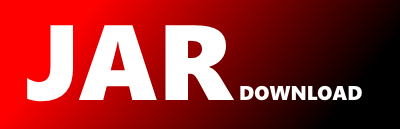
com.amazonaws.services.mediaconnect.model.UpdateFlowOutputRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws-java-sdk-mediaconnect Show documentation
Show all versions of aws-java-sdk-mediaconnect Show documentation
The AWS Java SDK for AWS MediaConnect module holds the client classes that are used for communicating with AWS MediaConnect Service
The newest version!
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.mediaconnect.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
* The fields that you want to update in the output.
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class UpdateFlowOutputRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
* The range of IP addresses that should be allowed to initiate output requests to this flow. These IP addresses
* should be in the form of a Classless Inter-Domain Routing (CIDR) block; for example, 10.0.0.0/16.
*/
private java.util.List cidrAllowList;
/**
* A description of the output. This description appears only on the AWS Elemental MediaConnect console and will not
* be seen by the end user.
*/
private String description;
/** The IP address where you want to send the output. */
private String destination;
/**
* The type of key used for the encryption. If no keyType is provided, the service will use the default setting
* (static-key). Allowable encryption types: static-key.
*/
private UpdateEncryption encryption;
/** The flow that is associated with the output that you want to update. */
private String flowArn;
/**
* The maximum latency in milliseconds. This parameter applies only to RIST-based, Zixi-based, and Fujitsu-based
* streams.
*/
private Integer maxLatency;
/** The media streams that are associated with the output, and the parameters for those associations. */
private java.util.List mediaStreamOutputConfigurations;
/**
* The minimum latency in milliseconds for SRT-based streams. In streams that use the SRT protocol, this value that
* you set on your MediaConnect source or output represents the minimal potential latency of that connection. The
* latency of the stream is set to the highest number between the sender’s minimum latency and the receiver’s minimum
* latency.
*/
private Integer minLatency;
/** The ARN of the output that you want to update. */
private String outputArn;
/** The port to use when content is distributed to this output. */
private Integer port;
/** The protocol to use for the output. */
private String protocol;
/** The remote ID for the Zixi-pull stream. */
private String remoteId;
/** The port that the flow uses to send outbound requests to initiate connection with the sender. */
private Integer senderControlPort;
/** The IP address that the flow communicates with to initiate connection with the sender. */
private String senderIpAddress;
/** The smoothing latency in milliseconds for RIST, RTP, and RTP-FEC streams. */
private Integer smoothingLatency;
/**
* The stream ID that you want to use for this transport. This parameter applies only to Zixi and SRT caller-based
* streams.
*/
private String streamId;
/** The name of the VPC interface attachment to use for this output. */
private VpcInterfaceAttachment vpcInterfaceAttachment;
/**
* An indication of whether the output should transmit data or not. If you don't specify the outputStatus field in
* your request, MediaConnect leaves the value unchanged.
*/
private String outputStatus;
/**
* The range of IP addresses that should be allowed to initiate output requests to this flow. These IP addresses
* should be in the form of a Classless Inter-Domain Routing (CIDR) block; for example, 10.0.0.0/16.
*
* @return The range of IP addresses that should be allowed to initiate output requests to this flow. These IP
* addresses should be in the form of a Classless Inter-Domain Routing (CIDR) block; for example,
* 10.0.0.0/16.
*/
public java.util.List getCidrAllowList() {
return cidrAllowList;
}
/**
* The range of IP addresses that should be allowed to initiate output requests to this flow. These IP addresses
* should be in the form of a Classless Inter-Domain Routing (CIDR) block; for example, 10.0.0.0/16.
*
* @param cidrAllowList
* The range of IP addresses that should be allowed to initiate output requests to this flow. These IP
* addresses should be in the form of a Classless Inter-Domain Routing (CIDR) block; for example,
* 10.0.0.0/16.
*/
public void setCidrAllowList(java.util.Collection cidrAllowList) {
if (cidrAllowList == null) {
this.cidrAllowList = null;
return;
}
this.cidrAllowList = new java.util.ArrayList(cidrAllowList);
}
/**
* The range of IP addresses that should be allowed to initiate output requests to this flow. These IP addresses
* should be in the form of a Classless Inter-Domain Routing (CIDR) block; for example, 10.0.0.0/16.
