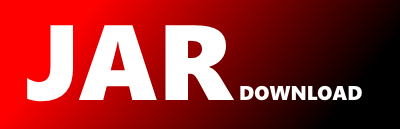
com.amazonaws.services.mediaconvert.model.AudioSelector Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws-java-sdk-mediaconvert Show documentation
Show all versions of aws-java-sdk-mediaconvert Show documentation
The AWS Java SDK for AWS Elemental MediaConvert module holds the client classes that are used for communicating with AWS Elemental MediaConvert Service
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.mediaconvert.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
* Use Audio selectors to specify a track or set of tracks from the input that you will use in your outputs. You can use
* multiple Audio selectors per input.
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AudioSelector implements Serializable, Cloneable, StructuredPojo {
/**
* Apply audio timing corrections to help synchronize audio and video in your output. To apply timing corrections,
* your input must meet the following requirements: * Container: MP4, or MOV, with an accurate time-to-sample (STTS)
* table. * Audio track: AAC. Choose from the following audio timing correction settings: * Disabled (Default): Apply
* no correction. * Auto: Recommended for most inputs. MediaConvert analyzes the audio timing in your input and
* determines which correction setting to use, if needed. * Track: Adjust the duration of each audio frame by a
* constant amount to align the audio track length with STTS duration. Track-level correction does not affect pitch,
* and is recommended for tonal audio content such as music. * Frame: Adjust the duration of each audio frame by a
* variable amount to align audio frames with STTS timestamps. No corrections are made to already-aligned frames.
* Frame-level correction may affect the pitch of corrected frames, and is recommended for atonal audio content such
* as speech or percussion.
*/
private String audioDurationCorrection;
/**
* Selects a specific language code from within an audio source, using the ISO 639-2 or ISO 639-3 three-letter
* language code
*/
private String customLanguageCode;
/**
* Enable this setting on one audio selector to set it as the default for the job. The service uses this default for
* outputs where it can't find the specified input audio. If you don't set a default, those outputs have no audio.
*/
private String defaultSelection;
/** Specifies audio data from an external file source. */
private String externalAudioFileInput;
/**
* Settings specific to audio sources in an HLS alternate rendition group. Specify the properties (renditionGroupId,
* renditionName or renditionLanguageCode) to identify the unique audio track among the alternative rendition groups
* present in the HLS manifest. If no unique track is found, or multiple tracks match the properties provided, the
* job fails. If no properties in hlsRenditionGroupSettings are specified, the default audio track within the video
* segment is chosen. If there is no audio within video segment, the alternative audio with DEFAULT=YES is chosen
* instead.
*/
private HlsRenditionGroupSettings hlsRenditionGroupSettings;
/** Selects a specific language code from within an audio source. */
private String languageCode;
/** Specifies a time delta in milliseconds to offset the audio from the input video. */
private Integer offset;
/** Selects a specific PID from within an audio source (e.g. 257 selects PID 0x101). */
private java.util.List pids;
/**
* Use this setting for input streams that contain Dolby E, to have the service extract specific program data from
* the track. To select multiple programs, create multiple selectors with the same Track and different Program
* numbers. In the console, this setting is visible when you set Selector type to Track. Choose the program number
* from the dropdown list. If your input file has incorrect metadata, you can choose All channels instead of a
* program number to have the service ignore the program IDs and include all the programs in the track.
*/
private Integer programSelection;
/**
* Use these settings to reorder the audio channels of one input to match those of another input. This allows you to
* combine the two files into a single output, one after the other.
*/
private RemixSettings remixSettings;
/** Specifies the type of the audio selector. */
private String selectorType;
/**
* Identify a track from the input audio to include in this selector by entering the track index number. To include
* several tracks in a single audio selector, specify multiple tracks as follows. Using the console, enter a
* comma-separated list. For example, type "1,2,3" to include tracks 1 through 3.
*/
private java.util.List tracks;
/**
* Apply audio timing corrections to help synchronize audio and video in your output. To apply timing corrections,
* your input must meet the following requirements: * Container: MP4, or MOV, with an accurate time-to-sample (STTS)
* table. * Audio track: AAC. Choose from the following audio timing correction settings: * Disabled (Default): Apply
* no correction. * Auto: Recommended for most inputs. MediaConvert analyzes the audio timing in your input and
* determines which correction setting to use, if needed. * Track: Adjust the duration of each audio frame by a
* constant amount to align the audio track length with STTS duration. Track-level correction does not affect pitch,
* and is recommended for tonal audio content such as music. * Frame: Adjust the duration of each audio frame by a
* variable amount to align audio frames with STTS timestamps. No corrections are made to already-aligned frames.
