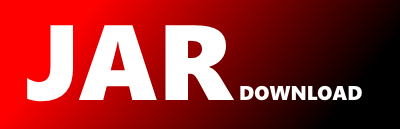
com.amazonaws.services.macie2.model.UserPausedDetails Maven / Gradle / Ivy
Show all versions of aws-java-sdk-macie2 Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.macie2.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Provides information about when a classification job was paused. For a one-time job, this object also specifies when
* the job will expire and be cancelled if it isn't resumed. For a recurring job, this object also specifies when the
* paused job run will expire and be cancelled if it isn't resumed. This object is present only if a job's current
* status (jobStatus) is USER_PAUSED. The information in this object applies only to a job that was paused while it had
* a status of RUNNING.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class UserPausedDetails implements Serializable, Cloneable, StructuredPojo {
/**
*
* The date and time, in UTC and extended ISO 8601 format, when the job or job run will expire and be cancelled if
* you don't resume it first.
*
*/
private java.util.Date jobExpiresAt;
/**
*
* The Amazon Resource Name (ARN) of the Health event that Amazon Macie sent to notify you of the job or job run's
* pending expiration and cancellation. This value is null if a job has been paused for less than 23 days.
*
*/
private String jobImminentExpirationHealthEventArn;
/**
*
* The date and time, in UTC and extended ISO 8601 format, when you paused the job.
*
*/
private java.util.Date jobPausedAt;
/**
*
* The date and time, in UTC and extended ISO 8601 format, when the job or job run will expire and be cancelled if
* you don't resume it first.
*
*
* @param jobExpiresAt
* The date and time, in UTC and extended ISO 8601 format, when the job or job run will expire and be
* cancelled if you don't resume it first.
*/
public void setJobExpiresAt(java.util.Date jobExpiresAt) {
this.jobExpiresAt = jobExpiresAt;
}
/**
*
* The date and time, in UTC and extended ISO 8601 format, when the job or job run will expire and be cancelled if
* you don't resume it first.
*
*
* @return The date and time, in UTC and extended ISO 8601 format, when the job or job run will expire and be
* cancelled if you don't resume it first.
*/
public java.util.Date getJobExpiresAt() {
return this.jobExpiresAt;
}
/**
*
* The date and time, in UTC and extended ISO 8601 format, when the job or job run will expire and be cancelled if
* you don't resume it first.
*
*
* @param jobExpiresAt
* The date and time, in UTC and extended ISO 8601 format, when the job or job run will expire and be
* cancelled if you don't resume it first.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UserPausedDetails withJobExpiresAt(java.util.Date jobExpiresAt) {
setJobExpiresAt(jobExpiresAt);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the Health event that Amazon Macie sent to notify you of the job or job run's
* pending expiration and cancellation. This value is null if a job has been paused for less than 23 days.
*
*
* @param jobImminentExpirationHealthEventArn
* The Amazon Resource Name (ARN) of the Health event that Amazon Macie sent to notify you of the job or job
* run's pending expiration and cancellation. This value is null if a job has been paused for less than 23
* days.
*/
public void setJobImminentExpirationHealthEventArn(String jobImminentExpirationHealthEventArn) {
this.jobImminentExpirationHealthEventArn = jobImminentExpirationHealthEventArn;
}
/**
*
* The Amazon Resource Name (ARN) of the Health event that Amazon Macie sent to notify you of the job or job run's
* pending expiration and cancellation. This value is null if a job has been paused for less than 23 days.
*
*
* @return The Amazon Resource Name (ARN) of the Health event that Amazon Macie sent to notify you of the job or job
* run's pending expiration and cancellation. This value is null if a job has been paused for less than 23
* days.
*/
public String getJobImminentExpirationHealthEventArn() {
return this.jobImminentExpirationHealthEventArn;
}
/**
*
* The Amazon Resource Name (ARN) of the Health event that Amazon Macie sent to notify you of the job or job run's
* pending expiration and cancellation. This value is null if a job has been paused for less than 23 days.
*
*
* @param jobImminentExpirationHealthEventArn
* The Amazon Resource Name (ARN) of the Health event that Amazon Macie sent to notify you of the job or job
* run's pending expiration and cancellation. This value is null if a job has been paused for less than 23
* days.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UserPausedDetails withJobImminentExpirationHealthEventArn(String jobImminentExpirationHealthEventArn) {
setJobImminentExpirationHealthEventArn(jobImminentExpirationHealthEventArn);
return this;
}
/**
*
* The date and time, in UTC and extended ISO 8601 format, when you paused the job.
*
*
* @param jobPausedAt
* The date and time, in UTC and extended ISO 8601 format, when you paused the job.
*/
public void setJobPausedAt(java.util.Date jobPausedAt) {
this.jobPausedAt = jobPausedAt;
}
/**
*
* The date and time, in UTC and extended ISO 8601 format, when you paused the job.
*
*
* @return The date and time, in UTC and extended ISO 8601 format, when you paused the job.
*/
public java.util.Date getJobPausedAt() {
return this.jobPausedAt;
}
/**
*
* The date and time, in UTC and extended ISO 8601 format, when you paused the job.
*
*
* @param jobPausedAt
* The date and time, in UTC and extended ISO 8601 format, when you paused the job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UserPausedDetails withJobPausedAt(java.util.Date jobPausedAt) {
setJobPausedAt(jobPausedAt);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getJobExpiresAt() != null)
sb.append("JobExpiresAt: ").append(getJobExpiresAt()).append(",");
if (getJobImminentExpirationHealthEventArn() != null)
sb.append("JobImminentExpirationHealthEventArn: ").append(getJobImminentExpirationHealthEventArn()).append(",");
if (getJobPausedAt() != null)
sb.append("JobPausedAt: ").append(getJobPausedAt());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof UserPausedDetails == false)
return false;
UserPausedDetails other = (UserPausedDetails) obj;
if (other.getJobExpiresAt() == null ^ this.getJobExpiresAt() == null)
return false;
if (other.getJobExpiresAt() != null && other.getJobExpiresAt().equals(this.getJobExpiresAt()) == false)
return false;
if (other.getJobImminentExpirationHealthEventArn() == null ^ this.getJobImminentExpirationHealthEventArn() == null)
return false;
if (other.getJobImminentExpirationHealthEventArn() != null
&& other.getJobImminentExpirationHealthEventArn().equals(this.getJobImminentExpirationHealthEventArn()) == false)
return false;
if (other.getJobPausedAt() == null ^ this.getJobPausedAt() == null)
return false;
if (other.getJobPausedAt() != null && other.getJobPausedAt().equals(this.getJobPausedAt()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getJobExpiresAt() == null) ? 0 : getJobExpiresAt().hashCode());
hashCode = prime * hashCode + ((getJobImminentExpirationHealthEventArn() == null) ? 0 : getJobImminentExpirationHealthEventArn().hashCode());
hashCode = prime * hashCode + ((getJobPausedAt() == null) ? 0 : getJobPausedAt().hashCode());
return hashCode;
}
@Override
public UserPausedDetails clone() {
try {
return (UserPausedDetails) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.macie2.model.transform.UserPausedDetailsMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}