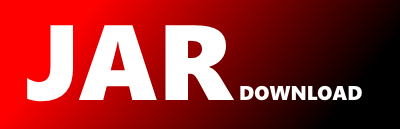
com.amazonaws.services.managedgrafana.model.WorkspaceDescription Maven / Gradle / Ivy
Show all versions of aws-java-sdk-managedgrafana Show documentation
/*
* Copyright 2018-2023 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.managedgrafana.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* A structure containing information about an Amazon Managed Grafana workspace in your account.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class WorkspaceDescription implements Serializable, Cloneable, StructuredPojo {
/**
*
* Specifies whether the workspace can access Amazon Web Services resources in this Amazon Web Services account
* only, or whether it can also access Amazon Web Services resources in other accounts in the same organization. If
* this is ORGANIZATION
, the workspaceOrganizationalUnits
parameter specifies which
* organizational units the workspace can access.
*
*/
private String accountAccessType;
/**
*
* A structure that describes whether the workspace uses SAML, IAM Identity Center, or both methods for user
* authentication.
*
*/
private AuthenticationSummary authentication;
/**
*
* The date that the workspace was created.
*
*/
private java.util.Date created;
/**
*
* Specifies the Amazon Web Services data sources that have been configured to have IAM roles and permissions
* created to allow Amazon Managed Grafana to read data from these sources.
*
*
* This list is only used when the workspace was created through the Amazon Web Services console, and the
* permissionType
is SERVICE_MANAGED
.
*
*/
private java.util.List dataSources;
/**
*
* The user-defined description of the workspace.
*
*/
private String description;
/**
*
* The URL that users can use to access the Grafana console in the workspace.
*
*/
private String endpoint;
/**
*
* Specifies whether this workspace has already fully used its free trial for Grafana Enterprise.
*
*/
private Boolean freeTrialConsumed;
/**
*
* If this workspace is currently in the free trial period for Grafana Enterprise, this value specifies when that
* free trial ends.
*
*/
private java.util.Date freeTrialExpiration;
/**
*
* The version of Grafana supported in this workspace.
*
*/
private String grafanaVersion;
/**
*
* The unique ID of this workspace.
*
*/
private String id;
/**
*
* If this workspace has a full Grafana Enterprise license, this specifies when the license ends and will need to be
* renewed.
*
*/
private java.util.Date licenseExpiration;
/**
*
* Specifies whether this workspace has a full Grafana Enterprise license or a free trial license.
*
*/
private String licenseType;
/**
*
* The most recent date that the workspace was modified.
*
*/
private java.util.Date modified;
/**
*
* The name of the workspace.
*
*/
private String name;
/**
*
* The configuration settings for network access to your workspace.
*
*/
private NetworkAccessConfiguration networkAccessControl;
/**
*
* The Amazon Web Services notification channels that Amazon Managed Grafana can automatically create IAM roles and
* permissions for, to allow Amazon Managed Grafana to use these channels.
*
*/
private java.util.List notificationDestinations;
/**
*
* The name of the IAM role that is used to access resources through Organizations.
*
*/
private String organizationRoleName;
/**
*
* Specifies the organizational units that this workspace is allowed to use data sources from, if this workspace is
* in an account that is part of an organization.
*
*/
private java.util.List organizationalUnits;
/**
*
* If this is SERVICE_MANAGED
, and the workplace was created through the Amazon Managed Grafana
* console, then Amazon Managed Grafana automatically creates the IAM roles and provisions the permissions that the
* workspace needs to use Amazon Web Services data sources and notification channels.
*
*
* If this is CUSTOMER_MANAGED
, you must manage those roles and permissions yourself.
*
*
* If you are working with a workspace in a member account of an organization and that account is not a delegated
* administrator account, and you want the workspace to access data sources in other Amazon Web Services accounts in
* the organization, this parameter must be set to CUSTOMER_MANAGED
.
*
*
* For more information about converting between customer and service managed, see Managing
* permissions for data sources and notification channels. For more information about the roles and permissions
* that must be managed for customer managed workspaces, see Amazon Managed Grafana
* permissions and policies for Amazon Web Services data sources and notification channels
*
*/
private String permissionType;
/**
*
* The name of the CloudFormation stack set that is used to generate IAM roles to be used for this workspace.
*
*/
private String stackSetName;
/**
*
* The current status of the workspace.
*
*/
private String status;
/**
*
* The list of tags associated with the workspace.
*
*/
private java.util.Map tags;
/**
*
* The configuration for connecting to data sources in a private VPC (Amazon Virtual Private Cloud).
*
*/
private VpcConfiguration vpcConfiguration;
/**
*
* The IAM role that grants permissions to the Amazon Web Services resources that the workspace will view data from.
* This role must already exist.
*
*/
private String workspaceRoleArn;
/**
*
* Specifies whether the workspace can access Amazon Web Services resources in this Amazon Web Services account
* only, or whether it can also access Amazon Web Services resources in other accounts in the same organization. If
* this is ORGANIZATION
, the workspaceOrganizationalUnits
parameter specifies which
* organizational units the workspace can access.
*
*
* @param accountAccessType
* Specifies whether the workspace can access Amazon Web Services resources in this Amazon Web Services
* account only, or whether it can also access Amazon Web Services resources in other accounts in the same
* organization. If this is ORGANIZATION
, the workspaceOrganizationalUnits
* parameter specifies which organizational units the workspace can access.
* @see AccountAccessType
*/
public void setAccountAccessType(String accountAccessType) {
this.accountAccessType = accountAccessType;
}
/**
*
* Specifies whether the workspace can access Amazon Web Services resources in this Amazon Web Services account
* only, or whether it can also access Amazon Web Services resources in other accounts in the same organization. If
* this is ORGANIZATION
, the workspaceOrganizationalUnits
parameter specifies which
* organizational units the workspace can access.
*
*
* @return Specifies whether the workspace can access Amazon Web Services resources in this Amazon Web Services
* account only, or whether it can also access Amazon Web Services resources in other accounts in the same
* organization. If this is ORGANIZATION
, the workspaceOrganizationalUnits
* parameter specifies which organizational units the workspace can access.
* @see AccountAccessType
*/
public String getAccountAccessType() {
return this.accountAccessType;
}
/**
*
* Specifies whether the workspace can access Amazon Web Services resources in this Amazon Web Services account
* only, or whether it can also access Amazon Web Services resources in other accounts in the same organization. If
* this is ORGANIZATION
, the workspaceOrganizationalUnits
parameter specifies which
* organizational units the workspace can access.
