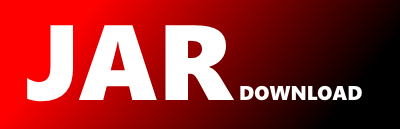
com.amazonaws.services.managedgrafana.AmazonManagedGrafanaClient Maven / Gradle / Ivy
Show all versions of aws-java-sdk-managedgrafana Show documentation
/*
* Copyright 2018-2023 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.managedgrafana;
import org.w3c.dom.*;
import java.net.*;
import java.util.*;
import javax.annotation.Generated;
import org.apache.commons.logging.*;
import com.amazonaws.*;
import com.amazonaws.annotation.SdkInternalApi;
import com.amazonaws.auth.*;
import com.amazonaws.handlers.*;
import com.amazonaws.http.*;
import com.amazonaws.internal.*;
import com.amazonaws.internal.auth.*;
import com.amazonaws.metrics.*;
import com.amazonaws.regions.*;
import com.amazonaws.transform.*;
import com.amazonaws.util.*;
import com.amazonaws.protocol.json.*;
import com.amazonaws.util.AWSRequestMetrics.Field;
import com.amazonaws.annotation.ThreadSafe;
import com.amazonaws.client.AwsSyncClientParams;
import com.amazonaws.client.builder.AdvancedConfig;
import com.amazonaws.services.managedgrafana.AmazonManagedGrafanaClientBuilder;
import com.amazonaws.AmazonServiceException;
import com.amazonaws.services.managedgrafana.model.*;
import com.amazonaws.services.managedgrafana.model.transform.*;
/**
* Client for accessing Amazon Managed Grafana. All service calls made using this client are blocking, and will not
* return until the service call completes.
*
*
* Amazon Managed Grafana is a fully managed and secure data visualization service that you can use to instantly query,
* correlate, and visualize operational metrics, logs, and traces from multiple sources. Amazon Managed Grafana makes it
* easy to deploy, operate, and scale Grafana, a widely deployed data visualization tool that is popular for its
* extensible data support.
*
*
* With Amazon Managed Grafana, you create logically isolated Grafana servers called workspaces. In a workspace,
* you can create Grafana dashboards and visualizations to analyze your metrics, logs, and traces without having to
* build, package, or deploy any hardware to run Grafana servers.
*
*/
@ThreadSafe
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AmazonManagedGrafanaClient extends AmazonWebServiceClient implements AmazonManagedGrafana {
/** Provider for AWS credentials. */
private final AWSCredentialsProvider awsCredentialsProvider;
private static final Log log = LogFactory.getLog(AmazonManagedGrafana.class);
/** Default signing name for the service. */
private static final String DEFAULT_SIGNING_NAME = "grafana";
/** Client configuration factory providing ClientConfigurations tailored to this client */
protected static final ClientConfigurationFactory configFactory = new ClientConfigurationFactory();
private final AdvancedConfig advancedConfig;
private static final com.amazonaws.protocol.json.SdkJsonProtocolFactory protocolFactory = new com.amazonaws.protocol.json.SdkJsonProtocolFactory(
new JsonClientMetadata()
.withProtocolVersion("1.1")
.withSupportsCbor(false)
.withSupportsIon(false)
.withContentTypeOverride("application/json")
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("AccessDeniedException").withExceptionUnmarshaller(
com.amazonaws.services.managedgrafana.model.transform.AccessDeniedExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ConflictException").withExceptionUnmarshaller(
com.amazonaws.services.managedgrafana.model.transform.ConflictExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ResourceNotFoundException").withExceptionUnmarshaller(
com.amazonaws.services.managedgrafana.model.transform.ResourceNotFoundExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ThrottlingException").withExceptionUnmarshaller(
com.amazonaws.services.managedgrafana.model.transform.ThrottlingExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ValidationException").withExceptionUnmarshaller(
com.amazonaws.services.managedgrafana.model.transform.ValidationExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ServiceQuotaExceededException").withExceptionUnmarshaller(
com.amazonaws.services.managedgrafana.model.transform.ServiceQuotaExceededExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InternalServerException").withExceptionUnmarshaller(
com.amazonaws.services.managedgrafana.model.transform.InternalServerExceptionUnmarshaller.getInstance()))
.withBaseServiceExceptionClass(com.amazonaws.services.managedgrafana.model.AmazonManagedGrafanaException.class));
public static AmazonManagedGrafanaClientBuilder builder() {
return AmazonManagedGrafanaClientBuilder.standard();
}
/**
* Constructs a new client to invoke service methods on Amazon Managed Grafana using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AmazonManagedGrafanaClient(AwsSyncClientParams clientParams) {
this(clientParams, false);
}
/**
