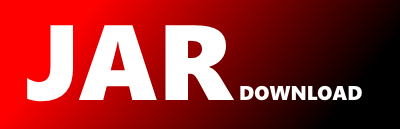
com.amazonaws.services.mediaconnect.model.Source Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws-java-sdk-mediaconnect Show documentation
Show all versions of aws-java-sdk-mediaconnect Show documentation
The AWS Java SDK for AWS MediaConnect module holds the client classes that are used for communicating with AWS MediaConnect Service
/*
* Copyright 2018-2023 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.mediaconnect.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
* The settings for the source of the flow.
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class Source implements Serializable, Cloneable, StructuredPojo {
/** Percentage from 0-100 of the data transfer cost to be billed to the subscriber. */
private Integer dataTransferSubscriberFeePercent;
/** The type of encryption that is used on the content ingested from this source. */
private Encryption decryption;
/**
* A description for the source. This value is not used or seen outside of the current AWS Elemental MediaConnect
* account.
*/
private String description;
/**
* The ARN of the entitlement that allows you to subscribe to content that comes from another AWS account. The
* entitlement is set by the content originator and the ARN is generated as part of the originator's flow.
*/
private String entitlementArn;
/** The IP address that the flow will be listening on for incoming content. */
private String ingestIp;
/** The port that the flow will be listening on for incoming content. */
private Integer ingestPort;
/** The media streams that are associated with the source, and the parameters for those associations. */
private java.util.List mediaStreamSourceConfigurations;
/** The name of the source. */
private String name;
/** The port that the flow uses to send outbound requests to initiate connection with the sender. */
private Integer senderControlPort;
/** The IP address that the flow communicates with to initiate connection with the sender. */
private String senderIpAddress;
/** The ARN of the source. */
private String sourceArn;
/** Attributes related to the transport stream that are used in the source. */
private Transport transport;
/** The name of the VPC interface that is used for this source. */
private String vpcInterfaceName;
/**
* The range of IP addresses that should be allowed to contribute content to your source. These IP addresses should
* be in the form of a Classless Inter-Domain Routing (CIDR) block; for example, 10.0.0.0/16.
*/
private String whitelistCidr;
/** The source configuration for cloud flows receiving a stream from a bridge. */
private GatewayBridgeSource gatewayBridgeSource;
/**
* Percentage from 0-100 of the data transfer cost to be billed to the subscriber.
*
* @param dataTransferSubscriberFeePercent
* Percentage from 0-100 of the data transfer cost to be billed to the subscriber.
*/
public void setDataTransferSubscriberFeePercent(Integer dataTransferSubscriberFeePercent) {
this.dataTransferSubscriberFeePercent = dataTransferSubscriberFeePercent;
}
/**
* Percentage from 0-100 of the data transfer cost to be billed to the subscriber.
*
* @return Percentage from 0-100 of the data transfer cost to be billed to the subscriber.
*/
public Integer getDataTransferSubscriberFeePercent() {
return this.dataTransferSubscriberFeePercent;
}
/**
* Percentage from 0-100 of the data transfer cost to be billed to the subscriber.
*
* @param dataTransferSubscriberFeePercent
* Percentage from 0-100 of the data transfer cost to be billed to the subscriber.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Source withDataTransferSubscriberFeePercent(Integer dataTransferSubscriberFeePercent) {
setDataTransferSubscriberFeePercent(dataTransferSubscriberFeePercent);
return this;
}
/**
* The type of encryption that is used on the content ingested from this source.
*
* @param decryption
* The type of encryption that is used on the content ingested from this source.
*/
public void setDecryption(Encryption decryption) {
this.decryption = decryption;
}
/**
* The type of encryption that is used on the content ingested from this source.
*
* @return The type of encryption that is used on the content ingested from this source.
*/
public Encryption getDecryption() {
return this.decryption;
}
/**
* The type of encryption that is used on the content ingested from this source.
*
* @param decryption
* The type of encryption that is used on the content ingested from this source.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Source withDecryption(Encryption decryption) {
setDecryption(decryption);
return this;
}
/**
* A description for the source. This value is not used or seen outside of the current AWS Elemental MediaConnect
* account.
*
* @param description
* A description for the source. This value is not used or seen outside of the current AWS Elemental
* MediaConnect account.
*/
public void setDescription(String description) {
this.description = description;
}
/**
* A description for the source. This value is not used or seen outside of the current AWS Elemental MediaConnect
* account.
