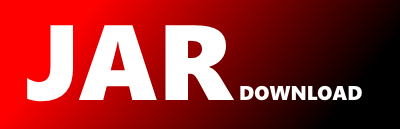
com.amazonaws.services.mediaconnect.AWSMediaConnect Maven / Gradle / Ivy
Show all versions of aws-java-sdk-mediaconnect Show documentation
/*
* Copyright 2018-2023 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.mediaconnect;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.mediaconnect.model.*;
import com.amazonaws.services.mediaconnect.waiters.AWSMediaConnectWaiters;
/**
* Interface for accessing AWS MediaConnect.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.mediaconnect.AbstractAWSMediaConnect} instead.
*
*
* API for AWS Elemental MediaConnect
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSMediaConnect {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "mediaconnect";
/**
* Adds outputs to an existing bridge.
*
* @param addBridgeOutputsRequest
* A request to add outputs to the specified bridge.
* @return Result of the AddBridgeOutputs operation returned by the service.
* @throws BadRequestException
* The request that you submitted is not valid.
* @throws InternalServerErrorException
* AWS Elemental MediaConnect can't fulfill your request because it encountered an unexpected condition.
* @throws ForbiddenException
* You don't have the required permissions to perform this operation.
* @throws NotFoundException
* AWS Elemental MediaConnect did not find the resource that you specified in the request.
* @throws ServiceUnavailableException
* AWS Elemental MediaConnect is currently unavailable. Try again later.
* @throws TooManyRequestsException
* You have exceeded the service request rate limit for your AWS Elemental MediaConnect account.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @sample AWSMediaConnect.AddBridgeOutputs
* @see AWS
* API Documentation
*/
AddBridgeOutputsResult addBridgeOutputs(AddBridgeOutputsRequest addBridgeOutputsRequest);
/**
* Adds sources to an existing bridge.
*
* @param addBridgeSourcesRequest
* A request to add sources to the specified bridge.
* @return Result of the AddBridgeSources operation returned by the service.
* @throws BadRequestException
* The request that you submitted is not valid.
* @throws InternalServerErrorException
* AWS Elemental MediaConnect can't fulfill your request because it encountered an unexpected condition.
* @throws ForbiddenException
* You don't have the required permissions to perform this operation.
* @throws NotFoundException
* AWS Elemental MediaConnect did not find the resource that you specified in the request.
* @throws ServiceUnavailableException
* AWS Elemental MediaConnect is currently unavailable. Try again later.
* @throws TooManyRequestsException
* You have exceeded the service request rate limit for your AWS Elemental MediaConnect account.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @sample AWSMediaConnect.AddBridgeSources
* @see AWS
* API Documentation
*/
AddBridgeSourcesResult addBridgeSources(AddBridgeSourcesRequest addBridgeSourcesRequest);
/**
* Adds media streams to an existing flow. After you add a media stream to a flow, you can associate it with a
* source and/or an output that uses the ST 2110 JPEG XS or CDI protocol.
*
* @param addFlowMediaStreamsRequest
* A request to add media streams to the flow.
* @return Result of the AddFlowMediaStreams operation returned by the service.
* @throws BadRequestException
* The request that you submitted is not valid.
* @throws InternalServerErrorException
* AWS Elemental MediaConnect can't fulfill your request because it encountered an unexpected condition.
* @throws ForbiddenException
* You don't have the required permissions to perform this operation.
* @throws NotFoundException
* AWS Elemental MediaConnect did not find the resource that you specified in the request.
* @throws ServiceUnavailableException
* AWS Elemental MediaConnect is currently unavailable. Try again later.
* @throws TooManyRequestsException
* You have exceeded the service request rate limit for your AWS Elemental MediaConnect account.
* @sample AWSMediaConnect.AddFlowMediaStreams
* @see AWS API Documentation
*/
AddFlowMediaStreamsResult addFlowMediaStreams(AddFlowMediaStreamsRequest addFlowMediaStreamsRequest);
/**
* Adds outputs to an existing flow. You can create up to 50 outputs per flow.
*
* @param addFlowOutputsRequest
* A request to add outputs to the specified flow.
* @return Result of the AddFlowOutputs operation returned by the service.
* @throws AddFlowOutputs420Exception
* AWS Elemental MediaConnect can't complete this request because this flow already has the maximum number
* of allowed outputs (50). For more information, contact AWS Customer Support.
* @throws BadRequestException
* The request that you submitted is not valid.
* @throws InternalServerErrorException
* AWS Elemental MediaConnect can't fulfill your request because it encountered an unexpected condition.
* @throws ForbiddenException
* You don't have the required permissions to perform this operation.
* @throws NotFoundException
* AWS Elemental MediaConnect did not find the resource that you specified in the request.
* @throws ServiceUnavailableException
* AWS Elemental MediaConnect is currently unavailable. Try again later.
* @throws TooManyRequestsException
* You have exceeded the service request rate limit for your AWS Elemental MediaConnect account.
* @sample AWSMediaConnect.AddFlowOutputs
* @see AWS
* API Documentation
*/
AddFlowOutputsResult addFlowOutputs(AddFlowOutputsRequest addFlowOutputsRequest);
/**
* Adds Sources to flow
*
* @param addFlowSourcesRequest
* A request to add sources to the flow.
* @return Result of the AddFlowSources operation returned by the service.
* @throws BadRequestException
* The request that you submitted is not valid.
* @throws InternalServerErrorException
* AWS Elemental MediaConnect can't fulfill your request because it encountered an unexpected condition.
* @throws ForbiddenException
* You don't have the required permissions to perform this operation.
* @throws NotFoundException
* AWS Elemental MediaConnect did not find the resource that you specified in the request.
* @throws ServiceUnavailableException
* AWS Elemental MediaConnect is currently unavailable. Try again later.
* @throws TooManyRequestsException
* You have exceeded the service request rate limit for your AWS Elemental MediaConnect account.
* @sample AWSMediaConnect.AddFlowSources
* @see AWS
* API Documentation
*/
AddFlowSourcesResult addFlowSources(AddFlowSourcesRequest addFlowSourcesRequest);
/**
* Adds VPC interfaces to flow
*
* @param addFlowVpcInterfacesRequest
* A request to add VPC interfaces to the flow.
