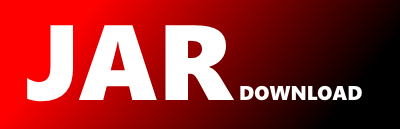
com.amazonaws.services.macie2.model.GetSensitiveDataOccurrencesAvailabilityResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-macie2 Show documentation
/*
* Copyright 2018-2023 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.macie2.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class GetSensitiveDataOccurrencesAvailabilityResult extends com.amazonaws.AmazonWebServiceResult implements
Serializable, Cloneable {
/**
*
* Specifies whether occurrences of sensitive data can be retrieved for the finding. Possible values are: AVAILABLE,
* the sensitive data can be retrieved; and, UNAVAILABLE, the sensitive data can't be retrieved. If this value is
* UNAVAILABLE, the reasons array indicates why the data can't be retrieved.
*
*/
private String code;
/**
*
* Specifies why occurrences of sensitive data can't be retrieved for the finding. Possible values are:
*
*
* -
*
* INVALID_CLASSIFICATION_RESULT - Amazon Macie can't verify the location of the sensitive data to retrieve. There
* isn't a corresponding sensitive data discovery result for the finding. Or the sensitive data discovery result
* specified by the classificationDetails.detailedResultsLocation field of the finding isn't available, is malformed
* or corrupted, or uses an unsupported storage format.
*
*
* -
*
* OBJECT_EXCEEDS_SIZE_QUOTA - The storage size of the affected S3 object exceeds the size quota for retrieving
* occurrences of sensitive data.
*
*
* -
*
* OBJECT_UNAVAILABLE - The affected S3 object isn't available. The object might have been renamed, moved, or
* deleted. Or the object was changed after Macie created the finding.
*
*
* -
*
* UNSUPPORTED_FINDING_TYPE - The specified finding isn't a sensitive data finding.
*
*
* -
*
* UNSUPPORTED_OBJECT_TYPE - The affected S3 object uses a file or storage format that Macie doesn't support for
* retrieving occurrences of sensitive data.
*
*
*
*
* This value is null if sensitive data can be retrieved for the finding.
*
*/
private java.util.List reasons;
/**
*
* Specifies whether occurrences of sensitive data can be retrieved for the finding. Possible values are: AVAILABLE,
* the sensitive data can be retrieved; and, UNAVAILABLE, the sensitive data can't be retrieved. If this value is
* UNAVAILABLE, the reasons array indicates why the data can't be retrieved.
*
*
* @param code
* Specifies whether occurrences of sensitive data can be retrieved for the finding. Possible values are:
* AVAILABLE, the sensitive data can be retrieved; and, UNAVAILABLE, the sensitive data can't be retrieved.
* If this value is UNAVAILABLE, the reasons array indicates why the data can't be retrieved.
* @see AvailabilityCode
*/
public void setCode(String code) {
this.code = code;
}
/**
*
* Specifies whether occurrences of sensitive data can be retrieved for the finding. Possible values are: AVAILABLE,
* the sensitive data can be retrieved; and, UNAVAILABLE, the sensitive data can't be retrieved. If this value is
* UNAVAILABLE, the reasons array indicates why the data can't be retrieved.
*
*
* @return Specifies whether occurrences of sensitive data can be retrieved for the finding. Possible values are:
* AVAILABLE, the sensitive data can be retrieved; and, UNAVAILABLE, the sensitive data can't be retrieved.
* If this value is UNAVAILABLE, the reasons array indicates why the data can't be retrieved.
* @see AvailabilityCode
*/
public String getCode() {
return this.code;
}
/**
*
* Specifies whether occurrences of sensitive data can be retrieved for the finding. Possible values are: AVAILABLE,
* the sensitive data can be retrieved; and, UNAVAILABLE, the sensitive data can't be retrieved. If this value is
* UNAVAILABLE, the reasons array indicates why the data can't be retrieved.
*
*
* @param code
* Specifies whether occurrences of sensitive data can be retrieved for the finding. Possible values are:
* AVAILABLE, the sensitive data can be retrieved; and, UNAVAILABLE, the sensitive data can't be retrieved.