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setCidrAllowList(java.util.Collection)} or {@link #withCidrAllowList(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param cidrAllowList
* The range of IP addresses that should be allowed to initiate output requests to this flow. These IP
* addresses should be in the form of a Classless Inter-Domain Routing (CIDR) block; for example,
* 10.0.0.0/16.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateFlowOutputRequest withCidrAllowList(String... cidrAllowList) {
if (this.cidrAllowList == null) {
setCidrAllowList(new java.util.ArrayList(cidrAllowList.length));
}
for (String ele : cidrAllowList) {
this.cidrAllowList.add(ele);
}
return this;
}
/**
* The range of IP addresses that should be allowed to initiate output requests to this flow. These IP addresses
* should be in the form of a Classless Inter-Domain Routing (CIDR) block; for example, 10.0.0.0/16.
*
* @param cidrAllowList
* The range of IP addresses that should be allowed to initiate output requests to this flow. These IP
* addresses should be in the form of a Classless Inter-Domain Routing (CIDR) block; for example,
* 10.0.0.0/16.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateFlowOutputRequest withCidrAllowList(java.util.Collection cidrAllowList) {
setCidrAllowList(cidrAllowList);
return this;
}
/**
* A description of the output. This description appears only on the AWS Elemental MediaConnect console and will not
* be seen by the end user.
*
* @param description
* A description of the output. This description appears only on the AWS Elemental MediaConnect console and
* will not be seen by the end user.
*/
public void setDescription(String description) {
this.description = description;
}
/**
* A description of the output. This description appears only on the AWS Elemental MediaConnect console and will not
* be seen by the end user.
*
* @return A description of the output. This description appears only on the AWS Elemental MediaConnect console and
* will not be seen by the end user.
*/
public String getDescription() {
return this.description;
}
/**
* A description of the output. This description appears only on the AWS Elemental MediaConnect console and will not
* be seen by the end user.
*
* @param description
* A description of the output. This description appears only on the AWS Elemental MediaConnect console and
* will not be seen by the end user.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateFlowOutputRequest withDescription(String description) {
setDescription(description);
return this;
}
/**
* The IP address where you want to send the output.
*
* @param destination
* The IP address where you want to send the output.
*/
public void setDestination(String destination) {
this.destination = destination;
}
/**
* The IP address where you want to send the output.
*
* @return The IP address where you want to send the output.
*/
public String getDestination() {
return this.destination;
}
/**
* The IP address where you want to send the output.
*
* @param destination
* The IP address where you want to send the output.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateFlowOutputRequest withDestination(String destination) {
setDestination(destination);
return this;
}
/**
* The type of key used for the encryption. If no keyType is provided, the service will use the default setting
* (static-key). Allowable encryption types: static-key.
*
* @param encryption
* The type of key used for the encryption. If no keyType is provided, the service will use the default
* setting (static-key). Allowable encryption types: static-key.
*/
public void setEncryption(UpdateEncryption encryption) {
this.encryption = encryption;
}
/**
* The type of key used for the encryption. If no keyType is provided, the service will use the default setting
* (static-key). Allowable encryption types: static-key.
*
* @return The type of key used for the encryption. If no keyType is provided, the service will use the default
* setting (static-key). Allowable encryption types: static-key.
*/
public UpdateEncryption getEncryption() {
return this.encryption;
}
/**
* The type of key used for the encryption. If no keyType is provided, the service will use the default setting
* (static-key). Allowable encryption types: static-key.
*
* @param encryption
* The type of key used for the encryption. If no keyType is provided, the service will use the default
* setting (static-key). Allowable encryption types: static-key.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateFlowOutputRequest withEncryption(UpdateEncryption encryption) {
setEncryption(encryption);
return this;
}
/**
* The flow that is associated with the output that you want to update.
*
* @param flowArn
* The flow that is associated with the output that you want to update.
*/
public void setFlowArn(String flowArn) {
this.flowArn = flowArn;
}
/**
* The flow that is associated with the output that you want to update.
*
* @return The flow that is associated with the output that you want to update.
*/
public String getFlowArn() {
return this.flowArn;
}
/**
* The flow that is associated with the output that you want to update.
*
* @param flowArn
* The flow that is associated with the output that you want to update.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateFlowOutputRequest withFlowArn(String flowArn) {
setFlowArn(flowArn);
return this;
}
/**
* The maximum latency in milliseconds. This parameter applies only to RIST-based, Zixi-based, and Fujitsu-based
* streams.