* Frame-level correction may affect the pitch of corrected frames, and is recommended for atonal audio content such
* as speech or percussion.
*
* @param audioDurationCorrection
* Apply audio timing corrections to help synchronize audio and video in your output. To apply timing
* corrections, your input must meet the following requirements: * Container: MP4, or MOV, with an accurate
* time-to-sample (STTS) table. * Audio track: AAC. Choose from the following audio timing correction
* settings: * Disabled (Default): Apply no correction. * Auto: Recommended for most inputs. MediaConvert
* analyzes the audio timing in your input and determines which correction setting to use, if needed. *
* Track: Adjust the duration of each audio frame by a constant amount to align the audio track length with
* STTS duration. Track-level correction does not affect pitch, and is recommended for tonal audio content
* such as music. * Frame: Adjust the duration of each audio frame by a variable amount to align audio frames
* with STTS timestamps. No corrections are made to already-aligned frames. Frame-level correction may affect
* the pitch of corrected frames, and is recommended for atonal audio content such as speech or percussion.
* @see AudioDurationCorrection
*/
public void setAudioDurationCorrection(String audioDurationCorrection) {
this.audioDurationCorrection = audioDurationCorrection;
}
/**
* Apply audio timing corrections to help synchronize audio and video in your output. To apply timing corrections,
* your input must meet the following requirements: * Container: MP4, or MOV, with an accurate time-to-sample (STTS)
* table. * Audio track: AAC. Choose from the following audio timing correction settings: * Disabled (Default): Apply
* no correction. * Auto: Recommended for most inputs. MediaConvert analyzes the audio timing in your input and
* determines which correction setting to use, if needed. * Track: Adjust the duration of each audio frame by a
* constant amount to align the audio track length with STTS duration. Track-level correction does not affect pitch,
* and is recommended for tonal audio content such as music. * Frame: Adjust the duration of each audio frame by a
* variable amount to align audio frames with STTS timestamps. No corrections are made to already-aligned frames.
* Frame-level correction may affect the pitch of corrected frames, and is recommended for atonal audio content such
* as speech or percussion.
*
* @return Apply audio timing corrections to help synchronize audio and video in your output. To apply timing
* corrections, your input must meet the following requirements: * Container: MP4, or MOV, with an accurate
* time-to-sample (STTS) table. * Audio track: AAC. Choose from the following audio timing correction
* settings: * Disabled (Default): Apply no correction. * Auto: Recommended for most inputs. MediaConvert
* analyzes the audio timing in your input and determines which correction setting to use, if needed. *
* Track: Adjust the duration of each audio frame by a constant amount to align the audio track length with
* STTS duration. Track-level correction does not affect pitch, and is recommended for tonal audio content
* such as music. * Frame: Adjust the duration of each audio frame by a variable amount to align audio
* frames with STTS timestamps. No corrections are made to already-aligned frames. Frame-level correction
* may affect the pitch of corrected frames, and is recommended for atonal audio content such as speech or
* percussion.
* @see AudioDurationCorrection
*/
public String getAudioDurationCorrection() {
return this.audioDurationCorrection;
}
/**
* Apply audio timing corrections to help synchronize audio and video in your output. To apply timing corrections,
* your input must meet the following requirements: * Container: MP4, or MOV, with an accurate time-to-sample (STTS)
* table. * Audio track: AAC. Choose from the following audio timing correction settings: * Disabled (Default): Apply
* no correction. * Auto: Recommended for most inputs. MediaConvert analyzes the audio timing in your input and
* determines which correction setting to use, if needed. * Track: Adjust the duration of each audio frame by a
* constant amount to align the audio track length with STTS duration. Track-level correction does not affect pitch,
* and is recommended for tonal audio content such as music. * Frame: Adjust the duration of each audio frame by a
* variable amount to align audio frames with STTS timestamps. No corrections are made to already-aligned frames.
* Frame-level correction may affect the pitch of corrected frames, and is recommended for atonal audio content such
* as speech or percussion.
