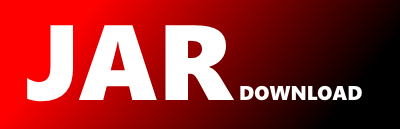
com.amazonaws.services.mediastoredata.model.GetObjectRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-mediastoredata Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.mediastoredata.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class GetObjectRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The path (including the file name) where the object is stored in the container. Format: <folder
* name>/<folder name>/<file name>
*
*
* For example, to upload the file mlaw.avi
to the folder path premium\canada
in the
* container movies
, enter the path premium/canada/mlaw.avi
.
*
*
* Do not include the container name in this path.
*
*
* If the path includes any folders that don't exist yet, the service creates them. For example, suppose you have an
* existing premium/usa
subfolder. If you specify premium/canada
, the service creates a
* canada
subfolder in the premium
folder. You then have two subfolders, usa
* and canada
, in the premium
folder.
*
*
* There is no correlation between the path to the source and the path (folders) in the container in AWS Elemental
* MediaStore.
*
*
* For more information about folders and how they exist in a container, see the AWS Elemental MediaStore User Guide.
*
*
* The file name is the name that is assigned to the file that you upload. The file can have the same name inside
* and outside of AWS Elemental MediaStore, or it can have the same name. The file name can include or omit an
* extension.
*
*/
private String path;
/**
*
* The range bytes of an object to retrieve. For more information about the Range
header, see http://www.w3.org/Protocols/rfc2616/rfc2616-sec14.html#sec14.35. AWS Elemental MediaStore ignores this
* header for partially uploaded objects that have streaming upload availability.
*
*/
private String range;
/**
*
* The path (including the file name) where the object is stored in the container. Format: <folder
* name>/<folder name>/<file name>
*
*
* For example, to upload the file mlaw.avi
to the folder path premium\canada
in the
* container movies
, enter the path premium/canada/mlaw.avi
.
*
*
* Do not include the container name in this path.
*
*
* If the path includes any folders that don't exist yet, the service creates them. For example, suppose you have an
* existing premium/usa
subfolder. If you specify premium/canada
, the service creates a
* canada
subfolder in the premium
folder. You then have two subfolders, usa
* and canada
, in the premium
folder.
*
*
* There is no correlation between the path to the source and the path (folders) in the container in AWS Elemental
* MediaStore.
*
*
* For more information about folders and how they exist in a container, see the AWS Elemental MediaStore User Guide.
*
*
* The file name is the name that is assigned to the file that you upload. The file can have the same name inside
* and outside of AWS Elemental MediaStore, or it can have the same name. The file name can include or omit an
* extension.
*
*
* @param path
* The path (including the file name) where the object is stored in the container. Format: <folder
* name>/<folder name>/<file name>
*
* For example, to upload the file mlaw.avi
to the folder path premium\canada
in
* the container movies
, enter the path premium/canada/mlaw.avi
.
*
*
* Do not include the container name in this path.
*
*
* If the path includes any folders that don't exist yet, the service creates them. For example, suppose you
* have an existing premium/usa
subfolder. If you specify premium/canada
, the
* service creates a canada
subfolder in the premium
folder. You then have two
* subfolders, usa
and canada
, in the premium
folder.
*
*
* There is no correlation between the path to the source and the path (folders) in the container in AWS
* Elemental MediaStore.
*
*
* For more information about folders and how they exist in a container, see the AWS Elemental MediaStore User Guide.
*
*
* The file name is the name that is assigned to the file that you upload. The file can have the same name
* inside and outside of AWS Elemental MediaStore, or it can have the same name. The file name can include or
* omit an extension.
*/
public void setPath(String path) {
this.path = path;
}
/**
*
* The path (including the file name) where the object is stored in the container. Format: <folder
* name>/<folder name>/<file name>
*
*
* For example, to upload the file mlaw.avi
to the folder path premium\canada
in the
* container movies
, enter the path premium/canada/mlaw.avi
.
*
*
* Do not include the container name in this path.
*
*
* If the path includes any folders that don't exist yet, the service creates them. For example, suppose you have an
* existing premium/usa
subfolder. If you specify premium/canada
, the service creates a
* canada
subfolder in the premium
folder. You then have two subfolders, usa
* and canada
, in the premium
folder.
*
*
* There is no correlation between the path to the source and the path (folders) in the container in AWS Elemental
* MediaStore.
*
*
* For more information about folders and how they exist in a container, see the AWS Elemental MediaStore User Guide.
*
*
* The file name is the name that is assigned to the file that you upload. The file can have the same name inside
* and outside of AWS Elemental MediaStore, or it can have the same name. The file name can include or omit an
* extension.
*
*
* @return The path (including the file name) where the object is stored in the container. Format: <folder
* name>/<folder name>/<file name>
*
* For example, to upload the file mlaw.avi
to the folder path premium\canada
in
* the container movies
, enter the path premium/canada/mlaw.avi
.
*
*
* Do not include the container name in this path.
*
*
* If the path includes any folders that don't exist yet, the service creates them. For example, suppose you
* have an existing premium/usa
subfolder. If you specify premium/canada
, the
* service creates a canada
subfolder in the premium
folder. You then have two
* subfolders, usa
and canada
, in the premium
folder.
