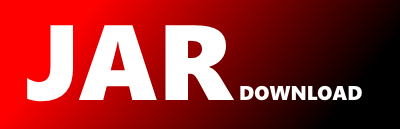
com.amazonaws.services.mediaconnect.AWSMediaConnectAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-mediaconnect Show documentation
/*
* Copyright 2018-2023 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.mediaconnect;
import javax.annotation.Generated;
import com.amazonaws.services.mediaconnect.model.*;
/**
* Interface for accessing AWS MediaConnect asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.mediaconnect.AbstractAWSMediaConnectAsync} instead.
*
*
* API for AWS Elemental MediaConnect
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSMediaConnectAsync extends AWSMediaConnect {
/**
* Adds outputs to an existing bridge.
*
* @param addBridgeOutputsRequest
* A request to add outputs to the specified bridge.
* @return A Java Future containing the result of the AddBridgeOutputs operation returned by the service.
* @sample AWSMediaConnectAsync.AddBridgeOutputs
* @see AWS
* API Documentation
*/
java.util.concurrent.Future addBridgeOutputsAsync(AddBridgeOutputsRequest addBridgeOutputsRequest);
/**
* Adds outputs to an existing bridge.
*
* @param addBridgeOutputsRequest
* A request to add outputs to the specified bridge.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AddBridgeOutputs operation returned by the service.
* @sample AWSMediaConnectAsyncHandler.AddBridgeOutputs
* @see AWS
* API Documentation
*/
java.util.concurrent.Future addBridgeOutputsAsync(AddBridgeOutputsRequest addBridgeOutputsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Adds sources to an existing bridge.
*
* @param addBridgeSourcesRequest
* A request to add sources to the specified bridge.
* @return A Java Future containing the result of the AddBridgeSources operation returned by the service.
* @sample AWSMediaConnectAsync.AddBridgeSources
* @see AWS
* API Documentation
*/
java.util.concurrent.Future addBridgeSourcesAsync(AddBridgeSourcesRequest addBridgeSourcesRequest);
/**
* Adds sources to an existing bridge.
*
* @param addBridgeSourcesRequest
* A request to add sources to the specified bridge.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AddBridgeSources operation returned by the service.
* @sample AWSMediaConnectAsyncHandler.AddBridgeSources
* @see AWS
* API Documentation
*/
java.util.concurrent.Future addBridgeSourcesAsync(AddBridgeSourcesRequest addBridgeSourcesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Adds media streams to an existing flow. After you add a media stream to a flow, you can associate it with a
* source and/or an output that uses the ST 2110 JPEG XS or CDI protocol.
*
* @param addFlowMediaStreamsRequest
* A request to add media streams to the flow.
* @return A Java Future containing the result of the AddFlowMediaStreams operation returned by the service.
* @sample AWSMediaConnectAsync.AddFlowMediaStreams
* @see AWS API Documentation
*/
java.util.concurrent.Future addFlowMediaStreamsAsync(AddFlowMediaStreamsRequest addFlowMediaStreamsRequest);
/**
* Adds media streams to an existing flow. After you add a media stream to a flow, you can associate it with a
* source and/or an output that uses the ST 2110 JPEG XS or CDI protocol.
*
* @param addFlowMediaStreamsRequest
* A request to add media streams to the flow.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AddFlowMediaStreams operation returned by the service.
* @sample AWSMediaConnectAsyncHandler.AddFlowMediaStreams
* @see AWS API Documentation
*/
java.util.concurrent.Future addFlowMediaStreamsAsync(AddFlowMediaStreamsRequest addFlowMediaStreamsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Adds outputs to an existing flow. You can create up to 50 outputs per flow.
*
* @param addFlowOutputsRequest
* A request to add outputs to the specified flow.
* @return A Java Future containing the result of the AddFlowOutputs operation returned by the service.
* @sample AWSMediaConnectAsync.AddFlowOutputs
* @see AWS
* API Documentation
*/
java.util.concurrent.Future addFlowOutputsAsync(AddFlowOutputsRequest addFlowOutputsRequest);
/**
* Adds outputs to an existing flow. You can create up to 50 outputs per flow.
*
* @param addFlowOutputsRequest
* A request to add outputs to the specified flow.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AddFlowOutputs operation returned by the service.
* @sample AWSMediaConnectAsyncHandler.AddFlowOutputs
* @see AWS
* API Documentation
*/
java.util.concurrent.Future addFlowOutputsAsync(AddFlowOutputsRequest addFlowOutputsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Adds Sources to flow
*
* @param addFlowSourcesRequest
* A request to add sources to the flow.
* @return A Java Future containing the result of the AddFlowSources operation returned by the service.
* @sample AWSMediaConnectAsync.AddFlowSources
* @see AWS
* API Documentation
*/
java.util.concurrent.Future addFlowSourcesAsync(AddFlowSourcesRequest addFlowSourcesRequest);
/**
* Adds Sources to flow
*
* @param addFlowSourcesRequest
* A request to add sources to the flow.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AddFlowSources operation returned by the service.
* @sample AWSMediaConnectAsyncHandler.AddFlowSources
* @see AWS
* API Documentation
*/
java.util.concurrent.Future addFlowSourcesAsync(AddFlowSourcesRequest addFlowSourcesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Adds VPC interfaces to flow
*
* @param addFlowVpcInterfacesRequest
* A request to add VPC interfaces to the flow.
* @return A Java Future containing the result of the AddFlowVpcInterfaces operation returned by the service.
* @sample AWSMediaConnectAsync.AddFlowVpcInterfaces
* @see AWS API Documentation
*/
java.util.concurrent.Future addFlowVpcInterfacesAsync(AddFlowVpcInterfacesRequest addFlowVpcInterfacesRequest);
/**
* Adds VPC interfaces to flow
*
* @param addFlowVpcInterfacesRequest
* A request to add VPC interfaces to the flow.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AddFlowVpcInterfaces operation returned by the service.
* @sample AWSMediaConnectAsyncHandler.AddFlowVpcInterfaces
* @see AWS API Documentation
*/
java.util.concurrent.Future addFlowVpcInterfacesAsync(AddFlowVpcInterfacesRequest addFlowVpcInterfacesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Creates a new bridge. The request must include one source.
*
* @param createBridgeRequest
* Creates a new bridge. The request must include one source.
* @return A Java Future containing the result of the CreateBridge operation returned by the service.
* @sample AWSMediaConnectAsync.CreateBridge
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createBridgeAsync(CreateBridgeRequest createBridgeRequest);
/**
* Creates a new bridge. The request must include one source.
*
* @param createBridgeRequest
* Creates a new bridge. The request must include one source.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateBridge operation returned by the service.
* @sample AWSMediaConnectAsyncHandler.CreateBridge
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createBridgeAsync(CreateBridgeRequest createBridgeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Creates a new flow. The request must include one source. The request optionally can include outputs (up to 50)
* and entitlements (up to 50).
*
* @param createFlowRequest
* Creates a new flow. The request must include one source. The request optionally can include outputs (up to
* 50) and entitlements (up to 50).
* @return A Java Future containing the result of the CreateFlow operation returned by the service.
* @sample AWSMediaConnectAsync.CreateFlow
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createFlowAsync(CreateFlowRequest createFlowRequest);
/**
* Creates a new flow. The request must include one source. The request optionally can include outputs (up to 50)
* and entitlements (up to 50).
*
* @param createFlowRequest
* Creates a new flow. The request must include one source. The request optionally can include outputs (up to
* 50) and entitlements (up to 50).
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateFlow operation returned by the service.
* @sample AWSMediaConnectAsyncHandler.CreateFlow
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createFlowAsync(CreateFlowRequest createFlowRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Creates a new gateway. The request must include at least one network (up to 4).
*
* @param createGatewayRequest
* Creates a new gateway. The request must include at least one network (up to 4).
* @return A Java Future containing the result of the CreateGateway operation returned by the service.
* @sample AWSMediaConnectAsync.CreateGateway
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createGatewayAsync(CreateGatewayRequest createGatewayRequest);
/**
* Creates a new gateway. The request must include at least one network (up to 4).
*
* @param createGatewayRequest
* Creates a new gateway. The request must include at least one network (up to 4).
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateGateway operation returned by the service.
* @sample AWSMediaConnectAsyncHandler.CreateGateway
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createGatewayAsync(CreateGatewayRequest createGatewayRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Deletes a bridge. Before you can delete a bridge, you must stop the bridge.
*
* @param deleteBridgeRequest
* @return A Java Future containing the result of the DeleteBridge operation returned by the service.
* @sample AWSMediaConnectAsync.DeleteBridge
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteBridgeAsync(DeleteBridgeRequest deleteBridgeRequest);
/**
* Deletes a bridge. Before you can delete a bridge, you must stop the bridge.
*
* @param deleteBridgeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteBridge operation returned by the service.
* @sample AWSMediaConnectAsyncHandler.DeleteBridge
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteBridgeAsync(DeleteBridgeRequest deleteBridgeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Deletes a flow. Before you can delete a flow, you must stop the flow.
*
* @param deleteFlowRequest
* @return A Java Future containing the result of the DeleteFlow operation returned by the service.
* @sample AWSMediaConnectAsync.DeleteFlow
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteFlowAsync(DeleteFlowRequest deleteFlowRequest);
/**
* Deletes a flow. Before you can delete a flow, you must stop the flow.
*
* @param deleteFlowRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteFlow operation returned by the service.
* @sample AWSMediaConnectAsyncHandler.DeleteFlow
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteFlowAsync(DeleteFlowRequest deleteFlowRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Deletes a gateway. Before you can delete a gateway, you must deregister its instances and delete its bridges.
*
* @param deleteGatewayRequest
* @return A Java Future containing the result of the DeleteGateway operation returned by the service.