*
* @param audioDurationCorrection
* Apply audio timing corrections to help synchronize audio and video in your output. To apply timing
* corrections, your input must meet the following requirements: * Container: MP4, or MOV, with an accurate
* time-to-sample (STTS) table. * Audio track: AAC. Choose from the following audio timing correction
* settings: * Disabled (Default): Apply no correction. * Auto: Recommended for most inputs. MediaConvert
* analyzes the audio timing in your input and determines which correction setting to use, if needed. *
* Track: Adjust the duration of each audio frame by a constant amount to align the audio track length with
* STTS duration. Track-level correction does not affect pitch, and is recommended for tonal audio content
* such as music. * Frame: Adjust the duration of each audio frame by a variable amount to align audio frames
* with STTS timestamps. No corrections are made to already-aligned frames. Frame-level correction may affect
* the pitch of corrected frames, and is recommended for atonal audio content such as speech or percussion.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AudioDurationCorrection
*/
public AudioSelector withAudioDurationCorrection(String audioDurationCorrection) {
setAudioDurationCorrection(audioDurationCorrection);
return this;
}
/**
* Apply audio timing corrections to help synchronize audio and video in your output. To apply timing corrections,
* your input must meet the following requirements: * Container: MP4, or MOV, with an accurate time-to-sample (STTS)
* table. * Audio track: AAC. Choose from the following audio timing correction settings: * Disabled (Default): Apply
* no correction. * Auto: Recommended for most inputs. MediaConvert analyzes the audio timing in your input and
* determines which correction setting to use, if needed. * Track: Adjust the duration of each audio frame by a
* constant amount to align the audio track length with STTS duration. Track-level correction does not affect pitch,
* and is recommended for tonal audio content such as music. * Frame: Adjust the duration of each audio frame by a
* variable amount to align audio frames with STTS timestamps. No corrections are made to already-aligned frames.
* Frame-level correction may affect the pitch of corrected frames, and is recommended for atonal audio content such
* as speech or percussion.
*
* @param audioDurationCorrection
* Apply audio timing corrections to help synchronize audio and video in your output. To apply timing
* corrections, your input must meet the following requirements: * Container: MP4, or MOV, with an accurate
* time-to-sample (STTS) table. * Audio track: AAC. Choose from the following audio timing correction
* settings: * Disabled (Default): Apply no correction. * Auto: Recommended for most inputs. MediaConvert
* analyzes the audio timing in your input and determines which correction setting to use, if needed. *
* Track: Adjust the duration of each audio frame by a constant amount to align the audio track length with
* STTS duration. Track-level correction does not affect pitch, and is recommended for tonal audio content
* such as music. * Frame: Adjust the duration of each audio frame by a variable amount to align audio frames
* with STTS timestamps. No corrections are made to already-aligned frames. Frame-level correction may affect
* the pitch of corrected frames, and is recommended for atonal audio content such as speech or percussion.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AudioDurationCorrection
*/
public AudioSelector withAudioDurationCorrection(AudioDurationCorrection audioDurationCorrection) {
this.audioDurationCorrection = audioDurationCorrection.toString();
return this;
}
/**
* Selects a specific language code from within an audio source, using the ISO 639-2 or ISO 639-3 three-letter
* language code
*
* @param customLanguageCode
* Selects a specific language code from within an audio source, using the ISO 639-2 or ISO 639-3
* three-letter language code
*/
public void setCustomLanguageCode(String customLanguageCode) {
this.customLanguageCode = customLanguageCode;
}
/**
* Selects a specific language code from within an audio source, using the ISO 639-2 or ISO 639-3 three-letter
* language code
*
* @return Selects a specific language code from within an audio source, using the ISO 639-2 or ISO 639-3
* three-letter language code
*/
public String getCustomLanguageCode() {
return this.customLanguageCode;
}
/**
* Selects a specific language code from within an audio source, using the ISO 639-2 or ISO 639-3 three-letter
* language code
*
* @param customLanguageCode
* Selects a specific language code from within an audio source, using the ISO 639-2 or ISO 639-3
* three-letter language code
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AudioSelector withCustomLanguageCode(String customLanguageCode) {
setCustomLanguageCode(customLanguageCode);
return this;
}
/**
* Enable this setting on one audio selector to set it as the default for the job. The service uses this default for
* outputs where it can't find the specified input audio. If you don't set a default, those outputs have no audio.
*
* @param defaultSelection
* Enable this setting on one audio selector to set it as the default for the job. The service uses this
* default for outputs where it can't find the specified input audio. If you don't set a default, those
* outputs have no audio.