*
* @return A description for the source. This value is not used or seen outside of the current AWS Elemental
* MediaConnect account.
*/
public String getDescription() {
return this.description;
}
/**
* A description for the source. This value is not used or seen outside of the current AWS Elemental MediaConnect
* account.
*
* @param description
* A description for the source. This value is not used or seen outside of the current AWS Elemental
* MediaConnect account.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Source withDescription(String description) {
setDescription(description);
return this;
}
/**
* The ARN of the entitlement that allows you to subscribe to content that comes from another AWS account. The
* entitlement is set by the content originator and the ARN is generated as part of the originator's flow.
*
* @param entitlementArn
* The ARN of the entitlement that allows you to subscribe to content that comes from another AWS account.
* The entitlement is set by the content originator and the ARN is generated as part of the originator's
* flow.
*/
public void setEntitlementArn(String entitlementArn) {
this.entitlementArn = entitlementArn;
}
/**
* The ARN of the entitlement that allows you to subscribe to content that comes from another AWS account. The
* entitlement is set by the content originator and the ARN is generated as part of the originator's flow.
*
* @return The ARN of the entitlement that allows you to subscribe to content that comes from another AWS account.
* The entitlement is set by the content originator and the ARN is generated as part of the originator's
* flow.
*/
public String getEntitlementArn() {
return this.entitlementArn;
}
/**
* The ARN of the entitlement that allows you to subscribe to content that comes from another AWS account. The
* entitlement is set by the content originator and the ARN is generated as part of the originator's flow.
*
* @param entitlementArn
* The ARN of the entitlement that allows you to subscribe to content that comes from another AWS account.
* The entitlement is set by the content originator and the ARN is generated as part of the originator's
* flow.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Source withEntitlementArn(String entitlementArn) {
setEntitlementArn(entitlementArn);
return this;
}
/**
* The IP address that the flow will be listening on for incoming content.
*
* @param ingestIp
* The IP address that the flow will be listening on for incoming content.
*/
public void setIngestIp(String ingestIp) {
this.ingestIp = ingestIp;
}
/**
* The IP address that the flow will be listening on for incoming content.
*
* @return The IP address that the flow will be listening on for incoming content.
*/
public String getIngestIp() {
return this.ingestIp;
}
/**
* The IP address that the flow will be listening on for incoming content.
*
* @param ingestIp
* The IP address that the flow will be listening on for incoming content.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Source withIngestIp(String ingestIp) {
setIngestIp(ingestIp);
return this;
}
/**
* The port that the flow will be listening on for incoming content.
*
* @param ingestPort
* The port that the flow will be listening on for incoming content.
*/
public void setIngestPort(Integer ingestPort) {
this.ingestPort = ingestPort;
}
/**
* The port that the flow will be listening on for incoming content.
*
* @return The port that the flow will be listening on for incoming content.
*/
public Integer getIngestPort() {
return this.ingestPort;
}
/**
* The port that the flow will be listening on for incoming content.
*
* @param ingestPort
* The port that the flow will be listening on for incoming content.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Source withIngestPort(Integer ingestPort) {
setIngestPort(ingestPort);
return this;
}
/**
* The media streams that are associated with the source, and the parameters for those associations.
*
* @return The media streams that are associated with the source, and the parameters for those associations.
*/
public java.util.List getMediaStreamSourceConfigurations() {
return mediaStreamSourceConfigurations;
}
/**
* The media streams that are associated with the source, and the parameters for those associations.
*
* @param mediaStreamSourceConfigurations
* The media streams that are associated with the source, and the parameters for those associations.
*/
public void setMediaStreamSourceConfigurations(java.util.Collection mediaStreamSourceConfigurations) {
if (mediaStreamSourceConfigurations == null) {
this.mediaStreamSourceConfigurations = null;
return;
}
this.mediaStreamSourceConfigurations = new java.util.ArrayList(mediaStreamSourceConfigurations);
}
/**
* The media streams that are associated with the source, and the parameters for those associations.
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setMediaStreamSourceConfigurations(java.util.Collection)} or
* {@link #withMediaStreamSourceConfigurations(java.util.Collection)} if you want to override the existing values.