* If this value is UNAVAILABLE, the reasons array indicates why the data can't be retrieved.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AvailabilityCode
*/
public GetSensitiveDataOccurrencesAvailabilityResult withCode(String code) {
setCode(code);
return this;
}
/**
*
* Specifies whether occurrences of sensitive data can be retrieved for the finding. Possible values are: AVAILABLE,
* the sensitive data can be retrieved; and, UNAVAILABLE, the sensitive data can't be retrieved. If this value is
* UNAVAILABLE, the reasons array indicates why the data can't be retrieved.
*
*
* @param code
* Specifies whether occurrences of sensitive data can be retrieved for the finding. Possible values are:
* AVAILABLE, the sensitive data can be retrieved; and, UNAVAILABLE, the sensitive data can't be retrieved.
* If this value is UNAVAILABLE, the reasons array indicates why the data can't be retrieved.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AvailabilityCode
*/
public GetSensitiveDataOccurrencesAvailabilityResult withCode(AvailabilityCode code) {
this.code = code.toString();
return this;
}
/**
*
* Specifies why occurrences of sensitive data can't be retrieved for the finding. Possible values are:
*
*
* -
*
* INVALID_CLASSIFICATION_RESULT - Amazon Macie can't verify the location of the sensitive data to retrieve. There
* isn't a corresponding sensitive data discovery result for the finding. Or the sensitive data discovery result
* specified by the classificationDetails.detailedResultsLocation field of the finding isn't available, is malformed
* or corrupted, or uses an unsupported storage format.
*
*
* -
*
* OBJECT_EXCEEDS_SIZE_QUOTA - The storage size of the affected S3 object exceeds the size quota for retrieving
* occurrences of sensitive data.
*
*
* -
*
* OBJECT_UNAVAILABLE - The affected S3 object isn't available. The object might have been renamed, moved, or
* deleted. Or the object was changed after Macie created the finding.
*
*
* -
*
* UNSUPPORTED_FINDING_TYPE - The specified finding isn't a sensitive data finding.
*
*
* -
*
* UNSUPPORTED_OBJECT_TYPE - The affected S3 object uses a file or storage format that Macie doesn't support for
* retrieving occurrences of sensitive data.
*
*
*
*
* This value is null if sensitive data can be retrieved for the finding.
*
*
* @return Specifies why occurrences of sensitive data can't be retrieved for the finding. Possible values are:
*
* -
*
* INVALID_CLASSIFICATION_RESULT - Amazon Macie can't verify the location of the sensitive data to retrieve.
* There isn't a corresponding sensitive data discovery result for the finding. Or the sensitive data
* discovery result specified by the classificationDetails.detailedResultsLocation field of the finding
* isn't available, is malformed or corrupted, or uses an unsupported storage format.
*
*
* -
*
* OBJECT_EXCEEDS_SIZE_QUOTA - The storage size of the affected S3 object exceeds the size quota for
* retrieving occurrences of sensitive data.
*
*
* -
*
* OBJECT_UNAVAILABLE - The affected S3 object isn't available. The object might have been renamed, moved,
* or deleted. Or the object was changed after Macie created the finding.
*
*
* -
*
* UNSUPPORTED_FINDING_TYPE - The specified finding isn't a sensitive data finding.
*
*
* -
*
* UNSUPPORTED_OBJECT_TYPE - The affected S3 object uses a file or storage format that Macie doesn't support
* for retrieving occurrences of sensitive data.
*
*
*
*
* This value is null if sensitive data can be retrieved for the finding.
* @see UnavailabilityReasonCode
*/
public java.util.List getReasons() {
return reasons;
}
/**
*
* Specifies why occurrences of sensitive data can't be retrieved for the finding. Possible values are:
*
*
* -
*
* INVALID_CLASSIFICATION_RESULT - Amazon Macie can't verify the location of the sensitive data to retrieve. There
* isn't a corresponding sensitive data discovery result for the finding. Or the sensitive data discovery result
* specified by the classificationDetails.detailedResultsLocation field of the finding isn't available, is malformed
* or corrupted, or uses an unsupported storage format.