* @see AudioDefaultSelection
*/
public void setDefaultSelection(String defaultSelection) {
this.defaultSelection = defaultSelection;
}
/**
* Enable this setting on one audio selector to set it as the default for the job. The service uses this default for
* outputs where it can't find the specified input audio. If you don't set a default, those outputs have no audio.
*
* @return Enable this setting on one audio selector to set it as the default for the job. The service uses this
* default for outputs where it can't find the specified input audio. If you don't set a default, those
* outputs have no audio.
* @see AudioDefaultSelection
*/
public String getDefaultSelection() {
return this.defaultSelection;
}
/**
* Enable this setting on one audio selector to set it as the default for the job. The service uses this default for
* outputs where it can't find the specified input audio. If you don't set a default, those outputs have no audio.
*
* @param defaultSelection
* Enable this setting on one audio selector to set it as the default for the job. The service uses this
* default for outputs where it can't find the specified input audio. If you don't set a default, those
* outputs have no audio.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AudioDefaultSelection
*/
public AudioSelector withDefaultSelection(String defaultSelection) {
setDefaultSelection(defaultSelection);
return this;
}
/**
* Enable this setting on one audio selector to set it as the default for the job. The service uses this default for
* outputs where it can't find the specified input audio. If you don't set a default, those outputs have no audio.
*
* @param defaultSelection
* Enable this setting on one audio selector to set it as the default for the job. The service uses this
* default for outputs where it can't find the specified input audio. If you don't set a default, those
* outputs have no audio.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AudioDefaultSelection
*/
public AudioSelector withDefaultSelection(AudioDefaultSelection defaultSelection) {
this.defaultSelection = defaultSelection.toString();
return this;
}
/**
* Specifies audio data from an external file source.
*
* @param externalAudioFileInput
* Specifies audio data from an external file source.
*/
public void setExternalAudioFileInput(String externalAudioFileInput) {
this.externalAudioFileInput = externalAudioFileInput;
}
/**
* Specifies audio data from an external file source.
*
* @return Specifies audio data from an external file source.
*/
public String getExternalAudioFileInput() {
return this.externalAudioFileInput;
}
/**
* Specifies audio data from an external file source.
*
* @param externalAudioFileInput
* Specifies audio data from an external file source.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AudioSelector withExternalAudioFileInput(String externalAudioFileInput) {
setExternalAudioFileInput(externalAudioFileInput);
return this;
}
/**
* Settings specific to audio sources in an HLS alternate rendition group. Specify the properties (renditionGroupId,
* renditionName or renditionLanguageCode) to identify the unique audio track among the alternative rendition groups
* present in the HLS manifest. If no unique track is found, or multiple tracks match the properties provided, the
* job fails. If no properties in hlsRenditionGroupSettings are specified, the default audio track within the video
* segment is chosen. If there is no audio within video segment, the alternative audio with DEFAULT=YES is chosen
* instead.
*
* @param hlsRenditionGroupSettings
* Settings specific to audio sources in an HLS alternate rendition group. Specify the properties
* (renditionGroupId, renditionName or renditionLanguageCode) to identify the unique audio track among the
* alternative rendition groups present in the HLS manifest. If no unique track is found, or multiple tracks
* match the properties provided, the job fails. If no properties in hlsRenditionGroupSettings are specified,
* the default audio track within the video segment is chosen. If there is no audio within video segment, the
* alternative audio with DEFAULT=YES is chosen instead.
*/
public void setHlsRenditionGroupSettings(HlsRenditionGroupSettings hlsRenditionGroupSettings) {
this.hlsRenditionGroupSettings = hlsRenditionGroupSettings;
}
/**
* Settings specific to audio sources in an HLS alternate rendition group. Specify the properties (renditionGroupId,
* renditionName or renditionLanguageCode) to identify the unique audio track among the alternative rendition groups
* present in the HLS manifest. If no unique track is found, or multiple tracks match the properties provided, the
* job fails. If no properties in hlsRenditionGroupSettings are specified, the default audio track within the video
* segment is chosen. If there is no audio within video segment, the alternative audio with DEFAULT=YES is chosen
* instead.
*
* @return Settings specific to audio sources in an HLS alternate rendition group. Specify the properties
* (renditionGroupId, renditionName or renditionLanguageCode) to identify the unique audio track among the
* alternative rendition groups present in the HLS manifest. If no unique track is found, or multiple tracks
* match the properties provided, the job fails. If no properties in hlsRenditionGroupSettings are
* specified, the default audio track within the video segment is chosen. If there is no audio within video
* segment, the alternative audio with DEFAULT=YES is chosen instead.