* @sample AWSMediaConnectAsync.DeleteGateway
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteGatewayAsync(DeleteGatewayRequest deleteGatewayRequest);
/**
* Deletes a gateway. Before you can delete a gateway, you must deregister its instances and delete its bridges.
*
* @param deleteGatewayRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteGateway operation returned by the service.
* @sample AWSMediaConnectAsyncHandler.DeleteGateway
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteGatewayAsync(DeleteGatewayRequest deleteGatewayRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Deregisters an instance. Before you deregister an instance, all bridges running on the instance must be stopped.
* If you want to deregister an instance without stopping the bridges, you must use the --force option.
*
* @param deregisterGatewayInstanceRequest
* @return A Java Future containing the result of the DeregisterGatewayInstance operation returned by the service.
* @sample AWSMediaConnectAsync.DeregisterGatewayInstance
* @see AWS API Documentation
*/
java.util.concurrent.Future deregisterGatewayInstanceAsync(
DeregisterGatewayInstanceRequest deregisterGatewayInstanceRequest);
/**
* Deregisters an instance. Before you deregister an instance, all bridges running on the instance must be stopped.
* If you want to deregister an instance without stopping the bridges, you must use the --force option.
*
* @param deregisterGatewayInstanceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeregisterGatewayInstance operation returned by the service.
* @sample AWSMediaConnectAsyncHandler.DeregisterGatewayInstance
* @see AWS API Documentation
*/
java.util.concurrent.Future deregisterGatewayInstanceAsync(
DeregisterGatewayInstanceRequest deregisterGatewayInstanceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Displays the details of a bridge.
*
* @param describeBridgeRequest
* @return A Java Future containing the result of the DescribeBridge operation returned by the service.
* @sample AWSMediaConnectAsync.DescribeBridge
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeBridgeAsync(DescribeBridgeRequest describeBridgeRequest);
/**
* Displays the details of a bridge.
*
* @param describeBridgeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeBridge operation returned by the service.
* @sample AWSMediaConnectAsyncHandler.DescribeBridge
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeBridgeAsync(DescribeBridgeRequest describeBridgeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Displays the details of a flow. The response includes the flow ARN, name, and Availability Zone, as well as
* details about the source, outputs, and entitlements.
*
* @param describeFlowRequest
* @return A Java Future containing the result of the DescribeFlow operation returned by the service.
* @sample AWSMediaConnectAsync.DescribeFlow
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeFlowAsync(DescribeFlowRequest describeFlowRequest);
/**
* Displays the details of a flow. The response includes the flow ARN, name, and Availability Zone, as well as
* details about the source, outputs, and entitlements.
*
* @param describeFlowRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeFlow operation returned by the service.
* @sample AWSMediaConnectAsyncHandler.DescribeFlow
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeFlowAsync(DescribeFlowRequest describeFlowRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Displays the details of a gateway. The response includes the gateway ARN, name, and CIDR blocks, as well as
* details about the networks.
*
* @param describeGatewayRequest
* @return A Java Future containing the result of the DescribeGateway operation returned by the service.
* @sample AWSMediaConnectAsync.DescribeGateway
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeGatewayAsync(DescribeGatewayRequest describeGatewayRequest);
/**
* Displays the details of a gateway. The response includes the gateway ARN, name, and CIDR blocks, as well as
* details about the networks.
*
* @param describeGatewayRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeGateway operation returned by the service.
* @sample AWSMediaConnectAsyncHandler.DescribeGateway
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeGatewayAsync(DescribeGatewayRequest describeGatewayRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Displays the details of an instance.
*
* @param describeGatewayInstanceRequest
* @return A Java Future containing the result of the DescribeGatewayInstance operation returned by the service.
* @sample AWSMediaConnectAsync.DescribeGatewayInstance
* @see AWS API Documentation
*/
java.util.concurrent.Future describeGatewayInstanceAsync(DescribeGatewayInstanceRequest describeGatewayInstanceRequest);
/**
* Displays the details of an instance.
*
* @param describeGatewayInstanceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeGatewayInstance operation returned by the service.
* @sample AWSMediaConnectAsyncHandler.DescribeGatewayInstance
* @see AWS API Documentation
*/
java.util.concurrent.Future describeGatewayInstanceAsync(DescribeGatewayInstanceRequest describeGatewayInstanceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Displays the details of an offering. The response includes the offering description, duration, outbound
* bandwidth, price, and Amazon Resource Name (ARN).
*
* @param describeOfferingRequest
* @return A Java Future containing the result of the DescribeOffering operation returned by the service.
* @sample AWSMediaConnectAsync.DescribeOffering
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeOfferingAsync(DescribeOfferingRequest describeOfferingRequest);
/**
* Displays the details of an offering. The response includes the offering description, duration, outbound
* bandwidth, price, and Amazon Resource Name (ARN).
*
* @param describeOfferingRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeOffering operation returned by the service.