*
* @param maxLatency
* The maximum latency in milliseconds. This parameter applies only to RIST-based, Zixi-based, and
* Fujitsu-based streams.
*/
public void setMaxLatency(Integer maxLatency) {
this.maxLatency = maxLatency;
}
/**
* The maximum latency in milliseconds. This parameter applies only to RIST-based, Zixi-based, and Fujitsu-based
* streams.
*
* @return The maximum latency in milliseconds. This parameter applies only to RIST-based, Zixi-based, and
* Fujitsu-based streams.
*/
public Integer getMaxLatency() {
return this.maxLatency;
}
/**
* The maximum latency in milliseconds. This parameter applies only to RIST-based, Zixi-based, and Fujitsu-based
* streams.
*
* @param maxLatency
* The maximum latency in milliseconds. This parameter applies only to RIST-based, Zixi-based, and
* Fujitsu-based streams.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateFlowOutputRequest withMaxLatency(Integer maxLatency) {
setMaxLatency(maxLatency);
return this;
}
/**
* The media streams that are associated with the output, and the parameters for those associations.
*
* @return The media streams that are associated with the output, and the parameters for those associations.
*/
public java.util.List getMediaStreamOutputConfigurations() {
return mediaStreamOutputConfigurations;
}
/**
* The media streams that are associated with the output, and the parameters for those associations.
*
* @param mediaStreamOutputConfigurations
* The media streams that are associated with the output, and the parameters for those associations.
*/
public void setMediaStreamOutputConfigurations(java.util.Collection mediaStreamOutputConfigurations) {
if (mediaStreamOutputConfigurations == null) {
this.mediaStreamOutputConfigurations = null;
return;
}
this.mediaStreamOutputConfigurations = new java.util.ArrayList(mediaStreamOutputConfigurations);
}
/**
* The media streams that are associated with the output, and the parameters for those associations.
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setMediaStreamOutputConfigurations(java.util.Collection)} or
* {@link #withMediaStreamOutputConfigurations(java.util.Collection)} if you want to override the existing values.
*
*
* @param mediaStreamOutputConfigurations
* The media streams that are associated with the output, and the parameters for those associations.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateFlowOutputRequest withMediaStreamOutputConfigurations(MediaStreamOutputConfigurationRequest... mediaStreamOutputConfigurations) {
if (this.mediaStreamOutputConfigurations == null) {
setMediaStreamOutputConfigurations(new java.util.ArrayList(mediaStreamOutputConfigurations.length));
}
for (MediaStreamOutputConfigurationRequest ele : mediaStreamOutputConfigurations) {
this.mediaStreamOutputConfigurations.add(ele);
}
return this;
}
/**
* The media streams that are associated with the output, and the parameters for those associations.
*
* @param mediaStreamOutputConfigurations
* The media streams that are associated with the output, and the parameters for those associations.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateFlowOutputRequest withMediaStreamOutputConfigurations(
java.util.Collection mediaStreamOutputConfigurations) {
setMediaStreamOutputConfigurations(mediaStreamOutputConfigurations);
return this;
}
/**
* The minimum latency in milliseconds for SRT-based streams. In streams that use the SRT protocol, this value that
* you set on your MediaConnect source or output represents the minimal potential latency of that connection. The
* latency of the stream is set to the highest number between the sender’s minimum latency and the receiver’s minimum
* latency.
*
* @param minLatency
* The minimum latency in milliseconds for SRT-based streams. In streams that use the SRT protocol, this
* value that you set on your MediaConnect source or output represents the minimal potential latency of that
* connection. The latency of the stream is set to the highest number between the sender’s minimum latency
* and the receiver’s minimum latency.
*/
public void setMinLatency(Integer minLatency) {
this.minLatency = minLatency;
}
/**
* The minimum latency in milliseconds for SRT-based streams. In streams that use the SRT protocol, this value that
* you set on your MediaConnect source or output represents the minimal potential latency of that connection. The
* latency of the stream is set to the highest number between the sender’s minimum latency and the receiver’s minimum
* latency.
*
* @return The minimum latency in milliseconds for SRT-based streams. In streams that use the SRT protocol, this
* value that you set on your MediaConnect source or output represents the minimal potential latency of that
* connection. The latency of the stream is set to the highest number between the sender’s minimum latency
* and the receiver’s minimum latency.
