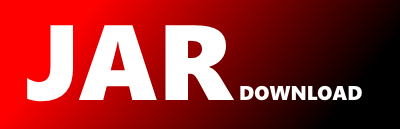
com.amazonaws.services.macie2.model.GetBucketStatisticsResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-macie2 Show documentation
/*
* Copyright 2018-2023 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.macie2.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class GetBucketStatisticsResult extends com.amazonaws.AmazonWebServiceResult implements Serializable, Cloneable {
/**
*
* The total number of buckets.
*
*/
private Long bucketCount;
/**
*
* The total number of buckets that are publicly accessible due to a combination of permissions settings for each
* bucket.
*
*/
private BucketCountByEffectivePermission bucketCountByEffectivePermission;
/**
*
* The total number of buckets whose settings do or don't specify default server-side encryption behavior for
* objects that are added to the buckets.
*
*/
private BucketCountByEncryptionType bucketCountByEncryptionType;
/**
*
* The total number of buckets whose bucket policies do or don't require server-side encryption of objects when
* objects are added to the buckets.
*
*/
private BucketCountPolicyAllowsUnencryptedObjectUploads bucketCountByObjectEncryptionRequirement;
/**
*
* The total number of buckets that are or aren't shared with other Amazon Web Services accounts, Amazon CloudFront
* origin access identities (OAIs), or CloudFront origin access controls (OACs).
*
*/
private BucketCountBySharedAccessType bucketCountBySharedAccessType;
/**
*
* The aggregated sensitive data discovery statistics for the buckets. If automated sensitive data discovery is
* currently disabled for your account, the value for each statistic is 0.
*
*/
private BucketStatisticsBySensitivity bucketStatisticsBySensitivity;
/**
*
* The total number of objects that Amazon Macie can analyze in the buckets. These objects use a supported storage
* class and have a file name extension for a supported file or storage format.
*
*/
private Long classifiableObjectCount;
/**
*
* The total storage size, in bytes, of all the objects that Amazon Macie can analyze in the buckets. These objects
* use a supported storage class and have a file name extension for a supported file or storage format.
*
*
* If versioning is enabled for any of the buckets, this value is based on the size of the latest version of each
* applicable object in the buckets. This value doesn't reflect the storage size of all versions of all applicable
* objects in the buckets.
*
*/
private Long classifiableSizeInBytes;
/**
*
* The date and time, in UTC and extended ISO 8601 format, when Amazon Macie most recently retrieved bucket or
* object metadata from Amazon S3 for the buckets.
*
*/
private java.util.Date lastUpdated;
/**
*
* The total number of objects in the buckets.
*
*/
private Long objectCount;
/**
*
* The total storage size, in bytes, of the buckets.
*
*
* If versioning is enabled for any of the buckets, this value is based on the size of the latest version of each
* object in the buckets. This value doesn't reflect the storage size of all versions of the objects in the buckets.
*
*/
private Long sizeInBytes;
/**
*
* The total storage size, in bytes, of the objects that are compressed (.gz, .gzip, .zip) files in the buckets.
*
*
* If versioning is enabled for any of the buckets, this value is based on the size of the latest version of each
* applicable object in the buckets. This value doesn't reflect the storage size of all versions of the applicable
* objects in the buckets.
*
*/
private Long sizeInBytesCompressed;
/**
*
* The total number of objects that Amazon Macie can't analyze in the buckets. These objects don't use a supported
* storage class or don't have a file name extension for a supported file or storage format.
*
*/
private ObjectLevelStatistics unclassifiableObjectCount;
/**
*
* The total storage size, in bytes, of the objects that Amazon Macie can't analyze in the buckets. These objects
* don't use a supported storage class or don't have a file name extension for a supported file or storage format.
*
*/
private ObjectLevelStatistics unclassifiableObjectSizeInBytes;
/**
*
* The total number of buckets.
*
*
* @param bucketCount
* The total number of buckets.
*/
public void setBucketCount(Long bucketCount) {
this.bucketCount = bucketCount;
}
/**
*
* The total number of buckets.
*
*
* @return The total number of buckets.
*/
public Long getBucketCount() {
return this.bucketCount;
}
/**
*
* The total number of buckets.