*
*
* @param accountAccessType
* Specifies whether the workspace can access Amazon Web Services resources in this Amazon Web Services
* account only, or whether it can also access Amazon Web Services resources in other accounts in the same
* organization. If this is ORGANIZATION
, the workspaceOrganizationalUnits
* parameter specifies which organizational units the workspace can access.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AccountAccessType
*/
public WorkspaceDescription withAccountAccessType(String accountAccessType) {
setAccountAccessType(accountAccessType);
return this;
}
/**
*
* Specifies whether the workspace can access Amazon Web Services resources in this Amazon Web Services account
* only, or whether it can also access Amazon Web Services resources in other accounts in the same organization. If
* this is ORGANIZATION
, the workspaceOrganizationalUnits
parameter specifies which
* organizational units the workspace can access.
*
*
* @param accountAccessType
* Specifies whether the workspace can access Amazon Web Services resources in this Amazon Web Services
* account only, or whether it can also access Amazon Web Services resources in other accounts in the same
* organization. If this is ORGANIZATION
, the workspaceOrganizationalUnits
* parameter specifies which organizational units the workspace can access.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AccountAccessType
*/
public WorkspaceDescription withAccountAccessType(AccountAccessType accountAccessType) {
this.accountAccessType = accountAccessType.toString();
return this;
}
/**
*
* A structure that describes whether the workspace uses SAML, IAM Identity Center, or both methods for user
* authentication.
*
*
* @param authentication
* A structure that describes whether the workspace uses SAML, IAM Identity Center, or both methods for user
* authentication.
*/
public void setAuthentication(AuthenticationSummary authentication) {
this.authentication = authentication;
}
/**
*
* A structure that describes whether the workspace uses SAML, IAM Identity Center, or both methods for user
* authentication.
*
*
* @return A structure that describes whether the workspace uses SAML, IAM Identity Center, or both methods for user
* authentication.
*/
public AuthenticationSummary getAuthentication() {
return this.authentication;
}
/**
*
* A structure that describes whether the workspace uses SAML, IAM Identity Center, or both methods for user
* authentication.
*
*
* @param authentication
* A structure that describes whether the workspace uses SAML, IAM Identity Center, or both methods for user
* authentication.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public WorkspaceDescription withAuthentication(AuthenticationSummary authentication) {
setAuthentication(authentication);
return this;
}
/**
*
* The date that the workspace was created.
*
*
* @param created
* The date that the workspace was created.
*/
public void setCreated(java.util.Date created) {
this.created = created;
}
/**
*
* The date that the workspace was created.
*
*
* @return The date that the workspace was created.
*/
public java.util.Date getCreated() {
return this.created;
}
/**
*
* The date that the workspace was created.
*
*
* @param created
* The date that the workspace was created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public WorkspaceDescription withCreated(java.util.Date created) {
setCreated(created);
return this;
}
/**
*
* Specifies the Amazon Web Services data sources that have been configured to have IAM roles and permissions
* created to allow Amazon Managed Grafana to read data from these sources.
*
*
* This list is only used when the workspace was created through the Amazon Web Services console, and the
* permissionType
is SERVICE_MANAGED
.
*
*
* @return Specifies the Amazon Web Services data sources that have been configured to have IAM roles and
* permissions created to allow Amazon Managed Grafana to read data from these sources.
*
* This list is only used when the workspace was created through the Amazon Web Services console, and the
* permissionType
is SERVICE_MANAGED
.
* @see DataSourceType
*/
public java.util.List getDataSources() {
return dataSources;
}
/**
*
* Specifies the Amazon Web Services data sources that have been configured to have IAM roles and permissions
* created to allow Amazon Managed Grafana to read data from these sources.
*
*
* This list is only used when the workspace was created through the Amazon Web Services console, and the
* permissionType
is SERVICE_MANAGED
.
*
*
* @param dataSources
* Specifies the Amazon Web Services data sources that have been configured to have IAM roles and permissions
* created to allow Amazon Managed Grafana to read data from these sources.
*
* This list is only used when the workspace was created through the Amazon Web Services console, and the
* permissionType
is SERVICE_MANAGED
.
* @see DataSourceType
*/
public void setDataSources(java.util.Collection dataSources) {
if (dataSources == null) {
this.dataSources = null;
return;
}
this.dataSources = new java.util.ArrayList(dataSources);
}
/**
*
* Specifies the Amazon Web Services data sources that have been configured to have IAM roles and permissions
* created to allow Amazon Managed Grafana to read data from these sources.
*
*
* This list is only used when the workspace was created through the Amazon Web Services console, and the
* permissionType
is SERVICE_MANAGED
.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setDataSources(java.util.Collection)} or {@link #withDataSources(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param dataSources
* Specifies the Amazon Web Services data sources that have been configured to have IAM roles and permissions
* created to allow Amazon Managed Grafana to read data from these sources.
*
* This list is only used when the workspace was created through the Amazon Web Services console, and the
* permissionType
is SERVICE_MANAGED
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see DataSourceType
*/
public WorkspaceDescription withDataSources(String... dataSources) {
if (this.dataSources == null) {
setDataSources(new java.util.ArrayList(dataSources.length));
}
for (String ele : dataSources) {
this.dataSources.add(ele);
}
return this;
}
/**
*
* Specifies the Amazon Web Services data sources that have been configured to have IAM roles and permissions
* created to allow Amazon Managed Grafana to read data from these sources.
*
*
* This list is only used when the workspace was created through the Amazon Web Services console, and the
* permissionType
is SERVICE_MANAGED
.
*
*
* @param dataSources
* Specifies the Amazon Web Services data sources that have been configured to have IAM roles and permissions
* created to allow Amazon Managed Grafana to read data from these sources.
*
* This list is only used when the workspace was created through the Amazon Web Services console, and the
* permissionType
is SERVICE_MANAGED
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see DataSourceType
*/
public WorkspaceDescription withDataSources(java.util.Collection dataSources) {
setDataSources(dataSources);
return this;
}
/**
*
* Specifies the Amazon Web Services data sources that have been configured to have IAM roles and permissions
* created to allow Amazon Managed Grafana to read data from these sources.