*/
public Integer getMinLatency() {
return this.minLatency;
}
/**
* The minimum latency in milliseconds for SRT-based streams. In streams that use the SRT protocol, this value that
* you set on your MediaConnect source or output represents the minimal potential latency of that connection. The
* latency of the stream is set to the highest number between the sender’s minimum latency and the receiver’s minimum
* latency.
*
* @param minLatency
* The minimum latency in milliseconds for SRT-based streams. In streams that use the SRT protocol, this
* value that you set on your MediaConnect source or output represents the minimal potential latency of that
* connection. The latency of the stream is set to the highest number between the sender’s minimum latency
* and the receiver’s minimum latency.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateFlowOutputRequest withMinLatency(Integer minLatency) {
setMinLatency(minLatency);
return this;
}
/**
* The ARN of the output that you want to update.
*
* @param outputArn
* The ARN of the output that you want to update.
*/
public void setOutputArn(String outputArn) {
this.outputArn = outputArn;
}
/**
* The ARN of the output that you want to update.
*
* @return The ARN of the output that you want to update.
*/
public String getOutputArn() {
return this.outputArn;
}
/**
* The ARN of the output that you want to update.
*
* @param outputArn
* The ARN of the output that you want to update.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateFlowOutputRequest withOutputArn(String outputArn) {
setOutputArn(outputArn);
return this;
}
/**
* The port to use when content is distributed to this output.
*
* @param port
* The port to use when content is distributed to this output.
*/
public void setPort(Integer port) {
this.port = port;
}
/**
* The port to use when content is distributed to this output.
*
* @return The port to use when content is distributed to this output.
*/
public Integer getPort() {
return this.port;
}
/**
* The port to use when content is distributed to this output.
*
* @param port
* The port to use when content is distributed to this output.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateFlowOutputRequest withPort(Integer port) {
setPort(port);
return this;
}
/**
* The protocol to use for the output.
*
* @param protocol
* The protocol to use for the output.
* @see Protocol
*/
public void setProtocol(String protocol) {
this.protocol = protocol;
}
/**
* The protocol to use for the output.
*
* @return The protocol to use for the output.
* @see Protocol
*/
public String getProtocol() {
return this.protocol;
}
/**
* The protocol to use for the output.
*
* @param protocol
* The protocol to use for the output.
* @return Returns a reference to this object so that method calls can be chained together.
* @see Protocol
*/
public UpdateFlowOutputRequest withProtocol(String protocol) {
setProtocol(protocol);
return this;
}
/**
* The protocol to use for the output.
*
* @param protocol
* The protocol to use for the output.
* @return Returns a reference to this object so that method calls can be chained together.
* @see Protocol
*/
public UpdateFlowOutputRequest withProtocol(Protocol protocol) {
this.protocol = protocol.toString();
return this;
}
/**
* The remote ID for the Zixi-pull stream.
*
* @param remoteId
* The remote ID for the Zixi-pull stream.
*/
public void setRemoteId(String remoteId) {
this.remoteId = remoteId;
}
/**
* The remote ID for the Zixi-pull stream.
*
* @return The remote ID for the Zixi-pull stream.
*/
public String getRemoteId() {
return this.remoteId;
}
/**
* The remote ID for the Zixi-pull stream.
*
* @param remoteId
* The remote ID for the Zixi-pull stream.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateFlowOutputRequest withRemoteId(String remoteId) {
setRemoteId(remoteId);
return this;
}
/**
* The port that the flow uses to send outbound requests to initiate connection with the sender.
*
* @param senderControlPort
* The port that the flow uses to send outbound requests to initiate connection with the sender.
*/
public void setSenderControlPort(Integer senderControlPort) {
this.senderControlPort = senderControlPort;
}
/**
* The port that the flow uses to send outbound requests to initiate connection with the sender.
*
* @return The port that the flow uses to send outbound requests to initiate connection with the sender.
*/
public Integer getSenderControlPort() {
return this.senderControlPort;
}
/**
* The port that the flow uses to send outbound requests to initiate connection with the sender.
*
* @param senderControlPort
* The port that the flow uses to send outbound requests to initiate connection with the sender.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateFlowOutputRequest withSenderControlPort(Integer senderControlPort) {
setSenderControlPort(senderControlPort);
return this;
}
/**
* The IP address that the flow communicates with to initiate connection with the sender.
