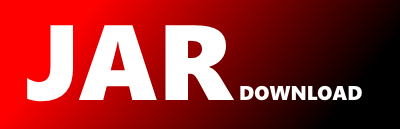
com.amazonaws.services.managedgrafana.AmazonManagedGrafanaAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-managedgrafana Show documentation
/*
* Copyright 2018-2023 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.managedgrafana;
import javax.annotation.Generated;
import com.amazonaws.services.managedgrafana.model.*;
/**
* Interface for accessing Amazon Managed Grafana asynchronously. Each asynchronous method will return a Java Future
* object representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.managedgrafana.AbstractAmazonManagedGrafanaAsync} instead.
*
*
*
* Amazon Managed Grafana is a fully managed and secure data visualization service that you can use to instantly query,
* correlate, and visualize operational metrics, logs, and traces from multiple sources. Amazon Managed Grafana makes it
* easy to deploy, operate, and scale Grafana, a widely deployed data visualization tool that is popular for its
* extensible data support.
*
*
* With Amazon Managed Grafana, you create logically isolated Grafana servers called workspaces. In a workspace,
* you can create Grafana dashboards and visualizations to analyze your metrics, logs, and traces without having to
* build, package, or deploy any hardware to run Grafana servers.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonManagedGrafanaAsync extends AmazonManagedGrafana {
/**
*
* Assigns a Grafana Enterprise license to a workspace. Upgrading to Grafana Enterprise incurs additional fees. For
* more information, see Upgrade a
* workspace to Grafana Enterprise.
*
*
* @param associateLicenseRequest
* @return A Java Future containing the result of the AssociateLicense operation returned by the service.
* @sample AmazonManagedGrafanaAsync.AssociateLicense
* @see AWS API
* Documentation
*/
java.util.concurrent.Future associateLicenseAsync(AssociateLicenseRequest associateLicenseRequest);
/**
*
* Assigns a Grafana Enterprise license to a workspace. Upgrading to Grafana Enterprise incurs additional fees. For
* more information, see Upgrade a
* workspace to Grafana Enterprise.
*
*
* @param associateLicenseRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateLicense operation returned by the service.
* @sample AmazonManagedGrafanaAsyncHandler.AssociateLicense
* @see AWS API
* Documentation
*/
java.util.concurrent.Future associateLicenseAsync(AssociateLicenseRequest associateLicenseRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a workspace. In a workspace, you can create Grafana dashboards and visualizations to analyze your
* metrics, logs, and traces. You don't have to build, package, or deploy any hardware to run the Grafana server.
*
*
* Don't use CreateWorkspace
to modify an existing workspace. Instead, use UpdateWorkspace.
*
*
* @param createWorkspaceRequest
* @return A Java Future containing the result of the CreateWorkspace operation returned by the service.
* @sample AmazonManagedGrafanaAsync.CreateWorkspace
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createWorkspaceAsync(CreateWorkspaceRequest createWorkspaceRequest);
/**
*
* Creates a workspace. In a workspace, you can create Grafana dashboards and visualizations to analyze your
* metrics, logs, and traces. You don't have to build, package, or deploy any hardware to run the Grafana server.
*
*
* Don't use CreateWorkspace
to modify an existing workspace. Instead, use UpdateWorkspace.
*
*
* @param createWorkspaceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateWorkspace operation returned by the service.
* @sample AmazonManagedGrafanaAsyncHandler.CreateWorkspace
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createWorkspaceAsync(CreateWorkspaceRequest createWorkspaceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a Grafana API key for the workspace. This key can be used to authenticate requests sent to the
* workspace's HTTP API. See https
* ://docs.aws.amazon.com/grafana/latest/userguide/Using-Grafana-APIs.html for available APIs and example
* requests.
*
*
* @param createWorkspaceApiKeyRequest
* @return A Java Future containing the result of the CreateWorkspaceApiKey operation returned by the service.
* @sample AmazonManagedGrafanaAsync.CreateWorkspaceApiKey
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createWorkspaceApiKeyAsync(CreateWorkspaceApiKeyRequest createWorkspaceApiKeyRequest);
/**
*
* Creates a Grafana API key for the workspace. This key can be used to authenticate requests sent to the
* workspace's HTTP API. See https
* ://docs.aws.amazon.com/grafana/latest/userguide/Using-Grafana-APIs.html for available APIs and example
* requests.
*
*
* @param createWorkspaceApiKeyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateWorkspaceApiKey operation returned by the service.
* @sample AmazonManagedGrafanaAsyncHandler.CreateWorkspaceApiKey
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createWorkspaceApiKeyAsync(CreateWorkspaceApiKeyRequest createWorkspaceApiKeyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an Amazon Managed Grafana workspace.
*
*
* @param deleteWorkspaceRequest
* @return A Java Future containing the result of the DeleteWorkspace operation returned by the service.
* @sample AmazonManagedGrafanaAsync.DeleteWorkspace
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteWorkspaceAsync(DeleteWorkspaceRequest deleteWorkspaceRequest);
/**
*
* Deletes an Amazon Managed Grafana workspace.
*
*
* @param deleteWorkspaceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteWorkspace operation returned by the service.
* @sample AmazonManagedGrafanaAsyncHandler.DeleteWorkspace
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteWorkspaceAsync(DeleteWorkspaceRequest deleteWorkspaceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a Grafana API key for the workspace.
*
*
* @param deleteWorkspaceApiKeyRequest
* @return A Java Future containing the result of the DeleteWorkspaceApiKey operation returned by the service.
* @sample AmazonManagedGrafanaAsync.DeleteWorkspaceApiKey
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteWorkspaceApiKeyAsync(DeleteWorkspaceApiKeyRequest deleteWorkspaceApiKeyRequest);
/**
*
* Deletes a Grafana API key for the workspace.
*
*
* @param deleteWorkspaceApiKeyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteWorkspaceApiKey operation returned by the service.
* @sample AmazonManagedGrafanaAsyncHandler.DeleteWorkspaceApiKey
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteWorkspaceApiKeyAsync(DeleteWorkspaceApiKeyRequest deleteWorkspaceApiKeyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Displays information about one Amazon Managed Grafana workspace.
*
*
* @param describeWorkspaceRequest
* @return A Java Future containing the result of the DescribeWorkspace operation returned by the service.
* @sample AmazonManagedGrafanaAsync.DescribeWorkspace
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeWorkspaceAsync(DescribeWorkspaceRequest describeWorkspaceRequest);
/**
*
* Displays information about one Amazon Managed Grafana workspace.
*
*
* @param describeWorkspaceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeWorkspace operation returned by the service.
* @sample AmazonManagedGrafanaAsyncHandler.DescribeWorkspace
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeWorkspaceAsync(DescribeWorkspaceRequest describeWorkspaceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Displays information about the authentication methods used in one Amazon Managed Grafana workspace.
*
*
* @param describeWorkspaceAuthenticationRequest
* @return A Java Future containing the result of the DescribeWorkspaceAuthentication operation returned by the
* service.
* @sample AmazonManagedGrafanaAsync.DescribeWorkspaceAuthentication
* @see AWS API Documentation
*/
java.util.concurrent.Future describeWorkspaceAuthenticationAsync(
DescribeWorkspaceAuthenticationRequest describeWorkspaceAuthenticationRequest);
/**
*
* Displays information about the authentication methods used in one Amazon Managed Grafana workspace.
*
*
* @param describeWorkspaceAuthenticationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeWorkspaceAuthentication operation returned by the
* service.
* @sample AmazonManagedGrafanaAsyncHandler.DescribeWorkspaceAuthentication
* @see AWS API Documentation
*/
java.util.concurrent.Future describeWorkspaceAuthenticationAsync(
DescribeWorkspaceAuthenticationRequest describeWorkspaceAuthenticationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets the current configuration string for the given workspace.
*
*
* @param describeWorkspaceConfigurationRequest
* @return A Java Future containing the result of the DescribeWorkspaceConfiguration operation returned by the
* service.
* @sample AmazonManagedGrafanaAsync.DescribeWorkspaceConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future describeWorkspaceConfigurationAsync(
DescribeWorkspaceConfigurationRequest describeWorkspaceConfigurationRequest);
/**
*
* Gets the current configuration string for the given workspace.
*
*
* @param describeWorkspaceConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeWorkspaceConfiguration operation returned by the
* service.
* @sample AmazonManagedGrafanaAsyncHandler.DescribeWorkspaceConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future describeWorkspaceConfigurationAsync(
DescribeWorkspaceConfigurationRequest describeWorkspaceConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes the Grafana Enterprise license from a workspace.
*
*
* @param disassociateLicenseRequest
* @return A Java Future containing the result of the DisassociateLicense operation returned by the service.
* @sample AmazonManagedGrafanaAsync.DisassociateLicense
* @see AWS
* API Documentation
*/
java.util.concurrent.Future disassociateLicenseAsync(DisassociateLicenseRequest disassociateLicenseRequest);
/**
*
* Removes the Grafana Enterprise license from a workspace.
*
*
* @param disassociateLicenseRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisassociateLicense operation returned by the service.
* @sample AmazonManagedGrafanaAsyncHandler.DisassociateLicense
* @see AWS
* API Documentation
*/
java.util.concurrent.Future disassociateLicenseAsync(DisassociateLicenseRequest disassociateLicenseRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the users and groups who have the Grafana Admin
and Editor
roles in this
* workspace. If you use this operation without specifying userId
or groupId
, the
* operation returns the roles of all users and groups. If you specify a userId
or a
* groupId
, only the roles for that user or group are returned. If you do this, you can specify only
* one userId
or one groupId
.
*
*
* @param listPermissionsRequest
* @return A Java Future containing the result of the ListPermissions operation returned by the service.
* @sample AmazonManagedGrafanaAsync.ListPermissions
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listPermissionsAsync(ListPermissionsRequest listPermissionsRequest);
/**
*
* Lists the users and groups who have the Grafana Admin
and Editor
roles in this
* workspace. If you use this operation without specifying userId
or groupId
, the
* operation returns the roles of all users and groups. If you specify a userId
or a
* groupId
, only the roles for that user or group are returned. If you do this, you can specify only
* one userId
or one groupId
.
*
*
* @param listPermissionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListPermissions operation returned by the service.
* @sample AmazonManagedGrafanaAsyncHandler.ListPermissions
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listPermissionsAsync(ListPermissionsRequest listPermissionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* The ListTagsForResource
operation returns the tags that are associated with the Amazon Managed
* Service for Grafana resource specified by the resourceArn
. Currently, the only resource that can be
* tagged is a workspace.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AmazonManagedGrafanaAsync.ListTagsForResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* The ListTagsForResource
operation returns the tags that are associated with the Amazon Managed
* Service for Grafana resource specified by the resourceArn
. Currently, the only resource that can be
* tagged is a workspace.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AmazonManagedGrafanaAsyncHandler.ListTagsForResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists available versions of Grafana. These are available when calling CreateWorkspace
. Optionally,
* include a workspace to list the versions to which it can be upgraded.
*
*
* @param listVersionsRequest
* @return A Java Future containing the result of the ListVersions operation returned by the service.
* @sample AmazonManagedGrafanaAsync.ListVersions
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listVersionsAsync(ListVersionsRequest listVersionsRequest);
/**
*
* Lists available versions of Grafana. These are available when calling CreateWorkspace
. Optionally,
* include a workspace to list the versions to which it can be upgraded.
*
*
* @param listVersionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListVersions operation returned by the service.
* @sample AmazonManagedGrafanaAsyncHandler.ListVersions
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listVersionsAsync(ListVersionsRequest listVersionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of Amazon Managed Grafana workspaces in the account, with some information about each workspace.
* For more complete information about one workspace, use DescribeWorkspace.
*
*
* @param listWorkspacesRequest
* @return A Java Future containing the result of the ListWorkspaces operation returned by the service.
* @sample AmazonManagedGrafanaAsync.ListWorkspaces
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listWorkspacesAsync(ListWorkspacesRequest listWorkspacesRequest);
/**
*
* Returns a list of Amazon Managed Grafana workspaces in the account, with some information about each workspace.
* For more complete information about one workspace, use DescribeWorkspace.
*
*
* @param listWorkspacesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListWorkspaces operation returned by the service.
* @sample AmazonManagedGrafanaAsyncHandler.ListWorkspaces
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listWorkspacesAsync(ListWorkspacesRequest listWorkspacesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* The TagResource
operation associates tags with an Amazon Managed Grafana resource. Currently, the
* only resource that can be tagged is workspaces.
*
*
* If you specify a new tag key for the resource, this tag is appended to the list of tags associated with the
* resource. If you specify a tag key that is already associated with the resource, the new tag value that you
* specify replaces the previous value for that tag.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AmazonManagedGrafanaAsync.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* The TagResource
operation associates tags with an Amazon Managed Grafana resource. Currently, the
* only resource that can be tagged is workspaces.
*
*
* If you specify a new tag key for the resource, this tag is appended to the list of tags associated with the
* resource. If you specify a tag key that is already associated with the resource, the new tag value that you
* specify replaces the previous value for that tag.
*
*
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AmazonManagedGrafanaAsyncHandler.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* The UntagResource
operation removes the association of the tag with the Amazon Managed Grafana
* resource.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AmazonManagedGrafanaAsync.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* The UntagResource
operation removes the association of the tag with the Amazon Managed Grafana
* resource.
*
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AmazonManagedGrafanaAsyncHandler.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates which users in a workspace have the Grafana Admin
or Editor
roles.
*
*
* @param updatePermissionsRequest
* @return A Java Future containing the result of the UpdatePermissions operation returned by the service.
* @sample AmazonManagedGrafanaAsync.UpdatePermissions
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updatePermissionsAsync(UpdatePermissionsRequest updatePermissionsRequest);
/**
*
* Updates which users in a workspace have the Grafana Admin
or Editor
roles.
*
*
* @param updatePermissionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdatePermissions operation returned by the service.
* @sample AmazonManagedGrafanaAsyncHandler.UpdatePermissions
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updatePermissionsAsync(UpdatePermissionsRequest updatePermissionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Modifies an existing Amazon Managed Grafana workspace. If you use this operation and omit any optional
* parameters, the existing values of those parameters are not changed.
*
*
* To modify the user authentication methods that the workspace uses, such as SAML or IAM Identity Center, use
* UpdateWorkspaceAuthentication.
*
*
* To modify which users in the workspace have the Admin
and Editor
Grafana roles, use UpdatePermissions.
*
*
* @param updateWorkspaceRequest
* @return A Java Future containing the result of the UpdateWorkspace operation returned by the service.
* @sample AmazonManagedGrafanaAsync.UpdateWorkspace
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateWorkspaceAsync(UpdateWorkspaceRequest updateWorkspaceRequest);
/**
*
* Modifies an existing Amazon Managed Grafana workspace. If you use this operation and omit any optional
* parameters, the existing values of those parameters are not changed.
*
*
* To modify the user authentication methods that the workspace uses, such as SAML or IAM Identity Center, use
* UpdateWorkspaceAuthentication.
*
*
* To modify which users in the workspace have the Admin
and Editor
Grafana roles, use UpdatePermissions.
*
*
* @param updateWorkspaceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateWorkspace operation returned by the service.
* @sample AmazonManagedGrafanaAsyncHandler.UpdateWorkspace
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateWorkspaceAsync(UpdateWorkspaceRequest updateWorkspaceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Use this operation to define the identity provider (IdP) that this workspace authenticates users from, using
* SAML. You can also map SAML assertion attributes to workspace user information and define which groups in the
* assertion attribute are to have the Admin
and Editor
roles in the workspace.
*
*
*
* Changes to the authentication method for a workspace may take a few minutes to take effect.
*
*
*
* @param updateWorkspaceAuthenticationRequest
* @return A Java Future containing the result of the UpdateWorkspaceAuthentication operation returned by the
* service.
* @sample AmazonManagedGrafanaAsync.UpdateWorkspaceAuthentication
* @see AWS API Documentation
*/
java.util.concurrent.Future updateWorkspaceAuthenticationAsync(
UpdateWorkspaceAuthenticationRequest updateWorkspaceAuthenticationRequest);
/**
*
* Use this operation to define the identity provider (IdP) that this workspace authenticates users from, using
* SAML. You can also map SAML assertion attributes to workspace user information and define which groups in the
* assertion attribute are to have the Admin
and Editor
roles in the workspace.
*
*
*
* Changes to the authentication method for a workspace may take a few minutes to take effect.
*
*
*
* @param updateWorkspaceAuthenticationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateWorkspaceAuthentication operation returned by the
* service.
* @sample AmazonManagedGrafanaAsyncHandler.UpdateWorkspaceAuthentication
* @see AWS API Documentation
*/
java.util.concurrent.Future updateWorkspaceAuthenticationAsync(
UpdateWorkspaceAuthenticationRequest updateWorkspaceAuthenticationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the configuration string for the given workspace
*
*
* @param updateWorkspaceConfigurationRequest
* @return A Java Future containing the result of the UpdateWorkspaceConfiguration operation returned by the
* service.
* @sample AmazonManagedGrafanaAsync.UpdateWorkspaceConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future updateWorkspaceConfigurationAsync(
UpdateWorkspaceConfigurationRequest updateWorkspaceConfigurationRequest);
/**
*
* Updates the configuration string for the given workspace
*
*
* @param updateWorkspaceConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateWorkspaceConfiguration operation returned by the
* service.
* @sample AmazonManagedGrafanaAsyncHandler.UpdateWorkspaceConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future updateWorkspaceConfigurationAsync(
UpdateWorkspaceConfigurationRequest updateWorkspaceConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}