*
*
* @param bucketCount
* The total number of buckets.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetBucketStatisticsResult withBucketCount(Long bucketCount) {
setBucketCount(bucketCount);
return this;
}
/**
*
* The total number of buckets that are publicly accessible due to a combination of permissions settings for each
* bucket.
*
*
* @param bucketCountByEffectivePermission
* The total number of buckets that are publicly accessible due to a combination of permissions settings for
* each bucket.
*/
public void setBucketCountByEffectivePermission(BucketCountByEffectivePermission bucketCountByEffectivePermission) {
this.bucketCountByEffectivePermission = bucketCountByEffectivePermission;
}
/**
*
* The total number of buckets that are publicly accessible due to a combination of permissions settings for each
* bucket.
*
*
* @return The total number of buckets that are publicly accessible due to a combination of permissions settings for
* each bucket.
*/
public BucketCountByEffectivePermission getBucketCountByEffectivePermission() {
return this.bucketCountByEffectivePermission;
}
/**
*
* The total number of buckets that are publicly accessible due to a combination of permissions settings for each
* bucket.
*
*
* @param bucketCountByEffectivePermission
* The total number of buckets that are publicly accessible due to a combination of permissions settings for
* each bucket.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetBucketStatisticsResult withBucketCountByEffectivePermission(BucketCountByEffectivePermission bucketCountByEffectivePermission) {
setBucketCountByEffectivePermission(bucketCountByEffectivePermission);
return this;
}
/**
*
* The total number of buckets whose settings do or don't specify default server-side encryption behavior for
* objects that are added to the buckets.
*
*
* @param bucketCountByEncryptionType
* The total number of buckets whose settings do or don't specify default server-side encryption behavior for
* objects that are added to the buckets.
*/
public void setBucketCountByEncryptionType(BucketCountByEncryptionType bucketCountByEncryptionType) {
this.bucketCountByEncryptionType = bucketCountByEncryptionType;
}
/**
*
* The total number of buckets whose settings do or don't specify default server-side encryption behavior for
* objects that are added to the buckets.
*
*
* @return The total number of buckets whose settings do or don't specify default server-side encryption behavior
* for objects that are added to the buckets.
*/
public BucketCountByEncryptionType getBucketCountByEncryptionType() {
return this.bucketCountByEncryptionType;
}
/**
*
* The total number of buckets whose settings do or don't specify default server-side encryption behavior for
* objects that are added to the buckets.
*
*
* @param bucketCountByEncryptionType
* The total number of buckets whose settings do or don't specify default server-side encryption behavior for
* objects that are added to the buckets.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetBucketStatisticsResult withBucketCountByEncryptionType(BucketCountByEncryptionType bucketCountByEncryptionType) {
setBucketCountByEncryptionType(bucketCountByEncryptionType);
return this;
}
/**
*
* The total number of buckets whose bucket policies do or don't require server-side encryption of objects when
* objects are added to the buckets.
*
*
* @param bucketCountByObjectEncryptionRequirement
* The total number of buckets whose bucket policies do or don't require server-side encryption of objects
* when objects are added to the buckets.
*/
public void setBucketCountByObjectEncryptionRequirement(BucketCountPolicyAllowsUnencryptedObjectUploads bucketCountByObjectEncryptionRequirement) {
this.bucketCountByObjectEncryptionRequirement = bucketCountByObjectEncryptionRequirement;
}
/**
*
* The total number of buckets whose bucket policies do or don't require server-side encryption of objects when
* objects are added to the buckets.
*
*
* @return The total number of buckets whose bucket policies do or don't require server-side encryption of objects
* when objects are added to the buckets.
*/
public BucketCountPolicyAllowsUnencryptedObjectUploads getBucketCountByObjectEncryptionRequirement() {
return this.bucketCountByObjectEncryptionRequirement;
}
/**
*
* The total number of buckets whose bucket policies do or don't require server-side encryption of objects when
* objects are added to the buckets.
*
*
* @param bucketCountByObjectEncryptionRequirement
* The total number of buckets whose bucket policies do or don't require server-side encryption of objects
* when objects are added to the buckets.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetBucketStatisticsResult withBucketCountByObjectEncryptionRequirement(
BucketCountPolicyAllowsUnencryptedObjectUploads bucketCountByObjectEncryptionRequirement) {
setBucketCountByObjectEncryptionRequirement(bucketCountByObjectEncryptionRequirement);
return this;
}
/**
*
* The total number of buckets that are or aren't shared with other Amazon Web Services accounts, Amazon CloudFront
* origin access identities (OAIs), or CloudFront origin access controls (OACs).
*
*
* @param bucketCountBySharedAccessType
* The total number of buckets that are or aren't shared with other Amazon Web Services accounts, Amazon
* CloudFront origin access identities (OAIs), or CloudFront origin access controls (OACs).
*/
public void setBucketCountBySharedAccessType(BucketCountBySharedAccessType bucketCountBySharedAccessType) {
this.bucketCountBySharedAccessType = bucketCountBySharedAccessType;
}
/**
*
* The total number of buckets that are or aren't shared with other Amazon Web Services accounts, Amazon CloudFront
* origin access identities (OAIs), or CloudFront origin access controls (OACs).
*
*
* @return The total number of buckets that are or aren't shared with other Amazon Web Services accounts, Amazon
* CloudFront origin access identities (OAIs), or CloudFront origin access controls (OACs).
*/
public BucketCountBySharedAccessType getBucketCountBySharedAccessType() {
return this.bucketCountBySharedAccessType;
}
/**
*
* The total number of buckets that are or aren't shared with other Amazon Web Services accounts, Amazon CloudFront
* origin access identities (OAIs), or CloudFront origin access controls (OACs).
*
*
* @param bucketCountBySharedAccessType
* The total number of buckets that are or aren't shared with other Amazon Web Services accounts, Amazon
* CloudFront origin access identities (OAIs), or CloudFront origin access controls (OACs).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetBucketStatisticsResult withBucketCountBySharedAccessType(BucketCountBySharedAccessType bucketCountBySharedAccessType) {
setBucketCountBySharedAccessType(bucketCountBySharedAccessType);
return this;
}
/**
*
* The aggregated sensitive data discovery statistics for the buckets. If automated sensitive data discovery is
* currently disabled for your account, the value for each statistic is 0.
*
*
* @param bucketStatisticsBySensitivity
* The aggregated sensitive data discovery statistics for the buckets. If automated sensitive data discovery
* is currently disabled for your account, the value for each statistic is 0.
*/
public void setBucketStatisticsBySensitivity(BucketStatisticsBySensitivity bucketStatisticsBySensitivity) {
this.bucketStatisticsBySensitivity = bucketStatisticsBySensitivity;
}
/**
*
* The aggregated sensitive data discovery statistics for the buckets. If automated sensitive data discovery is
* currently disabled for your account, the value for each statistic is 0.
*
*
* @return The aggregated sensitive data discovery statistics for the buckets. If automated sensitive data discovery
* is currently disabled for your account, the value for each statistic is 0.
*/
public BucketStatisticsBySensitivity getBucketStatisticsBySensitivity() {
return this.bucketStatisticsBySensitivity;
}
/**
*
* The aggregated sensitive data discovery statistics for the buckets. If automated sensitive data discovery is
* currently disabled for your account, the value for each statistic is 0.
*
*
* @param bucketStatisticsBySensitivity
* The aggregated sensitive data discovery statistics for the buckets. If automated sensitive data discovery
* is currently disabled for your account, the value for each statistic is 0.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetBucketStatisticsResult withBucketStatisticsBySensitivity(BucketStatisticsBySensitivity bucketStatisticsBySensitivity) {
setBucketStatisticsBySensitivity(bucketStatisticsBySensitivity);
return this;
}
/**
*
* The total number of objects that Amazon Macie can analyze in the buckets. These objects use a supported storage
* class and have a file name extension for a supported file or storage format.
*
*
* @param classifiableObjectCount
* The total number of objects that Amazon Macie can analyze in the buckets. These objects use a supported
* storage class and have a file name extension for a supported file or storage format.
*/
public void setClassifiableObjectCount(Long classifiableObjectCount) {
this.classifiableObjectCount = classifiableObjectCount;
}
/**
*
* The total number of objects that Amazon Macie can analyze in the buckets. These objects use a supported storage
* class and have a file name extension for a supported file or storage format.
*
*
* @return The total number of objects that Amazon Macie can analyze in the buckets. These objects use a supported
* storage class and have a file name extension for a supported file or storage format.