*
*
* This list is only used when the workspace was created through the Amazon Web Services console, and the
* permissionType
is SERVICE_MANAGED
.
*
*
* @param dataSources
* Specifies the Amazon Web Services data sources that have been configured to have IAM roles and permissions
* created to allow Amazon Managed Grafana to read data from these sources.
*
* This list is only used when the workspace was created through the Amazon Web Services console, and the
* permissionType
is SERVICE_MANAGED
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see DataSourceType
*/
public WorkspaceDescription withDataSources(DataSourceType... dataSources) {
java.util.ArrayList dataSourcesCopy = new java.util.ArrayList(dataSources.length);
for (DataSourceType value : dataSources) {
dataSourcesCopy.add(value.toString());
}
if (getDataSources() == null) {
setDataSources(dataSourcesCopy);
} else {
getDataSources().addAll(dataSourcesCopy);
}
return this;
}
/**
*
* The user-defined description of the workspace.
*
*
* @param description
* The user-defined description of the workspace.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* The user-defined description of the workspace.
*
*
* @return The user-defined description of the workspace.
*/
public String getDescription() {
return this.description;
}
/**
*
* The user-defined description of the workspace.
*
*
* @param description
* The user-defined description of the workspace.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public WorkspaceDescription withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* The URL that users can use to access the Grafana console in the workspace.
*
*
* @param endpoint
* The URL that users can use to access the Grafana console in the workspace.
*/
public void setEndpoint(String endpoint) {
this.endpoint = endpoint;
}
/**
*
* The URL that users can use to access the Grafana console in the workspace.
*
*
* @return The URL that users can use to access the Grafana console in the workspace.
*/
public String getEndpoint() {
return this.endpoint;
}
/**
*
* The URL that users can use to access the Grafana console in the workspace.
*
*
* @param endpoint
* The URL that users can use to access the Grafana console in the workspace.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public WorkspaceDescription withEndpoint(String endpoint) {
setEndpoint(endpoint);
return this;
}
/**
*
* Specifies whether this workspace has already fully used its free trial for Grafana Enterprise.
*
*
* @param freeTrialConsumed
* Specifies whether this workspace has already fully used its free trial for Grafana Enterprise.
*/
public void setFreeTrialConsumed(Boolean freeTrialConsumed) {
this.freeTrialConsumed = freeTrialConsumed;
}
/**
*
* Specifies whether this workspace has already fully used its free trial for Grafana Enterprise.
*
*
* @return Specifies whether this workspace has already fully used its free trial for Grafana Enterprise.
*/
public Boolean getFreeTrialConsumed() {
return this.freeTrialConsumed;
}
/**
*
* Specifies whether this workspace has already fully used its free trial for Grafana Enterprise.
*
*
* @param freeTrialConsumed
* Specifies whether this workspace has already fully used its free trial for Grafana Enterprise.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public WorkspaceDescription withFreeTrialConsumed(Boolean freeTrialConsumed) {
setFreeTrialConsumed(freeTrialConsumed);
return this;
}
/**
*
* Specifies whether this workspace has already fully used its free trial for Grafana Enterprise.
*
*
* @return Specifies whether this workspace has already fully used its free trial for Grafana Enterprise.
*/
public Boolean isFreeTrialConsumed() {
return this.freeTrialConsumed;
}
/**
*
* If this workspace is currently in the free trial period for Grafana Enterprise, this value specifies when that
* free trial ends.
*
*
* @param freeTrialExpiration
* If this workspace is currently in the free trial period for Grafana Enterprise, this value specifies when
* that free trial ends.
*/
public void setFreeTrialExpiration(java.util.Date freeTrialExpiration) {
this.freeTrialExpiration = freeTrialExpiration;
}
/**
*
* If this workspace is currently in the free trial period for Grafana Enterprise, this value specifies when that
* free trial ends.
*
*
* @return If this workspace is currently in the free trial period for Grafana Enterprise, this value specifies when
* that free trial ends.
*/
public java.util.Date getFreeTrialExpiration() {
return this.freeTrialExpiration;
}
/**
*
* If this workspace is currently in the free trial period for Grafana Enterprise, this value specifies when that
* free trial ends.
*
*
* @param freeTrialExpiration
* If this workspace is currently in the free trial period for Grafana Enterprise, this value specifies when
* that free trial ends.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public WorkspaceDescription withFreeTrialExpiration(java.util.Date freeTrialExpiration) {
setFreeTrialExpiration(freeTrialExpiration);
return this;
}
/**
*
* The version of Grafana supported in this workspace.
*
*
* @param grafanaVersion
* The version of Grafana supported in this workspace.
*/
public void setGrafanaVersion(String grafanaVersion) {
this.grafanaVersion = grafanaVersion;
}
/**
*
* The version of Grafana supported in this workspace.
*
*
* @return The version of Grafana supported in this workspace.
*/
public String getGrafanaVersion() {
return this.grafanaVersion;
}
/**
*
* The version of Grafana supported in this workspace.
*
*
* @param grafanaVersion
* The version of Grafana supported in this workspace.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public WorkspaceDescription withGrafanaVersion(String grafanaVersion) {
setGrafanaVersion(grafanaVersion);
return this;
}
/**
*
* The unique ID of this workspace.
*
*
* @param id
* The unique ID of this workspace.
*/
public void setId(String id) {
this.id = id;
}
/**
*
* The unique ID of this workspace.
*
*
* @return The unique ID of this workspace.
*/
public String getId() {
return this.id;
}
/**
*
* The unique ID of this workspace.
*
*
* @param id
* The unique ID of this workspace.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public WorkspaceDescription withId(String id) {
setId(id);
return this;
}
/**
*
* If this workspace has a full Grafana Enterprise license, this specifies when the license ends and will need to be
* renewed.
*
*
* @param licenseExpiration
* If this workspace has a full Grafana Enterprise license, this specifies when the license ends and will
* need to be renewed.
*/
public void setLicenseExpiration(java.util.Date licenseExpiration) {
this.licenseExpiration = licenseExpiration;
}
/**
*
* If this workspace has a full Grafana Enterprise license, this specifies when the license ends and will need to be
* renewed.
*
*
* @return If this workspace has a full Grafana Enterprise license, this specifies when the license ends and will
* need to be renewed.
*/
public java.util.Date getLicenseExpiration() {
return this.licenseExpiration;
}
/**
*
* If this workspace has a full Grafana Enterprise license, this specifies when the license ends and will need to be
* renewed.
