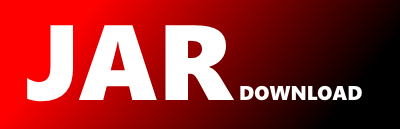
com.amazonaws.services.mturk.model.ListReviewPolicyResultsForHITRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-mechanicalturkrequester Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.mturk.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ListReviewPolicyResultsForHITRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The unique identifier of the HIT to retrieve review results for.
*
*/
private String hITId;
/**
*
* The Policy Level(s) to retrieve review results for - HIT or Assignment. If omitted, the default behavior is to
* retrieve all data for both policy levels. For a list of all the described policies, see Review Policies.
*
*/
private java.util.List policyLevels;
/**
*
* Specify if the operation should retrieve a list of the actions taken executing the Review Policies and their
* outcomes.
*
*/
private Boolean retrieveActions;
/**
*
* Specify if the operation should retrieve a list of the results computed by the Review Policies.
*
*/
private Boolean retrieveResults;
/**
*
* Pagination token
*
*/
private String nextToken;
/**
*
* Limit the number of results returned.
*
*/
private Integer maxResults;
/**
*
* The unique identifier of the HIT to retrieve review results for.
*
*
* @param hITId
* The unique identifier of the HIT to retrieve review results for.
*/
public void setHITId(String hITId) {
this.hITId = hITId;
}
/**
*
* The unique identifier of the HIT to retrieve review results for.
*
*
* @return The unique identifier of the HIT to retrieve review results for.
*/
public String getHITId() {
return this.hITId;
}
/**
*
* The unique identifier of the HIT to retrieve review results for.
*
*
* @param hITId
* The unique identifier of the HIT to retrieve review results for.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListReviewPolicyResultsForHITRequest withHITId(String hITId) {
setHITId(hITId);
return this;
}
/**
*
* The Policy Level(s) to retrieve review results for - HIT or Assignment. If omitted, the default behavior is to
* retrieve all data for both policy levels. For a list of all the described policies, see Review Policies.
*
*
* @return The Policy Level(s) to retrieve review results for - HIT or Assignment. If omitted, the default behavior
* is to retrieve all data for both policy levels. For a list of all the described policies, see Review
* Policies.
* @see ReviewPolicyLevel
*/
public java.util.List getPolicyLevels() {
return policyLevels;
}
/**
*
* The Policy Level(s) to retrieve review results for - HIT or Assignment. If omitted, the default behavior is to
* retrieve all data for both policy levels. For a list of all the described policies, see Review Policies.
*
*
* @param policyLevels
* The Policy Level(s) to retrieve review results for - HIT or Assignment. If omitted, the default behavior
* is to retrieve all data for both policy levels. For a list of all the described policies, see Review
* Policies.
* @see ReviewPolicyLevel
*/
public void setPolicyLevels(java.util.Collection policyLevels) {
if (policyLevels == null) {
this.policyLevels = null;
return;
}
this.policyLevels = new java.util.ArrayList(policyLevels);
}
/**
*
* The Policy Level(s) to retrieve review results for - HIT or Assignment. If omitted, the default behavior is to
* retrieve all data for both policy levels. For a list of all the described policies, see Review Policies.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setPolicyLevels(java.util.Collection)} or {@link #withPolicyLevels(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param policyLevels
* The Policy Level(s) to retrieve review results for - HIT or Assignment. If omitted, the default behavior
* is to retrieve all data for both policy levels. For a list of all the described policies, see Review
* Policies.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ReviewPolicyLevel
*/
public ListReviewPolicyResultsForHITRequest withPolicyLevels(String... policyLevels) {
if (this.policyLevels == null) {
setPolicyLevels(new java.util.ArrayList(policyLevels.length));
}
for (String ele : policyLevels) {
this.policyLevels.add(ele);
}
return this;
}
/**
*
* The Policy Level(s) to retrieve review results for - HIT or Assignment. If omitted, the default behavior is to
* retrieve all data for both policy levels. For a list of all the described policies, see Review Policies.