*
*
* -
*
* OBJECT_EXCEEDS_SIZE_QUOTA - The storage size of the affected S3 object exceeds the size quota for retrieving
* occurrences of sensitive data.
*
*
* -
*
* OBJECT_UNAVAILABLE - The affected S3 object isn't available. The object might have been renamed, moved, or
* deleted. Or the object was changed after Macie created the finding.
*
*
* -
*
* UNSUPPORTED_FINDING_TYPE - The specified finding isn't a sensitive data finding.
*
*
* -
*
* UNSUPPORTED_OBJECT_TYPE - The affected S3 object uses a file or storage format that Macie doesn't support for
* retrieving occurrences of sensitive data.
*
*
*
*
* This value is null if sensitive data can be retrieved for the finding.
*
*
* @param reasons
* Specifies why occurrences of sensitive data can't be retrieved for the finding. Possible values are:
*
* -
*
* INVALID_CLASSIFICATION_RESULT - Amazon Macie can't verify the location of the sensitive data to retrieve.
* There isn't a corresponding sensitive data discovery result for the finding. Or the sensitive data
* discovery result specified by the classificationDetails.detailedResultsLocation field of the finding isn't
* available, is malformed or corrupted, or uses an unsupported storage format.
*
*
* -
*
* OBJECT_EXCEEDS_SIZE_QUOTA - The storage size of the affected S3 object exceeds the size quota for
* retrieving occurrences of sensitive data.
*
*
* -
*
* OBJECT_UNAVAILABLE - The affected S3 object isn't available. The object might have been renamed, moved, or
* deleted. Or the object was changed after Macie created the finding.
*
*
* -
*
* UNSUPPORTED_FINDING_TYPE - The specified finding isn't a sensitive data finding.
*
*
* -
*
* UNSUPPORTED_OBJECT_TYPE - The affected S3 object uses a file or storage format that Macie doesn't support
* for retrieving occurrences of sensitive data.
*
*
*
*
* This value is null if sensitive data can be retrieved for the finding.
* @see UnavailabilityReasonCode
*/
public void setReasons(java.util.Collection reasons) {
if (reasons == null) {
this.reasons = null;
return;
}
this.reasons = new java.util.ArrayList(reasons);
}
/**
*
* Specifies why occurrences of sensitive data can't be retrieved for the finding. Possible values are:
*
*
* -
*
* INVALID_CLASSIFICATION_RESULT - Amazon Macie can't verify the location of the sensitive data to retrieve. There
* isn't a corresponding sensitive data discovery result for the finding. Or the sensitive data discovery result
* specified by the classificationDetails.detailedResultsLocation field of the finding isn't available, is malformed
* or corrupted, or uses an unsupported storage format.
*
*
* -
*
* OBJECT_EXCEEDS_SIZE_QUOTA - The storage size of the affected S3 object exceeds the size quota for retrieving
* occurrences of sensitive data.
*
*
* -
*
* OBJECT_UNAVAILABLE - The affected S3 object isn't available. The object might have been renamed, moved, or
* deleted. Or the object was changed after Macie created the finding.
*
*
* -
*
* UNSUPPORTED_FINDING_TYPE - The specified finding isn't a sensitive data finding.
*
*
* -
*
* UNSUPPORTED_OBJECT_TYPE - The affected S3 object uses a file or storage format that Macie doesn't support for
* retrieving occurrences of sensitive data.
*
*
*
*
* This value is null if sensitive data can be retrieved for the finding.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setReasons(java.util.Collection)} or {@link #withReasons(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param reasons
* Specifies why occurrences of sensitive data can't be retrieved for the finding. Possible values are:
*
* -
*
* INVALID_CLASSIFICATION_RESULT - Amazon Macie can't verify the location of the sensitive data to retrieve.