*
*
* @param licenseExpiration
* If this workspace has a full Grafana Enterprise license, this specifies when the license ends and will
* need to be renewed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public WorkspaceDescription withLicenseExpiration(java.util.Date licenseExpiration) {
setLicenseExpiration(licenseExpiration);
return this;
}
/**
*
* Specifies whether this workspace has a full Grafana Enterprise license or a free trial license.
*
*
* @param licenseType
* Specifies whether this workspace has a full Grafana Enterprise license or a free trial license.
* @see LicenseType
*/
public void setLicenseType(String licenseType) {
this.licenseType = licenseType;
}
/**
*
* Specifies whether this workspace has a full Grafana Enterprise license or a free trial license.
*
*
* @return Specifies whether this workspace has a full Grafana Enterprise license or a free trial license.
* @see LicenseType
*/
public String getLicenseType() {
return this.licenseType;
}
/**
*
* Specifies whether this workspace has a full Grafana Enterprise license or a free trial license.
*
*
* @param licenseType
* Specifies whether this workspace has a full Grafana Enterprise license or a free trial license.
* @return Returns a reference to this object so that method calls can be chained together.
* @see LicenseType
*/
public WorkspaceDescription withLicenseType(String licenseType) {
setLicenseType(licenseType);
return this;
}
/**
*
* Specifies whether this workspace has a full Grafana Enterprise license or a free trial license.
*
*
* @param licenseType
* Specifies whether this workspace has a full Grafana Enterprise license or a free trial license.
* @return Returns a reference to this object so that method calls can be chained together.
* @see LicenseType
*/
public WorkspaceDescription withLicenseType(LicenseType licenseType) {
this.licenseType = licenseType.toString();
return this;
}
/**
*
* The most recent date that the workspace was modified.
*
*
* @param modified
* The most recent date that the workspace was modified.
*/
public void setModified(java.util.Date modified) {
this.modified = modified;
}
/**
*
* The most recent date that the workspace was modified.
*
*
* @return The most recent date that the workspace was modified.
*/
public java.util.Date getModified() {
return this.modified;
}
/**
*
* The most recent date that the workspace was modified.
*
*
* @param modified
* The most recent date that the workspace was modified.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public WorkspaceDescription withModified(java.util.Date modified) {
setModified(modified);
return this;
}
/**
*
* The name of the workspace.
*
*
* @param name
* The name of the workspace.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The name of the workspace.
*
*
* @return The name of the workspace.
*/
public String getName() {
return this.name;
}
/**
*
* The name of the workspace.
*
*
* @param name
* The name of the workspace.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public WorkspaceDescription withName(String name) {
setName(name);
return this;
}
/**
*
* The configuration settings for network access to your workspace.
*
*
* @param networkAccessControl
* The configuration settings for network access to your workspace.
*/
public void setNetworkAccessControl(NetworkAccessConfiguration networkAccessControl) {
this.networkAccessControl = networkAccessControl;
}
/**
*
* The configuration settings for network access to your workspace.
*
*
* @return The configuration settings for network access to your workspace.
*/
public NetworkAccessConfiguration getNetworkAccessControl() {
return this.networkAccessControl;
}
/**
*
* The configuration settings for network access to your workspace.
*
*
* @param networkAccessControl
* The configuration settings for network access to your workspace.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public WorkspaceDescription withNetworkAccessControl(NetworkAccessConfiguration networkAccessControl) {
setNetworkAccessControl(networkAccessControl);
return this;
}
/**
*
* The Amazon Web Services notification channels that Amazon Managed Grafana can automatically create IAM roles and
* permissions for, to allow Amazon Managed Grafana to use these channels.
*
*
* @return The Amazon Web Services notification channels that Amazon Managed Grafana can automatically create IAM
* roles and permissions for, to allow Amazon Managed Grafana to use these channels.
* @see NotificationDestinationType
*/
public java.util.List getNotificationDestinations() {
return notificationDestinations;
}
/**
*
* The Amazon Web Services notification channels that Amazon Managed Grafana can automatically create IAM roles and
* permissions for, to allow Amazon Managed Grafana to use these channels.
*
*
* @param notificationDestinations
* The Amazon Web Services notification channels that Amazon Managed Grafana can automatically create IAM
* roles and permissions for, to allow Amazon Managed Grafana to use these channels.
* @see NotificationDestinationType
*/
public void setNotificationDestinations(java.util.Collection notificationDestinations) {
if (notificationDestinations == null) {
this.notificationDestinations = null;
return;
}
this.notificationDestinations = new java.util.ArrayList(notificationDestinations);
}
/**
*
* The Amazon Web Services notification channels that Amazon Managed Grafana can automatically create IAM roles and
* permissions for, to allow Amazon Managed Grafana to use these channels.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setNotificationDestinations(java.util.Collection)} or
* {@link #withNotificationDestinations(java.util.Collection)} if you want to override the existing values.
*
*
* @param notificationDestinations
* The Amazon Web Services notification channels that Amazon Managed Grafana can automatically create IAM
* roles and permissions for, to allow Amazon Managed Grafana to use these channels.
* @return Returns a reference to this object so that method calls can be chained together.
* @see NotificationDestinationType
*/
public WorkspaceDescription withNotificationDestinations(String... notificationDestinations) {
if (this.notificationDestinations == null) {
setNotificationDestinations(new java.util.ArrayList(notificationDestinations.length));
}
for (String ele : notificationDestinations) {
this.notificationDestinations.add(ele);
}
return this;
}
/**
*
* The Amazon Web Services notification channels that Amazon Managed Grafana can automatically create IAM roles and
* permissions for, to allow Amazon Managed Grafana to use these channels.
*
*
* @param notificationDestinations
* The Amazon Web Services notification channels that Amazon Managed Grafana can automatically create IAM
* roles and permissions for, to allow Amazon Managed Grafana to use these channels.
* @return Returns a reference to this object so that method calls can be chained together.
* @see NotificationDestinationType
*/
public WorkspaceDescription withNotificationDestinations(java.util.Collection notificationDestinations) {
setNotificationDestinations(notificationDestinations);
return this;
}
/**
*
* The Amazon Web Services notification channels that Amazon Managed Grafana can automatically create IAM roles and
* permissions for, to allow Amazon Managed Grafana to use these channels.