* Constructs a new client to invoke service methods on Amazon Managed Grafana using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AmazonManagedGrafanaClient(AwsSyncClientParams clientParams, boolean endpointDiscoveryEnabled) {
super(clientParams);
this.awsCredentialsProvider = clientParams.getCredentialsProvider();
this.advancedConfig = clientParams.getAdvancedConfig();
init();
}
private void init() {
setServiceNameIntern(DEFAULT_SIGNING_NAME);
setEndpointPrefix(ENDPOINT_PREFIX);
// calling this.setEndPoint(...) will also modify the signer accordingly
setEndpoint("grafana.us-east-1.amazonaws.com");
HandlerChainFactory chainFactory = new HandlerChainFactory();
requestHandler2s.addAll(chainFactory.newRequestHandlerChain("/com/amazonaws/services/managedgrafana/request.handlers"));
requestHandler2s.addAll(chainFactory.newRequestHandler2Chain("/com/amazonaws/services/managedgrafana/request.handler2s"));
requestHandler2s.addAll(chainFactory.getGlobalHandlers());
}
/**
*
* Assigns a Grafana Enterprise license to a workspace. Upgrading to Grafana Enterprise incurs additional fees. For
* more information, see Upgrade a
* workspace to Grafana Enterprise.
*
*
* @param associateLicenseRequest
* @return Result of the AssociateLicense operation returned by the service.
* @throws ResourceNotFoundException
* The request references a resource that does not exist.
* @throws ThrottlingException
* The request was denied because of request throttling. Retry the request.
* @throws ValidationException
* The value of a parameter in the request caused an error.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action.
* @throws InternalServerException
* Unexpected error while processing the request. Retry the request.
* @sample AmazonManagedGrafana.AssociateLicense
* @see AWS API
* Documentation
*/
@Override
public AssociateLicenseResult associateLicense(AssociateLicenseRequest request) {
request = beforeClientExecution(request);
return executeAssociateLicense(request);
}
@SdkInternalApi
final AssociateLicenseResult executeAssociateLicense(AssociateLicenseRequest associateLicenseRequest) {
ExecutionContext executionContext = createExecutionContext(associateLicenseRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AssociateLicenseRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(associateLicenseRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "grafana");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "AssociateLicense");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new AssociateLicenseResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a workspace. In a workspace, you can create Grafana dashboards and visualizations to analyze your
* metrics, logs, and traces. You don't have to build, package, or deploy any hardware to run the Grafana server.
*
*
* Don't use CreateWorkspace
to modify an existing workspace. Instead, use UpdateWorkspace.
*
*
* @param createWorkspaceRequest
* @return Result of the CreateWorkspace operation returned by the service.
* @throws ThrottlingException
* The request was denied because of request throttling. Retry the request.
* @throws ConflictException
* A resource was in an inconsistent state during an update or a deletion.
* @throws ValidationException
* The value of a parameter in the request caused an error.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action.
* @throws InternalServerException
* Unexpected error while processing the request. Retry the request.
* @throws ServiceQuotaExceededException
* The request would cause a service quota to be exceeded.
* @sample AmazonManagedGrafana.CreateWorkspace
* @see AWS API
* Documentation
*/
@Override
public CreateWorkspaceResult createWorkspace(CreateWorkspaceRequest request) {
request = beforeClientExecution(request);
return executeCreateWorkspace(request);
}
@SdkInternalApi
final CreateWorkspaceResult executeCreateWorkspace(CreateWorkspaceRequest createWorkspaceRequest) {
ExecutionContext executionContext = createExecutionContext(createWorkspaceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateWorkspaceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createWorkspaceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "grafana");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateWorkspace");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateWorkspaceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a Grafana API key for the workspace. This key can be used to authenticate requests sent to the
* workspace's HTTP API. See https
* ://docs.aws.amazon.com/grafana/latest/userguide/Using-Grafana-APIs.html for available APIs and example
* requests.
*
*
* @param createWorkspaceApiKeyRequest
* @return Result of the CreateWorkspaceApiKey operation returned by the service.
* @throws ResourceNotFoundException
* The request references a resource that does not exist.
