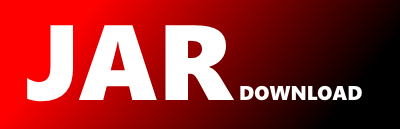
com.amazonaws.services.mediaconnect.model.UpdateFlowSourceRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws-java-sdk-mediaconnect Show documentation
Show all versions of aws-java-sdk-mediaconnect Show documentation
The AWS Java SDK for AWS MediaConnect module holds the client classes that are used for communicating with AWS MediaConnect Service
The newest version!
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.mediaconnect.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
* A request to update the source of a flow.
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class UpdateFlowSourceRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/** The type of encryption used on the content ingested from this source. Allowable encryption types: static-key. */
private UpdateEncryption decryption;
/**
* A description for the source. This value is not used or seen outside of the current AWS Elemental MediaConnect
* account.
*/
private String description;
/**
* The ARN of the entitlement that allows you to subscribe to this flow. The entitlement is set by the flow
* originator, and the ARN is generated as part of the originator's flow.
*/
private String entitlementArn;
/** The flow that is associated with the source that you want to update. */
private String flowArn;
/** The port that the flow will be listening on for incoming content. */
private Integer ingestPort;
/** The smoothing max bitrate (in bps) for RIST, RTP, and RTP-FEC streams. */
private Integer maxBitrate;
/**
* The maximum latency in milliseconds. This parameter applies only to RIST-based, Zixi-based, and Fujitsu-based
* streams.
*/
private Integer maxLatency;
/** The size of the buffer (in milliseconds) to use to sync incoming source data. */
private Integer maxSyncBuffer;
/** The media streams that are associated with the source, and the parameters for those associations. */
private java.util.List mediaStreamSourceConfigurations;
/**
* The minimum latency in milliseconds for SRT-based streams. In streams that use the SRT protocol, this value that
* you set on your MediaConnect source or output represents the minimal potential latency of that connection. The
* latency of the stream is set to the highest number between the sender’s minimum latency and the receiver’s minimum
* latency.
*/
private Integer minLatency;
/** The protocol that is used by the source. */
private String protocol;
/** The port that the flow uses to send outbound requests to initiate connection with the sender. */
private Integer senderControlPort;
/** The IP address that the flow communicates with to initiate connection with the sender. */
private String senderIpAddress;
/** The ARN of the source that you want to update. */
private String sourceArn;
/** Source IP or domain name for SRT-caller protocol. */
private String sourceListenerAddress;
/** Source port for SRT-caller protocol. */
private Integer sourceListenerPort;
/**
* The stream ID that you want to use for this transport. This parameter applies only to Zixi and SRT caller-based
* streams.
*/
private String streamId;
/** The name of the VPC interface to use for this source. */
private String vpcInterfaceName;
/**
* The range of IP addresses that should be allowed to contribute content to your source. These IP addresses should
* be in the form of a Classless Inter-Domain Routing (CIDR) block; for example, 10.0.0.0/16.
*/
private String whitelistCidr;
/** The source configuration for cloud flows receiving a stream from a bridge. */
private UpdateGatewayBridgeSourceRequest gatewayBridgeSource;
/**
* The type of encryption used on the content ingested from this source. Allowable encryption types: static-key.
*
* @param decryption
* The type of encryption used on the content ingested from this source. Allowable encryption types:
* static-key.
*/
public void setDecryption(UpdateEncryption decryption) {
this.decryption = decryption;
}
/**
* The type of encryption used on the content ingested from this source. Allowable encryption types: static-key.
*
* @return The type of encryption used on the content ingested from this source. Allowable encryption types:
* static-key.
*/
public UpdateEncryption getDecryption() {
return this.decryption;
}
/**
* The type of encryption used on the content ingested from this source. Allowable encryption types: static-key.
*
* @param decryption
* The type of encryption used on the content ingested from this source. Allowable encryption types:
* static-key.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateFlowSourceRequest withDecryption(UpdateEncryption decryption) {
setDecryption(decryption);
return this;
}
/**
* A description for the source. This value is not used or seen outside of the current AWS Elemental MediaConnect
* account.
*
* @param description
* A description for the source. This value is not used or seen outside of the current AWS Elemental
* MediaConnect account.
*/
public void setDescription(String description) {
this.description = description;
}
/**
* A description for the source. This value is not used or seen outside of the current AWS Elemental MediaConnect
* account.
*
* @return A description for the source. This value is not used or seen outside of the current AWS Elemental
* MediaConnect account.
*/
public String getDescription() {
return this.description;
}
/**
* A description for the source. This value is not used or seen outside of the current AWS Elemental MediaConnect
* account.
*
* @param description
* A description for the source. This value is not used or seen outside of the current AWS Elemental
* MediaConnect account.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateFlowSourceRequest withDescription(String description) {
setDescription(description);
return this;
}
/**
* The ARN of the entitlement that allows you to subscribe to this flow. The entitlement is set by the flow
* originator, and the ARN is generated as part of the originator's flow.
*
* @param entitlementArn
* The ARN of the entitlement that allows you to subscribe to this flow. The entitlement is set by the flow
* originator, and the ARN is generated as part of the originator's flow.
*/
public void setEntitlementArn(String entitlementArn) {
this.entitlementArn = entitlementArn;
}
/**
* The ARN of the entitlement that allows you to subscribe to this flow. The entitlement is set by the flow
* originator, and the ARN is generated as part of the originator's flow.
*
* @return The ARN of the entitlement that allows you to subscribe to this flow. The entitlement is set by the flow
* originator, and the ARN is generated as part of the originator's flow.
*/
public String getEntitlementArn() {
return this.entitlementArn;
}
/**
* The ARN of the entitlement that allows you to subscribe to this flow. The entitlement is set by the flow
* originator, and the ARN is generated as part of the originator's flow.
*
* @param entitlementArn
* The ARN of the entitlement that allows you to subscribe to this flow. The entitlement is set by the flow
* originator, and the ARN is generated as part of the originator's flow.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateFlowSourceRequest withEntitlementArn(String entitlementArn) {
setEntitlementArn(entitlementArn);
return this;
}
/**
* The flow that is associated with the source that you want to update.
*
* @param flowArn
* The flow that is associated with the source that you want to update.
*/
public void setFlowArn(String flowArn) {
this.flowArn = flowArn;
}
/**
* The flow that is associated with the source that you want to update.
*
* @return The flow that is associated with the source that you want to update.
*/
public String getFlowArn() {
return this.flowArn;
}
/**
* The flow that is associated with the source that you want to update.
*
* @param flowArn
* The flow that is associated with the source that you want to update.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateFlowSourceRequest withFlowArn(String flowArn) {
setFlowArn(flowArn);
return this;
}
/**
* The port that the flow will be listening on for incoming content.
*
* @param ingestPort
* The port that the flow will be listening on for incoming content.
*/
public void setIngestPort(Integer ingestPort) {
this.ingestPort = ingestPort;
}
/**
* The port that the flow will be listening on for incoming content.
*
* @return The port that the flow will be listening on for incoming content.
*/
public Integer getIngestPort() {
return this.ingestPort;
}
/**
* The port that the flow will be listening on for incoming content.
*
* @param ingestPort
* The port that the flow will be listening on for incoming content.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateFlowSourceRequest withIngestPort(Integer ingestPort) {
setIngestPort(ingestPort);
return this;
}
/**
* The smoothing max bitrate (in bps) for RIST, RTP, and RTP-FEC streams.
*
* @param maxBitrate
* The smoothing max bitrate (in bps) for RIST, RTP, and RTP-FEC streams.
*/
public void setMaxBitrate(Integer maxBitrate) {
this.maxBitrate = maxBitrate;
}
/**
* The smoothing max bitrate (in bps) for RIST, RTP, and RTP-FEC streams.
*
* @return The smoothing max bitrate (in bps) for RIST, RTP, and RTP-FEC streams.
*/
public Integer getMaxBitrate() {
return this.maxBitrate;
}
/**
* The smoothing max bitrate (in bps) for RIST, RTP, and RTP-FEC streams.
*
* @param maxBitrate
* The smoothing max bitrate (in bps) for RIST, RTP, and RTP-FEC streams.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateFlowSourceRequest withMaxBitrate(Integer maxBitrate) {
setMaxBitrate(maxBitrate);
return this;
}
/**
* The maximum latency in milliseconds. This parameter applies only to RIST-based, Zixi-based, and Fujitsu-based
* streams.
*
* @param maxLatency
* The maximum latency in milliseconds. This parameter applies only to RIST-based, Zixi-based, and
* Fujitsu-based streams.
*/
public void setMaxLatency(Integer maxLatency) {
this.maxLatency = maxLatency;
}
/**
* The maximum latency in milliseconds. This parameter applies only to RIST-based, Zixi-based, and Fujitsu-based
* streams.
*
* @return The maximum latency in milliseconds. This parameter applies only to RIST-based, Zixi-based, and
* Fujitsu-based streams.
*/
public Integer getMaxLatency() {
return this.maxLatency;
}
/**
* The maximum latency in milliseconds. This parameter applies only to RIST-based, Zixi-based, and Fujitsu-based
* streams.
*
* @param maxLatency
* The maximum latency in milliseconds. This parameter applies only to RIST-based, Zixi-based, and
* Fujitsu-based streams.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateFlowSourceRequest withMaxLatency(Integer maxLatency) {
setMaxLatency(maxLatency);
return this;
}
/**
* The size of the buffer (in milliseconds) to use to sync incoming source data.
*
* @param maxSyncBuffer
* The size of the buffer (in milliseconds) to use to sync incoming source data.
*/
public void setMaxSyncBuffer(Integer maxSyncBuffer) {
this.maxSyncBuffer = maxSyncBuffer;
}
/**
* The size of the buffer (in milliseconds) to use to sync incoming source data.
*
* @return The size of the buffer (in milliseconds) to use to sync incoming source data.
*/
public Integer getMaxSyncBuffer() {
return this.maxSyncBuffer;
}
/**
* The size of the buffer (in milliseconds) to use to sync incoming source data.
*
* @param maxSyncBuffer
* The size of the buffer (in milliseconds) to use to sync incoming source data.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateFlowSourceRequest withMaxSyncBuffer(Integer maxSyncBuffer) {
setMaxSyncBuffer(maxSyncBuffer);
return this;
}
/**
* The media streams that are associated with the source, and the parameters for those associations.
*
* @return The media streams that are associated with the source, and the parameters for those associations.
*/
public java.util.List getMediaStreamSourceConfigurations() {
return mediaStreamSourceConfigurations;
}
/**
* The media streams that are associated with the source, and the parameters for those associations.
*
* @param mediaStreamSourceConfigurations
* The media streams that are associated with the source, and the parameters for those associations.
*/
public void setMediaStreamSourceConfigurations(java.util.Collection mediaStreamSourceConfigurations) {
if (mediaStreamSourceConfigurations == null) {
this.mediaStreamSourceConfigurations = null;
return;
}
this.mediaStreamSourceConfigurations = new java.util.ArrayList(mediaStreamSourceConfigurations);
}
/**
* The media streams that are associated with the source, and the parameters for those associations.
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setMediaStreamSourceConfigurations(java.util.Collection)} or
* {@link #withMediaStreamSourceConfigurations(java.util.Collection)} if you want to override the existing values.
*
*
* @param mediaStreamSourceConfigurations
* The media streams that are associated with the source, and the parameters for those associations.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateFlowSourceRequest withMediaStreamSourceConfigurations(MediaStreamSourceConfigurationRequest... mediaStreamSourceConfigurations) {
if (this.mediaStreamSourceConfigurations == null) {
setMediaStreamSourceConfigurations(new java.util.ArrayList(mediaStreamSourceConfigurations.length));
}
for (MediaStreamSourceConfigurationRequest ele : mediaStreamSourceConfigurations) {
this.mediaStreamSourceConfigurations.add(ele);
}
return this;
}
/**
* The media streams that are associated with the source, and the parameters for those associations.
