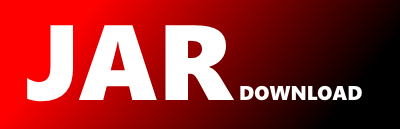
com.amazonaws.services.mediastore.AWSMediaStoreAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-mediastore Show documentation
/*
* Copyright 2013-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.mediastore;
import javax.annotation.Generated;
import com.amazonaws.services.mediastore.model.*;
/**
* Interface for accessing MediaStore asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.mediastore.AbstractAWSMediaStoreAsync} instead.
*
*
*
* An AWS Elemental MediaStore container is a namespace that holds folders and objects. You use a container endpoint to
* create, read, and delete objects.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSMediaStoreAsync extends AWSMediaStore {
/**
*
* Creates a storage container to hold objects. A container is similar to a bucket in the Amazon S3 service.
*
*
* @param createContainerRequest
* @return A Java Future containing the result of the CreateContainer operation returned by the service.
* @sample AWSMediaStoreAsync.CreateContainer
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createContainerAsync(CreateContainerRequest createContainerRequest);
/**
*
* Creates a storage container to hold objects. A container is similar to a bucket in the Amazon S3 service.
*
*
* @param createContainerRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateContainer operation returned by the service.
* @sample AWSMediaStoreAsyncHandler.CreateContainer
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createContainerAsync(CreateContainerRequest createContainerRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified container. Before you make a DeleteContainer
request, delete any objects in
* the container or in any folders in the container. You can delete only empty containers.
*
*
* @param deleteContainerRequest
* @return A Java Future containing the result of the DeleteContainer operation returned by the service.
* @sample AWSMediaStoreAsync.DeleteContainer
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteContainerAsync(DeleteContainerRequest deleteContainerRequest);
/**
*
* Deletes the specified container. Before you make a DeleteContainer
request, delete any objects in
* the container or in any folders in the container. You can delete only empty containers.
*
*
* @param deleteContainerRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteContainer operation returned by the service.
* @sample AWSMediaStoreAsyncHandler.DeleteContainer
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteContainerAsync(DeleteContainerRequest deleteContainerRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the access policy that is associated with the specified container.
*
*
* @param deleteContainerPolicyRequest
* @return A Java Future containing the result of the DeleteContainerPolicy operation returned by the service.
* @sample AWSMediaStoreAsync.DeleteContainerPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteContainerPolicyAsync(DeleteContainerPolicyRequest deleteContainerPolicyRequest);
/**
*
* Deletes the access policy that is associated with the specified container.
*
*
* @param deleteContainerPolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteContainerPolicy operation returned by the service.
* @sample AWSMediaStoreAsyncHandler.DeleteContainerPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteContainerPolicyAsync(DeleteContainerPolicyRequest deleteContainerPolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the properties of the requested container. This returns a single Container
object based on
* ContainerName
. To return all Container
objects that are associated with a specified AWS
* account, use ListContainers.
*
*
* @param describeContainerRequest
* @return A Java Future containing the result of the DescribeContainer operation returned by the service.
* @sample AWSMediaStoreAsync.DescribeContainer
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeContainerAsync(DescribeContainerRequest describeContainerRequest);
/**
*
* Retrieves the properties of the requested container. This returns a single Container
object based on
* ContainerName
. To return all Container
objects that are associated with a specified AWS
* account, use ListContainers.
*
*
* @param describeContainerRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeContainer operation returned by the service.
* @sample AWSMediaStoreAsyncHandler.DescribeContainer
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeContainerAsync(DescribeContainerRequest describeContainerRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the access policy for the specified container. For information about the data that is included in an
* access policy, see the AWS Identity and Access Management
* User Guide.
*
*
* @param getContainerPolicyRequest
* @return A Java Future containing the result of the GetContainerPolicy operation returned by the service.
* @sample AWSMediaStoreAsync.GetContainerPolicy
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getContainerPolicyAsync(GetContainerPolicyRequest getContainerPolicyRequest);
/**
*
* Retrieves the access policy for the specified container. For information about the data that is included in an
* access policy, see the AWS Identity and Access Management
* User Guide.
*
*
* @param getContainerPolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetContainerPolicy operation returned by the service.
* @sample AWSMediaStoreAsyncHandler.GetContainerPolicy
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getContainerPolicyAsync(GetContainerPolicyRequest getContainerPolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the properties of all containers in AWS Elemental MediaStore.
*
*
* You can query to receive all the containers in one response. Or you can include the MaxResults
* parameter to receive a limited number of containers in each response. In this case, the response includes a
* token. To get the next set of containers, send the command again, this time with the NextToken
* parameter (with the returned token as its value). The next set of responses appears, with a token if there are
* still more containers to receive.
*
*
* See also DescribeContainer, which gets the properties of one container.
*
*
* @param listContainersRequest
* @return A Java Future containing the result of the ListContainers operation returned by the service.
* @sample AWSMediaStoreAsync.ListContainers
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listContainersAsync(ListContainersRequest listContainersRequest);
/**
*
* Lists the properties of all containers in AWS Elemental MediaStore.
*
*
* You can query to receive all the containers in one response. Or you can include the MaxResults
* parameter to receive a limited number of containers in each response. In this case, the response includes a
* token. To get the next set of containers, send the command again, this time with the NextToken
* parameter (with the returned token as its value). The next set of responses appears, with a token if there are
* still more containers to receive.
*
*
* See also DescribeContainer, which gets the properties of one container.
*
*
* @param listContainersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListContainers operation returned by the service.
* @sample AWSMediaStoreAsyncHandler.ListContainers
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listContainersAsync(ListContainersRequest listContainersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an access policy for the specified container to restrict the users and clients that can access it. For
* information about the data that is included in an access policy, see the AWS Identity and Access Management User Guide.
*
*
* For this release of the REST API, you can create only one policy for a container. If you enter
* PutContainerPolicy
twice, the second command modifies the existing policy.
*
*
* @param putContainerPolicyRequest
* @return A Java Future containing the result of the PutContainerPolicy operation returned by the service.
* @sample AWSMediaStoreAsync.PutContainerPolicy
* @see AWS
* API Documentation
*/
java.util.concurrent.Future putContainerPolicyAsync(PutContainerPolicyRequest putContainerPolicyRequest);
/**
*
* Creates an access policy for the specified container to restrict the users and clients that can access it. For
* information about the data that is included in an access policy, see the AWS Identity and Access Management User Guide.
*
*
* For this release of the REST API, you can create only one policy for a container. If you enter
* PutContainerPolicy
twice, the second command modifies the existing policy.
*
*
* @param putContainerPolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutContainerPolicy operation returned by the service.
* @sample AWSMediaStoreAsyncHandler.PutContainerPolicy
* @see AWS
* API Documentation
*/
java.util.concurrent.Future putContainerPolicyAsync(PutContainerPolicyRequest putContainerPolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}