*/
public HlsRenditionGroupSettings getHlsRenditionGroupSettings() {
return this.hlsRenditionGroupSettings;
}
/**
* Settings specific to audio sources in an HLS alternate rendition group. Specify the properties (renditionGroupId,
* renditionName or renditionLanguageCode) to identify the unique audio track among the alternative rendition groups
* present in the HLS manifest. If no unique track is found, or multiple tracks match the properties provided, the
* job fails. If no properties in hlsRenditionGroupSettings are specified, the default audio track within the video
* segment is chosen. If there is no audio within video segment, the alternative audio with DEFAULT=YES is chosen
* instead.
*
* @param hlsRenditionGroupSettings
* Settings specific to audio sources in an HLS alternate rendition group. Specify the properties
* (renditionGroupId, renditionName or renditionLanguageCode) to identify the unique audio track among the
* alternative rendition groups present in the HLS manifest. If no unique track is found, or multiple tracks
* match the properties provided, the job fails. If no properties in hlsRenditionGroupSettings are specified,
* the default audio track within the video segment is chosen. If there is no audio within video segment, the
* alternative audio with DEFAULT=YES is chosen instead.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AudioSelector withHlsRenditionGroupSettings(HlsRenditionGroupSettings hlsRenditionGroupSettings) {
setHlsRenditionGroupSettings(hlsRenditionGroupSettings);
return this;
}
/**
* Selects a specific language code from within an audio source.
*
* @param languageCode
* Selects a specific language code from within an audio source.
* @see LanguageCode
*/
public void setLanguageCode(String languageCode) {
this.languageCode = languageCode;
}
/**
* Selects a specific language code from within an audio source.
*
* @return Selects a specific language code from within an audio source.
* @see LanguageCode
*/
public String getLanguageCode() {
return this.languageCode;
}
/**
* Selects a specific language code from within an audio source.
*
* @param languageCode
* Selects a specific language code from within an audio source.
* @return Returns a reference to this object so that method calls can be chained together.
* @see LanguageCode
*/
public AudioSelector withLanguageCode(String languageCode) {
setLanguageCode(languageCode);
return this;
}
/**
* Selects a specific language code from within an audio source.
*
* @param languageCode
* Selects a specific language code from within an audio source.
* @return Returns a reference to this object so that method calls can be chained together.
* @see LanguageCode
*/
public AudioSelector withLanguageCode(LanguageCode languageCode) {
this.languageCode = languageCode.toString();
return this;
}
/**
* Specifies a time delta in milliseconds to offset the audio from the input video.
*
* @param offset
* Specifies a time delta in milliseconds to offset the audio from the input video.
*/
public void setOffset(Integer offset) {
this.offset = offset;
}
/**
* Specifies a time delta in milliseconds to offset the audio from the input video.
*
* @return Specifies a time delta in milliseconds to offset the audio from the input video.
*/
public Integer getOffset() {
return this.offset;
}
/**
* Specifies a time delta in milliseconds to offset the audio from the input video.
*
* @param offset
* Specifies a time delta in milliseconds to offset the audio from the input video.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AudioSelector withOffset(Integer offset) {
setOffset(offset);
return this;
}
/**
* Selects a specific PID from within an audio source (e.g. 257 selects PID 0x101).
*
* @return Selects a specific PID from within an audio source (e.g. 257 selects PID 0x101).
*/
public java.util.List getPids() {
return pids;
}
/**
* Selects a specific PID from within an audio source (e.g. 257 selects PID 0x101).
*
* @param pids
* Selects a specific PID from within an audio source (e.g. 257 selects PID 0x101).
*/
public void setPids(java.util.Collection pids) {
if (pids == null) {
this.pids = null;
return;
}
this.pids = new java.util.ArrayList(pids);
}
/**
* Selects a specific PID from within an audio source (e.g. 257 selects PID 0x101).
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setPids(java.util.Collection)} or {@link #withPids(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param pids
* Selects a specific PID from within an audio source (e.g. 257 selects PID 0x101).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AudioSelector withPids(Integer... pids) {
if (this.pids == null) {
setPids(new java.util.ArrayList(pids.length));
}
for (Integer ele : pids) {
this.pids.add(ele);
}
return this;
}
/**
* Selects a specific PID from within an audio source (e.g. 257 selects PID 0x101).
