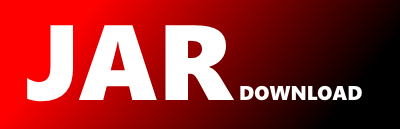
com.amazonaws.services.mailmanager.AWSMailManagerAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-mailmanager Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.mailmanager;
import javax.annotation.Generated;
import com.amazonaws.services.mailmanager.model.*;
/**
* Interface for accessing MailManager asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.mailmanager.AbstractAWSMailManagerAsync} instead.
*
*
*
* AWS SES Mail Manager API
*
* AWS SES Mail Manager API contains operations and data types that comprise the
* Mail Manager feature of Amazon Simple Email Service.
*
*
* Mail Manager is a set of Amazon SES email gateway features designed to help you strengthen your organization's email
* infrastructure, simplify email workflow management, and streamline email compliance control. To learn more, see the
* Mail Manager chapter in the Amazon SES Developer
* Guide.
*
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSMailManagerAsync extends AWSMailManager {
/**
*
* Creates an Add On instance for the subscription indicated in the request. The resulting Amazon Resource Name
* (ARN) can be used in a conditional statement for a rule set or traffic policy.
*
*
* @param createAddonInstanceRequest
* @return A Java Future containing the result of the CreateAddonInstance operation returned by the service.
* @sample AWSMailManagerAsync.CreateAddonInstance
* @see AWS API Documentation
*/
java.util.concurrent.Future createAddonInstanceAsync(CreateAddonInstanceRequest createAddonInstanceRequest);
/**
*
* Creates an Add On instance for the subscription indicated in the request. The resulting Amazon Resource Name
* (ARN) can be used in a conditional statement for a rule set or traffic policy.
*
*
* @param createAddonInstanceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateAddonInstance operation returned by the service.
* @sample AWSMailManagerAsyncHandler.CreateAddonInstance
* @see AWS API Documentation
*/
java.util.concurrent.Future createAddonInstanceAsync(CreateAddonInstanceRequest createAddonInstanceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a subscription for an Add On representing the acceptance of its terms of use and additional pricing. The
* subscription can then be used to create an instance for use in rule sets or traffic policies.
*
*
* @param createAddonSubscriptionRequest
* @return A Java Future containing the result of the CreateAddonSubscription operation returned by the service.
* @sample AWSMailManagerAsync.CreateAddonSubscription
* @see AWS API Documentation
*/
java.util.concurrent.Future createAddonSubscriptionAsync(CreateAddonSubscriptionRequest createAddonSubscriptionRequest);
/**
*
* Creates a subscription for an Add On representing the acceptance of its terms of use and additional pricing. The
* subscription can then be used to create an instance for use in rule sets or traffic policies.
*
*
* @param createAddonSubscriptionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateAddonSubscription operation returned by the service.
* @sample AWSMailManagerAsyncHandler.CreateAddonSubscription
* @see AWS API Documentation
*/
java.util.concurrent.Future createAddonSubscriptionAsync(CreateAddonSubscriptionRequest createAddonSubscriptionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new email archive resource for storing and retaining emails.
*
*
* @param createArchiveRequest
* The request to create a new email archive.
* @return A Java Future containing the result of the CreateArchive operation returned by the service.
* @sample AWSMailManagerAsync.CreateArchive
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createArchiveAsync(CreateArchiveRequest createArchiveRequest);
/**
*
* Creates a new email archive resource for storing and retaining emails.
*
*
* @param createArchiveRequest
* The request to create a new email archive.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateArchive operation returned by the service.
* @sample AWSMailManagerAsyncHandler.CreateArchive
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createArchiveAsync(CreateArchiveRequest createArchiveRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provision a new ingress endpoint resource.
*
*
* @param createIngressPointRequest
* @return A Java Future containing the result of the CreateIngressPoint operation returned by the service.
* @sample AWSMailManagerAsync.CreateIngressPoint
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createIngressPointAsync(CreateIngressPointRequest createIngressPointRequest);
/**
*
* Provision a new ingress endpoint resource.
*
*
* @param createIngressPointRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateIngressPoint operation returned by the service.
