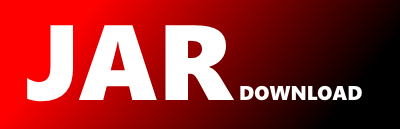
com.amazonaws.services.macie2.model.JobSummary Maven / Gradle / Ivy
Show all versions of aws-java-sdk-macie2 Show documentation
/*
* Copyright 2018-2023 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.macie2.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Provides information about a classification job, including the current status of the job.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class JobSummary implements Serializable, Cloneable, StructuredPojo {
/**
*
* The property- and tag-based conditions that determine which S3 buckets are included or excluded from the job's
* analysis. Each time the job runs, the job uses these criteria to determine which buckets to analyze. A job's
* definition can contain a bucketCriteria object or a bucketDefinitions array, not both.
*
*/
private S3BucketCriteriaForJob bucketCriteria;
/**
*
* An array of objects, one for each Amazon Web Services account that owns specific S3 buckets for the job to
* analyze. Each object specifies the account ID for an account and one or more buckets to analyze for that account.
* A job's definition can contain a bucketDefinitions array or a bucketCriteria object, not both.
*
*/
private java.util.List bucketDefinitions;
/**
*
* The date and time, in UTC and extended ISO 8601 format, when the job was created.
*
*/
private java.util.Date createdAt;
/**
*
* The unique identifier for the job.
*
*/
private String jobId;
/**
*
* The current status of the job. Possible values are:
*
*
* -
*
* CANCELLED - You cancelled the job or, if it's a one-time job, you paused the job and didn't resume it within 30
* days.
*
*
* -
*
* COMPLETE - For a one-time job, Amazon Macie finished processing the data specified for the job. This value
* doesn't apply to recurring jobs.
*
*
* -
*
* IDLE - For a recurring job, the previous scheduled run is complete and the next scheduled run is pending. This
* value doesn't apply to one-time jobs.
*
*
* -
*
* PAUSED - Macie started running the job but additional processing would exceed the monthly sensitive data
* discovery quota for your account or one or more member accounts that the job analyzes data for.
*
*
* -
*
* RUNNING - For a one-time job, the job is in progress. For a recurring job, a scheduled run is in progress.
*
*
* -
*
* USER_PAUSED - You paused the job. If you paused the job while it had a status of RUNNING and you don't resume it
* within 30 days of pausing it, the job or job run will expire and be cancelled, depending on the job's type. To
* check the expiration date, refer to the UserPausedDetails.jobExpiresAt property.
*
*
*
*/
private String jobStatus;
/**
*
* The schedule for running the job. Possible values are:
*
*
* -
*
* ONE_TIME - The job runs only once.
*
*
* -
*
* SCHEDULED - The job runs on a daily, weekly, or monthly basis.
*
*
*
*/
private String jobType;
/**
*
* Specifies whether any account- or bucket-level access errors occurred when the job ran. For a recurring job, this
* value indicates the error status of the job's most recent run.
*
*/
private LastRunErrorStatus lastRunErrorStatus;
/**
*
* The custom name of the job.
*
*/
private String name;
/**
*
* If the current status of the job is USER_PAUSED, specifies when the job was paused and when the job or job run
* will expire and be cancelled if it isn't resumed. This value is present only if the value for jobStatus is
* USER_PAUSED.
*
*/
private UserPausedDetails userPausedDetails;
/**
*
* The property- and tag-based conditions that determine which S3 buckets are included or excluded from the job's
* analysis. Each time the job runs, the job uses these criteria to determine which buckets to analyze. A job's
* definition can contain a bucketCriteria object or a bucketDefinitions array, not both.
*
*
* @param bucketCriteria
* The property- and tag-based conditions that determine which S3 buckets are included or excluded from the
* job's analysis. Each time the job runs, the job uses these criteria to determine which buckets to analyze.
* A job's definition can contain a bucketCriteria object or a bucketDefinitions array, not both.