*
*
* @param mediaStreamSourceConfigurations
* The media streams that are associated with the source, and the parameters for those associations.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Source withMediaStreamSourceConfigurations(MediaStreamSourceConfiguration... mediaStreamSourceConfigurations) {
if (this.mediaStreamSourceConfigurations == null) {
setMediaStreamSourceConfigurations(new java.util.ArrayList(mediaStreamSourceConfigurations.length));
}
for (MediaStreamSourceConfiguration ele : mediaStreamSourceConfigurations) {
this.mediaStreamSourceConfigurations.add(ele);
}
return this;
}
/**
* The media streams that are associated with the source, and the parameters for those associations.
*
* @param mediaStreamSourceConfigurations
* The media streams that are associated with the source, and the parameters for those associations.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Source withMediaStreamSourceConfigurations(java.util.Collection mediaStreamSourceConfigurations) {
setMediaStreamSourceConfigurations(mediaStreamSourceConfigurations);
return this;
}
/**
* The name of the source.
*
* @param name
* The name of the source.
*/
public void setName(String name) {
this.name = name;
}
/**
* The name of the source.
*
* @return The name of the source.
*/
public String getName() {
return this.name;
}
/**
* The name of the source.
*
* @param name
* The name of the source.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Source withName(String name) {
setName(name);
return this;
}
/**
* The port that the flow uses to send outbound requests to initiate connection with the sender.
*
* @param senderControlPort
* The port that the flow uses to send outbound requests to initiate connection with the sender.
*/
public void setSenderControlPort(Integer senderControlPort) {
this.senderControlPort = senderControlPort;
}
/**
* The port that the flow uses to send outbound requests to initiate connection with the sender.
*
* @return The port that the flow uses to send outbound requests to initiate connection with the sender.
*/
public Integer getSenderControlPort() {
return this.senderControlPort;
}
/**
* The port that the flow uses to send outbound requests to initiate connection with the sender.
*
* @param senderControlPort
* The port that the flow uses to send outbound requests to initiate connection with the sender.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Source withSenderControlPort(Integer senderControlPort) {
setSenderControlPort(senderControlPort);
return this;
}
/**
* The IP address that the flow communicates with to initiate connection with the sender.
*
* @param senderIpAddress
* The IP address that the flow communicates with to initiate connection with the sender.
*/
public void setSenderIpAddress(String senderIpAddress) {
this.senderIpAddress = senderIpAddress;
}
/**
* The IP address that the flow communicates with to initiate connection with the sender.
*
* @return The IP address that the flow communicates with to initiate connection with the sender.
*/
public String getSenderIpAddress() {
return this.senderIpAddress;
}
/**
* The IP address that the flow communicates with to initiate connection with the sender.
*
* @param senderIpAddress
* The IP address that the flow communicates with to initiate connection with the sender.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Source withSenderIpAddress(String senderIpAddress) {
setSenderIpAddress(senderIpAddress);
return this;
}
/**
* The ARN of the source.
*
* @param sourceArn
* The ARN of the source.
*/
public void setSourceArn(String sourceArn) {
this.sourceArn = sourceArn;
}
/**
* The ARN of the source.
*
* @return The ARN of the source.
*/
public String getSourceArn() {
return this.sourceArn;
}
/**
* The ARN of the source.
*
* @param sourceArn
* The ARN of the source.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Source withSourceArn(String sourceArn) {
setSourceArn(sourceArn);
return this;
}
/**
* Attributes related to the transport stream that are used in the source.
*
* @param transport
* Attributes related to the transport stream that are used in the source.
*/
public void setTransport(Transport transport) {
this.transport = transport;
}
/**
* Attributes related to the transport stream that are used in the source.
*
* @return Attributes related to the transport stream that are used in the source.
*/
public Transport getTransport() {
return this.transport;
}
/**
* Attributes related to the transport stream that are used in the source.
*
* @param transport
* Attributes related to the transport stream that are used in the source.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Source withTransport(Transport transport) {
setTransport(transport);
return this;
}
/**
* The name of the VPC interface that is used for this source.
*
* @param vpcInterfaceName
* The name of the VPC interface that is used for this source.
*/
public void setVpcInterfaceName(String vpcInterfaceName) {
this.vpcInterfaceName = vpcInterfaceName;
}
/**
* The name of the VPC interface that is used for this source.
*
* @return The name of the VPC interface that is used for this source.
*/
public String getVpcInterfaceName() {
return this.vpcInterfaceName;
}
/**
* The name of the VPC interface that is used for this source.
