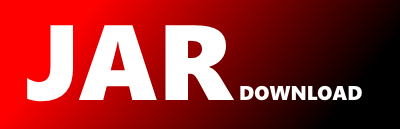
com.amazonaws.services.mturk.model.HIT Maven / Gradle / Ivy
Show all versions of aws-java-sdk-mechanicalturkrequester Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.mturk.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* The HIT data structure represents a single HIT, including all the information necessary for a Worker to accept and
* complete the HIT.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class HIT implements Serializable, Cloneable, StructuredPojo {
/**
*
* A unique identifier for the HIT.
*
*/
private String hITId;
/**
*
* The ID of the HIT type of this HIT
*
*/
private String hITTypeId;
/**
*
* The ID of the HIT Group of this HIT.
*
*/
private String hITGroupId;
/**
*
* The ID of the HIT Layout of this HIT.
*
*/
private String hITLayoutId;
/**
*
* The date and time the HIT was created.
*
*/
private java.util.Date creationTime;
/**
*
* The title of the HIT.
*
*/
private String title;
/**
*
* A general description of the HIT.
*
*/
private String description;
/**
*
* The data the Worker completing the HIT uses produce the results. This is either either a QuestionForm,
* HTMLQuestion or an ExternalQuestion data structure.
*
*/
private String question;
/**
*
* One or more words or phrases that describe the HIT, separated by commas. Search terms similar to the keywords of
* a HIT are more likely to have the HIT in the search results.
*
*/
private String keywords;
/**
*
* The status of the HIT and its assignments. Valid Values are Assignable | Unassignable | Reviewable | Reviewing |
* Disposed.
*
*/
private String hITStatus;
/**
*
* The number of times the HIT can be accepted and completed before the HIT becomes unavailable.
*
*/
private Integer maxAssignments;
private String reward;
/**
*
* The amount of time, in seconds, after the Worker submits an assignment for the HIT that the results are
* automatically approved by Amazon Mechanical Turk. This is the amount of time the Requester has to reject an
* assignment submitted by a Worker before the assignment is auto-approved and the Worker is paid.
*
*/
private Long autoApprovalDelayInSeconds;
/**
*
* The date and time the HIT expires.
*
*/
private java.util.Date expiration;
/**
*
* The length of time, in seconds, that a Worker has to complete the HIT after accepting it.
*
*/
private Long assignmentDurationInSeconds;
/**
*
* An arbitrary data field the Requester who created the HIT can use. This field is visible only to the creator of
* the HIT.
*
*/
private String requesterAnnotation;
/**
*
* Conditions that a Worker's Qualifications must meet in order to accept the HIT. A HIT can have between zero and
* ten Qualification requirements. All requirements must be met in order for a Worker to accept the HIT.
* Additionally, other actions can be restricted using the ActionsGuarded
field on each
* QualificationRequirement
structure.
*
*/
private java.util.List qualificationRequirements;
/**
*
* Indicates the review status of the HIT. Valid Values are NotReviewed | MarkedForReview | ReviewedAppropriate |
* ReviewedInappropriate.
*
*/
private String hITReviewStatus;
/**
*
* The number of assignments for this HIT that are being previewed or have been accepted by Workers, but have not
* yet been submitted, returned, or abandoned.
*
*/
private Integer numberOfAssignmentsPending;
/**
*
* The number of assignments for this HIT that are available for Workers to accept.
*
*/
private Integer numberOfAssignmentsAvailable;
/**
*
* The number of assignments for this HIT that have been approved or rejected.
*
*/
private Integer numberOfAssignmentsCompleted;
/**
*
* A unique identifier for the HIT.
*
*
* @param hITId
* A unique identifier for the HIT.
*/
public void setHITId(String hITId) {
this.hITId = hITId;
}
/**
*
* A unique identifier for the HIT.
*
*
* @return A unique identifier for the HIT.
*/
public String getHITId() {
return this.hITId;
}
/**
*
* A unique identifier for the HIT.
*
*
* @param hITId
* A unique identifier for the HIT.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HIT withHITId(String hITId) {
setHITId(hITId);
return this;
}
/**
*
* The ID of the HIT type of this HIT
*
*
* @param hITTypeId
* The ID of the HIT type of this HIT
*/
public void setHITTypeId(String hITTypeId) {
this.hITTypeId = hITTypeId;
}
/**
*
* The ID of the HIT type of this HIT
*
*
* @return The ID of the HIT type of this HIT
*/
public String getHITTypeId() {
return this.hITTypeId;
}
/**
*
* The ID of the HIT type of this HIT
*
*
* @param hITTypeId
* The ID of the HIT type of this HIT
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HIT withHITTypeId(String hITTypeId) {
setHITTypeId(hITTypeId);
return this;
}
/**
*
* The ID of the HIT Group of this HIT.
