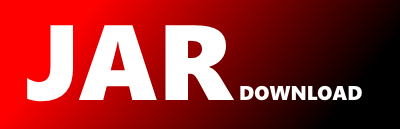
com.amazonaws.services.marketplaceagreement.model.DescribeAgreementResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-marketplaceagreement Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.marketplaceagreement.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class DescribeAgreementResult extends com.amazonaws.AmazonWebServiceResult implements Serializable, Cloneable {
/**
*
* The date and time the offer was accepted or the agreement was created.
*
*
*
* AcceptanceTime
and StartTime
can differ for future dated agreements (FDAs).
*
*
*/
private java.util.Date acceptanceTime;
/**
*
* The details of the party accepting the agreement terms. This is commonly the buyer for
* PurchaseAgreement
.
*
*/
private Acceptor acceptor;
/**
*
* The unique identifier of the agreement.
*
*/
private String agreementId;
/**
*
* The type of agreement. Values are PurchaseAgreement
or VendorInsightsAgreement
.
*
*/
private String agreementType;
/**
*
* The date and time when the agreement ends. The field is null
for pay-as-you-go agreements, which
* don’t have end dates.
*
*/
private java.util.Date endTime;
/**
*
* The estimated cost of the agreement.
*
*/
private EstimatedCharges estimatedCharges;
/**
*
* A summary of the proposal received from the proposer.
*
*/
private ProposalSummary proposalSummary;
/**
*
* The details of the party proposing the agreement terms. This is commonly the seller for
* PurchaseAgreement
.
*
*/
private Proposer proposer;
/**
*
* The date and time when the agreement starts.
*
*/
private java.util.Date startTime;
/**
*
* The current status of the agreement.
*
*
* Statuses include:
*
*
* -
*
* ACTIVE
– The terms of the agreement are active.
*
*
* -
*
* ARCHIVED
– The agreement ended without a specified reason.
*
*
* -
*
* CANCELLED
– The acceptor ended the agreement before the defined end date.
*
*
* -
*
* EXPIRED
– The agreement ended on the defined end date.
*
*
* -
*
* RENEWED
– The agreement was renewed into a new agreement (for example, an auto-renewal).
*
*
* -
*
* REPLACED
– The agreement was replaced using an agreement replacement offer.
*
*
* -
*
* ROLLED_BACK
(Only applicable to inactive agreement revisions) – The agreement revision has been
* rolled back because of an error. An earlier revision is now active.
*
*
* -
*
* SUPERCEDED
(Only applicable to inactive agreement revisions) – The agreement revision is no longer
* active and another agreement revision is now active.
*
*
* -
*
* TERMINATED
– The agreement ended before the defined end date because of an AWS termination (for
* example, a payment failure).
*
*
*
*/
private String status;
/**
*
* The date and time the offer was accepted or the agreement was created.
*
*
*
* AcceptanceTime
and StartTime
can differ for future dated agreements (FDAs).
*
*
*
* @param acceptanceTime
* The date and time the offer was accepted or the agreement was created.
*
* AcceptanceTime
and StartTime
can differ for future dated agreements (FDAs).
*
*/
public void setAcceptanceTime(java.util.Date acceptanceTime) {
this.acceptanceTime = acceptanceTime;
}
/**
*
* The date and time the offer was accepted or the agreement was created.
*
*
*
* AcceptanceTime
and StartTime
can differ for future dated agreements (FDAs).
*
*
*
* @return The date and time the offer was accepted or the agreement was created.
*
* AcceptanceTime
and StartTime
can differ for future dated agreements (FDAs).
*
*/
public java.util.Date getAcceptanceTime() {
return this.acceptanceTime;
}
/**
*
* The date and time the offer was accepted or the agreement was created.
*
*
*
* AcceptanceTime
and StartTime
can differ for future dated agreements (FDAs).
*
*
*
* @param acceptanceTime
* The date and time the offer was accepted or the agreement was created.
