobType = jobType;
}
/**
*
* The schedule for running the job. Possible values are:
*
*
* -
*
* ONE_TIME - The job runs only once.
*
*
* -
*
* SCHEDULED - The job runs on a daily, weekly, or monthly basis. The scheduleFrequency property indicates the
* recurrence pattern for the job.
*
*
*
*
* @return The schedule for running the job. Possible values are:
*
* -
*
* ONE_TIME - The job runs only once.
*
*
* -
*
* SCHEDULED - The job runs on a daily, weekly, or monthly basis. The scheduleFrequency property indicates
* the recurrence pattern for the job.
*
*
* @see JobType
*/
public String getJobType() {
return this.jobType;
}
/**
*
* The schedule for running the job. Possible values are:
*
*
* -
*
* ONE_TIME - The job runs only once.
*
*
* -
*
* SCHEDULED - The job runs on a daily, weekly, or monthly basis. The scheduleFrequency property indicates the
* recurrence pattern for the job.
*
*
*
*
* @param jobType
* The schedule for running the job. Possible values are:
*
* -
*
* ONE_TIME - The job runs only once.
*
*
* -
*
* SCHEDULED - The job runs on a daily, weekly, or monthly basis. The scheduleFrequency property indicates
* the recurrence pattern for the job.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see JobType
*/
public DescribeClassificationJobResult withJobType(String jobType) {
setJobType(jobType);
return this;
}
/**
*
* The schedule for running the job. Possible values are:
*
*
* -
*
* ONE_TIME - The job runs only once.
*
*
* -
*
* SCHEDULED - The job runs on a daily, weekly, or monthly basis. The scheduleFrequency property indicates the
* recurrence pattern for the job.
*
*
*
*
* @param jobType
* The schedule for running the job. Possible values are:
*
* -
*
* ONE_TIME - The job runs only once.
*
*
* -
*
* SCHEDULED - The job runs on a daily, weekly, or monthly basis. The scheduleFrequency property indicates
* the recurrence pattern for the job.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see JobType
*/
public DescribeClassificationJobResult withJobType(JobType jobType) {
this.jobType = jobType.toString();
return this;
}
/**
*
* Specifies whether any account- or bucket-level access errors occurred when the job ran. For a recurring job, this
* value indicates the error status of the job's most recent run.
*
*
* @param lastRunErrorStatus
* Specifies whether any account- or bucket-level access errors occurred when the job ran. For a recurring
* job, this value indicates the error status of the job's most recent run.
*/
public void setLastRunErrorStatus(LastRunErrorStatus lastRunErrorStatus) {
this.lastRunErrorStatus = lastRunErrorStatus;
}
/**
*
* Specifies whether any account- or bucket-level access errors occurred when the job ran. For a recurring job, this
* value indicates the error status of the job's most recent run.
*
*
* @return Specifies whether any account- or bucket-level access errors occurred when the job ran. For a recurring
* job, this value indicates the error status of the job's most recent run.
*/
public LastRunErrorStatus getLastRunErrorStatus() {
return this.lastRunErrorStatus;
}
/**
*
* Specifies whether any account- or bucket-level access errors occurred when the job ran. For a recurring job, this
* value indicates the error status of the job's most recent run.
*
*
* @param lastRunErrorStatus
* Specifies whether any account- or bucket-level access errors occurred when the job ran. For a recurring
* job, this value indicates the error status of the job's most recent run.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeClassificationJobResult withLastRunErrorStatus(LastRunErrorStatus lastRunErrorStatus) {
setLastRunErrorStatus(lastRunErrorStatus);
return this;
}
/**
*
* The date and time, in UTC and extended ISO 8601 format, when the job started. If the job is a recurring job, this
* value indicates when the most recent run started or, if the job hasn't run yet, when the job was created.
*
*
* @param lastRunTime
* The date and time, in UTC and extended ISO 8601 format, when the job started. If the job is a recurring
* job, this value indicates when the most recent run started or, if the job hasn't run yet, when the job was
* created.
*/
public void setLastRunTime(java.util.Date lastRunTime) {
this.lastRunTime = lastRunTime;
}
/**
*
* The date and time, in UTC and extended ISO 8601 format, when the job started. If the job is a recurring job, this
* value indicates when the most recent run started or, if the job hasn't run yet, when the job was created.
*
*
* @return The date and time, in UTC and extended ISO 8601 format, when the job started. If the job is a recurring
* job, this value indicates when the most recent run started or, if the job hasn't run yet, when the job
* was created.