*
* @param mediaStreamSourceConfigurations
* The media streams that are associated with the source, and the parameters for those associations.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateFlowSourceRequest withMediaStreamSourceConfigurations(
java.util.Collection mediaStreamSourceConfigurations) {
setMediaStreamSourceConfigurations(mediaStreamSourceConfigurations);
return this;
}
/**
* The minimum latency in milliseconds for SRT-based streams. In streams that use the SRT protocol, this value that
* you set on your MediaConnect source or output represents the minimal potential latency of that connection. The
* latency of the stream is set to the highest number between the sender’s minimum latency and the receiver’s minimum
* latency.
*
* @param minLatency
* The minimum latency in milliseconds for SRT-based streams. In streams that use the SRT protocol, this
* value that you set on your MediaConnect source or output represents the minimal potential latency of that
* connection. The latency of the stream is set to the highest number between the sender’s minimum latency
* and the receiver’s minimum latency.
*/
public void setMinLatency(Integer minLatency) {
this.minLatency = minLatency;
}
/**
* The minimum latency in milliseconds for SRT-based streams. In streams that use the SRT protocol, this value that
* you set on your MediaConnect source or output represents the minimal potential latency of that connection. The
* latency of the stream is set to the highest number between the sender’s minimum latency and the receiver’s minimum
* latency.
*
* @return The minimum latency in milliseconds for SRT-based streams. In streams that use the SRT protocol, this
* value that you set on your MediaConnect source or output represents the minimal potential latency of that
* connection. The latency of the stream is set to the highest number between the sender’s minimum latency
* and the receiver’s minimum latency.
*/
public Integer getMinLatency() {
return this.minLatency;
}
/**
* The minimum latency in milliseconds for SRT-based streams. In streams that use the SRT protocol, this value that
* you set on your MediaConnect source or output represents the minimal potential latency of that connection. The
* latency of the stream is set to the highest number between the sender’s minimum latency and the receiver’s minimum
* latency.
*
* @param minLatency
* The minimum latency in milliseconds for SRT-based streams. In streams that use the SRT protocol, this
* value that you set on your MediaConnect source or output represents the minimal potential latency of that
* connection. The latency of the stream is set to the highest number between the sender’s minimum latency
* and the receiver’s minimum latency.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateFlowSourceRequest withMinLatency(Integer minLatency) {
setMinLatency(minLatency);
return this;
}
/**
* The protocol that is used by the source.
*
* @param protocol
* The protocol that is used by the source.
* @see Protocol
*/
public void setProtocol(String protocol) {
this.protocol = protocol;
}
/**
* The protocol that is used by the source.
*
* @return The protocol that is used by the source.
* @see Protocol
*/
public String getProtocol() {
return this.protocol;
}
/**
* The protocol that is used by the source.
*
* @param protocol
* The protocol that is used by the source.
* @return Returns a reference to this object so that method calls can be chained together.
* @see Protocol
*/
public UpdateFlowSourceRequest withProtocol(String protocol) {
setProtocol(protocol);
return this;
}
/**
* The protocol that is used by the source.
*
* @param protocol
* The protocol that is used by the source.
* @return Returns a reference to this object so that method calls can be chained together.
* @see Protocol
*/
public UpdateFlowSourceRequest withProtocol(Protocol protocol) {
this.protocol = protocol.toString();
return this;
}
/**
* The port that the flow uses to send outbound requests to initiate connection with the sender.
*
* @param senderControlPort
* The port that the flow uses to send outbound requests to initiate connection with the sender.
*/
public void setSenderControlPort(Integer senderControlPort) {
this.senderControlPort = senderControlPort;
}
/**
* The port that the flow uses to send outbound requests to initiate connection with the sender.
*
* @return The port that the flow uses to send outbound requests to initiate connection with the sender.
*/
public Integer getSenderControlPort() {
return this.senderControlPort;
}
/**
* The port that the flow uses to send outbound requests to initiate connection with the sender.
*
* @param senderControlPort
* The port that the flow uses to send outbound requests to initiate connection with the sender.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateFlowSourceRequest withSenderControlPort(Integer senderControlPort) {
setSenderControlPort(senderControlPort);
return this;
}
/**
* The IP address that the flow communicates with to initiate connection with the sender.
*
* @param senderIpAddress
* The IP address that the flow communicates with to initiate connection with the sender.
*/
public void setSenderIpAddress(String senderIpAddress) {
this.senderIpAddress = senderIpAddress;
}
/**
* The IP address that the flow communicates with to initiate connection with the sender.
*
* @return The IP address that the flow communicates with to initiate connection with the sender.
*/
public String getSenderIpAddress() {
return this.senderIpAddress;
}
/**
* The IP address that the flow communicates with to initiate connection with the sender.
*
* @param senderIpAddress
* The IP address that the flow communicates with to initiate connection with the sender.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateFlowSourceRequest withSenderIpAddress(String senderIpAddress) {
setSenderIpAddress(senderIpAddress);
return this;
}
/**
* The ARN of the source that you want to update.
*
* @param sourceArn
* The ARN of the source that you want to update.
*/
public void setSourceArn(String sourceArn) {
this.sourceArn = sourceArn;
}
/**
* The ARN of the source that you want to update.
*
* @return The ARN of the source that you want to update.
*/
public String getSourceArn() {
return this.sourceArn;
}
/**
* The ARN of the source that you want to update.
*
* @param sourceArn
* The ARN of the source that you want to update.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateFlowSourceRequest withSourceArn(String sourceArn) {
setSourceArn(sourceArn);
return this;
}
/**
* Source IP or domain name for SRT-caller protocol.
*
* @param sourceListenerAddress
* Source IP or domain name for SRT-caller protocol.
*/
public void setSourceListenerAddress(String sourceListenerAddress) {
this.sourceListenerAddress = sourceListenerAddress;
}
/**
* Source IP or domain name for SRT-caller protocol.
*
* @return Source IP or domain name for SRT-caller protocol.
*/
public String getSourceListenerAddress() {
return this.sourceListenerAddress;
}
/**
* Source IP or domain name for SRT-caller protocol.
*
* @param sourceListenerAddress
* Source IP or domain name for SRT-caller protocol.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateFlowSourceRequest withSourceListenerAddress(String sourceListenerAddress) {
setSourceListenerAddress(sourceListenerAddress);
return this;
}
/**
* Source port for SRT-caller protocol.
*
* @param sourceListenerPort
* Source port for SRT-caller protocol.
*/
public void setSourceListenerPort(Integer sourceListenerPort) {
this.sourceListenerPort = sourceListenerPort;
}
/**
* Source port for SRT-caller protocol.
*
* @return Source port for SRT-caller protocol.
*/
public Integer getSourceListenerPort() {
return this.sourceListenerPort;
}
/**
* Source port for SRT-caller protocol.
*
* @param sourceListenerPort
* Source port for SRT-caller protocol.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateFlowSourceRequest withSourceListenerPort(Integer sourceListenerPort) {
setSourceListenerPort(sourceListenerPort);
return this;
}
/**
* The stream ID that you want to use for this transport. This parameter applies only to Zixi and SRT caller-based
* streams.
*
* @param streamId
* The stream ID that you want to use for this transport. This parameter applies only to Zixi and SRT
* caller-based streams.
*/
public void setStreamId(String streamId) {
this.streamId = streamId;
}
/**
* The stream ID that you want to use for this transport. This parameter applies only to Zixi and SRT caller-based
* streams.
*
* @return The stream ID that you want to use for this transport. This parameter applies only to Zixi and SRT
* caller-based streams.
*/
public String getStreamId() {
return this.streamId;
}
/**
* The stream ID that you want to use for this transport. This parameter applies only to Zixi and SRT caller-based
* streams.
*
* @param streamId
* The stream ID that you want to use for this transport. This parameter applies only to Zixi and SRT
* caller-based streams.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateFlowSourceRequest withStreamId(String streamId) {
setStreamId(streamId);
return this;
}
/**
* The name of the VPC interface to use for this source.