*
* AcceptanceTime
and StartTime
can differ for future dated agreements (FDAs).
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeAgreementResult withAcceptanceTime(java.util.Date acceptanceTime) {
setAcceptanceTime(acceptanceTime);
return this;
}
/**
*
* The details of the party accepting the agreement terms. This is commonly the buyer for
* PurchaseAgreement
.
*
*
* @param acceptor
* The details of the party accepting the agreement terms. This is commonly the buyer for
* PurchaseAgreement
.
*/
public void setAcceptor(Acceptor acceptor) {
this.acceptor = acceptor;
}
/**
*
* The details of the party accepting the agreement terms. This is commonly the buyer for
* PurchaseAgreement
.
*
*
* @return The details of the party accepting the agreement terms. This is commonly the buyer for
* PurchaseAgreement
.
*/
public Acceptor getAcceptor() {
return this.acceptor;
}
/**
*
* The details of the party accepting the agreement terms. This is commonly the buyer for
* PurchaseAgreement
.
*
*
* @param acceptor
* The details of the party accepting the agreement terms. This is commonly the buyer for
* PurchaseAgreement
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeAgreementResult withAcceptor(Acceptor acceptor) {
setAcceptor(acceptor);
return this;
}
/**
*
* The unique identifier of the agreement.
*
*
* @param agreementId
* The unique identifier of the agreement.
*/
public void setAgreementId(String agreementId) {
this.agreementId = agreementId;
}
/**
*
* The unique identifier of the agreement.
*
*
* @return The unique identifier of the agreement.
*/
public String getAgreementId() {
return this.agreementId;
}
/**
*
* The unique identifier of the agreement.
*
*
* @param agreementId
* The unique identifier of the agreement.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeAgreementResult withAgreementId(String agreementId) {
setAgreementId(agreementId);
return this;
}
/**
*
* The type of agreement. Values are PurchaseAgreement
or VendorInsightsAgreement
.
*
*
* @param agreementType
* The type of agreement. Values are PurchaseAgreement
or VendorInsightsAgreement
.
*/
public void setAgreementType(String agreementType) {
this.agreementType = agreementType;
}
/**
*
* The type of agreement. Values are PurchaseAgreement
or VendorInsightsAgreement
.
*
*
* @return The type of agreement. Values are PurchaseAgreement
or VendorInsightsAgreement
.
*/
public String getAgreementType() {
return this.agreementType;
}
/**
*
* The type of agreement. Values are PurchaseAgreement
or VendorInsightsAgreement
.
*
*
* @param agreementType
* The type of agreement. Values are PurchaseAgreement
or VendorInsightsAgreement
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeAgreementResult withAgreementType(String agreementType) {
setAgreementType(agreementType);
return this;
}
/**
*
* The date and time when the agreement ends. The field is null
for pay-as-you-go agreements, which
* don’t have end dates.
*
*
* @param endTime
* The date and time when the agreement ends. The field is null
for pay-as-you-go agreements,
* which don’t have end dates.
*/
public void setEndTime(java.util.Date endTime) {
this.endTime = endTime;
}
/**
*
* The date and time when the agreement ends. The field is null
for pay-as-you-go agreements, which
* don’t have end dates.
*
*
* @return The date and time when the agreement ends. The field is null
for pay-as-you-go agreements,
* which don’t have end dates.
*/
public java.util.Date getEndTime() {
return this.endTime;
}
/**
*
* The date and time when the agreement ends. The field is null
for pay-as-you-go agreements, which
* don’t have end dates.
*
*
* @param endTime
* The date and time when the agreement ends. The field is null
for pay-as-you-go agreements,
* which don’t have end dates.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeAgreementResult withEndTime(java.util.Date endTime) {
setEndTime(endTime);
return this;
}
/**
*
* The estimated cost of the agreement.
*
*
* @param estimatedCharges
* The estimated cost of the agreement.
*/
public void setEstimatedCharges(EstimatedCharges estimatedCharges) {
this.estimatedCharges = estimatedCharges;
}
/**
*
* The estimated cost of the agreement.
*
*
* @return The estimated cost of the agreement.
*/
public EstimatedCharges getEstimatedCharges() {
return this.estimatedCharges;
}
/**
*
* The estimated cost of the agreement.
*
*
* @param estimatedCharges
* The estimated cost of the agreement.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeAgreementResult withEstimatedCharges(EstimatedCharges estimatedCharges) {
setEstimatedCharges(estimatedCharges);
return this;
}
/**
*
* A summary of the proposal received from the proposer.
*
*
* @param proposalSummary
* A summary of the proposal received from the proposer.
*/
public void setProposalSummary(ProposalSummary proposalSummary) {
this.proposalSummary = proposalSummary;
}
/**
*
* A summary of the proposal received from the proposer.
*
*
* @return A summary of the proposal received from the proposer.
*/
public ProposalSummary getProposalSummary() {
return this.proposalSummary;
}
/**
*
* A summary of the proposal received from the proposer.
*
*
* @param proposalSummary
* A summary of the proposal received from the proposer.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeAgreementResult withProposalSummary(ProposalSummary proposalSummary) {
setProposalSummary(proposalSummary);
return this;
}
/**
*
* The details of the party proposing the agreement terms. This is commonly the seller for
* PurchaseAgreement
.
*
*
* @param proposer
* The details of the party proposing the agreement terms. This is commonly the seller for
* PurchaseAgreement
.
*/
public void setProposer(Proposer proposer) {
this.proposer = proposer;
}
/**
*
* The details of the party proposing the agreement terms. This is commonly the seller for
* PurchaseAgreement
.
*
*
* @return The details of the party proposing the agreement terms. This is commonly the seller for
* PurchaseAgreement
.
*/
public Proposer getProposer() {
return this.proposer;
}
/**
*
* The details of the party proposing the agreement terms. This is commonly the seller for
* PurchaseAgreement
.
*
*
* @param proposer
* The details of the party proposing the agreement terms. This is commonly the seller for
* PurchaseAgreement
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeAgreementResult withProposer(Proposer proposer) {
setProposer(proposer);
return this;
}
/**
*
* The date and time when the agreement starts.
*
*
* @param startTime
* The date and time when the agreement starts.
*/
public void setStartTime(java.util.Date startTime) {
this.startTime = startTime;
}
/**
*
* The date and time when the agreement starts.
*
*
* @return The date and time when the agreement starts.
*/
public java.util.Date getStartTime() {
return this.startTime;
}
/**
*
* The date and time when the agreement starts.
*
*
* @param startTime
* The date and time when the agreement starts.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeAgreementResult withStartTime(java.util.Date startTime) {
setStartTime(startTime);
return this;
}
/**
*
* The current status of the agreement.
*
*
* Statuses include:
*
*
* -
*
* ACTIVE
– The terms of the agreement are active.
*
*
* -
*
* ARCHIVED
– The agreement ended without a specified reason.
*
*
* -
*
* CANCELLED
– The acceptor ended the agreement before the defined end date.
*
*
* -
*
* EXPIRED
– The agreement ended on the defined end date.
*
*
* -
*
* RENEWED
– The agreement was renewed into a new agreement (for example, an auto-renewal).
*
*
* -
*
* REPLACED
– The agreement was replaced using an agreement replacement offer.
*
*
* -
*
* ROLLED_BACK
(Only applicable to inactive agreement revisions) – The agreement revision has been
* rolled back because of an error. An earlier revision is now active.
*
*
* -
*
* SUPERCEDED
(Only applicable to inactive agreement revisions) – The agreement revision is no longer
* active and another agreement revision is now active.
*
*
* -
*
* TERMINATED
– The agreement ended before the defined end date because of an AWS termination (for
* example, a payment failure).