*/
public java.util.Date getLastRunTime() {
return this.lastRunTime;
}
/**
*
* The date and time, in UTC and extended ISO 8601 format, when the job started. If the job is a recurring job, this
* value indicates when the most recent run started or, if the job hasn't run yet, when the job was created.
*
*
* @param lastRunTime
* The date and time, in UTC and extended ISO 8601 format, when the job started. If the job is a recurring
* job, this value indicates when the most recent run started or, if the job hasn't run yet, when the job was
* created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeClassificationJobResult withLastRunTime(java.util.Date lastRunTime) {
setLastRunTime(lastRunTime);
return this;
}
/**
*
* An array of unique identifiers, one for each managed data identifier that the job is explicitly configured to
* include (use) or exclude (not use) when it analyzes data. Inclusion or exclusion depends on the managed data
* identifier selection type specified for the job (managedDataIdentifierSelector).
*
*
* This value is null if the job's managed data identifier selection type is ALL, NONE, or RECOMMENDED.
*
*
* @return An array of unique identifiers, one for each managed data identifier that the job is explicitly
* configured to include (use) or exclude (not use) when it analyzes data. Inclusion or exclusion depends on
* the managed data identifier selection type specified for the job (managedDataIdentifierSelector).
*
* This value is null if the job's managed data identifier selection type is ALL, NONE, or RECOMMENDED.
*/
public java.util.List getManagedDataIdentifierIds() {
return managedDataIdentifierIds;
}
/**
*
* An array of unique identifiers, one for each managed data identifier that the job is explicitly configured to
* include (use) or exclude (not use) when it analyzes data. Inclusion or exclusion depends on the managed data
* identifier selection type specified for the job (managedDataIdentifierSelector).
*
*
* This value is null if the job's managed data identifier selection type is ALL, NONE, or RECOMMENDED.
*
*
* @param managedDataIdentifierIds
* An array of unique identifiers, one for each managed data identifier that the job is explicitly configured
* to include (use) or exclude (not use) when it analyzes data. Inclusion or exclusion depends on the managed
* data identifier selection type specified for the job (managedDataIdentifierSelector).
*
* This value is null if the job's managed data identifier selection type is ALL, NONE, or RECOMMENDED.
*/
public void setManagedDataIdentifierIds(java.util.Collection managedDataIdentifierIds) {
if (managedDataIdentifierIds == null) {
this.managedDataIdentifierIds = null;
return;
}
this.managedDataIdentifierIds = new java.util.ArrayList(managedDataIdentifierIds);
}
/**
*
* An array of unique identifiers, one for each managed data identifier that the job is explicitly configured to
* include (use) or exclude (not use) when it analyzes data. Inclusion or exclusion depends on the managed data
* identifier selection type specified for the job (managedDataIdentifierSelector).
*
*
* This value is null if the job's managed data identifier selection type is ALL, NONE, or RECOMMENDED.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setManagedDataIdentifierIds(java.util.Collection)} or
* {@link #withManagedDataIdentifierIds(java.util.Collection)} if you want to override the existing values.
*
*
* @param managedDataIdentifierIds
* An array of unique identifiers, one for each managed data identifier that the job is explicitly configured
* to include (use) or exclude (not use) when it analyzes data. Inclusion or exclusion depends on the managed
* data identifier selection type specified for the job (managedDataIdentifierSelector).
*
* This value is null if the job's managed data identifier selection type is ALL, NONE, or RECOMMENDED.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeClassificationJobResult withManagedDataIdentifierIds(String... managedDataIdentifierIds) {
if (this.managedDataIdentifierIds == null) {
setManagedDataIdentifierIds(new java.util.ArrayList(managedDataIdentifierIds.length));
}
for (String ele : managedDataIdentifierIds) {
this.managedDataIdentifierIds.add(ele);
}
return this;
}
/**
*
* An array of unique identifiers, one for each managed data identifier that the job is explicitly configured to
* include (use) or exclude (not use) when it analyzes data. Inclusion or exclusion depends on the managed data
* identifier selection type specified for the job (managedDataIdentifierSelector).
*
*
* This value is null if the job's managed data identifier selection type is ALL, NONE, or RECOMMENDED.
*
*
* @param managedDataIdentifierIds
* An array of unique identifiers, one for each managed data identifier that the job is explicitly configured
* to include (use) or exclude (not use) when it analyzes data. Inclusion or exclusion depends on the managed
* data identifier selection type specified for the job (managedDataIdentifierSelector).