* There isn't a corresponding sensitive data discovery result for the finding. Or the sensitive data
* discovery result specified by the classificationDetails.detailedResultsLocation field of the finding isn't
* available, is malformed or corrupted, or uses an unsupported storage format.
*
*
* -
*
* OBJECT_EXCEEDS_SIZE_QUOTA - The storage size of the affected S3 object exceeds the size quota for
* retrieving occurrences of sensitive data.
*
*
* -
*
* OBJECT_UNAVAILABLE - The affected S3 object isn't available. The object might have been renamed, moved, or
* deleted. Or the object was changed after Macie created the finding.
*
*
* -
*
* UNSUPPORTED_FINDING_TYPE - The specified finding isn't a sensitive data finding.
*
*
* -
*
* UNSUPPORTED_OBJECT_TYPE - The affected S3 object uses a file or storage format that Macie doesn't support
* for retrieving occurrences of sensitive data.
*
*
*
*
* This value is null if sensitive data can be retrieved for the finding.
* @return Returns a reference to this object so that method calls can be chained together.
* @see UnavailabilityReasonCode
*/
public GetSensitiveDataOccurrencesAvailabilityResult withReasons(String... reasons) {
if (this.reasons == null) {
setReasons(new java.util.ArrayList(reasons.length));
}
for (String ele : reasons) {
this.reasons.add(ele);
}
return this;
}
/**
*
* Specifies why occurrences of sensitive data can't be retrieved for the finding. Possible values are:
*
*
* -
*
* INVALID_CLASSIFICATION_RESULT - Amazon Macie can't verify the location of the sensitive data to retrieve. There
* isn't a corresponding sensitive data discovery result for the finding. Or the sensitive data discovery result
* specified by the classificationDetails.detailedResultsLocation field of the finding isn't available, is malformed
* or corrupted, or uses an unsupported storage format.
*
*
* -
*
* OBJECT_EXCEEDS_SIZE_QUOTA - The storage size of the affected S3 object exceeds the size quota for retrieving
* occurrences of sensitive data.
*
*
* -
*
* OBJECT_UNAVAILABLE - The affected S3 object isn't available. The object might have been renamed, moved, or
* deleted. Or the object was changed after Macie created the finding.
*
*
* -
*
* UNSUPPORTED_FINDING_TYPE - The specified finding isn't a sensitive data finding.
*
*
* -
*
* UNSUPPORTED_OBJECT_TYPE - The affected S3 object uses a file or storage format that Macie doesn't support for
* retrieving occurrences of sensitive data.
*
*
*
*
* This value is null if sensitive data can be retrieved for the finding.
*
*
* @param reasons
* Specifies why occurrences of sensitive data can't be retrieved for the finding. Possible values are:
*
* -
*
* INVALID_CLASSIFICATION_RESULT - Amazon Macie can't verify the location of the sensitive data to retrieve.
* There isn't a corresponding sensitive data discovery result for the finding. Or the sensitive data
* discovery result specified by the classificationDetails.detailedResultsLocation field of the finding isn't
* available, is malformed or corrupted, or uses an unsupported storage format.
*
*
* -
*
* OBJECT_EXCEEDS_SIZE_QUOTA - The storage size of the affected S3 object exceeds the size quota for
* retrieving occurrences of sensitive data.
*
*
* -
*
* OBJECT_UNAVAILABLE - The affected S3 object isn't available. The object might have been renamed, moved, or
* deleted. Or the object was changed after Macie created the finding.
*
*
* -
*
* UNSUPPORTED_FINDING_TYPE - The specified finding isn't a sensitive data finding.
*
*
* -
*
* UNSUPPORTED_OBJECT_TYPE - The affected S3 object uses a file or storage format that Macie doesn't support
* for retrieving occurrences of sensitive data.
*
*
*
*
* This value is null if sensitive data can be retrieved for the finding.
* @return Returns a reference to this object so that method calls can be chained together.