*/
public Long getClassifiableObjectCount() {
return this.classifiableObjectCount;
}
/**
*
* The total number of objects that Amazon Macie can analyze in the buckets. These objects use a supported storage
* class and have a file name extension for a supported file or storage format.
*
*
* @param classifiableObjectCount
* The total number of objects that Amazon Macie can analyze in the buckets. These objects use a supported
* storage class and have a file name extension for a supported file or storage format.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetBucketStatisticsResult withClassifiableObjectCount(Long classifiableObjectCount) {
setClassifiableObjectCount(classifiableObjectCount);
return this;
}
/**
*
* The total storage size, in bytes, of all the objects that Amazon Macie can analyze in the buckets. These objects
* use a supported storage class and have a file name extension for a supported file or storage format.
*
*
* If versioning is enabled for any of the buckets, this value is based on the size of the latest version of each
* applicable object in the buckets. This value doesn't reflect the storage size of all versions of all applicable
* objects in the buckets.
*
*
* @param classifiableSizeInBytes
* The total storage size, in bytes, of all the objects that Amazon Macie can analyze in the buckets. These
* objects use a supported storage class and have a file name extension for a supported file or storage
* format.
*
* If versioning is enabled for any of the buckets, this value is based on the size of the latest version of
* each applicable object in the buckets. This value doesn't reflect the storage size of all versions of all
* applicable objects in the buckets.
*/
public void setClassifiableSizeInBytes(Long classifiableSizeInBytes) {
this.classifiableSizeInBytes = classifiableSizeInBytes;
}
/**
*
* The total storage size, in bytes, of all the objects that Amazon Macie can analyze in the buckets. These objects
* use a supported storage class and have a file name extension for a supported file or storage format.
*
*
* If versioning is enabled for any of the buckets, this value is based on the size of the latest version of each
* applicable object in the buckets. This value doesn't reflect the storage size of all versions of all applicable
* objects in the buckets.
*
*
* @return The total storage size, in bytes, of all the objects that Amazon Macie can analyze in the buckets. These
* objects use a supported storage class and have a file name extension for a supported file or storage
* format.
*
* If versioning is enabled for any of the buckets, this value is based on the size of the latest version of
* each applicable object in the buckets. This value doesn't reflect the storage size of all versions of all
* applicable objects in the buckets.
*/
public Long getClassifiableSizeInBytes() {
return this.classifiableSizeInBytes;
}
/**
*
* The total storage size, in bytes, of all the objects that Amazon Macie can analyze in the buckets. These objects
* use a supported storage class and have a file name extension for a supported file or storage format.
*
*
* If versioning is enabled for any of the buckets, this value is based on the size of the latest version of each
* applicable object in the buckets. This value doesn't reflect the storage size of all versions of all applicable
* objects in the buckets.
*
*
* @param classifiableSizeInBytes
* The total storage size, in bytes, of all the objects that Amazon Macie can analyze in the buckets. These
* objects use a supported storage class and have a file name extension for a supported file or storage
* format.
*
* If versioning is enabled for any of the buckets, this value is based on the size of the latest version of
* each applicable object in the buckets. This value doesn't reflect the storage size of all versions of all
* applicable objects in the buckets.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetBucketStatisticsResult withClassifiableSizeInBytes(Long classifiableSizeInBytes) {
setClassifiableSizeInBytes(classifiableSizeInBytes);
return this;
}
/**
*
* The date and time, in UTC and extended ISO 8601 format, when Amazon Macie most recently retrieved bucket or
* object metadata from Amazon S3 for the buckets.
*
*
* @param lastUpdated
* The date and time, in UTC and extended ISO 8601 format, when Amazon Macie most recently retrieved bucket
* or object metadata from Amazon S3 for the buckets.
*/
public void setLastUpdated(java.util.Date lastUpdated) {
this.lastUpdated = lastUpdated;
}
/**
*
* The date and time, in UTC and extended ISO 8601 format, when Amazon Macie most recently retrieved bucket or
* object metadata from Amazon S3 for the buckets.
*
*
* @return The date and time, in UTC and extended ISO 8601 format, when Amazon Macie most recently retrieved bucket
* or object metadata from Amazon S3 for the buckets.