*
* This value is null if the job's managed data identifier selection type is ALL, NONE, or RECOMMENDED.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeClassificationJobResult withManagedDataIdentifierIds(java.util.Collection managedDataIdentifierIds) {
setManagedDataIdentifierIds(managedDataIdentifierIds);
return this;
}
/**
*
* The selection type that determines which managed data identifiers the job uses when it analyzes data. Possible
* values are:
*
*
* -
*
* ALL (default) - Use all managed data identifiers.
*
*
* -
*
* EXCLUDE - Use all managed data identifiers except the ones specified by the managedDataIdentifierIds property.
*
*
* -
*
* INCLUDE - Use only the managed data identifiers specified by the managedDataIdentifierIds property.
*
*
* -
*
* NONE - Don't use any managed data identifiers. Use only custom data identifiers (customDataIdentifierIds).
*
*
* -
*
* RECOMMENDED - Use only the set of managed data identifiers that Amazon Web Services recommends for jobs.
*
*
*
*
* If this value is null, the job uses all managed data identifiers.
*
*
* If the job is a recurring job and this value is null, ALL, or EXCLUDE, each job run automatically uses new
* managed data identifiers that are released after the job was created or the preceding run ended. If this value is
* RECOMMENDED for a recurring job, each job run uses all the managed data identifiers that are in the recommended
* set when the run starts.
*
*
* For information about individual managed data identifiers or to determine which ones are in the recommended set,
* see Using managed data
* identifiers and Recommended managed
* data identifiers in the Amazon Macie User Guide.
*
*
* @param managedDataIdentifierSelector
* The selection type that determines which managed data identifiers the job uses when it analyzes data.
* Possible values are:
*
* -
*
* ALL (default) - Use all managed data identifiers.
*
*
* -
*
* EXCLUDE - Use all managed data identifiers except the ones specified by the managedDataIdentifierIds
* property.
*
*
* -
*
* INCLUDE - Use only the managed data identifiers specified by the managedDataIdentifierIds property.
*
*
* -
*
* NONE - Don't use any managed data identifiers. Use only custom data identifiers (customDataIdentifierIds).
*
*
* -
*
* RECOMMENDED - Use only the set of managed data identifiers that Amazon Web Services recommends for jobs.
*
*
*
*
* If this value is null, the job uses all managed data identifiers.
*
*
* If the job is a recurring job and this value is null, ALL, or EXCLUDE, each job run automatically uses new
* managed data identifiers that are released after the job was created or the preceding run ended. If this
* value is RECOMMENDED for a recurring job, each job run uses all the managed data identifiers that are in
* the recommended set when the run starts.
*
*
* For information about individual managed data identifiers or to determine which ones are in the
* recommended set, see Using managed data
* identifiers and Recommended
* managed data identifiers in the Amazon Macie User Guide.
* @see ManagedDataIdentifierSelector
*/
public void setManagedDataIdentifierSelector(String managedDataIdentifierSelector) {
this.managedDataIdentifierSelector = managedDataIdentifierSelector;
}
/**
*
* The selection type that determines which managed data identifiers the job uses when it analyzes data. Possible
* values are:
*
*
* -
*
* ALL (default) - Use all managed data identifiers.
*
*
* -
*
* EXCLUDE - Use all managed data identifiers except the ones specified by the managedDataIdentifierIds property.
*
*
* -
*
* INCLUDE - Use only the managed data identifiers specified by the managedDataIdentifierIds property.
*
*
* -
*
* NONE - Don't use any managed data identifiers. Use only custom data identifiers (customDataIdentifierIds).
*
*
* -
*
* RECOMMENDED - Use only the set of managed data identifiers that Amazon Web Services recommends for jobs.
*
*
*
*
* If this value is null, the job uses all managed data identifiers.
*
*
* If the job is a recurring job and this value is null, ALL, or EXCLUDE, each job run automatically uses new
* managed data identifiers that are released after the job was created or the preceding run ended. If this value is
* RECOMMENDED for a recurring job, each job run uses all the managed data identifiers that are in the recommended
* set when the run starts.
*
*
* For information about individual managed data identifiers or to determine which ones are in the recommended set,
* see Using managed data
* identifiers and Recommended managed
* data identifiers in the Amazon Macie User Guide.
*
*
* @return The selection type that determines which managed data identifiers the job uses when it analyzes data.
* Possible values are:
*
* -
*
* ALL (default) - Use all managed data identifiers.
*
*
* -
*
* EXCLUDE - Use all managed data identifiers except the ones specified by the managedDataIdentifierIds
* property.
*
*
* -
*
* INCLUDE - Use only the managed data identifiers specified by the managedDataIdentifierIds property.
*
*
* -
*
* NONE - Don't use any managed data identifiers. Use only custom data identifiers
* (customDataIdentifierIds).
*
*
* -
*
* RECOMMENDED - Use only the set of managed data identifiers that Amazon Web Services recommends for jobs.
*
*
*
*
* If this value is null, the job uses all managed data identifiers.
*
*
* If the job is a recurring job and this value is null, ALL, or EXCLUDE, each job run automatically uses
* new managed data identifiers that are released after the job was created or the preceding run ended. If
* this value is RECOMMENDED for a recurring job, each job run uses all the managed data identifiers that
* are in the recommended set when the run starts.
*
*
* For information about individual managed data identifiers or to determine which ones are in the
* recommended set, see Using managed data
* identifiers and Recommended
* managed data identifiers in the Amazon Macie User Guide.
* @see ManagedDataIdentifierSelector
*/
public String getManagedDataIdentifierSelector() {
return this.managedDataIdentifierSelector;
}
/**
*
* The selection type that determines which managed data identifiers the job uses when it analyzes data. Possible
* values are:
*
*
* -
*
* ALL (default) - Use all managed data identifiers.
*
*
* -
*
* EXCLUDE - Use all managed data identifiers except the ones specified by the managedDataIdentifierIds property.
*
*
* -
*
* INCLUDE - Use only the managed data identifiers specified by the managedDataIdentifierIds property.
*
*
* -
*
* NONE - Don't use any managed data identifiers. Use only custom data identifiers (customDataIdentifierIds).
*
*
* -
*
* RECOMMENDED - Use only the set of managed data identifiers that Amazon Web Services recommends for jobs.
*
*
*
*
* If this value is null, the job uses all managed data identifiers.
*
*
* If the job is a recurring job and this value is null, ALL, or EXCLUDE, each job run automatically uses new
* managed data identifiers that are released after the job was created or the preceding run ended. If this value is
* RECOMMENDED for a recurring job, each job run uses all the managed data identifiers that are in the recommended
* set when the run starts.
*
*
* For information about individual managed data identifiers or to determine which ones are in the recommended set,
* see Using managed data
* identifiers and Recommended managed
* data identifiers in the Amazon Macie User Guide.
*
*
* @param managedDataIdentifierSelector
* The selection type that determines which managed data identifiers the job uses when it analyzes data.
* Possible values are:
*
* -
*
* ALL (default) - Use all managed data identifiers.
*
*
* -
*
* EXCLUDE - Use all managed data identifiers except the ones specified by the managedDataIdentifierIds
* property.
*
*
* -
*
* INCLUDE - Use only the managed data identifiers specified by the managedDataIdentifierIds property.
*
*
* -
*
* NONE - Don't use any managed data identifiers. Use only custom data identifiers (customDataIdentifierIds).
*
*
* -
*
* RECOMMENDED - Use only the set of managed data identifiers that Amazon Web Services recommends for jobs.
*
*
*
*
* If this value is null, the job uses all managed data identifiers.
*
*
* If the job is a recurring job and this value is null, ALL, or EXCLUDE, each job run automatically uses new
* managed data identifiers that are released after the job was created or the preceding run ended. If this
* value is RECOMMENDED for a recurring job, each job run uses all the managed data identifiers that are in
* the recommended set when the run starts.
*
*
* For information about individual managed data identifiers or to determine which ones are in the
* recommended set, see Using managed data
* identifiers and Recommended
* managed data identifiers in the Amazon Macie User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ManagedDataIdentifierSelector
*/
public DescribeClassificationJobResult withManagedDataIdentifierSelector(String managedDataIdentifierSelector) {
setManagedDataIdentifierSelector(managedDataIdentifierSelector);
return this;
}
/**
*
* The selection type that determines which managed data identifiers the job uses when it analyzes data. Possible
* values are:
*
*
* -
*
* ALL (default) - Use all managed data identifiers.
*
*
* -
*
* EXCLUDE - Use all managed data identifiers except the ones specified by the managedDataIdentifierIds property.
*
*
* -
*
* INCLUDE - Use only the managed data identifiers specified by the managedDataIdentifierIds property.