* @throws ThrottlingException
* The request was denied because of request throttling. Retry the request.
* @throws ConflictException
* A resource was in an inconsistent state during an update or a deletion.
* @throws ValidationException
* The value of a parameter in the request caused an error.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action.
* @throws InternalServerException
* Unexpected error while processing the request. Retry the request.
* @throws ServiceQuotaExceededException
* The request would cause a service quota to be exceeded.
* @sample AmazonManagedGrafana.CreateWorkspaceApiKey
* @see AWS
* API Documentation
*/
@Override
public CreateWorkspaceApiKeyResult createWorkspaceApiKey(CreateWorkspaceApiKeyRequest request) {
request = beforeClientExecution(request);
return executeCreateWorkspaceApiKey(request);
}
@SdkInternalApi
final CreateWorkspaceApiKeyResult executeCreateWorkspaceApiKey(CreateWorkspaceApiKeyRequest createWorkspaceApiKeyRequest) {
ExecutionContext executionContext = createExecutionContext(createWorkspaceApiKeyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateWorkspaceApiKeyRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createWorkspaceApiKeyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "grafana");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateWorkspaceApiKey");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateWorkspaceApiKeyResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes an Amazon Managed Grafana workspace.
*
*
* @param deleteWorkspaceRequest
* @return Result of the DeleteWorkspace operation returned by the service.
* @throws ResourceNotFoundException
* The request references a resource that does not exist.
* @throws ThrottlingException
* The request was denied because of request throttling. Retry the request.
* @throws ConflictException
* A resource was in an inconsistent state during an update or a deletion.
* @throws ValidationException
* The value of a parameter in the request caused an error.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action.
* @throws InternalServerException
* Unexpected error while processing the request. Retry the request.
* @sample AmazonManagedGrafana.DeleteWorkspace
* @see AWS API
* Documentation
*/
@Override
public DeleteWorkspaceResult deleteWorkspace(DeleteWorkspaceRequest request) {
request = beforeClientExecution(request);
return executeDeleteWorkspace(request);
}
@SdkInternalApi
final DeleteWorkspaceResult executeDeleteWorkspace(DeleteWorkspaceRequest deleteWorkspaceRequest) {
ExecutionContext executionContext = createExecutionContext(deleteWorkspaceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteWorkspaceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteWorkspaceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "grafana");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteWorkspace");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteWorkspaceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a Grafana API key for the workspace.
*
*
* @param deleteWorkspaceApiKeyRequest
* @return Result of the DeleteWorkspaceApiKey operation returned by the service.
* @throws ResourceNotFoundException
* The request references a resource that does not exist.
* @throws ThrottlingException
* The request was denied because of request throttling. Retry the request.
* @throws ConflictException
* A resource was in an inconsistent state during an update or a deletion.
* @throws ValidationException
* The value of a parameter in the request caused an error.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action.
* @throws InternalServerException
* Unexpected error while processing the request. Retry the request.
* @sample AmazonManagedGrafana.DeleteWorkspaceApiKey
* @see AWS
* API Documentation
*/
@Override
public DeleteWorkspaceApiKeyResult deleteWorkspaceApiKey(DeleteWorkspaceApiKeyRequest request) {
request = beforeClientExecution(request);
return executeDeleteWorkspaceApiKey(request);
}
@SdkInternalApi
final DeleteWorkspaceApiKeyResult executeDeleteWorkspaceApiKey(DeleteWorkspaceApiKeyRequest deleteWorkspaceApiKeyRequest) {
ExecutionContext executionContext = createExecutionContext(deleteWorkspaceApiKeyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteWorkspaceApiKeyRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteWorkspaceApiKeyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "grafana");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteWorkspaceApiKey");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteWorkspaceApiKeyResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Displays information about one Amazon Managed Grafana workspace.
*
*
* @param describeWorkspaceRequest
* @return Result of the DescribeWorkspace operation returned by the service.
* @throws ResourceNotFoundException
* The request references a resource that does not exist.
* @throws ThrottlingException
* The request was denied because of request throttling. Retry the request.
* @throws ValidationException
* The value of a parameter in the request caused an error.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action.
* @throws InternalServerException
* Unexpected error while processing the request. Retry the request.
* @sample AmazonManagedGrafana.DescribeWorkspace
* @see AWS API
* Documentation
*/
@Override
public DescribeWorkspaceResult describeWorkspace(DescribeWorkspaceRequest request) {
request = beforeClientExecution(request);
return executeDescribeWorkspace(request);
}
@SdkInternalApi
final DescribeWorkspaceResult executeDescribeWorkspace(DescribeWorkspaceRequest describeWorkspaceRequest) {
ExecutionContext executionContext = createExecutionContext(describeWorkspaceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeWorkspaceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeWorkspaceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "grafana");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeWorkspace");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeWorkspaceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Displays information about the authentication methods used in one Amazon Managed Grafana workspace.
*
*
* @param describeWorkspaceAuthenticationRequest
* @return Result of the DescribeWorkspaceAuthentication operation returned by the service.
* @throws ResourceNotFoundException
* The request references a resource that does not exist.
* @throws ThrottlingException
* The request was denied because of request throttling. Retry the request.
* @throws ValidationException
* The value of a parameter in the request caused an error.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action.
* @throws InternalServerException
* Unexpected error while processing the request. Retry the request.
* @sample AmazonManagedGrafana.DescribeWorkspaceAuthentication
* @see AWS API Documentation
*/
@Override
public DescribeWorkspaceAuthenticationResult describeWorkspaceAuthentication(DescribeWorkspaceAuthenticationRequest request) {
request = beforeClientExecution(request);
return executeDescribeWorkspaceAuthentication(request);
}
@SdkInternalApi
final DescribeWorkspaceAuthenticationResult executeDescribeWorkspaceAuthentication(
DescribeWorkspaceAuthenticationRequest describeWorkspaceAuthenticationRequest) {
ExecutionContext executionContext = createExecutionContext(describeWorkspaceAuthenticationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeWorkspaceAuthenticationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeWorkspaceAuthenticationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "grafana");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeWorkspaceAuthentication");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeWorkspaceAuthenticationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets the current configuration string for the given workspace.
*
*
* @param describeWorkspaceConfigurationRequest
* @return Result of the DescribeWorkspaceConfiguration operation returned by the service.
* @throws ResourceNotFoundException
* The request references a resource that does not exist.
* @throws ThrottlingException
* The request was denied because of request throttling. Retry the request.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action.
* @throws InternalServerException
* Unexpected error while processing the request. Retry the request.
* @sample AmazonManagedGrafana.DescribeWorkspaceConfiguration
* @see AWS API Documentation
*/
@Override
public DescribeWorkspaceConfigurationResult describeWorkspaceConfiguration(DescribeWorkspaceConfigurationRequest request) {
request = beforeClientExecution(request);
return executeDescribeWorkspaceConfiguration(request);
}
@SdkInternalApi
final DescribeWorkspaceConfigurationResult executeDescribeWorkspaceConfiguration(DescribeWorkspaceConfigurationRequest describeWorkspaceConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(describeWorkspaceConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeWorkspaceConfigurationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeWorkspaceConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "grafana");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeWorkspaceConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeWorkspaceConfigurationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Removes the Grafana Enterprise license from a workspace.
*
*
* @param disassociateLicenseRequest
* @return Result of the DisassociateLicense operation returned by the service.
* @throws ResourceNotFoundException
* The request references a resource that does not exist.
* @throws ThrottlingException
* The request was denied because of request throttling. Retry the request.
* @throws ValidationException
* The value of a parameter in the request caused an error.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action.
* @throws InternalServerException
* Unexpected error while processing the request. Retry the request.
* @sample AmazonManagedGrafana.DisassociateLicense
* @see AWS
* API Documentation
*/
@Override
public DisassociateLicenseResult disassociateLicense(DisassociateLicenseRequest request) {
request = beforeClientExecution(request);
return executeDisassociateLicense(request);
}
@SdkInternalApi
final DisassociateLicenseResult executeDisassociateLicense(DisassociateLicenseRequest disassociateLicenseRequest) {
ExecutionContext executionContext = createExecutionContext(disassociateLicenseRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DisassociateLicenseRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(disassociateLicenseRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "grafana");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DisassociateLicense");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DisassociateLicenseResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the users and groups who have the Grafana Admin
and Editor
roles in this
* workspace. If you use this operation without specifying userId
or groupId
, the
* operation returns the roles of all users and groups. If you specify a userId
or a
* groupId
, only the roles for that user or group are returned. If you do this, you can specify only
* one userId
or one groupId
.
*
*
* @param listPermissionsRequest
* @return Result of the ListPermissions operation returned by the service.
* @throws ResourceNotFoundException
* The request references a resource that does not exist.