*/
public java.util.Date getLastUpdated() {
return this.lastUpdated;
}
/**
*
* The date and time, in UTC and extended ISO 8601 format, when Amazon Macie most recently retrieved bucket or
* object metadata from Amazon S3 for the buckets.
*
*
* @param lastUpdated
* The date and time, in UTC and extended ISO 8601 format, when Amazon Macie most recently retrieved bucket
* or object metadata from Amazon S3 for the buckets.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetBucketStatisticsResult withLastUpdated(java.util.Date lastUpdated) {
setLastUpdated(lastUpdated);
return this;
}
/**
*
* The total number of objects in the buckets.
*
*
* @param objectCount
* The total number of objects in the buckets.
*/
public void setObjectCount(Long objectCount) {
this.objectCount = objectCount;
}
/**
*
* The total number of objects in the buckets.
*
*
* @return The total number of objects in the buckets.
*/
public Long getObjectCount() {
return this.objectCount;
}
/**
*
* The total number of objects in the buckets.
*
*
* @param objectCount
* The total number of objects in the buckets.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetBucketStatisticsResult withObjectCount(Long objectCount) {
setObjectCount(objectCount);
return this;
}
/**
*
* The total storage size, in bytes, of the buckets.
*
*
* If versioning is enabled for any of the buckets, this value is based on the size of the latest version of each
* object in the buckets. This value doesn't reflect the storage size of all versions of the objects in the buckets.
*
*
* @param sizeInBytes
* The total storage size, in bytes, of the buckets.
*
* If versioning is enabled for any of the buckets, this value is based on the size of the latest version of
* each object in the buckets. This value doesn't reflect the storage size of all versions of the objects in
* the buckets.
*/
public void setSizeInBytes(Long sizeInBytes) {
this.sizeInBytes = sizeInBytes;
}
/**
*
* The total storage size, in bytes, of the buckets.
*
*
* If versioning is enabled for any of the buckets, this value is based on the size of the latest version of each
* object in the buckets. This value doesn't reflect the storage size of all versions of the objects in the buckets.
*
*
* @return The total storage size, in bytes, of the buckets.
*
* If versioning is enabled for any of the buckets, this value is based on the size of the latest version of
* each object in the buckets. This value doesn't reflect the storage size of all versions of the objects in
* the buckets.
*/
public Long getSizeInBytes() {
return this.sizeInBytes;
}
/**
*
* The total storage size, in bytes, of the buckets.
*
*
* If versioning is enabled for any of the buckets, this value is based on the size of the latest version of each
* object in the buckets. This value doesn't reflect the storage size of all versions of the objects in the buckets.
*
*
* @param sizeInBytes
* The total storage size, in bytes, of the buckets.
*
* If versioning is enabled for any of the buckets, this value is based on the size of the latest version of
* each object in the buckets. This value doesn't reflect the storage size of all versions of the objects in
* the buckets.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetBucketStatisticsResult withSizeInBytes(Long sizeInBytes) {
setSizeInBytes(sizeInBytes);
return this;
}
/**
*
* The total storage size, in bytes, of the objects that are compressed (.gz, .gzip, .zip) files in the buckets.
*
*
* If versioning is enabled for any of the buckets, this value is based on the size of the latest version of each
* applicable object in the buckets. This value doesn't reflect the storage size of all versions of the applicable
* objects in the buckets.
*
*
* @param sizeInBytesCompressed
* The total storage size, in bytes, of the objects that are compressed (.gz, .gzip, .zip) files in the
* buckets.
*
* If versioning is enabled for any of the buckets, this value is based on the size of the latest version of
* each applicable object in the buckets. This value doesn't reflect the storage size of all versions of the
* applicable objects in the buckets.
*/
public void setSizeInBytesCompressed(Long sizeInBytesCompressed) {
this.sizeInBytesCompressed = sizeInBytesCompressed;
}
/**
*
* The total storage size, in bytes, of the objects that are compressed (.gz, .gzip, .zip) files in the buckets.
*
*
* If versioning is enabled for any of the buckets, this value is based on the size of the latest version of each
* applicable object in the buckets. This value doesn't reflect the storage size of all versions of the applicable
* objects in the buckets.