*
*
* -
*
* NONE - Don't use any managed data identifiers. Use only custom data identifiers (customDataIdentifierIds).
*
*
* -
*
* RECOMMENDED - Use only the set of managed data identifiers that Amazon Web Services recommends for jobs.
*
*
*
*
* If this value is null, the job uses all managed data identifiers.
*
*
* If the job is a recurring job and this value is null, ALL, or EXCLUDE, each job run automatically uses new
* managed data identifiers that are released after the job was created or the preceding run ended. If this value is
* RECOMMENDED for a recurring job, each job run uses all the managed data identifiers that are in the recommended
* set when the run starts.
*
*
* For information about individual managed data identifiers or to determine which ones are in the recommended set,
* see Using managed data
* identifiers and Recommended managed
* data identifiers in the Amazon Macie User Guide.
*
*
* @param managedDataIdentifierSelector
* The selection type that determines which managed data identifiers the job uses when it analyzes data.
* Possible values are:
*
* -
*
* ALL (default) - Use all managed data identifiers.
*
*
* -
*
* EXCLUDE - Use all managed data identifiers except the ones specified by the managedDataIdentifierIds
* property.
*
*
* -
*
* INCLUDE - Use only the managed data identifiers specified by the managedDataIdentifierIds property.
*
*
* -
*
* NONE - Don't use any managed data identifiers. Use only custom data identifiers (customDataIdentifierIds).
*
*
* -
*
* RECOMMENDED - Use only the set of managed data identifiers that Amazon Web Services recommends for jobs.
*
*
*
*
* If this value is null, the job uses all managed data identifiers.
*
*
* If the job is a recurring job and this value is null, ALL, or EXCLUDE, each job run automatically uses new
* managed data identifiers that are released after the job was created or the preceding run ended. If this
* value is RECOMMENDED for a recurring job, each job run uses all the managed data identifiers that are in
* the recommended set when the run starts.
*
*
* For information about individual managed data identifiers or to determine which ones are in the
* recommended set, see Using managed data
* identifiers and Recommended
* managed data identifiers in the Amazon Macie User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ManagedDataIdentifierSelector
*/
public DescribeClassificationJobResult withManagedDataIdentifierSelector(ManagedDataIdentifierSelector managedDataIdentifierSelector) {
this.managedDataIdentifierSelector = managedDataIdentifierSelector.toString();
return this;
}
/**
*
* The custom name of the job.
*
*
* @param name
* The custom name of the job.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The custom name of the job.
*
*
* @return The custom name of the job.
*/
public String getName() {
return this.name;
}
/**
*
* The custom name of the job.
*
*
* @param name
* The custom name of the job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeClassificationJobResult withName(String name) {
setName(name);
return this;
}
/**
*
* The S3 buckets that contain the objects to analyze, and the scope of that analysis.
*
*
* @param s3JobDefinition
* The S3 buckets that contain the objects to analyze, and the scope of that analysis.
*/
public void setS3JobDefinition(S3JobDefinition s3JobDefinition) {
this.s3JobDefinition = s3JobDefinition;
}
/**
*
* The S3 buckets that contain the objects to analyze, and the scope of that analysis.
*
*
* @return The S3 buckets that contain the objects to analyze, and the scope of that analysis.
*/
public S3JobDefinition getS3JobDefinition() {
return this.s3JobDefinition;
}
/**
*
* The S3 buckets that contain the objects to analyze, and the scope of that analysis.
*
*
* @param s3JobDefinition
* The S3 buckets that contain the objects to analyze, and the scope of that analysis.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeClassificationJobResult withS3JobDefinition(S3JobDefinition s3JobDefinition) {
setS3JobDefinition(s3JobDefinition);
return this;
}
/**
*
* The sampling depth, as a percentage, that determines the percentage of eligible objects that the job analyzes.
*
*
* @param samplingPercentage
* The sampling depth, as a percentage, that determines the percentage of eligible objects that the job
* analyzes.
*/
public void setSamplingPercentage(Integer samplingPercentage) {
this.samplingPercentage = samplingPercentage;
}
/**
*
* The sampling depth, as a percentage, that determines the percentage of eligible objects that the job analyzes.
*
*
* @return The sampling depth, as a percentage, that determines the percentage of eligible objects that the job
* analyzes.
*/
public Integer getSamplingPercentage() {
return this.samplingPercentage;
}
/**
*
* The sampling depth, as a percentage, that determines the percentage of eligible objects that the job analyzes.