*
* @param senderIpAddress
* The IP address that the flow communicates with to initiate connection with the sender.
*/
public void setSenderIpAddress(String senderIpAddress) {
this.senderIpAddress = senderIpAddress;
}
/**
* The IP address that the flow communicates with to initiate connection with the sender.
*
* @return The IP address that the flow communicates with to initiate connection with the sender.
*/
public String getSenderIpAddress() {
return this.senderIpAddress;
}
/**
* The IP address that the flow communicates with to initiate connection with the sender.
*
* @param senderIpAddress
* The IP address that the flow communicates with to initiate connection with the sender.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateFlowOutputRequest withSenderIpAddress(String senderIpAddress) {
setSenderIpAddress(senderIpAddress);
return this;
}
/**
* The smoothing latency in milliseconds for RIST, RTP, and RTP-FEC streams.
*
* @param smoothingLatency
* The smoothing latency in milliseconds for RIST, RTP, and RTP-FEC streams.
*/
public void setSmoothingLatency(Integer smoothingLatency) {
this.smoothingLatency = smoothingLatency;
}
/**
* The smoothing latency in milliseconds for RIST, RTP, and RTP-FEC streams.
*
* @return The smoothing latency in milliseconds for RIST, RTP, and RTP-FEC streams.
*/
public Integer getSmoothingLatency() {
return this.smoothingLatency;
}
/**
* The smoothing latency in milliseconds for RIST, RTP, and RTP-FEC streams.
*
* @param smoothingLatency
* The smoothing latency in milliseconds for RIST, RTP, and RTP-FEC streams.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateFlowOutputRequest withSmoothingLatency(Integer smoothingLatency) {
setSmoothingLatency(smoothingLatency);
return this;
}
/**
* The stream ID that you want to use for this transport. This parameter applies only to Zixi and SRT caller-based
* streams.
*
* @param streamId
* The stream ID that you want to use for this transport. This parameter applies only to Zixi and SRT
* caller-based streams.
*/
public void setStreamId(String streamId) {
this.streamId = streamId;
}
/**
* The stream ID that you want to use for this transport. This parameter applies only to Zixi and SRT caller-based
* streams.
*
* @return The stream ID that you want to use for this transport. This parameter applies only to Zixi and SRT
* caller-based streams.
*/
public String getStreamId() {
return this.streamId;
}
/**
* The stream ID that you want to use for this transport. This parameter applies only to Zixi and SRT caller-based
* streams.
*
* @param streamId
* The stream ID that you want to use for this transport. This parameter applies only to Zixi and SRT
* caller-based streams.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateFlowOutputRequest withStreamId(String streamId) {
setStreamId(streamId);
return this;
}
/**
* The name of the VPC interface attachment to use for this output.
*
* @param vpcInterfaceAttachment
* The name of the VPC interface attachment to use for this output.
*/
public void setVpcInterfaceAttachment(VpcInterfaceAttachment vpcInterfaceAttachment) {
this.vpcInterfaceAttachment = vpcInterfaceAttachment;
}
/**
* The name of the VPC interface attachment to use for this output.
*
* @return The name of the VPC interface attachment to use for this output.
*/
public VpcInterfaceAttachment getVpcInterfaceAttachment() {
return this.vpcInterfaceAttachment;
}
/**
* The name of the VPC interface attachment to use for this output.
*
* @param vpcInterfaceAttachment
* The name of the VPC interface attachment to use for this output.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateFlowOutputRequest withVpcInterfaceAttachment(VpcInterfaceAttachment vpcInterfaceAttachment) {
setVpcInterfaceAttachment(vpcInterfaceAttachment);
return this;
}
/**
* An indication of whether the output should transmit data or not. If you don't specify the outputStatus field in
* your request, MediaConnect leaves the value unchanged.
*
* @param outputStatus
* An indication of whether the output should transmit data or not. If you don't specify the outputStatus
* field in your request, MediaConnect leaves the value unchanged.
* @see OutputStatus
*/
public void setOutputStatus(String outputStatus) {
this.outputStatus = outputStatus;
}
/**
* An indication of whether the output should transmit data or not. If you don't specify the outputStatus field in
* your request, MediaConnect leaves the value unchanged.
*
* @return An indication of whether the output should transmit data or not. If you don't specify the outputStatus
* field in your request, MediaConnect leaves the value unchanged.