*/
public void setBucketCriteria(S3BucketCriteriaForJob bucketCriteria) {
this.bucketCriteria = bucketCriteria;
}
/**
*
* The property- and tag-based conditions that determine which S3 buckets are included or excluded from the job's
* analysis. Each time the job runs, the job uses these criteria to determine which buckets to analyze. A job's
* definition can contain a bucketCriteria object or a bucketDefinitions array, not both.
*
*
* @return The property- and tag-based conditions that determine which S3 buckets are included or excluded from the
* job's analysis. Each time the job runs, the job uses these criteria to determine which buckets to
* analyze. A job's definition can contain a bucketCriteria object or a bucketDefinitions array, not both.
*/
public S3BucketCriteriaForJob getBucketCriteria() {
return this.bucketCriteria;
}
/**
*
* The property- and tag-based conditions that determine which S3 buckets are included or excluded from the job's
* analysis. Each time the job runs, the job uses these criteria to determine which buckets to analyze. A job's
* definition can contain a bucketCriteria object or a bucketDefinitions array, not both.
*
*
* @param bucketCriteria
* The property- and tag-based conditions that determine which S3 buckets are included or excluded from the
* job's analysis. Each time the job runs, the job uses these criteria to determine which buckets to analyze.
* A job's definition can contain a bucketCriteria object or a bucketDefinitions array, not both.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public JobSummary withBucketCriteria(S3BucketCriteriaForJob bucketCriteria) {
setBucketCriteria(bucketCriteria);
return this;
}
/**
*
* An array of objects, one for each Amazon Web Services account that owns specific S3 buckets for the job to
* analyze. Each object specifies the account ID for an account and one or more buckets to analyze for that account.
* A job's definition can contain a bucketDefinitions array or a bucketCriteria object, not both.
*
*
* @return An array of objects, one for each Amazon Web Services account that owns specific S3 buckets for the job
* to analyze. Each object specifies the account ID for an account and one or more buckets to analyze for
* that account. A job's definition can contain a bucketDefinitions array or a bucketCriteria object, not
* both.
*/
public java.util.List getBucketDefinitions() {
return bucketDefinitions;
}
/**
*
* An array of objects, one for each Amazon Web Services account that owns specific S3 buckets for the job to
* analyze. Each object specifies the account ID for an account and one or more buckets to analyze for that account.
* A job's definition can contain a bucketDefinitions array or a bucketCriteria object, not both.
*
*
* @param bucketDefinitions
* An array of objects, one for each Amazon Web Services account that owns specific S3 buckets for the job to
* analyze. Each object specifies the account ID for an account and one or more buckets to analyze for that
* account. A job's definition can contain a bucketDefinitions array or a bucketCriteria object, not both.
*/
public void setBucketDefinitions(java.util.Collection bucketDefinitions) {
if (bucketDefinitions == null) {
this.bucketDefinitions = null;
return;
}
this.bucketDefinitions = new java.util.ArrayList(bucketDefinitions);
}
/**
*
* An array of objects, one for each Amazon Web Services account that owns specific S3 buckets for the job to
* analyze. Each object specifies the account ID for an account and one or more buckets to analyze for that account.
* A job's definition can contain a bucketDefinitions array or a bucketCriteria object, not both.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setBucketDefinitions(java.util.Collection)} or {@link #withBucketDefinitions(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param bucketDefinitions
* An array of objects, one for each Amazon Web Services account that owns specific S3 buckets for the job to
* analyze. Each object specifies the account ID for an account and one or more buckets to analyze for that
* account. A job's definition can contain a bucketDefinitions array or a bucketCriteria object, not both.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public JobSummary withBucketDefinitions(S3BucketDefinitionForJob... bucketDefinitions) {
if (this.bucketDefinitions == null) {
setBucketDefinitions(new java.util.ArrayList(bucketDefinitions.length));
}
for (S3BucketDefinitionForJob ele : bucketDefinitions) {
this.bucketDefinitions.add(ele);
}
return this;
}
/**
*
* An array of objects, one for each Amazon Web Services account that owns specific S3 buckets for the job to
* analyze. Each object specifies the account ID for an account and one or more buckets to analyze for that account.
* A job's definition can contain a bucketDefinitions array or a bucketCriteria object, not both.
*
*
* @param bucketDefinitions
* An array of objects, one for each Amazon Web Services account that owns specific S3 buckets for the job to
* analyze. Each object specifies the account ID for an account and one or more buckets to analyze for that
* account. A job's definition can contain a bucketDefinitions array or a bucketCriteria object, not both.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public JobSummary withBucketDefinitions(java.util.Collection bucketDefinitions) {
setBucketDefinitions(bucketDefinitions);
return this;
}
/**
*
* The date and time, in UTC and extended ISO 8601 format, when the job was created.
*
*
* @param createdAt
* The date and time, in UTC and extended ISO 8601 format, when the job was created.
*/
public void setCreatedAt(java.util.Date createdAt) {
this.createdAt = createdAt;
}
/**
*
* The date and time, in UTC and extended ISO 8601 format, when the job was created.
*
*
* @return The date and time, in UTC and extended ISO 8601 format, when the job was created.
*/
public java.util.Date getCreatedAt() {
return this.createdAt;
}
/**
*
* The date and time, in UTC and extended ISO 8601 format, when the job was created.
*
*
* @param createdAt
* The date and time, in UTC and extended ISO 8601 format, when the job was created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public JobSummary withCreatedAt(java.util.Date createdAt) {
setCreatedAt(createdAt);
return this;
}
/**
*
* The unique identifier for the job.
*
*
* @param jobId
* The unique identifier for the job.
*/
public void setJobId(String jobId) {
this.jobId = jobId;
}
/**
*
* The unique identifier for the job.
*
*
* @return The unique identifier for the job.
*/
public String getJobId() {
return this.jobId;
}
/**
*
* The unique identifier for the job.
*
*
* @param jobId
* The unique identifier for the job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public JobSummary withJobId(String jobId) {
setJobId(jobId);
return this;
}
/**
*
* The current status of the job. Possible values are:
*
*
* -
*
* CANCELLED - You cancelled the job or, if it's a one-time job, you paused the job and didn't resume it within 30
* days.
*
*
* -
*
* COMPLETE - For a one-time job, Amazon Macie finished processing the data specified for the job. This value
* doesn't apply to recurring jobs.
*
*
* -
*
* IDLE - For a recurring job, the previous scheduled run is complete and the next scheduled run is pending. This
* value doesn't apply to one-time jobs.
*
*
* -
*
* PAUSED - Macie started running the job but additional processing would exceed the monthly sensitive data
* discovery quota for your account or one or more member accounts that the job analyzes data for.
*
*
* -
*
* RUNNING - For a one-time job, the job is in progress. For a recurring job, a scheduled run is in progress.
*
*
* -
*
* USER_PAUSED - You paused the job. If you paused the job while it had a status of RUNNING and you don't resume it
* within 30 days of pausing it, the job or job run will expire and be cancelled, depending on the job's type. To
* check the expiration date, refer to the UserPausedDetails.jobExpiresAt property.
*
*
*
*
* @param jobStatus
* The current status of the job. Possible values are:
*
* -
*
* CANCELLED - You cancelled the job or, if it's a one-time job, you paused the job and didn't resume it
* within 30 days.
*
*
* -
*
* COMPLETE - For a one-time job, Amazon Macie finished processing the data specified for the job. This value
* doesn't apply to recurring jobs.
*
*
* -
*
* IDLE - For a recurring job, the previous scheduled run is complete and the next scheduled run is pending.
* This value doesn't apply to one-time jobs.
*
*
* -
*
* PAUSED - Macie started running the job but additional processing would exceed the monthly sensitive data
* discovery quota for your account or one or more member accounts that the job analyzes data for.
*
*
* -
*
* RUNNING - For a one-time job, the job is in progress. For a recurring job, a scheduled run is in progress.
*
*
* -
*
* USER_PAUSED - You paused the job. If you paused the job while it had a status of RUNNING and you don't
* resume it within 30 days of pausing it, the job or job run will expire and be cancelled, depending on the
* job's type. To check the expiration date, refer to the UserPausedDetails.jobExpiresAt property.
*
*
* @see JobStatus
*/
public void setJobStatus(String jobStatus) {
this.jobStatus = jobStatus;
}
/**
*
* The current status of the job. Possible values are:
*
*
* -
*
* CANCELLED - You cancelled the job or, if it's a one-time job, you paused the job and didn't resume it within 30
* days.
*
*
* -
*
* COMPLETE - For a one-time job, Amazon Macie finished processing the data specified for the job. This value
* doesn't apply to recurring jobs.
*
*
* -
*
* IDLE - For a recurring job, the previous scheduled run is complete and the next scheduled run is pending. This
* value doesn't apply to one-time jobs.
*
*
* -
*
* PAUSED - Macie started running the job but additional processing would exceed the monthly sensitive data
* discovery quota for your account or one or more member accounts that the job analyzes data for.
*
*
* -
*
* RUNNING - For a one-time job, the job is in progress. For a recurring job, a scheduled run is in progress.
*
*
* -
*
* USER_PAUSED - You paused the job. If you paused the job while it had a status of RUNNING and you don't resume it
* within 30 days of pausing it, the job or job run will expire and be cancelled, depending on the job's type. To
* check the expiration date, refer to the UserPausedDetails.jobExpiresAt property.
*
*
*
*
* @return The current status of the job. Possible values are:
*
* -
*
* CANCELLED - You cancelled the job or, if it's a one-time job, you paused the job and didn't resume it
* within 30 days.
*
*
* -
*
* COMPLETE - For a one-time job, Amazon Macie finished processing the data specified for the job. This
* value doesn't apply to recurring jobs.
*
*
* -
*
* IDLE - For a recurring job, the previous scheduled run is complete and the next scheduled run is pending.
* This value doesn't apply to one-time jobs.
*
*
* -
*
* PAUSED - Macie started running the job but additional processing would exceed the monthly sensitive data
* discovery quota for your account or one or more member accounts that the job analyzes data for.
*
*
* -
*
* RUNNING - For a one-time job, the job is in progress. For a recurring job, a scheduled run is in
* progress.
*
*
* -
*
* USER_PAUSED - You paused the job. If you paused the job while it had a status of RUNNING and you don't
* resume it within 30 days of pausing it, the job or job run will expire and be cancelled, depending on the
* job's type. To check the expiration date, refer to the UserPausedDetails.jobExpiresAt property.
*
*
* @see JobStatus
*/
public String getJobStatus() {
return this.jobStatus;
}
/**
*
* The current status of the job. Possible values are:
*
*
* -
*
* CANCELLED - You cancelled the job or, if it's a one-time job, you paused the job and didn't resume it within 30
* days.
*
*
* -
*
* COMPLETE - For a one-time job, Amazon Macie finished processing the data specified for the job. This value
* doesn't apply to recurring jobs.
*
*
* -
*
* IDLE - For a recurring job, the previous scheduled run is complete and the next scheduled run is pending. This
* value doesn't apply to one-time jobs.
*
*
* -
*
* PAUSED - Macie started running the job but additional processing would exceed the monthly sensitive data
* discovery quota for your account or one or more member accounts that the job analyzes data for.
*
*
* -
*
* RUNNING - For a one-time job, the job is in progress. For a recurring job, a scheduled run is in progress.
*
*
* -
*
* USER_PAUSED - You paused the job. If you paused the job while it had a status of RUNNING and you don't resume it
* within 30 days of pausing it, the job or job run will expire and be cancelled, depending on the job's type. To
* check the expiration date, refer to the UserPausedDetails.jobExpiresAt property.
*
*
*
*
* @param jobStatus
* The current status of the job. Possible values are:
*
* -
*
* CANCELLED - You cancelled the job or, if it's a one-time job, you paused the job and didn't resume it
* within 30 days.
*
*
* -
*
* COMPLETE - For a one-time job, Amazon Macie finished processing the data specified for the job. This value
* doesn't apply to recurring jobs.
*
*
* -
*
* IDLE - For a recurring job, the previous scheduled run is complete and the next scheduled run is pending.
* This value doesn't apply to one-time jobs.
*
*
* -
*
* PAUSED - Macie started running the job but additional processing would exceed the monthly sensitive data
* discovery quota for your account or one or more member accounts that the job analyzes data for.
