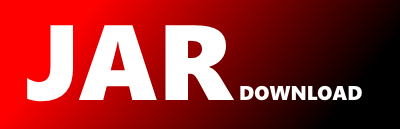
com.amazonaws.services.mediapackagev2.model.GetChannelResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-mediapackagev2 Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.mediapackagev2.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class GetChannelResult extends com.amazonaws.AmazonWebServiceResult implements Serializable, Cloneable {
/**
*
* The Amazon Resource Name (ARN) associated with the resource.
*
*/
private String arn;
/**
*
* The name that describes the channel. The name is the primary identifier for the channel, and must be unique for
* your account in the AWS Region and channel group.
*
*/
private String channelName;
/**
*
* The name that describes the channel group. The name is the primary identifier for the channel group, and must be
* unique for your account in the AWS Region.
*
*/
private String channelGroupName;
/**
*
* The date and time the channel was created.
*
*/
private java.util.Date createdAt;
/**
*
* The date and time the channel was modified.
*
*/
private java.util.Date modifiedAt;
/**
*
* The description for your channel.
*
*/
private String description;
private java.util.List ingestEndpoints;
/**
*
* The input type will be an immutable field which will be used to define whether the channel will allow CMAF ingest
* or HLS ingest. If unprovided, it will default to HLS to preserve current behavior.
*
*
* The allowed values are:
*
*
* -
*
* HLS
- The HLS streaming specification (which defines M3U8 manifests and TS segments).
*
*
* -
*
* CMAF
- The DASH-IF CMAF Ingest specification (which defines CMAF segments with optional DASH
* manifests).
*
*
*
*/
private String inputType;
/**
*
* The current Entity Tag (ETag) associated with this resource. The entity tag can be used to safely make concurrent
* updates to the resource.
*
*/
private String eTag;
/**
*
* The comma-separated list of tag key:value pairs assigned to the channel.
*
*/
private java.util.Map tags;
/**
*
* The Amazon Resource Name (ARN) associated with the resource.
*
*
* @param arn
* The Amazon Resource Name (ARN) associated with the resource.
*/
public void setArn(String arn) {
this.arn = arn;
}
/**
*
* The Amazon Resource Name (ARN) associated with the resource.
*
*
* @return The Amazon Resource Name (ARN) associated with the resource.
*/
public String getArn() {
return this.arn;
}
/**
*
* The Amazon Resource Name (ARN) associated with the resource.
*
*
* @param arn
* The Amazon Resource Name (ARN) associated with the resource.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetChannelResult withArn(String arn) {
setArn(arn);
return this;
}
/**
*
* The name that describes the channel. The name is the primary identifier for the channel, and must be unique for
* your account in the AWS Region and channel group.
*
*
* @param channelName
* The name that describes the channel. The name is the primary identifier for the channel, and must be
* unique for your account in the AWS Region and channel group.
*/
public void setChannelName(String channelName) {
this.channelName = channelName;
}
/**
*
* The name that describes the channel. The name is the primary identifier for the channel, and must be unique for
* your account in the AWS Region and channel group.
*
*
* @return The name that describes the channel. The name is the primary identifier for the channel, and must be
* unique for your account in the AWS Region and channel group.
*/
public String getChannelName() {
return this.channelName;
}
/**
*
* The name that describes the channel. The name is the primary identifier for the channel, and must be unique for
* your account in the AWS Region and channel group.
*
*
* @param channelName
* The name that describes the channel. The name is the primary identifier for the channel, and must be
* unique for your account in the AWS Region and channel group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetChannelResult withChannelName(String channelName) {
setChannelName(channelName);
return this;
}
/**
*
* The name that describes the channel group. The name is the primary identifier for the channel group, and must be
* unique for your account in the AWS Region.
*
*
* @param channelGroupName
* The name that describes the channel group. The name is the primary identifier for the channel group, and
* must be unique for your account in the AWS Region.
*/
public void setChannelGroupName(String channelGroupName) {
this.channelGroupName = channelGroupName;
}
/**
*
* The name that describes the channel group. The name is the primary identifier for the channel group, and must be
* unique for your account in the AWS Region.
*
*
* @return The name that describes the channel group. The name is the primary identifier for the channel group, and
* must be unique for your account in the AWS Region.