* @throws ThrottlingException
* The request was denied because of request throttling. Retry the request.
* @throws ValidationException
* The value of a parameter in the request caused an error.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action.
* @throws InternalServerException
* Unexpected error while processing the request. Retry the request.
* @sample AmazonManagedGrafana.ListPermissions
* @see AWS API
* Documentation
*/
@Override
public ListPermissionsResult listPermissions(ListPermissionsRequest request) {
request = beforeClientExecution(request);
return executeListPermissions(request);
}
@SdkInternalApi
final ListPermissionsResult executeListPermissions(ListPermissionsRequest listPermissionsRequest) {
ExecutionContext executionContext = createExecutionContext(listPermissionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListPermissionsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listPermissionsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "grafana");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListPermissions");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListPermissionsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* The ListTagsForResource
operation returns the tags that are associated with the Amazon Managed
* Service for Grafana resource specified by the resourceArn
. Currently, the only resource that can be
* tagged is a workspace.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws ResourceNotFoundException
* The request references a resource that does not exist.
* @throws ThrottlingException
* The request was denied because of request throttling. Retry the request.
* @throws ValidationException
* The value of a parameter in the request caused an error.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action.
* @throws InternalServerException
* Unexpected error while processing the request. Retry the request.
* @sample AmazonManagedGrafana.ListTagsForResource
* @see AWS
* API Documentation
*/
@Override
public ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest request) {
request = beforeClientExecution(request);
return executeListTagsForResource(request);
}
@SdkInternalApi
final ListTagsForResourceResult executeListTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest) {
ExecutionContext executionContext = createExecutionContext(listTagsForResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListTagsForResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listTagsForResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "grafana");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListTagsForResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListTagsForResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists available versions of Grafana. These are available when calling CreateWorkspace
. Optionally,
* include a workspace to list the versions to which it can be upgraded.
*
*
* @param listVersionsRequest
* @return Result of the ListVersions operation returned by the service.
* @throws ResourceNotFoundException
* The request references a resource that does not exist.
* @throws ThrottlingException
* The request was denied because of request throttling. Retry the request.
* @throws ValidationException
* The value of a parameter in the request caused an error.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action.
* @throws InternalServerException
* Unexpected error while processing the request. Retry the request.
* @sample AmazonManagedGrafana.ListVersions
* @see AWS API
* Documentation
*/
@Override
public ListVersionsResult listVersions(ListVersionsRequest request) {
request = beforeClientExecution(request);
return executeListVersions(request);
}
@SdkInternalApi
final ListVersionsResult executeListVersions(ListVersionsRequest listVersionsRequest) {
ExecutionContext executionContext = createExecutionContext(listVersionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListVersionsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listVersionsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "grafana");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListVersions");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListVersionsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of Amazon Managed Grafana workspaces in the account, with some information about each workspace.
* For more complete information about one workspace, use DescribeWorkspace.
*
*
* @param listWorkspacesRequest
* @return Result of the ListWorkspaces operation returned by the service.
* @throws ThrottlingException
* The request was denied because of request throttling. Retry the request.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action.
* @throws InternalServerException
* Unexpected error while processing the request. Retry the request.
* @sample AmazonManagedGrafana.ListWorkspaces
* @see AWS API
* Documentation
*/
@Override
public ListWorkspacesResult listWorkspaces(ListWorkspacesRequest request) {
request = beforeClientExecution(request);
return executeListWorkspaces(request);
}
@SdkInternalApi
final ListWorkspacesResult executeListWorkspaces(ListWorkspacesRequest listWorkspacesRequest) {
ExecutionContext executionContext = createExecutionContext(listWorkspacesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListWorkspacesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listWorkspacesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "grafana");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListWorkspaces");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListWorkspacesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* The TagResource
operation associates tags with an Amazon Managed Grafana resource. Currently, the
* only resource that can be tagged is workspaces.
*
*
* If you specify a new tag key for the resource, this tag is appended to the list of tags associated with the
* resource. If you specify a tag key that is already associated with the resource, the new tag value that you
* specify replaces the previous value for that tag.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws ResourceNotFoundException
* The request references a resource that does not exist.
* @throws ThrottlingException
* The request was denied because of request throttling. Retry the request.
* @throws ValidationException
* The value of a parameter in the request caused an error.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action.
* @throws InternalServerException
* Unexpected error while processing the request. Retry the request.
* @sample AmazonManagedGrafana.TagResource
* @see AWS API
* Documentation
*/
@Override
public TagResourceResult tagResource(TagResourceRequest request) {
request = beforeClientExecution(request);
return executeTagResource(request);
}
@SdkInternalApi
final TagResourceResult executeTagResource(TagResourceRequest tagResourceRequest) {
ExecutionContext executionContext = createExecutionContext(tagResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new TagResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(tagResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "grafana");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "TagResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new TagResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* The UntagResource
operation removes the association of the tag with the Amazon Managed Grafana
* resource.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws ResourceNotFoundException
* The request references a resource that does not exist.
* @throws ThrottlingException
* The request was denied because of request throttling. Retry the request.
* @throws ValidationException
* The value of a parameter in the request caused an error.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action.
* @throws InternalServerException
* Unexpected error while processing the request. Retry the request.
* @sample AmazonManagedGrafana.UntagResource
* @see AWS API
* Documentation
*/
@Override
public UntagResourceResult untagResource(UntagResourceRequest request) {
request = beforeClientExecution(request);
return executeUntagResource(request);
}
@SdkInternalApi
final UntagResourceResult executeUntagResource(UntagResourceRequest untagResourceRequest) {
ExecutionContext executionContext = createExecutionContext(untagResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UntagResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(untagResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "grafana");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UntagResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UntagResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates which users in a workspace have the Grafana Admin
or Editor
roles.
*
*
* @param updatePermissionsRequest
* @return Result of the UpdatePermissions operation returned by the service.
* @throws ResourceNotFoundException
* The request references a resource that does not exist.
* @throws ThrottlingException
* The request was denied because of request throttling. Retry the request.
* @throws ValidationException
* The value of a parameter in the request caused an error.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action.
* @throws InternalServerException
* Unexpected error while processing the request. Retry the request.
* @sample AmazonManagedGrafana.UpdatePermissions
* @see AWS API
* Documentation
*/
@Override
public UpdatePermissionsResult updatePermissions(UpdatePermissionsRequest request) {
request = beforeClientExecution(request);
return executeUpdatePermissions(request);
}
@SdkInternalApi
final UpdatePermissionsResult executeUpdatePermissions(UpdatePermissionsRequest updatePermissionsRequest) {
ExecutionContext executionContext = createExecutionContext(updatePermissionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdatePermissionsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updatePermissionsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "grafana");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdatePermissions");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdatePermissionsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Modifies an existing Amazon Managed Grafana workspace. If you use this operation and omit any optional
* parameters, the existing values of those parameters are not changed.
*
*
* To modify the user authentication methods that the workspace uses, such as SAML or IAM Identity Center, use
* UpdateWorkspaceAuthentication.
*
*
* To modify which users in the workspace have the Admin
and Editor
Grafana roles, use UpdatePermissions.
*
*
* @param updateWorkspaceRequest
* @return Result of the UpdateWorkspace operation returned by the service.
* @throws ResourceNotFoundException
* The request references a resource that does not exist.
* @throws ThrottlingException
* The request was denied because of request throttling. Retry the request.
* @throws ConflictException
* A resource was in an inconsistent state during an update or a deletion.
* @throws ValidationException
* The value of a parameter in the request caused an error.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action.
* @throws InternalServerException
* Unexpected error while processing the request. Retry the request.
* @sample AmazonManagedGrafana.UpdateWorkspace
* @see AWS API
* Documentation
*/
@Override
public UpdateWorkspaceResult updateWorkspace(UpdateWorkspaceRequest request) {
request = beforeClientExecution(request);
return executeUpdateWorkspace(request);
}
@SdkInternalApi
final UpdateWorkspaceResult executeUpdateWorkspace(UpdateWorkspaceRequest updateWorkspaceRequest) {
ExecutionContext executionContext = createExecutionContext(updateWorkspaceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateWorkspaceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateWorkspaceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "grafana");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateWorkspace");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateWorkspaceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Use this operation to define the identity provider (IdP) that this workspace authenticates users from, using
* SAML. You can also map SAML assertion attributes to workspace user information and define which groups in the
* assertion attribute are to have the Admin
and Editor
roles in the workspace.
*
*
*
* Changes to the authentication method for a workspace may take a few minutes to take effect.
*
*
*
* @param updateWorkspaceAuthenticationRequest
* @return Result of the UpdateWorkspaceAuthentication operation returned by the service.
