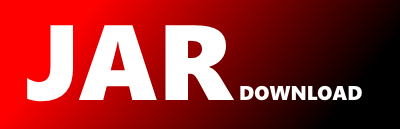
com.amazonaws.services.mediapackagev2.model.CreateDashManifestConfiguration Maven / Gradle / Ivy
Show all versions of aws-java-sdk-mediapackagev2 Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.mediapackagev2.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Create a DASH manifest configuration.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateDashManifestConfiguration implements Serializable, Cloneable, StructuredPojo {
/**
*
* A short string that's appended to the endpoint URL. The child manifest name creates a unique path to this
* endpoint.
*
*/
private String manifestName;
/**
*
* The total duration (in seconds) of the manifest's content.
*
*/
private Integer manifestWindowSeconds;
private FilterConfiguration filterConfiguration;
/**
*
* Minimum amount of time (in seconds) that the player should wait before requesting updates to the manifest.
*
*/
private Integer minUpdatePeriodSeconds;
/**
*
* Minimum amount of content (in seconds) that a player must keep available in the buffer.
*
*/
private Integer minBufferTimeSeconds;
/**
*
* The amount of time (in seconds) that the player should be from the end of the manifest.
*
*/
private Integer suggestedPresentationDelaySeconds;
/**
*
* Determines the type of variable used in the media
URL of the SegmentTemplate
tag in the
* manifest. Also specifies if segment timeline information is included in SegmentTimeline
or
* SegmentTemplate
.
*
*
* Value description:
*
*
* -
*
* NUMBER_WITH_TIMELINE
- The $Number$
variable is used in the media
URL. The
* value of this variable is the sequential number of the segment. A full SegmentTimeline
object is
* presented in each SegmentTemplate
.
*
*
*
*/
private String segmentTemplateFormat;
/**
*
* A list of triggers that controls when AWS Elemental MediaPackage separates the MPEG-DASH manifest into multiple
* periods. Type ADS
to indicate that AWS Elemental MediaPackage must create periods in the output
* manifest that correspond to SCTE-35 ad markers in the input source. Leave this value empty to indicate that the
* manifest is contained all in one period. For more information about periods in the DASH manifest, see Multi-period DASH in AWS
* Elemental MediaPackage.
*
*/
private java.util.List periodTriggers;
/**
*
* The SCTE configuration.
*
*/
private ScteDash scteDash;
/**
*
* Determines how the DASH manifest signals the DRM content.
*
*/
private String drmSignaling;
/**
*
* Determines the type of UTC timing included in the DASH Media Presentation Description (MPD).
*
*/
private DashUtcTiming utcTiming;
/**
*
* A short string that's appended to the endpoint URL. The child manifest name creates a unique path to this
* endpoint.
*
*
* @param manifestName
* A short string that's appended to the endpoint URL. The child manifest name creates a unique path to this
* endpoint.
*/
public void setManifestName(String manifestName) {
this.manifestName = manifestName;
}
/**
*
* A short string that's appended to the endpoint URL. The child manifest name creates a unique path to this
* endpoint.
*
*
* @return A short string that's appended to the endpoint URL. The child manifest name creates a unique path to this
* endpoint.
*/
public String getManifestName() {
return this.manifestName;
}
/**
*
* A short string that's appended to the endpoint URL. The child manifest name creates a unique path to this
* endpoint.
*
*
* @param manifestName
* A short string that's appended to the endpoint URL. The child manifest name creates a unique path to this
* endpoint.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDashManifestConfiguration withManifestName(String manifestName) {
setManifestName(manifestName);
return this;
}
/**
*
* The total duration (in seconds) of the manifest's content.
*
*
* @param manifestWindowSeconds
* The total duration (in seconds) of the manifest's content.
*/
public void setManifestWindowSeconds(Integer manifestWindowSeconds) {
this.manifestWindowSeconds = manifestWindowSeconds;
}
/**
*
* The total duration (in seconds) of the manifest's content.
*
*
* @return The total duration (in seconds) of the manifest's content.