*
*
*
*
* @param status
* The current status of the agreement.
*
* Statuses include:
*
*
* -
*
* ACTIVE
– The terms of the agreement are active.
*
*
* -
*
* ARCHIVED
– The agreement ended without a specified reason.
*
*
* -
*
* CANCELLED
– The acceptor ended the agreement before the defined end date.
*
*
* -
*
* EXPIRED
– The agreement ended on the defined end date.
*
*
* -
*
* RENEWED
– The agreement was renewed into a new agreement (for example, an auto-renewal).
*
*
* -
*
* REPLACED
– The agreement was replaced using an agreement replacement offer.
*
*
* -
*
* ROLLED_BACK
(Only applicable to inactive agreement revisions) – The agreement revision has
* been rolled back because of an error. An earlier revision is now active.
*
*
* -
*
* SUPERCEDED
(Only applicable to inactive agreement revisions) – The agreement revision is no
* longer active and another agreement revision is now active.
*
*
* -
*
* TERMINATED
– The agreement ended before the defined end date because of an AWS termination
* (for example, a payment failure).
*
*
* @see AgreementStatus
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* The current status of the agreement.
*
*
* Statuses include:
*
*
* -
*
* ACTIVE
– The terms of the agreement are active.
*
*
* -
*
* ARCHIVED
– The agreement ended without a specified reason.
*
*
* -
*
* CANCELLED
– The acceptor ended the agreement before the defined end date.
*
*
* -
*
* EXPIRED
– The agreement ended on the defined end date.
*
*
* -
*
* RENEWED
– The agreement was renewed into a new agreement (for example, an auto-renewal).
*
*
* -
*
* REPLACED
– The agreement was replaced using an agreement replacement offer.
*
*
* -
*
* ROLLED_BACK
(Only applicable to inactive agreement revisions) – The agreement revision has been
* rolled back because of an error. An earlier revision is now active.
*
*
* -
*
* SUPERCEDED
(Only applicable to inactive agreement revisions) – The agreement revision is no longer
* active and another agreement revision is now active.
*
*
* -
*
* TERMINATED
– The agreement ended before the defined end date because of an AWS termination (for
* example, a payment failure).
*
*
*
*
* @return The current status of the agreement.
*
* Statuses include:
*
*
* -
*
* ACTIVE
– The terms of the agreement are active.
*
*
* -
*
* ARCHIVED
– The agreement ended without a specified reason.
*
*
* -
*
* CANCELLED
– The acceptor ended the agreement before the defined end date.
*
*
* -
*
* EXPIRED
– The agreement ended on the defined end date.
*
*
* -
*
* RENEWED
– The agreement was renewed into a new agreement (for example, an auto-renewal).
*
*
* -
*
* REPLACED
– The agreement was replaced using an agreement replacement offer.
*
*
* -
*
* ROLLED_BACK
(Only applicable to inactive agreement revisions) – The agreement revision has
* been rolled back because of an error. An earlier revision is now active.
*
*
* -
*
* SUPERCEDED
(Only applicable to inactive agreement revisions) – The agreement revision is no
* longer active and another agreement revision is now active.
*
*
* -
*
* TERMINATED
– The agreement ended before the defined end date because of an AWS termination
* (for example, a payment failure).
*
*
* @see AgreementStatus
*/
public String getStatus() {
return this.status;
}
/**
*
* The current status of the agreement.
*
*
* Statuses include:
*
*
* -
*
* ACTIVE
– The terms of the agreement are active.
*
*
* -
*
* ARCHIVED
– The agreement ended without a specified reason.
*
*
* -
*
* CANCELLED
– The acceptor ended the agreement before the defined end date.
*
*
* -
*
* EXPIRED
– The agreement ended on the defined end date.
*
*
* -
*
* RENEWED
– The agreement was renewed into a new agreement (for example, an auto-renewal).
*
*
* -
*
* REPLACED
– The agreement was replaced using an agreement replacement offer.
*
*
* -
*
* ROLLED_BACK
(Only applicable to inactive agreement revisions) – The agreement revision has been
* rolled back because of an error. An earlier revision is now active.
*
*
* -
*
* SUPERCEDED
(Only applicable to inactive agreement revisions) – The agreement revision is no longer
* active and another agreement revision is now active.
*
*
* -
*
* TERMINATED
– The agreement ended before the defined end date because of an AWS termination (for
* example, a payment failure).
*
*
*
*
* @param status
* The current status of the agreement.
*
* Statuses include:
*
*
* -
*
* ACTIVE
– The terms of the agreement are active.
*
*
* -
*
* ARCHIVED
– The agreement ended without a specified reason.
*
*
* -
*
* CANCELLED
– The acceptor ended the agreement before the defined end date.
*
*
* -
*
* EXPIRED
– The agreement ended on the defined end date.
*
*
* -
*
* RENEWED
– The agreement was renewed into a new agreement (for example, an auto-renewal).
*
*
* -
*
* REPLACED
– The agreement was replaced using an agreement replacement offer.
*
*
* -
*
* ROLLED_BACK
(Only applicable to inactive agreement revisions) – The agreement revision has
* been rolled back because of an error. An earlier revision is now active.
*
*
* -
*
* SUPERCEDED
(Only applicable to inactive agreement revisions) – The agreement revision is no
* longer active and another agreement revision is now active.
*
*
* -
*
* TERMINATED
– The agreement ended before the defined end date because of an AWS termination
* (for example, a payment failure).
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see AgreementStatus
*/
public DescribeAgreementResult withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* The current status of the agreement.
*
*
* Statuses include:
*
*
* -
*
* ACTIVE
– The terms of the agreement are active.
*
*
* -
*
* ARCHIVED
– The agreement ended without a specified reason.
*
*
* -
*
* CANCELLED
– The acceptor ended the agreement before the defined end date.
*
*
* -
*
* EXPIRED
– The agreement ended on the defined end date.
*
*
* -
*
* RENEWED
– The agreement was renewed into a new agreement (for example, an auto-renewal).
*
*
* -
*
* REPLACED
– The agreement was replaced using an agreement replacement offer.
*
*
* -
*
* ROLLED_BACK
(Only applicable to inactive agreement revisions) – The agreement revision has been
* rolled back because of an error. An earlier revision is now active.
*
*
* -
*
* SUPERCEDED
(Only applicable to inactive agreement revisions) – The agreement revision is no longer
* active and another agreement revision is now active.
*
*
* -
*
* TERMINATED
– The agreement ended before the defined end date because of an AWS termination (for
* example, a payment failure).
*
*
*
*
* @param status
* The current status of the agreement.
*
* Statuses include:
*
*
* -
*
* ACTIVE
– The terms of the agreement are active.
*
*
* -
*
* ARCHIVED
– The agreement ended without a specified reason.
*
*
* -
*
* CANCELLED
– The acceptor ended the agreement before the defined end date.
*
*
* -
*
* EXPIRED
– The agreement ended on the defined end date.
*
*
* -
*
* RENEWED
– The agreement was renewed into a new agreement (for example, an auto-renewal).
*
*
* -
*
* REPLACED
– The agreement was replaced using an agreement replacement offer.
*
*
* -
*
* ROLLED_BACK
(Only applicable to inactive agreement revisions) – The agreement revision has
* been rolled back because of an error. An earlier revision is now active.
*
*
* -
*
* SUPERCEDED
(Only applicable to inactive agreement revisions) – The agreement revision is no
* longer active and another agreement revision is now active.
*
*
* -
*
* TERMINATED
– The agreement ended before the defined end date because of an AWS termination
* (for example, a payment failure).
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see AgreementStatus
*/
public DescribeAgreementResult withStatus(AgreementStatus status) {
this.status = status.toString();
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getAcceptanceTime() != null)
sb.append("AcceptanceTime: ").append(getAcceptanceTime()).append(",");
if (getAcceptor() != null)
sb.append("Acceptor: ").append(getAcceptor()).append(",");
if (getAgreementId() != null)
sb.append("AgreementId: ").append(getAgreementId()).append(",");
if (getAgreementType() != null)
sb.append("AgreementType: ").append(getAgreementType()).append(",");
if (getEndTime() != null)
sb.append("EndTime: ").append(getEndTime()).append(",");
if (getEstimatedCharges() != null)
sb.append("EstimatedCharges: ").append(getEstimatedCharges()).append(",");
if (getProposalSummary() != null)
sb.append("ProposalSummary: ").append(getProposalSummary()).append(",");
if (getProposer() != null)
sb.append("Proposer: ").append(getProposer()).append(",");
if (getStartTime() != null)
sb.append("StartTime: ").append(getStartTime()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof DescribeAgreementResult == false)
return false;
DescribeAgreementResult other = (DescribeAgreementResult) obj;
if (other.getAcceptanceTime() == null ^ this.getAcceptanceTime() == null)
return false;
if (other.getAcceptanceTime() != null && other.getAcceptanceTime().equals(this.getAcceptanceTime()) == false)
return false;
if (other.getAcceptor() == null ^ this.getAcceptor() == null)
return false;
if (other.getAcceptor() != null && other.getAcceptor().equals(this.getAcceptor()) == false)
return false;
if (other.getAgreementId() == null ^ this.getAgreementId() == null)
return false;
if (other.getAgreementId() != null && other.getAgreementId().equals(this.getAgreementId()) == false)
return false;
if (other.getAgreementType() == null ^ this.getAgreementType() == null)
return false;
if (other.getAgreementType() != null && other.getAgreementType().equals(this.getAgreementType()) == false)
return false;
if (other.getEndTime() == null ^ this.getEndTime() == null)
return false;
if (other.getEndTime() != null && other.getEndTime().equals(this.getEndTime()) == false)
return false;
if (other.getEstimatedCharges() == null ^ this.getEstimatedCharges() == null)
return false;
if (other.getEstimatedCharges() != null && other.getEstimatedCharges().equals(this.getEstimatedCharges()) == false)
return false;
if (other.getProposalSummary() == null ^ this.getProposalSummary() == null)
return false;
if (other.getProposalSummary() != null && other.getProposalSummary().equals(this.getProposalSummary()) == false)
return false;
if (other.getProposer() == null ^ this.getProposer() == null)
return false;
if (other.getProposer() != null && other.getProposer().equals(this.getProposer()) == false)
return false;
if (other.getStartTime() == null ^ this.getStartTime() == null)
return false;
if (other.getStartTime() != null && other.getStartTime().equals(this.getStartTime()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getAcceptanceTime() == null) ? 0 : getAcceptanceTime().hashCode());
hashCode = prime * hashCode + ((getAcceptor() == null) ? 0 : getAcceptor().hashCode());
hashCode = prime * hashCode + ((getAgreementId() == null) ? 0 : getAgreementId().hashCode());
hashCode = prime * hashCode + ((getAgreementType() == null) ? 0 : getAgreementType().hashCode());
hashCode = prime * hashCode + ((getEndTime() == null) ? 0 : getEndTime().hashCode());
hashCode = prime * hashCode + ((getEstimatedCharges() == null) ? 0 : getEstimatedCharges().hashCode());
hashCode = prime * hashCode + ((getProposalSummary() == null) ? 0 : getProposalSummary().hashCode());
hashCode = prime * hashCode + ((getProposer() == null) ? 0 : getProposer().hashCode());
hashCode = prime * hashCode + ((getStartTime() == null) ? 0 : getStartTime().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
return hashCode;
}
@Override
public DescribeAgreementResult clone() {
try {
return (DescribeAgreementResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}