* @see UnavailabilityReasonCode
*/
public GetSensitiveDataOccurrencesAvailabilityResult withReasons(java.util.Collection reasons) {
setReasons(reasons);
return this;
}
/**
*
* Specifies why occurrences of sensitive data can't be retrieved for the finding. Possible values are:
*
*
* -
*
* INVALID_CLASSIFICATION_RESULT - Amazon Macie can't verify the location of the sensitive data to retrieve. There
* isn't a corresponding sensitive data discovery result for the finding. Or the sensitive data discovery result
* specified by the classificationDetails.detailedResultsLocation field of the finding isn't available, is malformed
* or corrupted, or uses an unsupported storage format.
*
*
* -
*
* OBJECT_EXCEEDS_SIZE_QUOTA - The storage size of the affected S3 object exceeds the size quota for retrieving
* occurrences of sensitive data.
*
*
* -
*
* OBJECT_UNAVAILABLE - The affected S3 object isn't available. The object might have been renamed, moved, or
* deleted. Or the object was changed after Macie created the finding.
*
*
* -
*
* UNSUPPORTED_FINDING_TYPE - The specified finding isn't a sensitive data finding.
*
*
* -
*
* UNSUPPORTED_OBJECT_TYPE - The affected S3 object uses a file or storage format that Macie doesn't support for
* retrieving occurrences of sensitive data.
*
*
*
*
* This value is null if sensitive data can be retrieved for the finding.
*
*
* @param reasons
* Specifies why occurrences of sensitive data can't be retrieved for the finding. Possible values are:
*
* -
*
* INVALID_CLASSIFICATION_RESULT - Amazon Macie can't verify the location of the sensitive data to retrieve.
* There isn't a corresponding sensitive data discovery result for the finding. Or the sensitive data
* discovery result specified by the classificationDetails.detailedResultsLocation field of the finding isn't
* available, is malformed or corrupted, or uses an unsupported storage format.
*
*
* -
*
* OBJECT_EXCEEDS_SIZE_QUOTA - The storage size of the affected S3 object exceeds the size quota for
* retrieving occurrences of sensitive data.
*
*
* -
*
* OBJECT_UNAVAILABLE - The affected S3 object isn't available. The object might have been renamed, moved, or
* deleted. Or the object was changed after Macie created the finding.
*
*
* -
*
* UNSUPPORTED_FINDING_TYPE - The specified finding isn't a sensitive data finding.
*
*
* -
*
* UNSUPPORTED_OBJECT_TYPE - The affected S3 object uses a file or storage format that Macie doesn't support
* for retrieving occurrences of sensitive data.
*
*
*
*
* This value is null if sensitive data can be retrieved for the finding.
* @return Returns a reference to this object so that method calls can be chained together.
* @see UnavailabilityReasonCode
*/
public GetSensitiveDataOccurrencesAvailabilityResult withReasons(UnavailabilityReasonCode... reasons) {
java.util.ArrayList reasonsCopy = new java.util.ArrayList(reasons.length);
for (UnavailabilityReasonCode value : reasons) {
reasonsCopy.add(value.toString());
}
if (getReasons() == null) {
setReasons(reasonsCopy);
} else {
getReasons().addAll(reasonsCopy);
}
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getCode() != null)
sb.append("Code: ").append(getCode()).append(",");
if (getReasons() != null)
sb.append("Reasons: ").append(getReasons());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof GetSensitiveDataOccurrencesAvailabilityResult == false)
return false;
GetSensitiveDataOccurrencesAvailabilityResult other = (GetSensitiveDataOccurrencesAvailabilityResult) obj;
if (other.getCode() == null ^ this.getCode() == null)
return false;
if (other.getCode() != null && other.getCode().equals(this.getCode()) == false)
return false;
if (other.getReasons() == null ^ this.getReasons() == null)
return false;
if (other.getReasons() != null && other.getReasons().equals(this.getReasons()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getCode() == null) ? 0 : getCode().hashCode());
hashCode = prime * hashCode + ((getReasons() == null) ? 0 : getReasons().hashCode());
return hashCode;
}
@Override
public GetSensitiveDataOccurrencesAvailabilityResult clone() {
try {
return (GetSensitiveDataOccurrencesAvailabilityResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}