*
* @param pids
* Selects a specific PID from within an audio source (e.g. 257 selects PID 0x101).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AudioSelector withPids(java.util.Collection pids) {
setPids(pids);
return this;
}
/**
* Use this setting for input streams that contain Dolby E, to have the service extract specific program data from
* the track. To select multiple programs, create multiple selectors with the same Track and different Program
* numbers. In the console, this setting is visible when you set Selector type to Track. Choose the program number
* from the dropdown list. If your input file has incorrect metadata, you can choose All channels instead of a
* program number to have the service ignore the program IDs and include all the programs in the track.
*
* @param programSelection
* Use this setting for input streams that contain Dolby E, to have the service extract specific program data
* from the track. To select multiple programs, create multiple selectors with the same Track and different
* Program numbers. In the console, this setting is visible when you set Selector type to Track. Choose the
* program number from the dropdown list. If your input file has incorrect metadata, you can choose All
* channels instead of a program number to have the service ignore the program IDs and include all the
* programs in the track.
*/
public void setProgramSelection(Integer programSelection) {
this.programSelection = programSelection;
}
/**
* Use this setting for input streams that contain Dolby E, to have the service extract specific program data from
* the track. To select multiple programs, create multiple selectors with the same Track and different Program
* numbers. In the console, this setting is visible when you set Selector type to Track. Choose the program number
* from the dropdown list. If your input file has incorrect metadata, you can choose All channels instead of a
* program number to have the service ignore the program IDs and include all the programs in the track.
*
* @return Use this setting for input streams that contain Dolby E, to have the service extract specific program
* data from the track. To select multiple programs, create multiple selectors with the same Track and
* different Program numbers. In the console, this setting is visible when you set Selector type to Track.
* Choose the program number from the dropdown list. If your input file has incorrect metadata, you can
* choose All channels instead of a program number to have the service ignore the program IDs and include
* all the programs in the track.
*/
public Integer getProgramSelection() {
return this.programSelection;
}
/**
* Use this setting for input streams that contain Dolby E, to have the service extract specific program data from
* the track. To select multiple programs, create multiple selectors with the same Track and different Program
* numbers. In the console, this setting is visible when you set Selector type to Track. Choose the program number
* from the dropdown list. If your input file has incorrect metadata, you can choose All channels instead of a
* program number to have the service ignore the program IDs and include all the programs in the track.
*
* @param programSelection
* Use this setting for input streams that contain Dolby E, to have the service extract specific program data
* from the track. To select multiple programs, create multiple selectors with the same Track and different
* Program numbers. In the console, this setting is visible when you set Selector type to Track. Choose the
* program number from the dropdown list. If your input file has incorrect metadata, you can choose All
* channels instead of a program number to have the service ignore the program IDs and include all the
* programs in the track.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AudioSelector withProgramSelection(Integer programSelection) {
setProgramSelection(programSelection);
return this;
}
/**
* Use these settings to reorder the audio channels of one input to match those of another input. This allows you to
* combine the two files into a single output, one after the other.
*
* @param remixSettings
* Use these settings to reorder the audio channels of one input to match those of another input. This allows
* you to combine the two files into a single output, one after the other.
*/
public void setRemixSettings(RemixSettings remixSettings) {
this.remixSettings = remixSettings;
}
/**
* Use these settings to reorder the audio channels of one input to match those of another input. This allows you to
* combine the two files into a single output, one after the other.
*
* @return Use these settings to reorder the audio channels of one input to match those of another input. This
* allows you to combine the two files into a single output, one after the other.
*/
public RemixSettings getRemixSettings() {
return this.remixSettings;
}
/**
* Use these settings to reorder the audio channels of one input to match those of another input. This allows you to
* combine the two files into a single output, one after the other.
*
* @param remixSettings
* Use these settings to reorder the audio channels of one input to match those of another input. This allows
* you to combine the two files into a single output, one after the other.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AudioSelector withRemixSettings(RemixSettings remixSettings) {
setRemixSettings(remixSettings);
return this;
}
/**
* Specifies the type of the audio selector.
*
* @param selectorType
* Specifies the type of the audio selector.
* @see AudioSelectorType
*/
public void setSelectorType(String selectorType) {
this.selectorType = selectorType;
}
/**
* Specifies the type of the audio selector.
*
* @return Specifies the type of the audio selector.
* @see AudioSelectorType
*/
public String getSelectorType() {
return this.selectorType;
}
/**
* Specifies the type of the audio selector.
*
* @param selectorType
* Specifies the type of the audio selector.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AudioSelectorType
*/
public AudioSelector withSelectorType(String selectorType) {
setSelectorType(selectorType);
return this;
}
/**
* Specifies the type of the audio selector.
*
* @param selectorType
* Specifies the type of the audio selector.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AudioSelectorType
*/
public AudioSelector withSelectorType(AudioSelectorType selectorType) {
this.selectorType = selectorType.toString();
return this;
}
/**
* Identify a track from the input audio to include in this selector by entering the track index number. To include
* several tracks in a single audio selector, specify multiple tracks as follows. Using the console, enter a
* comma-separated list. For example, type "1,2,3" to include tracks 1 through 3.
*
* @return Identify a track from the input audio to include in this selector by entering the track index number. To
* include several tracks in a single audio selector, specify multiple tracks as follows. Using the console,
* enter a comma-separated list. For example, type "1,2,3" to include tracks 1 through 3.
*/
public java.util.List getTracks() {
return tracks;
}
/**
* Identify a track from the input audio to include in this selector by entering the track index number. To include
* several tracks in a single audio selector, specify multiple tracks as follows. Using the console, enter a
* comma-separated list. For example, type "1,2,3" to include tracks 1 through 3.
*
* @param tracks
* Identify a track from the input audio to include in this selector by entering the track index number. To
* include several tracks in a single audio selector, specify multiple tracks as follows. Using the console,
* enter a comma-separated list. For example, type "1,2,3" to include tracks 1 through 3.
*/
public void setTracks(java.util.Collection tracks) {
if (tracks == null) {
this.tracks = null;
return;
}
this.tracks = new java.util.ArrayList(tracks);
}
/**
* Identify a track from the input audio to include in this selector by entering the track index number. To include
* several tracks in a single audio selector, specify multiple tracks as follows. Using the console, enter a
* comma-separated list. For example, type "1,2,3" to include tracks 1 through 3.
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTracks(java.util.Collection)} or {@link #withTracks(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tracks
* Identify a track from the input audio to include in this selector by entering the track index number. To
* include several tracks in a single audio selector, specify multiple tracks as follows. Using the console,
* enter a comma-separated list. For example, type "1,2,3" to include tracks 1 through 3.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AudioSelector withTracks(Integer... tracks) {
if (this.tracks == null) {
setTracks(new java.util.ArrayList(tracks.length));
}
for (Integer ele : tracks) {
this.tracks.add(ele);
}
return this;
}
/**
* Identify a track from the input audio to include in this selector by entering the track index number. To include
* several tracks in a single audio selector, specify multiple tracks as follows. Using the console, enter a
* comma-separated list. For example, type "1,2,3" to include tracks 1 through 3.
*
* @param tracks
* Identify a track from the input audio to include in this selector by entering the track index number. To
* include several tracks in a single audio selector, specify multiple tracks as follows. Using the console,
* enter a comma-separated list. For example, type "1,2,3" to include tracks 1 through 3.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AudioSelector withTracks(java.util.Collection tracks) {
setTracks(tracks);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getAudioDurationCorrection() != null)
sb.append("AudioDurationCorrection: ").append(getAudioDurationCorrection()).append(",");
if (getCustomLanguageCode() != null)
sb.append("CustomLanguageCode: ").append(getCustomLanguageCode()).append(",");
if (getDefaultSelection() != null)
sb.append("DefaultSelection: ").append(getDefaultSelection()).append(",");
if (getExternalAudioFileInput() != null)
sb.append("ExternalAudioFileInput: ").append(getExternalAudioFileInput()).append(",");
if (getHlsRenditionGroupSettings() != null)
sb.append("HlsRenditionGroupSettings: ").append(getHlsRenditionGroupSettings()).append(",");
if (getLanguageCode() != null)
sb.append("LanguageCode: ").append(getLanguageCode()).append(",");
if (getOffset() != null)
sb.append("Offset: ").append(getOffset()).append(",");
if (getPids() != null)
sb.append("Pids: ").append(getPids()).append(",");
if (getProgramSelection() != null)
sb.append("ProgramSelection: ").append(getProgramSelection()).append(",");
if (getRemixSettings() != null)
sb.append("RemixSettings: ").append(getRemixSettings()).append(",");
if (getSelectorType() != null)
sb.append("SelectorType: ").append(getSelectorType()).append(",");
if (getTracks() != null)
sb.append("Tracks: ").append(getTracks());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof AudioSelector == false)
return false;
AudioSelector other = (AudioSelector) obj;
if (other.getAudioDurationCorrection() == null ^ this.getAudioDurationCorrection() == null)
return false;
if (other.getAudioDurationCorrection() != null && other.getAudioDurationCorrection().equals(this.getAudioDurationCorrection()) == false)
return false;
if (other.getCustomLanguageCode() == null ^ this.getCustomLanguageCode() == null)
return false;
if (other.getCustomLanguageCode() != null && other.getCustomLanguageCode().equals(this.getCustomLanguageCode()) == false)
return false;
if (other.getDefaultSelection() == null ^ this.getDefaultSelection() == null)
return false;
if (other.getDefaultSelection() != null && other.getDefaultSelection().equals(this.getDefaultSelection()) == false)
return false;
if (other.getExternalAudioFileInput() == null ^ this.getExternalAudioFileInput() == null)
return false;
if (other.getExternalAudioFileInput() != null && other.getExternalAudioFileInput().equals(this.getExternalAudioFileInput()) == false)
return false;
if (other.getHlsRenditionGroupSettings() == null ^ this.getHlsRenditionGroupSettings() == null)
return false;
if (other.getHlsRenditionGroupSettings() != null && other.getHlsRenditionGroupSettings().equals(this.getHlsRenditionGroupSettings()) == false)
return false;
if (other.getLanguageCode() == null ^ this.getLanguageCode() == null)
return false;
if (other.getLanguageCode() != null && other.getLanguageCode().equals(this.getLanguageCode()) == false)
return false;
if (other.getOffset() == null ^ this.getOffset() == null)
return false;
if (other.getOffset() != null && other.getOffset().equals(this.getOffset()) == false)
return false;
if (other.getPids() == null ^ this.getPids() == null)
return false;
if (other.getPids() != null && other.getPids().equals(this.getPids()) == false)
return false;
if (other.getProgramSelection() == null ^ this.getProgramSelection() == null)
return false;
if (other.getProgramSelection() != null && other.getProgramSelection().equals(this.getProgramSelection()) == false)
return false;
if (other.getRemixSettings() == null ^ this.getRemixSettings() == null)
return false;
if (other.getRemixSettings() != null && other.getRemixSettings().equals(this.getRemixSettings()) == false)
return false;
if (other.getSelectorType() == null ^ this.getSelectorType() == null)
return false;
if (other.getSelectorType() != null && other.getSelectorType().equals(this.getSelectorType()) == false)
return false;
if (other.getTracks() == null ^ this.getTracks() == null)
return false;
if (other.getTracks() != null && other.getTracks().equals(this.getTracks()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getAudioDurationCorrection() == null) ? 0 : getAudioDurationCorrection().hashCode());
hashCode = prime * hashCode + ((getCustomLanguageCode() == null) ? 0 : getCustomLanguageCode().hashCode());
hashCode = prime * hashCode + ((getDefaultSelection() == null) ? 0 : getDefaultSelection().hashCode());
hashCode = prime * hashCode + ((getExternalAudioFileInput() == null) ? 0 : getExternalAudioFileInput().hashCode());
hashCode = prime * hashCode + ((getHlsRenditionGroupSettings() == null) ? 0 : getHlsRenditionGroupSettings().hashCode());
hashCode = prime * hashCode + ((getLanguageCode() == null) ? 0 : getLanguageCode().hashCode());
hashCode = prime * hashCode + ((getOffset() == null) ? 0 : getOffset().hashCode());
hashCode = prime * hashCode + ((getPids() == null) ? 0 : getPids().hashCode());
hashCode = prime * hashCode + ((getProgramSelection() == null) ? 0 : getProgramSelection().hashCode());
hashCode = prime * hashCode + ((getRemixSettings() == null) ? 0 : getRemixSettings().hashCode());
hashCode = prime * hashCode + ((getSelectorType() == null) ? 0 : getSelectorType().hashCode());
hashCode = prime * hashCode + ((getTracks() == null) ? 0 : getTracks().hashCode());
return hashCode;
}
@Override
public AudioSelector clone() {
try {
return (AudioSelector) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.mediaconvert.model.transform.AudioSelectorMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy