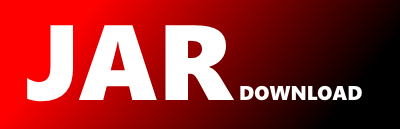
com.amazonaws.services.macie2.model.MatchingBucket Maven / Gradle / Ivy
Show all versions of aws-java-sdk-macie2 Show documentation
/*
* Copyright 2018-2023 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.macie2.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Provides statistical data and other information about an S3 bucket that Amazon Macie monitors and analyzes for your
* account. By default, object count and storage size values include data for object parts that are the result of
* incomplete multipart uploads. For more information, see How Macie monitors Amazon S3
* data security in the Amazon Macie User Guide.
*
*
* If an error occurs when Macie attempts to retrieve and process information about the bucket or the bucket's objects,
* the value for most of these properties is null. Key exceptions are accountId and bucketName. To identify the cause of
* the error, refer to the errorCode and errorMessage values.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class MatchingBucket implements Serializable, Cloneable, StructuredPojo {
/**
*
* The unique identifier for the Amazon Web Services account that owns the bucket.
*
*/
private String accountId;
/**
*
* The name of the bucket.
*
*/
private String bucketName;
/**
*
* The total number of objects that Amazon Macie can analyze in the bucket. These objects use a supported storage
* class and have a file name extension for a supported file or storage format.
*
*/
private Long classifiableObjectCount;
/**
*
* The total storage size, in bytes, of the objects that Amazon Macie can analyze in the bucket. These objects use a
* supported storage class and have a file name extension for a supported file or storage format.
*
*
* If versioning is enabled for the bucket, Macie calculates this value based on the size of the latest version of
* each applicable object in the bucket. This value doesn't reflect the storage size of all versions of each
* applicable object in the bucket.
*
*/
private Long classifiableSizeInBytes;
/**
*
* The error code for an error that prevented Amazon Macie from retrieving and processing information about the
* bucket and the bucket's objects. If this value is ACCESS_DENIED, Macie doesn't have permission to retrieve the
* information. For example, the bucket has a restrictive bucket policy and Amazon S3 denied the request. If this
* value is null, Macie was able to retrieve and process the information.
*
*/
private String errorCode;
/**
*
* A brief description of the error (errorCode) that prevented Amazon Macie from retrieving and processing
* information about the bucket and the bucket's objects. This value is null if Macie was able to retrieve and
* process the information.
*
*/
private String errorMessage;
/**
*
* Specifies whether any one-time or recurring classification jobs are configured to analyze objects in the bucket,
* and, if so, the details of the job that ran most recently.
*
*/
private JobDetails jobDetails;
/**
*
* The date and time, in UTC and extended ISO 8601 format, when Amazon Macie most recently analyzed data in the
* bucket while performing automated sensitive data discovery for your account. This value is null if automated
* sensitive data discovery is currently disabled for your account.
*
*/
private java.util.Date lastAutomatedDiscoveryTime;
/**
*
* The total number of objects in the bucket.
*
*/
private Long objectCount;
/**
*
* The total number of objects in the bucket, grouped by server-side encryption type. This includes a grouping that
* reports the total number of objects that aren't encrypted or use client-side encryption.
*
*/
private ObjectCountByEncryptionType objectCountByEncryptionType;
/**
*
* The current sensitivity score for the bucket, ranging from -1 (classification error) to 100 (sensitive). This
* value is null if automated sensitive data discovery is currently disabled for your account.
*
*/
private Integer sensitivityScore;
/**
*
* The total storage size, in bytes, of the bucket.
*
*
* If versioning is enabled for the bucket, Amazon Macie calculates this value based on the size of the latest
* version of each object in the bucket. This value doesn't reflect the storage size of all versions of each object
* in the bucket.
*
*/
private Long sizeInBytes;
/**
*
* The total storage size, in bytes, of the objects that are compressed (.gz, .gzip, .zip) files in the bucket.
*
*
* If versioning is enabled for the bucket, Amazon Macie calculates this value based on the size of the latest
* version of each applicable object in the bucket. This value doesn't reflect the storage size of all versions of
* each applicable object in the bucket.
*
*/
private Long sizeInBytesCompressed;
/**
*
* The total number of objects that Amazon Macie can't analyze in the bucket. These objects don't use a supported
* storage class or don't have a file name extension for a supported file or storage format.
*
*/
private ObjectLevelStatistics unclassifiableObjectCount;
/**
*
* The total storage size, in bytes, of the objects that Amazon Macie can't analyze in the bucket. These objects
* don't use a supported storage class or don't have a file name extension for a supported file or storage format.
*
*/
private ObjectLevelStatistics unclassifiableObjectSizeInBytes;
/**
*
* The unique identifier for the Amazon Web Services account that owns the bucket.
*
*
* @param accountId
* The unique identifier for the Amazon Web Services account that owns the bucket.
*/
public void setAccountId(String accountId) {
this.accountId = accountId;
}
/**
*
* The unique identifier for the Amazon Web Services account that owns the bucket.
*
*
* @return The unique identifier for the Amazon Web Services account that owns the bucket.
*/
public String getAccountId() {
return this.accountId;
}
/**
*
* The unique identifier for the Amazon Web Services account that owns the bucket.
*
*
* @param accountId
* The unique identifier for the Amazon Web Services account that owns the bucket.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MatchingBucket withAccountId(String accountId) {
setAccountId(accountId);
return this;
}
/**
*
* The name of the bucket.
*
*
* @param bucketName
* The name of the bucket.
*/
public void setBucketName(String bucketName) {
this.bucketName = bucketName;
}
/**
*
* The name of the bucket.
*
*
* @return The name of the bucket.
*/
public String getBucketName() {
return this.bucketName;
}
/**
*
* The name of the bucket.
*
*
* @param bucketName
* The name of the bucket.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MatchingBucket withBucketName(String bucketName) {
setBucketName(bucketName);
return this;
}
/**
*
* The total number of objects that Amazon Macie can analyze in the bucket. These objects use a supported storage
* class and have a file name extension for a supported file or storage format.
*
*
* @param classifiableObjectCount
* The total number of objects that Amazon Macie can analyze in the bucket. These objects use a supported
* storage class and have a file name extension for a supported file or storage format.
*/
public void setClassifiableObjectCount(Long classifiableObjectCount) {
this.classifiableObjectCount = classifiableObjectCount;
}
/**
*
* The total number of objects that Amazon Macie can analyze in the bucket. These objects use a supported storage
* class and have a file name extension for a supported file or storage format.
*
*
* @return The total number of objects that Amazon Macie can analyze in the bucket. These objects use a supported
* storage class and have a file name extension for a supported file or storage format.
*/
public Long getClassifiableObjectCount() {
return this.classifiableObjectCount;
}
/**
*
* The total number of objects that Amazon Macie can analyze in the bucket. These objects use a supported storage
* class and have a file name extension for a supported file or storage format.
*
*
* @param classifiableObjectCount
* The total number of objects that Amazon Macie can analyze in the bucket. These objects use a supported
* storage class and have a file name extension for a supported file or storage format.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MatchingBucket withClassifiableObjectCount(Long classifiableObjectCount) {
setClassifiableObjectCount(classifiableObjectCount);
return this;
}
/**
*
* The total storage size, in bytes, of the objects that Amazon Macie can analyze in the bucket. These objects use a
* supported storage class and have a file name extension for a supported file or storage format.
*
*
* If versioning is enabled for the bucket, Macie calculates this value based on the size of the latest version of
* each applicable object in the bucket. This value doesn't reflect the storage size of all versions of each
* applicable object in the bucket.
*
*
* @param classifiableSizeInBytes
* The total storage size, in bytes, of the objects that Amazon Macie can analyze in the bucket. These
* objects use a supported storage class and have a file name extension for a supported file or storage
* format.
*
* If versioning is enabled for the bucket, Macie calculates this value based on the size of the latest
* version of each applicable object in the bucket. This value doesn't reflect the storage size of all
* versions of each applicable object in the bucket.
*/
public void setClassifiableSizeInBytes(Long classifiableSizeInBytes) {
this.classifiableSizeInBytes = classifiableSizeInBytes;
}
/**
*
* The total storage size, in bytes, of the objects that Amazon Macie can analyze in the bucket. These objects use a
* supported storage class and have a file name extension for a supported file or storage format.
*
*
* If versioning is enabled for the bucket, Macie calculates this value based on the size of the latest version of
* each applicable object in the bucket. This value doesn't reflect the storage size of all versions of each
* applicable object in the bucket.
*
*
* @return The total storage size, in bytes, of the objects that Amazon Macie can analyze in the bucket. These
* objects use a supported storage class and have a file name extension for a supported file or storage
* format.
*
* If versioning is enabled for the bucket, Macie calculates this value based on the size of the latest
* version of each applicable object in the bucket. This value doesn't reflect the storage size of all
* versions of each applicable object in the bucket.
*/
public Long getClassifiableSizeInBytes() {
return this.classifiableSizeInBytes;
}
/**
*
* The total storage size, in bytes, of the objects that Amazon Macie can analyze in the bucket. These objects use a
* supported storage class and have a file name extension for a supported file or storage format.
*
*
* If versioning is enabled for the bucket, Macie calculates this value based on the size of the latest version of
* each applicable object in the bucket. This value doesn't reflect the storage size of all versions of each
* applicable object in the bucket.
*
*
* @param classifiableSizeInBytes
* The total storage size, in bytes, of the objects that Amazon Macie can analyze in the bucket. These
* objects use a supported storage class and have a file name extension for a supported file or storage
* format.
*
* If versioning is enabled for the bucket, Macie calculates this value based on the size of the latest
* version of each applicable object in the bucket. This value doesn't reflect the storage size of all
* versions of each applicable object in the bucket.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MatchingBucket withClassifiableSizeInBytes(Long classifiableSizeInBytes) {
setClassifiableSizeInBytes(classifiableSizeInBytes);
return this;
}
/**
*
* The error code for an error that prevented Amazon Macie from retrieving and processing information about the
* bucket and the bucket's objects. If this value is ACCESS_DENIED, Macie doesn't have permission to retrieve the
* information. For example, the bucket has a restrictive bucket policy and Amazon S3 denied the request. If this
* value is null, Macie was able to retrieve and process the information.
*
*
* @param errorCode
* The error code for an error that prevented Amazon Macie from retrieving and processing information about
* the bucket and the bucket's objects. If this value is ACCESS_DENIED, Macie doesn't have permission to
* retrieve the information. For example, the bucket has a restrictive bucket policy and Amazon S3 denied the
* request. If this value is null, Macie was able to retrieve and process the information.
* @see BucketMetadataErrorCode
*/
public void setErrorCode(String errorCode) {
this.errorCode = errorCode;
}
/**
*
* The error code for an error that prevented Amazon Macie from retrieving and processing information about the
* bucket and the bucket's objects. If this value is ACCESS_DENIED, Macie doesn't have permission to retrieve the
* information. For example, the bucket has a restrictive bucket policy and Amazon S3 denied the request. If this
* value is null, Macie was able to retrieve and process the information.
*
*
* @return The error code for an error that prevented Amazon Macie from retrieving and processing information about
* the bucket and the bucket's objects. If this value is ACCESS_DENIED, Macie doesn't have permission to
* retrieve the information. For example, the bucket has a restrictive bucket policy and Amazon S3 denied
* the request. If this value is null, Macie was able to retrieve and process the information.
* @see BucketMetadataErrorCode
*/
public String getErrorCode() {
return this.errorCode;
}
/**
*
* The error code for an error that prevented Amazon Macie from retrieving and processing information about the
* bucket and the bucket's objects. If this value is ACCESS_DENIED, Macie doesn't have permission to retrieve the
* information. For example, the bucket has a restrictive bucket policy and Amazon S3 denied the request. If this
* value is null, Macie was able to retrieve and process the information.
*
*
* @param errorCode
* The error code for an error that prevented Amazon Macie from retrieving and processing information about
* the bucket and the bucket's objects. If this value is ACCESS_DENIED, Macie doesn't have permission to
* retrieve the information. For example, the bucket has a restrictive bucket policy and Amazon S3 denied the
* request. If this value is null, Macie was able to retrieve and process the information.
* @return Returns a reference to this object so that method calls can be chained together.
* @see BucketMetadataErrorCode
*/
public MatchingBucket withErrorCode(String errorCode) {
setErrorCode(errorCode);
return this;
}
/**
*
* The error code for an error that prevented Amazon Macie from retrieving and processing information about the
* bucket and the bucket's objects. If this value is ACCESS_DENIED, Macie doesn't have permission to retrieve the
* information. For example, the bucket has a restrictive bucket policy and Amazon S3 denied the request. If this
* value is null, Macie was able to retrieve and process the information.
*
*
* @param errorCode
* The error code for an error that prevented Amazon Macie from retrieving and processing information about
* the bucket and the bucket's objects. If this value is ACCESS_DENIED, Macie doesn't have permission to
* retrieve the information. For example, the bucket has a restrictive bucket policy and Amazon S3 denied the
* request. If this value is null, Macie was able to retrieve and process the information.
* @return Returns a reference to this object so that method calls can be chained together.
* @see BucketMetadataErrorCode
*/
public MatchingBucket withErrorCode(BucketMetadataErrorCode errorCode) {
this.errorCode = errorCode.toString();
return this;
}
/**
*
* A brief description of the error (errorCode) that prevented Amazon Macie from retrieving and processing
* information about the bucket and the bucket's objects. This value is null if Macie was able to retrieve and
* process the information.
*
*
* @param errorMessage
* A brief description of the error (errorCode) that prevented Amazon Macie from retrieving and processing
* information about the bucket and the bucket's objects. This value is null if Macie was able to retrieve
* and process the information.
*/
public void setErrorMessage(String errorMessage) {
this.errorMessage = errorMessage;
}
/**
*
* A brief description of the error (errorCode) that prevented Amazon Macie from retrieving and processing
* information about the bucket and the bucket's objects. This value is null if Macie was able to retrieve and
* process the information.
*
*
* @return A brief description of the error (errorCode) that prevented Amazon Macie from retrieving and processing
* information about the bucket and the bucket's objects. This value is null if Macie was able to retrieve
* and process the information.
*/
public String getErrorMessage() {
return this.errorMessage;
}
/**
*
* A brief description of the error (errorCode) that prevented Amazon Macie from retrieving and processing
* information about the bucket and the bucket's objects. This value is null if Macie was able to retrieve and
* process the information.
*
*
* @param errorMessage
* A brief description of the error (errorCode) that prevented Amazon Macie from retrieving and processing
* information about the bucket and the bucket's objects. This value is null if Macie was able to retrieve
* and process the information.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MatchingBucket withErrorMessage(String errorMessage) {
setErrorMessage(errorMessage);
return this;
}
/**
*
* Specifies whether any one-time or recurring classification jobs are configured to analyze objects in the bucket,
* and, if so, the details of the job that ran most recently.
*
*
* @param jobDetails
* Specifies whether any one-time or recurring classification jobs are configured to analyze objects in the
* bucket, and, if so, the details of the job that ran most recently.
*/
public void setJobDetails(JobDetails jobDetails) {
this.jobDetails = jobDetails;
}
/**
*
* Specifies whether any one-time or recurring classification jobs are configured to analyze objects in the bucket,
* and, if so, the details of the job that ran most recently.
*
*
* @return Specifies whether any one-time or recurring classification jobs are configured to analyze objects in the
* bucket, and, if so, the details of the job that ran most recently.
*/
public JobDetails getJobDetails() {
return this.jobDetails;
}
/**
*
* Specifies whether any one-time or recurring classification jobs are configured to analyze objects in the bucket,
* and, if so, the details of the job that ran most recently.
*
*
* @param jobDetails
* Specifies whether any one-time or recurring classification jobs are configured to analyze objects in the
* bucket, and, if so, the details of the job that ran most recently.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MatchingBucket withJobDetails(JobDetails jobDetails) {
setJobDetails(jobDetails);
return this;
}
/**
*
* The date and time, in UTC and extended ISO 8601 format, when Amazon Macie most recently analyzed data in the
* bucket while performing automated sensitive data discovery for your account. This value is null if automated
* sensitive data discovery is currently disabled for your account.
*
*
* @param lastAutomatedDiscoveryTime
* The date and time, in UTC and extended ISO 8601 format, when Amazon Macie most recently analyzed data in
* the bucket while performing automated sensitive data discovery for your account. This value is null if
* automated sensitive data discovery is currently disabled for your account.
*/
public void setLastAutomatedDiscoveryTime(java.util.Date lastAutomatedDiscoveryTime) {
this.lastAutomatedDiscoveryTime = lastAutomatedDiscoveryTime;
}
/**
*
* The date and time, in UTC and extended ISO 8601 format, when Amazon Macie most recently analyzed data in the
* bucket while performing automated sensitive data discovery for your account. This value is null if automated
* sensitive data discovery is currently disabled for your account.
*
*
* @return The date and time, in UTC and extended ISO 8601 format, when Amazon Macie most recently analyzed data in
* the bucket while performing automated sensitive data discovery for your account. This value is null if
* automated sensitive data discovery is currently disabled for your account.
*/
public java.util.Date getLastAutomatedDiscoveryTime() {
return this.lastAutomatedDiscoveryTime;
}
/**
*
* The date and time, in UTC and extended ISO 8601 format, when Amazon Macie most recently analyzed data in the
* bucket while performing automated sensitive data discovery for your account. This value is null if automated
* sensitive data discovery is currently disabled for your account.
*
*
* @param lastAutomatedDiscoveryTime
* The date and time, in UTC and extended ISO 8601 format, when Amazon Macie most recently analyzed data in
* the bucket while performing automated sensitive data discovery for your account. This value is null if
* automated sensitive data discovery is currently disabled for your account.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MatchingBucket withLastAutomatedDiscoveryTime(java.util.Date lastAutomatedDiscoveryTime) {
setLastAutomatedDiscoveryTime(lastAutomatedDiscoveryTime);
return this;
}
/**
*
* The total number of objects in the bucket.
*
*
* @param objectCount
* The total number of objects in the bucket.
*/
public void setObjectCount(Long objectCount) {
this.objectCount = objectCount;
}
/**
*
* The total number of objects in the bucket.
*
*
* @return The total number of objects in the bucket.
*/
public Long getObjectCount() {
return this.objectCount;
}
/**
*
* The total number of objects in the bucket.
*
*
* @param objectCount
* The total number of objects in the bucket.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MatchingBucket withObjectCount(Long objectCount) {
setObjectCount(objectCount);
return this;
}
/**
*
* The total number of objects in the bucket, grouped by server-side encryption type. This includes a grouping that
* reports the total number of objects that aren't encrypted or use client-side encryption.
*
*
* @param objectCountByEncryptionType
* The total number of objects in the bucket, grouped by server-side encryption type. This includes a
* grouping that reports the total number of objects that aren't encrypted or use client-side encryption.
*/
public void setObjectCountByEncryptionType(ObjectCountByEncryptionType objectCountByEncryptionType) {
this.objectCountByEncryptionType = objectCountByEncryptionType;
}
/**
*
* The total number of objects in the bucket, grouped by server-side encryption type. This includes a grouping that
* reports the total number of objects that aren't encrypted or use client-side encryption.
*
*
* @return The total number of objects in the bucket, grouped by server-side encryption type. This includes a
* grouping that reports the total number of objects that aren't encrypted or use client-side encryption.
*/
public ObjectCountByEncryptionType getObjectCountByEncryptionType() {
return this.objectCountByEncryptionType;
}
/**
*
* The total number of objects in the bucket, grouped by server-side encryption type. This includes a grouping that
* reports the total number of objects that aren't encrypted or use client-side encryption.
*
*
* @param objectCountByEncryptionType
* The total number of objects in the bucket, grouped by server-side encryption type. This includes a
* grouping that reports the total number of objects that aren't encrypted or use client-side encryption.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MatchingBucket withObjectCountByEncryptionType(ObjectCountByEncryptionType objectCountByEncryptionType) {
setObjectCountByEncryptionType(objectCountByEncryptionType);
return this;
}
/**
*
* The current sensitivity score for the bucket, ranging from -1 (classification error) to 100 (sensitive). This
* value is null if automated sensitive data discovery is currently disabled for your account.
*
*
* @param sensitivityScore
* The current sensitivity score for the bucket, ranging from -1 (classification error) to 100 (sensitive).
* This value is null if automated sensitive data discovery is currently disabled for your account.
*/
public void setSensitivityScore(Integer sensitivityScore) {
this.sensitivityScore = sensitivityScore;
}
/**
*
* The current sensitivity score for the bucket, ranging from -1 (classification error) to 100 (sensitive). This
* value is null if automated sensitive data discovery is currently disabled for your account.
*
*
* @return The current sensitivity score for the bucket, ranging from -1 (classification error) to 100 (sensitive).
* This value is null if automated sensitive data discovery is currently disabled for your account.
*/
public Integer getSensitivityScore() {
return this.sensitivityScore;
}
/**
*
* The current sensitivity score for the bucket, ranging from -1 (classification error) to 100 (sensitive). This
* value is null if automated sensitive data discovery is currently disabled for your account.
*
*
* @param sensitivityScore
* The current sensitivity score for the bucket, ranging from -1 (classification error) to 100 (sensitive).
* This value is null if automated sensitive data discovery is currently disabled for your account.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MatchingBucket withSensitivityScore(Integer sensitivityScore) {
setSensitivityScore(sensitivityScore);
return this;
}
/**
*
* The total storage size, in bytes, of the bucket.
*
*
* If versioning is enabled for the bucket, Amazon Macie calculates this value based on the size of the latest
* version of each object in the bucket. This value doesn't reflect the storage size of all versions of each object
* in the bucket.
*
*
* @param sizeInBytes
* The total storage size, in bytes, of the bucket.
*
* If versioning is enabled for the bucket, Amazon Macie calculates this value based on the size of the
* latest version of each object in the bucket. This value doesn't reflect the storage size of all versions
* of each object in the bucket.
*/
public void setSizeInBytes(Long sizeInBytes) {
this.sizeInBytes = sizeInBytes;
}
/**
*
* The total storage size, in bytes, of the bucket.
*
*
* If versioning is enabled for the bucket, Amazon Macie calculates this value based on the size of the latest
* version of each object in the bucket. This value doesn't reflect the storage size of all versions of each object
* in the bucket.
*
*
* @return The total storage size, in bytes, of the bucket.
*
* If versioning is enabled for the bucket, Amazon Macie calculates this value based on the size of the
* latest version of each object in the bucket. This value doesn't reflect the storage size of all versions
* of each object in the bucket.
*/
public Long getSizeInBytes() {
return this.sizeInBytes;
}
/**
*
* The total storage size, in bytes, of the bucket.
*
*
* If versioning is enabled for the bucket, Amazon Macie calculates this value based on the size of the latest
* version of each object in the bucket. This value doesn't reflect the storage size of all versions of each object
* in the bucket.
*
*
* @param sizeInBytes
* The total storage size, in bytes, of the bucket.
*
* If versioning is enabled for the bucket, Amazon Macie calculates this value based on the size of the
* latest version of each object in the bucket. This value doesn't reflect the storage size of all versions
* of each object in the bucket.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MatchingBucket withSizeInBytes(Long sizeInBytes) {
setSizeInBytes(sizeInBytes);
return this;
}
/**
*
* The total storage size, in bytes, of the objects that are compressed (.gz, .gzip, .zip) files in the bucket.
*
*
* If versioning is enabled for the bucket, Amazon Macie calculates this value based on the size of the latest
* version of each applicable object in the bucket. This value doesn't reflect the storage size of all versions of
* each applicable object in the bucket.
*
*
* @param sizeInBytesCompressed
* The total storage size, in bytes, of the objects that are compressed (.gz, .gzip, .zip) files in the
* bucket.
*
* If versioning is enabled for the bucket, Amazon Macie calculates this value based on the size of the
* latest version of each applicable object in the bucket. This value doesn't reflect the storage size of all
* versions of each applicable object in the bucket.
*/
public void setSizeInBytesCompressed(Long sizeInBytesCompressed) {
this.sizeInBytesCompressed = sizeInBytesCompressed;
}
/**
*
* The total storage size, in bytes, of the objects that are compressed (.gz, .gzip, .zip) files in the bucket.
*
*
* If versioning is enabled for the bucket, Amazon Macie calculates this value based on the size of the latest
* version of each applicable object in the bucket. This value doesn't reflect the storage size of all versions of
* each applicable object in the bucket.
*
*
* @return The total storage size, in bytes, of the objects that are compressed (.gz, .gzip, .zip) files in the
* bucket.
*
* If versioning is enabled for the bucket, Amazon Macie calculates this value based on the size of the
* latest version of each applicable object in the bucket. This value doesn't reflect the storage size of
* all versions of each applicable object in the bucket.
*/
public Long getSizeInBytesCompressed() {
return this.sizeInBytesCompressed;
}
/**
*
* The total storage size, in bytes, of the objects that are compressed (.gz, .gzip, .zip) files in the bucket.
*
*
* If versioning is enabled for the bucket, Amazon Macie calculates this value based on the size of the latest
* version of each applicable object in the bucket. This value doesn't reflect the storage size of all versions of
* each applicable object in the bucket.
*
*
* @param sizeInBytesCompressed
* The total storage size, in bytes, of the objects that are compressed (.gz, .gzip, .zip) files in the
* bucket.
*
* If versioning is enabled for the bucket, Amazon Macie calculates this value based on the size of the
* latest version of each applicable object in the bucket. This value doesn't reflect the storage size of all
* versions of each applicable object in the bucket.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MatchingBucket withSizeInBytesCompressed(Long sizeInBytesCompressed) {
setSizeInBytesCompressed(sizeInBytesCompressed);
return this;
}
/**
*
* The total number of objects that Amazon Macie can't analyze in the bucket. These objects don't use a supported
* storage class or don't have a file name extension for a supported file or storage format.
*
*
* @param unclassifiableObjectCount
* The total number of objects that Amazon Macie can't analyze in the bucket. These objects don't use a
* supported storage class or don't have a file name extension for a supported file or storage format.
*/
public void setUnclassifiableObjectCount(ObjectLevelStatistics unclassifiableObjectCount) {
this.unclassifiableObjectCount = unclassifiableObjectCount;
}
/**
*
* The total number of objects that Amazon Macie can't analyze in the bucket. These objects don't use a supported
* storage class or don't have a file name extension for a supported file or storage format.
*
*
* @return The total number of objects that Amazon Macie can't analyze in the bucket. These objects don't use a
* supported storage class or don't have a file name extension for a supported file or storage format.
*/
public ObjectLevelStatistics getUnclassifiableObjectCount() {
return this.unclassifiableObjectCount;
}
/**
*
* The total number of objects that Amazon Macie can't analyze in the bucket. These objects don't use a supported
* storage class or don't have a file name extension for a supported file or storage format.
*
*
* @param unclassifiableObjectCount
* The total number of objects that Amazon Macie can't analyze in the bucket. These objects don't use a
* supported storage class or don't have a file name extension for a supported file or storage format.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MatchingBucket withUnclassifiableObjectCount(ObjectLevelStatistics unclassifiableObjectCount) {
setUnclassifiableObjectCount(unclassifiableObjectCount);
return this;
}
/**
*
* The total storage size, in bytes, of the objects that Amazon Macie can't analyze in the bucket. These objects
* don't use a supported storage class or don't have a file name extension for a supported file or storage format.
*
*
* @param unclassifiableObjectSizeInBytes
* The total storage size, in bytes, of the objects that Amazon Macie can't analyze in the bucket. These
* objects don't use a supported storage class or don't have a file name extension for a supported file or
* storage format.
*/
public void setUnclassifiableObjectSizeInBytes(ObjectLevelStatistics unclassifiableObjectSizeInBytes) {
this.unclassifiableObjectSizeInBytes = unclassifiableObjectSizeInBytes;
}
/**
*
* The total storage size, in bytes, of the objects that Amazon Macie can't analyze in the bucket. These objects
* don't use a supported storage class or don't have a file name extension for a supported file or storage format.
*
*
* @return The total storage size, in bytes, of the objects that Amazon Macie can't analyze in the bucket. These
* objects don't use a supported storage class or don't have a file name extension for a supported file or
* storage format.
*/
public ObjectLevelStatistics getUnclassifiableObjectSizeInBytes() {
return this.unclassifiableObjectSizeInBytes;
}
/**
*
* The total storage size, in bytes, of the objects that Amazon Macie can't analyze in the bucket. These objects
* don't use a supported storage class or don't have a file name extension for a supported file or storage format.
*
*
* @param unclassifiableObjectSizeInBytes
* The total storage size, in bytes, of the objects that Amazon Macie can't analyze in the bucket. These
* objects don't use a supported storage class or don't have a file name extension for a supported file or
* storage format.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MatchingBucket withUnclassifiableObjectSizeInBytes(ObjectLevelStatistics unclassifiableObjectSizeInBytes) {
setUnclassifiableObjectSizeInBytes(unclassifiableObjectSizeInBytes);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getAccountId() != null)
sb.append("AccountId: ").append(getAccountId()).append(",");
if (getBucketName() != null)
sb.append("BucketName: ").append(getBucketName()).append(",");
if (getClassifiableObjectCount() != null)
sb.append("ClassifiableObjectCount: ").append(getClassifiableObjectCount()).append(",");
if (getClassifiableSizeInBytes() != null)
sb.append("ClassifiableSizeInBytes: ").append(getClassifiableSizeInBytes()).append(",");
if (getErrorCode() != null)
sb.append("ErrorCode: ").append(getErrorCode()).append(",");
if (getErrorMessage() != null)
sb.append("ErrorMessage: ").append(getErrorMessage()).append(",");
if (getJobDetails() != null)
sb.append("JobDetails: ").append(getJobDetails()).append(",");
if (getLastAutomatedDiscoveryTime() != null)
sb.append("LastAutomatedDiscoveryTime: ").append(getLastAutomatedDiscoveryTime()).append(",");
if (getObjectCount() != null)
sb.append("ObjectCount: ").append(getObjectCount()).append(",");
if (getObjectCountByEncryptionType() != null)
sb.append("ObjectCountByEncryptionType: ").append(getObjectCountByEncryptionType()).append(",");
if (getSensitivityScore() != null)
sb.append("SensitivityScore: ").append(getSensitivityScore()).append(",");
if (getSizeInBytes() != null)
sb.append("SizeInBytes: ").append(getSizeInBytes()).append(",");
if (getSizeInBytesCompressed() != null)
sb.append("SizeInBytesCompressed: ").append(getSizeInBytesCompressed()).append(",");
if (getUnclassifiableObjectCount() != null)
sb.append("UnclassifiableObjectCount: ").append(getUnclassifiableObjectCount()).append(",");
if (getUnclassifiableObjectSizeInBytes() != null)
sb.append("UnclassifiableObjectSizeInBytes: ").append(getUnclassifiableObjectSizeInBytes());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof MatchingBucket == false)
return false;
MatchingBucket other = (MatchingBucket) obj;
if (other.getAccountId() == null ^ this.getAccountId() == null)
return false;
if (other.getAccountId() != null && other.getAccountId().equals(this.getAccountId()) == false)
return false;
if (other.getBucketName() == null ^ this.getBucketName() == null)
return false;
if (other.getBucketName() != null && other.getBucketName().equals(this.getBucketName()) == false)
return false;
if (other.getClassifiableObjectCount() == null ^ this.getClassifiableObjectCount() == null)
return false;
if (other.getClassifiableObjectCount() != null && other.getClassifiableObjectCount().equals(this.getClassifiableObjectCount()) == false)
return false;
if (other.getClassifiableSizeInBytes() == null ^ this.getClassifiableSizeInBytes() == null)
return false;
if (other.getClassifiableSizeInBytes() != null && other.getClassifiableSizeInBytes().equals(this.getClassifiableSizeInBytes()) == false)
return false;
if (other.getErrorCode() == null ^ this.getErrorCode() == null)
return false;
if (other.getErrorCode() != null && other.getErrorCode().equals(this.getErrorCode()) == false)
return false;
if (other.getErrorMessage() == null ^ this.getErrorMessage() == null)
return false;
if (other.getErrorMessage() != null && other.getErrorMessage().equals(this.getErrorMessage()) == false)
return false;
if (other.getJobDetails() == null ^ this.getJobDetails() == null)
return false;
if (other.getJobDetails() != null && other.getJobDetails().equals(this.getJobDetails()) == false)
return false;
if (other.getLastAutomatedDiscoveryTime() == null ^ this.getLastAutomatedDiscoveryTime() == null)
return false;
if (other.getLastAutomatedDiscoveryTime() != null && other.getLastAutomatedDiscoveryTime().equals(this.getLastAutomatedDiscoveryTime()) == false)
return false;
if (other.getObjectCount() == null ^ this.getObjectCount() == null)
return false;
if (other.getObjectCount() != null && other.getObjectCount().equals(this.getObjectCount()) == false)
return false;
if (other.getObjectCountByEncryptionType() == null ^ this.getObjectCountByEncryptionType() == null)
return false;
if (other.getObjectCountByEncryptionType() != null && other.getObjectCountByEncryptionType().equals(this.getObjectCountByEncryptionType()) == false)
return false;
if (other.getSensitivityScore() == null ^ this.getSensitivityScore() == null)
return false;
if (other.getSensitivityScore() != null && other.getSensitivityScore().equals(this.getSensitivityScore()) == false)
return false;
if (other.getSizeInBytes() == null ^ this.getSizeInBytes() == null)
return false;
if (other.getSizeInBytes() != null && other.getSizeInBytes().equals(this.getSizeInBytes()) == false)
return false;
if (other.getSizeInBytesCompressed() == null ^ this.getSizeInBytesCompressed() == null)
return false;
if (other.getSizeInBytesCompressed() != null && other.getSizeInBytesCompressed().equals(this.getSizeInBytesCompressed()) == false)
return false;
if (other.getUnclassifiableObjectCount() == null ^ this.getUnclassifiableObjectCount() == null)
return false;
if (other.getUnclassifiableObjectCount() != null && other.getUnclassifiableObjectCount().equals(this.getUnclassifiableObjectCount()) == false)
return false;
if (other.getUnclassifiableObjectSizeInBytes() == null ^ this.getUnclassifiableObjectSizeInBytes() == null)
return false;
if (other.getUnclassifiableObjectSizeInBytes() != null
&& other.getUnclassifiableObjectSizeInBytes().equals(this.getUnclassifiableObjectSizeInBytes()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getAccountId() == null) ? 0 : getAccountId().hashCode());
hashCode = prime * hashCode + ((getBucketName() == null) ? 0 : getBucketName().hashCode());
hashCode = prime * hashCode + ((getClassifiableObjectCount() == null) ? 0 : getClassifiableObjectCount().hashCode());
hashCode = prime * hashCode + ((getClassifiableSizeInBytes() == null) ? 0 : getClassifiableSizeInBytes().hashCode());
hashCode = prime * hashCode + ((getErrorCode() == null) ? 0 : getErrorCode().hashCode());
hashCode = prime * hashCode + ((getErrorMessage() == null) ? 0 : getErrorMessage().hashCode());
hashCode = prime * hashCode + ((getJobDetails() == null) ? 0 : getJobDetails().hashCode());
hashCode = prime * hashCode + ((getLastAutomatedDiscoveryTime() == null) ? 0 : getLastAutomatedDiscoveryTime().hashCode());
hashCode = prime * hashCode + ((getObjectCount() == null) ? 0 : getObjectCount().hashCode());
hashCode = prime * hashCode + ((getObjectCountByEncryptionType() == null) ? 0 : getObjectCountByEncryptionType().hashCode());
hashCode = prime * hashCode + ((getSensitivityScore() == null) ? 0 : getSensitivityScore().hashCode());
hashCode = prime * hashCode + ((getSizeInBytes() == null) ? 0 : getSizeInBytes().hashCode());
hashCode = prime * hashCode + ((getSizeInBytesCompressed() == null) ? 0 : getSizeInBytesCompressed().hashCode());
hashCode = prime * hashCode + ((getUnclassifiableObjectCount() == null) ? 0 : getUnclassifiableObjectCount().hashCode());
hashCode = prime * hashCode + ((getUnclassifiableObjectSizeInBytes() == null) ? 0 : getUnclassifiableObjectSizeInBytes().hashCode());
return hashCode;
}
@Override
public MatchingBucket clone() {
try {
return (MatchingBucket) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.macie2.model.transform.MatchingBucketMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}