*
* @param vpcInterfaceName
* The name of the VPC interface that is used for this source.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Source withVpcInterfaceName(String vpcInterfaceName) {
setVpcInterfaceName(vpcInterfaceName);
return this;
}
/**
* The range of IP addresses that should be allowed to contribute content to your source. These IP addresses should
* be in the form of a Classless Inter-Domain Routing (CIDR) block; for example, 10.0.0.0/16.
*
* @param whitelistCidr
* The range of IP addresses that should be allowed to contribute content to your source. These IP addresses
* should be in the form of a Classless Inter-Domain Routing (CIDR) block; for example, 10.0.0.0/16.
*/
public void setWhitelistCidr(String whitelistCidr) {
this.whitelistCidr = whitelistCidr;
}
/**
* The range of IP addresses that should be allowed to contribute content to your source. These IP addresses should
* be in the form of a Classless Inter-Domain Routing (CIDR) block; for example, 10.0.0.0/16.
*
* @return The range of IP addresses that should be allowed to contribute content to your source. These IP addresses
* should be in the form of a Classless Inter-Domain Routing (CIDR) block; for example, 10.0.0.0/16.
*/
public String getWhitelistCidr() {
return this.whitelistCidr;
}
/**
* The range of IP addresses that should be allowed to contribute content to your source. These IP addresses should
* be in the form of a Classless Inter-Domain Routing (CIDR) block; for example, 10.0.0.0/16.
*
* @param whitelistCidr
* The range of IP addresses that should be allowed to contribute content to your source. These IP addresses
* should be in the form of a Classless Inter-Domain Routing (CIDR) block; for example, 10.0.0.0/16.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Source withWhitelistCidr(String whitelistCidr) {
setWhitelistCidr(whitelistCidr);
return this;
}
/**
* The source configuration for cloud flows receiving a stream from a bridge.
*
* @param gatewayBridgeSource
* The source configuration for cloud flows receiving a stream from a bridge.
*/
public void setGatewayBridgeSource(GatewayBridgeSource gatewayBridgeSource) {
this.gatewayBridgeSource = gatewayBridgeSource;
}
/**
* The source configuration for cloud flows receiving a stream from a bridge.
*
* @return The source configuration for cloud flows receiving a stream from a bridge.
*/
public GatewayBridgeSource getGatewayBridgeSource() {
return this.gatewayBridgeSource;
}
/**
* The source configuration for cloud flows receiving a stream from a bridge.
*
* @param gatewayBridgeSource
* The source configuration for cloud flows receiving a stream from a bridge.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Source withGatewayBridgeSource(GatewayBridgeSource gatewayBridgeSource) {
setGatewayBridgeSource(gatewayBridgeSource);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getDataTransferSubscriberFeePercent() != null)
sb.append("DataTransferSubscriberFeePercent: ").append(getDataTransferSubscriberFeePercent()).append(",");
if (getDecryption() != null)
sb.append("Decryption: ").append(getDecryption()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getEntitlementArn() != null)
sb.append("EntitlementArn: ").append(getEntitlementArn()).append(",");
if (getIngestIp() != null)
sb.append("IngestIp: ").append(getIngestIp()).append(",");
if (getIngestPort() != null)
sb.append("IngestPort: ").append(getIngestPort()).append(",");
if (getMediaStreamSourceConfigurations() != null)
sb.append("MediaStreamSourceConfigurations: ").append(getMediaStreamSourceConfigurations()).append(",");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getSenderControlPort() != null)
sb.append("SenderControlPort: ").append(getSenderControlPort()).append(",");
if (getSenderIpAddress() != null)
sb.append("SenderIpAddress: ").append(getSenderIpAddress()).append(",");
if (getSourceArn() != null)
sb.append("SourceArn: ").append(getSourceArn()).append(",");
if (getTransport() != null)
sb.append("Transport: ").append(getTransport()).append(",");
if (getVpcInterfaceName() != null)
sb.append("VpcInterfaceName: ").append(getVpcInterfaceName()).append(",");
if (getWhitelistCidr() != null)
sb.append("WhitelistCidr: ").append(getWhitelistCidr()).append(",");
if (getGatewayBridgeSource() != null)
sb.append("GatewayBridgeSource: ").append(getGatewayBridgeSource());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof Source == false)
return false;
Source other = (Source) obj;