* @sample AWSMailManagerAsyncHandler.CreateIngressPoint
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createIngressPointAsync(CreateIngressPointRequest createIngressPointRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a relay resource which can be used in rules to relay incoming emails to defined relay destinations.
*
*
* @param createRelayRequest
* @return A Java Future containing the result of the CreateRelay operation returned by the service.
* @sample AWSMailManagerAsync.CreateRelay
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createRelayAsync(CreateRelayRequest createRelayRequest);
/**
*
* Creates a relay resource which can be used in rules to relay incoming emails to defined relay destinations.
*
*
* @param createRelayRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateRelay operation returned by the service.
* @sample AWSMailManagerAsyncHandler.CreateRelay
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createRelayAsync(CreateRelayRequest createRelayRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provision a new rule set.
*
*
* @param createRuleSetRequest
* @return A Java Future containing the result of the CreateRuleSet operation returned by the service.
* @sample AWSMailManagerAsync.CreateRuleSet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createRuleSetAsync(CreateRuleSetRequest createRuleSetRequest);
/**
*
* Provision a new rule set.
*
*
* @param createRuleSetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateRuleSet operation returned by the service.
* @sample AWSMailManagerAsyncHandler.CreateRuleSet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createRuleSetAsync(CreateRuleSetRequest createRuleSetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provision a new traffic policy resource.
*
*
* @param createTrafficPolicyRequest
* @return A Java Future containing the result of the CreateTrafficPolicy operation returned by the service.
* @sample AWSMailManagerAsync.CreateTrafficPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future createTrafficPolicyAsync(CreateTrafficPolicyRequest createTrafficPolicyRequest);
/**
*
* Provision a new traffic policy resource.
*
*
* @param createTrafficPolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateTrafficPolicy operation returned by the service.
* @sample AWSMailManagerAsyncHandler.CreateTrafficPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future createTrafficPolicyAsync(CreateTrafficPolicyRequest createTrafficPolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an Add On instance.
*
*
* @param deleteAddonInstanceRequest
* @return A Java Future containing the result of the DeleteAddonInstance operation returned by the service.
* @sample AWSMailManagerAsync.DeleteAddonInstance
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteAddonInstanceAsync(DeleteAddonInstanceRequest deleteAddonInstanceRequest);
/**
*
* Deletes an Add On instance.
*
*
* @param deleteAddonInstanceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteAddonInstance operation returned by the service.
* @sample AWSMailManagerAsyncHandler.DeleteAddonInstance
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteAddonInstanceAsync(DeleteAddonInstanceRequest deleteAddonInstanceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an Add On subscription.
*
*
* @param deleteAddonSubscriptionRequest
* @return A Java Future containing the result of the DeleteAddonSubscription operation returned by the service.
* @sample AWSMailManagerAsync.DeleteAddonSubscription
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteAddonSubscriptionAsync(DeleteAddonSubscriptionRequest deleteAddonSubscriptionRequest);
/**
*
* Deletes an Add On subscription.
*
*
* @param deleteAddonSubscriptionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteAddonSubscription operation returned by the service.
* @sample AWSMailManagerAsyncHandler.DeleteAddonSubscription
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteAddonSubscriptionAsync(DeleteAddonSubscriptionRequest deleteAddonSubscriptionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Initiates deletion of an email archive. This changes the archive state to pending deletion. In this state, no new
* emails can be added, and existing archived emails become inaccessible (search, export, download). The archive and
* all of its contents will be permanently deleted 30 days after entering the pending deletion state, regardless of
* the configured retention period.
*
*
* @param deleteArchiveRequest
* The request to initiate deletion of an email archive.
* @return A Java Future containing the result of the DeleteArchive operation returned by the service.
* @sample AWSMailManagerAsync.DeleteArchive
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteArchiveAsync(DeleteArchiveRequest deleteArchiveRequest);
/**
*
* Initiates deletion of an email archive. This changes the archive state to pending deletion. In this state, no new
* emails can be added, and existing archived emails become inaccessible (search, export, download). The archive and
* all of its contents will be permanently deleted 30 days after entering the pending deletion state, regardless of
* the configured retention period.
*
*
* @param deleteArchiveRequest
* The request to initiate deletion of an email archive.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteArchive operation returned by the service.
* @sample AWSMailManagerAsyncHandler.DeleteArchive
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteArchiveAsync(DeleteArchiveRequest deleteArchiveRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Delete an ingress endpoint resource.
*
*
* @param deleteIngressPointRequest
* @return A Java Future containing the result of the DeleteIngressPoint operation returned by the service.
* @sample AWSMailManagerAsync.DeleteIngressPoint
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteIngressPointAsync(DeleteIngressPointRequest deleteIngressPointRequest);
/**
*
* Delete an ingress endpoint resource.
*
*
* @param deleteIngressPointRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteIngressPoint operation returned by the service.
* @sample AWSMailManagerAsyncHandler.DeleteIngressPoint
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteIngressPointAsync(DeleteIngressPointRequest deleteIngressPointRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an existing relay resource.
*
*
* @param deleteRelayRequest
* @return A Java Future containing the result of the DeleteRelay operation returned by the service.
* @sample AWSMailManagerAsync.DeleteRelay
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteRelayAsync(DeleteRelayRequest deleteRelayRequest);
/**
*
* Deletes an existing relay resource.
*
*
* @param deleteRelayRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteRelay operation returned by the service.
* @sample AWSMailManagerAsyncHandler.DeleteRelay
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteRelayAsync(DeleteRelayRequest deleteRelayRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Delete a rule set.
*
*
* @param deleteRuleSetRequest
* @return A Java Future containing the result of the DeleteRuleSet operation returned by the service.
* @sample AWSMailManagerAsync.DeleteRuleSet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteRuleSetAsync(DeleteRuleSetRequest deleteRuleSetRequest);
/**
*
* Delete a rule set.
*
*
* @param deleteRuleSetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteRuleSet operation returned by the service.
* @sample AWSMailManagerAsyncHandler.DeleteRuleSet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteRuleSetAsync(DeleteRuleSetRequest deleteRuleSetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Delete a traffic policy resource.
*
*
* @param deleteTrafficPolicyRequest
* @return A Java Future containing the result of the DeleteTrafficPolicy operation returned by the service.
* @sample AWSMailManagerAsync.DeleteTrafficPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteTrafficPolicyAsync(DeleteTrafficPolicyRequest deleteTrafficPolicyRequest);
/**
*
* Delete a traffic policy resource.
*
*
* @param deleteTrafficPolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteTrafficPolicy operation returned by the service.
* @sample AWSMailManagerAsyncHandler.DeleteTrafficPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteTrafficPolicyAsync(DeleteTrafficPolicyRequest deleteTrafficPolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets detailed information about an Add On instance.
*
*
* @param getAddonInstanceRequest
* @return A Java Future containing the result of the GetAddonInstance operation returned by the service.
* @sample AWSMailManagerAsync.GetAddonInstance
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getAddonInstanceAsync(GetAddonInstanceRequest getAddonInstanceRequest);
/**
*
* Gets detailed information about an Add On instance.
*
*
* @param getAddonInstanceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetAddonInstance operation returned by the service.
* @sample AWSMailManagerAsyncHandler.GetAddonInstance
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getAddonInstanceAsync(GetAddonInstanceRequest getAddonInstanceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets detailed information about an Add On subscription.
*
*
* @param getAddonSubscriptionRequest
* @return A Java Future containing the result of the GetAddonSubscription operation returned by the service.
* @sample AWSMailManagerAsync.GetAddonSubscription
* @see AWS API Documentation
*/
java.util.concurrent.Future getAddonSubscriptionAsync(GetAddonSubscriptionRequest getAddonSubscriptionRequest);
/**
*
* Gets detailed information about an Add On subscription.
*
*
* @param getAddonSubscriptionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetAddonSubscription operation returned by the service.
* @sample AWSMailManagerAsyncHandler.GetAddonSubscription
* @see AWS API Documentation
*/
java.util.concurrent.Future getAddonSubscriptionAsync(GetAddonSubscriptionRequest getAddonSubscriptionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the full details and current state of a specified email archive.
*
*
* @param getArchiveRequest
* The request to retrieve details of an email archive.
* @return A Java Future containing the result of the GetArchive operation returned by the service.
* @sample AWSMailManagerAsync.GetArchive
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getArchiveAsync(GetArchiveRequest getArchiveRequest);
/**
*
* Retrieves the full details and current state of a specified email archive.
*
*
* @param getArchiveRequest
* The request to retrieve details of an email archive.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetArchive operation returned by the service.
* @sample AWSMailManagerAsyncHandler.GetArchive
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getArchiveAsync(GetArchiveRequest getArchiveRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the details and current status of a specific email archive export job.
*
*
* @param getArchiveExportRequest
* The request to retrieve details of a specific archive export job.
* @return A Java Future containing the result of the GetArchiveExport operation returned by the service.
* @sample AWSMailManagerAsync.GetArchiveExport
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getArchiveExportAsync(GetArchiveExportRequest getArchiveExportRequest);
/**
*
* Retrieves the details and current status of a specific email archive export job.
*
*
* @param getArchiveExportRequest
* The request to retrieve details of a specific archive export job.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetArchiveExport operation returned by the service.
* @sample AWSMailManagerAsyncHandler.GetArchiveExport
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getArchiveExportAsync(GetArchiveExportRequest getArchiveExportRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a pre-signed URL that provides temporary download access to the specific email message stored in the
* archive.
*
*
* @param getArchiveMessageRequest
* The request to get details of a specific email message stored in an archive.
* @return A Java Future containing the result of the GetArchiveMessage operation returned by the service.
* @sample AWSMailManagerAsync.GetArchiveMessage
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getArchiveMessageAsync(GetArchiveMessageRequest getArchiveMessageRequest);
/**
*
* Returns a pre-signed URL that provides temporary download access to the specific email message stored in the
* archive.
*
*
* @param getArchiveMessageRequest
* The request to get details of a specific email message stored in an archive.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetArchiveMessage operation returned by the service.
* @sample AWSMailManagerAsyncHandler.GetArchiveMessage
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getArchiveMessageAsync(GetArchiveMessageRequest getArchiveMessageRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the textual content of a specific email message stored in the archive. Attachments are not included.
*
*
* @param getArchiveMessageContentRequest
* The request to get the textual content of a specific email message stored in an archive.
* @return A Java Future containing the result of the GetArchiveMessageContent operation returned by the service.
* @sample AWSMailManagerAsync.GetArchiveMessageContent
* @see AWS API Documentation
*/
java.util.concurrent.Future getArchiveMessageContentAsync(GetArchiveMessageContentRequest getArchiveMessageContentRequest);
/**
*
* Returns the textual content of a specific email message stored in the archive. Attachments are not included.
*
*
* @param getArchiveMessageContentRequest
* The request to get the textual content of a specific email message stored in an archive.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetArchiveMessageContent operation returned by the service.
* @sample AWSMailManagerAsyncHandler.GetArchiveMessageContent
* @see AWS API Documentation
*/
java.util.concurrent.Future getArchiveMessageContentAsync(GetArchiveMessageContentRequest getArchiveMessageContentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the details and current status of a specific email archive search job.
*
*
* @param getArchiveSearchRequest
* The request to retrieve details of a specific archive search job.
* @return A Java Future containing the result of the GetArchiveSearch operation returned by the service.
* @sample AWSMailManagerAsync.GetArchiveSearch
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getArchiveSearchAsync(GetArchiveSearchRequest getArchiveSearchRequest);
/**
*
* Retrieves the details and current status of a specific email archive search job.
*
*
* @param getArchiveSearchRequest
* The request to retrieve details of a specific archive search job.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetArchiveSearch operation returned by the service.
* @sample AWSMailManagerAsyncHandler.GetArchiveSearch
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getArchiveSearchAsync(GetArchiveSearchRequest getArchiveSearchRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the results of a completed email archive search job.
*
*
* @param getArchiveSearchResultsRequest
* The request to retrieve results from a completed archive search job.
* @return A Java Future containing the result of the GetArchiveSearchResults operation returned by the service.
* @sample AWSMailManagerAsync.GetArchiveSearchResults
* @see AWS API Documentation
*/
java.util.concurrent.Future getArchiveSearchResultsAsync(GetArchiveSearchResultsRequest getArchiveSearchResultsRequest);
/**
*
* Returns the results of a completed email archive search job.
*
*
* @param getArchiveSearchResultsRequest
* The request to retrieve results from a completed archive search job.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetArchiveSearchResults operation returned by the service.
* @sample AWSMailManagerAsyncHandler.GetArchiveSearchResults
* @see AWS API Documentation
*/
java.util.concurrent.Future getArchiveSearchResultsAsync(GetArchiveSearchResultsRequest getArchiveSearchResultsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Fetch ingress endpoint resource attributes.
*
*
* @param getIngressPointRequest
* @return A Java Future containing the result of the GetIngressPoint operation returned by the service.
* @sample AWSMailManagerAsync.GetIngressPoint
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getIngressPointAsync(GetIngressPointRequest getIngressPointRequest);
/**
*
* Fetch ingress endpoint resource attributes.
*
*
* @param getIngressPointRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetIngressPoint operation returned by the service.
* @sample AWSMailManagerAsyncHandler.GetIngressPoint
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getIngressPointAsync(GetIngressPointRequest getIngressPointRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Fetch the relay resource and it's attributes.
*
*
* @param getRelayRequest
* @return A Java Future containing the result of the GetRelay operation returned by the service.
* @sample AWSMailManagerAsync.GetRelay
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getRelayAsync(GetRelayRequest getRelayRequest);
/**
*
* Fetch the relay resource and it's attributes.
*
*
* @param getRelayRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetRelay operation returned by the service.
* @sample AWSMailManagerAsyncHandler.GetRelay
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getRelayAsync(GetRelayRequest getRelayRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Fetch attributes of a rule set.
*
*
* @param getRuleSetRequest
* @return A Java Future containing the result of the GetRuleSet operation returned by the service.
* @sample AWSMailManagerAsync.GetRuleSet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getRuleSetAsync(GetRuleSetRequest getRuleSetRequest);
/**
*
* Fetch attributes of a rule set.
*
*
* @param getRuleSetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetRuleSet operation returned by the service.
* @sample AWSMailManagerAsyncHandler.GetRuleSet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getRuleSetAsync(GetRuleSetRequest getRuleSetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Fetch attributes of a traffic policy resource.
*
*
* @param getTrafficPolicyRequest
* @return A Java Future containing the result of the GetTrafficPolicy operation returned by the service.
* @sample AWSMailManagerAsync.GetTrafficPolicy
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getTrafficPolicyAsync(GetTrafficPolicyRequest getTrafficPolicyRequest);
/**
*
* Fetch attributes of a traffic policy resource.
*
*
* @param getTrafficPolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetTrafficPolicy operation returned by the service.
* @sample AWSMailManagerAsyncHandler.GetTrafficPolicy
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getTrafficPolicyAsync(GetTrafficPolicyRequest getTrafficPolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all Add On instances in your account.
*
*
* @param listAddonInstancesRequest
* @return A Java Future containing the result of the ListAddonInstances operation returned by the service.
* @sample AWSMailManagerAsync.ListAddonInstances
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listAddonInstancesAsync(ListAddonInstancesRequest listAddonInstancesRequest);
/**
*
* Lists all Add On instances in your account.
*
*
* @param listAddonInstancesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListAddonInstances operation returned by the service.
* @sample AWSMailManagerAsyncHandler.ListAddonInstances
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listAddonInstancesAsync(ListAddonInstancesRequest listAddonInstancesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all Add On subscriptions in your account.
*
*
* @param listAddonSubscriptionsRequest
* @return A Java Future containing the result of the ListAddonSubscriptions operation returned by the service.
* @sample AWSMailManagerAsync.ListAddonSubscriptions
* @see AWS API Documentation
*/
java.util.concurrent.Future listAddonSubscriptionsAsync(ListAddonSubscriptionsRequest listAddonSubscriptionsRequest);
/**
*
* Lists all Add On subscriptions in your account.
*
*
* @param listAddonSubscriptionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListAddonSubscriptions operation returned by the service.
* @sample AWSMailManagerAsyncHandler.ListAddonSubscriptions
* @see AWS API Documentation
*/
java.util.concurrent.Future listAddonSubscriptionsAsync(ListAddonSubscriptionsRequest listAddonSubscriptionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of email archive export jobs.
*
*
* @param listArchiveExportsRequest
* The request to list archive export jobs in your account.
* @return A Java Future containing the result of the ListArchiveExports operation returned by the service.
* @sample AWSMailManagerAsync.ListArchiveExports
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listArchiveExportsAsync(ListArchiveExportsRequest listArchiveExportsRequest);
/**
*
* Returns a list of email archive export jobs.
*
*
* @param listArchiveExportsRequest
* The request to list archive export jobs in your account.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListArchiveExports operation returned by the service.
* @sample AWSMailManagerAsyncHandler.ListArchiveExports
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listArchiveExportsAsync(ListArchiveExportsRequest listArchiveExportsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of email archive search jobs.
*
*
* @param listArchiveSearchesRequest
* The request to list archive search jobs in your account.
* @return A Java Future containing the result of the ListArchiveSearches operation returned by the service.
* @sample AWSMailManagerAsync.ListArchiveSearches
* @see AWS API Documentation
*/
java.util.concurrent.Future listArchiveSearchesAsync(ListArchiveSearchesRequest listArchiveSearchesRequest);
/**
*
* Returns a list of email archive search jobs.
*
*
* @param listArchiveSearchesRequest
* The request to list archive search jobs in your account.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListArchiveSearches operation returned by the service.
* @sample AWSMailManagerAsyncHandler.ListArchiveSearches
* @see AWS API Documentation
*/
java.util.concurrent.Future listArchiveSearchesAsync(ListArchiveSearchesRequest listArchiveSearchesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of all email archives in your account.
*
*
* @param listArchivesRequest
* The request to list email archives in your account.
* @return A Java Future containing the result of the ListArchives operation returned by the service.
* @sample AWSMailManagerAsync.ListArchives
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listArchivesAsync(ListArchivesRequest listArchivesRequest);
/**
*
* Returns a list of all email archives in your account.
*
*
* @param listArchivesRequest
* The request to list email archives in your account.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListArchives operation returned by the service.
* @sample AWSMailManagerAsyncHandler.ListArchives
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listArchivesAsync(ListArchivesRequest listArchivesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* List all ingress endpoint resources.
*
*
* @param listIngressPointsRequest
* @return A Java Future containing the result of the ListIngressPoints operation returned by the service.
* @sample AWSMailManagerAsync.ListIngressPoints
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listIngressPointsAsync(ListIngressPointsRequest listIngressPointsRequest);
/**
*
* List all ingress endpoint resources.
*
*
* @param listIngressPointsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListIngressPoints operation returned by the service.
* @sample AWSMailManagerAsyncHandler.ListIngressPoints
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listIngressPointsAsync(ListIngressPointsRequest listIngressPointsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all the existing relay resources.
*
*
* @param listRelaysRequest
* @return A Java Future containing the result of the ListRelays operation returned by the service.
* @sample AWSMailManagerAsync.ListRelays
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listRelaysAsync(ListRelaysRequest listRelaysRequest);
/**
*
* Lists all the existing relay resources.
*
*
* @param listRelaysRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListRelays operation returned by the service.
* @sample AWSMailManagerAsyncHandler.ListRelays
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listRelaysAsync(ListRelaysRequest listRelaysRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* List rule sets for this account.
*
*
* @param listRuleSetsRequest
* @return A Java Future containing the result of the ListRuleSets operation returned by the service.
* @sample AWSMailManagerAsync.ListRuleSets
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listRuleSetsAsync(ListRuleSetsRequest listRuleSetsRequest);
/**
*
* List rule sets for this account.
*
*
* @param listRuleSetsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListRuleSets operation returned by the service.
* @sample AWSMailManagerAsyncHandler.ListRuleSets
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listRuleSetsAsync(ListRuleSetsRequest listRuleSetsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the list of tags (keys and values) assigned to the resource.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSMailManagerAsync.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Retrieves the list of tags (keys and values) assigned to the resource.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSMailManagerAsyncHandler.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* List traffic policy resources.
*
*
* @param listTrafficPoliciesRequest
* @return A Java Future containing the result of the ListTrafficPolicies operation returned by the service.
* @sample AWSMailManagerAsync.ListTrafficPolicies
* @see AWS API Documentation
*/
java.util.concurrent.Future listTrafficPoliciesAsync(ListTrafficPoliciesRequest listTrafficPoliciesRequest);
/**
*
* List traffic policy resources.
*
*
* @param listTrafficPoliciesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTrafficPolicies operation returned by the service.
* @sample AWSMailManagerAsyncHandler.ListTrafficPolicies
* @see AWS API Documentation
*/
java.util.concurrent.Future listTrafficPoliciesAsync(ListTrafficPoliciesRequest listTrafficPoliciesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Initiates an export of emails from the specified archive.
*
*
* @param startArchiveExportRequest
* The request to initiate an export of emails from an archive.
* @return A Java Future containing the result of the StartArchiveExport operation returned by the service.
* @sample AWSMailManagerAsync.StartArchiveExport
* @see AWS
* API Documentation
*/
java.util.concurrent.Future startArchiveExportAsync(StartArchiveExportRequest startArchiveExportRequest);
/**
*
* Initiates an export of emails from the specified archive.
*
*
* @param startArchiveExportRequest
* The request to initiate an export of emails from an archive.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StartArchiveExport operation returned by the service.
* @sample AWSMailManagerAsyncHandler.StartArchiveExport
* @see AWS
* API Documentation
*/
java.util.concurrent.Future startArchiveExportAsync(StartArchiveExportRequest startArchiveExportRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Initiates a search across emails in the specified archive.
*
*
* @param startArchiveSearchRequest
* The request to initiate a search across emails in an archive.
* @return A Java Future containing the result of the StartArchiveSearch operation returned by the service.
* @sample AWSMailManagerAsync.StartArchiveSearch
* @see AWS
* API Documentation
*/
java.util.concurrent.Future startArchiveSearchAsync(StartArchiveSearchRequest startArchiveSearchRequest);
/**
*
* Initiates a search across emails in the specified archive.
*
*
* @param startArchiveSearchRequest
* The request to initiate a search across emails in an archive.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StartArchiveSearch operation returned by the service.
* @sample AWSMailManagerAsyncHandler.StartArchiveSearch
* @see AWS
* API Documentation
*/
java.util.concurrent.Future startArchiveSearchAsync(StartArchiveSearchRequest startArchiveSearchRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Stops an in-progress export of emails from an archive.
*
*
* @param stopArchiveExportRequest
* The request to stop an in-progress archive export job.
* @return A Java Future containing the result of the StopArchiveExport operation returned by the service.
* @sample AWSMailManagerAsync.StopArchiveExport
* @see AWS
* API Documentation
*/
java.util.concurrent.Future stopArchiveExportAsync(StopArchiveExportRequest stopArchiveExportRequest);
/**
*
* Stops an in-progress export of emails from an archive.
*
*
* @param stopArchiveExportRequest
* The request to stop an in-progress archive export job.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StopArchiveExport operation returned by the service.
* @sample AWSMailManagerAsyncHandler.StopArchiveExport
* @see AWS
* API Documentation
*/
java.util.concurrent.Future stopArchiveExportAsync(StopArchiveExportRequest stopArchiveExportRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Stops an in-progress archive search job.
*
*
* @param stopArchiveSearchRequest
* The request to stop an in-progress archive search job.
* @return A Java Future containing the result of the StopArchiveSearch operation returned by the service.
* @sample AWSMailManagerAsync.StopArchiveSearch
* @see AWS
* API Documentation
*/
java.util.concurrent.Future stopArchiveSearchAsync(StopArchiveSearchRequest stopArchiveSearchRequest);
/**
*
* Stops an in-progress archive search job.
*
*
* @param stopArchiveSearchRequest
* The request to stop an in-progress archive search job.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StopArchiveSearch operation returned by the service.
* @sample AWSMailManagerAsyncHandler.StopArchiveSearch
* @see AWS
* API Documentation
*/
java.util.concurrent.Future stopArchiveSearchAsync(StopArchiveSearchRequest stopArchiveSearchRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds one or more tags (keys and values) to a specified resource.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSMailManagerAsync.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* Adds one or more tags (keys and values) to a specified resource.
*
*
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSMailManagerAsyncHandler.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Remove one or more tags (keys and values) from a specified resource.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSMailManagerAsync.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* Remove one or more tags (keys and values) from a specified resource.
*
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSMailManagerAsyncHandler.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the attributes of an existing email archive.
*
*
* @param updateArchiveRequest
* The request to update properties of an existing email archive.
* @return A Java Future containing the result of the UpdateArchive operation returned by the service.
* @sample AWSMailManagerAsync.UpdateArchive
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateArchiveAsync(UpdateArchiveRequest updateArchiveRequest);
/**
*
* Updates the attributes of an existing email archive.
*
*
* @param updateArchiveRequest
* The request to update properties of an existing email archive.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateArchive operation returned by the service.
* @sample AWSMailManagerAsyncHandler.UpdateArchive
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateArchiveAsync(UpdateArchiveRequest updateArchiveRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Update attributes of a provisioned ingress endpoint resource.
*
*
* @param updateIngressPointRequest
* @return A Java Future containing the result of the UpdateIngressPoint operation returned by the service.
* @sample AWSMailManagerAsync.UpdateIngressPoint
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateIngressPointAsync(UpdateIngressPointRequest updateIngressPointRequest);
/**
*
* Update attributes of a provisioned ingress endpoint resource.
*
*
* @param updateIngressPointRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateIngressPoint operation returned by the service.
* @sample AWSMailManagerAsyncHandler.UpdateIngressPoint
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateIngressPointAsync(UpdateIngressPointRequest updateIngressPointRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the attributes of an existing relay resource.
*
*
* @param updateRelayRequest
* @return A Java Future containing the result of the UpdateRelay operation returned by the service.
* @sample AWSMailManagerAsync.UpdateRelay
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateRelayAsync(UpdateRelayRequest updateRelayRequest);
/**
*
* Updates the attributes of an existing relay resource.
*
*
* @param updateRelayRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateRelay operation returned by the service.
* @sample AWSMailManagerAsyncHandler.UpdateRelay
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateRelayAsync(UpdateRelayRequest updateRelayRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* >Update attributes of an already provisioned rule set.
*
*
* @param updateRuleSetRequest
* @return A Java Future containing the result of the UpdateRuleSet operation returned by the service.
* @sample AWSMailManagerAsync.UpdateRuleSet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateRuleSetAsync(UpdateRuleSetRequest updateRuleSetRequest);
/**
*
* >Update attributes of an already provisioned rule set.
*
*
* @param updateRuleSetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateRuleSet operation returned by the service.
* @sample AWSMailManagerAsyncHandler.UpdateRuleSet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateRuleSetAsync(UpdateRuleSetRequest updateRuleSetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Update attributes of an already provisioned traffic policy resource.
*
*
* @param updateTrafficPolicyRequest
* @return A Java Future containing the result of the UpdateTrafficPolicy operation returned by the service.
* @sample AWSMailManagerAsync.UpdateTrafficPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future updateTrafficPolicyAsync(UpdateTrafficPolicyRequest updateTrafficPolicyRequest);
/**
*
* Update attributes of an already provisioned traffic policy resource.
*
*
* @param updateTrafficPolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateTrafficPolicy operation returned by the service.
* @sample AWSMailManagerAsyncHandler.UpdateTrafficPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future updateTrafficPolicyAsync(UpdateTrafficPolicyRequest updateTrafficPolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}