*
*
* @param samplingPercentage
* The sampling depth, as a percentage, that determines the percentage of eligible objects that the job
* analyzes.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeClassificationJobResult withSamplingPercentage(Integer samplingPercentage) {
setSamplingPercentage(samplingPercentage);
return this;
}
/**
*
* The recurrence pattern for running the job. This value is null if the job is configured to run only once.
*
*
* @param scheduleFrequency
* The recurrence pattern for running the job. This value is null if the job is configured to run only once.
*/
public void setScheduleFrequency(JobScheduleFrequency scheduleFrequency) {
this.scheduleFrequency = scheduleFrequency;
}
/**
*
* The recurrence pattern for running the job. This value is null if the job is configured to run only once.
*
*
* @return The recurrence pattern for running the job. This value is null if the job is configured to run only once.
*/
public JobScheduleFrequency getScheduleFrequency() {
return this.scheduleFrequency;
}
/**
*
* The recurrence pattern for running the job. This value is null if the job is configured to run only once.
*
*
* @param scheduleFrequency
* The recurrence pattern for running the job. This value is null if the job is configured to run only once.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeClassificationJobResult withScheduleFrequency(JobScheduleFrequency scheduleFrequency) {
setScheduleFrequency(scheduleFrequency);
return this;
}
/**
*
* The number of times that the job has run and processing statistics for the job's current run.
*
*
* @param statistics
* The number of times that the job has run and processing statistics for the job's current run.
*/
public void setStatistics(Statistics statistics) {
this.statistics = statistics;
}
/**
*
* The number of times that the job has run and processing statistics for the job's current run.
*
*
* @return The number of times that the job has run and processing statistics for the job's current run.
*/
public Statistics getStatistics() {
return this.statistics;
}
/**
*
* The number of times that the job has run and processing statistics for the job's current run.
*
*
* @param statistics
* The number of times that the job has run and processing statistics for the job's current run.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeClassificationJobResult withStatistics(Statistics statistics) {
setStatistics(statistics);
return this;
}
/**
*
* A map of key-value pairs that specifies which tags (keys and values) are associated with the classification job.
*
*
* @return A map of key-value pairs that specifies which tags (keys and values) are associated with the
* classification job.
*/
public java.util.Map getTags() {
return tags;
}
/**
*
* A map of key-value pairs that specifies which tags (keys and values) are associated with the classification job.
*
*
* @param tags
* A map of key-value pairs that specifies which tags (keys and values) are associated with the
* classification job.
*/
public void setTags(java.util.Map tags) {
this.tags = tags;
}
/**
*
* A map of key-value pairs that specifies which tags (keys and values) are associated with the classification job.
*
*
* @param tags
* A map of key-value pairs that specifies which tags (keys and values) are associated with the
* classification job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeClassificationJobResult withTags(java.util.Map tags) {
setTags(tags);
return this;
}
/**
* Add a single Tags entry
*
* @see DescribeClassificationJobResult#withTags
* @returns a reference to this object so that method calls can be chained together.
*/
public DescribeClassificationJobResult addTagsEntry(String key, String value) {
if (null == this.tags) {
this.tags = new java.util.HashMap();
}
if (this.tags.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.tags.put(key, value);
return this;
}
/**
* Removes all the entries added into Tags.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeClassificationJobResult clearTagsEntries() {
this.tags = null;
return this;
}
/**
*
* If the current status of the job is USER_PAUSED, specifies when the job was paused and when the job or job run
* will expire and be cancelled if it isn't resumed. This value is present only if the value for jobStatus is
* USER_PAUSED.
*
*
* @param userPausedDetails
* If the current status of the job is USER_PAUSED, specifies when the job was paused and when the job or job
* run will expire and be cancelled if it isn't resumed. This value is present only if the value for
* jobStatus is USER_PAUSED.
*/
public void setUserPausedDetails(UserPausedDetails userPausedDetails) {
this.userPausedDetails = userPausedDetails;
}
/**
*
* If the current status of the job is USER_PAUSED, specifies when the job was paused and when the job or job run
* will expire and be cancelled if it isn't resumed. This value is present only if the value for jobStatus is
* USER_PAUSED.
*
*
* @return If the current status of the job is USER_PAUSED, specifies when the job was paused and when the job or
* job run will expire and be cancelled if it isn't resumed. This value is present only if the value for
* jobStatus is USER_PAUSED.
*/
public UserPausedDetails getUserPausedDetails() {
return this.userPausedDetails;
}
/**
*
* If the current status of the job is USER_PAUSED, specifies when the job was paused and when the job or job run
* will expire and be cancelled if it isn't resumed. This value is present only if the value for jobStatus is
* USER_PAUSED.
*
*
* @param userPausedDetails
* If the current status of the job is USER_PAUSED, specifies when the job was paused and when the job or job
* run will expire and be cancelled if it isn't resumed. This value is present only if the value for
* jobStatus is USER_PAUSED.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeClassificationJobResult withUserPausedDetails(UserPausedDetails userPausedDetails) {
setUserPausedDetails(userPausedDetails);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getAllowListIds() != null)
sb.append("AllowListIds: ").append(getAllowListIds()).append(",");
if (getClientToken() != null)
sb.append("ClientToken: ").append(getClientToken()).append(",");
if (getCreatedAt() != null)
sb.append("CreatedAt: ").append(getCreatedAt()).append(",");
if (getCustomDataIdentifierIds() != null)
sb.append("CustomDataIdentifierIds: ").append(getCustomDataIdentifierIds()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getInitialRun() != null)
sb.append("InitialRun: ").append(getInitialRun()).append(",");
if (getJobArn() != null)
sb.append("JobArn: ").append(getJobArn()).append(",");
if (getJobId() != null)
sb.append("JobId: ").append(getJobId()).append(",");
if (getJobStatus() != null)
sb.append("JobStatus: ").append(getJobStatus()).append(",");
if (getJobType() != null)
sb.append("JobType: ").append(getJobType()).append(",");
if (getLastRunErrorStatus() != null)
sb.append("LastRunErrorStatus: ").append(getLastRunErrorStatus()).append(",");
if (getLastRunTime() != null)
sb.append("LastRunTime: ").append(getLastRunTime()).append(",");
if (getManagedDataIdentifierIds() != null)
sb.append("ManagedDataIdentifierIds: ").append(getManagedDataIdentifierIds()).append(",");
if (getManagedDataIdentifierSelector() != null)
sb.append("ManagedDataIdentifierSelector: ").append(getManagedDataIdentifierSelector()).append(",");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getS3JobDefinition() != null)
sb.append("S3JobDefinition: ").append(getS3JobDefinition()).append(",");
if (getSamplingPercentage() != null)
sb.append("SamplingPercentage: ").append(getSamplingPercentage()).append(",");
if (getScheduleFrequency() != null)
sb.append("ScheduleFrequency: ").append(getScheduleFrequency()).append(",");
if (getStatistics() != null)
sb.append("Statistics: ").append(getStatistics()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags()).append(",");
if (getUserPausedDetails() != null)
sb.append("UserPausedDetails: ").append(getUserPausedDetails());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof DescribeClassificationJobResult == false)
return false;
DescribeClassificationJobResult other = (DescribeClassificationJobResult) obj;
if (other.getAllowListIds() == null ^ this.getAllowListIds() == null)
return false;
if (other.getAllowListIds() != null && other.getAllowListIds().equals(this.getAllowListIds()) == false)
return false;
if (other.getClientToken() == null ^ this.getClientToken() == null)
return false;
if (other.getClientToken() != null && other.getClientToken().equals(this.getClientToken()) == false)
return false;
if (other.getCreatedAt() == null ^ this.getCreatedAt() == null)
return false;
if (other.getCreatedAt() != null && other.getCreatedAt().equals(this.getCreatedAt()) == false)
return false;
if (other.getCustomDataIdentifierIds() == null ^ this.getCustomDataIdentifierIds() == null)
return false;
if (other.getCustomDataIdentifierIds() != null && other.getCustomDataIdentifierIds().equals(this.getCustomDataIdentifierIds()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getInitialRun() == null ^ this.getInitialRun() == null)
return false;
if (other.getInitialRun() != null && other.getInitialRun().equals(this.getInitialRun()) == false)
return false;
if (other.getJobArn() == null ^ this.getJobArn() == null)
return false;
if (other.getJobArn() != null && other.getJobArn().equals(this.getJobArn()) == false)
return false;
if (other.getJobId() == null ^ this.getJobId() == null)
return false;
if (other.getJobId() != null && other.getJobId().equals(this.getJobId()) == false)
return false;
if (other.getJobStatus() == null ^ this.getJobStatus() == null)
return false;
if (other.getJobStatus() != null && other.getJobStatus().equals(this.getJobStatus()) == false)
return false;
if (other.getJobType() == null ^ this.getJobType() == null)
return false;
if (other.getJobType() != null && other.getJobType().equals(this.getJobType()) == false)
return false;
if (other.getLastRunErrorStatus() == null ^ this.getLastRunErrorStatus() == null)
return false;
if (other.getLastRunErrorStatus() != null && other.getLastRunErrorStatus().equals(this.getLastRunErrorStatus()) == false)
return false;
if (other.getLastRunTime() == null ^ this.getLastRunTime() == null)
return false;
if (other.getLastRunTime() != null && other.getLastRunTime().equals(this.getLastRunTime()) == false)
return false;
if (other.getManagedDataIdentifierIds() == null ^ this.getManagedDataIdentifierIds() == null)
return false;
if (other.getManagedDataIdentifierIds() != null && other.getManagedDataIdentifierIds().equals(this.getManagedDataIdentifierIds()) == false)
return false;
if (other.getManagedDataIdentifierSelector() == null ^ this.getManagedDataIdentifierSelector() == null)
return false;
if (other.getManagedDataIdentifierSelector() != null
&& other.getManagedDataIdentifierSelector().equals(this.getManagedDataIdentifierSelector()) == false)
return false;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getS3JobDefinition() == null ^ this.getS3JobDefinition() == null)
return false;
if (other.getS3JobDefinition() != null && other.getS3JobDefinition().equals(this.getS3JobDefinition()) == false)
return false;
if (other.getSamplingPercentage() == null ^ this.getSamplingPercentage() == null)
return false;
if (other.getSamplingPercentage() != null && other.getSamplingPercentage().equals(this.getSamplingPercentage()) == false)
return false;
if (other.getScheduleFrequency() == null ^ this.getScheduleFrequency() == null)
return false;
if (other.getScheduleFrequency() != null && other.getScheduleFrequency().equals(this.getScheduleFrequency()) == false)
return false;
if (other.getStatistics() == null ^ this.getStatistics() == null)
return false;
if (other.getStatistics() != null && other.getStatistics().equals(this.getStatistics()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
if (other.getUserPausedDetails() == null ^ this.getUserPausedDetails() == null)
return false;
if (other.getUserPausedDetails() != null && other.getUserPausedDetails().equals(this.getUserPausedDetails()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getAllowListIds() == null) ? 0 : getAllowListIds().hashCode());
hashCode = prime * hashCode + ((getClientToken() == null) ? 0 : getClientToken().hashCode());
hashCode = prime * hashCode + ((getCreatedAt() == null) ? 0 : getCreatedAt().hashCode());
hashCode = prime * hashCode + ((getCustomDataIdentifierIds() == null) ? 0 : getCustomDataIdentifierIds().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getInitialRun() == null) ? 0 : getInitialRun().hashCode());
hashCode = prime * hashCode + ((getJobArn() == null) ? 0 : getJobArn().hashCode());
hashCode = prime * hashCode + ((getJobId() == null) ? 0 : getJobId().hashCode());
hashCode = prime * hashCode + ((getJobStatus() == null) ? 0 : getJobStatus().hashCode());
hashCode = prime * hashCode + ((getJobType() == null) ? 0 : getJobType().hashCode());
hashCode = prime * hashCode + ((getLastRunErrorStatus() == null) ? 0 : getLastRunErrorStatus().hashCode());
hashCode = prime * hashCode + ((getLastRunTime() == null) ? 0 : getLastRunTime().hashCode());
hashCode = prime * hashCode + ((getManagedDataIdentifierIds() == null) ? 0 : getManagedDataIdentifierIds().hashCode());
hashCode = prime * hashCode + ((getManagedDataIdentifierSelector() == null) ? 0 : getManagedDataIdentifierSelector().hashCode());
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getS3JobDefinition() == null) ? 0 : getS3JobDefinition().hashCode());
hashCode = prime * hashCode + ((getSamplingPercentage() == null) ? 0 : getSamplingPercentage().hashCode());
hashCode = prime * hashCode + ((getScheduleFrequency() == null) ? 0 : getScheduleFrequency().hashCode());
hashCode = prime * hashCode + ((getStatistics() == null) ? 0 : getStatistics().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
hashCode = prime * hashCode + ((getUserPausedDetails() == null) ? 0 : getUserPausedDetails().hashCode());
return hashCode;
}
@Override
public DescribeClassificationJobResult clone() {
try {
return (DescribeClassificationJobResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}