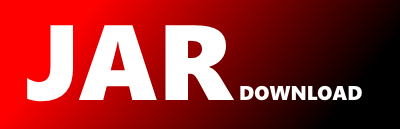
com.amazonaws.services.marketplacemetering.model.MeterUsageRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-marketplacemeteringservice Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.marketplacemetering.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class MeterUsageRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* Product code is used to uniquely identify a product in AWS Marketplace. The product code should be the same as
* the one used during the publishing of a new product.
*
*/
private String productCode;
/**
*
* Timestamp, in UTC, for which the usage is being reported. Your application can meter usage for up to one hour in
* the past. Make sure the timestamp
value is not before the start of the software usage.
*
*/
private java.util.Date timestamp;
/**
*
* It will be one of the fcp dimension name provided during the publishing of the product.
*
*/
private String usageDimension;
/**
*
* Consumption value for the hour. Defaults to 0
if not specified.
*
*/
private Integer usageQuantity;
/**
*
* Checks whether you have the permissions required for the action, but does not make the request. If you have the
* permissions, the request returns DryRunOperation
; otherwise, it returns
* UnauthorizedException
. Defaults to false
if not specified.
*
*/
private Boolean dryRun;
/**
*
* The set of UsageAllocations
to submit.
*
*
* The sum of all UsageAllocation
quantities must equal the UsageQuantity
of the
* MeterUsage
request, and each UsageAllocation
must have a unique set of tags (include no
* tags).
*
*/
private java.util.List usageAllocations;
/**
*
* Product code is used to uniquely identify a product in AWS Marketplace. The product code should be the same as
* the one used during the publishing of a new product.
*
*
* @param productCode
* Product code is used to uniquely identify a product in AWS Marketplace. The product code should be the
* same as the one used during the publishing of a new product.
*/
public void setProductCode(String productCode) {
this.productCode = productCode;
}
/**
*
* Product code is used to uniquely identify a product in AWS Marketplace. The product code should be the same as
* the one used during the publishing of a new product.
*
*
* @return Product code is used to uniquely identify a product in AWS Marketplace. The product code should be the
* same as the one used during the publishing of a new product.
*/
public String getProductCode() {
return this.productCode;
}
/**
*
* Product code is used to uniquely identify a product in AWS Marketplace. The product code should be the same as
* the one used during the publishing of a new product.
*
*
* @param productCode
* Product code is used to uniquely identify a product in AWS Marketplace. The product code should be the
* same as the one used during the publishing of a new product.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MeterUsageRequest withProductCode(String productCode) {
setProductCode(productCode);
return this;
}
/**
*
* Timestamp, in UTC, for which the usage is being reported. Your application can meter usage for up to one hour in
* the past. Make sure the timestamp
value is not before the start of the software usage.
*
*
* @param timestamp
* Timestamp, in UTC, for which the usage is being reported. Your application can meter usage for up to one
* hour in the past. Make sure the timestamp
value is not before the start of the software
* usage.
*/
public void setTimestamp(java.util.Date timestamp) {
this.timestamp = timestamp;
}
/**
*
* Timestamp, in UTC, for which the usage is being reported. Your application can meter usage for up to one hour in
* the past. Make sure the timestamp
value is not before the start of the software usage.
*
*
* @return Timestamp, in UTC, for which the usage is being reported. Your application can meter usage for up to one
* hour in the past. Make sure the timestamp
value is not before the start of the software
* usage.
*/
public java.util.Date getTimestamp() {
return this.timestamp;
}
/**
*
* Timestamp, in UTC, for which the usage is being reported. Your application can meter usage for up to one hour in
* the past. Make sure the timestamp
value is not before the start of the software usage.
*
*
* @param timestamp
* Timestamp, in UTC, for which the usage is being reported. Your application can meter usage for up to one
* hour in the past. Make sure the timestamp
value is not before the start of the software
* usage.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MeterUsageRequest withTimestamp(java.util.Date timestamp) {
setTimestamp(timestamp);
return this;
}
/**
*
* It will be one of the fcp dimension name provided during the publishing of the product.
*
*
* @param usageDimension
* It will be one of the fcp dimension name provided during the publishing of the product.
*/
public void setUsageDimension(String usageDimension) {
this.usageDimension = usageDimension;
}
/**
*
* It will be one of the fcp dimension name provided during the publishing of the product.
*
*
* @return It will be one of the fcp dimension name provided during the publishing of the product.
*/
public String getUsageDimension() {
return this.usageDimension;
}
/**
*
* It will be one of the fcp dimension name provided during the publishing of the product.
*
*
* @param usageDimension
* It will be one of the fcp dimension name provided during the publishing of the product.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MeterUsageRequest withUsageDimension(String usageDimension) {
setUsageDimension(usageDimension);
return this;
}
/**
*
* Consumption value for the hour. Defaults to 0
if not specified.
*
*
* @param usageQuantity
* Consumption value for the hour. Defaults to 0
if not specified.
*/
public void setUsageQuantity(Integer usageQuantity) {
this.usageQuantity = usageQuantity;
}
/**
*
* Consumption value for the hour. Defaults to 0
if not specified.
*
*
* @return Consumption value for the hour. Defaults to 0
if not specified.
*/
public Integer getUsageQuantity() {
return this.usageQuantity;
}
/**
*
* Consumption value for the hour. Defaults to 0
if not specified.
*
*
* @param usageQuantity
* Consumption value for the hour. Defaults to 0
if not specified.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MeterUsageRequest withUsageQuantity(Integer usageQuantity) {
setUsageQuantity(usageQuantity);
return this;
}
/**
*
* Checks whether you have the permissions required for the action, but does not make the request. If you have the
* permissions, the request returns DryRunOperation
; otherwise, it returns
* UnauthorizedException
. Defaults to false
if not specified.
*
*
* @param dryRun
* Checks whether you have the permissions required for the action, but does not make the request. If you
* have the permissions, the request returns DryRunOperation
; otherwise, it returns
* UnauthorizedException
. Defaults to false
if not specified.
*/
public void setDryRun(Boolean dryRun) {
this.dryRun = dryRun;
}
/**
*
* Checks whether you have the permissions required for the action, but does not make the request. If you have the
* permissions, the request returns DryRunOperation
; otherwise, it returns
* UnauthorizedException
. Defaults to false
if not specified.
*
*
* @return Checks whether you have the permissions required for the action, but does not make the request. If you
* have the permissions, the request returns DryRunOperation
; otherwise, it returns
* UnauthorizedException
. Defaults to false
if not specified.
*/
public Boolean getDryRun() {
return this.dryRun;
}
/**
*
* Checks whether you have the permissions required for the action, but does not make the request. If you have the
* permissions, the request returns DryRunOperation
; otherwise, it returns
* UnauthorizedException
. Defaults to false
if not specified.
*
*
* @param dryRun
* Checks whether you have the permissions required for the action, but does not make the request. If you
* have the permissions, the request returns DryRunOperation
; otherwise, it returns
* UnauthorizedException
. Defaults to false
if not specified.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MeterUsageRequest withDryRun(Boolean dryRun) {
setDryRun(dryRun);
return this;
}
/**
*
* Checks whether you have the permissions required for the action, but does not make the request. If you have the
* permissions, the request returns DryRunOperation
; otherwise, it returns
* UnauthorizedException
. Defaults to false
if not specified.
*
*
* @return Checks whether you have the permissions required for the action, but does not make the request. If you
* have the permissions, the request returns DryRunOperation
; otherwise, it returns
* UnauthorizedException
. Defaults to false
if not specified.
*/
public Boolean isDryRun() {
return this.dryRun;
}
/**
*
* The set of UsageAllocations
to submit.
*
*
* The sum of all UsageAllocation
quantities must equal the UsageQuantity
of the
* MeterUsage
request, and each UsageAllocation
must have a unique set of tags (include no
* tags).
*
*
* @return The set of UsageAllocations
to submit.
*
* The sum of all UsageAllocation
quantities must equal the UsageQuantity
of the
* MeterUsage
request, and each UsageAllocation
must have a unique set of tags
* (include no tags).
*/
public java.util.List getUsageAllocations() {
return usageAllocations;
}
/**
*
* The set of UsageAllocations
to submit.
*
*
* The sum of all UsageAllocation
quantities must equal the UsageQuantity
of the
* MeterUsage
request, and each UsageAllocation
must have a unique set of tags (include no
* tags).
*
*
* @param usageAllocations
* The set of UsageAllocations
to submit.
*
* The sum of all UsageAllocation
quantities must equal the UsageQuantity
of the
* MeterUsage
request, and each UsageAllocation
must have a unique set of tags
* (include no tags).
*/
public void setUsageAllocations(java.util.Collection usageAllocations) {
if (usageAllocations == null) {
this.usageAllocations = null;
return;
}
this.usageAllocations = new java.util.ArrayList(usageAllocations);
}
/**
*
* The set of UsageAllocations
to submit.
*
*
* The sum of all UsageAllocation
quantities must equal the UsageQuantity
of the
* MeterUsage
request, and each UsageAllocation
must have a unique set of tags (include no
* tags).
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setUsageAllocations(java.util.Collection)} or {@link #withUsageAllocations(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param usageAllocations
* The set of UsageAllocations
to submit.
*
* The sum of all UsageAllocation
quantities must equal the UsageQuantity
of the
* MeterUsage
request, and each UsageAllocation
must have a unique set of tags
* (include no tags).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MeterUsageRequest withUsageAllocations(UsageAllocation... usageAllocations) {
if (this.usageAllocations == null) {
setUsageAllocations(new java.util.ArrayList(usageAllocations.length));
}
for (UsageAllocation ele : usageAllocations) {
this.usageAllocations.add(ele);
}
return this;
}
/**
*
* The set of UsageAllocations
to submit.
*
*
* The sum of all UsageAllocation
quantities must equal the UsageQuantity
of the
* MeterUsage
request, and each UsageAllocation
must have a unique set of tags (include no
* tags).
*
*
* @param usageAllocations
* The set of UsageAllocations
to submit.
*
* The sum of all UsageAllocation
quantities must equal the UsageQuantity
of the
* MeterUsage
request, and each UsageAllocation
must have a unique set of tags
* (include no tags).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MeterUsageRequest withUsageAllocations(java.util.Collection usageAllocations) {
setUsageAllocations(usageAllocations);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getProductCode() != null)
sb.append("ProductCode: ").append(getProductCode()).append(",");
if (getTimestamp() != null)
sb.append("Timestamp: ").append(getTimestamp()).append(",");
if (getUsageDimension() != null)
sb.append("UsageDimension: ").append(getUsageDimension()).append(",");
if (getUsageQuantity() != null)
sb.append("UsageQuantity: ").append(getUsageQuantity()).append(",");
if (getDryRun() != null)
sb.append("DryRun: ").append(getDryRun()).append(",");
if (getUsageAllocations() != null)
sb.append("UsageAllocations: ").append(getUsageAllocations());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof MeterUsageRequest == false)
return false;
MeterUsageRequest other = (MeterUsageRequest) obj;
if (other.getProductCode() == null ^ this.getProductCode() == null)
return false;
if (other.getProductCode() != null && other.getProductCode().equals(this.getProductCode()) == false)
return false;
if (other.getTimestamp() == null ^ this.getTimestamp() == null)
return false;
if (other.getTimestamp() != null && other.getTimestamp().equals(this.getTimestamp()) == false)
return false;
if (other.getUsageDimension() == null ^ this.getUsageDimension() == null)
return false;
if (other.getUsageDimension() != null && other.getUsageDimension().equals(this.getUsageDimension()) == false)
return false;
if (other.getUsageQuantity() == null ^ this.getUsageQuantity() == null)
return false;
if (other.getUsageQuantity() != null && other.getUsageQuantity().equals(this.getUsageQuantity()) == false)
return false;
if (other.getDryRun() == null ^ this.getDryRun() == null)
return false;
if (other.getDryRun() != null && other.getDryRun().equals(this.getDryRun()) == false)
return false;
if (other.getUsageAllocations() == null ^ this.getUsageAllocations() == null)
return false;
if (other.getUsageAllocations() != null && other.getUsageAllocations().equals(this.getUsageAllocations()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getProductCode() == null) ? 0 : getProductCode().hashCode());
hashCode = prime * hashCode + ((getTimestamp() == null) ? 0 : getTimestamp().hashCode());
hashCode = prime * hashCode + ((getUsageDimension() == null) ? 0 : getUsageDimension().hashCode());
hashCode = prime * hashCode + ((getUsageQuantity() == null) ? 0 : getUsageQuantity().hashCode());
hashCode = prime * hashCode + ((getDryRun() == null) ? 0 : getDryRun().hashCode());
hashCode = prime * hashCode + ((getUsageAllocations() == null) ? 0 : getUsageAllocations().hashCode());
return hashCode;
}
@Override
public MeterUsageRequest clone() {
return (MeterUsageRequest) super.clone();
}
}