*
*
* @param policyLevels
* The Policy Level(s) to retrieve review results for - HIT or Assignment. If omitted, the default behavior
* is to retrieve all data for both policy levels. For a list of all the described policies, see Review
* Policies.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ReviewPolicyLevel
*/
public ListReviewPolicyResultsForHITRequest withPolicyLevels(java.util.Collection policyLevels) {
setPolicyLevels(policyLevels);
return this;
}
/**
*
* The Policy Level(s) to retrieve review results for - HIT or Assignment. If omitted, the default behavior is to
* retrieve all data for both policy levels. For a list of all the described policies, see Review Policies.
*
*
* @param policyLevels
* The Policy Level(s) to retrieve review results for - HIT or Assignment. If omitted, the default behavior
* is to retrieve all data for both policy levels. For a list of all the described policies, see Review
* Policies.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ReviewPolicyLevel
*/
public ListReviewPolicyResultsForHITRequest withPolicyLevels(ReviewPolicyLevel... policyLevels) {
java.util.ArrayList policyLevelsCopy = new java.util.ArrayList(policyLevels.length);
for (ReviewPolicyLevel value : policyLevels) {
policyLevelsCopy.add(value.toString());
}
if (getPolicyLevels() == null) {
setPolicyLevels(policyLevelsCopy);
} else {
getPolicyLevels().addAll(policyLevelsCopy);
}
return this;
}
/**
*
* Specify if the operation should retrieve a list of the actions taken executing the Review Policies and their
* outcomes.
*
*
* @param retrieveActions
* Specify if the operation should retrieve a list of the actions taken executing the Review Policies and
* their outcomes.
*/
public void setRetrieveActions(Boolean retrieveActions) {
this.retrieveActions = retrieveActions;
}
/**
*
* Specify if the operation should retrieve a list of the actions taken executing the Review Policies and their
* outcomes.
*
*
* @return Specify if the operation should retrieve a list of the actions taken executing the Review Policies and
* their outcomes.
*/
public Boolean getRetrieveActions() {
return this.retrieveActions;
}
/**
*
* Specify if the operation should retrieve a list of the actions taken executing the Review Policies and their
* outcomes.
*
*
* @param retrieveActions
* Specify if the operation should retrieve a list of the actions taken executing the Review Policies and
* their outcomes.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListReviewPolicyResultsForHITRequest withRetrieveActions(Boolean retrieveActions) {
setRetrieveActions(retrieveActions);
return this;
}
/**
*
* Specify if the operation should retrieve a list of the actions taken executing the Review Policies and their
* outcomes.
*
*
* @return Specify if the operation should retrieve a list of the actions taken executing the Review Policies and
* their outcomes.
*/
public Boolean isRetrieveActions() {
return this.retrieveActions;
}
/**
*
* Specify if the operation should retrieve a list of the results computed by the Review Policies.
*
*
* @param retrieveResults
* Specify if the operation should retrieve a list of the results computed by the Review Policies.
*/
public void setRetrieveResults(Boolean retrieveResults) {
this.retrieveResults = retrieveResults;
}
/**
*
* Specify if the operation should retrieve a list of the results computed by the Review Policies.
*
*
* @return Specify if the operation should retrieve a list of the results computed by the Review Policies.
*/
public Boolean getRetrieveResults() {
return this.retrieveResults;
}
/**
*
* Specify if the operation should retrieve a list of the results computed by the Review Policies.
*
*
* @param retrieveResults
* Specify if the operation should retrieve a list of the results computed by the Review Policies.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListReviewPolicyResultsForHITRequest withRetrieveResults(Boolean retrieveResults) {
setRetrieveResults(retrieveResults);
return this;
}
/**
*
* Specify if the operation should retrieve a list of the results computed by the Review Policies.
*
*
* @return Specify if the operation should retrieve a list of the results computed by the Review Policies.
*/
public Boolean isRetrieveResults() {
return this.retrieveResults;
}
/**
*
* Pagination token
*
*
* @param nextToken
* Pagination token
*/
public void setNextToken(String nextToken) {
this.nextToken = nextToken;
}
/**
*
* Pagination token
*
*
* @return Pagination token
*/
public String getNextToken() {
return this.nextToken;
}
/**
*
* Pagination token
*
*
* @param nextToken
* Pagination token
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListReviewPolicyResultsForHITRequest withNextToken(String nextToken) {
setNextToken(nextToken);
return this;
}
/**
*
* Limit the number of results returned.
*
*
* @param maxResults
* Limit the number of results returned.
*/
public void setMaxResults(Integer maxResults) {
this.maxResults = maxResults;
}
/**
*
* Limit the number of results returned.
*
*
* @return Limit the number of results returned.
*/
public Integer getMaxResults() {
return this.maxResults;
}
/**
*
* Limit the number of results returned.
*
*
* @param maxResults
* Limit the number of results returned.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListReviewPolicyResultsForHITRequest withMaxResults(Integer maxResults) {
setMaxResults(maxResults);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getHITId() != null)
sb.append("HITId: ").append(getHITId()).append(",");
if (getPolicyLevels() != null)
sb.append("PolicyLevels: ").append(getPolicyLevels()).append(",");
if (getRetrieveActions() != null)
sb.append("RetrieveActions: ").append(getRetrieveActions()).append(",");
if (getRetrieveResults() != null)
sb.append("RetrieveResults: ").append(getRetrieveResults()).append(",");
if (getNextToken() != null)
sb.append("NextToken: ").append(getNextToken()).append(",");
if (getMaxResults() != null)
sb.append("MaxResults: ").append(getMaxResults());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ListReviewPolicyResultsForHITRequest == false)
return false;
ListReviewPolicyResultsForHITRequest other = (ListReviewPolicyResultsForHITRequest) obj;
if (other.getHITId() == null ^ this.getHITId() == null)
return false;
if (other.getHITId() != null && other.getHITId().equals(this.getHITId()) == false)
return false;
if (other.getPolicyLevels() == null ^ this.getPolicyLevels() == null)
return false;
if (other.getPolicyLevels() != null && other.getPolicyLevels().equals(this.getPolicyLevels()) == false)
return false;
if (other.getRetrieveActions() == null ^ this.getRetrieveActions() == null)
return false;
if (other.getRetrieveActions() != null && other.getRetrieveActions().equals(this.getRetrieveActions()) == false)
return false;
if (other.getRetrieveResults() == null ^ this.getRetrieveResults() == null)
return false;
if (other.getRetrieveResults() != null && other.getRetrieveResults().equals(this.getRetrieveResults()) == false)
return false;
if (other.getNextToken() == null ^ this.getNextToken() == null)
return false;
if (other.getNextToken() != null && other.getNextToken().equals(this.getNextToken()) == false)
return false;
if (other.getMaxResults() == null ^ this.getMaxResults() == null)
return false;
if (other.getMaxResults() != null && other.getMaxResults().equals(this.getMaxResults()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getHITId() == null) ? 0 : getHITId().hashCode());
hashCode = prime * hashCode + ((getPolicyLevels() == null) ? 0 : getPolicyLevels().hashCode());
hashCode = prime * hashCode + ((getRetrieveActions() == null) ? 0 : getRetrieveActions().hashCode());
hashCode = prime * hashCode + ((getRetrieveResults() == null) ? 0 : getRetrieveResults().hashCode());
hashCode = prime * hashCode + ((getNextToken() == null) ? 0 : getNextToken().hashCode());
hashCode = prime * hashCode + ((getMaxResults() == null) ? 0 : getMaxResults().hashCode());
return hashCode;
}
@Override
public ListReviewPolicyResultsForHITRequest clone() {
return (ListReviewPolicyResultsForHITRequest) super.clone();
}
}