*
*
* @return The total storage size, in bytes, of the objects that are compressed (.gz, .gzip, .zip) files in the
* buckets.
*
* If versioning is enabled for any of the buckets, this value is based on the size of the latest version of
* each applicable object in the buckets. This value doesn't reflect the storage size of all versions of the
* applicable objects in the buckets.
*/
public Long getSizeInBytesCompressed() {
return this.sizeInBytesCompressed;
}
/**
*
* The total storage size, in bytes, of the objects that are compressed (.gz, .gzip, .zip) files in the buckets.
*
*
* If versioning is enabled for any of the buckets, this value is based on the size of the latest version of each
* applicable object in the buckets. This value doesn't reflect the storage size of all versions of the applicable
* objects in the buckets.
*
*
* @param sizeInBytesCompressed
* The total storage size, in bytes, of the objects that are compressed (.gz, .gzip, .zip) files in the
* buckets.
*
* If versioning is enabled for any of the buckets, this value is based on the size of the latest version of
* each applicable object in the buckets. This value doesn't reflect the storage size of all versions of the
* applicable objects in the buckets.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetBucketStatisticsResult withSizeInBytesCompressed(Long sizeInBytesCompressed) {
setSizeInBytesCompressed(sizeInBytesCompressed);
return this;
}
/**
*
* The total number of objects that Amazon Macie can't analyze in the buckets. These objects don't use a supported
* storage class or don't have a file name extension for a supported file or storage format.
*
*
* @param unclassifiableObjectCount
* The total number of objects that Amazon Macie can't analyze in the buckets. These objects don't use a
* supported storage class or don't have a file name extension for a supported file or storage format.
*/
public void setUnclassifiableObjectCount(ObjectLevelStatistics unclassifiableObjectCount) {
this.unclassifiableObjectCount = unclassifiableObjectCount;
}
/**
*
* The total number of objects that Amazon Macie can't analyze in the buckets. These objects don't use a supported
* storage class or don't have a file name extension for a supported file or storage format.
*
*
* @return The total number of objects that Amazon Macie can't analyze in the buckets. These objects don't use a
* supported storage class or don't have a file name extension for a supported file or storage format.
*/
public ObjectLevelStatistics getUnclassifiableObjectCount() {
return this.unclassifiableObjectCount;
}
/**
*
* The total number of objects that Amazon Macie can't analyze in the buckets. These objects don't use a supported
* storage class or don't have a file name extension for a supported file or storage format.
*
*
* @param unclassifiableObjectCount
* The total number of objects that Amazon Macie can't analyze in the buckets. These objects don't use a
* supported storage class or don't have a file name extension for a supported file or storage format.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetBucketStatisticsResult withUnclassifiableObjectCount(ObjectLevelStatistics unclassifiableObjectCount) {
setUnclassifiableObjectCount(unclassifiableObjectCount);
return this;
}
/**
*
* The total storage size, in bytes, of the objects that Amazon Macie can't analyze in the buckets. These objects
* don't use a supported storage class or don't have a file name extension for a supported file or storage format.
*
*
* @param unclassifiableObjectSizeInBytes
* The total storage size, in bytes, of the objects that Amazon Macie can't analyze in the buckets. These
* objects don't use a supported storage class or don't have a file name extension for a supported file or
* storage format.
*/
public void setUnclassifiableObjectSizeInBytes(ObjectLevelStatistics unclassifiableObjectSizeInBytes) {
this.unclassifiableObjectSizeInBytes = unclassifiableObjectSizeInBytes;
}
/**
*
* The total storage size, in bytes, of the objects that Amazon Macie can't analyze in the buckets. These objects
* don't use a supported storage class or don't have a file name extension for a supported file or storage format.
*
*
* @return The total storage size, in bytes, of the objects that Amazon Macie can't analyze in the buckets. These
* objects don't use a supported storage class or don't have a file name extension for a supported file or
* storage format.
*/
public ObjectLevelStatistics getUnclassifiableObjectSizeInBytes() {
return this.unclassifiableObjectSizeInBytes;
}
/**
*
* The total storage size, in bytes, of the objects that Amazon Macie can't analyze in the buckets. These objects
* don't use a supported storage class or don't have a file name extension for a supported file or storage format.
*
*
* @param unclassifiableObjectSizeInBytes
* The total storage size, in bytes, of the objects that Amazon Macie can't analyze in the buckets. These
* objects don't use a supported storage class or don't have a file name extension for a supported file or
* storage format.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetBucketStatisticsResult withUnclassifiableObjectSizeInBytes(ObjectLevelStatistics unclassifiableObjectSizeInBytes) {
setUnclassifiableObjectSizeInBytes(unclassifiableObjectSizeInBytes);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getBucketCount() != null)
sb.append("BucketCount: ").append(getBucketCount()).append(",");
if (getBucketCountByEffectivePermission() != null)
sb.append("BucketCountByEffectivePermission: ").append(getBucketCountByEffectivePermission()).append(",");
if (getBucketCountByEncryptionType() != null)
sb.append("BucketCountByEncryptionType: ").append(getBucketCountByEncryptionType()).append(",");
if (getBucketCountByObjectEncryptionRequirement() != null)
sb.append("BucketCountByObjectEncryptionRequirement: ").append(getBucketCountByObjectEncryptionRequirement()).append(",");
if (getBucketCountBySharedAccessType() != null)
sb.append("BucketCountBySharedAccessType: ").append(getBucketCountBySharedAccessType()).append(",");
if (getBucketStatisticsBySensitivity() != null)
sb.append("BucketStatisticsBySensitivity: ").append(getBucketStatisticsBySensitivity()).append(",");
if (getClassifiableObjectCount() != null)
sb.append("ClassifiableObjectCount: ").append(getClassifiableObjectCount()).append(",");
if (getClassifiableSizeInBytes() != null)
sb.append("ClassifiableSizeInBytes: ").append(getClassifiableSizeInBytes()).append(",");
if (getLastUpdated() != null)
sb.append("LastUpdated: ").append(getLastUpdated()).append(",");
if (getObjectCount() != null)
sb.append("ObjectCount: ").append(getObjectCount()).append(",");
if (getSizeInBytes() != null)
sb.append("SizeInBytes: ").append(getSizeInBytes()).append(",");
if (getSizeInBytesCompressed() != null)
sb.append("SizeInBytesCompressed: ").append(getSizeInBytesCompressed()).append(",");
if (getUnclassifiableObjectCount() != null)
sb.append("UnclassifiableObjectCount: ").append(getUnclassifiableObjectCount()).append(",");
if (getUnclassifiableObjectSizeInBytes() != null)
sb.append("UnclassifiableObjectSizeInBytes: ").append(getUnclassifiableObjectSizeInBytes());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof GetBucketStatisticsResult == false)
return false;
GetBucketStatisticsResult other = (GetBucketStatisticsResult) obj;
if (other.getBucketCount() == null ^ this.getBucketCount() == null)
return false;
if (other.getBucketCount() != null && other.getBucketCount().equals(this.getBucketCount()) == false)
return false;
if (other.getBucketCountByEffectivePermission() == null ^ this.getBucketCountByEffectivePermission() == null)
return false;
if (other.getBucketCountByEffectivePermission() != null
&& other.getBucketCountByEffectivePermission().equals(this.getBucketCountByEffectivePermission()) == false)
return false;
if (other.getBucketCountByEncryptionType() == null ^ this.getBucketCountByEncryptionType() == null)
return false;
if (other.getBucketCountByEncryptionType() != null && other.getBucketCountByEncryptionType().equals(this.getBucketCountByEncryptionType()) == false)
return false;
if (other.getBucketCountByObjectEncryptionRequirement() == null ^ this.getBucketCountByObjectEncryptionRequirement() == null)
return false;
if (other.getBucketCountByObjectEncryptionRequirement() != null
&& other.getBucketCountByObjectEncryptionRequirement().equals(this.getBucketCountByObjectEncryptionRequirement()) == false)
return false;
if (other.getBucketCountBySharedAccessType() == null ^ this.getBucketCountBySharedAccessType() == null)
return false;
if (other.getBucketCountBySharedAccessType() != null
&& other.getBucketCountBySharedAccessType().equals(this.getBucketCountBySharedAccessType()) == false)
return false;