*
*
* There is no correlation between the path to the source and the path (folders) in the container in AWS
* Elemental MediaStore.
*
*
* For more information about folders and how they exist in a container, see the AWS Elemental MediaStore User Guide.
*
*
* The file name is the name that is assigned to the file that you upload. The file can have the same name
* inside and outside of AWS Elemental MediaStore, or it can have the same name. The file name can include
* or omit an extension.
*/
public String getPath() {
return this.path;
}
/**
*
* The path (including the file name) where the object is stored in the container. Format: <folder
* name>/<folder name>/<file name>
*
*
* For example, to upload the file mlaw.avi
to the folder path premium\canada
in the
* container movies
, enter the path premium/canada/mlaw.avi
.
*
*
* Do not include the container name in this path.
*
*
* If the path includes any folders that don't exist yet, the service creates them. For example, suppose you have an
* existing premium/usa
subfolder. If you specify premium/canada
, the service creates a
* canada
subfolder in the premium
folder. You then have two subfolders, usa
* and canada
, in the premium
folder.
*
*
* There is no correlation between the path to the source and the path (folders) in the container in AWS Elemental
* MediaStore.
*
*
* For more information about folders and how they exist in a container, see the AWS Elemental MediaStore User Guide.
*
*
* The file name is the name that is assigned to the file that you upload. The file can have the same name inside
* and outside of AWS Elemental MediaStore, or it can have the same name. The file name can include or omit an
* extension.
*
*
* @param path
* The path (including the file name) where the object is stored in the container. Format: <folder
* name>/<folder name>/<file name>
*
* For example, to upload the file mlaw.avi
to the folder path premium\canada
in
* the container movies
, enter the path premium/canada/mlaw.avi
.
*
*
* Do not include the container name in this path.
*
*
* If the path includes any folders that don't exist yet, the service creates them. For example, suppose you
* have an existing premium/usa
subfolder. If you specify premium/canada
, the
* service creates a canada
subfolder in the premium
folder. You then have two
* subfolders, usa
and canada
, in the premium
folder.
*
*
* There is no correlation between the path to the source and the path (folders) in the container in AWS
* Elemental MediaStore.
*
*
* For more information about folders and how they exist in a container, see the AWS Elemental MediaStore User Guide.
*
*
* The file name is the name that is assigned to the file that you upload. The file can have the same name
* inside and outside of AWS Elemental MediaStore, or it can have the same name. The file name can include or
* omit an extension.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetObjectRequest withPath(String path) {
setPath(path);
return this;
}
/**
*
* The range bytes of an object to retrieve. For more information about the Range
header, see http://www.w3.org/Protocols/rfc2616/rfc2616-sec14.html#sec14.35. AWS Elemental MediaStore ignores this
* header for partially uploaded objects that have streaming upload availability.
*
*
* @param range
* The range bytes of an object to retrieve. For more information about the Range
header, see http://www.w3.org/Protocols/rfc2616/
* rfc2616-sec14.html#sec14.35. AWS Elemental MediaStore ignores this header for partially uploaded
* objects that have streaming upload availability.
*/
public void setRange(String range) {
this.range = range;
}
/**
*
* The range bytes of an object to retrieve. For more information about the Range
header, see http://www.w3.org/Protocols/rfc2616/rfc2616-sec14.html#sec14.35. AWS Elemental MediaStore ignores this
* header for partially uploaded objects that have streaming upload availability.
*
*
* @return The range bytes of an object to retrieve. For more information about the Range
header, see
* http://www.w3.org/Protocols/
* rfc2616/rfc2616-sec14.html#sec14.35. AWS Elemental MediaStore ignores this header for partially
* uploaded objects that have streaming upload availability.
*/
public String getRange() {
return this.range;
}
/**
*
* The range bytes of an object to retrieve. For more information about the Range
header, see http://www.w3.org/Protocols/rfc2616/rfc2616-sec14.html#sec14.35. AWS Elemental MediaStore ignores this
* header for partially uploaded objects that have streaming upload availability.
*
*
* @param range
* The range bytes of an object to retrieve. For more information about the Range
header, see http://www.w3.org/Protocols/rfc2616/
* rfc2616-sec14.html#sec14.35. AWS Elemental MediaStore ignores this header for partially uploaded
* objects that have streaming upload availability.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetObjectRequest withRange(String range) {
setRange(range);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getPath() != null)
sb.append("Path: ").append(getPath()).append(",");
if (getRange() != null)
sb.append("Range: ").append(getRange());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof GetObjectRequest == false)
return false;
GetObjectRequest other = (GetObjectRequest) obj;
if (other.getPath() == null ^ this.getPath() == null)
return false;
if (other.getPath() != null && other.getPath().equals(this.getPath()) == false)
return false;
if (other.getRange() == null ^ this.getRange() == null)
return false;
if (other.getRange() != null && other.getRange().equals(this.getRange()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getPath() == null) ? 0 : getPath().hashCode());
hashCode = prime * hashCode + ((getRange() == null) ? 0 : getRange().hashCode());
return hashCode;
}
@Override
public GetObjectRequest clone() {
return (GetObjectRequest) super.clone();
}
}