* @see OutputStatus
*/
public String getOutputStatus() {
return this.outputStatus;
}
/**
* An indication of whether the output should transmit data or not. If you don't specify the outputStatus field in
* your request, MediaConnect leaves the value unchanged.
*
* @param outputStatus
* An indication of whether the output should transmit data or not. If you don't specify the outputStatus
* field in your request, MediaConnect leaves the value unchanged.
* @return Returns a reference to this object so that method calls can be chained together.
* @see OutputStatus
*/
public UpdateFlowOutputRequest withOutputStatus(String outputStatus) {
setOutputStatus(outputStatus);
return this;
}
/**
* An indication of whether the output should transmit data or not. If you don't specify the outputStatus field in
* your request, MediaConnect leaves the value unchanged.
*
* @param outputStatus
* An indication of whether the output should transmit data or not. If you don't specify the outputStatus
* field in your request, MediaConnect leaves the value unchanged.
* @return Returns a reference to this object so that method calls can be chained together.
* @see OutputStatus
*/
public UpdateFlowOutputRequest withOutputStatus(OutputStatus outputStatus) {
this.outputStatus = outputStatus.toString();
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getCidrAllowList() != null)
sb.append("CidrAllowList: ").append(getCidrAllowList()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getDestination() != null)
sb.append("Destination: ").append(getDestination()).append(",");
if (getEncryption() != null)
sb.append("Encryption: ").append(getEncryption()).append(",");
if (getFlowArn() != null)
sb.append("FlowArn: ").append(getFlowArn()).append(",");
if (getMaxLatency() != null)
sb.append("MaxLatency: ").append(getMaxLatency()).append(",");
if (getMediaStreamOutputConfigurations() != null)
sb.append("MediaStreamOutputConfigurations: ").append(getMediaStreamOutputConfigurations()).append(",");
if (getMinLatency() != null)
sb.append("MinLatency: ").append(getMinLatency()).append(",");
if (getOutputArn() != null)
sb.append("OutputArn: ").append(getOutputArn()).append(",");
if (getPort() != null)
sb.append("Port: ").append(getPort()).append(",");
if (getProtocol() != null)
sb.append("Protocol: ").append(getProtocol()).append(",");
if (getRemoteId() != null)
sb.append("RemoteId: ").append(getRemoteId()).append(",");
if (getSenderControlPort() != null)
sb.append("SenderControlPort: ").append(getSenderControlPort()).append(",");
if (getSenderIpAddress() != null)
sb.append("SenderIpAddress: ").append(getSenderIpAddress()).append(",");
if (getSmoothingLatency() != null)
sb.append("SmoothingLatency: ").append(getSmoothingLatency()).append(",");
if (getStreamId() != null)
sb.append("StreamId: ").append(getStreamId()).append(",");
if (getVpcInterfaceAttachment() != null)
sb.append("VpcInterfaceAttachment: ").append(getVpcInterfaceAttachment()).append(",");
if (getOutputStatus() != null)
sb.append("OutputStatus: ").append(getOutputStatus());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof UpdateFlowOutputRequest == false)
return false;
UpdateFlowOutputRequest other = (UpdateFlowOutputRequest) obj;
if (other.getCidrAllowList() == null ^ this.getCidrAllowList() == null)
return false;
if (other.getCidrAllowList() != null && other.getCidrAllowList().equals(this.getCidrAllowList()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getDestination() == null ^ this.getDestination() == null)
return false;
if (other.getDestination() != null && other.getDestination().equals(this.getDestination()) == false)
return false;
if (other.getEncryption() == null ^ this.getEncryption() == null)
return false;
if (other.getEncryption() != null && other.getEncryption().equals(this.getEncryption()) == false)
return false;
if (other.getFlowArn() == null ^ this.getFlowArn() == null)
return false;
if (other.getFlowArn() != null && other.getFlowArn().equals(this.getFlowArn()) == false)
return false;
if (other.getMaxLatency() == null ^ this.getMaxLatency() == null)
return false;
if (other.getMaxLatency() != null && other.getMaxLatency().equals(this.getMaxLatency()) == false)
return false;
if (other.getMediaStreamOutputConfigurations() == null ^ this.getMediaStreamOutputConfigurations() == null)
