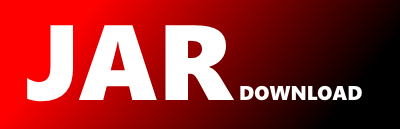
com.amazonaws.services.mediapackage.AWSMediaPackageAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-mediapackage Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.mediapackage;
import javax.annotation.Generated;
import com.amazonaws.services.mediapackage.model.*;
/**
* Interface for accessing MediaPackage asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.mediapackage.AbstractAWSMediaPackageAsync} instead.
*
*
* AWS Elemental MediaPackage
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSMediaPackageAsync extends AWSMediaPackage {
/**
* Changes the Channel's properities to configure log subscription
*
* @param configureLogsRequest
* the option to configure log subscription.
* @return A Java Future containing the result of the ConfigureLogs operation returned by the service.
* @sample AWSMediaPackageAsync.ConfigureLogs
* @see AWS API
* Documentation
*/
java.util.concurrent.Future configureLogsAsync(ConfigureLogsRequest configureLogsRequest);
/**
* Changes the Channel's properities to configure log subscription
*
* @param configureLogsRequest
* the option to configure log subscription.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ConfigureLogs operation returned by the service.
* @sample AWSMediaPackageAsyncHandler.ConfigureLogs
* @see AWS API
* Documentation
*/
java.util.concurrent.Future configureLogsAsync(ConfigureLogsRequest configureLogsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Creates a new Channel.
*
* @param createChannelRequest
* A new Channel configuration.
* @return A Java Future containing the result of the CreateChannel operation returned by the service.
* @sample AWSMediaPackageAsync.CreateChannel
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createChannelAsync(CreateChannelRequest createChannelRequest);
/**
* Creates a new Channel.
*
* @param createChannelRequest
* A new Channel configuration.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateChannel operation returned by the service.
* @sample AWSMediaPackageAsyncHandler.CreateChannel
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createChannelAsync(CreateChannelRequest createChannelRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Creates a new HarvestJob record.
*
* @param createHarvestJobRequest
* Configuration parameters used to create a new HarvestJob.
* @return A Java Future containing the result of the CreateHarvestJob operation returned by the service.
* @sample AWSMediaPackageAsync.CreateHarvestJob
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createHarvestJobAsync(CreateHarvestJobRequest createHarvestJobRequest);
/**
* Creates a new HarvestJob record.
*
* @param createHarvestJobRequest
* Configuration parameters used to create a new HarvestJob.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateHarvestJob operation returned by the service.
* @sample AWSMediaPackageAsyncHandler.CreateHarvestJob
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createHarvestJobAsync(CreateHarvestJobRequest createHarvestJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Creates a new OriginEndpoint record.
*
* @param createOriginEndpointRequest
* Configuration parameters used to create a new OriginEndpoint.
* @return A Java Future containing the result of the CreateOriginEndpoint operation returned by the service.
* @sample AWSMediaPackageAsync.CreateOriginEndpoint
* @see AWS API Documentation
*/
java.util.concurrent.Future createOriginEndpointAsync(CreateOriginEndpointRequest createOriginEndpointRequest);
/**
* Creates a new OriginEndpoint record.
*
* @param createOriginEndpointRequest
* Configuration parameters used to create a new OriginEndpoint.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateOriginEndpoint operation returned by the service.
* @sample AWSMediaPackageAsyncHandler.CreateOriginEndpoint
* @see AWS API Documentation
*/
java.util.concurrent.Future createOriginEndpointAsync(CreateOriginEndpointRequest createOriginEndpointRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Deletes an existing Channel.
*
* @param deleteChannelRequest
* @return A Java Future containing the result of the DeleteChannel operation returned by the service.
* @sample AWSMediaPackageAsync.DeleteChannel
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteChannelAsync(DeleteChannelRequest deleteChannelRequest);
/**
* Deletes an existing Channel.
*
* @param deleteChannelRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteChannel operation returned by the service.
* @sample AWSMediaPackageAsyncHandler.DeleteChannel
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteChannelAsync(DeleteChannelRequest deleteChannelRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Deletes an existing OriginEndpoint.
*
* @param deleteOriginEndpointRequest
* @return A Java Future containing the result of the DeleteOriginEndpoint operation returned by the service.
* @sample AWSMediaPackageAsync.DeleteOriginEndpoint
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteOriginEndpointAsync(DeleteOriginEndpointRequest deleteOriginEndpointRequest);
/**
* Deletes an existing OriginEndpoint.
*
* @param deleteOriginEndpointRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteOriginEndpoint operation returned by the service.
* @sample AWSMediaPackageAsyncHandler.DeleteOriginEndpoint
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteOriginEndpointAsync(DeleteOriginEndpointRequest deleteOriginEndpointRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Gets details about a Channel.
*
* @param describeChannelRequest
* @return A Java Future containing the result of the DescribeChannel operation returned by the service.
* @sample AWSMediaPackageAsync.DescribeChannel
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeChannelAsync(DescribeChannelRequest describeChannelRequest);
/**
* Gets details about a Channel.
*
* @param describeChannelRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeChannel operation returned by the service.
* @sample AWSMediaPackageAsyncHandler.DescribeChannel
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeChannelAsync(DescribeChannelRequest describeChannelRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Gets details about an existing HarvestJob.
*
* @param describeHarvestJobRequest
* @return A Java Future containing the result of the DescribeHarvestJob operation returned by the service.
* @sample AWSMediaPackageAsync.DescribeHarvestJob
* @see AWS API Documentation
*/
java.util.concurrent.Future describeHarvestJobAsync(DescribeHarvestJobRequest describeHarvestJobRequest);
/**
* Gets details about an existing HarvestJob.
*
* @param describeHarvestJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeHarvestJob operation returned by the service.
* @sample AWSMediaPackageAsyncHandler.DescribeHarvestJob
* @see AWS API Documentation
*/
java.util.concurrent.Future describeHarvestJobAsync(DescribeHarvestJobRequest describeHarvestJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Gets details about an existing OriginEndpoint.
*
* @param describeOriginEndpointRequest
* @return A Java Future containing the result of the DescribeOriginEndpoint operation returned by the service.
* @sample AWSMediaPackageAsync.DescribeOriginEndpoint
* @see AWS API Documentation
*/
java.util.concurrent.Future describeOriginEndpointAsync(DescribeOriginEndpointRequest describeOriginEndpointRequest);
/**
* Gets details about an existing OriginEndpoint.
*
* @param describeOriginEndpointRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeOriginEndpoint operation returned by the service.
* @sample AWSMediaPackageAsyncHandler.DescribeOriginEndpoint
* @see AWS API Documentation
*/
java.util.concurrent.Future describeOriginEndpointAsync(DescribeOriginEndpointRequest describeOriginEndpointRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Returns a collection of Channels.
*
* @param listChannelsRequest
* @return A Java Future containing the result of the ListChannels operation returned by the service.
* @sample AWSMediaPackageAsync.ListChannels
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listChannelsAsync(ListChannelsRequest listChannelsRequest);
/**
* Returns a collection of Channels.
*
* @param listChannelsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListChannels operation returned by the service.
* @sample AWSMediaPackageAsyncHandler.ListChannels
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listChannelsAsync(ListChannelsRequest listChannelsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Returns a collection of HarvestJob records.
*
* @param listHarvestJobsRequest
* @return A Java Future containing the result of the ListHarvestJobs operation returned by the service.
* @sample AWSMediaPackageAsync.ListHarvestJobs
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listHarvestJobsAsync(ListHarvestJobsRequest listHarvestJobsRequest);
/**
* Returns a collection of HarvestJob records.
*
* @param listHarvestJobsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListHarvestJobs operation returned by the service.
* @sample AWSMediaPackageAsyncHandler.ListHarvestJobs
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listHarvestJobsAsync(ListHarvestJobsRequest listHarvestJobsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Returns a collection of OriginEndpoint records.
*
* @param listOriginEndpointsRequest
* @return A Java Future containing the result of the ListOriginEndpoints operation returned by the service.
* @sample AWSMediaPackageAsync.ListOriginEndpoints
* @see AWS API Documentation
*/
java.util.concurrent.Future listOriginEndpointsAsync(ListOriginEndpointsRequest listOriginEndpointsRequest);
/**
* Returns a collection of OriginEndpoint records.
*
* @param listOriginEndpointsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListOriginEndpoints operation returned by the service.
* @sample AWSMediaPackageAsyncHandler.ListOriginEndpoints
* @see AWS API Documentation
*/
java.util.concurrent.Future listOriginEndpointsAsync(ListOriginEndpointsRequest listOriginEndpointsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSMediaPackageAsync.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSMediaPackageAsyncHandler.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Changes the Channel's first IngestEndpoint's username and password. WARNING - This API is deprecated. Please use
* RotateIngestEndpointCredentials instead
*
* @param rotateChannelCredentialsRequest
* @return A Java Future containing the result of the RotateChannelCredentials operation returned by the service.
* @sample AWSMediaPackageAsync.RotateChannelCredentials
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future rotateChannelCredentialsAsync(RotateChannelCredentialsRequest rotateChannelCredentialsRequest);
/**
* Changes the Channel's first IngestEndpoint's username and password. WARNING - This API is deprecated. Please use
* RotateIngestEndpointCredentials instead
*
* @param rotateChannelCredentialsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RotateChannelCredentials operation returned by the service.
* @sample AWSMediaPackageAsyncHandler.RotateChannelCredentials
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future rotateChannelCredentialsAsync(RotateChannelCredentialsRequest rotateChannelCredentialsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Rotate the IngestEndpoint's username and password, as specified by the IngestEndpoint's id.
*
* @param rotateIngestEndpointCredentialsRequest
* @return A Java Future containing the result of the RotateIngestEndpointCredentials operation returned by the
* service.
* @sample AWSMediaPackageAsync.RotateIngestEndpointCredentials
* @see AWS API Documentation
*/
java.util.concurrent.Future rotateIngestEndpointCredentialsAsync(
RotateIngestEndpointCredentialsRequest rotateIngestEndpointCredentialsRequest);
/**
* Rotate the IngestEndpoint's username and password, as specified by the IngestEndpoint's id.
*
* @param rotateIngestEndpointCredentialsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RotateIngestEndpointCredentials operation returned by the
* service.
* @sample AWSMediaPackageAsyncHandler.RotateIngestEndpointCredentials
* @see AWS API Documentation
*/
java.util.concurrent.Future rotateIngestEndpointCredentialsAsync(
RotateIngestEndpointCredentialsRequest rotateIngestEndpointCredentialsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSMediaPackageAsync.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSMediaPackageAsyncHandler.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSMediaPackageAsync.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSMediaPackageAsyncHandler.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Updates an existing Channel.
*
* @param updateChannelRequest
* Configuration parameters used to update the Channel.
* @return A Java Future containing the result of the UpdateChannel operation returned by the service.
* @sample AWSMediaPackageAsync.UpdateChannel
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateChannelAsync(UpdateChannelRequest updateChannelRequest);
/**
* Updates an existing Channel.
*
* @param updateChannelRequest
* Configuration parameters used to update the Channel.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateChannel operation returned by the service.
* @sample AWSMediaPackageAsyncHandler.UpdateChannel
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateChannelAsync(UpdateChannelRequest updateChannelRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Updates an existing OriginEndpoint.
*
* @param updateOriginEndpointRequest
* Configuration parameters used to update an existing OriginEndpoint.
* @return A Java Future containing the result of the UpdateOriginEndpoint operation returned by the service.
* @sample AWSMediaPackageAsync.UpdateOriginEndpoint
* @see AWS API Documentation
*/
java.util.concurrent.Future updateOriginEndpointAsync(UpdateOriginEndpointRequest updateOriginEndpointRequest);
/**
* Updates an existing OriginEndpoint.
*
* @param updateOriginEndpointRequest
* Configuration parameters used to update an existing OriginEndpoint.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateOriginEndpoint operation returned by the service.
* @sample AWSMediaPackageAsyncHandler.UpdateOriginEndpoint
* @see AWS API Documentation
*/
java.util.concurrent.Future updateOriginEndpointAsync(UpdateOriginEndpointRequest updateOriginEndpointRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}