*
*
* -
*
* RUNNING - For a one-time job, the job is in progress. For a recurring job, a scheduled run is in progress.
*
*
* -
*
* USER_PAUSED - You paused the job. If you paused the job while it had a status of RUNNING and you don't
* resume it within 30 days of pausing it, the job or job run will expire and be cancelled, depending on the
* job's type. To check the expiration date, refer to the UserPausedDetails.jobExpiresAt property.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see JobStatus
*/
public JobSummary withJobStatus(String jobStatus) {
setJobStatus(jobStatus);
return this;
}
/**
*
* The current status of the job. Possible values are:
*
*
* -
*
* CANCELLED - You cancelled the job or, if it's a one-time job, you paused the job and didn't resume it within 30
* days.
*
*
* -
*
* COMPLETE - For a one-time job, Amazon Macie finished processing the data specified for the job. This value
* doesn't apply to recurring jobs.
*
*
* -
*
* IDLE - For a recurring job, the previous scheduled run is complete and the next scheduled run is pending. This
* value doesn't apply to one-time jobs.
*
*
* -
*
* PAUSED - Macie started running the job but additional processing would exceed the monthly sensitive data
* discovery quota for your account or one or more member accounts that the job analyzes data for.
*
*
* -
*
* RUNNING - For a one-time job, the job is in progress. For a recurring job, a scheduled run is in progress.
*
*
* -
*
* USER_PAUSED - You paused the job. If you paused the job while it had a status of RUNNING and you don't resume it
* within 30 days of pausing it, the job or job run will expire and be cancelled, depending on the job's type. To
* check the expiration date, refer to the UserPausedDetails.jobExpiresAt property.
*
*
*
*
* @param jobStatus
* The current status of the job. Possible values are:
*
* -
*
* CANCELLED - You cancelled the job or, if it's a one-time job, you paused the job and didn't resume it
* within 30 days.
*
*
* -
*
* COMPLETE - For a one-time job, Amazon Macie finished processing the data specified for the job. This value
* doesn't apply to recurring jobs.
*
*
* -
*
* IDLE - For a recurring job, the previous scheduled run is complete and the next scheduled run is pending.
* This value doesn't apply to one-time jobs.
*
*
* -
*
* PAUSED - Macie started running the job but additional processing would exceed the monthly sensitive data
* discovery quota for your account or one or more member accounts that the job analyzes data for.
*
*
* -
*
* RUNNING - For a one-time job, the job is in progress. For a recurring job, a scheduled run is in progress.
*
*
* -
*
* USER_PAUSED - You paused the job. If you paused the job while it had a status of RUNNING and you don't
* resume it within 30 days of pausing it, the job or job run will expire and be cancelled, depending on the
* job's type. To check the expiration date, refer to the UserPausedDetails.jobExpiresAt property.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see JobStatus
*/
public JobSummary withJobStatus(JobStatus jobStatus) {
this.jobStatus = jobStatus.toString();
return this;
}
/**
*
* The schedule for running the job. Possible values are:
*
*
* -
*
* ONE_TIME - The job runs only once.
*
*
* -
*
* SCHEDULED - The job runs on a daily, weekly, or monthly basis.
*
*
*
*
* @param jobType
* The schedule for running the job. Possible values are:
*
* -
*
* ONE_TIME - The job runs only once.
*
*
* -
*
* SCHEDULED - The job runs on a daily, weekly, or monthly basis.
*
*
* @see JobType
*/
public void setJobType(String jobType) {
this.jobType = jobType;
}
/**
*
* The schedule for running the job. Possible values are:
*
*
* -
*
* ONE_TIME - The job runs only once.
*
*
* -
*
* SCHEDULED - The job runs on a daily, weekly, or monthly basis.
*
*
*
*
* @return The schedule for running the job. Possible values are:
*
* -
*
* ONE_TIME - The job runs only once.
*
*
* -
*
* SCHEDULED - The job runs on a daily, weekly, or monthly basis.
*
*
* @see JobType
*/
public String getJobType() {
return this.jobType;
}
/**
*
* The schedule for running the job. Possible values are:
*
*
* -
*
* ONE_TIME - The job runs only once.
*
*
* -
*
* SCHEDULED - The job runs on a daily, weekly, or monthly basis.
*
*
*
*
* @param jobType
* The schedule for running the job. Possible values are:
*
* -
*
* ONE_TIME - The job runs only once.
*
*
* -
*
* SCHEDULED - The job runs on a daily, weekly, or monthly basis.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see JobType
*/
public JobSummary withJobType(String jobType) {
setJobType(jobType);
return this;
}
/**
*
* The schedule for running the job. Possible values are:
*
*
* -
*
* ONE_TIME - The job runs only once.
*
*
* -
*
* SCHEDULED - The job runs on a daily, weekly, or monthly basis.
*
*
*
*
* @param jobType
* The schedule for running the job. Possible values are:
*
* -
*
* ONE_TIME - The job runs only once.
*
*
* -
*
* SCHEDULED - The job runs on a daily, weekly, or monthly basis.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see JobType
*/
public JobSummary withJobType(JobType jobType) {
this.jobType = jobType.toString();
return this;
}
/**
*
* Specifies whether any account- or bucket-level access errors occurred when the job ran. For a recurring job, this
* value indicates the error status of the job's most recent run.
*
*
* @param lastRunErrorStatus
* Specifies whether any account- or bucket-level access errors occurred when the job ran. For a recurring
* job, this value indicates the error status of the job's most recent run.
*/
public void setLastRunErrorStatus(LastRunErrorStatus lastRunErrorStatus) {
this.lastRunErrorStatus = lastRunErrorStatus;
}
/**
*
* Specifies whether any account- or bucket-level access errors occurred when the job ran. For a recurring job, this
* value indicates the error status of the job's most recent run.
*
*
* @return Specifies whether any account- or bucket-level access errors occurred when the job ran. For a recurring
* job, this value indicates the error status of the job's most recent run.
*/
public LastRunErrorStatus getLastRunErrorStatus() {
return this.lastRunErrorStatus;
}
/**
*
* Specifies whether any account- or bucket-level access errors occurred when the job ran. For a recurring job, this
* value indicates the error status of the job's most recent run.
*
*
* @param lastRunErrorStatus
* Specifies whether any account- or bucket-level access errors occurred when the job ran. For a recurring
* job, this value indicates the error status of the job's most recent run.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public JobSummary withLastRunErrorStatus(LastRunErrorStatus lastRunErrorStatus) {
setLastRunErrorStatus(lastRunErrorStatus);
return this;
}
/**
*
* The custom name of the job.
*
*
* @param name
* The custom name of the job.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The custom name of the job.
*
*
* @return The custom name of the job.
*/
public String getName() {
return this.name;
}
/**
*
* The custom name of the job.
*
*
* @param name
* The custom name of the job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public JobSummary withName(String name) {
setName(name);
return this;
}
/**
*
* If the current status of the job is USER_PAUSED, specifies when the job was paused and when the job or job run
* will expire and be cancelled if it isn't resumed. This value is present only if the value for jobStatus is
* USER_PAUSED.
*
*
* @param userPausedDetails
* If the current status of the job is USER_PAUSED, specifies when the job was paused and when the job or job
* run will expire and be cancelled if it isn't resumed. This value is present only if the value for
* jobStatus is USER_PAUSED.
*/
public void setUserPausedDetails(UserPausedDetails userPausedDetails) {
this.userPausedDetails = userPausedDetails;
}
/**
*
* If the current status of the job is USER_PAUSED, specifies when the job was paused and when the job or job run
* will expire and be cancelled if it isn't resumed. This value is present only if the value for jobStatus is
* USER_PAUSED.
*
*
* @return If the current status of the job is USER_PAUSED, specifies when the job was paused and when the job or
* job run will expire and be cancelled if it isn't resumed. This value is present only if the value for
* jobStatus is USER_PAUSED.
*/
public UserPausedDetails getUserPausedDetails() {
return this.userPausedDetails;
}
/**
*
* If the current status of the job is USER_PAUSED, specifies when the job was paused and when the job or job run
* will expire and be cancelled if it isn't resumed. This value is present only if the value for jobStatus is
* USER_PAUSED.
*
*
* @param userPausedDetails
* If the current status of the job is USER_PAUSED, specifies when the job was paused and when the job or job
* run will expire and be cancelled if it isn't resumed. This value is present only if the value for
* jobStatus is USER_PAUSED.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public JobSummary withUserPausedDetails(UserPausedDetails userPausedDetails) {
setUserPausedDetails(userPausedDetails);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getBucketCriteria() != null)
sb.append("BucketCriteria: ").append(getBucketCriteria()).append(",");
if (getBucketDefinitions() != null)
sb.append("BucketDefinitions: ").append(getBucketDefinitions()).append(",");
if (getCreatedAt() != null)
sb.append("CreatedAt: ").append(getCreatedAt()).append(",");
if (getJobId() != null)
sb.append("JobId: ").append(getJobId()).append(",");
if (getJobStatus() != null)
sb.append("JobStatus: ").append(getJobStatus()).append(",");
if (getJobType() != null)
sb.append("JobType: ").append(getJobType()).append(",");
if (getLastRunErrorStatus() != null)
sb.append("LastRunErrorStatus: ").append(getLastRunErrorStatus()).append(",");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getUserPausedDetails() != null)
sb.append("UserPausedDetails: ").append(getUserPausedDetails());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof JobSummary == false)
return false;
JobSummary other = (JobSummary) obj;
if (other.getBucketCriteria() == null ^ this.getBucketCriteria() == null)
return false;
if (other.getBucketCriteria() != null && other.getBucketCriteria().equals(this.getBucketCriteria()) == false)
return false;
if (other.getBucketDefinitions() == null ^ this.getBucketDefinitions() == null)
return false;
if (other.getBucketDefinitions() != null && other.getBucketDefinitions().equals(this.getBucketDefinitions()) == false)
return false;
if (other.getCreatedAt() == null ^ this.getCreatedAt() == null)
return false;
if (other.getCreatedAt() != null && other.getCreatedAt().equals(this.getCreatedAt()) == false)
return false;
if (other.getJobId() == null ^ this.getJobId() == null)
return false;
if (other.getJobId() != null && other.getJobId().equals(this.getJobId()) == false)
return false;
if (other.getJobStatus() == null ^ this.getJobStatus() == null)
return false;
if (other.getJobStatus() != null && other.getJobStatus().equals(this.getJobStatus()) == false)
return false;
if (other.getJobType() == null ^ this.getJobType() == null)
return false;
if (other.getJobType() != null && other.getJobType().equals(this.getJobType()) == false)
return false;
if (other.getLastRunErrorStatus() == null ^ this.getLastRunErrorStatus() == null)
return false;
if (other.getLastRunErrorStatus() != null && other.getLastRunErrorStatus().equals(this.getLastRunErrorStatus()) == false)
return false;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getUserPausedDetails() == null ^ this.getUserPausedDetails() == null)
return false;
if (other.getUserPausedDetails() != null && other.getUserPausedDetails().equals(this.getUserPausedDetails()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getBucketCriteria() == null) ? 0 : getBucketCriteria().hashCode());
hashCode = prime * hashCode + ((getBucketDefinitions() == null) ? 0 : getBucketDefinitions().hashCode());
hashCode = prime * hashCode + ((getCreatedAt() == null) ? 0 : getCreatedAt().hashCode());
hashCode = prime * hashCode + ((getJobId() == null) ? 0 : getJobId().hashCode());
hashCode = prime * hashCode + ((getJobStatus() == null) ? 0 : getJobStatus().hashCode());
hashCode = prime * hashCode + ((getJobType() == null) ? 0 : getJobType().hashCode());
hashCode = prime * hashCode + ((getLastRunErrorStatus() == null) ? 0 : getLastRunErrorStatus().hashCode());
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getUserPausedDetails() == null) ? 0 : getUserPausedDetails().hashCode());
return hashCode;
}
@Override
public JobSummary clone() {
try {
return (JobSummary) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.macie2.model.transform.JobSummaryMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}