*
*
* @param hITGroupId
* The ID of the HIT Group of this HIT.
*/
public void setHITGroupId(String hITGroupId) {
this.hITGroupId = hITGroupId;
}
/**
*
* The ID of the HIT Group of this HIT.
*
*
* @return The ID of the HIT Group of this HIT.
*/
public String getHITGroupId() {
return this.hITGroupId;
}
/**
*
* The ID of the HIT Group of this HIT.
*
*
* @param hITGroupId
* The ID of the HIT Group of this HIT.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HIT withHITGroupId(String hITGroupId) {
setHITGroupId(hITGroupId);
return this;
}
/**
*
* The ID of the HIT Layout of this HIT.
*
*
* @param hITLayoutId
* The ID of the HIT Layout of this HIT.
*/
public void setHITLayoutId(String hITLayoutId) {
this.hITLayoutId = hITLayoutId;
}
/**
*
* The ID of the HIT Layout of this HIT.
*
*
* @return The ID of the HIT Layout of this HIT.
*/
public String getHITLayoutId() {
return this.hITLayoutId;
}
/**
*
* The ID of the HIT Layout of this HIT.
*
*
* @param hITLayoutId
* The ID of the HIT Layout of this HIT.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HIT withHITLayoutId(String hITLayoutId) {
setHITLayoutId(hITLayoutId);
return this;
}
/**
*
* The date and time the HIT was created.
*
*
* @param creationTime
* The date and time the HIT was created.
*/
public void setCreationTime(java.util.Date creationTime) {
this.creationTime = creationTime;
}
/**
*
* The date and time the HIT was created.
*
*
* @return The date and time the HIT was created.
*/
public java.util.Date getCreationTime() {
return this.creationTime;
}
/**
*
* The date and time the HIT was created.
*
*
* @param creationTime
* The date and time the HIT was created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HIT withCreationTime(java.util.Date creationTime) {
setCreationTime(creationTime);
return this;
}
/**
*
* The title of the HIT.
*
*
* @param title
* The title of the HIT.
*/
public void setTitle(String title) {
this.title = title;
}
/**
*
* The title of the HIT.
*
*
* @return The title of the HIT.
*/
public String getTitle() {
return this.title;
}
/**
*
* The title of the HIT.
*
*
* @param title
* The title of the HIT.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HIT withTitle(String title) {
setTitle(title);
return this;
}
/**
*
* A general description of the HIT.
*
*
* @param description
* A general description of the HIT.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* A general description of the HIT.
*
*
* @return A general description of the HIT.
*/
public String getDescription() {
return this.description;
}
/**
*
* A general description of the HIT.
*
*
* @param description
* A general description of the HIT.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HIT withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* The data the Worker completing the HIT uses produce the results. This is either either a QuestionForm,
* HTMLQuestion or an ExternalQuestion data structure.
*
*
* @param question
* The data the Worker completing the HIT uses produce the results. This is either either a QuestionForm,
* HTMLQuestion or an ExternalQuestion data structure.
*/
public void setQuestion(String question) {
this.question = question;
}
/**
*
* The data the Worker completing the HIT uses produce the results. This is either either a QuestionForm,
* HTMLQuestion or an ExternalQuestion data structure.
*
*
* @return The data the Worker completing the HIT uses produce the results. This is either either a QuestionForm,
* HTMLQuestion or an ExternalQuestion data structure.
*/
public String getQuestion() {
return this.question;
}
/**
*
* The data the Worker completing the HIT uses produce the results. This is either either a QuestionForm,
* HTMLQuestion or an ExternalQuestion data structure.
*
*
* @param question
* The data the Worker completing the HIT uses produce the results. This is either either a QuestionForm,
* HTMLQuestion or an ExternalQuestion data structure.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HIT withQuestion(String question) {
setQuestion(question);
return this;
}
/**
*
* One or more words or phrases that describe the HIT, separated by commas. Search terms similar to the keywords of
* a HIT are more likely to have the HIT in the search results.
*
*
* @param keywords
* One or more words or phrases that describe the HIT, separated by commas. Search terms similar to the
* keywords of a HIT are more likely to have the HIT in the search results.
*/
public void setKeywords(String keywords) {
this.keywords = keywords;
}
/**
*
* One or more words or phrases that describe the HIT, separated by commas. Search terms similar to the keywords of
* a HIT are more likely to have the HIT in the search results.
*
*
* @return One or more words or phrases that describe the HIT, separated by commas. Search terms similar to the
* keywords of a HIT are more likely to have the HIT in the search results.