*
*
* @param notificationDestinations
* The Amazon Web Services notification channels that Amazon Managed Grafana can automatically create IAM
* roles and permissions for, to allow Amazon Managed Grafana to use these channels.
* @return Returns a reference to this object so that method calls can be chained together.
* @see NotificationDestinationType
*/
public WorkspaceDescription withNotificationDestinations(NotificationDestinationType... notificationDestinations) {
java.util.ArrayList notificationDestinationsCopy = new java.util.ArrayList(notificationDestinations.length);
for (NotificationDestinationType value : notificationDestinations) {
notificationDestinationsCopy.add(value.toString());
}
if (getNotificationDestinations() == null) {
setNotificationDestinations(notificationDestinationsCopy);
} else {
getNotificationDestinations().addAll(notificationDestinationsCopy);
}
return this;
}
/**
*
* The name of the IAM role that is used to access resources through Organizations.
*
*
* @param organizationRoleName
* The name of the IAM role that is used to access resources through Organizations.
*/
public void setOrganizationRoleName(String organizationRoleName) {
this.organizationRoleName = organizationRoleName;
}
/**
*
* The name of the IAM role that is used to access resources through Organizations.
*
*
* @return The name of the IAM role that is used to access resources through Organizations.
*/
public String getOrganizationRoleName() {
return this.organizationRoleName;
}
/**
*
* The name of the IAM role that is used to access resources through Organizations.
*
*
* @param organizationRoleName
* The name of the IAM role that is used to access resources through Organizations.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public WorkspaceDescription withOrganizationRoleName(String organizationRoleName) {
setOrganizationRoleName(organizationRoleName);
return this;
}
/**
*
* Specifies the organizational units that this workspace is allowed to use data sources from, if this workspace is
* in an account that is part of an organization.
*
*
* @return Specifies the organizational units that this workspace is allowed to use data sources from, if this
* workspace is in an account that is part of an organization.
*/
public java.util.List getOrganizationalUnits() {
return organizationalUnits;
}
/**
*
* Specifies the organizational units that this workspace is allowed to use data sources from, if this workspace is
* in an account that is part of an organization.
*
*
* @param organizationalUnits
* Specifies the organizational units that this workspace is allowed to use data sources from, if this
* workspace is in an account that is part of an organization.
*/
public void setOrganizationalUnits(java.util.Collection organizationalUnits) {
if (organizationalUnits == null) {
this.organizationalUnits = null;
return;
}
this.organizationalUnits = new java.util.ArrayList(organizationalUnits);
}
/**
*
* Specifies the organizational units that this workspace is allowed to use data sources from, if this workspace is
* in an account that is part of an organization.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setOrganizationalUnits(java.util.Collection)} or {@link #withOrganizationalUnits(java.util.Collection)}
* if you want to override the existing values.
*
*
* @param organizationalUnits
* Specifies the organizational units that this workspace is allowed to use data sources from, if this
* workspace is in an account that is part of an organization.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public WorkspaceDescription withOrganizationalUnits(String... organizationalUnits) {
if (this.organizationalUnits == null) {
setOrganizationalUnits(new java.util.ArrayList(organizationalUnits.length));
}
for (String ele : organizationalUnits) {
this.organizationalUnits.add(ele);
}
return this;
}
/**
*
* Specifies the organizational units that this workspace is allowed to use data sources from, if this workspace is
* in an account that is part of an organization.
*
*
* @param organizationalUnits
* Specifies the organizational units that this workspace is allowed to use data sources from, if this
* workspace is in an account that is part of an organization.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public WorkspaceDescription withOrganizationalUnits(java.util.Collection organizationalUnits) {
setOrganizationalUnits(organizationalUnits);
return this;
}
/**
*
* If this is SERVICE_MANAGED
, and the workplace was created through the Amazon Managed Grafana
* console, then Amazon Managed Grafana automatically creates the IAM roles and provisions the permissions that the
* workspace needs to use Amazon Web Services data sources and notification channels.
*
*
* If this is CUSTOMER_MANAGED
, you must manage those roles and permissions yourself.
*
*
* If you are working with a workspace in a member account of an organization and that account is not a delegated
* administrator account, and you want the workspace to access data sources in other Amazon Web Services accounts in
* the organization, this parameter must be set to CUSTOMER_MANAGED
.
*
*
* For more information about converting between customer and service managed, see Managing
* permissions for data sources and notification channels. For more information about the roles and permissions
* that must be managed for customer managed workspaces, see Amazon Managed Grafana
* permissions and policies for Amazon Web Services data sources and notification channels
*
*
* @param permissionType
* If this is SERVICE_MANAGED
, and the workplace was created through the Amazon Managed Grafana
* console, then Amazon Managed Grafana automatically creates the IAM roles and provisions the permissions
* that the workspace needs to use Amazon Web Services data sources and notification channels.
*
* If this is CUSTOMER_MANAGED
, you must manage those roles and permissions yourself.
*
*
* If you are working with a workspace in a member account of an organization and that account is not a
* delegated administrator account, and you want the workspace to access data sources in other Amazon Web
* Services accounts in the organization, this parameter must be set to CUSTOMER_MANAGED
.
*
*
* For more information about converting between customer and service managed, see Managing
* permissions for data sources and notification channels. For more information about the roles and
* permissions that must be managed for customer managed workspaces, see Amazon Managed
* Grafana permissions and policies for Amazon Web Services data sources and notification channels
* @see PermissionType
*/
public void setPermissionType(String permissionType) {
this.permissionType = permissionType;
}
/**
*
* If this is SERVICE_MANAGED
, and the workplace was created through the Amazon Managed Grafana
* console, then Amazon Managed Grafana automatically creates the IAM roles and provisions the permissions that the
* workspace needs to use Amazon Web Services data sources and notification channels.
*
*
* If this is CUSTOMER_MANAGED
, you must manage those roles and permissions yourself.
*
*
* If you are working with a workspace in a member account of an organization and that account is not a delegated
* administrator account, and you want the workspace to access data sources in other Amazon Web Services accounts in
* the organization, this parameter must be set to CUSTOMER_MANAGED
.
*
*
* For more information about converting between customer and service managed, see Managing
* permissions for data sources and notification channels. For more information about the roles and permissions
* that must be managed for customer managed workspaces, see Amazon Managed Grafana
* permissions and policies for Amazon Web Services data sources and notification channels
*
*
* @return If this is SERVICE_MANAGED
, and the workplace was created through the Amazon Managed Grafana
* console, then Amazon Managed Grafana automatically creates the IAM roles and provisions the permissions
* that the workspace needs to use Amazon Web Services data sources and notification channels.
*
* If this is CUSTOMER_MANAGED
, you must manage those roles and permissions yourself.
*
*
* If you are working with a workspace in a member account of an organization and that account is not a
* delegated administrator account, and you want the workspace to access data sources in other Amazon Web
* Services accounts in the organization, this parameter must be set to CUSTOMER_MANAGED
.
*
*
* For more information about converting between customer and service managed, see Managing
* permissions for data sources and notification channels. For more information about the roles and
* permissions that must be managed for customer managed workspaces, see Amazon Managed
* Grafana permissions and policies for Amazon Web Services data sources and notification channels
* @see PermissionType
*/
public String getPermissionType() {
return this.permissionType;
}
/**
*
* If this is SERVICE_MANAGED
, and the workplace was created through the Amazon Managed Grafana
* console, then Amazon Managed Grafana automatically creates the IAM roles and provisions the permissions that the
* workspace needs to use Amazon Web Services data sources and notification channels.
*
*
* If this is CUSTOMER_MANAGED
, you must manage those roles and permissions yourself.
*
*
* If you are working with a workspace in a member account of an organization and that account is not a delegated
* administrator account, and you want the workspace to access data sources in other Amazon Web Services accounts in
* the organization, this parameter must be set to CUSTOMER_MANAGED
.
*
*
* For more information about converting between customer and service managed, see Managing
* permissions for data sources and notification channels. For more information about the roles and permissions
* that must be managed for customer managed workspaces, see Amazon Managed Grafana
* permissions and policies for Amazon Web Services data sources and notification channels
*
*
* @param permissionType
* If this is SERVICE_MANAGED
, and the workplace was created through the Amazon Managed Grafana
* console, then Amazon Managed Grafana automatically creates the IAM roles and provisions the permissions
* that the workspace needs to use Amazon Web Services data sources and notification channels.
*
* If this is CUSTOMER_MANAGED
, you must manage those roles and permissions yourself.
*
*
* If you are working with a workspace in a member account of an organization and that account is not a
* delegated administrator account, and you want the workspace to access data sources in other Amazon Web
* Services accounts in the organization, this parameter must be set to CUSTOMER_MANAGED
.
*
*
* For more information about converting between customer and service managed, see Managing
* permissions for data sources and notification channels. For more information about the roles and
* permissions that must be managed for customer managed workspaces, see Amazon Managed
* Grafana permissions and policies for Amazon Web Services data sources and notification channels
* @return Returns a reference to this object so that method calls can be chained together.
* @see PermissionType
*/
public WorkspaceDescription withPermissionType(String permissionType) {
setPermissionType(permissionType);
return this;
}
/**
*
* If this is SERVICE_MANAGED
, and the workplace was created through the Amazon Managed Grafana
* console, then Amazon Managed Grafana automatically creates the IAM roles and provisions the permissions that the
* workspace needs to use Amazon Web Services data sources and notification channels.
*
*
* If this is CUSTOMER_MANAGED
, you must manage those roles and permissions yourself.
*
*
* If you are working with a workspace in a member account of an organization and that account is not a delegated
* administrator account, and you want the workspace to access data sources in other Amazon Web Services accounts in
* the organization, this parameter must be set to CUSTOMER_MANAGED
.
*
*
* For more information about converting between customer and service managed, see Managing
* permissions for data sources and notification channels. For more information about the roles and permissions
* that must be managed for customer managed workspaces, see Amazon Managed Grafana
* permissions and policies for Amazon Web Services data sources and notification channels
*
*
* @param permissionType
* If this is SERVICE_MANAGED
, and the workplace was created through the Amazon Managed Grafana
* console, then Amazon Managed Grafana automatically creates the IAM roles and provisions the permissions
* that the workspace needs to use Amazon Web Services data sources and notification channels.
*
* If this is CUSTOMER_MANAGED
, you must manage those roles and permissions yourself.
*
*
* If you are working with a workspace in a member account of an organization and that account is not a
* delegated administrator account, and you want the workspace to access data sources in other Amazon Web
* Services accounts in the organization, this parameter must be set to CUSTOMER_MANAGED
.
*
*
* For more information about converting between customer and service managed, see Managing
* permissions for data sources and notification channels. For more information about the roles and
* permissions that must be managed for customer managed workspaces, see Amazon Managed
* Grafana permissions and policies for Amazon Web Services data sources and notification channels
* @return Returns a reference to this object so that method calls can be chained together.
* @see PermissionType
*/
public WorkspaceDescription withPermissionType(PermissionType permissionType) {
this.permissionType = permissionType.toString();
return this;
}
/**
*
* The name of the CloudFormation stack set that is used to generate IAM roles to be used for this workspace.
*
*
* @param stackSetName
* The name of the CloudFormation stack set that is used to generate IAM roles to be used for this workspace.
*/
public void setStackSetName(String stackSetName) {
this.stackSetName = stackSetName;
}
/**
*
* The name of the CloudFormation stack set that is used to generate IAM roles to be used for this workspace.
*
*
* @return The name of the CloudFormation stack set that is used to generate IAM roles to be used for this
* workspace.
*/
public String getStackSetName() {
return this.stackSetName;
}
/**
*
* The name of the CloudFormation stack set that is used to generate IAM roles to be used for this workspace.
*
*
* @param stackSetName
* The name of the CloudFormation stack set that is used to generate IAM roles to be used for this workspace.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public WorkspaceDescription withStackSetName(String stackSetName) {
setStackSetName(stackSetName);
return this;
}
/**
*
* The current status of the workspace.
*
*
* @param status
* The current status of the workspace.
* @see WorkspaceStatus
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* The current status of the workspace.
*
*
* @return The current status of the workspace.
* @see WorkspaceStatus
*/
public String getStatus() {
return this.status;
}
/**
*
* The current status of the workspace.
*
*
* @param status
* The current status of the workspace.
* @return Returns a reference to this object so that method calls can be chained together.
* @see WorkspaceStatus
*/
public WorkspaceDescription withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* The current status of the workspace.
*
*
* @param status
* The current status of the workspace.
* @return Returns a reference to this object so that method calls can be chained together.
* @see WorkspaceStatus
*/
public WorkspaceDescription withStatus(WorkspaceStatus status) {
this.status = status.toString();
return this;
}
/**
*
* The list of tags associated with the workspace.
*
*
* @return The list of tags associated with the workspace.
*/
public java.util.Map getTags() {
return tags;
}
/**
*
* The list of tags associated with the workspace.
*
*
* @param tags
* The list of tags associated with the workspace.
*/
public void setTags(java.util.Map tags) {
this.tags = tags;
}
/**
*
* The list of tags associated with the workspace.
*
*
* @param tags
* The list of tags associated with the workspace.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public WorkspaceDescription withTags(java.util.Map tags) {
setTags(tags);
return this;
}
/**
* Add a single Tags entry
*
* @see WorkspaceDescription#withTags
* @returns a reference to this object so that method calls can be chained together.
*/
public WorkspaceDescription addTagsEntry(String key, String value) {
if (null == this.tags) {
this.tags = new java.util.HashMap();
}
if (this.tags.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.tags.put(key, value);
return this;
}
/**
* Removes all the entries added into Tags.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public WorkspaceDescription clearTagsEntries() {
this.tags = null;
return this;
}
/**
*
* The configuration for connecting to data sources in a private VPC (Amazon Virtual Private Cloud).
*
*
* @param vpcConfiguration
* The configuration for connecting to data sources in a private VPC (Amazon Virtual Private Cloud).
*/
public void setVpcConfiguration(VpcConfiguration vpcConfiguration) {
this.vpcConfiguration = vpcConfiguration;
}
/**
*
* The configuration for connecting to data sources in a private VPC (Amazon Virtual Private Cloud).
*
*
* @return The configuration for connecting to data sources in a private VPC (Amazon Virtual Private Cloud).
*/
public VpcConfiguration getVpcConfiguration() {
return this.vpcConfiguration;
}
/**
*
* The configuration for connecting to data sources in a private VPC (Amazon Virtual Private Cloud).
*
*
* @param vpcConfiguration
* The configuration for connecting to data sources in a private VPC (Amazon Virtual Private Cloud).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public WorkspaceDescription withVpcConfiguration(VpcConfiguration vpcConfiguration) {
setVpcConfiguration(vpcConfiguration);
return this;
}
/**
*
* The IAM role that grants permissions to the Amazon Web Services resources that the workspace will view data from.
* This role must already exist.
*
*
* @param workspaceRoleArn
* The IAM role that grants permissions to the Amazon Web Services resources that the workspace will view
* data from. This role must already exist.
*/
public void setWorkspaceRoleArn(String workspaceRoleArn) {
this.workspaceRoleArn = workspaceRoleArn;
}
/**
*
* The IAM role that grants permissions to the Amazon Web Services resources that the workspace will view data from.
* This role must already exist.
*
*
* @return The IAM role that grants permissions to the Amazon Web Services resources that the workspace will view
* data from. This role must already exist.
*/
public String getWorkspaceRoleArn() {
return this.workspaceRoleArn;
}
/**
*
* The IAM role that grants permissions to the Amazon Web Services resources that the workspace will view data from.
* This role must already exist.
*
*
* @param workspaceRoleArn
* The IAM role that grants permissions to the Amazon Web Services resources that the workspace will view
* data from. This role must already exist.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public WorkspaceDescription withWorkspaceRoleArn(String workspaceRoleArn) {
setWorkspaceRoleArn(workspaceRoleArn);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getAccountAccessType() != null)
sb.append("AccountAccessType: ").append(getAccountAccessType()).append(",");
if (getAuthentication() != null)
sb.append("Authentication: ").append(getAuthentication()).append(",");
if (getCreated() != null)
sb.append("Created: ").append(getCreated()).append(",");
if (getDataSources() != null)
sb.append("DataSources: ").append(getDataSources()).append(",");
if (getDescription() != null)
sb.append("Description: ").append("***Sensitive Data Redacted***").append(",");
if (getEndpoint() != null)
sb.append("Endpoint: ").append(getEndpoint()).append(",");
if (getFreeTrialConsumed() != null)
sb.append("FreeTrialConsumed: ").append(getFreeTrialConsumed()).append(",");
if (getFreeTrialExpiration() != null)
sb.append("FreeTrialExpiration: ").append(getFreeTrialExpiration()).append(",");
if (getGrafanaVersion() != null)
sb.append("GrafanaVersion: ").append(getGrafanaVersion()).append(",");
if (getId() != null)
sb.append("Id: ").append(getId()).append(",");
if (getLicenseExpiration() != null)
sb.append("LicenseExpiration: ").append(getLicenseExpiration()).append(",");
if (getLicenseType() != null)
sb.append("LicenseType: ").append(getLicenseType()).append(",");
if (getModified() != null)
sb.append("Modified: ").append(getModified()).append(",");
if (getName() != null)
sb.append("Name: ").append("***Sensitive Data Redacted***").append(",");
if (getNetworkAccessControl() != null)
sb.append("NetworkAccessControl: ").append(getNetworkAccessControl()).append(",");
if (getNotificationDestinations() != null)
sb.append("NotificationDestinations: ").append(getNotificationDestinations()).append(",");
if (getOrganizationRoleName() != null)
sb.append("OrganizationRoleName: ").append("***Sensitive Data Redacted***").append(",");
if (getOrganizationalUnits() != null)
sb.append("OrganizationalUnits: ").append("***Sensitive Data Redacted***").append(",");
if (getPermissionType() != null)
sb.append("PermissionType: ").append(getPermissionType()).append(",");
if (getStackSetName() != null)
sb.append("StackSetName: ").append(getStackSetName()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags()).append(",");
if (getVpcConfiguration() != null)
sb.append("VpcConfiguration: ").append(getVpcConfiguration()).append(",");
if (getWorkspaceRoleArn() != null)
sb.append("WorkspaceRoleArn: ").append("***Sensitive Data Redacted***");
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof WorkspaceDescription == false)
return false;
WorkspaceDescription other = (WorkspaceDescription) obj;
if (other.getAccountAccessType() == null ^ this.getAccountAccessType() == null)
return false;
if (other.getAccountAccessType() != null && other.getAccountAccessType().equals(this.getAccountAccessType()) == false)
return false;
if (other.getAuthentication() == null ^ this.getAuthentication() == null)
return false;
if (other.getAuthentication() != null && other.getAuthentication().equals(this.getAuthentication()) == false)
return false;
if (other.getCreated() == null ^ this.getCreated() == null)
return false;
if (other.getCreated() != null && other.getCreated().equals(this.getCreated()) == false)
return false;
if (other.getDataSources() == null ^ this.getDataSources() == null)
return false;
if (other.getDataSources() != null && other.getDataSources().equals(this.getDataSources()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getEndpoint() == null ^ this.getEndpoint() == null)
return false;
if (other.getEndpoint() != null && other.getEndpoint().equals(this.getEndpoint()) == false)
return false;
if (other.getFreeTrialConsumed() == null ^ this.getFreeTrialConsumed() == null)
return false;
if (other.getFreeTrialConsumed() != null && other.getFreeTrialConsumed().equals(this.getFreeTrialConsumed()) == false)
return false;
if (other.getFreeTrialExpiration() == null ^ this.getFreeTrialExpiration() == null)
return false;
if (other.getFreeTrialExpiration() != null && other.getFreeTrialExpiration().equals(this.getFreeTrialExpiration()) == false)
return false;
if (other.getGrafanaVersion() == null ^ this.getGrafanaVersion() == null)
return false;
if (other.getGrafanaVersion() != null && other.getGrafanaVersion().equals(this.getGrafanaVersion()) == false)
return false;
if (other.getId() == null ^ this.getId() == null)
return false;
if (other.getId() != null && other.getId().equals(this.getId()) == false)
return false;
if (other.getLicenseExpiration() == null ^ this.getLicenseExpiration() == null)
return false;
if (other.getLicenseExpiration() != null && other.getLicenseExpiration().equals(this.getLicenseExpiration()) == false)
return false;
if (other.getLicenseType() == null ^ this.getLicenseType() == null)
return false;
if (other.getLicenseType() != null && other.getLicenseType().equals(this.getLicenseType()) == false)
return false;
if (other.getModified() == null ^ this.getModified() == null)
return false;
if (other.getModified() != null && other.getModified().equals(this.getModified()) == false)
return false;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getNetworkAccessControl() == null ^ this.getNetworkAccessControl() == null)
return false;
if (other.getNetworkAccessControl() != null && other.getNetworkAccessControl().equals(this.getNetworkAccessControl()) == false)
return false;
if (other.getNotificationDestinations() == null ^ this.getNotificationDestinations() == null)
return false;
if (other.getNotificationDestinations() != null && other.getNotificationDestinations().equals(this.getNotificationDestinations()) == false)
return false;
if (other.getOrganizationRoleName() == null ^ this.getOrganizationRoleName() == null)
return false;
if (other.getOrganizationRoleName() != null && other.getOrganizationRoleName().equals(this.getOrganizationRoleName()) == false)
return false;
if (other.getOrganizationalUnits() == null ^ this.getOrganizationalUnits() == null)
return false;
if (other.getOrganizationalUnits() != null && other.getOrganizationalUnits().equals(this.getOrganizationalUnits()) == false)
return false;
if (other.getPermissionType() == null ^ this.getPermissionType() == null)
return false;
if (other.getPermissionType() != null && other.getPermissionType().equals(this.getPermissionType()) == false)
return false;
if (other.getStackSetName() == null ^ this.getStackSetName() == null)
return false;
if (other.getStackSetName() != null && other.getStackSetName().equals(this.getStackSetName()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
if (other.getVpcConfiguration() == null ^ this.getVpcConfiguration() == null)
return false;
if (other.getVpcConfiguration() != null && other.getVpcConfiguration().equals(this.getVpcConfiguration()) == false)
return false;
if (other.getWorkspaceRoleArn() == null ^ this.getWorkspaceRoleArn() == null)
return false;
if (other.getWorkspaceRoleArn() != null && other.getWorkspaceRoleArn().equals(this.getWorkspaceRoleArn()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getAccountAccessType() == null) ? 0 : getAccountAccessType().hashCode());
hashCode = prime * hashCode + ((getAuthentication() == null) ? 0 : getAuthentication().hashCode());
hashCode = prime * hashCode + ((getCreated() == null) ? 0 : getCreated().hashCode());
hashCode = prime * hashCode + ((getDataSources() == null) ? 0 : getDataSources().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getEndpoint() == null) ? 0 : getEndpoint().hashCode());
hashCode = prime * hashCode + ((getFreeTrialConsumed() == null) ? 0 : getFreeTrialConsumed().hashCode());
hashCode = prime * hashCode + ((getFreeTrialExpiration() == null) ? 0 : getFreeTrialExpiration().hashCode());
hashCode = prime * hashCode + ((getGrafanaVersion() == null) ? 0 : getGrafanaVersion().hashCode());
hashCode = prime * hashCode + ((getId() == null) ? 0 : getId().hashCode());
hashCode = prime * hashCode + ((getLicenseExpiration() == null) ? 0 : getLicenseExpiration().hashCode());
hashCode = prime * hashCode + ((getLicenseType() == null) ? 0 : getLicenseType().hashCode());
hashCode = prime * hashCode + ((getModified() == null) ? 0 : getModified().hashCode());
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getNetworkAccessControl() == null) ? 0 : getNetworkAccessControl().hashCode());
hashCode = prime * hashCode + ((getNotificationDestinations() == null) ? 0 : getNotificationDestinations().hashCode());
hashCode = prime * hashCode + ((getOrganizationRoleName() == null) ? 0 : getOrganizationRoleName().hashCode());
hashCode = prime * hashCode + ((getOrganizationalUnits() == null) ? 0 : getOrganizationalUnits().hashCode());
hashCode = prime * hashCode + ((getPermissionType() == null) ? 0 : getPermissionType().hashCode());
hashCode = prime * hashCode + ((getStackSetName() == null) ? 0 : getStackSetName().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
hashCode = prime * hashCode + ((getVpcConfiguration() == null) ? 0 : getVpcConfiguration().hashCode());
hashCode = prime * hashCode + ((getWorkspaceRoleArn() == null) ? 0 : getWorkspaceRoleArn().hashCode());
return hashCode;
}
@Override
public WorkspaceDescription clone() {
try {
return (WorkspaceDescription) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.managedgrafana.model.transform.WorkspaceDescriptionMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}