* @throws ResourceNotFoundException
* The request references a resource that does not exist.
* @throws ThrottlingException
* The request was denied because of request throttling. Retry the request.
* @throws ConflictException
* A resource was in an inconsistent state during an update or a deletion.
* @throws ValidationException
* The value of a parameter in the request caused an error.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action.
* @throws InternalServerException
* Unexpected error while processing the request. Retry the request.
* @sample AmazonManagedGrafana.UpdateWorkspaceAuthentication
* @see AWS API Documentation
*/
@Override
public UpdateWorkspaceAuthenticationResult updateWorkspaceAuthentication(UpdateWorkspaceAuthenticationRequest request) {
request = beforeClientExecution(request);
return executeUpdateWorkspaceAuthentication(request);
}
@SdkInternalApi
final UpdateWorkspaceAuthenticationResult executeUpdateWorkspaceAuthentication(UpdateWorkspaceAuthenticationRequest updateWorkspaceAuthenticationRequest) {
ExecutionContext executionContext = createExecutionContext(updateWorkspaceAuthenticationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateWorkspaceAuthenticationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(updateWorkspaceAuthenticationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "grafana");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateWorkspaceAuthentication");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new UpdateWorkspaceAuthenticationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates the configuration string for the given workspace
*
*
* @param updateWorkspaceConfigurationRequest
* @return Result of the UpdateWorkspaceConfiguration operation returned by the service.
* @throws ResourceNotFoundException
* The request references a resource that does not exist.
* @throws ThrottlingException
* The request was denied because of request throttling. Retry the request.
* @throws ConflictException
* A resource was in an inconsistent state during an update or a deletion.
* @throws ValidationException
* The value of a parameter in the request caused an error.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action.
* @throws InternalServerException
* Unexpected error while processing the request. Retry the request.
* @sample AmazonManagedGrafana.UpdateWorkspaceConfiguration
* @see AWS API Documentation
*/
@Override
public UpdateWorkspaceConfigurationResult updateWorkspaceConfiguration(UpdateWorkspaceConfigurationRequest request) {
request = beforeClientExecution(request);
return executeUpdateWorkspaceConfiguration(request);
}
@SdkInternalApi
final UpdateWorkspaceConfigurationResult executeUpdateWorkspaceConfiguration(UpdateWorkspaceConfigurationRequest updateWorkspaceConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(updateWorkspaceConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateWorkspaceConfigurationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(updateWorkspaceConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "grafana");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateWorkspaceConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new UpdateWorkspaceConfigurationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
* Returns additional metadata for a previously executed successful, request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing the request.
*
* @param request
* The originally executed request
*
* @return The response metadata for the specified request, or null if none is available.
*/
public ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request) {
return client.getResponseMetadataForRequest(request);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext) {
return invoke(request, responseHandler, executionContext, null, null);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI cachedEndpoint, URI uriFromEndpointTrait) {
executionContext.setCredentialsProvider(CredentialUtils.getCredentialsProvider(request.getOriginalRequest(), awsCredentialsProvider));
return doInvoke(request, responseHandler, executionContext, cachedEndpoint, uriFromEndpointTrait);
}
/**
* Invoke with no authentication. Credentials are not required and any credentials set on the client or request will
* be ignored for this operation.
**/
private Response anonymousInvoke(Request request,
HttpResponseHandler> responseHandler, ExecutionContext executionContext) {
return doInvoke(request, responseHandler, executionContext, null, null);
}
/**
* Invoke the request using the http client. Assumes credentials (or lack thereof) have been configured in the
* ExecutionContext beforehand.
**/
private Response doInvoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI discoveredEndpoint, URI uriFromEndpointTrait) {
if (discoveredEndpoint != null) {
request.setEndpoint(discoveredEndpoint);
request.getOriginalRequest().getRequestClientOptions().appendUserAgent("endpoint-discovery");
} else if (uriFromEndpointTrait != null) {
request.setEndpoint(uriFromEndpointTrait);
} else {
request.setEndpoint(endpoint);
}
request.setTimeOffset(timeOffset);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler(new JsonErrorResponseMetadata());
return client.execute(request, responseHandler, errorResponseHandler, executionContext);
}
@com.amazonaws.annotation.SdkInternalApi
static com.amazonaws.protocol.json.SdkJsonProtocolFactory getProtocolFactory() {
return protocolFactory;
}
@Override
public void shutdown() {
super.shutdown();
}
}