if (other.getBucketStatisticsBySensitivity() == null ^ this.getBucketStatisticsBySensitivity() == null)
return false;
if (other.getBucketStatisticsBySensitivity() != null
&& other.getBucketStatisticsBySensitivity().equals(this.getBucketStatisticsBySensitivity()) == false)
return false;
if (other.getClassifiableObjectCount() == null ^ this.getClassifiableObjectCount() == null)
return false;
if (other.getClassifiableObjectCount() != null && other.getClassifiableObjectCount().equals(this.getClassifiableObjectCount()) == false)
return false;
if (other.getClassifiableSizeInBytes() == null ^ this.getClassifiableSizeInBytes() == null)
return false;
if (other.getClassifiableSizeInBytes() != null && other.getClassifiableSizeInBytes().equals(this.getClassifiableSizeInBytes()) == false)
return false;
if (other.getLastUpdated() == null ^ this.getLastUpdated() == null)
return false;
if (other.getLastUpdated() != null && other.getLastUpdated().equals(this.getLastUpdated()) == false)
return false;
if (other.getObjectCount() == null ^ this.getObjectCount() == null)
return false;
if (other.getObjectCount() != null && other.getObjectCount().equals(this.getObjectCount()) == false)
return false;
if (other.getSizeInBytes() == null ^ this.getSizeInBytes() == null)
return false;
if (other.getSizeInBytes() != null && other.getSizeInBytes().equals(this.getSizeInBytes()) == false)
return false;
if (other.getSizeInBytesCompressed() == null ^ this.getSizeInBytesCompressed() == null)
return false;
if (other.getSizeInBytesCompressed() != null && other.getSizeInBytesCompressed().equals(this.getSizeInBytesCompressed()) == false)
return false;
if (other.getUnclassifiableObjectCount() == null ^ this.getUnclassifiableObjectCount() == null)
return false;
if (other.getUnclassifiableObjectCount() != null && other.getUnclassifiableObjectCount().equals(this.getUnclassifiableObjectCount()) == false)
return false;
if (other.getUnclassifiableObjectSizeInBytes() == null ^ this.getUnclassifiableObjectSizeInBytes() == null)
return false;
if (other.getUnclassifiableObjectSizeInBytes() != null
&& other.getUnclassifiableObjectSizeInBytes().equals(this.getUnclassifiableObjectSizeInBytes()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getBucketCount() == null) ? 0 : getBucketCount().hashCode());
hashCode = prime * hashCode + ((getBucketCountByEffectivePermission() == null) ? 0 : getBucketCountByEffectivePermission().hashCode());
hashCode = prime * hashCode + ((getBucketCountByEncryptionType() == null) ? 0 : getBucketCountByEncryptionType().hashCode());
hashCode = prime * hashCode + ((getBucketCountByObjectEncryptionRequirement() == null) ? 0 : getBucketCountByObjectEncryptionRequirement().hashCode());
hashCode = prime * hashCode + ((getBucketCountBySharedAccessType() == null) ? 0 : getBucketCountBySharedAccessType().hashCode());
hashCode = prime * hashCode + ((getBucketStatisticsBySensitivity() == null) ? 0 : getBucketStatisticsBySensitivity().hashCode());
hashCode = prime * hashCode + ((getClassifiableObjectCount() == null) ? 0 : getClassifiableObjectCount().hashCode());
hashCode = prime * hashCode + ((getClassifiableSizeInBytes() == null) ? 0 : getClassifiableSizeInBytes().hashCode());
hashCode = prime * hashCode + ((getLastUpdated() == null) ? 0 : getLastUpdated().hashCode());
hashCode = prime * hashCode + ((getObjectCount() == null) ? 0 : getObjectCount().hashCode());
hashCode = prime * hashCode + ((getSizeInBytes() == null) ? 0 : getSizeInBytes().hashCode());
hashCode = prime * hashCode + ((getSizeInBytesCompressed() == null) ? 0 : getSizeInBytesCompressed().hashCode());
hashCode = prime * hashCode + ((getUnclassifiableObjectCount() == null) ? 0 : getUnclassifiableObjectCount().hashCode());
hashCode = prime * hashCode + ((getUnclassifiableObjectSizeInBytes() == null) ? 0 : getUnclassifiableObjectSizeInBytes().hashCode());
return hashCode;
}
@Override
public GetBucketStatisticsResult clone() {
try {
return (GetBucketStatisticsResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}