*/
public String getChannelGroupName() {
return this.channelGroupName;
}
/**
*
* The name that describes the channel group. The name is the primary identifier for the channel group, and must be
* unique for your account in the AWS Region.
*
*
* @param channelGroupName
* The name that describes the channel group. The name is the primary identifier for the channel group, and
* must be unique for your account in the AWS Region.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetChannelResult withChannelGroupName(String channelGroupName) {
setChannelGroupName(channelGroupName);
return this;
}
/**
*
* The date and time the channel was created.
*
*
* @param createdAt
* The date and time the channel was created.
*/
public void setCreatedAt(java.util.Date createdAt) {
this.createdAt = createdAt;
}
/**
*
* The date and time the channel was created.
*
*
* @return The date and time the channel was created.
*/
public java.util.Date getCreatedAt() {
return this.createdAt;
}
/**
*
* The date and time the channel was created.
*
*
* @param createdAt
* The date and time the channel was created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetChannelResult withCreatedAt(java.util.Date createdAt) {
setCreatedAt(createdAt);
return this;
}
/**
*
* The date and time the channel was modified.
*
*
* @param modifiedAt
* The date and time the channel was modified.
*/
public void setModifiedAt(java.util.Date modifiedAt) {
this.modifiedAt = modifiedAt;
}
/**
*
* The date and time the channel was modified.
*
*
* @return The date and time the channel was modified.
*/
public java.util.Date getModifiedAt() {
return this.modifiedAt;
}
/**
*
* The date and time the channel was modified.
*
*
* @param modifiedAt
* The date and time the channel was modified.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetChannelResult withModifiedAt(java.util.Date modifiedAt) {
setModifiedAt(modifiedAt);
return this;
}
/**
*
* The description for your channel.
*
*
* @param description
* The description for your channel.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* The description for your channel.
*
*
* @return The description for your channel.
*/
public String getDescription() {
return this.description;
}
/**
*
* The description for your channel.
*
*
* @param description
* The description for your channel.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetChannelResult withDescription(String description) {
setDescription(description);
return this;
}
/**
* @return
*/
public java.util.List getIngestEndpoints() {
return ingestEndpoints;
}
/**
* @param ingestEndpoints
*/
public void setIngestEndpoints(java.util.Collection ingestEndpoints) {
if (ingestEndpoints == null) {
this.ingestEndpoints = null;
return;
}
this.ingestEndpoints = new java.util.ArrayList(ingestEndpoints);
}
/**
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setIngestEndpoints(java.util.Collection)} or {@link #withIngestEndpoints(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param ingestEndpoints
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetChannelResult withIngestEndpoints(IngestEndpoint... ingestEndpoints) {
if (this.ingestEndpoints == null) {
setIngestEndpoints(new java.util.ArrayList(ingestEndpoints.length));
}
for (IngestEndpoint ele : ingestEndpoints) {
this.ingestEndpoints.add(ele);
}
return this;
}
/**
* @param ingestEndpoints
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetChannelResult withIngestEndpoints(java.util.Collection ingestEndpoints) {
setIngestEndpoints(ingestEndpoints);
return this;
}
/**
*
* The input type will be an immutable field which will be used to define whether the channel will allow CMAF ingest
* or HLS ingest. If unprovided, it will default to HLS to preserve current behavior.
*
*
* The allowed values are:
*
*
* -
*
* HLS
- The HLS streaming specification (which defines M3U8 manifests and TS segments).
*
*
* -
*
* CMAF
- The DASH-IF CMAF Ingest specification (which defines CMAF segments with optional DASH
* manifests).
*
*
*
*
* @param inputType
* The input type will be an immutable field which will be used to define whether the channel will allow CMAF
* ingest or HLS ingest. If unprovided, it will default to HLS to preserve current behavior.
*
* The allowed values are:
*
*
* -
*
* HLS
- The HLS streaming specification (which defines M3U8 manifests and TS segments).
*
*
* -
*
* CMAF
- The DASH-IF CMAF Ingest specification (which defines CMAF segments with optional DASH
* manifests).
*
*
* @see InputType
*/
public void setInputType(String inputType) {
this.inputType = inputType;
}
/**
*
* The input type will be an immutable field which will be used to define whether the channel will allow CMAF ingest
* or HLS ingest. If unprovided, it will default to HLS to preserve current behavior.
*
*
* The allowed values are:
*
*
* -
*
* HLS
- The HLS streaming specification (which defines M3U8 manifests and TS segments).
*
*
* -
*
* CMAF
- The DASH-IF CMAF Ingest specification (which defines CMAF segments with optional DASH
* manifests).
*
*
*
*
* @return The input type will be an immutable field which will be used to define whether the channel will allow
* CMAF ingest or HLS ingest. If unprovided, it will default to HLS to preserve current behavior.
*
* The allowed values are:
*
*
* -
*
* HLS
- The HLS streaming specification (which defines M3U8 manifests and TS segments).
*
*
* -
*
* CMAF
- The DASH-IF CMAF Ingest specification (which defines CMAF segments with optional DASH
* manifests).
*
*
* @see InputType
*/
public String getInputType() {
return this.inputType;
}
/**
*
* The input type will be an immutable field which will be used to define whether the channel will allow CMAF ingest
* or HLS ingest. If unprovided, it will default to HLS to preserve current behavior.
*
*
* The allowed values are:
*
*
* -
*
* HLS
- The HLS streaming specification (which defines M3U8 manifests and TS segments).
*
*
* -
*
* CMAF
- The DASH-IF CMAF Ingest specification (which defines CMAF segments with optional DASH
* manifests).
*
*
*
*
* @param inputType
* The input type will be an immutable field which will be used to define whether the channel will allow CMAF
* ingest or HLS ingest. If unprovided, it will default to HLS to preserve current behavior.
*
* The allowed values are:
*
*
* -
*
* HLS
- The HLS streaming specification (which defines M3U8 manifests and TS segments).
*
*
* -
*
* CMAF
- The DASH-IF CMAF Ingest specification (which defines CMAF segments with optional DASH
* manifests).
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see InputType
*/
public GetChannelResult withInputType(String inputType) {
setInputType(inputType);
return this;
}
/**
*
* The input type will be an immutable field which will be used to define whether the channel will allow CMAF ingest
* or HLS ingest. If unprovided, it will default to HLS to preserve current behavior.
*
*
* The allowed values are:
*
*
* -
*
* HLS
- The HLS streaming specification (which defines M3U8 manifests and TS segments).
*
*
* -
*
* CMAF
- The DASH-IF CMAF Ingest specification (which defines CMAF segments with optional DASH
* manifests).
*
*
*
*
* @param inputType
* The input type will be an immutable field which will be used to define whether the channel will allow CMAF
* ingest or HLS ingest. If unprovided, it will default to HLS to preserve current behavior.
*
* The allowed values are:
*
*
* -
*
* HLS
- The HLS streaming specification (which defines M3U8 manifests and TS segments).
*
*
* -
*
* CMAF
- The DASH-IF CMAF Ingest specification (which defines CMAF segments with optional DASH
* manifests).
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see InputType
*/
public GetChannelResult withInputType(InputType inputType) {
this.inputType = inputType.toString();
return this;
}
/**
*
* The current Entity Tag (ETag) associated with this resource. The entity tag can be used to safely make concurrent
* updates to the resource.
*
*
* @param eTag
* The current Entity Tag (ETag) associated with this resource. The entity tag can be used to safely make
* concurrent updates to the resource.
*/
public void setETag(String eTag) {
this.eTag = eTag;
}
/**
*
* The current Entity Tag (ETag) associated with this resource. The entity tag can be used to safely make concurrent
* updates to the resource.
*
*
* @return The current Entity Tag (ETag) associated with this resource. The entity tag can be used to safely make
* concurrent updates to the resource.
*/
public String getETag() {
return this.eTag;
}
/**
*
* The current Entity Tag (ETag) associated with this resource. The entity tag can be used to safely make concurrent
* updates to the resource.
*
*
* @param eTag
* The current Entity Tag (ETag) associated with this resource. The entity tag can be used to safely make
* concurrent updates to the resource.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetChannelResult withETag(String eTag) {
setETag(eTag);
return this;
}
/**
*
* The comma-separated list of tag key:value pairs assigned to the channel.
*
*
* @return The comma-separated list of tag key:value pairs assigned to the channel.
*/
public java.util.Map getTags() {
return tags;
}
/**
*
* The comma-separated list of tag key:value pairs assigned to the channel.
*
*
* @param tags
* The comma-separated list of tag key:value pairs assigned to the channel.
*/
public void setTags(java.util.Map tags) {
this.tags = tags;
}
/**
*
* The comma-separated list of tag key:value pairs assigned to the channel.
*
*
* @param tags
* The comma-separated list of tag key:value pairs assigned to the channel.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetChannelResult withTags(java.util.Map tags) {
setTags(tags);
return this;
}
/**
* Add a single Tags entry
*
* @see GetChannelResult#withTags
* @returns a reference to this object so that method calls can be chained together.
*/
public GetChannelResult addTagsEntry(String key, String value) {
if (null == this.tags) {
this.tags = new java.util.HashMap();
}
if (this.tags.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.tags.put(key, value);
return this;
}
/**
* Removes all the entries added into Tags.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetChannelResult clearTagsEntries() {
this.tags = null;
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getArn() != null)
sb.append("Arn: ").append(getArn()).append(",");
if (getChannelName() != null)
sb.append("ChannelName: ").append(getChannelName()).append(",");
if (getChannelGroupName() != null)
sb.append("ChannelGroupName: ").append(getChannelGroupName()).append(",");
if (getCreatedAt() != null)
sb.append("CreatedAt: ").append(getCreatedAt()).append(",");
if (getModifiedAt() != null)
sb.append("ModifiedAt: ").append(getModifiedAt()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getIngestEndpoints() != null)
sb.append("IngestEndpoints: ").append(getIngestEndpoints()).append(",");
if (getInputType() != null)
sb.append("InputType: ").append(getInputType()).append(",");
if (getETag() != null)
sb.append("ETag: ").append(getETag()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof GetChannelResult == false)
return false;
GetChannelResult other = (GetChannelResult) obj;
if (other.getArn() == null ^ this.getArn() == null)
return false;
if (other.getArn() != null && other.getArn().equals(this.getArn()) == false)
return false;
if (other.getChannelName() == null ^ this.getChannelName() == null)
return false;
if (other.getChannelName() != null && other.getChannelName().equals(this.getChannelName()) == false)
return false;
if (other.getChannelGroupName() == null ^ this.getChannelGroupName() == null)
return false;
if (other.getChannelGroupName() != null && other.getChannelGroupName().equals(this.getChannelGroupName()) == false)
return false;
if (other.getCreatedAt() == null ^ this.getCreatedAt() == null)
return false;
if (other.getCreatedAt() != null && other.getCreatedAt().equals(this.getCreatedAt()) == false)
return false;
if (other.getModifiedAt() == null ^ this.getModifiedAt() == null)
return false;
if (other.getModifiedAt() != null && other.getModifiedAt().equals(this.getModifiedAt()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getIngestEndpoints() == null ^ this.getIngestEndpoints() == null)
return false;
if (other.getIngestEndpoints() != null && other.getIngestEndpoints().equals(this.getIngestEndpoints()) == false)
return false;
if (other.getInputType() == null ^ this.getInputType() == null)
return false;
if (other.getInputType() != null && other.getInputType().equals(this.getInputType()) == false)
return false;
if (other.getETag() == null ^ this.getETag() == null)
return false;
if (other.getETag() != null && other.getETag().equals(this.getETag()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getArn() == null) ? 0 : getArn().hashCode());
hashCode = prime * hashCode + ((getChannelName() == null) ? 0 : getChannelName().hashCode());
hashCode = prime * hashCode + ((getChannelGroupName() == null) ? 0 : getChannelGroupName().hashCode());
hashCode = prime * hashCode + ((getCreatedAt() == null) ? 0 : getCreatedAt().hashCode());
hashCode = prime * hashCode + ((getModifiedAt() == null) ? 0 : getModifiedAt().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getIngestEndpoints() == null) ? 0 : getIngestEndpoints().hashCode());
hashCode = prime * hashCode + ((getInputType() == null) ? 0 : getInputType().hashCode());
hashCode = prime * hashCode + ((getETag() == null) ? 0 : getETag().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
return hashCode;
}
@Override
public GetChannelResult clone() {
try {
return (GetChannelResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}