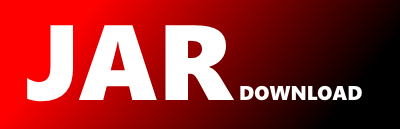
com.amazonaws.services.mediapackagevod.AWSMediaPackageVodAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-mediapackagevod Show documentation
/*
* Copyright 2015-2020 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.mediapackagevod;
import javax.annotation.Generated;
import com.amazonaws.services.mediapackagevod.model.*;
/**
* Interface for accessing MediaPackage Vod asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.mediapackagevod.AbstractAWSMediaPackageVodAsync} instead.
*
*
* AWS Elemental MediaPackage VOD
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSMediaPackageVodAsync extends AWSMediaPackageVod {
/**
* Creates a new MediaPackage VOD Asset resource.
*
* @param createAssetRequest
* A new MediaPackage VOD Asset configuration.
* @return A Java Future containing the result of the CreateAsset operation returned by the service.
* @sample AWSMediaPackageVodAsync.CreateAsset
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createAssetAsync(CreateAssetRequest createAssetRequest);
/**
* Creates a new MediaPackage VOD Asset resource.
*
* @param createAssetRequest
* A new MediaPackage VOD Asset configuration.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateAsset operation returned by the service.
* @sample AWSMediaPackageVodAsyncHandler.CreateAsset
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createAssetAsync(CreateAssetRequest createAssetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Creates a new MediaPackage VOD PackagingConfiguration resource.
*
* @param createPackagingConfigurationRequest
* A new MediaPackage VOD PackagingConfiguration resource configuration.
* @return A Java Future containing the result of the CreatePackagingConfiguration operation returned by the
* service.
* @sample AWSMediaPackageVodAsync.CreatePackagingConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future createPackagingConfigurationAsync(
CreatePackagingConfigurationRequest createPackagingConfigurationRequest);
/**
* Creates a new MediaPackage VOD PackagingConfiguration resource.
*
* @param createPackagingConfigurationRequest
* A new MediaPackage VOD PackagingConfiguration resource configuration.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreatePackagingConfiguration operation returned by the
* service.
* @sample AWSMediaPackageVodAsyncHandler.CreatePackagingConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future createPackagingConfigurationAsync(
CreatePackagingConfigurationRequest createPackagingConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Creates a new MediaPackage VOD PackagingGroup resource.
*
* @param createPackagingGroupRequest
* A new MediaPackage VOD PackagingGroup resource configuration.
* @return A Java Future containing the result of the CreatePackagingGroup operation returned by the service.
* @sample AWSMediaPackageVodAsync.CreatePackagingGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future createPackagingGroupAsync(CreatePackagingGroupRequest createPackagingGroupRequest);
/**
* Creates a new MediaPackage VOD PackagingGroup resource.
*
* @param createPackagingGroupRequest
* A new MediaPackage VOD PackagingGroup resource configuration.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreatePackagingGroup operation returned by the service.
* @sample AWSMediaPackageVodAsyncHandler.CreatePackagingGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future createPackagingGroupAsync(CreatePackagingGroupRequest createPackagingGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Deletes an existing MediaPackage VOD Asset resource.
*
* @param deleteAssetRequest
* @return A Java Future containing the result of the DeleteAsset operation returned by the service.
* @sample AWSMediaPackageVodAsync.DeleteAsset
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteAssetAsync(DeleteAssetRequest deleteAssetRequest);
/**
* Deletes an existing MediaPackage VOD Asset resource.
*
* @param deleteAssetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteAsset operation returned by the service.
* @sample AWSMediaPackageVodAsyncHandler.DeleteAsset
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteAssetAsync(DeleteAssetRequest deleteAssetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Deletes a MediaPackage VOD PackagingConfiguration resource.
*
* @param deletePackagingConfigurationRequest
* @return A Java Future containing the result of the DeletePackagingConfiguration operation returned by the
* service.
* @sample AWSMediaPackageVodAsync.DeletePackagingConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future deletePackagingConfigurationAsync(
DeletePackagingConfigurationRequest deletePackagingConfigurationRequest);
/**
* Deletes a MediaPackage VOD PackagingConfiguration resource.
*
* @param deletePackagingConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeletePackagingConfiguration operation returned by the
* service.
* @sample AWSMediaPackageVodAsyncHandler.DeletePackagingConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future deletePackagingConfigurationAsync(
DeletePackagingConfigurationRequest deletePackagingConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Deletes a MediaPackage VOD PackagingGroup resource.
*
* @param deletePackagingGroupRequest
* @return A Java Future containing the result of the DeletePackagingGroup operation returned by the service.
* @sample AWSMediaPackageVodAsync.DeletePackagingGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future deletePackagingGroupAsync(DeletePackagingGroupRequest deletePackagingGroupRequest);
/**
* Deletes a MediaPackage VOD PackagingGroup resource.
*
* @param deletePackagingGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeletePackagingGroup operation returned by the service.
* @sample AWSMediaPackageVodAsyncHandler.DeletePackagingGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future deletePackagingGroupAsync(DeletePackagingGroupRequest deletePackagingGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Returns a description of a MediaPackage VOD Asset resource.
*
* @param describeAssetRequest
* @return A Java Future containing the result of the DescribeAsset operation returned by the service.
* @sample AWSMediaPackageVodAsync.DescribeAsset
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeAssetAsync(DescribeAssetRequest describeAssetRequest);
/**
* Returns a description of a MediaPackage VOD Asset resource.