*
* @param vpcInterfaceName
* The name of the VPC interface to use for this source.
*/
public void setVpcInterfaceName(String vpcInterfaceName) {
this.vpcInterfaceName = vpcInterfaceName;
}
/**
* The name of the VPC interface to use for this source.
*
* @return The name of the VPC interface to use for this source.
*/
public String getVpcInterfaceName() {
return this.vpcInterfaceName;
}
/**
* The name of the VPC interface to use for this source.
*
* @param vpcInterfaceName
* The name of the VPC interface to use for this source.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateFlowSourceRequest withVpcInterfaceName(String vpcInterfaceName) {
setVpcInterfaceName(vpcInterfaceName);
return this;
}
/**
* The range of IP addresses that should be allowed to contribute content to your source. These IP addresses should
* be in the form of a Classless Inter-Domain Routing (CIDR) block; for example, 10.0.0.0/16.
*
* @param whitelistCidr
* The range of IP addresses that should be allowed to contribute content to your source. These IP addresses
* should be in the form of a Classless Inter-Domain Routing (CIDR) block; for example, 10.0.0.0/16.
*/
public void setWhitelistCidr(String whitelistCidr) {
this.whitelistCidr = whitelistCidr;
}
/**
* The range of IP addresses that should be allowed to contribute content to your source. These IP addresses should
* be in the form of a Classless Inter-Domain Routing (CIDR) block; for example, 10.0.0.0/16.
*
* @return The range of IP addresses that should be allowed to contribute content to your source. These IP addresses
* should be in the form of a Classless Inter-Domain Routing (CIDR) block; for example, 10.0.0.0/16.
*/
public String getWhitelistCidr() {
return this.whitelistCidr;
}
/**
* The range of IP addresses that should be allowed to contribute content to your source. These IP addresses should
* be in the form of a Classless Inter-Domain Routing (CIDR) block; for example, 10.0.0.0/16.
*
* @param whitelistCidr
* The range of IP addresses that should be allowed to contribute content to your source. These IP addresses
* should be in the form of a Classless Inter-Domain Routing (CIDR) block; for example, 10.0.0.0/16.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateFlowSourceRequest withWhitelistCidr(String whitelistCidr) {
setWhitelistCidr(whitelistCidr);
return this;
}
/**
* The source configuration for cloud flows receiving a stream from a bridge.
*
* @param gatewayBridgeSource
* The source configuration for cloud flows receiving a stream from a bridge.
*/
public void setGatewayBridgeSource(UpdateGatewayBridgeSourceRequest gatewayBridgeSource) {
this.gatewayBridgeSource = gatewayBridgeSource;
}
/**
* The source configuration for cloud flows receiving a stream from a bridge.
*
* @return The source configuration for cloud flows receiving a stream from a bridge.
*/
public UpdateGatewayBridgeSourceRequest getGatewayBridgeSource() {
return this.gatewayBridgeSource;
}
/**
* The source configuration for cloud flows receiving a stream from a bridge.
*
* @param gatewayBridgeSource
* The source configuration for cloud flows receiving a stream from a bridge.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateFlowSourceRequest withGatewayBridgeSource(UpdateGatewayBridgeSourceRequest gatewayBridgeSource) {
setGatewayBridgeSource(gatewayBridgeSource);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getDecryption() != null)
sb.append("Decryption: ").append(getDecryption()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getEntitlementArn() != null)
sb.append("EntitlementArn: ").append(getEntitlementArn()).append(",");
if (getFlowArn() != null)
sb.append("FlowArn: ").append(getFlowArn()).append(",");
if (getIngestPort() != null)
sb.append("IngestPort: ").append(getIngestPort()).append(",");
if (getMaxBitrate() != null)
sb.append("MaxBitrate: ").append(getMaxBitrate()).append(",");
if (getMaxLatency() != null)
sb.append("MaxLatency: ").append(getMaxLatency()).append(",");
if (getMaxSyncBuffer() != null)
sb.append("MaxSyncBuffer: ").append(getMaxSyncBuffer()).append(",");
if (getMediaStreamSourceConfigurations() != null)
sb.append("MediaStreamSourceConfigurations: ").append(getMediaStreamSourceConfigurations()).append(",");
if (getMinLatency() != null)
sb.append("MinLatency: ").append(getMinLatency()).append(",");
if (getProtocol() != null)
sb.append("Protocol: ").append(getProtocol()).append(",");
if (getSenderControlPort() != null)
sb.append("SenderControlPort: ").append(getSenderControlPort()).append(",");
if (getSenderIpAddress() != null)
sb.append("SenderIpAddress: ").append(getSenderIpAddress()).append(",");
if (getSourceArn() != null)
sb.append("SourceArn: ").append(getSourceArn()).append(",");
if (getSourceListenerAddress() != null)
sb.append("SourceListenerAddress: ").append(getSourceListenerAddress()).append(",");
if (getSourceListenerPort() != null)
sb.append("SourceListenerPort: ").append(getSourceListenerPort()).append(",");
if (getStreamId() != null)
sb.append("StreamId: ").append(getStreamId()).append(",");
if (getVpcInterfaceName() != null)
sb.append("VpcInterfaceName: ").append(getVpcInterfaceName()).append(",");
if (getWhitelistCidr() != null)
sb.append("WhitelistCidr: ").append(getWhitelistCidr()).append(",");
if (getGatewayBridgeSource() != null)
sb.append("GatewayBridgeSource: ").append(getGatewayBridgeSource());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof UpdateFlowSourceRequest == false)
return false;
UpdateFlowSourceRequest other = (UpdateFlowSourceRequest) obj;
if (other.getDecryption() == null ^ this.getDecryption() == null)
return false;
if (other.getDecryption() != null && other.getDecryption().equals(this.getDecryption()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getEntitlementArn() == null ^ this.getEntitlementArn() == null)
return false;
if (other.getEntitlementArn() != null && other.getEntitlementArn().equals(this.getEntitlementArn()) == false)
return false;
if (other.getFlowArn() == null ^ this.getFlowArn() == null)
return false;
if (other.getFlowArn() != null && other.getFlowArn().equals(this.getFlowArn()) == false)
return false;
if (other.getIngestPort() == null ^ this.getIngestPort() == null)
return false;
if (other.getIngestPort() != null && other.getIngestPort().equals(this.getIngestPort()) == false)
return false;
if (other.getMaxBitrate() == null ^ this.getMaxBitrate() == null)
return false;
if (other.getMaxBitrate() != null && other.getMaxBitrate().equals(this.getMaxBitrate()) == false)
return false;
if (other.getMaxLatency() == null ^ this.getMaxLatency() == null)
return false;
if (other.getMaxLatency() != null && other.getMaxLatency().equals(this.getMaxLatency()) == false)
return false;
if (other.getMaxSyncBuffer() == null ^ this.getMaxSyncBuffer() == null)
return false;
if (other.getMaxSyncBuffer() != null && other.getMaxSyncBuffer().equals(this.getMaxSyncBuffer()) == false)
return false;
if (other.getMediaStreamSourceConfigurations() == null ^ this.getMediaStreamSourceConfigurations() == null)
return false;
if (other.getMediaStreamSourceConfigurations() != null
&& other.getMediaStreamSourceConfigurations().equals(this.getMediaStreamSourceConfigurations()) == false)
return false;
if (other.getMinLatency() == null ^ this.getMinLatency() == null)
return false;
if (other.getMinLatency() != null && other.getMinLatency().equals(this.getMinLatency()) == false)
return false;
if (other.getProtocol() == null ^ this.getProtocol() == null)
return false;
if (other.getProtocol() != null && other.getProtocol().equals(this.getProtocol()) == false)
return false;
if (other.getSenderControlPort() == null ^ this.getSenderControlPort() == null)
return false;
if (other.getSenderControlPort() != null && other.getSenderControlPort().equals(this.getSenderControlPort()) == false)
return false;
if (other.getSenderIpAddress() == null ^ this.getSenderIpAddress() == null)
return false;
if (other.getSenderIpAddress() != null && other.getSenderIpAddress().equals(this.getSenderIpAddress()) == false)
return false;
if (other.getSourceArn() == null ^ this.getSourceArn() == null)
return false;
if (other.getSourceArn() != null && other.getSourceArn().equals(this.getSourceArn()) == false)
return false;
if (other.getSourceListenerAddress() == null ^ this.getSourceListenerAddress() == null)
return false;
if (other.getSourceListenerAddress() != null && other.getSourceListenerAddress().equals(this.getSourceListenerAddress()) == false)
return false;
if (other.getSourceListenerPort() == null ^ this.getSourceListenerPort() == null)
return false;
if (other.getSourceListenerPort() != null && other.getSourceListenerPort().equals(this.getSourceListenerPort()) == false)
return false;
if (other.getStreamId() == null ^ this.getStreamId() == null)
return false;
if (other.getStreamId() != null && other.getStreamId().equals(this.getStreamId()) == false)
return false;
if (other.getVpcInterfaceName() == null ^ this.getVpcInterfaceName() == null)
return false;
if (other.getVpcInterfaceName() != null && other.getVpcInterfaceName().equals(this.getVpcInterfaceName()) == false)
return false;
if (other.getWhitelistCidr() == null ^ this.getWhitelistCidr() == null)
return false;
if (other.getWhitelistCidr() != null && other.getWhitelistCidr().equals(this.getWhitelistCidr()) == false)
return false;
if (other.getGatewayBridgeSource() == null ^ this.getGatewayBridgeSource() == null)
return false;
if (other.getGatewayBridgeSource() != null && other.getGatewayBridgeSource().equals(this.getGatewayBridgeSource()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getDecryption() == null) ? 0 : getDecryption().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getEntitlementArn() == null) ? 0 : getEntitlementArn().hashCode());
hashCode = prime * hashCode + ((getFlowArn() == null) ? 0 : getFlowArn().hashCode());
hashCode = prime * hashCode + ((getIngestPort() == null) ? 0 : getIngestPort().hashCode());
hashCode = prime * hashCode + ((getMaxBitrate() == null) ? 0 : getMaxBitrate().hashCode());
hashCode = prime * hashCode + ((getMaxLatency() == null) ? 0 : getMaxLatency().hashCode());
hashCode = prime * hashCode + ((getMaxSyncBuffer() == null) ? 0 : getMaxSyncBuffer().hashCode());
hashCode = prime * hashCode + ((getMediaStreamSourceConfigurations() == null) ? 0 : getMediaStreamSourceConfigurations().hashCode());
hashCode = prime * hashCode + ((getMinLatency() == null) ? 0 : getMinLatency().hashCode());
hashCode = prime * hashCode + ((getProtocol() == null) ? 0 : getProtocol().hashCode());
hashCode = prime * hashCode + ((getSenderControlPort() == null) ? 0 : getSenderControlPort().hashCode());
hashCode = prime * hashCode + ((getSenderIpAddress() == null) ? 0 : getSenderIpAddress().hashCode());
hashCode = prime * hashCode + ((getSourceArn() == null) ? 0 : getSourceArn().hashCode());
hashCode = prime * hashCode + ((getSourceListenerAddress() == null) ? 0 : getSourceListenerAddress().hashCode());
hashCode = prime * hashCode + ((getSourceListenerPort() == null) ? 0 : getSourceListenerPort().hashCode());
hashCode = prime * hashCode + ((getStreamId() == null) ? 0 : getStreamId().hashCode());
hashCode = prime * hashCode + ((getVpcInterfaceName() == null) ? 0 : getVpcInterfaceName().hashCode());
hashCode = prime * hashCode + ((getWhitelistCidr() == null) ? 0 : getWhitelistCidr().hashCode());
hashCode = prime * hashCode + ((getGatewayBridgeSource() == null) ? 0 : getGatewayBridgeSource().hashCode());
return hashCode;
}
@Override
public UpdateFlowSourceRequest clone() {
return (UpdateFlowSourceRequest) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy