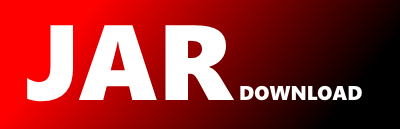
com.amazonaws.services.macie2.AmazonMacie2 Maven / Gradle / Ivy
Show all versions of aws-java-sdk-macie2 Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.macie2;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.macie2.model.*;
import com.amazonaws.services.macie2.waiters.AmazonMacie2Waiters;
/**
* Interface for accessing Amazon Macie 2.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.macie2.AbstractAmazonMacie2} instead.
*
*
*
* Amazon Macie
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonMacie2 {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "macie2";
/**
*
* Accepts an Amazon Macie membership invitation that was received from a specific account.
*
*
* @param acceptInvitationRequest
* @return Result of the AcceptInvitation operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.AcceptInvitation
* @see AWS API
* Documentation
*/
AcceptInvitationResult acceptInvitation(AcceptInvitationRequest acceptInvitationRequest);
/**
*
* Retrieves information about one or more custom data identifiers.
*
*
* @param batchGetCustomDataIdentifiersRequest
* @return Result of the BatchGetCustomDataIdentifiers operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.BatchGetCustomDataIdentifiers
* @see AWS API Documentation
*/
BatchGetCustomDataIdentifiersResult batchGetCustomDataIdentifiers(BatchGetCustomDataIdentifiersRequest batchGetCustomDataIdentifiersRequest);
/**
*
* Changes the status of automated sensitive data discovery for one or more accounts.
*
*
* @param batchUpdateAutomatedDiscoveryAccountsRequest
* @return Result of the BatchUpdateAutomatedDiscoveryAccounts operation returned by the service.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @sample AmazonMacie2.BatchUpdateAutomatedDiscoveryAccounts
* @see AWS API Documentation
*/
BatchUpdateAutomatedDiscoveryAccountsResult batchUpdateAutomatedDiscoveryAccounts(
BatchUpdateAutomatedDiscoveryAccountsRequest batchUpdateAutomatedDiscoveryAccountsRequest);
/**
*
* Creates and defines the settings for an allow list.
*
*
* @param createAllowListRequest
* @return Result of the CreateAllowList operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.CreateAllowList
* @see AWS API
* Documentation
*/
CreateAllowListResult createAllowList(CreateAllowListRequest createAllowListRequest);
/**
*
* Creates and defines the settings for a classification job.
*
*
* @param createClassificationJobRequest
* @return Result of the CreateClassificationJob operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.CreateClassificationJob
* @see AWS
* API Documentation
*/
CreateClassificationJobResult createClassificationJob(CreateClassificationJobRequest createClassificationJobRequest);
/**
*
* Creates and defines the criteria and other settings for a custom data identifier.
*
*
* @param createCustomDataIdentifierRequest
* @return Result of the CreateCustomDataIdentifier operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.CreateCustomDataIdentifier
* @see AWS API Documentation
*/
CreateCustomDataIdentifierResult createCustomDataIdentifier(CreateCustomDataIdentifierRequest createCustomDataIdentifierRequest);
/**
*
* Creates and defines the criteria and other settings for a findings filter.
*
*
* @param createFindingsFilterRequest
* @return Result of the CreateFindingsFilter operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.CreateFindingsFilter
* @see AWS
* API Documentation
*/
CreateFindingsFilterResult createFindingsFilter(CreateFindingsFilterRequest createFindingsFilterRequest);
/**
*
* Sends an Amazon Macie membership invitation to one or more accounts.
*
*
* @param createInvitationsRequest
* @return Result of the CreateInvitations operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.CreateInvitations
* @see AWS API
* Documentation
*/
CreateInvitationsResult createInvitations(CreateInvitationsRequest createInvitationsRequest);
/**
*
* Associates an account with an Amazon Macie administrator account.
*
*
* @param createMemberRequest
* @return Result of the CreateMember operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.CreateMember
* @see AWS API
* Documentation
*/
CreateMemberResult createMember(CreateMemberRequest createMemberRequest);
/**
*
* Creates sample findings.