*
* @param describeAssetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeAsset operation returned by the service.
* @sample AWSMediaPackageVodAsyncHandler.DescribeAsset
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeAssetAsync(DescribeAssetRequest describeAssetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Returns a description of a MediaPackage VOD PackagingConfiguration resource.
*
* @param describePackagingConfigurationRequest
* @return A Java Future containing the result of the DescribePackagingConfiguration operation returned by the
* service.
* @sample AWSMediaPackageVodAsync.DescribePackagingConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future describePackagingConfigurationAsync(
DescribePackagingConfigurationRequest describePackagingConfigurationRequest);
/**
* Returns a description of a MediaPackage VOD PackagingConfiguration resource.
*
* @param describePackagingConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribePackagingConfiguration operation returned by the
* service.
* @sample AWSMediaPackageVodAsyncHandler.DescribePackagingConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future describePackagingConfigurationAsync(
DescribePackagingConfigurationRequest describePackagingConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Returns a description of a MediaPackage VOD PackagingGroup resource.
*
* @param describePackagingGroupRequest
* @return A Java Future containing the result of the DescribePackagingGroup operation returned by the service.
* @sample AWSMediaPackageVodAsync.DescribePackagingGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future describePackagingGroupAsync(DescribePackagingGroupRequest describePackagingGroupRequest);
/**
* Returns a description of a MediaPackage VOD PackagingGroup resource.
*
* @param describePackagingGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribePackagingGroup operation returned by the service.
* @sample AWSMediaPackageVodAsyncHandler.DescribePackagingGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future describePackagingGroupAsync(DescribePackagingGroupRequest describePackagingGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Returns a collection of MediaPackage VOD Asset resources.
*
* @param listAssetsRequest
* @return A Java Future containing the result of the ListAssets operation returned by the service.
* @sample AWSMediaPackageVodAsync.ListAssets
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listAssetsAsync(ListAssetsRequest listAssetsRequest);
/**
* Returns a collection of MediaPackage VOD Asset resources.
*
* @param listAssetsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListAssets operation returned by the service.
* @sample AWSMediaPackageVodAsyncHandler.ListAssets
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listAssetsAsync(ListAssetsRequest listAssetsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Returns a collection of MediaPackage VOD PackagingConfiguration resources.
*
* @param listPackagingConfigurationsRequest
* @return A Java Future containing the result of the ListPackagingConfigurations operation returned by the service.
* @sample AWSMediaPackageVodAsync.ListPackagingConfigurations
* @see AWS API Documentation
*/
java.util.concurrent.Future listPackagingConfigurationsAsync(
ListPackagingConfigurationsRequest listPackagingConfigurationsRequest);
/**
* Returns a collection of MediaPackage VOD PackagingConfiguration resources.
*
* @param listPackagingConfigurationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListPackagingConfigurations operation returned by the service.
* @sample AWSMediaPackageVodAsyncHandler.ListPackagingConfigurations
* @see AWS API Documentation
*/
java.util.concurrent.Future listPackagingConfigurationsAsync(
ListPackagingConfigurationsRequest listPackagingConfigurationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Returns a collection of MediaPackage VOD PackagingGroup resources.
*
* @param listPackagingGroupsRequest
* @return A Java Future containing the result of the ListPackagingGroups operation returned by the service.
* @sample AWSMediaPackageVodAsync.ListPackagingGroups
* @see AWS API Documentation
*/
java.util.concurrent.Future listPackagingGroupsAsync(ListPackagingGroupsRequest listPackagingGroupsRequest);
/**
* Returns a collection of MediaPackage VOD PackagingGroup resources.
*
* @param listPackagingGroupsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListPackagingGroups operation returned by the service.
* @sample AWSMediaPackageVodAsyncHandler.ListPackagingGroups
* @see AWS API Documentation
*/
java.util.concurrent.Future listPackagingGroupsAsync(ListPackagingGroupsRequest listPackagingGroupsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Returns a list of the tags assigned to the specified resource.
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSMediaPackageVodAsync.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
* Returns a list of the tags assigned to the specified resource.
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSMediaPackageVodAsyncHandler.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Adds tags to the specified resource. You can specify one or more tags to add.
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSMediaPackageVodAsync.TagResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
* Adds tags to the specified resource. You can specify one or more tags to add.
*
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSMediaPackageVodAsyncHandler.TagResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Removes tags from the specified resource. You can specify one or more tags to remove.
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSMediaPackageVodAsync.UntagResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
* Removes tags from the specified resource. You can specify one or more tags to remove.
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSMediaPackageVodAsyncHandler.UntagResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Updates a specific packaging group. You can't change the id attribute or any other system-generated attributes.
*
* @param updatePackagingGroupRequest
* A MediaPackage VOD PackagingGroup resource configuration.
* @return A Java Future containing the result of the UpdatePackagingGroup operation returned by the service.
* @sample AWSMediaPackageVodAsync.UpdatePackagingGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future updatePackagingGroupAsync(UpdatePackagingGroupRequest updatePackagingGroupRequest);
/**
* Updates a specific packaging group. You can't change the id attribute or any other system-generated attributes.
*
* @param updatePackagingGroupRequest
* A MediaPackage VOD PackagingGroup resource configuration.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdatePackagingGroup operation returned by the service.
* @sample AWSMediaPackageVodAsyncHandler.UpdatePackagingGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future updatePackagingGroupAsync(UpdatePackagingGroupRequest updatePackagingGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}