* @sample AWSMediaConnectAsyncHandler.DescribeOffering
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeOfferingAsync(DescribeOfferingRequest describeOfferingRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Displays the details of a reservation. The response includes the reservation name, state, start date and time,
* and the details of the offering that make up the rest of the reservation (such as price, duration, and outbound
* bandwidth).
*
* @param describeReservationRequest
* @return A Java Future containing the result of the DescribeReservation operation returned by the service.
* @sample AWSMediaConnectAsync.DescribeReservation
* @see AWS API Documentation
*/
java.util.concurrent.Future describeReservationAsync(DescribeReservationRequest describeReservationRequest);
/**
* Displays the details of a reservation. The response includes the reservation name, state, start date and time,
* and the details of the offering that make up the rest of the reservation (such as price, duration, and outbound
* bandwidth).
*
* @param describeReservationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeReservation operation returned by the service.
* @sample AWSMediaConnectAsyncHandler.DescribeReservation
* @see AWS API Documentation
*/
java.util.concurrent.Future describeReservationAsync(DescribeReservationRequest describeReservationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Grants entitlements to an existing flow.
*
* @param grantFlowEntitlementsRequest
* A request to grant entitlements on a flow.
* @return A Java Future containing the result of the GrantFlowEntitlements operation returned by the service.
* @sample AWSMediaConnectAsync.GrantFlowEntitlements
* @see AWS API Documentation
*/
java.util.concurrent.Future grantFlowEntitlementsAsync(GrantFlowEntitlementsRequest grantFlowEntitlementsRequest);
/**
* Grants entitlements to an existing flow.
*
* @param grantFlowEntitlementsRequest
* A request to grant entitlements on a flow.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GrantFlowEntitlements operation returned by the service.
* @sample AWSMediaConnectAsyncHandler.GrantFlowEntitlements
* @see AWS API Documentation
*/
java.util.concurrent.Future grantFlowEntitlementsAsync(GrantFlowEntitlementsRequest grantFlowEntitlementsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Displays a list of bridges that are associated with this account and an optionally specified Arn. This request
* returns a paginated result.
*
* @param listBridgesRequest
* @return A Java Future containing the result of the ListBridges operation returned by the service.
* @sample AWSMediaConnectAsync.ListBridges
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listBridgesAsync(ListBridgesRequest listBridgesRequest);
/**
* Displays a list of bridges that are associated with this account and an optionally specified Arn. This request
* returns a paginated result.
*
* @param listBridgesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListBridges operation returned by the service.
* @sample AWSMediaConnectAsyncHandler.ListBridges
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listBridgesAsync(ListBridgesRequest listBridgesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Displays a list of all entitlements that have been granted to this account. This request returns 20 results per
* page.
*
* @param listEntitlementsRequest
* @return A Java Future containing the result of the ListEntitlements operation returned by the service.
* @sample AWSMediaConnectAsync.ListEntitlements
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listEntitlementsAsync(ListEntitlementsRequest listEntitlementsRequest);
/**
* Displays a list of all entitlements that have been granted to this account. This request returns 20 results per
* page.
*
* @param listEntitlementsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListEntitlements operation returned by the service.
* @sample AWSMediaConnectAsyncHandler.ListEntitlements
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listEntitlementsAsync(ListEntitlementsRequest listEntitlementsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Displays a list of flows that are associated with this account. This request returns a paginated result.
*
* @param listFlowsRequest
* @return A Java Future containing the result of the ListFlows operation returned by the service.
* @sample AWSMediaConnectAsync.ListFlows
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listFlowsAsync(ListFlowsRequest listFlowsRequest);
/**
* Displays a list of flows that are associated with this account. This request returns a paginated result.
*
* @param listFlowsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListFlows operation returned by the service.
* @sample AWSMediaConnectAsyncHandler.ListFlows
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listFlowsAsync(ListFlowsRequest listFlowsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Displays a list of instances associated with the AWS account. This request returns a paginated result. You can
* use the filterArn property to display only the instances associated with the selected Gateway Amazon Resource
* Name (ARN).
*
* @param listGatewayInstancesRequest
* @return A Java Future containing the result of the ListGatewayInstances operation returned by the service.
* @sample AWSMediaConnectAsync.ListGatewayInstances
* @see AWS API Documentation
*/
java.util.concurrent.Future listGatewayInstancesAsync(ListGatewayInstancesRequest listGatewayInstancesRequest);
/**
* Displays a list of instances associated with the AWS account. This request returns a paginated result. You can
* use the filterArn property to display only the instances associated with the selected Gateway Amazon Resource
* Name (ARN).
*
* @param listGatewayInstancesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListGatewayInstances operation returned by the service.
* @sample AWSMediaConnectAsyncHandler.ListGatewayInstances
* @see AWS API Documentation
*/
java.util.concurrent.Future listGatewayInstancesAsync(ListGatewayInstancesRequest listGatewayInstancesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Displays a list of gateways that are associated with this account. This request returns a paginated result.