*
*
* @param createSampleFindingsRequest
* @return Result of the CreateSampleFindings operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.CreateSampleFindings
* @see AWS
* API Documentation
*/
CreateSampleFindingsResult createSampleFindings(CreateSampleFindingsRequest createSampleFindingsRequest);
/**
*
* Declines Amazon Macie membership invitations that were received from specific accounts.
*
*
* @param declineInvitationsRequest
* @return Result of the DeclineInvitations operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.DeclineInvitations
* @see AWS API
* Documentation
*/
DeclineInvitationsResult declineInvitations(DeclineInvitationsRequest declineInvitationsRequest);
/**
*
* Deletes an allow list.
*
*
* @param deleteAllowListRequest
* @return Result of the DeleteAllowList operation returned by the service.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @sample AmazonMacie2.DeleteAllowList
* @see AWS API
* Documentation
*/
DeleteAllowListResult deleteAllowList(DeleteAllowListRequest deleteAllowListRequest);
/**
*
* Soft deletes a custom data identifier.
*
*
* @param deleteCustomDataIdentifierRequest
* @return Result of the DeleteCustomDataIdentifier operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.DeleteCustomDataIdentifier
* @see AWS API Documentation
*/
DeleteCustomDataIdentifierResult deleteCustomDataIdentifier(DeleteCustomDataIdentifierRequest deleteCustomDataIdentifierRequest);
/**
*
* Deletes a findings filter.
*
*
* @param deleteFindingsFilterRequest
* @return Result of the DeleteFindingsFilter operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.DeleteFindingsFilter
* @see AWS
* API Documentation
*/
DeleteFindingsFilterResult deleteFindingsFilter(DeleteFindingsFilterRequest deleteFindingsFilterRequest);
/**
*
* Deletes Amazon Macie membership invitations that were received from specific accounts.
*
*
* @param deleteInvitationsRequest
* @return Result of the DeleteInvitations operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.DeleteInvitations
* @see AWS API
* Documentation
*/
DeleteInvitationsResult deleteInvitations(DeleteInvitationsRequest deleteInvitationsRequest);
/**
*
* Deletes the association between an Amazon Macie administrator account and an account.
*
*
* @param deleteMemberRequest
* @return Result of the DeleteMember operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.DeleteMember
* @see AWS API
* Documentation
*/
DeleteMemberResult deleteMember(DeleteMemberRequest deleteMemberRequest);
/**
*
* Retrieves (queries) statistical data and other information about one or more S3 buckets that Amazon Macie
* monitors and analyzes for an account.
*
*
* @param describeBucketsRequest
* @return Result of the DescribeBuckets operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.DescribeBuckets
* @see AWS API
* Documentation
*/
DescribeBucketsResult describeBuckets(DescribeBucketsRequest describeBucketsRequest);
/**
*
* Retrieves the status and settings for a classification job.
*
*
* @param describeClassificationJobRequest
* @return Result of the DescribeClassificationJob operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.DescribeClassificationJob
* @see AWS API Documentation
*/
DescribeClassificationJobResult describeClassificationJob(DescribeClassificationJobRequest describeClassificationJobRequest);
/**
*
* Retrieves the Amazon Macie configuration settings for an organization in Organizations.
*
*
* @param describeOrganizationConfigurationRequest
* @return Result of the DescribeOrganizationConfiguration operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.DescribeOrganizationConfiguration
* @see AWS API Documentation
*/
DescribeOrganizationConfigurationResult describeOrganizationConfiguration(DescribeOrganizationConfigurationRequest describeOrganizationConfigurationRequest);
/**
*
* Disables Amazon Macie and deletes all settings and resources for a Macie account.
*
*
* @param disableMacieRequest
* @return Result of the DisableMacie operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.DisableMacie
* @see AWS API
* Documentation
*/
DisableMacieResult disableMacie(DisableMacieRequest disableMacieRequest);
/**
*
* Disables an account as the delegated Amazon Macie administrator account for an organization in Organizations.
*
*
* @param disableOrganizationAdminAccountRequest
* @return Result of the DisableOrganizationAdminAccount operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.DisableOrganizationAdminAccount
* @see AWS API Documentation
*/
DisableOrganizationAdminAccountResult disableOrganizationAdminAccount(DisableOrganizationAdminAccountRequest disableOrganizationAdminAccountRequest);
/**
*
* Disassociates a member account from its Amazon Macie administrator account.
*
*
* @param disassociateFromAdministratorAccountRequest
* @return Result of the DisassociateFromAdministratorAccount operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.DisassociateFromAdministratorAccount
* @see AWS API Documentation
*/
DisassociateFromAdministratorAccountResult disassociateFromAdministratorAccount(
DisassociateFromAdministratorAccountRequest disassociateFromAdministratorAccountRequest);
/**
*
* (Deprecated) Disassociates a member account from its Amazon Macie administrator account. This operation has been
* replaced by the DisassociateFromAdministratorAccount
* operation.
*
*
* @param disassociateFromMasterAccountRequest
* @return Result of the DisassociateFromMasterAccount operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.DisassociateFromMasterAccount
* @see AWS API Documentation
*/
DisassociateFromMasterAccountResult disassociateFromMasterAccount(DisassociateFromMasterAccountRequest disassociateFromMasterAccountRequest);
/**
*
* Disassociates an Amazon Macie administrator account from a member account.
*
*
* @param disassociateMemberRequest
* @return Result of the DisassociateMember operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.DisassociateMember
* @see AWS API
* Documentation
*/
DisassociateMemberResult disassociateMember(DisassociateMemberRequest disassociateMemberRequest);
/**
*
* Enables Amazon Macie and specifies the configuration settings for a Macie account.
*
*
* @param enableMacieRequest
* @return Result of the EnableMacie operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.EnableMacie
* @see AWS API
* Documentation
*/
EnableMacieResult enableMacie(EnableMacieRequest enableMacieRequest);
/**
*
* Designates an account as the delegated Amazon Macie administrator account for an organization in Organizations.
*
*
* @param enableOrganizationAdminAccountRequest
* @return Result of the EnableOrganizationAdminAccount operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.EnableOrganizationAdminAccount
* @see AWS API Documentation
*/
EnableOrganizationAdminAccountResult enableOrganizationAdminAccount(EnableOrganizationAdminAccountRequest enableOrganizationAdminAccountRequest);
/**
*
* Retrieves information about the Amazon Macie administrator account for an account.
*
*
* @param getAdministratorAccountRequest
* @return Result of the GetAdministratorAccount operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.GetAdministratorAccount
* @see AWS
* API Documentation
*/
GetAdministratorAccountResult getAdministratorAccount(GetAdministratorAccountRequest getAdministratorAccountRequest);
/**
*
* Retrieves the settings and status of an allow list.
*
*
* @param getAllowListRequest
* @return Result of the GetAllowList operation returned by the service.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @sample AmazonMacie2.GetAllowList
* @see AWS API
* Documentation
*/
GetAllowListResult getAllowList(GetAllowListRequest getAllowListRequest);
/**
*
* Retrieves the configuration settings and status of automated sensitive data discovery for an organization or
* standalone account.
*
*
* @param getAutomatedDiscoveryConfigurationRequest
* @return Result of the GetAutomatedDiscoveryConfiguration operation returned by the service.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @sample AmazonMacie2.GetAutomatedDiscoveryConfiguration
* @see AWS API Documentation
*/
GetAutomatedDiscoveryConfigurationResult getAutomatedDiscoveryConfiguration(
GetAutomatedDiscoveryConfigurationRequest getAutomatedDiscoveryConfigurationRequest);
/**
*
* Retrieves (queries) aggregated statistical data about all the S3 buckets that Amazon Macie monitors and analyzes
* for an account.
*
*
* @param getBucketStatisticsRequest
* @return Result of the GetBucketStatistics operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.GetBucketStatistics
* @see AWS API
* Documentation
*/
GetBucketStatisticsResult getBucketStatistics(GetBucketStatisticsRequest getBucketStatisticsRequest);
/**
*
* Retrieves the configuration settings for storing data classification results.
*
*
* @param getClassificationExportConfigurationRequest
* @return Result of the GetClassificationExportConfiguration operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.GetClassificationExportConfiguration
* @see AWS API Documentation
*/
GetClassificationExportConfigurationResult getClassificationExportConfiguration(
GetClassificationExportConfigurationRequest getClassificationExportConfigurationRequest);
/**
*
* Retrieves the classification scope settings for an account.
*
*
* @param getClassificationScopeRequest
* @return Result of the GetClassificationScope operation returned by the service.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @sample AmazonMacie2.GetClassificationScope
* @see AWS
* API Documentation
*/
GetClassificationScopeResult getClassificationScope(GetClassificationScopeRequest getClassificationScopeRequest);
/**
*
* Retrieves the criteria and other settings for a custom data identifier.
*
*
* @param getCustomDataIdentifierRequest
* @return Result of the GetCustomDataIdentifier operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.GetCustomDataIdentifier
* @see AWS
* API Documentation
*/
GetCustomDataIdentifierResult getCustomDataIdentifier(GetCustomDataIdentifierRequest getCustomDataIdentifierRequest);
/**
*
* Retrieves (queries) aggregated statistical data about findings.
*
*
* @param getFindingStatisticsRequest
* @return Result of the GetFindingStatistics operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.GetFindingStatistics
* @see AWS
* API Documentation
*/
GetFindingStatisticsResult getFindingStatistics(GetFindingStatisticsRequest getFindingStatisticsRequest);
/**
*
* Retrieves the details of one or more findings.
*
*
* @param getFindingsRequest
* @return Result of the GetFindings operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.GetFindings
* @see AWS API
* Documentation
*/
GetFindingsResult getFindings(GetFindingsRequest getFindingsRequest);
/**
*
* Retrieves the criteria and other settings for a findings filter.
*
*
* @param getFindingsFilterRequest
* @return Result of the GetFindingsFilter operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.GetFindingsFilter
* @see AWS API
* Documentation
*/
GetFindingsFilterResult getFindingsFilter(GetFindingsFilterRequest getFindingsFilterRequest);
/**
*
* Retrieves the configuration settings for publishing findings to Security Hub.
*
*
* @param getFindingsPublicationConfigurationRequest
* @return Result of the GetFindingsPublicationConfiguration operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.GetFindingsPublicationConfiguration
* @see AWS API Documentation
*/
GetFindingsPublicationConfigurationResult getFindingsPublicationConfiguration(
GetFindingsPublicationConfigurationRequest getFindingsPublicationConfigurationRequest);
/**
*
* Retrieves the count of Amazon Macie membership invitations that were received by an account.
*
*
* @param getInvitationsCountRequest
* @return Result of the GetInvitationsCount operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.GetInvitationsCount
* @see AWS API
* Documentation
*/
GetInvitationsCountResult getInvitationsCount(GetInvitationsCountRequest getInvitationsCountRequest);
/**
*
* Retrieves the status and configuration settings for an Amazon Macie account.
*
*
* @param getMacieSessionRequest
* @return Result of the GetMacieSession operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.GetMacieSession
* @see AWS API
* Documentation
*/
GetMacieSessionResult getMacieSession(GetMacieSessionRequest getMacieSessionRequest);
/**
*
* (Deprecated) Retrieves information about the Amazon Macie administrator account for an account. This operation
* has been replaced by the GetAdministratorAccount operation.
*
*
* @param getMasterAccountRequest
* @return Result of the GetMasterAccount operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.GetMasterAccount
* @see AWS API
* Documentation
*/
GetMasterAccountResult getMasterAccount(GetMasterAccountRequest getMasterAccountRequest);
/**
*
* Retrieves information about an account that's associated with an Amazon Macie administrator account.
*
*
* @param getMemberRequest
* @return Result of the GetMember operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.GetMember
* @see AWS API
* Documentation
*/
GetMemberResult getMember(GetMemberRequest getMemberRequest);
/**
*
* Retrieves (queries) sensitive data discovery statistics and the sensitivity score for an S3 bucket.
*
*
* @param getResourceProfileRequest
* @return Result of the GetResourceProfile operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @sample AmazonMacie2.GetResourceProfile
* @see AWS API
* Documentation
*/
GetResourceProfileResult getResourceProfile(GetResourceProfileRequest getResourceProfileRequest);
/**
*
* Retrieves the status and configuration settings for retrieving occurrences of sensitive data reported by
* findings.
*
*
* @param getRevealConfigurationRequest
* @return Result of the GetRevealConfiguration operation returned by the service.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @sample AmazonMacie2.GetRevealConfiguration
* @see AWS
* API Documentation
*/
GetRevealConfigurationResult getRevealConfiguration(GetRevealConfigurationRequest getRevealConfigurationRequest);
/**
*
* Retrieves occurrences of sensitive data reported by a finding.
*
*
* @param getSensitiveDataOccurrencesRequest
* @return Result of the GetSensitiveDataOccurrences operation returned by the service.
* @throws UnprocessableEntityException
* The request failed because it contains instructions that Amazon Macie can't process (Unprocessable
* Entity).
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @sample AmazonMacie2.GetSensitiveDataOccurrences
* @see AWS API Documentation
*/
GetSensitiveDataOccurrencesResult getSensitiveDataOccurrences(GetSensitiveDataOccurrencesRequest getSensitiveDataOccurrencesRequest);
/**
*
* Checks whether occurrences of sensitive data can be retrieved for a finding.
*
*
* @param getSensitiveDataOccurrencesAvailabilityRequest
* @return Result of the GetSensitiveDataOccurrencesAvailability operation returned by the service.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @sample AmazonMacie2.GetSensitiveDataOccurrencesAvailability
* @see AWS API Documentation
*/
GetSensitiveDataOccurrencesAvailabilityResult getSensitiveDataOccurrencesAvailability(
GetSensitiveDataOccurrencesAvailabilityRequest getSensitiveDataOccurrencesAvailabilityRequest);
/**
*
* Retrieves the settings for the sensitivity inspection template for an account.
*
*
* @param getSensitivityInspectionTemplateRequest
* @return Result of the GetSensitivityInspectionTemplate operation returned by the service.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @sample AmazonMacie2.GetSensitivityInspectionTemplate
* @see AWS API Documentation
*/
GetSensitivityInspectionTemplateResult getSensitivityInspectionTemplate(GetSensitivityInspectionTemplateRequest getSensitivityInspectionTemplateRequest);
/**
*
* Retrieves (queries) quotas and aggregated usage data for one or more accounts.
*
*
* @param getUsageStatisticsRequest
* @return Result of the GetUsageStatistics operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.GetUsageStatistics
* @see AWS API
* Documentation
*/
GetUsageStatisticsResult getUsageStatistics(GetUsageStatisticsRequest getUsageStatisticsRequest);
/**
*
* Retrieves (queries) aggregated usage data for an account.
*
*
* @param getUsageTotalsRequest
* @return Result of the GetUsageTotals operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.GetUsageTotals
* @see AWS API
* Documentation
*/
GetUsageTotalsResult getUsageTotals(GetUsageTotalsRequest getUsageTotalsRequest);
/**
*
* Retrieves a subset of information about all the allow lists for an account.
*
*
* @param listAllowListsRequest
* @return Result of the ListAllowLists operation returned by the service.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @sample AmazonMacie2.ListAllowLists
* @see AWS API
* Documentation
*/
ListAllowListsResult listAllowLists(ListAllowListsRequest listAllowListsRequest);
/**
*
* Retrieves the status of automated sensitive data discovery for one or more accounts.
*
*
* @param listAutomatedDiscoveryAccountsRequest
* @return Result of the ListAutomatedDiscoveryAccounts operation returned by the service.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @sample AmazonMacie2.ListAutomatedDiscoveryAccounts
* @see AWS API Documentation
*/
ListAutomatedDiscoveryAccountsResult listAutomatedDiscoveryAccounts(ListAutomatedDiscoveryAccountsRequest listAutomatedDiscoveryAccountsRequest);
/**
*
* Retrieves a subset of information about one or more classification jobs.
*
*
* @param listClassificationJobsRequest
* @return Result of the ListClassificationJobs operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.ListClassificationJobs
* @see AWS
* API Documentation
*/
ListClassificationJobsResult listClassificationJobs(ListClassificationJobsRequest listClassificationJobsRequest);
/**
*
* Retrieves a subset of information about the classification scope for an account.
*
*
* @param listClassificationScopesRequest
* @return Result of the ListClassificationScopes operation returned by the service.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @sample AmazonMacie2.ListClassificationScopes
* @see AWS API Documentation
*/
ListClassificationScopesResult listClassificationScopes(ListClassificationScopesRequest listClassificationScopesRequest);
/**
*
* Retrieves a subset of information about all the custom data identifiers for an account.
*
*
* @param listCustomDataIdentifiersRequest
* @return Result of the ListCustomDataIdentifiers operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.ListCustomDataIdentifiers
* @see AWS API Documentation
*/
ListCustomDataIdentifiersResult listCustomDataIdentifiers(ListCustomDataIdentifiersRequest listCustomDataIdentifiersRequest);
/**
*
* Retrieves a subset of information about one or more findings.
*
*
* @param listFindingsRequest
* @return Result of the ListFindings operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.ListFindings
* @see AWS API
* Documentation
*/
ListFindingsResult listFindings(ListFindingsRequest listFindingsRequest);
/**
*
* Retrieves a subset of information about all the findings filters for an account.
*
*
* @param listFindingsFiltersRequest
* @return Result of the ListFindingsFilters operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.ListFindingsFilters
* @see AWS API
* Documentation
*/
ListFindingsFiltersResult listFindingsFilters(ListFindingsFiltersRequest listFindingsFiltersRequest);
/**
*
* Retrieves information about Amazon Macie membership invitations that were received by an account.
*
*
* @param listInvitationsRequest
* @return Result of the ListInvitations operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.ListInvitations
* @see AWS API
* Documentation
*/
ListInvitationsResult listInvitations(ListInvitationsRequest listInvitationsRequest);
/**
*
* Retrieves information about all the managed data identifiers that Amazon Macie currently provides.
*
*
* @param listManagedDataIdentifiersRequest
* @return Result of the ListManagedDataIdentifiers operation returned by the service.
* @sample AmazonMacie2.ListManagedDataIdentifiers
* @see AWS API Documentation
*/
ListManagedDataIdentifiersResult listManagedDataIdentifiers(ListManagedDataIdentifiersRequest listManagedDataIdentifiersRequest);
/**
*
* Retrieves information about the accounts that are associated with an Amazon Macie administrator account.
*
*
* @param listMembersRequest
* @return Result of the ListMembers operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.ListMembers
* @see AWS API
* Documentation
*/
ListMembersResult listMembers(ListMembersRequest listMembersRequest);
/**
*
* Retrieves information about the delegated Amazon Macie administrator account for an organization in
* Organizations.
*
*
* @param listOrganizationAdminAccountsRequest
* @return Result of the ListOrganizationAdminAccounts operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.ListOrganizationAdminAccounts
* @see AWS API Documentation
*/
ListOrganizationAdminAccountsResult listOrganizationAdminAccounts(ListOrganizationAdminAccountsRequest listOrganizationAdminAccountsRequest);
/**
*
* Retrieves information about objects that Amazon Macie selected from an S3 bucket for automated sensitive data
* discovery.
*
*
* @param listResourceProfileArtifactsRequest
* @return Result of the ListResourceProfileArtifacts operation returned by the service.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @sample AmazonMacie2.ListResourceProfileArtifacts
* @see AWS API Documentation
*/
ListResourceProfileArtifactsResult listResourceProfileArtifacts(ListResourceProfileArtifactsRequest listResourceProfileArtifactsRequest);
/**
*
* Retrieves information about the types and amount of sensitive data that Amazon Macie found in an S3 bucket.
*
*
* @param listResourceProfileDetectionsRequest
* @return Result of the ListResourceProfileDetections operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @sample AmazonMacie2.ListResourceProfileDetections
* @see AWS API Documentation
*/
ListResourceProfileDetectionsResult listResourceProfileDetections(ListResourceProfileDetectionsRequest listResourceProfileDetectionsRequest);
/**
*
* Retrieves a subset of information about the sensitivity inspection template for an account.
*
*
* @param listSensitivityInspectionTemplatesRequest
* @return Result of the ListSensitivityInspectionTemplates operation returned by the service.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @sample AmazonMacie2.ListSensitivityInspectionTemplates
* @see AWS API Documentation
*/
ListSensitivityInspectionTemplatesResult listSensitivityInspectionTemplates(
ListSensitivityInspectionTemplatesRequest listSensitivityInspectionTemplatesRequest);
/**
*
* Retrieves the tags (keys and values) that are associated with an Amazon Macie resource.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @sample AmazonMacie2.ListTagsForResource
* @see AWS API
* Documentation
*/
ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Adds or updates the configuration settings for storing data classification results.
*
*
* @param putClassificationExportConfigurationRequest
* @return Result of the PutClassificationExportConfiguration operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.PutClassificationExportConfiguration
* @see AWS API Documentation
*/
PutClassificationExportConfigurationResult putClassificationExportConfiguration(
PutClassificationExportConfigurationRequest putClassificationExportConfigurationRequest);
/**
*
* Updates the configuration settings for publishing findings to Security Hub.
*
*
* @param putFindingsPublicationConfigurationRequest
* @return Result of the PutFindingsPublicationConfiguration operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.PutFindingsPublicationConfiguration
* @see AWS API Documentation
*/
PutFindingsPublicationConfigurationResult putFindingsPublicationConfiguration(
PutFindingsPublicationConfigurationRequest putFindingsPublicationConfigurationRequest);
/**
*
* Retrieves (queries) statistical data and other information about Amazon Web Services resources that Amazon Macie
* monitors and analyzes.
*
*
* @param searchResourcesRequest
* @return Result of the SearchResources operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.SearchResources
* @see AWS API
* Documentation
*/
SearchResourcesResult searchResources(SearchResourcesRequest searchResourcesRequest);
/**
*
* Adds or updates one or more tags (keys and values) that are associated with an Amazon Macie resource.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @sample AmazonMacie2.TagResource
* @see AWS API
* Documentation
*/
TagResourceResult tagResource(TagResourceRequest tagResourceRequest);
/**
*
* Tests criteria for a custom data identifier.
*
*
* @param testCustomDataIdentifierRequest
* @return Result of the TestCustomDataIdentifier operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.TestCustomDataIdentifier
* @see AWS API Documentation
*/
TestCustomDataIdentifierResult testCustomDataIdentifier(TestCustomDataIdentifierRequest testCustomDataIdentifierRequest);
/**
*
* Removes one or more tags (keys and values) from an Amazon Macie resource.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @sample AmazonMacie2.UntagResource
* @see AWS API
* Documentation
*/
UntagResourceResult untagResource(UntagResourceRequest untagResourceRequest);
/**
*
* Updates the settings for an allow list.
*
*
* @param updateAllowListRequest
* @return Result of the UpdateAllowList operation returned by the service.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @sample AmazonMacie2.UpdateAllowList
* @see AWS API
* Documentation
*/
UpdateAllowListResult updateAllowList(UpdateAllowListRequest updateAllowListRequest);
/**
*
* Changes the configuration settings and status of automated sensitive data discovery for an organization or
* standalone account.
*
*
* @param updateAutomatedDiscoveryConfigurationRequest
* @return Result of the UpdateAutomatedDiscoveryConfiguration operation returned by the service.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @sample AmazonMacie2.UpdateAutomatedDiscoveryConfiguration
* @see AWS API Documentation
*/
UpdateAutomatedDiscoveryConfigurationResult updateAutomatedDiscoveryConfiguration(
UpdateAutomatedDiscoveryConfigurationRequest updateAutomatedDiscoveryConfigurationRequest);
/**
*
* Changes the status of a classification job.
*
*
* @param updateClassificationJobRequest
* @return Result of the UpdateClassificationJob operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.UpdateClassificationJob
* @see AWS
* API Documentation
*/
UpdateClassificationJobResult updateClassificationJob(UpdateClassificationJobRequest updateClassificationJobRequest);
/**
*
* Updates the classification scope settings for an account.
*
*
* @param updateClassificationScopeRequest
* @return Result of the UpdateClassificationScope operation returned by the service.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @sample AmazonMacie2.UpdateClassificationScope
* @see AWS API Documentation
*/
UpdateClassificationScopeResult updateClassificationScope(UpdateClassificationScopeRequest updateClassificationScopeRequest);
/**
*
* Updates the criteria and other settings for a findings filter.
*
*
* @param updateFindingsFilterRequest
* @return Result of the UpdateFindingsFilter operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.UpdateFindingsFilter
* @see AWS
* API Documentation
*/
UpdateFindingsFilterResult updateFindingsFilter(UpdateFindingsFilterRequest updateFindingsFilterRequest);
/**
*
* Suspends or re-enables Amazon Macie, or updates the configuration settings for a Macie account.
*
*
* @param updateMacieSessionRequest
* @return Result of the UpdateMacieSession operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.UpdateMacieSession
* @see AWS API
* Documentation
*/
UpdateMacieSessionResult updateMacieSession(UpdateMacieSessionRequest updateMacieSessionRequest);
/**
*
* Enables an Amazon Macie administrator to suspend or re-enable Macie for a member account.
*
*
* @param updateMemberSessionRequest
* @return Result of the UpdateMemberSession operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.UpdateMemberSession
* @see AWS API
* Documentation
*/
UpdateMemberSessionResult updateMemberSession(UpdateMemberSessionRequest updateMemberSessionRequest);
/**
*
* Updates the Amazon Macie configuration settings for an organization in Organizations.
*
*
* @param updateOrganizationConfigurationRequest
* @return Result of the UpdateOrganizationConfiguration operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ConflictException
* The request failed because it conflicts with the current state of the specified resource.
* @sample AmazonMacie2.UpdateOrganizationConfiguration
* @see AWS API Documentation
*/
UpdateOrganizationConfigurationResult updateOrganizationConfiguration(UpdateOrganizationConfigurationRequest updateOrganizationConfigurationRequest);
/**
*
* Updates the sensitivity score for an S3 bucket.
*
*
* @param updateResourceProfileRequest
* @return Result of the UpdateResourceProfile operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @sample AmazonMacie2.UpdateResourceProfile
* @see AWS
* API Documentation
*/
UpdateResourceProfileResult updateResourceProfile(UpdateResourceProfileRequest updateResourceProfileRequest);
/**
*
* Updates the sensitivity scoring settings for an S3 bucket.
*
*
* @param updateResourceProfileDetectionsRequest
* @return Result of the UpdateResourceProfileDetections operation returned by the service.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws ServiceQuotaExceededException
* The request failed because fulfilling the request would exceed one or more service quotas for your
* account.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @sample AmazonMacie2.UpdateResourceProfileDetections
* @see AWS API Documentation
*/
UpdateResourceProfileDetectionsResult updateResourceProfileDetections(UpdateResourceProfileDetectionsRequest updateResourceProfileDetectionsRequest);
/**
*
* Updates the status and configuration settings for retrieving occurrences of sensitive data reported by findings.
*
*
* @param updateRevealConfigurationRequest
* @return Result of the UpdateRevealConfiguration operation returned by the service.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @sample AmazonMacie2.UpdateRevealConfiguration
* @see AWS API Documentation
*/
UpdateRevealConfigurationResult updateRevealConfiguration(UpdateRevealConfigurationRequest updateRevealConfigurationRequest);
/**
*
* Updates the settings for the sensitivity inspection template for an account.
*
*
* @param updateSensitivityInspectionTemplateRequest
* @return Result of the UpdateSensitivityInspectionTemplate operation returned by the service.
* @throws ResourceNotFoundException
* The request failed because the specified resource wasn't found.
* @throws ThrottlingException
* The request failed because you sent too many requests during a certain amount of time.
* @throws ValidationException
* The request failed because the input doesn't satisfy the constraints specified by the service.
* @throws InternalServerException
* The request failed due to an unknown internal server error, exception, or failure.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient access to the specified resource.
* @sample AmazonMacie2.UpdateSensitivityInspectionTemplate
* @see AWS API Documentation
*/
UpdateSensitivityInspectionTemplateResult updateSensitivityInspectionTemplate(
UpdateSensitivityInspectionTemplateRequest updateSensitivityInspectionTemplateRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
AmazonMacie2Waiters waiters();
}