*/
public Integer getManifestWindowSeconds() {
return this.manifestWindowSeconds;
}
/**
*
* The total duration (in seconds) of the manifest's content.
*
*
* @param manifestWindowSeconds
* The total duration (in seconds) of the manifest's content.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDashManifestConfiguration withManifestWindowSeconds(Integer manifestWindowSeconds) {
setManifestWindowSeconds(manifestWindowSeconds);
return this;
}
/**
* @param filterConfiguration
*/
public void setFilterConfiguration(FilterConfiguration filterConfiguration) {
this.filterConfiguration = filterConfiguration;
}
/**
* @return
*/
public FilterConfiguration getFilterConfiguration() {
return this.filterConfiguration;
}
/**
* @param filterConfiguration
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDashManifestConfiguration withFilterConfiguration(FilterConfiguration filterConfiguration) {
setFilterConfiguration(filterConfiguration);
return this;
}
/**
*
* Minimum amount of time (in seconds) that the player should wait before requesting updates to the manifest.
*
*
* @param minUpdatePeriodSeconds
* Minimum amount of time (in seconds) that the player should wait before requesting updates to the manifest.
*/
public void setMinUpdatePeriodSeconds(Integer minUpdatePeriodSeconds) {
this.minUpdatePeriodSeconds = minUpdatePeriodSeconds;
}
/**
*
* Minimum amount of time (in seconds) that the player should wait before requesting updates to the manifest.
*
*
* @return Minimum amount of time (in seconds) that the player should wait before requesting updates to the
* manifest.
*/
public Integer getMinUpdatePeriodSeconds() {
return this.minUpdatePeriodSeconds;
}
/**
*
* Minimum amount of time (in seconds) that the player should wait before requesting updates to the manifest.
*
*
* @param minUpdatePeriodSeconds
* Minimum amount of time (in seconds) that the player should wait before requesting updates to the manifest.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDashManifestConfiguration withMinUpdatePeriodSeconds(Integer minUpdatePeriodSeconds) {
setMinUpdatePeriodSeconds(minUpdatePeriodSeconds);
return this;
}
/**
*
* Minimum amount of content (in seconds) that a player must keep available in the buffer.
*
*
* @param minBufferTimeSeconds
* Minimum amount of content (in seconds) that a player must keep available in the buffer.
*/
public void setMinBufferTimeSeconds(Integer minBufferTimeSeconds) {
this.minBufferTimeSeconds = minBufferTimeSeconds;
}
/**
*
* Minimum amount of content (in seconds) that a player must keep available in the buffer.
*
*
* @return Minimum amount of content (in seconds) that a player must keep available in the buffer.
*/
public Integer getMinBufferTimeSeconds() {
return this.minBufferTimeSeconds;
}
/**
*
* Minimum amount of content (in seconds) that a player must keep available in the buffer.
*
*
* @param minBufferTimeSeconds
* Minimum amount of content (in seconds) that a player must keep available in the buffer.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDashManifestConfiguration withMinBufferTimeSeconds(Integer minBufferTimeSeconds) {
setMinBufferTimeSeconds(minBufferTimeSeconds);
return this;
}
/**
*
* The amount of time (in seconds) that the player should be from the end of the manifest.
*
*
* @param suggestedPresentationDelaySeconds
* The amount of time (in seconds) that the player should be from the end of the manifest.
*/
public void setSuggestedPresentationDelaySeconds(Integer suggestedPresentationDelaySeconds) {
this.suggestedPresentationDelaySeconds = suggestedPresentationDelaySeconds;
}
/**
*
* The amount of time (in seconds) that the player should be from the end of the manifest.
*
*
* @return The amount of time (in seconds) that the player should be from the end of the manifest.
*/
public Integer getSuggestedPresentationDelaySeconds() {
return this.suggestedPresentationDelaySeconds;
}
/**
*
* The amount of time (in seconds) that the player should be from the end of the manifest.
*
*
* @param suggestedPresentationDelaySeconds
* The amount of time (in seconds) that the player should be from the end of the manifest.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDashManifestConfiguration withSuggestedPresentationDelaySeconds(Integer suggestedPresentationDelaySeconds) {
setSuggestedPresentationDelaySeconds(suggestedPresentationDelaySeconds);
return this;
}
/**
*
* Determines the type of variable used in the media
URL of the SegmentTemplate
tag in the
* manifest. Also specifies if segment timeline information is included in SegmentTimeline
or
* SegmentTemplate
.
*
*
* Value description:
*
*
* -
*
* NUMBER_WITH_TIMELINE
- The $Number$
variable is used in the media
URL. The
* value of this variable is the sequential number of the segment. A full SegmentTimeline
object is
* presented in each SegmentTemplate
.
*
*
*
*
* @param segmentTemplateFormat
* Determines the type of variable used in the media
URL of the SegmentTemplate
tag
* in the manifest. Also specifies if segment timeline information is included in
* SegmentTimeline
or SegmentTemplate
.
*
* Value description:
*
*
* -
*
* NUMBER_WITH_TIMELINE
- The $Number$
variable is used in the media
* URL. The value of this variable is the sequential number of the segment. A full
* SegmentTimeline
object is presented in each SegmentTemplate
.
*
*
* @see DashSegmentTemplateFormat
*/
public void setSegmentTemplateFormat(String segmentTemplateFormat) {
this.segmentTemplateFormat = segmentTemplateFormat;
}
/**
*
* Determines the type of variable used in the media
URL of the SegmentTemplate
tag in the
* manifest. Also specifies if segment timeline information is included in SegmentTimeline
or
* SegmentTemplate
.
*
*
* Value description:
*
*
* -
*
* NUMBER_WITH_TIMELINE
- The $Number$
variable is used in the media
URL. The
* value of this variable is the sequential number of the segment. A full SegmentTimeline
object is
* presented in each SegmentTemplate
.
*
*
*
*
* @return Determines the type of variable used in the media
URL of the SegmentTemplate
* tag in the manifest. Also specifies if segment timeline information is included in
* SegmentTimeline
or SegmentTemplate
.
*
* Value description:
*
*
* -
*
* NUMBER_WITH_TIMELINE
- The $Number$
variable is used in the media
* URL. The value of this variable is the sequential number of the segment. A full
* SegmentTimeline
object is presented in each SegmentTemplate
.
*
*
* @see DashSegmentTemplateFormat
*/
public String getSegmentTemplateFormat() {
return this.segmentTemplateFormat;
}
/**
*
* Determines the type of variable used in the media
URL of the SegmentTemplate
tag in the
* manifest. Also specifies if segment timeline information is included in SegmentTimeline
or
* SegmentTemplate
.
*
*
* Value description:
*
*
* -
*
* NUMBER_WITH_TIMELINE
- The $Number$
variable is used in the media
URL. The
* value of this variable is the sequential number of the segment. A full SegmentTimeline
object is
* presented in each SegmentTemplate
.
*
*
*
*
* @param segmentTemplateFormat
* Determines the type of variable used in the media
URL of the SegmentTemplate
tag
* in the manifest. Also specifies if segment timeline information is included in
* SegmentTimeline
or SegmentTemplate
.
*
* Value description:
*
*
* -
*
* NUMBER_WITH_TIMELINE
- The $Number$
variable is used in the media
* URL. The value of this variable is the sequential number of the segment. A full
* SegmentTimeline
object is presented in each SegmentTemplate
.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see DashSegmentTemplateFormat
*/
public CreateDashManifestConfiguration withSegmentTemplateFormat(String segmentTemplateFormat) {
setSegmentTemplateFormat(segmentTemplateFormat);
return this;
}
/**
*
* Determines the type of variable used in the media
URL of the SegmentTemplate
tag in the
* manifest. Also specifies if segment timeline information is included in SegmentTimeline
or
* SegmentTemplate
.
*
*
* Value description:
*
*
* -
*
* NUMBER_WITH_TIMELINE
- The $Number$
variable is used in the media
URL. The
* value of this variable is the sequential number of the segment. A full SegmentTimeline
object is
* presented in each SegmentTemplate
.
*
*
*
*
* @param segmentTemplateFormat
* Determines the type of variable used in the media
URL of the SegmentTemplate
tag
* in the manifest. Also specifies if segment timeline information is included in
* SegmentTimeline
or SegmentTemplate
.
*
* Value description:
*
*
* -
*
* NUMBER_WITH_TIMELINE
- The $Number$
variable is used in the media
* URL. The value of this variable is the sequential number of the segment. A full
* SegmentTimeline
object is presented in each SegmentTemplate
.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see DashSegmentTemplateFormat
*/
public CreateDashManifestConfiguration withSegmentTemplateFormat(DashSegmentTemplateFormat segmentTemplateFormat) {
this.segmentTemplateFormat = segmentTemplateFormat.toString();
return this;
}
/**
*
* A list of triggers that controls when AWS Elemental MediaPackage separates the MPEG-DASH manifest into multiple
* periods. Type ADS
to indicate that AWS Elemental MediaPackage must create periods in the output
* manifest that correspond to SCTE-35 ad markers in the input source. Leave this value empty to indicate that the
* manifest is contained all in one period. For more information about periods in the DASH manifest, see Multi-period DASH in AWS
* Elemental MediaPackage.
*
*
* @return A list of triggers that controls when AWS Elemental MediaPackage separates the MPEG-DASH manifest into
* multiple periods. Type ADS
to indicate that AWS Elemental MediaPackage must create periods
* in the output manifest that correspond to SCTE-35 ad markers in the input source. Leave this value empty
* to indicate that the manifest is contained all in one period. For more information about periods in the
* DASH manifest, see Multi-period DASH in
* AWS Elemental MediaPackage.
* @see DashPeriodTrigger
*/
public java.util.List getPeriodTriggers() {
return periodTriggers;
}
/**
*
* A list of triggers that controls when AWS Elemental MediaPackage separates the MPEG-DASH manifest into multiple
* periods. Type ADS
to indicate that AWS Elemental MediaPackage must create periods in the output
* manifest that correspond to SCTE-35 ad markers in the input source. Leave this value empty to indicate that the
* manifest is contained all in one period. For more information about periods in the DASH manifest, see Multi-period DASH in AWS
* Elemental MediaPackage.
*
*
* @param periodTriggers
* A list of triggers that controls when AWS Elemental MediaPackage separates the MPEG-DASH manifest into
* multiple periods. Type ADS
to indicate that AWS Elemental MediaPackage must create periods in
* the output manifest that correspond to SCTE-35 ad markers in the input source. Leave this value empty to
* indicate that the manifest is contained all in one period. For more information about periods in the DASH
* manifest, see Multi-period DASH in
* AWS Elemental MediaPackage.
* @see DashPeriodTrigger
*/
public void setPeriodTriggers(java.util.Collection periodTriggers) {
if (periodTriggers == null) {
this.periodTriggers = null;
return;
}
this.periodTriggers = new java.util.ArrayList(periodTriggers);
}
/**
*
* A list of triggers that controls when AWS Elemental MediaPackage separates the MPEG-DASH manifest into multiple
* periods. Type ADS
to indicate that AWS Elemental MediaPackage must create periods in the output
* manifest that correspond to SCTE-35 ad markers in the input source. Leave this value empty to indicate that the
* manifest is contained all in one period. For more information about periods in the DASH manifest, see Multi-period DASH in AWS
* Elemental MediaPackage.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setPeriodTriggers(java.util.Collection)} or {@link #withPeriodTriggers(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param periodTriggers
* A list of triggers that controls when AWS Elemental MediaPackage separates the MPEG-DASH manifest into
* multiple periods. Type ADS
to indicate that AWS Elemental MediaPackage must create periods in
* the output manifest that correspond to SCTE-35 ad markers in the input source. Leave this value empty to
* indicate that the manifest is contained all in one period. For more information about periods in the DASH
* manifest, see Multi-period DASH in
* AWS Elemental MediaPackage.
* @return Returns a reference to this object so that method calls can be chained together.
* @see DashPeriodTrigger
*/
public CreateDashManifestConfiguration withPeriodTriggers(String... periodTriggers) {
if (this.periodTriggers == null) {
setPeriodTriggers(new java.util.ArrayList(periodTriggers.length));
}
for (String ele : periodTriggers) {
this.periodTriggers.add(ele);
}
return this;
}
/**
*
* A list of triggers that controls when AWS Elemental MediaPackage separates the MPEG-DASH manifest into multiple
* periods. Type ADS
to indicate that AWS Elemental MediaPackage must create periods in the output
* manifest that correspond to SCTE-35 ad markers in the input source. Leave this value empty to indicate that the
* manifest is contained all in one period. For more information about periods in the DASH manifest, see Multi-period DASH in AWS
* Elemental MediaPackage.
*
*
* @param periodTriggers
* A list of triggers that controls when AWS Elemental MediaPackage separates the MPEG-DASH manifest into
* multiple periods. Type ADS
to indicate that AWS Elemental MediaPackage must create periods in
* the output manifest that correspond to SCTE-35 ad markers in the input source. Leave this value empty to
* indicate that the manifest is contained all in one period. For more information about periods in the DASH
* manifest, see Multi-period DASH in
* AWS Elemental MediaPackage.
* @return Returns a reference to this object so that method calls can be chained together.
* @see DashPeriodTrigger
*/
public CreateDashManifestConfiguration withPeriodTriggers(java.util.Collection periodTriggers) {
setPeriodTriggers(periodTriggers);
return this;
}
/**
*
* A list of triggers that controls when AWS Elemental MediaPackage separates the MPEG-DASH manifest into multiple
* periods. Type ADS
to indicate that AWS Elemental MediaPackage must create periods in the output
* manifest that correspond to SCTE-35 ad markers in the input source. Leave this value empty to indicate that the
* manifest is contained all in one period. For more information about periods in the DASH manifest, see Multi-period DASH in AWS
* Elemental MediaPackage.
*
*
* @param periodTriggers
* A list of triggers that controls when AWS Elemental MediaPackage separates the MPEG-DASH manifest into
* multiple periods. Type ADS
to indicate that AWS Elemental MediaPackage must create periods in
* the output manifest that correspond to SCTE-35 ad markers in the input source. Leave this value empty to
* indicate that the manifest is contained all in one period. For more information about periods in the DASH
* manifest, see Multi-period DASH in
* AWS Elemental MediaPackage.
* @return Returns a reference to this object so that method calls can be chained together.
* @see DashPeriodTrigger
*/
public CreateDashManifestConfiguration withPeriodTriggers(DashPeriodTrigger... periodTriggers) {
java.util.ArrayList periodTriggersCopy = new java.util.ArrayList(periodTriggers.length);
for (DashPeriodTrigger value : periodTriggers) {
periodTriggersCopy.add(value.toString());
}
if (getPeriodTriggers() == null) {
setPeriodTriggers(periodTriggersCopy);
} else {
getPeriodTriggers().addAll(periodTriggersCopy);
}
return this;
}
/**
*
* The SCTE configuration.
*
*
* @param scteDash
* The SCTE configuration.
*/
public void setScteDash(ScteDash scteDash) {
this.scteDash = scteDash;
}
/**
*
* The SCTE configuration.
*
*
* @return The SCTE configuration.
*/
public ScteDash getScteDash() {
return this.scteDash;
}
/**
*
* The SCTE configuration.
*
*
* @param scteDash
* The SCTE configuration.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDashManifestConfiguration withScteDash(ScteDash scteDash) {
setScteDash(scteDash);
return this;
}
/**
*
* Determines how the DASH manifest signals the DRM content.
*
*
* @param drmSignaling
* Determines how the DASH manifest signals the DRM content.
* @see DashDrmSignaling
*/
public void setDrmSignaling(String drmSignaling) {
this.drmSignaling = drmSignaling;
}
/**
*
* Determines how the DASH manifest signals the DRM content.
*
*
* @return Determines how the DASH manifest signals the DRM content.
* @see DashDrmSignaling
*/
public String getDrmSignaling() {
return this.drmSignaling;
}
/**
*
* Determines how the DASH manifest signals the DRM content.
*
*
* @param drmSignaling
* Determines how the DASH manifest signals the DRM content.
* @return Returns a reference to this object so that method calls can be chained together.
* @see DashDrmSignaling
*/
public CreateDashManifestConfiguration withDrmSignaling(String drmSignaling) {
setDrmSignaling(drmSignaling);
return this;
}
/**
*
* Determines how the DASH manifest signals the DRM content.
*
*
* @param drmSignaling
* Determines how the DASH manifest signals the DRM content.
* @return Returns a reference to this object so that method calls can be chained together.
* @see DashDrmSignaling
*/
public CreateDashManifestConfiguration withDrmSignaling(DashDrmSignaling drmSignaling) {
this.drmSignaling = drmSignaling.toString();
return this;
}
/**
*
* Determines the type of UTC timing included in the DASH Media Presentation Description (MPD).
*
*
* @param utcTiming
* Determines the type of UTC timing included in the DASH Media Presentation Description (MPD).
*/
public void setUtcTiming(DashUtcTiming utcTiming) {
this.utcTiming = utcTiming;
}
/**
*
* Determines the type of UTC timing included in the DASH Media Presentation Description (MPD).
*
*
* @return Determines the type of UTC timing included in the DASH Media Presentation Description (MPD).
*/
public DashUtcTiming getUtcTiming() {
return this.utcTiming;
}
/**
*
* Determines the type of UTC timing included in the DASH Media Presentation Description (MPD).
*
*
* @param utcTiming
* Determines the type of UTC timing included in the DASH Media Presentation Description (MPD).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDashManifestConfiguration withUtcTiming(DashUtcTiming utcTiming) {
setUtcTiming(utcTiming);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getManifestName() != null)
sb.append("ManifestName: ").append(getManifestName()).append(",");
if (getManifestWindowSeconds() != null)
sb.append("ManifestWindowSeconds: ").append(getManifestWindowSeconds()).append(",");
if (getFilterConfiguration() != null)
sb.append("FilterConfiguration: ").append(getFilterConfiguration()).append(",");
if (getMinUpdatePeriodSeconds() != null)
sb.append("MinUpdatePeriodSeconds: ").append(getMinUpdatePeriodSeconds()).append(",");
if (getMinBufferTimeSeconds() != null)
sb.append("MinBufferTimeSeconds: ").append(getMinBufferTimeSeconds()).append(",");
if (getSuggestedPresentationDelaySeconds() != null)
sb.append("SuggestedPresentationDelaySeconds: ").append(getSuggestedPresentationDelaySeconds()).append(",");
if (getSegmentTemplateFormat() != null)
sb.append("SegmentTemplateFormat: ").append(getSegmentTemplateFormat()).append(",");
if (getPeriodTriggers() != null)
sb.append("PeriodTriggers: ").append(getPeriodTriggers()).append(",");
if (getScteDash() != null)
sb.append("ScteDash: ").append(getScteDash()).append(",");
if (getDrmSignaling() != null)
sb.append("DrmSignaling: ").append(getDrmSignaling()).append(",");
if (getUtcTiming() != null)
sb.append("UtcTiming: ").append(getUtcTiming());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateDashManifestConfiguration == false)
return false;
CreateDashManifestConfiguration other = (CreateDashManifestConfiguration) obj;
if (other.getManifestName() == null ^ this.getManifestName() == null)
return false;
if (other.getManifestName() != null && other.getManifestName().equals(this.getManifestName()) == false)
return false;
if (other.getManifestWindowSeconds() == null ^ this.getManifestWindowSeconds() == null)
return false;
if (other.getManifestWindowSeconds() != null && other.getManifestWindowSeconds().equals(this.getManifestWindowSeconds()) == false)
return false;
if (other.getFilterConfiguration() == null ^ this.getFilterConfiguration() == null)
return false;
if (other.getFilterConfiguration() != null && other.getFilterConfiguration().equals(this.getFilterConfiguration()) == false)
return false;
if (other.getMinUpdatePeriodSeconds() == null ^ this.getMinUpdatePeriodSeconds() == null)
return false;
if (other.getMinUpdatePeriodSeconds() != null && other.getMinUpdatePeriodSeconds().equals(this.getMinUpdatePeriodSeconds()) == false)
return false;
if (other.getMinBufferTimeSeconds() == null ^ this.getMinBufferTimeSeconds() == null)
return false;
if (other.getMinBufferTimeSeconds() != null && other.getMinBufferTimeSeconds().equals(this.getMinBufferTimeSeconds()) == false)
return false;
if (other.getSuggestedPresentationDelaySeconds() == null ^ this.getSuggestedPresentationDelaySeconds() == null)
return false;
if (other.getSuggestedPresentationDelaySeconds() != null
&& other.getSuggestedPresentationDelaySeconds().equals(this.getSuggestedPresentationDelaySeconds()) == false)
return false;
if (other.getSegmentTemplateFormat() == null ^ this.getSegmentTemplateFormat() == null)
return false;
if (other.getSegmentTemplateFormat() != null && other.getSegmentTemplateFormat().equals(this.getSegmentTemplateFormat()) == false)
return false;
if (other.getPeriodTriggers() == null ^ this.getPeriodTriggers() == null)
return false;
if (other.getPeriodTriggers() != null && other.getPeriodTriggers().equals(this.getPeriodTriggers()) == false)
return false;
if (other.getScteDash() == null ^ this.getScteDash() == null)
return false;
if (other.getScteDash() != null && other.getScteDash().equals(this.getScteDash()) == false)
return false;
if (other.getDrmSignaling() == null ^ this.getDrmSignaling() == null)
return false;
if (other.getDrmSignaling() != null && other.getDrmSignaling().equals(this.getDrmSignaling()) == false)
return false;
if (other.getUtcTiming() == null ^ this.getUtcTiming() == null)
return false;
if (other.getUtcTiming() != null && other.getUtcTiming().equals(this.getUtcTiming()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getManifestName() == null) ? 0 : getManifestName().hashCode());
hashCode = prime * hashCode + ((getManifestWindowSeconds() == null) ? 0 : getManifestWindowSeconds().hashCode());
hashCode = prime * hashCode + ((getFilterConfiguration() == null) ? 0 : getFilterConfiguration().hashCode());
hashCode = prime * hashCode + ((getMinUpdatePeriodSeconds() == null) ? 0 : getMinUpdatePeriodSeconds().hashCode());
hashCode = prime * hashCode + ((getMinBufferTimeSeconds() == null) ? 0 : getMinBufferTimeSeconds().hashCode());
hashCode = prime * hashCode + ((getSuggestedPresentationDelaySeconds() == null) ? 0 : getSuggestedPresentationDelaySeconds().hashCode());
hashCode = prime * hashCode + ((getSegmentTemplateFormat() == null) ? 0 : getSegmentTemplateFormat().hashCode());
hashCode = prime * hashCode + ((getPeriodTriggers() == null) ? 0 : getPeriodTriggers().hashCode());
hashCode = prime * hashCode + ((getScteDash() == null) ? 0 : getScteDash().hashCode());
hashCode = prime * hashCode + ((getDrmSignaling() == null) ? 0 : getDrmSignaling().hashCode());
hashCode = prime * hashCode + ((getUtcTiming() == null) ? 0 : getUtcTiming().hashCode());
return hashCode;
}
@Override
public CreateDashManifestConfiguration clone() {
try {
return (CreateDashManifestConfiguration) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.mediapackagev2.model.transform.CreateDashManifestConfigurationMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}