* @return Result of the AddFlowVpcInterfaces operation returned by the service.
* @throws BadRequestException
* The request that you submitted is not valid.
* @throws InternalServerErrorException
* AWS Elemental MediaConnect can't fulfill your request because it encountered an unexpected condition.
* @throws ForbiddenException
* You don't have the required permissions to perform this operation.
* @throws NotFoundException
* AWS Elemental MediaConnect did not find the resource that you specified in the request.
* @throws ServiceUnavailableException
* AWS Elemental MediaConnect is currently unavailable. Try again later.
* @throws TooManyRequestsException
* You have exceeded the service request rate limit for your AWS Elemental MediaConnect account.
* @sample AWSMediaConnect.AddFlowVpcInterfaces
* @see AWS API Documentation
*/
AddFlowVpcInterfacesResult addFlowVpcInterfaces(AddFlowVpcInterfacesRequest addFlowVpcInterfacesRequest);
/**
* Creates a new bridge. The request must include one source.
*
* @param createBridgeRequest
* Creates a new bridge. The request must include one source.
* @return Result of the CreateBridge operation returned by the service.
* @throws CreateBridge420Exception
* Your account already contains the maximum number of bridges per account, per Region. For more
* information, contact AWS Customer Support.
* @throws BadRequestException
* The request that you submitted is not valid.
* @throws InternalServerErrorException
* AWS Elemental MediaConnect can't fulfill your request because it encountered an unexpected condition.
* @throws ForbiddenException
* You don't have the required permissions to perform this operation.
* @throws ServiceUnavailableException
* AWS Elemental MediaConnect is currently unavailable. Try again later.
* @throws TooManyRequestsException
* You have exceeded the service request rate limit for your AWS Elemental MediaConnect account.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @sample AWSMediaConnect.CreateBridge
* @see AWS API
* Documentation
*/
CreateBridgeResult createBridge(CreateBridgeRequest createBridgeRequest);
/**
* Creates a new flow. The request must include one source. The request optionally can include outputs (up to 50)
* and entitlements (up to 50).
*
* @param createFlowRequest
* Creates a new flow. The request must include one source. The request optionally can include outputs (up to
* 50) and entitlements (up to 50).
* @return Result of the CreateFlow operation returned by the service.
* @throws CreateFlow420Exception
* Your account already contains the maximum number of 20 flows per account, per Region. For more
* information, contact AWS Customer Support.
* @throws BadRequestException
* The request that you submitted is not valid.
* @throws InternalServerErrorException
* AWS Elemental MediaConnect can't fulfill your request because it encountered an unexpected condition.
* @throws ForbiddenException
* You don't have the required permissions to perform this operation.
* @throws ServiceUnavailableException
* AWS Elemental MediaConnect is currently unavailable. Try again later.
* @throws TooManyRequestsException
* You have exceeded the service request rate limit for your AWS Elemental MediaConnect account.
* @sample AWSMediaConnect.CreateFlow
* @see AWS API
* Documentation
*/
CreateFlowResult createFlow(CreateFlowRequest createFlowRequest);
/**
* Creates a new gateway. The request must include at least one network (up to 4).
*
* @param createGatewayRequest
* Creates a new gateway. The request must include at least one network (up to 4).
* @return Result of the CreateGateway operation returned by the service.
* @throws CreateGateway420Exception
* Your account already contains the maximum number of gateways per account, per Region. For more
* information, contact AWS Customer Support.
* @throws BadRequestException
* The request that you submitted is not valid.
* @throws InternalServerErrorException
* AWS Elemental MediaConnect can't fulfill your request because it encountered an unexpected condition.
* @throws ForbiddenException
* You don't have the required permissions to perform this operation.
* @throws ServiceUnavailableException
* AWS Elemental MediaConnect is currently unavailable. Try again later.
* @throws TooManyRequestsException
* You have exceeded the service request rate limit for your AWS Elemental MediaConnect account.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @sample AWSMediaConnect.CreateGateway
* @see AWS API
* Documentation
*/
CreateGatewayResult createGateway(CreateGatewayRequest createGatewayRequest);
/**
* Deletes a bridge. Before you can delete a bridge, you must stop the bridge.
*
* @param deleteBridgeRequest
* @return Result of the DeleteBridge operation returned by the service.
* @throws BadRequestException
* The request that you submitted is not valid.
* @throws InternalServerErrorException
* AWS Elemental MediaConnect can't fulfill your request because it encountered an unexpected condition.
* @throws ForbiddenException
* You don't have the required permissions to perform this operation.
* @throws NotFoundException
* AWS Elemental MediaConnect did not find the resource that you specified in the request.
* @throws ServiceUnavailableException
* AWS Elemental MediaConnect is currently unavailable. Try again later.
* @throws TooManyRequestsException
* You have exceeded the service request rate limit for your AWS Elemental MediaConnect account.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @sample AWSMediaConnect.DeleteBridge
* @see AWS API
* Documentation
*/
DeleteBridgeResult deleteBridge(DeleteBridgeRequest deleteBridgeRequest);
/**
* Deletes a flow. Before you can delete a flow, you must stop the flow.
*
* @param deleteFlowRequest
* @return Result of the DeleteFlow operation returned by the service.
* @throws BadRequestException
* The request that you submitted is not valid.
* @throws InternalServerErrorException
* AWS Elemental MediaConnect can't fulfill your request because it encountered an unexpected condition.
* @throws ForbiddenException
* You don't have the required permissions to perform this operation.
* @throws NotFoundException
* AWS Elemental MediaConnect did not find the resource that you specified in the request.
* @throws ServiceUnavailableException
* AWS Elemental MediaConnect is currently unavailable. Try again later.
* @throws TooManyRequestsException
* You have exceeded the service request rate limit for your AWS Elemental MediaConnect account.
* @sample AWSMediaConnect.DeleteFlow
* @see AWS API
* Documentation
*/
DeleteFlowResult deleteFlow(DeleteFlowRequest deleteFlowRequest);
/**
* Deletes a gateway. Before you can delete a gateway, you must deregister its instances and delete its bridges.
*
* @param deleteGatewayRequest
* @return Result of the DeleteGateway operation returned by the service.
* @throws BadRequestException
* The request that you submitted is not valid.
* @throws InternalServerErrorException
* AWS Elemental MediaConnect can't fulfill your request because it encountered an unexpected condition.
* @throws ForbiddenException
* You don't have the required permissions to perform this operation.
* @throws NotFoundException
* AWS Elemental MediaConnect did not find the resource that you specified in the request.
* @throws ServiceUnavailableException
* AWS Elemental MediaConnect is currently unavailable. Try again later.
* @throws TooManyRequestsException
* You have exceeded the service request rate limit for your AWS Elemental MediaConnect account.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @sample AWSMediaConnect.DeleteGateway
* @see AWS API
* Documentation
*/
DeleteGatewayResult deleteGateway(DeleteGatewayRequest deleteGatewayRequest);
/**
* Deregisters an instance. Before you deregister an instance, all bridges running on the instance must be stopped.
* If you want to deregister an instance without stopping the bridges, you must use the --force option.
*
* @param deregisterGatewayInstanceRequest
* @return Result of the DeregisterGatewayInstance operation returned by the service.
* @throws BadRequestException
* The request that you submitted is not valid.
* @throws InternalServerErrorException
* AWS Elemental MediaConnect can't fulfill your request because it encountered an unexpected condition.
* @throws ForbiddenException
* You don't have the required permissions to perform this operation.
* @throws NotFoundException
* AWS Elemental MediaConnect did not find the resource that you specified in the request.
* @throws ServiceUnavailableException
* AWS Elemental MediaConnect is currently unavailable. Try again later.
* @throws TooManyRequestsException
* You have exceeded the service request rate limit for your AWS Elemental MediaConnect account.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @sample AWSMediaConnect.DeregisterGatewayInstance
* @see AWS API Documentation
*/
DeregisterGatewayInstanceResult deregisterGatewayInstance(DeregisterGatewayInstanceRequest deregisterGatewayInstanceRequest);
/**
* Displays the details of a bridge.
*
* @param describeBridgeRequest
* @return Result of the DescribeBridge operation returned by the service.
* @throws BadRequestException
* The request that you submitted is not valid.
* @throws InternalServerErrorException
* AWS Elemental MediaConnect can't fulfill your request because it encountered an unexpected condition.
* @throws ForbiddenException
* You don't have the required permissions to perform this operation.
* @throws NotFoundException
* AWS Elemental MediaConnect did not find the resource that you specified in the request.
* @throws ServiceUnavailableException
* AWS Elemental MediaConnect is currently unavailable. Try again later.
* @throws TooManyRequestsException
* You have exceeded the service request rate limit for your AWS Elemental MediaConnect account.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @sample AWSMediaConnect.DescribeBridge
* @see AWS
* API Documentation
*/
DescribeBridgeResult describeBridge(DescribeBridgeRequest describeBridgeRequest);
/**
* Displays the details of a flow. The response includes the flow ARN, name, and Availability Zone, as well as
* details about the source, outputs, and entitlements.
*
* @param describeFlowRequest
* @return Result of the DescribeFlow operation returned by the service.
* @throws BadRequestException
* The request that you submitted is not valid.
* @throws InternalServerErrorException
* AWS Elemental MediaConnect can't fulfill your request because it encountered an unexpected condition.
* @throws ForbiddenException
* You don't have the required permissions to perform this operation.
* @throws NotFoundException
* AWS Elemental MediaConnect did not find the resource that you specified in the request.
* @throws ServiceUnavailableException
* AWS Elemental MediaConnect is currently unavailable. Try again later.
* @throws TooManyRequestsException
* You have exceeded the service request rate limit for your AWS Elemental MediaConnect account.
* @sample AWSMediaConnect.DescribeFlow
* @see AWS API
* Documentation
*/
DescribeFlowResult describeFlow(DescribeFlowRequest describeFlowRequest);
/**
* Displays the details of a gateway. The response includes the gateway ARN, name, and CIDR blocks, as well as
* details about the networks.
*
* @param describeGatewayRequest
* @return Result of the DescribeGateway operation returned by the service.
* @throws BadRequestException
* The request that you submitted is not valid.
* @throws InternalServerErrorException
* AWS Elemental MediaConnect can't fulfill your request because it encountered an unexpected condition.
* @throws ForbiddenException
* You don't have the required permissions to perform this operation.
* @throws NotFoundException
* AWS Elemental MediaConnect did not find the resource that you specified in the request.
* @throws ServiceUnavailableException
* AWS Elemental MediaConnect is currently unavailable. Try again later.
* @throws TooManyRequestsException
* You have exceeded the service request rate limit for your AWS Elemental MediaConnect account.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @sample AWSMediaConnect.DescribeGateway
* @see AWS
* API Documentation
*/
DescribeGatewayResult describeGateway(DescribeGatewayRequest describeGatewayRequest);
/**
* Displays the details of an instance.
*
* @param describeGatewayInstanceRequest
* @return Result of the DescribeGatewayInstance operation returned by the service.
* @throws BadRequestException
* The request that you submitted is not valid.
* @throws InternalServerErrorException
* AWS Elemental MediaConnect can't fulfill your request because it encountered an unexpected condition.
* @throws ForbiddenException
* You don't have the required permissions to perform this operation.
* @throws NotFoundException
* AWS Elemental MediaConnect did not find the resource that you specified in the request.
* @throws ServiceUnavailableException
* AWS Elemental MediaConnect is currently unavailable. Try again later.
* @throws TooManyRequestsException
* You have exceeded the service request rate limit for your AWS Elemental MediaConnect account.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @sample AWSMediaConnect.DescribeGatewayInstance
* @see AWS API Documentation
*/
DescribeGatewayInstanceResult describeGatewayInstance(DescribeGatewayInstanceRequest describeGatewayInstanceRequest);
/**
* Displays the details of an offering. The response includes the offering description, duration, outbound
* bandwidth, price, and Amazon Resource Name (ARN).
*
* @param describeOfferingRequest
* @return Result of the DescribeOffering operation returned by the service.
* @throws NotFoundException
* AWS Elemental MediaConnect did not find the resource that you specified in the request.
* @throws ServiceUnavailableException
* AWS Elemental MediaConnect is currently unavailable. Try again later.
* @throws TooManyRequestsException
* You have exceeded the service request rate limit for your AWS Elemental MediaConnect account.
* @throws BadRequestException
* The request that you submitted is not valid.
* @throws InternalServerErrorException
* AWS Elemental MediaConnect can't fulfill your request because it encountered an unexpected condition.
* @sample AWSMediaConnect.DescribeOffering
* @see AWS
* API Documentation
*/
DescribeOfferingResult describeOffering(DescribeOfferingRequest describeOfferingRequest);
/**
* Displays the details of a reservation. The response includes the reservation name, state, start date and time,
* and the details of the offering that make up the rest of the reservation (such as price, duration, and outbound
* bandwidth).
*
* @param describeReservationRequest
* @return Result of the DescribeReservation operation returned by the service.
* @throws NotFoundException
* AWS Elemental MediaConnect did not find the resource that you specified in the request.
* @throws ServiceUnavailableException
* AWS Elemental MediaConnect is currently unavailable. Try again later.
* @throws TooManyRequestsException
* You have exceeded the service request rate limit for your AWS Elemental MediaConnect account.
* @throws BadRequestException
* The request that you submitted is not valid.
* @throws InternalServerErrorException
* AWS Elemental MediaConnect can't fulfill your request because it encountered an unexpected condition.
* @sample AWSMediaConnect.DescribeReservation
* @see AWS API Documentation
*/
DescribeReservationResult describeReservation(DescribeReservationRequest describeReservationRequest);
/**
* Grants entitlements to an existing flow.
*
* @param grantFlowEntitlementsRequest
* A request to grant entitlements on a flow.
* @return Result of the GrantFlowEntitlements operation returned by the service.
* @throws GrantFlowEntitlements420Exception
* AWS Elemental MediaConnect can't complete this request because this flow already has the maximum number
* of allowed entitlements (50). For more information, contact AWS Customer Support.
* @throws BadRequestException
* The request that you submitted is not valid.
* @throws InternalServerErrorException
* AWS Elemental MediaConnect can't fulfill your request because it encountered an unexpected condition.
* @throws ForbiddenException
* You don't have the required permissions to perform this operation.
* @throws NotFoundException
* AWS Elemental MediaConnect did not find the resource that you specified in the request.
* @throws ServiceUnavailableException
* AWS Elemental MediaConnect is currently unavailable. Try again later.
* @throws TooManyRequestsException
* You have exceeded the service request rate limit for your AWS Elemental MediaConnect account.
* @sample AWSMediaConnect.GrantFlowEntitlements
* @see AWS API Documentation
*/
GrantFlowEntitlementsResult grantFlowEntitlements(GrantFlowEntitlementsRequest grantFlowEntitlementsRequest);
/**
* Displays a list of bridges that are associated with this account and an optionally specified Arn. This request
* returns a paginated result.
*
* @param listBridgesRequest
* @return Result of the ListBridges operation returned by the service.
* @throws ServiceUnavailableException
* AWS Elemental MediaConnect is currently unavailable. Try again later.
* @throws TooManyRequestsException
* You have exceeded the service request rate limit for your AWS Elemental MediaConnect account.
* @throws BadRequestException
* The request that you submitted is not valid.
* @throws InternalServerErrorException
* AWS Elemental MediaConnect can't fulfill your request because it encountered an unexpected condition.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @sample AWSMediaConnect.ListBridges
* @see AWS API
* Documentation
*/
ListBridgesResult listBridges(ListBridgesRequest listBridgesRequest);
/**
* Displays a list of all entitlements that have been granted to this account. This request returns 20 results per
* page.
*
* @param listEntitlementsRequest
* @return Result of the ListEntitlements operation returned by the service.
* @throws ServiceUnavailableException
* AWS Elemental MediaConnect is currently unavailable. Try again later.
* @throws TooManyRequestsException
* You have exceeded the service request rate limit for your AWS Elemental MediaConnect account.
* @throws BadRequestException
* The request that you submitted is not valid.
* @throws InternalServerErrorException
* AWS Elemental MediaConnect can't fulfill your request because it encountered an unexpected condition.
* @sample AWSMediaConnect.ListEntitlements
* @see AWS
* API Documentation
*/
ListEntitlementsResult listEntitlements(ListEntitlementsRequest listEntitlementsRequest);
/**
* Displays a list of flows that are associated with this account. This request returns a paginated result.
*
* @param listFlowsRequest
* @return Result of the ListFlows operation returned by the service.
* @throws ServiceUnavailableException
* AWS Elemental MediaConnect is currently unavailable. Try again later.
* @throws TooManyRequestsException
* You have exceeded the service request rate limit for your AWS Elemental MediaConnect account.
* @throws BadRequestException
* The request that you submitted is not valid.
* @throws InternalServerErrorException
* AWS Elemental MediaConnect can't fulfill your request because it encountered an unexpected condition.
* @sample AWSMediaConnect.ListFlows
* @see AWS API
* Documentation
*/
ListFlowsResult listFlows(ListFlowsRequest listFlowsRequest);
/**
* Displays a list of instances associated with the AWS account. This request returns a paginated result. You can
* use the filterArn property to display only the instances associated with the selected Gateway Amazon Resource
* Name (ARN).
*
* @param listGatewayInstancesRequest
* @return Result of the ListGatewayInstances operation returned by the service.
* @throws ServiceUnavailableException
* AWS Elemental MediaConnect is currently unavailable. Try again later.
* @throws TooManyRequestsException
* You have exceeded the service request rate limit for your AWS Elemental MediaConnect account.
* @throws BadRequestException
* The request that you submitted is not valid.
* @throws InternalServerErrorException
* AWS Elemental MediaConnect can't fulfill your request because it encountered an unexpected condition.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @sample AWSMediaConnect.ListGatewayInstances
* @see AWS API Documentation
*/
ListGatewayInstancesResult listGatewayInstances(ListGatewayInstancesRequest listGatewayInstancesRequest);
/**
* Displays a list of gateways that are associated with this account. This request returns a paginated result.
*
* @param listGatewaysRequest
* @return Result of the ListGateways operation returned by the service.
* @throws ServiceUnavailableException
* AWS Elemental MediaConnect is currently unavailable. Try again later.
* @throws TooManyRequestsException
* You have exceeded the service request rate limit for your AWS Elemental MediaConnect account.
* @throws BadRequestException
* The request that you submitted is not valid.
* @throws InternalServerErrorException
* AWS Elemental MediaConnect can't fulfill your request because it encountered an unexpected condition.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @sample AWSMediaConnect.ListGateways
* @see AWS API
* Documentation
*/
ListGatewaysResult listGateways(ListGatewaysRequest listGatewaysRequest);
/**
* Displays a list of all offerings that are available to this account in the current AWS Region. If you have an
* active reservation (which means you've purchased an offering that has already started and hasn't expired yet),
* your account isn't eligible for other offerings.
*
* @param listOfferingsRequest
* @return Result of the ListOfferings operation returned by the service.
* @throws ServiceUnavailableException
* AWS Elemental MediaConnect is currently unavailable. Try again later.
* @throws TooManyRequestsException
* You have exceeded the service request rate limit for your AWS Elemental MediaConnect account.
* @throws BadRequestException
* The request that you submitted is not valid.
* @throws InternalServerErrorException
* AWS Elemental MediaConnect can't fulfill your request because it encountered an unexpected condition.
* @sample AWSMediaConnect.ListOfferings
* @see AWS API
* Documentation
*/
ListOfferingsResult listOfferings(ListOfferingsRequest listOfferingsRequest);
/**
* Displays a list of all reservations that have been purchased by this account in the current AWS Region. This list
* includes all reservations in all states (such as active and expired).
*
* @param listReservationsRequest
* @return Result of the ListReservations operation returned by the service.
* @throws ServiceUnavailableException
* AWS Elemental MediaConnect is currently unavailable. Try again later.
* @throws TooManyRequestsException
* You have exceeded the service request rate limit for your AWS Elemental MediaConnect account.
* @throws BadRequestException
* The request that you submitted is not valid.
* @throws InternalServerErrorException
* AWS Elemental MediaConnect can't fulfill your request because it encountered an unexpected condition.
* @sample AWSMediaConnect.ListReservations
* @see AWS
* API Documentation
*/
ListReservationsResult listReservations(ListReservationsRequest listReservationsRequest);
/**
* List all tags on an AWS Elemental MediaConnect resource
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws NotFoundException
* The requested resource was not found
* @throws BadRequestException
* The client performed an invalid request
* @throws InternalServerErrorException
* Internal service error
* @sample AWSMediaConnect.ListTagsForResource
* @see AWS API Documentation
*/
ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest);
/**
* Submits a request to purchase an offering. If you already have an active reservation, you can't purchase another
* offering.
*
* @param purchaseOfferingRequest
* A request to purchase a offering.
* @return Result of the PurchaseOffering operation returned by the service.
* @throws BadRequestException
* The request that you submitted is not valid.
* @throws InternalServerErrorException
* AWS Elemental MediaConnect can't fulfill your request because it encountered an unexpected condition.
* @throws ForbiddenException
* You don't have the required permissions to perform this operation.
* @throws NotFoundException
* AWS Elemental MediaConnect did not find the resource that you specified in the request.
* @throws ServiceUnavailableException
* AWS Elemental MediaConnect is currently unavailable. Try again later.
* @throws TooManyRequestsException
* You have exceeded the service request rate limit for your AWS Elemental MediaConnect account.
* @sample AWSMediaConnect.PurchaseOffering
* @see AWS
* API Documentation
*/
PurchaseOfferingResult purchaseOffering(PurchaseOfferingRequest purchaseOfferingRequest);
/**
* Removes an output from a bridge.
*
* @param removeBridgeOutputRequest
* @return Result of the RemoveBridgeOutput operation returned by the service.
* @throws BadRequestException
* The request that you submitted is not valid.
* @throws InternalServerErrorException
* AWS Elemental MediaConnect can't fulfill your request because it encountered an unexpected condition.
* @throws ForbiddenException
* You don't have the required permissions to perform this operation.
* @throws NotFoundException
* AWS Elemental MediaConnect did not find the resource that you specified in the request.
* @throws ServiceUnavailableException
* AWS Elemental MediaConnect is currently unavailable. Try again later.
* @throws TooManyRequestsException
* You have exceeded the service request rate limit for your AWS Elemental MediaConnect account.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @sample AWSMediaConnect.RemoveBridgeOutput
* @see AWS API Documentation
*/
RemoveBridgeOutputResult removeBridgeOutput(RemoveBridgeOutputRequest removeBridgeOutputRequest);
/**
* Removes a source from a bridge.
*
* @param removeBridgeSourceRequest
* @return Result of the RemoveBridgeSource operation returned by the service.
* @throws BadRequestException
* The request that you submitted is not valid.
* @throws InternalServerErrorException
* AWS Elemental MediaConnect can't fulfill your request because it encountered an unexpected condition.
* @throws ForbiddenException
* You don't have the required permissions to perform this operation.
* @throws NotFoundException
* AWS Elemental MediaConnect did not find the resource that you specified in the request.
* @throws ServiceUnavailableException
* AWS Elemental MediaConnect is currently unavailable. Try again later.
* @throws TooManyRequestsException
* You have exceeded the service request rate limit for your AWS Elemental MediaConnect account.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @sample AWSMediaConnect.RemoveBridgeSource
* @see AWS API Documentation
*/
RemoveBridgeSourceResult removeBridgeSource(RemoveBridgeSourceRequest removeBridgeSourceRequest);
/**
* Removes a media stream from a flow. This action is only available if the media stream is not associated with a
* source or output.
*
* @param removeFlowMediaStreamRequest
* @return Result of the RemoveFlowMediaStream operation returned by the service.
* @throws BadRequestException
* The request that you submitted is not valid.
* @throws InternalServerErrorException
* AWS Elemental MediaConnect can't fulfill your request because it encountered an unexpected condition.
* @throws ForbiddenException
* You don't have the required permissions to perform this operation.
* @throws NotFoundException
* AWS Elemental MediaConnect did not find the resource that you specified in the request.
* @throws ServiceUnavailableException
* AWS Elemental MediaConnect is currently unavailable. Try again later.
* @throws TooManyRequestsException
* You have exceeded the service request rate limit for your AWS Elemental MediaConnect account.
* @sample AWSMediaConnect.RemoveFlowMediaStream
* @see AWS API Documentation
*/
RemoveFlowMediaStreamResult removeFlowMediaStream(RemoveFlowMediaStreamRequest removeFlowMediaStreamRequest);
/**
* Removes an output from an existing flow. This request can be made only on an output that does not have an
* entitlement associated with it. If the output has an entitlement, you must revoke the entitlement instead. When
* an entitlement is revoked from a flow, the service automatically removes the associated output.
*
* @param removeFlowOutputRequest
* @return Result of the RemoveFlowOutput operation returned by the service.
* @throws BadRequestException
* The request that you submitted is not valid.
* @throws InternalServerErrorException
* AWS Elemental MediaConnect can't fulfill your request because it encountered an unexpected condition.
* @throws ForbiddenException
* You don't have the required permissions to perform this operation.
* @throws NotFoundException
* AWS Elemental MediaConnect did not find the resource that you specified in the request.
* @throws ServiceUnavailableException
* AWS Elemental MediaConnect is currently unavailable. Try again later.
* @throws TooManyRequestsException
* You have exceeded the service request rate limit for your AWS Elemental MediaConnect account.
* @sample AWSMediaConnect.RemoveFlowOutput
* @see AWS
* API Documentation
*/
RemoveFlowOutputResult removeFlowOutput(RemoveFlowOutputRequest removeFlowOutputRequest);
/**
* Removes a source from an existing flow. This request can be made only if there is more than one source on the
* flow.
*
* @param removeFlowSourceRequest
* @return Result of the RemoveFlowSource operation returned by the service.
* @throws BadRequestException
* The request that you submitted is not valid.
* @throws InternalServerErrorException
* AWS Elemental MediaConnect can't fulfill your request because it encountered an unexpected condition.
* @throws ForbiddenException
* You don't have the required permissions to perform this operation.
* @throws NotFoundException
* AWS Elemental MediaConnect did not find the resource that you specified in the request.
* @throws ServiceUnavailableException
* AWS Elemental MediaConnect is currently unavailable. Try again later.
* @throws TooManyRequestsException
* You have exceeded the service request rate limit for your AWS Elemental MediaConnect account.
* @sample AWSMediaConnect.RemoveFlowSource
* @see AWS
* API Documentation
*/
RemoveFlowSourceResult removeFlowSource(RemoveFlowSourceRequest removeFlowSourceRequest);
/**
* Removes a VPC Interface from an existing flow. This request can be made only on a VPC interface that does not
* have a Source or Output associated with it. If the VPC interface is referenced by a Source or Output, you must
* first delete or update the Source or Output to no longer reference the VPC interface.
*
* @param removeFlowVpcInterfaceRequest
* @return Result of the RemoveFlowVpcInterface operation returned by the service.
* @throws BadRequestException
* The request that you submitted is not valid.
* @throws InternalServerErrorException
* AWS Elemental MediaConnect can't fulfill your request because it encountered an unexpected condition.
* @throws ForbiddenException
* You don't have the required permissions to perform this operation.
* @throws NotFoundException
* AWS Elemental MediaConnect did not find the resource that you specified in the request.
* @throws ServiceUnavailableException
* AWS Elemental MediaConnect is currently unavailable. Try again later.
* @throws TooManyRequestsException
* You have exceeded the service request rate limit for your AWS Elemental MediaConnect account.
* @sample AWSMediaConnect.RemoveFlowVpcInterface
* @see AWS API Documentation
*/
RemoveFlowVpcInterfaceResult removeFlowVpcInterface(RemoveFlowVpcInterfaceRequest removeFlowVpcInterfaceRequest);
/**
* Revokes an entitlement from a flow. Once an entitlement is revoked, the content becomes unavailable to the
* subscriber and the associated output is removed.
*
* @param revokeFlowEntitlementRequest
* @return Result of the RevokeFlowEntitlement operation returned by the service.
* @throws BadRequestException
* The request that you submitted is not valid.
* @throws InternalServerErrorException
* AWS Elemental MediaConnect can't fulfill your request because it encountered an unexpected condition.
* @throws ForbiddenException
* You don't have the required permissions to perform this operation.
* @throws NotFoundException
* AWS Elemental MediaConnect did not find the resource that you specified in the request.
* @throws ServiceUnavailableException
* AWS Elemental MediaConnect is currently unavailable. Try again later.
* @throws TooManyRequestsException
* You have exceeded the service request rate limit for your AWS Elemental MediaConnect account.
* @sample AWSMediaConnect.RevokeFlowEntitlement
* @see AWS API Documentation
*/
RevokeFlowEntitlementResult revokeFlowEntitlement(RevokeFlowEntitlementRequest revokeFlowEntitlementRequest);
/**
* Starts a flow.
*
* @param startFlowRequest
* @return Result of the StartFlow operation returned by the service.
* @throws BadRequestException
* The request that you submitted is not valid.
* @throws InternalServerErrorException
* AWS Elemental MediaConnect can't fulfill your request because it encountered an unexpected condition.
* @throws ForbiddenException
* You don't have the required permissions to perform this operation.
* @throws NotFoundException
* AWS Elemental MediaConnect did not find the resource that you specified in the request.
* @throws ServiceUnavailableException
* AWS Elemental MediaConnect is currently unavailable. Try again later.
* @throws TooManyRequestsException
* You have exceeded the service request rate limit for your AWS Elemental MediaConnect account.
* @sample AWSMediaConnect.StartFlow
* @see AWS API
* Documentation
*/
StartFlowResult startFlow(StartFlowRequest startFlowRequest);
/**
* Stops a flow.
*
* @param stopFlowRequest
* @return Result of the StopFlow operation returned by the service.
* @throws BadRequestException
* The request that you submitted is not valid.
* @throws InternalServerErrorException
* AWS Elemental MediaConnect can't fulfill your request because it encountered an unexpected condition.
* @throws ForbiddenException
* You don't have the required permissions to perform this operation.
* @throws NotFoundException
* AWS Elemental MediaConnect did not find the resource that you specified in the request.
* @throws ServiceUnavailableException
* AWS Elemental MediaConnect is currently unavailable. Try again later.
* @throws TooManyRequestsException
* You have exceeded the service request rate limit for your AWS Elemental MediaConnect account.
* @sample AWSMediaConnect.StopFlow
* @see AWS API
* Documentation
*/
StopFlowResult stopFlow(StopFlowRequest stopFlowRequest);
/**
* Associates the specified tags to a resource with the specified resourceArn. If existing tags on a resource are
* not specified in the request parameters, they are not changed. When a resource is deleted, the tags associated
* with that resource are deleted as well.
*
* @param tagResourceRequest
* The tags to add to the resource. A tag is an array of key-value pairs. Tag keys can have a maximum
* character length of 128 characters, and tag values can have a maximum length of 256 characters.
* @return Result of the TagResource operation returned by the service.
* @throws NotFoundException
* The requested resource was not found
* @throws BadRequestException
* The client performed an invalid request
* @throws InternalServerErrorException
* Internal service error
* @sample AWSMediaConnect.TagResource
* @see AWS API
* Documentation
*/
TagResourceResult tagResource(TagResourceRequest tagResourceRequest);
/**
* Deletes specified tags from a resource.
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws NotFoundException
* The requested resource was not found
* @throws BadRequestException
* The client performed an invalid request
* @throws InternalServerErrorException
* Internal service error
* @sample AWSMediaConnect.UntagResource
* @see AWS API
* Documentation
*/
UntagResourceResult untagResource(UntagResourceRequest untagResourceRequest);
/**
* Updates the bridge
*
* @param updateBridgeRequest
* A request to update the bridge.
* @return Result of the UpdateBridge operation returned by the service.
* @throws BadRequestException
* The request that you submitted is not valid.
* @throws InternalServerErrorException
* AWS Elemental MediaConnect can't fulfill your request because it encountered an unexpected condition.
* @throws ForbiddenException
* You don't have the required permissions to perform this operation.
* @throws NotFoundException
* AWS Elemental MediaConnect did not find the resource that you specified in the request.
* @throws ServiceUnavailableException
* AWS Elemental MediaConnect is currently unavailable. Try again later.
* @throws TooManyRequestsException
* You have exceeded the service request rate limit for your AWS Elemental MediaConnect account.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @sample AWSMediaConnect.UpdateBridge
* @see AWS API
* Documentation
*/
UpdateBridgeResult updateBridge(UpdateBridgeRequest updateBridgeRequest);
/**
* Updates an existing bridge output.
*
* @param updateBridgeOutputRequest
* The fields that you want to update in the bridge output.
* @return Result of the UpdateBridgeOutput operation returned by the service.
* @throws BadRequestException
* The request that you submitted is not valid.
* @throws InternalServerErrorException
* AWS Elemental MediaConnect can't fulfill your request because it encountered an unexpected condition.
* @throws ForbiddenException
* You don't have the required permissions to perform this operation.
* @throws NotFoundException
* AWS Elemental MediaConnect did not find the resource that you specified in the request.
* @throws ServiceUnavailableException
* AWS Elemental MediaConnect is currently unavailable. Try again later.
* @throws TooManyRequestsException
* You have exceeded the service request rate limit for your AWS Elemental MediaConnect account.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @sample AWSMediaConnect.UpdateBridgeOutput
* @see AWS API Documentation
*/
UpdateBridgeOutputResult updateBridgeOutput(UpdateBridgeOutputRequest updateBridgeOutputRequest);
/**
* Updates an existing bridge source.
*
* @param updateBridgeSourceRequest
* The fields that you want to update in the bridge source.
* @return Result of the UpdateBridgeSource operation returned by the service.
* @throws BadRequestException
* The request that you submitted is not valid.
* @throws InternalServerErrorException
* AWS Elemental MediaConnect can't fulfill your request because it encountered an unexpected condition.
* @throws ForbiddenException
* You don't have the required permissions to perform this operation.
* @throws NotFoundException
* AWS Elemental MediaConnect did not find the resource that you specified in the request.
* @throws ServiceUnavailableException
* AWS Elemental MediaConnect is currently unavailable. Try again later.
* @throws TooManyRequestsException
* You have exceeded the service request rate limit for your AWS Elemental MediaConnect account.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @sample AWSMediaConnect.UpdateBridgeSource
* @see AWS API Documentation
*/
UpdateBridgeSourceResult updateBridgeSource(UpdateBridgeSourceRequest updateBridgeSourceRequest);
/**
* Updates the bridge state
*
* @param updateBridgeStateRequest
* A request to update the bridge state.
* @return Result of the UpdateBridgeState operation returned by the service.
* @throws BadRequestException
* The request that you submitted is not valid.
* @throws InternalServerErrorException
* AWS Elemental MediaConnect can't fulfill your request because it encountered an unexpected condition.
* @throws ForbiddenException
* You don't have the required permissions to perform this operation.
* @throws NotFoundException
* AWS Elemental MediaConnect did not find the resource that you specified in the request.
* @throws ServiceUnavailableException
* AWS Elemental MediaConnect is currently unavailable. Try again later.
* @throws TooManyRequestsException
* You have exceeded the service request rate limit for your AWS Elemental MediaConnect account.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @sample AWSMediaConnect.UpdateBridgeState
* @see AWS
* API Documentation
*/
UpdateBridgeStateResult updateBridgeState(UpdateBridgeStateRequest updateBridgeStateRequest);
/**
* Updates flow
*
* @param updateFlowRequest
* A request to update flow.
* @return Result of the UpdateFlow operation returned by the service.
* @throws BadRequestException
* The request that you submitted is not valid.
* @throws InternalServerErrorException
* AWS Elemental MediaConnect can't fulfill your request because it encountered an unexpected condition.
* @throws ForbiddenException
* You don't have the required permissions to perform this operation.
* @throws NotFoundException
* AWS Elemental MediaConnect did not find the resource that you specified in the request.
* @throws ServiceUnavailableException
* AWS Elemental MediaConnect is currently unavailable. Try again later.
* @throws TooManyRequestsException
* You have exceeded the service request rate limit for your AWS Elemental MediaConnect account.
* @sample AWSMediaConnect.UpdateFlow
* @see AWS API
* Documentation
*/
UpdateFlowResult updateFlow(UpdateFlowRequest updateFlowRequest);
/**
* You can change an entitlement's description, subscribers, and encryption. If you change the subscribers, the
* service will remove the outputs that are are used by the subscribers that are removed.
*
* @param updateFlowEntitlementRequest
* The entitlement fields that you want to update.
* @return Result of the UpdateFlowEntitlement operation returned by the service.
* @throws BadRequestException
* The request that you submitted is not valid.
* @throws InternalServerErrorException
* AWS Elemental MediaConnect can't fulfill your request because it encountered an unexpected condition.
* @throws ForbiddenException
* You don't have the required permissions to perform this operation.
* @throws NotFoundException
* AWS Elemental MediaConnect did not find the resource that you specified in the request.
* @throws ServiceUnavailableException
* AWS Elemental MediaConnect is currently unavailable. Try again later.
* @throws TooManyRequestsException
* You have exceeded the service request rate limit for your AWS Elemental MediaConnect account.
* @sample AWSMediaConnect.UpdateFlowEntitlement
* @see AWS API Documentation
*/
UpdateFlowEntitlementResult updateFlowEntitlement(UpdateFlowEntitlementRequest updateFlowEntitlementRequest);
/**
* Updates an existing media stream.
*
* @param updateFlowMediaStreamRequest
* The fields that you want to update in the media stream.
* @return Result of the UpdateFlowMediaStream operation returned by the service.
* @throws BadRequestException
* The request that you submitted is not valid.
* @throws InternalServerErrorException
* AWS Elemental MediaConnect can't fulfill your request because it encountered an unexpected condition.
* @throws ForbiddenException
* You don't have the required permissions to perform this operation.
* @throws NotFoundException
* AWS Elemental MediaConnect did not find the resource that you specified in the request.
* @throws ServiceUnavailableException
* AWS Elemental MediaConnect is currently unavailable. Try again later.
* @throws TooManyRequestsException
* You have exceeded the service request rate limit for your AWS Elemental MediaConnect account.
* @sample AWSMediaConnect.UpdateFlowMediaStream
* @see AWS API Documentation
*/
UpdateFlowMediaStreamResult updateFlowMediaStream(UpdateFlowMediaStreamRequest updateFlowMediaStreamRequest);
/**
* Updates an existing flow output.
*
* @param updateFlowOutputRequest
* The fields that you want to update in the output.
* @return Result of the UpdateFlowOutput operation returned by the service.
* @throws BadRequestException
* The request that you submitted is not valid.
* @throws InternalServerErrorException
* AWS Elemental MediaConnect can't fulfill your request because it encountered an unexpected condition.
* @throws ForbiddenException
* You don't have the required permissions to perform this operation.
* @throws NotFoundException
* AWS Elemental MediaConnect did not find the resource that you specified in the request.
* @throws ServiceUnavailableException
* AWS Elemental MediaConnect is currently unavailable. Try again later.
* @throws TooManyRequestsException
* You have exceeded the service request rate limit for your AWS Elemental MediaConnect account.
* @sample AWSMediaConnect.UpdateFlowOutput
* @see AWS
* API Documentation
*/
UpdateFlowOutputResult updateFlowOutput(UpdateFlowOutputRequest updateFlowOutputRequest);
/**
* Updates the source of a flow.
*
* @param updateFlowSourceRequest
* A request to update the source of a flow.
* @return Result of the UpdateFlowSource operation returned by the service.
* @throws BadRequestException
* The request that you submitted is not valid.
* @throws InternalServerErrorException
* AWS Elemental MediaConnect can't fulfill your request because it encountered an unexpected condition.
* @throws ForbiddenException
* You don't have the required permissions to perform this operation.
* @throws NotFoundException
* AWS Elemental MediaConnect did not find the resource that you specified in the request.
* @throws ServiceUnavailableException
* AWS Elemental MediaConnect is currently unavailable. Try again later.
* @throws TooManyRequestsException
* You have exceeded the service request rate limit for your AWS Elemental MediaConnect account.
* @sample AWSMediaConnect.UpdateFlowSource
* @see AWS
* API Documentation
*/
UpdateFlowSourceResult updateFlowSource(UpdateFlowSourceRequest updateFlowSourceRequest);
/**
* Updates the configuration of an existing Gateway Instance.
*
* @param updateGatewayInstanceRequest
* A request to update gateway instance state.
* @return Result of the UpdateGatewayInstance operation returned by the service.
* @throws BadRequestException
* The request that you submitted is not valid.
* @throws InternalServerErrorException
* AWS Elemental MediaConnect can't fulfill your request because it encountered an unexpected condition.
* @throws ForbiddenException
* You don't have the required permissions to perform this operation.
* @throws NotFoundException
* AWS Elemental MediaConnect did not find the resource that you specified in the request.
* @throws ServiceUnavailableException
* AWS Elemental MediaConnect is currently unavailable. Try again later.
* @throws TooManyRequestsException
* You have exceeded the service request rate limit for your AWS Elemental MediaConnect account.
* @throws ConflictException
* The request could not be completed due to a conflict with the current state of the target resource.
* @sample AWSMediaConnect.UpdateGatewayInstance
* @see AWS API Documentation
*/
UpdateGatewayInstanceResult updateGatewayInstance(UpdateGatewayInstanceRequest updateGatewayInstanceRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
AWSMediaConnectWaiters waiters();
}