return false;
if (other.getMediaStreamOutputConfigurations() != null
&& other.getMediaStreamOutputConfigurations().equals(this.getMediaStreamOutputConfigurations()) == false)
return false;
if (other.getMinLatency() == null ^ this.getMinLatency() == null)
return false;
if (other.getMinLatency() != null && other.getMinLatency().equals(this.getMinLatency()) == false)
return false;
if (other.getOutputArn() == null ^ this.getOutputArn() == null)
return false;
if (other.getOutputArn() != null && other.getOutputArn().equals(this.getOutputArn()) == false)
return false;
if (other.getPort() == null ^ this.getPort() == null)
return false;
if (other.getPort() != null && other.getPort().equals(this.getPort()) == false)
return false;
if (other.getProtocol() == null ^ this.getProtocol() == null)
return false;
if (other.getProtocol() != null && other.getProtocol().equals(this.getProtocol()) == false)
return false;
if (other.getRemoteId() == null ^ this.getRemoteId() == null)
return false;
if (other.getRemoteId() != null && other.getRemoteId().equals(this.getRemoteId()) == false)
return false;
if (other.getSenderControlPort() == null ^ this.getSenderControlPort() == null)
return false;
if (other.getSenderControlPort() != null && other.getSenderControlPort().equals(this.getSenderControlPort()) == false)
return false;
if (other.getSenderIpAddress() == null ^ this.getSenderIpAddress() == null)
return false;
if (other.getSenderIpAddress() != null && other.getSenderIpAddress().equals(this.getSenderIpAddress()) == false)
return false;
if (other.getSmoothingLatency() == null ^ this.getSmoothingLatency() == null)
return false;
if (other.getSmoothingLatency() != null && other.getSmoothingLatency().equals(this.getSmoothingLatency()) == false)
return false;
if (other.getStreamId() == null ^ this.getStreamId() == null)
return false;
if (other.getStreamId() != null && other.getStreamId().equals(this.getStreamId()) == false)
return false;
if (other.getVpcInterfaceAttachment() == null ^ this.getVpcInterfaceAttachment() == null)
return false;
if (other.getVpcInterfaceAttachment() != null && other.getVpcInterfaceAttachment().equals(this.getVpcInterfaceAttachment()) == false)
return false;
if (other.getOutputStatus() == null ^ this.getOutputStatus() == null)
return false;
if (other.getOutputStatus() != null && other.getOutputStatus().equals(this.getOutputStatus()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getCidrAllowList() == null) ? 0 : getCidrAllowList().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getDestination() == null) ? 0 : getDestination().hashCode());
hashCode = prime * hashCode + ((getEncryption() == null) ? 0 : getEncryption().hashCode());
hashCode = prime * hashCode + ((getFlowArn() == null) ? 0 : getFlowArn().hashCode());
hashCode = prime * hashCode + ((getMaxLatency() == null) ? 0 : getMaxLatency().hashCode());
hashCode = prime * hashCode + ((getMediaStreamOutputConfigurations() == null) ? 0 : getMediaStreamOutputConfigurations().hashCode());
hashCode = prime * hashCode + ((getMinLatency() == null) ? 0 : getMinLatency().hashCode());
hashCode = prime * hashCode + ((getOutputArn() == null) ? 0 : getOutputArn().hashCode());
hashCode = prime * hashCode + ((getPort() == null) ? 0 : getPort().hashCode());
hashCode = prime * hashCode + ((getProtocol() == null) ? 0 : getProtocol().hashCode());
hashCode = prime * hashCode + ((getRemoteId() == null) ? 0 : getRemoteId().hashCode());
hashCode = prime * hashCode + ((getSenderControlPort() == null) ? 0 : getSenderControlPort().hashCode());
hashCode = prime * hashCode + ((getSenderIpAddress() == null) ? 0 : getSenderIpAddress().hashCode());
hashCode = prime * hashCode + ((getSmoothingLatency() == null) ? 0 : getSmoothingLatency().hashCode());
hashCode = prime * hashCode + ((getStreamId() == null) ? 0 : getStreamId().hashCode());
hashCode = prime * hashCode + ((getVpcInterfaceAttachment() == null) ? 0 : getVpcInterfaceAttachment().hashCode());
hashCode = prime * hashCode + ((getOutputStatus() == null) ? 0 : getOutputStatus().hashCode());
return hashCode;
}
@Override
public UpdateFlowOutputRequest clone() {
return (UpdateFlowOutputRequest) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy