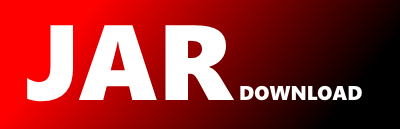
com.amazonaws.services.mediastore.AWSMediaStore Maven / Gradle / Ivy
Show all versions of aws-java-sdk-mediastore Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.mediastore;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.mediastore.model.*;
/**
* Interface for accessing MediaStore.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.mediastore.AbstractAWSMediaStore} instead.
*
*
*
* An AWS Elemental MediaStore container is a namespace that holds folders and objects. You use a container endpoint to
* create, read, and delete objects.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSMediaStore {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "mediastore";
/**
*
* Creates a storage container to hold objects. A container is similar to a bucket in the Amazon S3 service.
*
*
* @param createContainerRequest
* @return Result of the CreateContainer operation returned by the service.
* @throws ContainerInUseException
* The container that you specified in the request already exists or is being updated.
* @throws LimitExceededException
* A service limit has been exceeded.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @sample AWSMediaStore.CreateContainer
* @see AWS API
* Documentation
*/
CreateContainerResult createContainer(CreateContainerRequest createContainerRequest);
/**
*
* Deletes the specified container. Before you make a DeleteContainer
request, delete any objects in
* the container or in any folders in the container. You can delete only empty containers.
*
*
* @param deleteContainerRequest
* @return Result of the DeleteContainer operation returned by the service.
* @throws ContainerInUseException
* The container that you specified in the request already exists or is being updated.
* @throws ContainerNotFoundException
* The container that you specified in the request does not exist.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @sample AWSMediaStore.DeleteContainer
* @see AWS API
* Documentation
*/
DeleteContainerResult deleteContainer(DeleteContainerRequest deleteContainerRequest);
/**
*
* Deletes the access policy that is associated with the specified container.
*
*
* @param deleteContainerPolicyRequest
* @return Result of the DeleteContainerPolicy operation returned by the service.
* @throws ContainerInUseException
* The container that you specified in the request already exists or is being updated.
* @throws ContainerNotFoundException
* The container that you specified in the request does not exist.
* @throws PolicyNotFoundException
* The policy that you specified in the request does not exist.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @sample AWSMediaStore.DeleteContainerPolicy
* @see AWS API Documentation
*/
DeleteContainerPolicyResult deleteContainerPolicy(DeleteContainerPolicyRequest deleteContainerPolicyRequest);
/**
*
* Deletes the cross-origin resource sharing (CORS) configuration information that is set for the container.
*
*
* To use this operation, you must have permission to perform the MediaStore:DeleteCorsPolicy
action.
* The container owner has this permission by default and can grant this permission to others.
*
*
* @param deleteCorsPolicyRequest
* @return Result of the DeleteCorsPolicy operation returned by the service.
* @throws ContainerInUseException
* The container that you specified in the request already exists or is being updated.
* @throws ContainerNotFoundException
* The container that you specified in the request does not exist.
* @throws CorsPolicyNotFoundException
* The CORS policy that you specified in the request does not exist.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @sample AWSMediaStore.DeleteCorsPolicy
* @see AWS
* API Documentation
*/
DeleteCorsPolicyResult deleteCorsPolicy(DeleteCorsPolicyRequest deleteCorsPolicyRequest);
/**
*
* Removes an object lifecycle policy from a container. It takes up to 20 minutes for the change to take effect.
*
*
* @param deleteLifecyclePolicyRequest
* @return Result of the DeleteLifecyclePolicy operation returned by the service.
* @throws ContainerInUseException
* The container that you specified in the request already exists or is being updated.
* @throws ContainerNotFoundException
* The container that you specified in the request does not exist.
* @throws PolicyNotFoundException
* The policy that you specified in the request does not exist.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @sample AWSMediaStore.DeleteLifecyclePolicy
* @see AWS API Documentation
*/
DeleteLifecyclePolicyResult deleteLifecyclePolicy(DeleteLifecyclePolicyRequest deleteLifecyclePolicyRequest);
/**
*
* Deletes the metric policy that is associated with the specified container. If there is no metric policy
* associated with the container, MediaStore doesn't send metrics to CloudWatch.
*
*
* @param deleteMetricPolicyRequest
* @return Result of the DeleteMetricPolicy operation returned by the service.
* @throws ContainerInUseException
* The container that you specified in the request already exists or is being updated.
* @throws ContainerNotFoundException
* The container that you specified in the request does not exist.
* @throws PolicyNotFoundException
* The policy that you specified in the request does not exist.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @sample AWSMediaStore.DeleteMetricPolicy
* @see AWS
* API Documentation
*/
DeleteMetricPolicyResult deleteMetricPolicy(DeleteMetricPolicyRequest deleteMetricPolicyRequest);
/**
*
* Retrieves the properties of the requested container. This request is commonly used to retrieve the endpoint of a
* container. An endpoint is a value assigned by the service when a new container is created. A container's endpoint
* does not change after it has been assigned. The DescribeContainer
request returns a single
* Container
object based on ContainerName
. To return all Container
objects
* that are associated with a specified AWS account, use ListContainers.
*
*
* @param describeContainerRequest
* @return Result of the DescribeContainer operation returned by the service.
* @throws ContainerNotFoundException
* The container that you specified in the request does not exist.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @sample AWSMediaStore.DescribeContainer
* @see AWS
* API Documentation
*/
DescribeContainerResult describeContainer(DescribeContainerRequest describeContainerRequest);
/**
*
* Retrieves the access policy for the specified container. For information about the data that is included in an
* access policy, see the AWS Identity and Access Management
* User Guide.
*
*
* @param getContainerPolicyRequest
* @return Result of the GetContainerPolicy operation returned by the service.
* @throws ContainerInUseException
* The container that you specified in the request already exists or is being updated.
* @throws ContainerNotFoundException
* The container that you specified in the request does not exist.
* @throws PolicyNotFoundException
* The policy that you specified in the request does not exist.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @sample AWSMediaStore.GetContainerPolicy
* @see AWS
* API Documentation
*/
GetContainerPolicyResult getContainerPolicy(GetContainerPolicyRequest getContainerPolicyRequest);
/**
*
* Returns the cross-origin resource sharing (CORS) configuration information that is set for the container.
*
*
* To use this operation, you must have permission to perform the MediaStore:GetCorsPolicy
action. By
* default, the container owner has this permission and can grant it to others.
*
*
* @param getCorsPolicyRequest
* @return Result of the GetCorsPolicy operation returned by the service.
* @throws ContainerInUseException
* The container that you specified in the request already exists or is being updated.
* @throws ContainerNotFoundException
* The container that you specified in the request does not exist.
* @throws CorsPolicyNotFoundException
* The CORS policy that you specified in the request does not exist.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @sample AWSMediaStore.GetCorsPolicy
* @see AWS API
* Documentation
*/
GetCorsPolicyResult getCorsPolicy(GetCorsPolicyRequest getCorsPolicyRequest);
/**
*
* Retrieves the object lifecycle policy that is assigned to a container.
*
*
* @param getLifecyclePolicyRequest
* @return Result of the GetLifecyclePolicy operation returned by the service.
* @throws ContainerInUseException
* The container that you specified in the request already exists or is being updated.
* @throws ContainerNotFoundException
* The container that you specified in the request does not exist.
* @throws PolicyNotFoundException
* The policy that you specified in the request does not exist.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @sample AWSMediaStore.GetLifecyclePolicy
* @see AWS
* API Documentation
*/
GetLifecyclePolicyResult getLifecyclePolicy(GetLifecyclePolicyRequest getLifecyclePolicyRequest);
/**
*
* Returns the metric policy for the specified container.
*
*
* @param getMetricPolicyRequest
* @return Result of the GetMetricPolicy operation returned by the service.
* @throws ContainerNotFoundException
* The container that you specified in the request does not exist.
* @throws PolicyNotFoundException
* The policy that you specified in the request does not exist.
* @throws ContainerInUseException
* The container that you specified in the request already exists or is being updated.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @sample AWSMediaStore.GetMetricPolicy
* @see AWS API
* Documentation
*/
GetMetricPolicyResult getMetricPolicy(GetMetricPolicyRequest getMetricPolicyRequest);
/**
*
* Lists the properties of all containers in AWS Elemental MediaStore.
*
*
* You can query to receive all the containers in one response. Or you can include the MaxResults
* parameter to receive a limited number of containers in each response. In this case, the response includes a
* token. To get the next set of containers, send the command again, this time with the NextToken
* parameter (with the returned token as its value). The next set of responses appears, with a token if there are
* still more containers to receive.
*
*
* See also DescribeContainer, which gets the properties of one container.
*
*
* @param listContainersRequest
* @return Result of the ListContainers operation returned by the service.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @sample AWSMediaStore.ListContainers
* @see AWS API
* Documentation
*/
ListContainersResult listContainers(ListContainersRequest listContainersRequest);
/**
*
* Returns a list of the tags assigned to the specified container.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws ContainerInUseException
* The container that you specified in the request already exists or is being updated.
* @throws ContainerNotFoundException
* The container that you specified in the request does not exist.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @sample AWSMediaStore.ListTagsForResource
* @see AWS
* API Documentation
*/
ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Creates an access policy for the specified container to restrict the users and clients that can access it. For
* information about the data that is included in an access policy, see the AWS Identity and Access Management User Guide.
*
*
* For this release of the REST API, you can create only one policy for a container. If you enter
* PutContainerPolicy
twice, the second command modifies the existing policy.
*
*
* @param putContainerPolicyRequest
* @return Result of the PutContainerPolicy operation returned by the service.
* @throws ContainerNotFoundException
* The container that you specified in the request does not exist.
* @throws ContainerInUseException
* The container that you specified in the request already exists or is being updated.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @sample AWSMediaStore.PutContainerPolicy
* @see AWS
* API Documentation
*/
PutContainerPolicyResult putContainerPolicy(PutContainerPolicyRequest putContainerPolicyRequest);
/**
*
* Sets the cross-origin resource sharing (CORS) configuration on a container so that the container can service
* cross-origin requests. For example, you might want to enable a request whose origin is http://www.example.com to
* access your AWS Elemental MediaStore container at my.example.container.com by using the browser's XMLHttpRequest
* capability.
*
*
* To enable CORS on a container, you attach a CORS policy to the container. In the CORS policy, you configure rules
* that identify origins and the HTTP methods that can be executed on your container. The policy can contain up to
* 398,000 characters. You can add up to 100 rules to a CORS policy. If more than one rule applies, the service uses
* the first applicable rule listed.
*
*
* To learn more about CORS, see Cross-Origin Resource Sharing (CORS) in
* AWS Elemental MediaStore.
*
*
* @param putCorsPolicyRequest
* @return Result of the PutCorsPolicy operation returned by the service.
* @throws ContainerNotFoundException
* The container that you specified in the request does not exist.
* @throws ContainerInUseException
* The container that you specified in the request already exists or is being updated.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @sample AWSMediaStore.PutCorsPolicy
* @see AWS API
* Documentation
*/
PutCorsPolicyResult putCorsPolicy(PutCorsPolicyRequest putCorsPolicyRequest);
/**
*
* Writes an object lifecycle policy to a container. If the container already has an object lifecycle policy, the
* service replaces the existing policy with the new policy. It takes up to 20 minutes for the change to take
* effect.
*
*
* For information about how to construct an object lifecycle policy, see Components of
* an Object Lifecycle Policy.
*
*
* @param putLifecyclePolicyRequest
* @return Result of the PutLifecyclePolicy operation returned by the service.
* @throws ContainerInUseException
* The container that you specified in the request already exists or is being updated.
* @throws ContainerNotFoundException
* The container that you specified in the request does not exist.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @sample AWSMediaStore.PutLifecyclePolicy
* @see AWS
* API Documentation
*/
PutLifecyclePolicyResult putLifecyclePolicy(PutLifecyclePolicyRequest putLifecyclePolicyRequest);
/**
*
* The metric policy that you want to add to the container. A metric policy allows AWS Elemental MediaStore to send
* metrics to Amazon CloudWatch. It takes up to 20 minutes for the new policy to take effect.
*
*
* @param putMetricPolicyRequest
* @return Result of the PutMetricPolicy operation returned by the service.
* @throws ContainerInUseException
* The container that you specified in the request already exists or is being updated.
* @throws ContainerNotFoundException
* The container that you specified in the request does not exist.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @sample AWSMediaStore.PutMetricPolicy
* @see AWS API
* Documentation
*/
PutMetricPolicyResult putMetricPolicy(PutMetricPolicyRequest putMetricPolicyRequest);
/**
*
* Starts access logging on the specified container. When you enable access logging on a container, MediaStore
* delivers access logs for objects stored in that container to Amazon CloudWatch Logs.
*
*
* @param startAccessLoggingRequest
* @return Result of the StartAccessLogging operation returned by the service.
* @throws ContainerInUseException
* The container that you specified in the request already exists or is being updated.
* @throws ContainerNotFoundException
* The container that you specified in the request does not exist.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @sample AWSMediaStore.StartAccessLogging
* @see AWS
* API Documentation
*/
StartAccessLoggingResult startAccessLogging(StartAccessLoggingRequest startAccessLoggingRequest);
/**
*
* Stops access logging on the specified container. When you stop access logging on a container, MediaStore stops
* sending access logs to Amazon CloudWatch Logs. These access logs are not saved and are not retrievable.
*
*
* @param stopAccessLoggingRequest
* @return Result of the StopAccessLogging operation returned by the service.
* @throws ContainerInUseException
* The container that you specified in the request already exists or is being updated.
* @throws ContainerNotFoundException
* The container that you specified in the request does not exist.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @sample AWSMediaStore.StopAccessLogging
* @see AWS
* API Documentation
*/
StopAccessLoggingResult stopAccessLogging(StopAccessLoggingRequest stopAccessLoggingRequest);
/**
*
* Adds tags to the specified AWS Elemental MediaStore container. Tags are key:value pairs that you can associate
* with AWS resources. For example, the tag key might be "customer" and the tag value might be "companyA." You can
* specify one or more tags to add to each container. You can add up to 50 tags to each container. For more
* information about tagging, including naming and usage conventions, see Tagging Resources in MediaStore.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws ContainerInUseException
* The container that you specified in the request already exists or is being updated.
* @throws ContainerNotFoundException
* The container that you specified in the request does not exist.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @sample AWSMediaStore.TagResource
* @see AWS API
* Documentation
*/
TagResourceResult tagResource(TagResourceRequest tagResourceRequest);
/**
*
* Removes tags from the specified container. You can specify one or more tags to remove.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws ContainerInUseException
* The container that you specified in the request already exists or is being updated.
* @throws ContainerNotFoundException
* The container that you specified in the request does not exist.
* @throws InternalServerErrorException
* The service is temporarily unavailable.
* @sample AWSMediaStore.UntagResource
* @see AWS API
* Documentation
*/
UntagResourceResult untagResource(UntagResourceRequest untagResourceRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}