*
* @param listGatewaysRequest
* @return A Java Future containing the result of the ListGateways operation returned by the service.
* @sample AWSMediaConnectAsync.ListGateways
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listGatewaysAsync(ListGatewaysRequest listGatewaysRequest);
/**
* Displays a list of gateways that are associated with this account. This request returns a paginated result.
*
* @param listGatewaysRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListGateways operation returned by the service.
* @sample AWSMediaConnectAsyncHandler.ListGateways
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listGatewaysAsync(ListGatewaysRequest listGatewaysRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Displays a list of all offerings that are available to this account in the current AWS Region. If you have an
* active reservation (which means you've purchased an offering that has already started and hasn't expired yet),
* your account isn't eligible for other offerings.
*
* @param listOfferingsRequest
* @return A Java Future containing the result of the ListOfferings operation returned by the service.
* @sample AWSMediaConnectAsync.ListOfferings
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listOfferingsAsync(ListOfferingsRequest listOfferingsRequest);
/**
* Displays a list of all offerings that are available to this account in the current AWS Region. If you have an
* active reservation (which means you've purchased an offering that has already started and hasn't expired yet),
* your account isn't eligible for other offerings.
*
* @param listOfferingsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListOfferings operation returned by the service.
* @sample AWSMediaConnectAsyncHandler.ListOfferings
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listOfferingsAsync(ListOfferingsRequest listOfferingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Displays a list of all reservations that have been purchased by this account in the current AWS Region. This list
* includes all reservations in all states (such as active and expired).
*
* @param listReservationsRequest
* @return A Java Future containing the result of the ListReservations operation returned by the service.
* @sample AWSMediaConnectAsync.ListReservations
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listReservationsAsync(ListReservationsRequest listReservationsRequest);
/**
* Displays a list of all reservations that have been purchased by this account in the current AWS Region. This list
* includes all reservations in all states (such as active and expired).
*
* @param listReservationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListReservations operation returned by the service.
* @sample AWSMediaConnectAsyncHandler.ListReservations
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listReservationsAsync(ListReservationsRequest listReservationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* List all tags on an AWS Elemental MediaConnect resource
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSMediaConnectAsync.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
* List all tags on an AWS Elemental MediaConnect resource
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSMediaConnectAsyncHandler.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Submits a request to purchase an offering. If you already have an active reservation, you can't purchase another
* offering.
*
* @param purchaseOfferingRequest
* A request to purchase a offering.
* @return A Java Future containing the result of the PurchaseOffering operation returned by the service.
* @sample AWSMediaConnectAsync.PurchaseOffering
* @see AWS
* API Documentation
*/
java.util.concurrent.Future purchaseOfferingAsync(PurchaseOfferingRequest purchaseOfferingRequest);
/**
* Submits a request to purchase an offering. If you already have an active reservation, you can't purchase another
* offering.
*
* @param purchaseOfferingRequest
* A request to purchase a offering.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PurchaseOffering operation returned by the service.
* @sample AWSMediaConnectAsyncHandler.PurchaseOffering
* @see AWS
* API Documentation
*/
java.util.concurrent.Future purchaseOfferingAsync(PurchaseOfferingRequest purchaseOfferingRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Removes an output from a bridge.
*
* @param removeBridgeOutputRequest
* @return A Java Future containing the result of the RemoveBridgeOutput operation returned by the service.
* @sample AWSMediaConnectAsync.RemoveBridgeOutput
* @see AWS API Documentation
*/
java.util.concurrent.Future removeBridgeOutputAsync(RemoveBridgeOutputRequest removeBridgeOutputRequest);
/**
* Removes an output from a bridge.
*
* @param removeBridgeOutputRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RemoveBridgeOutput operation returned by the service.
* @sample AWSMediaConnectAsyncHandler.RemoveBridgeOutput
* @see AWS API Documentation
*/
java.util.concurrent.Future removeBridgeOutputAsync(RemoveBridgeOutputRequest removeBridgeOutputRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Removes a source from a bridge.
*
* @param removeBridgeSourceRequest
* @return A Java Future containing the result of the RemoveBridgeSource operation returned by the service.
* @sample AWSMediaConnectAsync.RemoveBridgeSource
* @see AWS API Documentation
*/
java.util.concurrent.Future removeBridgeSourceAsync(RemoveBridgeSourceRequest removeBridgeSourceRequest);
/**
* Removes a source from a bridge.
*
* @param removeBridgeSourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RemoveBridgeSource operation returned by the service.
* @sample AWSMediaConnectAsyncHandler.RemoveBridgeSource
* @see AWS API Documentation
*/
java.util.concurrent.Future removeBridgeSourceAsync(RemoveBridgeSourceRequest removeBridgeSourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Removes a media stream from a flow. This action is only available if the media stream is not associated with a
* source or output.
*
* @param removeFlowMediaStreamRequest
* @return A Java Future containing the result of the RemoveFlowMediaStream operation returned by the service.
* @sample AWSMediaConnectAsync.RemoveFlowMediaStream
* @see AWS API Documentation
*/
java.util.concurrent.Future removeFlowMediaStreamAsync(RemoveFlowMediaStreamRequest removeFlowMediaStreamRequest);
/**
* Removes a media stream from a flow. This action is only available if the media stream is not associated with a
* source or output.
*
* @param removeFlowMediaStreamRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RemoveFlowMediaStream operation returned by the service.
* @sample AWSMediaConnectAsyncHandler.RemoveFlowMediaStream
* @see AWS API Documentation
*/
java.util.concurrent.Future removeFlowMediaStreamAsync(RemoveFlowMediaStreamRequest removeFlowMediaStreamRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Removes an output from an existing flow. This request can be made only on an output that does not have an
* entitlement associated with it. If the output has an entitlement, you must revoke the entitlement instead. When
* an entitlement is revoked from a flow, the service automatically removes the associated output.
*
* @param removeFlowOutputRequest
* @return A Java Future containing the result of the RemoveFlowOutput operation returned by the service.
* @sample AWSMediaConnectAsync.RemoveFlowOutput
* @see AWS
* API Documentation
*/
java.util.concurrent.Future removeFlowOutputAsync(RemoveFlowOutputRequest removeFlowOutputRequest);
/**
* Removes an output from an existing flow. This request can be made only on an output that does not have an
* entitlement associated with it. If the output has an entitlement, you must revoke the entitlement instead. When
* an entitlement is revoked from a flow, the service automatically removes the associated output.
*
* @param removeFlowOutputRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RemoveFlowOutput operation returned by the service.
* @sample AWSMediaConnectAsyncHandler.RemoveFlowOutput
* @see AWS
* API Documentation
*/
java.util.concurrent.Future removeFlowOutputAsync(RemoveFlowOutputRequest removeFlowOutputRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Removes a source from an existing flow. This request can be made only if there is more than one source on the
* flow.
*
* @param removeFlowSourceRequest
* @return A Java Future containing the result of the RemoveFlowSource operation returned by the service.
* @sample AWSMediaConnectAsync.RemoveFlowSource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future removeFlowSourceAsync(RemoveFlowSourceRequest removeFlowSourceRequest);
/**
* Removes a source from an existing flow. This request can be made only if there is more than one source on the
* flow.
*
* @param removeFlowSourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RemoveFlowSource operation returned by the service.
* @sample AWSMediaConnectAsyncHandler.RemoveFlowSource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future removeFlowSourceAsync(RemoveFlowSourceRequest removeFlowSourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Removes a VPC Interface from an existing flow. This request can be made only on a VPC interface that does not
* have a Source or Output associated with it. If the VPC interface is referenced by a Source or Output, you must
* first delete or update the Source or Output to no longer reference the VPC interface.
*
* @param removeFlowVpcInterfaceRequest
* @return A Java Future containing the result of the RemoveFlowVpcInterface operation returned by the service.
* @sample AWSMediaConnectAsync.RemoveFlowVpcInterface
* @see AWS API Documentation
*/
java.util.concurrent.Future removeFlowVpcInterfaceAsync(RemoveFlowVpcInterfaceRequest removeFlowVpcInterfaceRequest);
/**
* Removes a VPC Interface from an existing flow. This request can be made only on a VPC interface that does not
* have a Source or Output associated with it. If the VPC interface is referenced by a Source or Output, you must
* first delete or update the Source or Output to no longer reference the VPC interface.
*
* @param removeFlowVpcInterfaceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RemoveFlowVpcInterface operation returned by the service.
* @sample AWSMediaConnectAsyncHandler.RemoveFlowVpcInterface
* @see AWS API Documentation
*/
java.util.concurrent.Future removeFlowVpcInterfaceAsync(RemoveFlowVpcInterfaceRequest removeFlowVpcInterfaceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Revokes an entitlement from a flow. Once an entitlement is revoked, the content becomes unavailable to the
* subscriber and the associated output is removed.
*
* @param revokeFlowEntitlementRequest
* @return A Java Future containing the result of the RevokeFlowEntitlement operation returned by the service.
* @sample AWSMediaConnectAsync.RevokeFlowEntitlement
* @see AWS API Documentation
*/
java.util.concurrent.Future revokeFlowEntitlementAsync(RevokeFlowEntitlementRequest revokeFlowEntitlementRequest);
/**
* Revokes an entitlement from a flow. Once an entitlement is revoked, the content becomes unavailable to the
* subscriber and the associated output is removed.
*
* @param revokeFlowEntitlementRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RevokeFlowEntitlement operation returned by the service.
* @sample AWSMediaConnectAsyncHandler.RevokeFlowEntitlement
* @see AWS API Documentation
*/
java.util.concurrent.Future revokeFlowEntitlementAsync(RevokeFlowEntitlementRequest revokeFlowEntitlementRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Starts a flow.
*
* @param startFlowRequest
* @return A Java Future containing the result of the StartFlow operation returned by the service.
* @sample AWSMediaConnectAsync.StartFlow
* @see AWS API
* Documentation
*/
java.util.concurrent.Future startFlowAsync(StartFlowRequest startFlowRequest);
/**
* Starts a flow.
*
* @param startFlowRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StartFlow operation returned by the service.
* @sample AWSMediaConnectAsyncHandler.StartFlow
* @see AWS API
* Documentation
*/
java.util.concurrent.Future startFlowAsync(StartFlowRequest startFlowRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Stops a flow.
*
* @param stopFlowRequest
* @return A Java Future containing the result of the StopFlow operation returned by the service.
* @sample AWSMediaConnectAsync.StopFlow
* @see AWS API
* Documentation
*/
java.util.concurrent.Future stopFlowAsync(StopFlowRequest stopFlowRequest);
/**
* Stops a flow.
*
* @param stopFlowRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StopFlow operation returned by the service.
* @sample AWSMediaConnectAsyncHandler.StopFlow
* @see AWS API
* Documentation
*/
java.util.concurrent.Future stopFlowAsync(StopFlowRequest stopFlowRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Associates the specified tags to a resource with the specified resourceArn. If existing tags on a resource are
* not specified in the request parameters, they are not changed. When a resource is deleted, the tags associated
* with that resource are deleted as well.
*
* @param tagResourceRequest
* The tags to add to the resource. A tag is an array of key-value pairs. Tag keys can have a maximum
* character length of 128 characters, and tag values can have a maximum length of 256 characters.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSMediaConnectAsync.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
* Associates the specified tags to a resource with the specified resourceArn. If existing tags on a resource are
* not specified in the request parameters, they are not changed. When a resource is deleted, the tags associated
* with that resource are deleted as well.
*
* @param tagResourceRequest
* The tags to add to the resource. A tag is an array of key-value pairs. Tag keys can have a maximum
* character length of 128 characters, and tag values can have a maximum length of 256 characters.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSMediaConnectAsyncHandler.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Deletes specified tags from a resource.
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSMediaConnectAsync.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
* Deletes specified tags from a resource.
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSMediaConnectAsyncHandler.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Updates the bridge
*
* @param updateBridgeRequest
* A request to update the bridge.
* @return A Java Future containing the result of the UpdateBridge operation returned by the service.
* @sample AWSMediaConnectAsync.UpdateBridge
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateBridgeAsync(UpdateBridgeRequest updateBridgeRequest);
/**
* Updates the bridge
*
* @param updateBridgeRequest
* A request to update the bridge.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateBridge operation returned by the service.
* @sample AWSMediaConnectAsyncHandler.UpdateBridge
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateBridgeAsync(UpdateBridgeRequest updateBridgeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Updates an existing bridge output.
*
* @param updateBridgeOutputRequest
* The fields that you want to update in the bridge output.
* @return A Java Future containing the result of the UpdateBridgeOutput operation returned by the service.
* @sample AWSMediaConnectAsync.UpdateBridgeOutput
* @see AWS API Documentation
*/
java.util.concurrent.Future updateBridgeOutputAsync(UpdateBridgeOutputRequest updateBridgeOutputRequest);
/**
* Updates an existing bridge output.
*
* @param updateBridgeOutputRequest
* The fields that you want to update in the bridge output.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateBridgeOutput operation returned by the service.
* @sample AWSMediaConnectAsyncHandler.UpdateBridgeOutput
* @see AWS API Documentation
*/
java.util.concurrent.Future updateBridgeOutputAsync(UpdateBridgeOutputRequest updateBridgeOutputRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Updates an existing bridge source.
*
* @param updateBridgeSourceRequest
* The fields that you want to update in the bridge source.
* @return A Java Future containing the result of the UpdateBridgeSource operation returned by the service.
* @sample AWSMediaConnectAsync.UpdateBridgeSource
* @see AWS API Documentation
*/
java.util.concurrent.Future updateBridgeSourceAsync(UpdateBridgeSourceRequest updateBridgeSourceRequest);
/**
* Updates an existing bridge source.
*
* @param updateBridgeSourceRequest
* The fields that you want to update in the bridge source.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateBridgeSource operation returned by the service.
* @sample AWSMediaConnectAsyncHandler.UpdateBridgeSource
* @see AWS API Documentation
*/
java.util.concurrent.Future updateBridgeSourceAsync(UpdateBridgeSourceRequest updateBridgeSourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Updates the bridge state
*
* @param updateBridgeStateRequest
* A request to update the bridge state.
* @return A Java Future containing the result of the UpdateBridgeState operation returned by the service.
* @sample AWSMediaConnectAsync.UpdateBridgeState
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateBridgeStateAsync(UpdateBridgeStateRequest updateBridgeStateRequest);
/**
* Updates the bridge state
*
* @param updateBridgeStateRequest
* A request to update the bridge state.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateBridgeState operation returned by the service.
* @sample AWSMediaConnectAsyncHandler.UpdateBridgeState
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateBridgeStateAsync(UpdateBridgeStateRequest updateBridgeStateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Updates flow
*
* @param updateFlowRequest
* A request to update flow.
* @return A Java Future containing the result of the UpdateFlow operation returned by the service.
* @sample AWSMediaConnectAsync.UpdateFlow
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateFlowAsync(UpdateFlowRequest updateFlowRequest);
/**
* Updates flow
*
* @param updateFlowRequest
* A request to update flow.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateFlow operation returned by the service.
* @sample AWSMediaConnectAsyncHandler.UpdateFlow
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateFlowAsync(UpdateFlowRequest updateFlowRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* You can change an entitlement's description, subscribers, and encryption. If you change the subscribers, the
* service will remove the outputs that are are used by the subscribers that are removed.
*
* @param updateFlowEntitlementRequest
* The entitlement fields that you want to update.
* @return A Java Future containing the result of the UpdateFlowEntitlement operation returned by the service.
* @sample AWSMediaConnectAsync.UpdateFlowEntitlement
* @see AWS API Documentation
*/
java.util.concurrent.Future updateFlowEntitlementAsync(UpdateFlowEntitlementRequest updateFlowEntitlementRequest);
/**
* You can change an entitlement's description, subscribers, and encryption. If you change the subscribers, the
* service will remove the outputs that are are used by the subscribers that are removed.
*
* @param updateFlowEntitlementRequest
* The entitlement fields that you want to update.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateFlowEntitlement operation returned by the service.
* @sample AWSMediaConnectAsyncHandler.UpdateFlowEntitlement
* @see AWS API Documentation
*/
java.util.concurrent.Future updateFlowEntitlementAsync(UpdateFlowEntitlementRequest updateFlowEntitlementRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Updates an existing media stream.
*
* @param updateFlowMediaStreamRequest
* The fields that you want to update in the media stream.
* @return A Java Future containing the result of the UpdateFlowMediaStream operation returned by the service.
* @sample AWSMediaConnectAsync.UpdateFlowMediaStream
* @see AWS API Documentation
*/
java.util.concurrent.Future updateFlowMediaStreamAsync(UpdateFlowMediaStreamRequest updateFlowMediaStreamRequest);
/**
* Updates an existing media stream.
*
* @param updateFlowMediaStreamRequest
* The fields that you want to update in the media stream.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateFlowMediaStream operation returned by the service.
* @sample AWSMediaConnectAsyncHandler.UpdateFlowMediaStream
* @see AWS API Documentation
*/
java.util.concurrent.Future updateFlowMediaStreamAsync(UpdateFlowMediaStreamRequest updateFlowMediaStreamRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Updates an existing flow output.
*
* @param updateFlowOutputRequest
* The fields that you want to update in the output.
* @return A Java Future containing the result of the UpdateFlowOutput operation returned by the service.
* @sample AWSMediaConnectAsync.UpdateFlowOutput
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateFlowOutputAsync(UpdateFlowOutputRequest updateFlowOutputRequest);
/**
* Updates an existing flow output.
*
* @param updateFlowOutputRequest
* The fields that you want to update in the output.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateFlowOutput operation returned by the service.
* @sample AWSMediaConnectAsyncHandler.UpdateFlowOutput
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateFlowOutputAsync(UpdateFlowOutputRequest updateFlowOutputRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Updates the source of a flow.
*
* @param updateFlowSourceRequest
* A request to update the source of a flow.
* @return A Java Future containing the result of the UpdateFlowSource operation returned by the service.
* @sample AWSMediaConnectAsync.UpdateFlowSource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateFlowSourceAsync(UpdateFlowSourceRequest updateFlowSourceRequest);
/**
* Updates the source of a flow.
*
* @param updateFlowSourceRequest
* A request to update the source of a flow.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateFlowSource operation returned by the service.
* @sample AWSMediaConnectAsyncHandler.UpdateFlowSource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateFlowSourceAsync(UpdateFlowSourceRequest updateFlowSourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Updates the configuration of an existing Gateway Instance.
*
* @param updateGatewayInstanceRequest
* A request to update gateway instance state.
* @return A Java Future containing the result of the UpdateGatewayInstance operation returned by the service.
* @sample AWSMediaConnectAsync.UpdateGatewayInstance
* @see AWS API Documentation
*/
java.util.concurrent.Future updateGatewayInstanceAsync(UpdateGatewayInstanceRequest updateGatewayInstanceRequest);
/**
* Updates the configuration of an existing Gateway Instance.
*
* @param updateGatewayInstanceRequest
* A request to update gateway instance state.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateGatewayInstance operation returned by the service.
* @sample AWSMediaConnectAsyncHandler.UpdateGatewayInstance
* @see AWS API Documentation
*/
java.util.concurrent.Future updateGatewayInstanceAsync(UpdateGatewayInstanceRequest updateGatewayInstanceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}