*/
public String getKeywords() {
return this.keywords;
}
/**
*
* One or more words or phrases that describe the HIT, separated by commas. Search terms similar to the keywords of
* a HIT are more likely to have the HIT in the search results.
*
*
* @param keywords
* One or more words or phrases that describe the HIT, separated by commas. Search terms similar to the
* keywords of a HIT are more likely to have the HIT in the search results.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HIT withKeywords(String keywords) {
setKeywords(keywords);
return this;
}
/**
*
* The status of the HIT and its assignments. Valid Values are Assignable | Unassignable | Reviewable | Reviewing |
* Disposed.
*
*
* @param hITStatus
* The status of the HIT and its assignments. Valid Values are Assignable | Unassignable | Reviewable |
* Reviewing | Disposed.
* @see HITStatus
*/
public void setHITStatus(String hITStatus) {
this.hITStatus = hITStatus;
}
/**
*
* The status of the HIT and its assignments. Valid Values are Assignable | Unassignable | Reviewable | Reviewing |
* Disposed.
*
*
* @return The status of the HIT and its assignments. Valid Values are Assignable | Unassignable | Reviewable |
* Reviewing | Disposed.
* @see HITStatus
*/
public String getHITStatus() {
return this.hITStatus;
}
/**
*
* The status of the HIT and its assignments. Valid Values are Assignable | Unassignable | Reviewable | Reviewing |
* Disposed.
*
*
* @param hITStatus
* The status of the HIT and its assignments. Valid Values are Assignable | Unassignable | Reviewable |
* Reviewing | Disposed.
* @return Returns a reference to this object so that method calls can be chained together.
* @see HITStatus
*/
public HIT withHITStatus(String hITStatus) {
setHITStatus(hITStatus);
return this;
}
/**
*
* The status of the HIT and its assignments. Valid Values are Assignable | Unassignable | Reviewable | Reviewing |
* Disposed.
*
*
* @param hITStatus
* The status of the HIT and its assignments. Valid Values are Assignable | Unassignable | Reviewable |
* Reviewing | Disposed.
* @see HITStatus
*/
public void setHITStatus(HITStatus hITStatus) {
withHITStatus(hITStatus);
}
/**
*
* The status of the HIT and its assignments. Valid Values are Assignable | Unassignable | Reviewable | Reviewing |
* Disposed.
*
*
* @param hITStatus
* The status of the HIT and its assignments. Valid Values are Assignable | Unassignable | Reviewable |
* Reviewing | Disposed.
* @return Returns a reference to this object so that method calls can be chained together.
* @see HITStatus
*/
public HIT withHITStatus(HITStatus hITStatus) {
this.hITStatus = hITStatus.toString();
return this;
}
/**
*
* The number of times the HIT can be accepted and completed before the HIT becomes unavailable.
*
*
* @param maxAssignments
* The number of times the HIT can be accepted and completed before the HIT becomes unavailable.
*/
public void setMaxAssignments(Integer maxAssignments) {
this.maxAssignments = maxAssignments;
}
/**
*
* The number of times the HIT can be accepted and completed before the HIT becomes unavailable.
*
*
* @return The number of times the HIT can be accepted and completed before the HIT becomes unavailable.
*/
public Integer getMaxAssignments() {
return this.maxAssignments;
}
/**
*
* The number of times the HIT can be accepted and completed before the HIT becomes unavailable.
*
*
* @param maxAssignments
* The number of times the HIT can be accepted and completed before the HIT becomes unavailable.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HIT withMaxAssignments(Integer maxAssignments) {
setMaxAssignments(maxAssignments);
return this;
}
/**
* @param reward
*/
public void setReward(String reward) {
this.reward = reward;
}
/**
* @return
*/
public String getReward() {
return this.reward;
}
/**
* @param reward
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HIT withReward(String reward) {
setReward(reward);
return this;
}
/**
*
* The amount of time, in seconds, after the Worker submits an assignment for the HIT that the results are
* automatically approved by Amazon Mechanical Turk. This is the amount of time the Requester has to reject an
* assignment submitted by a Worker before the assignment is auto-approved and the Worker is paid.
*
*
* @param autoApprovalDelayInSeconds
* The amount of time, in seconds, after the Worker submits an assignment for the HIT that the results are
* automatically approved by Amazon Mechanical Turk. This is the amount of time the Requester has to reject
* an assignment submitted by a Worker before the assignment is auto-approved and the Worker is paid.
*/
public void setAutoApprovalDelayInSeconds(Long autoApprovalDelayInSeconds) {
this.autoApprovalDelayInSeconds = autoApprovalDelayInSeconds;
}
/**
*
* The amount of time, in seconds, after the Worker submits an assignment for the HIT that the results are
* automatically approved by Amazon Mechanical Turk. This is the amount of time the Requester has to reject an
* assignment submitted by a Worker before the assignment is auto-approved and the Worker is paid.
*
*
* @return The amount of time, in seconds, after the Worker submits an assignment for the HIT that the results are
* automatically approved by Amazon Mechanical Turk. This is the amount of time the Requester has to reject
* an assignment submitted by a Worker before the assignment is auto-approved and the Worker is paid.
*/
public Long getAutoApprovalDelayInSeconds() {
return this.autoApprovalDelayInSeconds;
}
/**
*
* The amount of time, in seconds, after the Worker submits an assignment for the HIT that the results are
* automatically approved by Amazon Mechanical Turk. This is the amount of time the Requester has to reject an
* assignment submitted by a Worker before the assignment is auto-approved and the Worker is paid.
*
*
* @param autoApprovalDelayInSeconds
* The amount of time, in seconds, after the Worker submits an assignment for the HIT that the results are
* automatically approved by Amazon Mechanical Turk. This is the amount of time the Requester has to reject
* an assignment submitted by a Worker before the assignment is auto-approved and the Worker is paid.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HIT withAutoApprovalDelayInSeconds(Long autoApprovalDelayInSeconds) {
setAutoApprovalDelayInSeconds(autoApprovalDelayInSeconds);
return this;
}
/**
*
* The date and time the HIT expires.
*
*
* @param expiration
* The date and time the HIT expires.
*/
public void setExpiration(java.util.Date expiration) {
this.expiration = expiration;
}
/**
*
* The date and time the HIT expires.
*
*
* @return The date and time the HIT expires.
*/
public java.util.Date getExpiration() {
return this.expiration;
}
/**
*
* The date and time the HIT expires.
*
*
* @param expiration
* The date and time the HIT expires.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HIT withExpiration(java.util.Date expiration) {
setExpiration(expiration);
return this;
}
/**
*
* The length of time, in seconds, that a Worker has to complete the HIT after accepting it.
*
*
* @param assignmentDurationInSeconds
* The length of time, in seconds, that a Worker has to complete the HIT after accepting it.
*/
public void setAssignmentDurationInSeconds(Long assignmentDurationInSeconds) {
this.assignmentDurationInSeconds = assignmentDurationInSeconds;
}
/**
*
* The length of time, in seconds, that a Worker has to complete the HIT after accepting it.
*
*
* @return The length of time, in seconds, that a Worker has to complete the HIT after accepting it.
*/
public Long getAssignmentDurationInSeconds() {
return this.assignmentDurationInSeconds;
}
/**
*
* The length of time, in seconds, that a Worker has to complete the HIT after accepting it.
*
*
* @param assignmentDurationInSeconds
* The length of time, in seconds, that a Worker has to complete the HIT after accepting it.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HIT withAssignmentDurationInSeconds(Long assignmentDurationInSeconds) {
setAssignmentDurationInSeconds(assignmentDurationInSeconds);
return this;
}
/**
*
* An arbitrary data field the Requester who created the HIT can use. This field is visible only to the creator of
* the HIT.
*
*
* @param requesterAnnotation
* An arbitrary data field the Requester who created the HIT can use. This field is visible only to the
* creator of the HIT.
*/
public void setRequesterAnnotation(String requesterAnnotation) {
this.requesterAnnotation = requesterAnnotation;
}
/**
*
* An arbitrary data field the Requester who created the HIT can use. This field is visible only to the creator of
* the HIT.
*
*
* @return An arbitrary data field the Requester who created the HIT can use. This field is visible only to the
* creator of the HIT.
*/
public String getRequesterAnnotation() {
return this.requesterAnnotation;
}
/**
*
* An arbitrary data field the Requester who created the HIT can use. This field is visible only to the creator of
* the HIT.
*
*
* @param requesterAnnotation
* An arbitrary data field the Requester who created the HIT can use. This field is visible only to the
* creator of the HIT.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HIT withRequesterAnnotation(String requesterAnnotation) {
setRequesterAnnotation(requesterAnnotation);
return this;
}
/**
*
* Conditions that a Worker's Qualifications must meet in order to accept the HIT. A HIT can have between zero and
* ten Qualification requirements. All requirements must be met in order for a Worker to accept the HIT.
* Additionally, other actions can be restricted using the ActionsGuarded
field on each
* QualificationRequirement
structure.
*
*
* @return Conditions that a Worker's Qualifications must meet in order to accept the HIT. A HIT can have between
* zero and ten Qualification requirements. All requirements must be met in order for a Worker to accept the
* HIT. Additionally, other actions can be restricted using the ActionsGuarded
field on each
* QualificationRequirement
structure.
*/
public java.util.List getQualificationRequirements() {
return qualificationRequirements;
}
/**
*
* Conditions that a Worker's Qualifications must meet in order to accept the HIT. A HIT can have between zero and
* ten Qualification requirements. All requirements must be met in order for a Worker to accept the HIT.
* Additionally, other actions can be restricted using the ActionsGuarded
field on each
* QualificationRequirement
structure.
*
*
* @param qualificationRequirements
* Conditions that a Worker's Qualifications must meet in order to accept the HIT. A HIT can have between
* zero and ten Qualification requirements. All requirements must be met in order for a Worker to accept the
* HIT. Additionally, other actions can be restricted using the ActionsGuarded
field on each
* QualificationRequirement
structure.
*/
public void setQualificationRequirements(java.util.Collection qualificationRequirements) {
if (qualificationRequirements == null) {
this.qualificationRequirements = null;
return;
}
this.qualificationRequirements = new java.util.ArrayList(qualificationRequirements);
}
/**
*
* Conditions that a Worker's Qualifications must meet in order to accept the HIT. A HIT can have between zero and
* ten Qualification requirements. All requirements must be met in order for a Worker to accept the HIT.
* Additionally, other actions can be restricted using the ActionsGuarded
field on each
* QualificationRequirement
structure.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setQualificationRequirements(java.util.Collection)} or
* {@link #withQualificationRequirements(java.util.Collection)} if you want to override the existing values.
*
*
* @param qualificationRequirements
* Conditions that a Worker's Qualifications must meet in order to accept the HIT. A HIT can have between
* zero and ten Qualification requirements. All requirements must be met in order for a Worker to accept the
* HIT. Additionally, other actions can be restricted using the ActionsGuarded
field on each
* QualificationRequirement
structure.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HIT withQualificationRequirements(QualificationRequirement... qualificationRequirements) {
if (this.qualificationRequirements == null) {
setQualificationRequirements(new java.util.ArrayList(qualificationRequirements.length));
}
for (QualificationRequirement ele : qualificationRequirements) {
this.qualificationRequirements.add(ele);
}
return this;
}
/**
*
* Conditions that a Worker's Qualifications must meet in order to accept the HIT. A HIT can have between zero and
* ten Qualification requirements. All requirements must be met in order for a Worker to accept the HIT.
* Additionally, other actions can be restricted using the ActionsGuarded
field on each
* QualificationRequirement
structure.
*
*
* @param qualificationRequirements
* Conditions that a Worker's Qualifications must meet in order to accept the HIT. A HIT can have between
* zero and ten Qualification requirements. All requirements must be met in order for a Worker to accept the
* HIT. Additionally, other actions can be restricted using the ActionsGuarded
field on each
* QualificationRequirement
structure.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HIT withQualificationRequirements(java.util.Collection qualificationRequirements) {
setQualificationRequirements(qualificationRequirements);
return this;
}
/**
*
* Indicates the review status of the HIT. Valid Values are NotReviewed | MarkedForReview | ReviewedAppropriate |
* ReviewedInappropriate.
*
*
* @param hITReviewStatus
* Indicates the review status of the HIT. Valid Values are NotReviewed | MarkedForReview |
* ReviewedAppropriate | ReviewedInappropriate.
* @see HITReviewStatus
*/
public void setHITReviewStatus(String hITReviewStatus) {
this.hITReviewStatus = hITReviewStatus;
}
/**
*
* Indicates the review status of the HIT. Valid Values are NotReviewed | MarkedForReview | ReviewedAppropriate |
* ReviewedInappropriate.
*
*
* @return Indicates the review status of the HIT. Valid Values are NotReviewed | MarkedForReview |
* ReviewedAppropriate | ReviewedInappropriate.
* @see HITReviewStatus
*/
public String getHITReviewStatus() {
return this.hITReviewStatus;
}
/**
*
* Indicates the review status of the HIT. Valid Values are NotReviewed | MarkedForReview | ReviewedAppropriate |
* ReviewedInappropriate.
*
*
* @param hITReviewStatus
* Indicates the review status of the HIT. Valid Values are NotReviewed | MarkedForReview |
* ReviewedAppropriate | ReviewedInappropriate.
* @return Returns a reference to this object so that method calls can be chained together.
* @see HITReviewStatus
*/
public HIT withHITReviewStatus(String hITReviewStatus) {
setHITReviewStatus(hITReviewStatus);
return this;
}
/**
*
* Indicates the review status of the HIT. Valid Values are NotReviewed | MarkedForReview | ReviewedAppropriate |
* ReviewedInappropriate.
*
*
* @param hITReviewStatus
* Indicates the review status of the HIT. Valid Values are NotReviewed | MarkedForReview |
* ReviewedAppropriate | ReviewedInappropriate.
* @see HITReviewStatus
*/
public void setHITReviewStatus(HITReviewStatus hITReviewStatus) {
withHITReviewStatus(hITReviewStatus);
}
/**
*
* Indicates the review status of the HIT. Valid Values are NotReviewed | MarkedForReview | ReviewedAppropriate |
* ReviewedInappropriate.
*
*
* @param hITReviewStatus
* Indicates the review status of the HIT. Valid Values are NotReviewed | MarkedForReview |
* ReviewedAppropriate | ReviewedInappropriate.
* @return Returns a reference to this object so that method calls can be chained together.
* @see HITReviewStatus
*/
public HIT withHITReviewStatus(HITReviewStatus hITReviewStatus) {
this.hITReviewStatus = hITReviewStatus.toString();
return this;
}
/**
*
* The number of assignments for this HIT that are being previewed or have been accepted by Workers, but have not
* yet been submitted, returned, or abandoned.
*
*
* @param numberOfAssignmentsPending
* The number of assignments for this HIT that are being previewed or have been accepted by Workers, but have
* not yet been submitted, returned, or abandoned.
*/
public void setNumberOfAssignmentsPending(Integer numberOfAssignmentsPending) {
this.numberOfAssignmentsPending = numberOfAssignmentsPending;
}
/**
*
* The number of assignments for this HIT that are being previewed or have been accepted by Workers, but have not
* yet been submitted, returned, or abandoned.
*
*
* @return The number of assignments for this HIT that are being previewed or have been accepted by Workers, but
* have not yet been submitted, returned, or abandoned.
*/
public Integer getNumberOfAssignmentsPending() {
return this.numberOfAssignmentsPending;
}
/**
*
* The number of assignments for this HIT that are being previewed or have been accepted by Workers, but have not
* yet been submitted, returned, or abandoned.
*
*
* @param numberOfAssignmentsPending
* The number of assignments for this HIT that are being previewed or have been accepted by Workers, but have
* not yet been submitted, returned, or abandoned.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HIT withNumberOfAssignmentsPending(Integer numberOfAssignmentsPending) {
setNumberOfAssignmentsPending(numberOfAssignmentsPending);
return this;
}
/**
*
* The number of assignments for this HIT that are available for Workers to accept.
*
*
* @param numberOfAssignmentsAvailable
* The number of assignments for this HIT that are available for Workers to accept.
*/
public void setNumberOfAssignmentsAvailable(Integer numberOfAssignmentsAvailable) {
this.numberOfAssignmentsAvailable = numberOfAssignmentsAvailable;
}
/**
*
* The number of assignments for this HIT that are available for Workers to accept.
*
*
* @return The number of assignments for this HIT that are available for Workers to accept.
*/
public Integer getNumberOfAssignmentsAvailable() {
return this.numberOfAssignmentsAvailable;
}
/**
*
* The number of assignments for this HIT that are available for Workers to accept.
*
*
* @param numberOfAssignmentsAvailable
* The number of assignments for this HIT that are available for Workers to accept.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HIT withNumberOfAssignmentsAvailable(Integer numberOfAssignmentsAvailable) {
setNumberOfAssignmentsAvailable(numberOfAssignmentsAvailable);
return this;
}
/**
*
* The number of assignments for this HIT that have been approved or rejected.
*
*
* @param numberOfAssignmentsCompleted
* The number of assignments for this HIT that have been approved or rejected.
*/
public void setNumberOfAssignmentsCompleted(Integer numberOfAssignmentsCompleted) {
this.numberOfAssignmentsCompleted = numberOfAssignmentsCompleted;
}
/**
*
* The number of assignments for this HIT that have been approved or rejected.
*
*
* @return The number of assignments for this HIT that have been approved or rejected.
*/
public Integer getNumberOfAssignmentsCompleted() {
return this.numberOfAssignmentsCompleted;
}
/**
*
* The number of assignments for this HIT that have been approved or rejected.
*
*
* @param numberOfAssignmentsCompleted
* The number of assignments for this HIT that have been approved or rejected.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HIT withNumberOfAssignmentsCompleted(Integer numberOfAssignmentsCompleted) {
setNumberOfAssignmentsCompleted(numberOfAssignmentsCompleted);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getHITId() != null)
sb.append("HITId: ").append(getHITId()).append(",");
if (getHITTypeId() != null)
sb.append("HITTypeId: ").append(getHITTypeId()).append(",");
if (getHITGroupId() != null)
sb.append("HITGroupId: ").append(getHITGroupId()).append(",");
if (getHITLayoutId() != null)
sb.append("HITLayoutId: ").append(getHITLayoutId()).append(",");
if (getCreationTime() != null)
sb.append("CreationTime: ").append(getCreationTime()).append(",");
if (getTitle() != null)
sb.append("Title: ").append(getTitle()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getQuestion() != null)
sb.append("Question: ").append(getQuestion()).append(",");
if (getKeywords() != null)
sb.append("Keywords: ").append(getKeywords()).append(",");
if (getHITStatus() != null)
sb.append("HITStatus: ").append(getHITStatus()).append(",");
if (getMaxAssignments() != null)
sb.append("MaxAssignments: ").append(getMaxAssignments()).append(",");
if (getReward() != null)
sb.append("Reward: ").append(getReward()).append(",");
if (getAutoApprovalDelayInSeconds() != null)
sb.append("AutoApprovalDelayInSeconds: ").append(getAutoApprovalDelayInSeconds()).append(",");
if (getExpiration() != null)
sb.append("Expiration: ").append(getExpiration()).append(",");
if (getAssignmentDurationInSeconds() != null)
sb.append("AssignmentDurationInSeconds: ").append(getAssignmentDurationInSeconds()).append(",");
if (getRequesterAnnotation() != null)
sb.append("RequesterAnnotation: ").append(getRequesterAnnotation()).append(",");
if (getQualificationRequirements() != null)
sb.append("QualificationRequirements: ").append(getQualificationRequirements()).append(",");
if (getHITReviewStatus() != null)
sb.append("HITReviewStatus: ").append(getHITReviewStatus()).append(",");
if (getNumberOfAssignmentsPending() != null)
sb.append("NumberOfAssignmentsPending: ").append(getNumberOfAssignmentsPending()).append(",");
if (getNumberOfAssignmentsAvailable() != null)
sb.append("NumberOfAssignmentsAvailable: ").append(getNumberOfAssignmentsAvailable()).append(",");
if (getNumberOfAssignmentsCompleted() != null)
sb.append("NumberOfAssignmentsCompleted: ").append(getNumberOfAssignmentsCompleted());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof HIT == false)
return false;
HIT other = (HIT) obj;
if (other.getHITId() == null ^ this.getHITId() == null)
return false;
if (other.getHITId() != null && other.getHITId().equals(this.getHITId()) == false)
return false;
if (other.getHITTypeId() == null ^ this.getHITTypeId() == null)
return false;
if (other.getHITTypeId() != null && other.getHITTypeId().equals(this.getHITTypeId()) == false)
return false;
if (other.getHITGroupId() == null ^ this.getHITGroupId() == null)
return false;
if (other.getHITGroupId() != null && other.getHITGroupId().equals(this.getHITGroupId()) == false)
return false;
if (other.getHITLayoutId() == null ^ this.getHITLayoutId() == null)
return false;
if (other.getHITLayoutId() != null && other.getHITLayoutId().equals(this.getHITLayoutId()) == false)
return false;
if (other.getCreationTime() == null ^ this.getCreationTime() == null)
return false;
if (other.getCreationTime() != null && other.getCreationTime().equals(this.getCreationTime()) == false)
return false;
if (other.getTitle() == null ^ this.getTitle() == null)
return false;
if (other.getTitle() != null && other.getTitle().equals(this.getTitle()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getQuestion() == null ^ this.getQuestion() == null)
return false;
if (other.getQuestion() != null && other.getQuestion().equals(this.getQuestion()) == false)
return false;
if (other.getKeywords() == null ^ this.getKeywords() == null)
return false;
if (other.getKeywords() != null && other.getKeywords().equals(this.getKeywords()) == false)
return false;
if (other.getHITStatus() == null ^ this.getHITStatus() == null)
return false;
if (other.getHITStatus() != null && other.getHITStatus().equals(this.getHITStatus()) == false)
return false;
if (other.getMaxAssignments() == null ^ this.getMaxAssignments() == null)
return false;
if (other.getMaxAssignments() != null && other.getMaxAssignments().equals(this.getMaxAssignments()) == false)
return false;
if (other.getReward() == null ^ this.getReward() == null)
return false;
if (other.getReward() != null && other.getReward().equals(this.getReward()) == false)
return false;
if (other.getAutoApprovalDelayInSeconds() == null ^ this.getAutoApprovalDelayInSeconds() == null)
return false;
if (other.getAutoApprovalDelayInSeconds() != null && other.getAutoApprovalDelayInSeconds().equals(this.getAutoApprovalDelayInSeconds()) == false)
return false;
if (other.getExpiration() == null ^ this.getExpiration() == null)
return false;
if (other.getExpiration() != null && other.getExpiration().equals(this.getExpiration()) == false)
return false;
if (other.getAssignmentDurationInSeconds() == null ^ this.getAssignmentDurationInSeconds() == null)
return false;
if (other.getAssignmentDurationInSeconds() != null && other.getAssignmentDurationInSeconds().equals(this.getAssignmentDurationInSeconds()) == false)
return false;
if (other.getRequesterAnnotation() == null ^ this.getRequesterAnnotation() == null)
return false;
if (other.getRequesterAnnotation() != null && other.getRequesterAnnotation().equals(this.getRequesterAnnotation()) == false)
return false;
if (other.getQualificationRequirements() == null ^ this.getQualificationRequirements() == null)
return false;
if (other.getQualificationRequirements() != null && other.getQualificationRequirements().equals(this.getQualificationRequirements()) == false)
return false;
if (other.getHITReviewStatus() == null ^ this.getHITReviewStatus() == null)
return false;
if (other.getHITReviewStatus() != null && other.getHITReviewStatus().equals(this.getHITReviewStatus()) == false)
return false;
if (other.getNumberOfAssignmentsPending() == null ^ this.getNumberOfAssignmentsPending() == null)
return false;
if (other.getNumberOfAssignmentsPending() != null && other.getNumberOfAssignmentsPending().equals(this.getNumberOfAssignmentsPending()) == false)
return false;
if (other.getNumberOfAssignmentsAvailable() == null ^ this.getNumberOfAssignmentsAvailable() == null)
return false;
if (other.getNumberOfAssignmentsAvailable() != null && other.getNumberOfAssignmentsAvailable().equals(this.getNumberOfAssignmentsAvailable()) == false)
return false;
if (other.getNumberOfAssignmentsCompleted() == null ^ this.getNumberOfAssignmentsCompleted() == null)
return false;
if (other.getNumberOfAssignmentsCompleted() != null && other.getNumberOfAssignmentsCompleted().equals(this.getNumberOfAssignmentsCompleted()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getHITId() == null) ? 0 : getHITId().hashCode());
hashCode = prime * hashCode + ((getHITTypeId() == null) ? 0 : getHITTypeId().hashCode());
hashCode = prime * hashCode + ((getHITGroupId() == null) ? 0 : getHITGroupId().hashCode());
hashCode = prime * hashCode + ((getHITLayoutId() == null) ? 0 : getHITLayoutId().hashCode());
hashCode = prime * hashCode + ((getCreationTime() == null) ? 0 : getCreationTime().hashCode());
hashCode = prime * hashCode + ((getTitle() == null) ? 0 : getTitle().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getQuestion() == null) ? 0 : getQuestion().hashCode());
hashCode = prime * hashCode + ((getKeywords() == null) ? 0 : getKeywords().hashCode());
hashCode = prime * hashCode + ((getHITStatus() == null) ? 0 : getHITStatus().hashCode());
hashCode = prime * hashCode + ((getMaxAssignments() == null) ? 0 : getMaxAssignments().hashCode());
hashCode = prime * hashCode + ((getReward() == null) ? 0 : getReward().hashCode());
hashCode = prime * hashCode + ((getAutoApprovalDelayInSeconds() == null) ? 0 : getAutoApprovalDelayInSeconds().hashCode());
hashCode = prime * hashCode + ((getExpiration() == null) ? 0 : getExpiration().hashCode());
hashCode = prime * hashCode + ((getAssignmentDurationInSeconds() == null) ? 0 : getAssignmentDurationInSeconds().hashCode());
hashCode = prime * hashCode + ((getRequesterAnnotation() == null) ? 0 : getRequesterAnnotation().hashCode());
hashCode = prime * hashCode + ((getQualificationRequirements() == null) ? 0 : getQualificationRequirements().hashCode());
hashCode = prime * hashCode + ((getHITReviewStatus() == null) ? 0 : getHITReviewStatus().hashCode());
hashCode = prime * hashCode + ((getNumberOfAssignmentsPending() == null) ? 0 : getNumberOfAssignmentsPending().hashCode());
hashCode = prime * hashCode + ((getNumberOfAssignmentsAvailable() == null) ? 0 : getNumberOfAssignmentsAvailable().hashCode());
hashCode = prime * hashCode + ((getNumberOfAssignmentsCompleted() == null) ? 0 : getNumberOfAssignmentsCompleted().hashCode());
return hashCode;
}
@Override
public HIT clone() {
try {
return (HIT) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.mturk.model.transform.HITMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}