if (other.getDataTransferSubscriberFeePercent() == null ^ this.getDataTransferSubscriberFeePercent() == null)
return false;
if (other.getDataTransferSubscriberFeePercent() != null
&& other.getDataTransferSubscriberFeePercent().equals(this.getDataTransferSubscriberFeePercent()) == false)
return false;
if (other.getDecryption() == null ^ this.getDecryption() == null)
return false;
if (other.getDecryption() != null && other.getDecryption().equals(this.getDecryption()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getEntitlementArn() == null ^ this.getEntitlementArn() == null)
return false;
if (other.getEntitlementArn() != null && other.getEntitlementArn().equals(this.getEntitlementArn()) == false)
return false;
if (other.getIngestIp() == null ^ this.getIngestIp() == null)
return false;
if (other.getIngestIp() != null && other.getIngestIp().equals(this.getIngestIp()) == false)
return false;
if (other.getIngestPort() == null ^ this.getIngestPort() == null)
return false;
if (other.getIngestPort() != null && other.getIngestPort().equals(this.getIngestPort()) == false)
return false;
if (other.getMediaStreamSourceConfigurations() == null ^ this.getMediaStreamSourceConfigurations() == null)
return false;
if (other.getMediaStreamSourceConfigurations() != null
&& other.getMediaStreamSourceConfigurations().equals(this.getMediaStreamSourceConfigurations()) == false)
return false;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getSenderControlPort() == null ^ this.getSenderControlPort() == null)
return false;
if (other.getSenderControlPort() != null && other.getSenderControlPort().equals(this.getSenderControlPort()) == false)
return false;
if (other.getSenderIpAddress() == null ^ this.getSenderIpAddress() == null)
return false;
if (other.getSenderIpAddress() != null && other.getSenderIpAddress().equals(this.getSenderIpAddress()) == false)
return false;
if (other.getSourceArn() == null ^ this.getSourceArn() == null)
return false;
if (other.getSourceArn() != null && other.getSourceArn().equals(this.getSourceArn()) == false)
return false;
if (other.getTransport() == null ^ this.getTransport() == null)
return false;
if (other.getTransport() != null && other.getTransport().equals(this.getTransport()) == false)
return false;
if (other.getVpcInterfaceName() == null ^ this.getVpcInterfaceName() == null)
return false;
if (other.getVpcInterfaceName() != null && other.getVpcInterfaceName().equals(this.getVpcInterfaceName()) == false)
return false;
if (other.getWhitelistCidr() == null ^ this.getWhitelistCidr() == null)
return false;
if (other.getWhitelistCidr() != null && other.getWhitelistCidr().equals(this.getWhitelistCidr()) == false)
return false;
if (other.getGatewayBridgeSource() == null ^ this.getGatewayBridgeSource() == null)
return false;
if (other.getGatewayBridgeSource() != null && other.getGatewayBridgeSource().equals(this.getGatewayBridgeSource()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getDataTransferSubscriberFeePercent() == null) ? 0 : getDataTransferSubscriberFeePercent().hashCode());
hashCode = prime * hashCode + ((getDecryption() == null) ? 0 : getDecryption().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getEntitlementArn() == null) ? 0 : getEntitlementArn().hashCode());
hashCode = prime * hashCode + ((getIngestIp() == null) ? 0 : getIngestIp().hashCode());
hashCode = prime * hashCode + ((getIngestPort() == null) ? 0 : getIngestPort().hashCode());
hashCode = prime * hashCode + ((getMediaStreamSourceConfigurations() == null) ? 0 : getMediaStreamSourceConfigurations().hashCode());
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getSenderControlPort() == null) ? 0 : getSenderControlPort().hashCode());
hashCode = prime * hashCode + ((getSenderIpAddress() == null) ? 0 : getSenderIpAddress().hashCode());
hashCode = prime * hashCode + ((getSourceArn() == null) ? 0 : getSourceArn().hashCode());
hashCode = prime * hashCode + ((getTransport() == null) ? 0 : getTransport().hashCode());
hashCode = prime * hashCode + ((getVpcInterfaceName() == null) ? 0 : getVpcInterfaceName().hashCode());
hashCode = prime * hashCode + ((getWhitelistCidr() == null) ? 0 : getWhitelistCidr().hashCode());
hashCode = prime * hashCode + ((getGatewayBridgeSource() == null) ? 0 : getGatewayBridgeSource().hashCode());
return hashCode;
}
@Override
public Source clone() {
try {
return (Source) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.mediaconnect.model.transform.SourceMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy